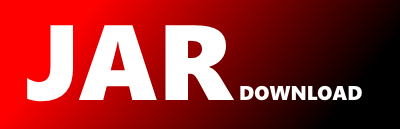
software.amazon.awssdk.services.dax.model.CreateClusterRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dax.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateClusterRequest extends DaxRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateClusterRequest::clusterName)).setter(setter(Builder::clusterName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterName").build()).build();
private static final SdkField NODE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateClusterRequest::nodeType)).setter(setter(Builder::nodeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NodeType").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateClusterRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField REPLICATION_FACTOR_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(CreateClusterRequest::replicationFactor)).setter(setter(Builder::replicationFactor))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationFactor").build()).build();
private static final SdkField> AVAILABILITY_ZONES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateClusterRequest::availabilityZones))
.setter(setter(Builder::availabilityZones))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZones").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateClusterRequest::subnetGroupName)).setter(setter(Builder::subnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetGroupName").build()).build();
private static final SdkField> SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateClusterRequest::securityGroupIds))
.setter(setter(Builder::securityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(CreateClusterRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField NOTIFICATION_TOPIC_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateClusterRequest::notificationTopicArn)).setter(setter(Builder::notificationTopicArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotificationTopicArn").build())
.build();
private static final SdkField IAM_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateClusterRequest::iamRoleArn)).setter(setter(Builder::iamRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamRoleArn").build()).build();
private static final SdkField PARAMETER_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateClusterRequest::parameterGroupName)).setter(setter(Builder::parameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParameterGroupName").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateClusterRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SSE_SPECIFICATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(CreateClusterRequest::sseSpecification))
.setter(setter(Builder::sseSpecification)).constructor(SSESpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SSESpecification").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_NAME_FIELD,
NODE_TYPE_FIELD, DESCRIPTION_FIELD, REPLICATION_FACTOR_FIELD, AVAILABILITY_ZONES_FIELD, SUBNET_GROUP_NAME_FIELD,
SECURITY_GROUP_IDS_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD, NOTIFICATION_TOPIC_ARN_FIELD, IAM_ROLE_ARN_FIELD,
PARAMETER_GROUP_NAME_FIELD, TAGS_FIELD, SSE_SPECIFICATION_FIELD));
private final String clusterName;
private final String nodeType;
private final String description;
private final Integer replicationFactor;
private final List availabilityZones;
private final String subnetGroupName;
private final List securityGroupIds;
private final String preferredMaintenanceWindow;
private final String notificationTopicArn;
private final String iamRoleArn;
private final String parameterGroupName;
private final List tags;
private final SSESpecification sseSpecification;
private CreateClusterRequest(BuilderImpl builder) {
super(builder);
this.clusterName = builder.clusterName;
this.nodeType = builder.nodeType;
this.description = builder.description;
this.replicationFactor = builder.replicationFactor;
this.availabilityZones = builder.availabilityZones;
this.subnetGroupName = builder.subnetGroupName;
this.securityGroupIds = builder.securityGroupIds;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.notificationTopicArn = builder.notificationTopicArn;
this.iamRoleArn = builder.iamRoleArn;
this.parameterGroupName = builder.parameterGroupName;
this.tags = builder.tags;
this.sseSpecification = builder.sseSpecification;
}
/**
*
* The cluster identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 20 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @return The cluster identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* A name must contain from 1 to 20 alphanumeric characters or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* A name cannot end with a hyphen or contain two consecutive hyphens.
*
*
*/
public String clusterName() {
return clusterName;
}
/**
*
* The compute and memory capacity of the nodes in the cluster.
*
*
* @return The compute and memory capacity of the nodes in the cluster.
*/
public String nodeType() {
return nodeType;
}
/**
*
* A description of the cluster.
*
*
* @return A description of the cluster.
*/
public String description() {
return description;
}
/**
*
* The number of nodes in the DAX cluster. A replication factor of 1 will create a single-node cluster, without any
* read replicas. For additional fault tolerance, you can create a multiple node cluster with one or more read
* replicas. To do this, set ReplicationFactor
to a number between 3 (one primary and two read
* replicas) and 10 (one primary and nine read replicas). If the AvailabilityZones
parameter is
* provided, its length must equal the ReplicationFactor
.
*
*
*
* AWS recommends that you have at least two read replicas per cluster.
*
*
*
* @return The number of nodes in the DAX cluster. A replication factor of 1 will create a single-node cluster,
* without any read replicas. For additional fault tolerance, you can create a multiple node cluster with
* one or more read replicas. To do this, set ReplicationFactor
to a number between 3 (one
* primary and two read replicas) and 10 (one primary and nine read replicas).
* If the AvailabilityZones
parameter is provided, its length must equal the
* ReplicationFactor
.
*
* AWS recommends that you have at least two read replicas per cluster.
*
*/
public Integer replicationFactor() {
return replicationFactor;
}
/**
* Returns true if the AvailabilityZones property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasAvailabilityZones() {
return availabilityZones != null && !(availabilityZones instanceof SdkAutoConstructList);
}
/**
*
* The Availability Zones (AZs) in which the cluster nodes will reside after the cluster has been created or
* updated. If provided, the length of this list must equal the ReplicationFactor
parameter. If you
* omit this parameter, DAX will spread the nodes across Availability Zones for the highest availability.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAvailabilityZones()} to see if a value was sent in this field.
*
*
* @return The Availability Zones (AZs) in which the cluster nodes will reside after the cluster has been created or
* updated. If provided, the length of this list must equal the ReplicationFactor
parameter. If
* you omit this parameter, DAX will spread the nodes across Availability Zones for the highest
* availability.
*/
public List availabilityZones() {
return availabilityZones;
}
/**
*
* The name of the subnet group to be used for the replication group.
*
*
*
* DAX clusters can only run in an Amazon VPC environment. All of the subnets that you specify in a subnet group
* must exist in the same VPC.
*
*
*
* @return The name of the subnet group to be used for the replication group.
*
* DAX clusters can only run in an Amazon VPC environment. All of the subnets that you specify in a subnet
* group must exist in the same VPC.
*
*/
public String subnetGroupName() {
return subnetGroupName;
}
/**
* Returns true if the SecurityGroupIds property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasSecurityGroupIds() {
return securityGroupIds != null && !(securityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* A list of security group IDs to be assigned to each node in the DAX cluster. (Each of the security group ID is
* system-generated.)
*
*
* If this parameter is not specified, DAX assigns the default VPC security group to each node.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSecurityGroupIds()} to see if a value was sent in this field.
*
*
* @return A list of security group IDs to be assigned to each node in the DAX cluster. (Each of the security group
* ID is system-generated.)
*
* If this parameter is not specified, DAX assigns the default VPC security group to each node.
*/
public List securityGroupIds() {
return securityGroupIds;
}
/**
*
* Specifies the weekly time range during which maintenance on the DAX cluster is performed. It is specified as a
* range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute
* period. Valid values for ddd
are:
*
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:05:00-sun:09:00
*
*
*
* If you don't specify a preferred maintenance window when you create or modify a cache cluster, DAX assigns a
* 60-minute maintenance window on a randomly selected day of the week.
*
*
*
* @return Specifies the weekly time range during which maintenance on the DAX cluster is performed. It is specified
* as a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60
* minute period. Valid values for ddd
are:
*
* -
*
* sun
*
*
* -
*
* mon
*
*
* -
*
* tue
*
*
* -
*
* wed
*
*
* -
*
* thu
*
*
* -
*
* fri
*
*
* -
*
* sat
*
*
*
*
* Example: sun:05:00-sun:09:00
*
*
*
* If you don't specify a preferred maintenance window when you create or modify a cache cluster, DAX
* assigns a 60-minute maintenance window on a randomly selected day of the week.
*
*/
public String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications will be sent.
*
*
*
* The Amazon SNS topic owner must be same as the DAX cluster owner.
*
*
*
* @return The Amazon Resource Name (ARN) of the Amazon SNS topic to which notifications will be sent.
*
* The Amazon SNS topic owner must be same as the DAX cluster owner.
*
*/
public String notificationTopicArn() {
return notificationTopicArn;
}
/**
*
* A valid Amazon Resource Name (ARN) that identifies an IAM role. At runtime, DAX will assume this role and use the
* role's permissions to access DynamoDB on your behalf.
*
*
* @return A valid Amazon Resource Name (ARN) that identifies an IAM role. At runtime, DAX will assume this role and
* use the role's permissions to access DynamoDB on your behalf.
*/
public String iamRoleArn() {
return iamRoleArn;
}
/**
*
* The parameter group to be associated with the DAX cluster.
*
*
* @return The parameter group to be associated with the DAX cluster.
*/
public String parameterGroupName() {
return parameterGroupName;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A set of tags to associate with the DAX cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return A set of tags to associate with the DAX cluster.
*/
public List tags() {
return tags;
}
/**
*
* Represents the settings used to enable server-side encryption on the cluster.
*
*
* @return Represents the settings used to enable server-side encryption on the cluster.
*/
public SSESpecification sseSpecification() {
return sseSpecification;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(clusterName());
hashCode = 31 * hashCode + Objects.hashCode(nodeType());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(replicationFactor());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZones());
hashCode = 31 * hashCode + Objects.hashCode(subnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(securityGroupIds());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(notificationTopicArn());
hashCode = 31 * hashCode + Objects.hashCode(iamRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(parameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(tags());
hashCode = 31 * hashCode + Objects.hashCode(sseSpecification());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateClusterRequest)) {
return false;
}
CreateClusterRequest other = (CreateClusterRequest) obj;
return Objects.equals(clusterName(), other.clusterName()) && Objects.equals(nodeType(), other.nodeType())
&& Objects.equals(description(), other.description())
&& Objects.equals(replicationFactor(), other.replicationFactor())
&& Objects.equals(availabilityZones(), other.availabilityZones())
&& Objects.equals(subnetGroupName(), other.subnetGroupName())
&& Objects.equals(securityGroupIds(), other.securityGroupIds())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(notificationTopicArn(), other.notificationTopicArn())
&& Objects.equals(iamRoleArn(), other.iamRoleArn())
&& Objects.equals(parameterGroupName(), other.parameterGroupName()) && Objects.equals(tags(), other.tags())
&& Objects.equals(sseSpecification(), other.sseSpecification());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CreateClusterRequest").add("ClusterName", clusterName()).add("NodeType", nodeType())
.add("Description", description()).add("ReplicationFactor", replicationFactor())
.add("AvailabilityZones", availabilityZones()).add("SubnetGroupName", subnetGroupName())
.add("SecurityGroupIds", securityGroupIds()).add("PreferredMaintenanceWindow", preferredMaintenanceWindow())
.add("NotificationTopicArn", notificationTopicArn()).add("IamRoleArn", iamRoleArn())
.add("ParameterGroupName", parameterGroupName()).add("Tags", tags()).add("SSESpecification", sseSpecification())
.build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClusterName":
return Optional.ofNullable(clazz.cast(clusterName()));
case "NodeType":
return Optional.ofNullable(clazz.cast(nodeType()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "ReplicationFactor":
return Optional.ofNullable(clazz.cast(replicationFactor()));
case "AvailabilityZones":
return Optional.ofNullable(clazz.cast(availabilityZones()));
case "SubnetGroupName":
return Optional.ofNullable(clazz.cast(subnetGroupName()));
case "SecurityGroupIds":
return Optional.ofNullable(clazz.cast(securityGroupIds()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "NotificationTopicArn":
return Optional.ofNullable(clazz.cast(notificationTopicArn()));
case "IamRoleArn":
return Optional.ofNullable(clazz.cast(iamRoleArn()));
case "ParameterGroupName":
return Optional.ofNullable(clazz.cast(parameterGroupName()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "SSESpecification":
return Optional.ofNullable(clazz.cast(sseSpecification()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function