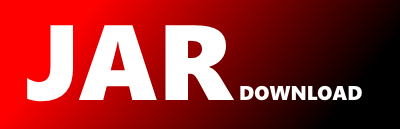
software.amazon.awssdk.services.databasemigration.model.DataProviderSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigration Show documentation
Show all versions of databasemigration Show documentation
The AWS Java SDK for AWS Database Migration Service module holds the client classes that are used for
communicating with AWS Database Migration Service.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructMap;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information that defines a data provider.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DataProviderSettings implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField REDSHIFT_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RedshiftSettings")
.getter(getter(DataProviderSettings::redshiftSettings)).setter(setter(Builder::redshiftSettings))
.constructor(RedshiftDataProviderSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RedshiftSettings").build()).build();
private static final SdkField POSTGRE_SQL_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PostgreSqlSettings")
.getter(getter(DataProviderSettings::postgreSqlSettings)).setter(setter(Builder::postgreSqlSettings))
.constructor(PostgreSqlDataProviderSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PostgreSqlSettings").build())
.build();
private static final SdkField MY_SQL_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("MySqlSettings")
.getter(getter(DataProviderSettings::mySqlSettings)).setter(setter(Builder::mySqlSettings))
.constructor(MySqlDataProviderSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MySqlSettings").build()).build();
private static final SdkField ORACLE_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OracleSettings")
.getter(getter(DataProviderSettings::oracleSettings)).setter(setter(Builder::oracleSettings))
.constructor(OracleDataProviderSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OracleSettings").build()).build();
private static final SdkField MICROSOFT_SQL_SERVER_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("MicrosoftSqlServerSettings")
.getter(getter(DataProviderSettings::microsoftSqlServerSettings))
.setter(setter(Builder::microsoftSqlServerSettings))
.constructor(MicrosoftSqlServerDataProviderSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MicrosoftSqlServerSettings").build())
.build();
private static final SdkField DOC_DB_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DocDbSettings")
.getter(getter(DataProviderSettings::docDbSettings)).setter(setter(Builder::docDbSettings))
.constructor(DocDbDataProviderSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DocDbSettings").build()).build();
private static final SdkField MARIA_DB_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("MariaDbSettings")
.getter(getter(DataProviderSettings::mariaDbSettings)).setter(setter(Builder::mariaDbSettings))
.constructor(MariaDbDataProviderSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MariaDbSettings").build()).build();
private static final SdkField MONGO_DB_SETTINGS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("MongoDbSettings")
.getter(getter(DataProviderSettings::mongoDbSettings)).setter(setter(Builder::mongoDbSettings))
.constructor(MongoDbDataProviderSettings::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MongoDbSettings").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REDSHIFT_SETTINGS_FIELD,
POSTGRE_SQL_SETTINGS_FIELD, MY_SQL_SETTINGS_FIELD, ORACLE_SETTINGS_FIELD, MICROSOFT_SQL_SERVER_SETTINGS_FIELD,
DOC_DB_SETTINGS_FIELD, MARIA_DB_SETTINGS_FIELD, MONGO_DB_SETTINGS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final RedshiftDataProviderSettings redshiftSettings;
private final PostgreSqlDataProviderSettings postgreSqlSettings;
private final MySqlDataProviderSettings mySqlSettings;
private final OracleDataProviderSettings oracleSettings;
private final MicrosoftSqlServerDataProviderSettings microsoftSqlServerSettings;
private final DocDbDataProviderSettings docDbSettings;
private final MariaDbDataProviderSettings mariaDbSettings;
private final MongoDbDataProviderSettings mongoDbSettings;
private final Type type;
private DataProviderSettings(BuilderImpl builder) {
this.redshiftSettings = builder.redshiftSettings;
this.postgreSqlSettings = builder.postgreSqlSettings;
this.mySqlSettings = builder.mySqlSettings;
this.oracleSettings = builder.oracleSettings;
this.microsoftSqlServerSettings = builder.microsoftSqlServerSettings;
this.docDbSettings = builder.docDbSettings;
this.mariaDbSettings = builder.mariaDbSettings;
this.mongoDbSettings = builder.mongoDbSettings;
this.type = builder.type;
}
/**
* Returns the value of the RedshiftSettings property for this object.
*
* @return The value of the RedshiftSettings property for this object.
*/
public final RedshiftDataProviderSettings redshiftSettings() {
return redshiftSettings;
}
/**
* Returns the value of the PostgreSqlSettings property for this object.
*
* @return The value of the PostgreSqlSettings property for this object.
*/
public final PostgreSqlDataProviderSettings postgreSqlSettings() {
return postgreSqlSettings;
}
/**
* Returns the value of the MySqlSettings property for this object.
*
* @return The value of the MySqlSettings property for this object.
*/
public final MySqlDataProviderSettings mySqlSettings() {
return mySqlSettings;
}
/**
* Returns the value of the OracleSettings property for this object.
*
* @return The value of the OracleSettings property for this object.
*/
public final OracleDataProviderSettings oracleSettings() {
return oracleSettings;
}
/**
* Returns the value of the MicrosoftSqlServerSettings property for this object.
*
* @return The value of the MicrosoftSqlServerSettings property for this object.
*/
public final MicrosoftSqlServerDataProviderSettings microsoftSqlServerSettings() {
return microsoftSqlServerSettings;
}
/**
* Returns the value of the DocDbSettings property for this object.
*
* @return The value of the DocDbSettings property for this object.
*/
public final DocDbDataProviderSettings docDbSettings() {
return docDbSettings;
}
/**
*
* Provides information that defines a MariaDB data provider.
*
*
* @return Provides information that defines a MariaDB data provider.
*/
public final MariaDbDataProviderSettings mariaDbSettings() {
return mariaDbSettings;
}
/**
*
* Provides information that defines a MongoDB data provider.
*
*
* @return Provides information that defines a MongoDB data provider.
*/
public final MongoDbDataProviderSettings mongoDbSettings() {
return mongoDbSettings;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(redshiftSettings());
hashCode = 31 * hashCode + Objects.hashCode(postgreSqlSettings());
hashCode = 31 * hashCode + Objects.hashCode(mySqlSettings());
hashCode = 31 * hashCode + Objects.hashCode(oracleSettings());
hashCode = 31 * hashCode + Objects.hashCode(microsoftSqlServerSettings());
hashCode = 31 * hashCode + Objects.hashCode(docDbSettings());
hashCode = 31 * hashCode + Objects.hashCode(mariaDbSettings());
hashCode = 31 * hashCode + Objects.hashCode(mongoDbSettings());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DataProviderSettings)) {
return false;
}
DataProviderSettings other = (DataProviderSettings) obj;
return Objects.equals(redshiftSettings(), other.redshiftSettings())
&& Objects.equals(postgreSqlSettings(), other.postgreSqlSettings())
&& Objects.equals(mySqlSettings(), other.mySqlSettings())
&& Objects.equals(oracleSettings(), other.oracleSettings())
&& Objects.equals(microsoftSqlServerSettings(), other.microsoftSqlServerSettings())
&& Objects.equals(docDbSettings(), other.docDbSettings())
&& Objects.equals(mariaDbSettings(), other.mariaDbSettings())
&& Objects.equals(mongoDbSettings(), other.mongoDbSettings());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DataProviderSettings").add("RedshiftSettings", redshiftSettings())
.add("PostgreSqlSettings", postgreSqlSettings()).add("MySqlSettings", mySqlSettings())
.add("OracleSettings", oracleSettings()).add("MicrosoftSqlServerSettings", microsoftSqlServerSettings())
.add("DocDbSettings", docDbSettings()).add("MariaDbSettings", mariaDbSettings())
.add("MongoDbSettings", mongoDbSettings()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RedshiftSettings":
return Optional.ofNullable(clazz.cast(redshiftSettings()));
case "PostgreSqlSettings":
return Optional.ofNullable(clazz.cast(postgreSqlSettings()));
case "MySqlSettings":
return Optional.ofNullable(clazz.cast(mySqlSettings()));
case "OracleSettings":
return Optional.ofNullable(clazz.cast(oracleSettings()));
case "MicrosoftSqlServerSettings":
return Optional.ofNullable(clazz.cast(microsoftSqlServerSettings()));
case "DocDbSettings":
return Optional.ofNullable(clazz.cast(docDbSettings()));
case "MariaDbSettings":
return Optional.ofNullable(clazz.cast(mariaDbSettings()));
case "MongoDbSettings":
return Optional.ofNullable(clazz.cast(mongoDbSettings()));
default:
return Optional.empty();
}
}
/**
* Create an instance of this class with {@link #redshiftSettings()} initialized to the given value.
*
* Sets the value of the RedshiftSettings property for this object.
*
* @param redshiftSettings
* The new value for the RedshiftSettings property for this object.
*/
public static DataProviderSettings fromRedshiftSettings(RedshiftDataProviderSettings redshiftSettings) {
return builder().redshiftSettings(redshiftSettings).build();
}
/**
* Create an instance of this class with {@link #redshiftSettings()} initialized to the given value.
*
* Sets the value of the RedshiftSettings property for this object.
*
* @param redshiftSettings
* The new value for the RedshiftSettings property for this object.
*/
public static DataProviderSettings fromRedshiftSettings(Consumer redshiftSettings) {
RedshiftDataProviderSettings.Builder builder = RedshiftDataProviderSettings.builder();
redshiftSettings.accept(builder);
return fromRedshiftSettings(builder.build());
}
/**
* Create an instance of this class with {@link #postgreSqlSettings()} initialized to the given value.
*
* Sets the value of the PostgreSqlSettings property for this object.
*
* @param postgreSqlSettings
* The new value for the PostgreSqlSettings property for this object.
*/
public static DataProviderSettings fromPostgreSqlSettings(PostgreSqlDataProviderSettings postgreSqlSettings) {
return builder().postgreSqlSettings(postgreSqlSettings).build();
}
/**
* Create an instance of this class with {@link #postgreSqlSettings()} initialized to the given value.
*
* Sets the value of the PostgreSqlSettings property for this object.
*
* @param postgreSqlSettings
* The new value for the PostgreSqlSettings property for this object.
*/
public static DataProviderSettings fromPostgreSqlSettings(Consumer postgreSqlSettings) {
PostgreSqlDataProviderSettings.Builder builder = PostgreSqlDataProviderSettings.builder();
postgreSqlSettings.accept(builder);
return fromPostgreSqlSettings(builder.build());
}
/**
* Create an instance of this class with {@link #mySqlSettings()} initialized to the given value.
*
* Sets the value of the MySqlSettings property for this object.
*
* @param mySqlSettings
* The new value for the MySqlSettings property for this object.
*/
public static DataProviderSettings fromMySqlSettings(MySqlDataProviderSettings mySqlSettings) {
return builder().mySqlSettings(mySqlSettings).build();
}
/**
* Create an instance of this class with {@link #mySqlSettings()} initialized to the given value.
*
* Sets the value of the MySqlSettings property for this object.
*
* @param mySqlSettings
* The new value for the MySqlSettings property for this object.
*/
public static DataProviderSettings fromMySqlSettings(Consumer mySqlSettings) {
MySqlDataProviderSettings.Builder builder = MySqlDataProviderSettings.builder();
mySqlSettings.accept(builder);
return fromMySqlSettings(builder.build());
}
/**
* Create an instance of this class with {@link #oracleSettings()} initialized to the given value.
*
* Sets the value of the OracleSettings property for this object.
*
* @param oracleSettings
* The new value for the OracleSettings property for this object.
*/
public static DataProviderSettings fromOracleSettings(OracleDataProviderSettings oracleSettings) {
return builder().oracleSettings(oracleSettings).build();
}
/**
* Create an instance of this class with {@link #oracleSettings()} initialized to the given value.
*
* Sets the value of the OracleSettings property for this object.
*
* @param oracleSettings
* The new value for the OracleSettings property for this object.
*/
public static DataProviderSettings fromOracleSettings(Consumer oracleSettings) {
OracleDataProviderSettings.Builder builder = OracleDataProviderSettings.builder();
oracleSettings.accept(builder);
return fromOracleSettings(builder.build());
}
/**
* Create an instance of this class with {@link #microsoftSqlServerSettings()} initialized to the given value.
*
* Sets the value of the MicrosoftSqlServerSettings property for this object.
*
* @param microsoftSqlServerSettings
* The new value for the MicrosoftSqlServerSettings property for this object.
*/
public static DataProviderSettings fromMicrosoftSqlServerSettings(
MicrosoftSqlServerDataProviderSettings microsoftSqlServerSettings) {
return builder().microsoftSqlServerSettings(microsoftSqlServerSettings).build();
}
/**
* Create an instance of this class with {@link #microsoftSqlServerSettings()} initialized to the given value.
*
* Sets the value of the MicrosoftSqlServerSettings property for this object.
*
* @param microsoftSqlServerSettings
* The new value for the MicrosoftSqlServerSettings property for this object.
*/
public static DataProviderSettings fromMicrosoftSqlServerSettings(
Consumer microsoftSqlServerSettings) {
MicrosoftSqlServerDataProviderSettings.Builder builder = MicrosoftSqlServerDataProviderSettings.builder();
microsoftSqlServerSettings.accept(builder);
return fromMicrosoftSqlServerSettings(builder.build());
}
/**
* Create an instance of this class with {@link #docDbSettings()} initialized to the given value.
*
* Sets the value of the DocDbSettings property for this object.
*
* @param docDbSettings
* The new value for the DocDbSettings property for this object.
*/
public static DataProviderSettings fromDocDbSettings(DocDbDataProviderSettings docDbSettings) {
return builder().docDbSettings(docDbSettings).build();
}
/**
* Create an instance of this class with {@link #docDbSettings()} initialized to the given value.
*
* Sets the value of the DocDbSettings property for this object.
*
* @param docDbSettings
* The new value for the DocDbSettings property for this object.
*/
public static DataProviderSettings fromDocDbSettings(Consumer docDbSettings) {
DocDbDataProviderSettings.Builder builder = DocDbDataProviderSettings.builder();
docDbSettings.accept(builder);
return fromDocDbSettings(builder.build());
}
/**
* Create an instance of this class with {@link #mariaDbSettings()} initialized to the given value.
*
*
* Provides information that defines a MariaDB data provider.
*
*
* @param mariaDbSettings
* Provides information that defines a MariaDB data provider.
*/
public static DataProviderSettings fromMariaDbSettings(MariaDbDataProviderSettings mariaDbSettings) {
return builder().mariaDbSettings(mariaDbSettings).build();
}
/**
* Create an instance of this class with {@link #mariaDbSettings()} initialized to the given value.
*
*
* Provides information that defines a MariaDB data provider.
*
*
* @param mariaDbSettings
* Provides information that defines a MariaDB data provider.
*/
public static DataProviderSettings fromMariaDbSettings(Consumer mariaDbSettings) {
MariaDbDataProviderSettings.Builder builder = MariaDbDataProviderSettings.builder();
mariaDbSettings.accept(builder);
return fromMariaDbSettings(builder.build());
}
/**
* Create an instance of this class with {@link #mongoDbSettings()} initialized to the given value.
*
*
* Provides information that defines a MongoDB data provider.
*
*
* @param mongoDbSettings
* Provides information that defines a MongoDB data provider.
*/
public static DataProviderSettings fromMongoDbSettings(MongoDbDataProviderSettings mongoDbSettings) {
return builder().mongoDbSettings(mongoDbSettings).build();
}
/**
* Create an instance of this class with {@link #mongoDbSettings()} initialized to the given value.
*
*
* Provides information that defines a MongoDB data provider.
*
*
* @param mongoDbSettings
* Provides information that defines a MongoDB data provider.
*/
public static DataProviderSettings fromMongoDbSettings(Consumer mongoDbSettings) {
MongoDbDataProviderSettings.Builder builder = MongoDbDataProviderSettings.builder();
mongoDbSettings.accept(builder);
return fromMongoDbSettings(builder.build());
}
/**
* Retrieve an enum value representing which member of this object is populated.
*
* When this class is returned in a service response, this will be {@link Type#UNKNOWN_TO_SDK_VERSION} if the
* service returned a member that is only known to a newer SDK version.
*
* When this class is created directly in your code, this will be {@link Type#UNKNOWN_TO_SDK_VERSION} if zero
* members are set, and {@code null} if more than one member is set.
*/
public Type type() {
return type;
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("RedshiftSettings", REDSHIFT_SETTINGS_FIELD);
map.put("PostgreSqlSettings", POSTGRE_SQL_SETTINGS_FIELD);
map.put("MySqlSettings", MY_SQL_SETTINGS_FIELD);
map.put("OracleSettings", ORACLE_SETTINGS_FIELD);
map.put("MicrosoftSqlServerSettings", MICROSOFT_SQL_SERVER_SETTINGS_FIELD);
map.put("DocDbSettings", DOC_DB_SETTINGS_FIELD);
map.put("MariaDbSettings", MARIA_DB_SETTINGS_FIELD);
map.put("MongoDbSettings", MONGO_DB_SETTINGS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy