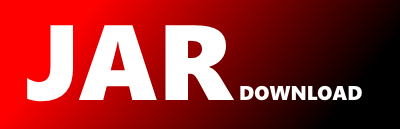
software.amazon.awssdk.services.databasemigration.model.MigrationProject Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information that defines a migration project.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MigrationProject implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField MIGRATION_PROJECT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MigrationProjectName").getter(getter(MigrationProject::migrationProjectName))
.setter(setter(Builder::migrationProjectName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MigrationProjectName").build())
.build();
private static final SdkField MIGRATION_PROJECT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MigrationProjectArn").getter(getter(MigrationProject::migrationProjectArn))
.setter(setter(Builder::migrationProjectArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MigrationProjectArn").build())
.build();
private static final SdkField MIGRATION_PROJECT_CREATION_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("MigrationProjectCreationTime")
.getter(getter(MigrationProject::migrationProjectCreationTime))
.setter(setter(Builder::migrationProjectCreationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MigrationProjectCreationTime")
.build(), TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField> SOURCE_DATA_PROVIDER_DESCRIPTORS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SourceDataProviderDescriptors")
.getter(getter(MigrationProject::sourceDataProviderDescriptors))
.setter(setter(Builder::sourceDataProviderDescriptors))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceDataProviderDescriptors")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DataProviderDescriptor::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TARGET_DATA_PROVIDER_DESCRIPTORS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TargetDataProviderDescriptors")
.getter(getter(MigrationProject::targetDataProviderDescriptors))
.setter(setter(Builder::targetDataProviderDescriptors))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetDataProviderDescriptors")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DataProviderDescriptor::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INSTANCE_PROFILE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceProfileArn").getter(getter(MigrationProject::instanceProfileArn))
.setter(setter(Builder::instanceProfileArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceProfileArn").build())
.build();
private static final SdkField INSTANCE_PROFILE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceProfileName").getter(getter(MigrationProject::instanceProfileName))
.setter(setter(Builder::instanceProfileName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceProfileName").build())
.build();
private static final SdkField TRANSFORMATION_RULES_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TransformationRules").getter(getter(MigrationProject::transformationRules))
.setter(setter(Builder::transformationRules))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransformationRules").build())
.build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(MigrationProject::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField SCHEMA_CONVERSION_APPLICATION_ATTRIBUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("SchemaConversionApplicationAttributes")
.getter(getter(MigrationProject::schemaConversionApplicationAttributes))
.setter(setter(Builder::schemaConversionApplicationAttributes))
.constructor(SCApplicationAttributes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("SchemaConversionApplicationAttributes").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MIGRATION_PROJECT_NAME_FIELD,
MIGRATION_PROJECT_ARN_FIELD, MIGRATION_PROJECT_CREATION_TIME_FIELD, SOURCE_DATA_PROVIDER_DESCRIPTORS_FIELD,
TARGET_DATA_PROVIDER_DESCRIPTORS_FIELD, INSTANCE_PROFILE_ARN_FIELD, INSTANCE_PROFILE_NAME_FIELD,
TRANSFORMATION_RULES_FIELD, DESCRIPTION_FIELD, SCHEMA_CONVERSION_APPLICATION_ATTRIBUTES_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String migrationProjectName;
private final String migrationProjectArn;
private final Instant migrationProjectCreationTime;
private final List sourceDataProviderDescriptors;
private final List targetDataProviderDescriptors;
private final String instanceProfileArn;
private final String instanceProfileName;
private final String transformationRules;
private final String description;
private final SCApplicationAttributes schemaConversionApplicationAttributes;
private MigrationProject(BuilderImpl builder) {
this.migrationProjectName = builder.migrationProjectName;
this.migrationProjectArn = builder.migrationProjectArn;
this.migrationProjectCreationTime = builder.migrationProjectCreationTime;
this.sourceDataProviderDescriptors = builder.sourceDataProviderDescriptors;
this.targetDataProviderDescriptors = builder.targetDataProviderDescriptors;
this.instanceProfileArn = builder.instanceProfileArn;
this.instanceProfileName = builder.instanceProfileName;
this.transformationRules = builder.transformationRules;
this.description = builder.description;
this.schemaConversionApplicationAttributes = builder.schemaConversionApplicationAttributes;
}
/**
*
* The name of the migration project.
*
*
* @return The name of the migration project.
*/
public final String migrationProjectName() {
return migrationProjectName;
}
/**
*
* The ARN string that uniquely identifies the migration project.
*
*
* @return The ARN string that uniquely identifies the migration project.
*/
public final String migrationProjectArn() {
return migrationProjectArn;
}
/**
*
* The time when the migration project was created.
*
*
* @return The time when the migration project was created.
*/
public final Instant migrationProjectCreationTime() {
return migrationProjectCreationTime;
}
/**
* For responses, this returns true if the service returned a value for the SourceDataProviderDescriptors property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasSourceDataProviderDescriptors() {
return sourceDataProviderDescriptors != null && !(sourceDataProviderDescriptors instanceof SdkAutoConstructList);
}
/**
*
* Information about the source data provider, including the name or ARN, and Secrets Manager parameters.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSourceDataProviderDescriptors} method.
*
*
* @return Information about the source data provider, including the name or ARN, and Secrets Manager parameters.
*/
public final List sourceDataProviderDescriptors() {
return sourceDataProviderDescriptors;
}
/**
* For responses, this returns true if the service returned a value for the TargetDataProviderDescriptors property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasTargetDataProviderDescriptors() {
return targetDataProviderDescriptors != null && !(targetDataProviderDescriptors instanceof SdkAutoConstructList);
}
/**
*
* Information about the target data provider, including the name or ARN, and Secrets Manager parameters.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTargetDataProviderDescriptors} method.
*
*
* @return Information about the target data provider, including the name or ARN, and Secrets Manager parameters.
*/
public final List targetDataProviderDescriptors() {
return targetDataProviderDescriptors;
}
/**
*
* The Amazon Resource Name (ARN) of the instance profile for your migration project.
*
*
* @return The Amazon Resource Name (ARN) of the instance profile for your migration project.
*/
public final String instanceProfileArn() {
return instanceProfileArn;
}
/**
*
* The name of the associated instance profile.
*
*
* @return The name of the associated instance profile.
*/
public final String instanceProfileName() {
return instanceProfileName;
}
/**
*
* The settings in JSON format for migration rules. Migration rules make it possible for you to change the object
* names according to the rules that you specify. For example, you can change an object name to lowercase or
* uppercase, add or remove a prefix or suffix, or rename objects.
*
*
* @return The settings in JSON format for migration rules. Migration rules make it possible for you to change the
* object names according to the rules that you specify. For example, you can change an object name to
* lowercase or uppercase, add or remove a prefix or suffix, or rename objects.
*/
public final String transformationRules() {
return transformationRules;
}
/**
*
* A user-friendly description of the migration project.
*
*
* @return A user-friendly description of the migration project.
*/
public final String description() {
return description;
}
/**
*
* The schema conversion application attributes, including the Amazon S3 bucket name and Amazon S3 role ARN.
*
*
* @return The schema conversion application attributes, including the Amazon S3 bucket name and Amazon S3 role ARN.
*/
public final SCApplicationAttributes schemaConversionApplicationAttributes() {
return schemaConversionApplicationAttributes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(migrationProjectName());
hashCode = 31 * hashCode + Objects.hashCode(migrationProjectArn());
hashCode = 31 * hashCode + Objects.hashCode(migrationProjectCreationTime());
hashCode = 31 * hashCode + Objects.hashCode(hasSourceDataProviderDescriptors() ? sourceDataProviderDescriptors() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTargetDataProviderDescriptors() ? targetDataProviderDescriptors() : null);
hashCode = 31 * hashCode + Objects.hashCode(instanceProfileArn());
hashCode = 31 * hashCode + Objects.hashCode(instanceProfileName());
hashCode = 31 * hashCode + Objects.hashCode(transformationRules());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(schemaConversionApplicationAttributes());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MigrationProject)) {
return false;
}
MigrationProject other = (MigrationProject) obj;
return Objects.equals(migrationProjectName(), other.migrationProjectName())
&& Objects.equals(migrationProjectArn(), other.migrationProjectArn())
&& Objects.equals(migrationProjectCreationTime(), other.migrationProjectCreationTime())
&& hasSourceDataProviderDescriptors() == other.hasSourceDataProviderDescriptors()
&& Objects.equals(sourceDataProviderDescriptors(), other.sourceDataProviderDescriptors())
&& hasTargetDataProviderDescriptors() == other.hasTargetDataProviderDescriptors()
&& Objects.equals(targetDataProviderDescriptors(), other.targetDataProviderDescriptors())
&& Objects.equals(instanceProfileArn(), other.instanceProfileArn())
&& Objects.equals(instanceProfileName(), other.instanceProfileName())
&& Objects.equals(transformationRules(), other.transformationRules())
&& Objects.equals(description(), other.description())
&& Objects.equals(schemaConversionApplicationAttributes(), other.schemaConversionApplicationAttributes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("MigrationProject")
.add("MigrationProjectName", migrationProjectName())
.add("MigrationProjectArn", migrationProjectArn())
.add("MigrationProjectCreationTime", migrationProjectCreationTime())
.add("SourceDataProviderDescriptors", hasSourceDataProviderDescriptors() ? sourceDataProviderDescriptors() : null)
.add("TargetDataProviderDescriptors", hasTargetDataProviderDescriptors() ? targetDataProviderDescriptors() : null)
.add("InstanceProfileArn", instanceProfileArn()).add("InstanceProfileName", instanceProfileName())
.add("TransformationRules", transformationRules()).add("Description", description())
.add("SchemaConversionApplicationAttributes", schemaConversionApplicationAttributes()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MigrationProjectName":
return Optional.ofNullable(clazz.cast(migrationProjectName()));
case "MigrationProjectArn":
return Optional.ofNullable(clazz.cast(migrationProjectArn()));
case "MigrationProjectCreationTime":
return Optional.ofNullable(clazz.cast(migrationProjectCreationTime()));
case "SourceDataProviderDescriptors":
return Optional.ofNullable(clazz.cast(sourceDataProviderDescriptors()));
case "TargetDataProviderDescriptors":
return Optional.ofNullable(clazz.cast(targetDataProviderDescriptors()));
case "InstanceProfileArn":
return Optional.ofNullable(clazz.cast(instanceProfileArn()));
case "InstanceProfileName":
return Optional.ofNullable(clazz.cast(instanceProfileName()));
case "TransformationRules":
return Optional.ofNullable(clazz.cast(transformationRules()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "SchemaConversionApplicationAttributes":
return Optional.ofNullable(clazz.cast(schemaConversionApplicationAttributes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("MigrationProjectName", MIGRATION_PROJECT_NAME_FIELD);
map.put("MigrationProjectArn", MIGRATION_PROJECT_ARN_FIELD);
map.put("MigrationProjectCreationTime", MIGRATION_PROJECT_CREATION_TIME_FIELD);
map.put("SourceDataProviderDescriptors", SOURCE_DATA_PROVIDER_DESCRIPTORS_FIELD);
map.put("TargetDataProviderDescriptors", TARGET_DATA_PROVIDER_DESCRIPTORS_FIELD);
map.put("InstanceProfileArn", INSTANCE_PROFILE_ARN_FIELD);
map.put("InstanceProfileName", INSTANCE_PROFILE_NAME_FIELD);
map.put("TransformationRules", TRANSFORMATION_RULES_FIELD);
map.put("Description", DESCRIPTION_FIELD);
map.put("SchemaConversionApplicationAttributes", SCHEMA_CONVERSION_APPLICATION_ATTRIBUTES_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function