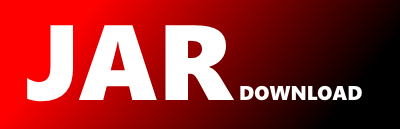
software.amazon.awssdk.services.dataexchange.model.ListDataSetsResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dataexchange.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListDataSetsResponse extends DataExchangeResponse implements
ToCopyableBuilder {
private static final SdkField> DATA_SETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DataSets")
.getter(getter(ListDataSetsResponse::dataSets))
.setter(setter(Builder::dataSets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DataSetEntry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NextToken").getter(getter(ListDataSetsResponse::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NextToken").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATA_SETS_FIELD,
NEXT_TOKEN_FIELD));
private final List dataSets;
private final String nextToken;
private ListDataSetsResponse(BuilderImpl builder) {
super(builder);
this.dataSets = builder.dataSets;
this.nextToken = builder.nextToken;
}
/**
* For responses, this returns true if the service returned a value for the DataSets property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasDataSets() {
return dataSets != null && !(dataSets instanceof SdkAutoConstructList);
}
/**
*
* The data set objects listed by the request.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDataSets} method.
*
*
* @return The data set objects listed by the request.
*/
public final List dataSets() {
return dataSets;
}
/**
*
* The token value retrieved from a previous call to access the next page of results.
*
*
* @return The token value retrieved from a previous call to access the next page of results.
*/
public final String nextToken() {
return nextToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hasDataSets() ? dataSets() : null);
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListDataSetsResponse)) {
return false;
}
ListDataSetsResponse other = (ListDataSetsResponse) obj;
return hasDataSets() == other.hasDataSets() && Objects.equals(dataSets(), other.dataSets())
&& Objects.equals(nextToken(), other.nextToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListDataSetsResponse").add("DataSets", hasDataSets() ? dataSets() : null)
.add("NextToken", nextToken()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DataSets":
return Optional.ofNullable(clazz.cast(dataSets()));
case "NextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy