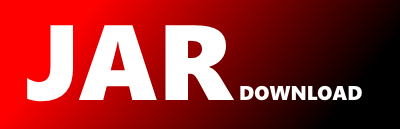
software.amazon.awssdk.services.dataexchange.model.GetJobResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dataexchange.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetJobResponse extends DataExchangeResponse implements
ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(GetJobResponse::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("CreatedAt")
.getter(getter(GetJobResponse::createdAt))
.setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField DETAILS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Details").getter(getter(GetJobResponse::details)).setter(setter(Builder::details))
.constructor(ResponseDetails::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Details").build()).build();
private static final SdkField> ERRORS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Errors")
.getter(getter(GetJobResponse::errors))
.setter(setter(Builder::errors))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Errors").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(JobError::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(GetJobResponse::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("State")
.getter(getter(GetJobResponse::stateAsString)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("State").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(GetJobResponse::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("UpdatedAt")
.getter(getter(GetJobResponse::updatedAt))
.setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdatedAt").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, CREATED_AT_FIELD,
DETAILS_FIELD, ERRORS_FIELD, ID_FIELD, STATE_FIELD, TYPE_FIELD, UPDATED_AT_FIELD));
private final String arn;
private final Instant createdAt;
private final ResponseDetails details;
private final List errors;
private final String id;
private final String state;
private final String type;
private final Instant updatedAt;
private GetJobResponse(BuilderImpl builder) {
super(builder);
this.arn = builder.arn;
this.createdAt = builder.createdAt;
this.details = builder.details;
this.errors = builder.errors;
this.id = builder.id;
this.state = builder.state;
this.type = builder.type;
this.updatedAt = builder.updatedAt;
}
/**
*
* The ARN for the job.
*
*
* @return The ARN for the job.
*/
public final String arn() {
return arn;
}
/**
*
* The date and time that the job was created, in ISO 8601 format.
*
*
* @return The date and time that the job was created, in ISO 8601 format.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* Details about the job.
*
*
* @return Details about the job.
*/
public final ResponseDetails details() {
return details;
}
/**
* For responses, this returns true if the service returned a value for the Errors property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasErrors() {
return errors != null && !(errors instanceof SdkAutoConstructList);
}
/**
*
* The errors associated with jobs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasErrors} method.
*
*
* @return The errors associated with jobs.
*/
public final List errors() {
return errors;
}
/**
*
* The unique identifier for the job.
*
*
* @return The unique identifier for the job.
*/
public final String id() {
return id;
}
/**
*
* The state of the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link State#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the job.
* @see State
*/
public final State state() {
return State.fromValue(state);
}
/**
*
* The state of the job.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link State#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the job.
* @see State
*/
public final String stateAsString() {
return state;
}
/**
*
* The job type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link Type#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The job type.
* @see Type
*/
public final Type type() {
return Type.fromValue(type);
}
/**
*
* The job type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link Type#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The job type.
* @see Type
*/
public final String typeAsString() {
return type;
}
/**
*
* The date and time that the job was last updated, in ISO 8601 format.
*
*
* @return The date and time that the job was last updated, in ISO 8601 format.
*/
public final Instant updatedAt() {
return updatedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(details());
hashCode = 31 * hashCode + Objects.hashCode(hasErrors() ? errors() : null);
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetJobResponse)) {
return false;
}
GetJobResponse other = (GetJobResponse) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(details(), other.details()) && hasErrors() == other.hasErrors()
&& Objects.equals(errors(), other.errors()) && Objects.equals(id(), other.id())
&& Objects.equals(stateAsString(), other.stateAsString()) && Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(updatedAt(), other.updatedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetJobResponse").add("Arn", arn()).add("CreatedAt", createdAt()).add("Details", details())
.add("Errors", hasErrors() ? errors() : null).add("Id", id()).add("State", stateAsString())
.add("Type", typeAsString()).add("UpdatedAt", updatedAt()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "Details":
return Optional.ofNullable(clazz.cast(details()));
case "Errors":
return Optional.ofNullable(clazz.cast(errors()));
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "State":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "UpdatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function