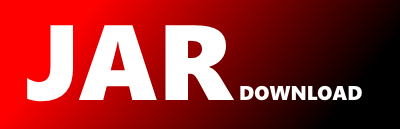
software.amazon.awssdk.services.dax.DefaultDaxClient Maven / Gradle / Ivy
Show all versions of dax Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dax;
import java.util.Collections;
import java.util.List;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.dax.model.ClusterAlreadyExistsException;
import software.amazon.awssdk.services.dax.model.ClusterNotFoundException;
import software.amazon.awssdk.services.dax.model.ClusterQuotaForCustomerExceededException;
import software.amazon.awssdk.services.dax.model.CreateClusterRequest;
import software.amazon.awssdk.services.dax.model.CreateClusterResponse;
import software.amazon.awssdk.services.dax.model.CreateParameterGroupRequest;
import software.amazon.awssdk.services.dax.model.CreateParameterGroupResponse;
import software.amazon.awssdk.services.dax.model.CreateSubnetGroupRequest;
import software.amazon.awssdk.services.dax.model.CreateSubnetGroupResponse;
import software.amazon.awssdk.services.dax.model.DaxException;
import software.amazon.awssdk.services.dax.model.DecreaseReplicationFactorRequest;
import software.amazon.awssdk.services.dax.model.DecreaseReplicationFactorResponse;
import software.amazon.awssdk.services.dax.model.DeleteClusterRequest;
import software.amazon.awssdk.services.dax.model.DeleteClusterResponse;
import software.amazon.awssdk.services.dax.model.DeleteParameterGroupRequest;
import software.amazon.awssdk.services.dax.model.DeleteParameterGroupResponse;
import software.amazon.awssdk.services.dax.model.DeleteSubnetGroupRequest;
import software.amazon.awssdk.services.dax.model.DeleteSubnetGroupResponse;
import software.amazon.awssdk.services.dax.model.DescribeClustersRequest;
import software.amazon.awssdk.services.dax.model.DescribeClustersResponse;
import software.amazon.awssdk.services.dax.model.DescribeDefaultParametersRequest;
import software.amazon.awssdk.services.dax.model.DescribeDefaultParametersResponse;
import software.amazon.awssdk.services.dax.model.DescribeEventsRequest;
import software.amazon.awssdk.services.dax.model.DescribeEventsResponse;
import software.amazon.awssdk.services.dax.model.DescribeParameterGroupsRequest;
import software.amazon.awssdk.services.dax.model.DescribeParameterGroupsResponse;
import software.amazon.awssdk.services.dax.model.DescribeParametersRequest;
import software.amazon.awssdk.services.dax.model.DescribeParametersResponse;
import software.amazon.awssdk.services.dax.model.DescribeSubnetGroupsRequest;
import software.amazon.awssdk.services.dax.model.DescribeSubnetGroupsResponse;
import software.amazon.awssdk.services.dax.model.IncreaseReplicationFactorRequest;
import software.amazon.awssdk.services.dax.model.IncreaseReplicationFactorResponse;
import software.amazon.awssdk.services.dax.model.InsufficientClusterCapacityException;
import software.amazon.awssdk.services.dax.model.InvalidArnException;
import software.amazon.awssdk.services.dax.model.InvalidClusterStateException;
import software.amazon.awssdk.services.dax.model.InvalidParameterCombinationException;
import software.amazon.awssdk.services.dax.model.InvalidParameterGroupStateException;
import software.amazon.awssdk.services.dax.model.InvalidParameterValueException;
import software.amazon.awssdk.services.dax.model.InvalidSubnetException;
import software.amazon.awssdk.services.dax.model.InvalidVpcNetworkStateException;
import software.amazon.awssdk.services.dax.model.ListTagsRequest;
import software.amazon.awssdk.services.dax.model.ListTagsResponse;
import software.amazon.awssdk.services.dax.model.NodeNotFoundException;
import software.amazon.awssdk.services.dax.model.NodeQuotaForClusterExceededException;
import software.amazon.awssdk.services.dax.model.NodeQuotaForCustomerExceededException;
import software.amazon.awssdk.services.dax.model.ParameterGroupAlreadyExistsException;
import software.amazon.awssdk.services.dax.model.ParameterGroupNotFoundException;
import software.amazon.awssdk.services.dax.model.ParameterGroupQuotaExceededException;
import software.amazon.awssdk.services.dax.model.RebootNodeRequest;
import software.amazon.awssdk.services.dax.model.RebootNodeResponse;
import software.amazon.awssdk.services.dax.model.ServiceLinkedRoleNotFoundException;
import software.amazon.awssdk.services.dax.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.dax.model.SubnetGroupAlreadyExistsException;
import software.amazon.awssdk.services.dax.model.SubnetGroupInUseException;
import software.amazon.awssdk.services.dax.model.SubnetGroupNotFoundException;
import software.amazon.awssdk.services.dax.model.SubnetGroupQuotaExceededException;
import software.amazon.awssdk.services.dax.model.SubnetInUseException;
import software.amazon.awssdk.services.dax.model.SubnetQuotaExceededException;
import software.amazon.awssdk.services.dax.model.TagNotFoundException;
import software.amazon.awssdk.services.dax.model.TagQuotaPerResourceExceededException;
import software.amazon.awssdk.services.dax.model.TagResourceRequest;
import software.amazon.awssdk.services.dax.model.TagResourceResponse;
import software.amazon.awssdk.services.dax.model.UntagResourceRequest;
import software.amazon.awssdk.services.dax.model.UntagResourceResponse;
import software.amazon.awssdk.services.dax.model.UpdateClusterRequest;
import software.amazon.awssdk.services.dax.model.UpdateClusterResponse;
import software.amazon.awssdk.services.dax.model.UpdateParameterGroupRequest;
import software.amazon.awssdk.services.dax.model.UpdateParameterGroupResponse;
import software.amazon.awssdk.services.dax.model.UpdateSubnetGroupRequest;
import software.amazon.awssdk.services.dax.model.UpdateSubnetGroupResponse;
import software.amazon.awssdk.services.dax.transform.CreateClusterRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.CreateParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.CreateSubnetGroupRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DecreaseReplicationFactorRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DeleteClusterRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DeleteParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DeleteSubnetGroupRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DescribeClustersRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DescribeDefaultParametersRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DescribeEventsRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DescribeParameterGroupsRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DescribeParametersRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.DescribeSubnetGroupsRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.IncreaseReplicationFactorRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.ListTagsRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.RebootNodeRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.UpdateClusterRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.UpdateParameterGroupRequestMarshaller;
import software.amazon.awssdk.services.dax.transform.UpdateSubnetGroupRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link DaxClient}.
*
* @see DaxClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultDaxClient implements DaxClient {
private static final Logger log = Logger.loggerFor(DefaultDaxClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultDaxClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates a DAX cluster. All nodes in the cluster run the same DAX caching software.
*
*
* @param createClusterRequest
* @return Result of the CreateCluster operation returned by the service.
* @throws ClusterAlreadyExistsException
* You already have a DAX cluster with the given identifier.
* @throws InvalidClusterStateException
* The requested DAX cluster is not in the available state.
* @throws InsufficientClusterCapacityException
* There are not enough system resources to create the cluster you requested (or to resize an
* already-existing cluster).
* @throws SubnetGroupNotFoundException
* The requested subnet group name does not refer to an existing subnet group.
* @throws InvalidParameterGroupStateException
* One or more parameters in a parameter group are in an invalid state.
* @throws ParameterGroupNotFoundException
* The specified parameter group does not exist.
* @throws ClusterQuotaForCustomerExceededException
* You have attempted to exceed the maximum number of DAX clusters for your AWS account.
* @throws NodeQuotaForClusterExceededException
* You have attempted to exceed the maximum number of nodes for a DAX cluster.
* @throws NodeQuotaForCustomerExceededException
* You have attempted to exceed the maximum number of nodes for your AWS account.
* @throws InvalidVpcNetworkStateException
* The VPC network is in an invalid state.
* @throws TagQuotaPerResourceExceededException
* You have exceeded the maximum number of tags for this DAX cluster.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws ServiceQuotaExceededException
* You have reached the maximum number of x509 certificates that can be created for encrypted clusters in a
* 30 day period. Contact AWS customer support to discuss options for continuing to create encrypted
* clusters.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.CreateCluster
* @see AWS API
* Documentation
*/
@Override
public CreateClusterResponse createCluster(CreateClusterRequest createClusterRequest) throws ClusterAlreadyExistsException,
InvalidClusterStateException, InsufficientClusterCapacityException, SubnetGroupNotFoundException,
InvalidParameterGroupStateException, ParameterGroupNotFoundException, ClusterQuotaForCustomerExceededException,
NodeQuotaForClusterExceededException, NodeQuotaForCustomerExceededException, InvalidVpcNetworkStateException,
TagQuotaPerResourceExceededException, ServiceLinkedRoleNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, ServiceQuotaExceededException, AwsServiceException, SdkClientException,
DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateCluster").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new parameter group. A parameter group is a collection of parameters that you apply to all of the nodes
* in a DAX cluster.
*
*
* @param createParameterGroupRequest
* @return Result of the CreateParameterGroup operation returned by the service.
* @throws ParameterGroupQuotaExceededException
* You have attempted to exceed the maximum number of parameter groups.
* @throws ParameterGroupAlreadyExistsException
* The specified parameter group already exists.
* @throws InvalidParameterGroupStateException
* One or more parameters in a parameter group are in an invalid state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.CreateParameterGroup
* @see AWS API
* Documentation
*/
@Override
public CreateParameterGroupResponse createParameterGroup(CreateParameterGroupRequest createParameterGroupRequest)
throws ParameterGroupQuotaExceededException, ParameterGroupAlreadyExistsException,
InvalidParameterGroupStateException, ServiceLinkedRoleNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateParameterGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createParameterGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateParameterGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateParameterGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createParameterGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateParameterGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new subnet group.
*
*
* @param createSubnetGroupRequest
* @return Result of the CreateSubnetGroup operation returned by the service.
* @throws SubnetGroupAlreadyExistsException
* The specified subnet group already exists.
* @throws SubnetGroupQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of subnets in a subnet group.
* @throws SubnetQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of subnets in a subnet group.
* @throws InvalidSubnetException
* An invalid subnet identifier was specified.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.CreateSubnetGroup
* @see AWS API
* Documentation
*/
@Override
public CreateSubnetGroupResponse createSubnetGroup(CreateSubnetGroupRequest createSubnetGroupRequest)
throws SubnetGroupAlreadyExistsException, SubnetGroupQuotaExceededException, SubnetQuotaExceededException,
InvalidSubnetException, ServiceLinkedRoleNotFoundException, AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateSubnetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSubnetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSubnetGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateSubnetGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createSubnetGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateSubnetGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes one or more nodes from a DAX cluster.
*
*
*
* You cannot use DecreaseReplicationFactor
to remove the last node in a DAX cluster. If you need to do
* this, use DeleteCluster
instead.
*
*
*
* @param decreaseReplicationFactorRequest
* @return Result of the DecreaseReplicationFactor operation returned by the service.
* @throws ClusterNotFoundException
* The requested cluster ID does not refer to an existing DAX cluster.
* @throws NodeNotFoundException
* None of the nodes in the cluster have the given node ID.
* @throws InvalidClusterStateException
* The requested DAX cluster is not in the available state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DecreaseReplicationFactor
* @see AWS
* API Documentation
*/
@Override
public DecreaseReplicationFactorResponse decreaseReplicationFactor(
DecreaseReplicationFactorRequest decreaseReplicationFactorRequest) throws ClusterNotFoundException,
NodeNotFoundException, InvalidClusterStateException, ServiceLinkedRoleNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DecreaseReplicationFactorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, decreaseReplicationFactorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DecreaseReplicationFactor");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DecreaseReplicationFactor").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(decreaseReplicationFactorRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DecreaseReplicationFactorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a previously provisioned DAX cluster. DeleteCluster deletes all associated nodes, node endpoints
* and the DAX cluster itself. When you receive a successful response from this action, DAX immediately begins
* deleting the cluster; you cannot cancel or revert this action.
*
*
* @param deleteClusterRequest
* @return Result of the DeleteCluster operation returned by the service.
* @throws ClusterNotFoundException
* The requested cluster ID does not refer to an existing DAX cluster.
* @throws InvalidClusterStateException
* The requested DAX cluster is not in the available state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DeleteCluster
* @see AWS API
* Documentation
*/
@Override
public DeleteClusterResponse deleteCluster(DeleteClusterRequest deleteClusterRequest) throws ClusterNotFoundException,
InvalidClusterStateException, ServiceLinkedRoleNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteCluster").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified parameter group. You cannot delete a parameter group if it is associated with any DAX
* clusters.
*
*
* @param deleteParameterGroupRequest
* @return Result of the DeleteParameterGroup operation returned by the service.
* @throws InvalidParameterGroupStateException
* One or more parameters in a parameter group are in an invalid state.
* @throws ParameterGroupNotFoundException
* The specified parameter group does not exist.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DeleteParameterGroup
* @see AWS API
* Documentation
*/
@Override
public DeleteParameterGroupResponse deleteParameterGroup(DeleteParameterGroupRequest deleteParameterGroupRequest)
throws InvalidParameterGroupStateException, ParameterGroupNotFoundException, ServiceLinkedRoleNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteParameterGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteParameterGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteParameterGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteParameterGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteParameterGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteParameterGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a subnet group.
*
*
*
* You cannot delete a subnet group if it is associated with any DAX clusters.
*
*
*
* @param deleteSubnetGroupRequest
* @return Result of the DeleteSubnetGroup operation returned by the service.
* @throws SubnetGroupInUseException
* The specified subnet group is currently in use.
* @throws SubnetGroupNotFoundException
* The requested subnet group name does not refer to an existing subnet group.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DeleteSubnetGroup
* @see AWS API
* Documentation
*/
@Override
public DeleteSubnetGroupResponse deleteSubnetGroup(DeleteSubnetGroupRequest deleteSubnetGroupRequest)
throws SubnetGroupInUseException, SubnetGroupNotFoundException, ServiceLinkedRoleNotFoundException,
AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteSubnetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSubnetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSubnetGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteSubnetGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteSubnetGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteSubnetGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about all provisioned DAX clusters if no cluster identifier is specified, or about a specific
* DAX cluster if a cluster identifier is supplied.
*
*
* If the cluster is in the CREATING state, only cluster level information will be displayed until all of the nodes
* are successfully provisioned.
*
*
* If the cluster is in the DELETING state, only cluster level information will be displayed.
*
*
* If nodes are currently being added to the DAX cluster, node endpoint information and creation time for the
* additional nodes will not be displayed until they are completely provisioned. When the DAX cluster state is
* available, the cluster is ready for use.
*
*
* If nodes are currently being removed from the DAX cluster, no endpoint information for the removed nodes is
* displayed.
*
*
* @param describeClustersRequest
* @return Result of the DescribeClusters operation returned by the service.
* @throws ClusterNotFoundException
* The requested cluster ID does not refer to an existing DAX cluster.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DescribeClusters
* @see AWS API
* Documentation
*/
@Override
public DescribeClustersResponse describeClusters(DescribeClustersRequest describeClustersRequest)
throws ClusterNotFoundException, ServiceLinkedRoleNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeClustersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeClustersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeClusters");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeClusters").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeClustersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeClustersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the default system parameter information for the DAX caching software.
*
*
* @param describeDefaultParametersRequest
* @return Result of the DescribeDefaultParameters operation returned by the service.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DescribeDefaultParameters
* @see AWS
* API Documentation
*/
@Override
public DescribeDefaultParametersResponse describeDefaultParameters(
DescribeDefaultParametersRequest describeDefaultParametersRequest) throws ServiceLinkedRoleNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDefaultParametersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDefaultParametersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDefaultParameters");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDefaultParameters").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeDefaultParametersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeDefaultParametersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns events related to DAX clusters and parameter groups. You can obtain events specific to a particular DAX
* cluster or parameter group by providing the name as a parameter.
*
*
* By default, only the events occurring within the last 24 hours are returned; however, you can retrieve up to 14
* days' worth of events if necessary.
*
*
* @param describeEventsRequest
* @return Result of the DescribeEvents operation returned by the service.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DescribeEvents
* @see AWS API
* Documentation
*/
@Override
public DescribeEventsResponse describeEvents(DescribeEventsRequest describeEventsRequest)
throws ServiceLinkedRoleNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeEventsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEventsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEvents");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeEvents").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeEventsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeEventsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of parameter group descriptions. If a parameter group name is specified, the list will contain
* only the descriptions for that group.
*
*
* @param describeParameterGroupsRequest
* @return Result of the DescribeParameterGroups operation returned by the service.
* @throws ParameterGroupNotFoundException
* The specified parameter group does not exist.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DescribeParameterGroups
* @see AWS
* API Documentation
*/
@Override
public DescribeParameterGroupsResponse describeParameterGroups(DescribeParameterGroupsRequest describeParameterGroupsRequest)
throws ParameterGroupNotFoundException, ServiceLinkedRoleNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeParameterGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeParameterGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeParameterGroups");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeParameterGroups").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeParameterGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeParameterGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the detailed parameter list for a particular parameter group.
*
*
* @param describeParametersRequest
* @return Result of the DescribeParameters operation returned by the service.
* @throws ParameterGroupNotFoundException
* The specified parameter group does not exist.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DescribeParameters
* @see AWS API
* Documentation
*/
@Override
public DescribeParametersResponse describeParameters(DescribeParametersRequest describeParametersRequest)
throws ParameterGroupNotFoundException, ServiceLinkedRoleNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeParametersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeParametersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeParameters");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeParameters").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeParametersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeParametersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of subnet group descriptions. If a subnet group name is specified, the list will contain only the
* description of that group.
*
*
* @param describeSubnetGroupsRequest
* @return Result of the DescribeSubnetGroups operation returned by the service.
* @throws SubnetGroupNotFoundException
* The requested subnet group name does not refer to an existing subnet group.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.DescribeSubnetGroups
* @see AWS API
* Documentation
*/
@Override
public DescribeSubnetGroupsResponse describeSubnetGroups(DescribeSubnetGroupsRequest describeSubnetGroupsRequest)
throws SubnetGroupNotFoundException, ServiceLinkedRoleNotFoundException, AwsServiceException, SdkClientException,
DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSubnetGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSubnetGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSubnetGroups");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeSubnetGroups").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeSubnetGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeSubnetGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds one or more nodes to a DAX cluster.
*
*
* @param increaseReplicationFactorRequest
* @return Result of the IncreaseReplicationFactor operation returned by the service.
* @throws ClusterNotFoundException
* The requested cluster ID does not refer to an existing DAX cluster.
* @throws InvalidClusterStateException
* The requested DAX cluster is not in the available state.
* @throws InsufficientClusterCapacityException
* There are not enough system resources to create the cluster you requested (or to resize an
* already-existing cluster).
* @throws InvalidVpcNetworkStateException
* The VPC network is in an invalid state.
* @throws NodeQuotaForClusterExceededException
* You have attempted to exceed the maximum number of nodes for a DAX cluster.
* @throws NodeQuotaForCustomerExceededException
* You have attempted to exceed the maximum number of nodes for your AWS account.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.IncreaseReplicationFactor
* @see AWS
* API Documentation
*/
@Override
public IncreaseReplicationFactorResponse increaseReplicationFactor(
IncreaseReplicationFactorRequest increaseReplicationFactorRequest) throws ClusterNotFoundException,
InvalidClusterStateException, InsufficientClusterCapacityException, InvalidVpcNetworkStateException,
NodeQuotaForClusterExceededException, NodeQuotaForCustomerExceededException, ServiceLinkedRoleNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, IncreaseReplicationFactorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, increaseReplicationFactorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "IncreaseReplicationFactor");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("IncreaseReplicationFactor").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(increaseReplicationFactorRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new IncreaseReplicationFactorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* List all of the tags for a DAX cluster. You can call ListTags
up to 10 times per second, per
* account.
*
*
* @param listTagsRequest
* @return Result of the ListTags operation returned by the service.
* @throws ClusterNotFoundException
* The requested cluster ID does not refer to an existing DAX cluster.
* @throws InvalidArnException
* The Amazon Resource Name (ARN) supplied in the request is not valid.
* @throws InvalidClusterStateException
* The requested DAX cluster is not in the available state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.ListTags
* @see AWS API
* Documentation
*/
@Override
public ListTagsResponse listTags(ListTagsRequest listTagsRequest) throws ClusterNotFoundException, InvalidArnException,
InvalidClusterStateException, ServiceLinkedRoleNotFoundException, InvalidParameterValueException,
InvalidParameterCombinationException, AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTags");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTags").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new ListTagsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Reboots a single node of a DAX cluster. The reboot action takes place as soon as possible. During the reboot, the
* node status is set to REBOOTING.
*
*
*
* RebootNode
restarts the DAX engine process and does not remove the contents of the cache.
*
*
*
* @param rebootNodeRequest
* @return Result of the RebootNode operation returned by the service.
* @throws ClusterNotFoundException
* The requested cluster ID does not refer to an existing DAX cluster.
* @throws NodeNotFoundException
* None of the nodes in the cluster have the given node ID.
* @throws InvalidClusterStateException
* The requested DAX cluster is not in the available state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.RebootNode
* @see AWS API
* Documentation
*/
@Override
public RebootNodeResponse rebootNode(RebootNodeRequest rebootNodeRequest) throws ClusterNotFoundException,
NodeNotFoundException, InvalidClusterStateException, ServiceLinkedRoleNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RebootNodeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, rebootNodeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RebootNode");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("RebootNode")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(rebootNodeRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RebootNodeRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates a set of tags with a DAX resource. You can call TagResource
up to 5 times per second, per
* account.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ClusterNotFoundException
* The requested cluster ID does not refer to an existing DAX cluster.
* @throws TagQuotaPerResourceExceededException
* You have exceeded the maximum number of tags for this DAX cluster.
* @throws InvalidArnException
* The Amazon Resource Name (ARN) supplied in the request is not valid.
* @throws InvalidClusterStateException
* The requested DAX cluster is not in the available state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ClusterNotFoundException,
TagQuotaPerResourceExceededException, InvalidArnException, InvalidClusterStateException,
ServiceLinkedRoleNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the association of tags from a DAX resource. You can call UntagResource
up to 5 times per
* second, per account.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ClusterNotFoundException
* The requested cluster ID does not refer to an existing DAX cluster.
* @throws InvalidArnException
* The Amazon Resource Name (ARN) supplied in the request is not valid.
* @throws TagNotFoundException
* The tag does not exist.
* @throws InvalidClusterStateException
* The requested DAX cluster is not in the available state.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ClusterNotFoundException,
InvalidArnException, TagNotFoundException, InvalidClusterStateException, ServiceLinkedRoleNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the settings for a DAX cluster. You can use this action to change one or more cluster configuration
* parameters by specifying the parameters and the new values.
*
*
* @param updateClusterRequest
* @return Result of the UpdateCluster operation returned by the service.
* @throws InvalidClusterStateException
* The requested DAX cluster is not in the available state.
* @throws ClusterNotFoundException
* The requested cluster ID does not refer to an existing DAX cluster.
* @throws InvalidParameterGroupStateException
* One or more parameters in a parameter group are in an invalid state.
* @throws ParameterGroupNotFoundException
* The specified parameter group does not exist.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.UpdateCluster
* @see AWS API
* Documentation
*/
@Override
public UpdateClusterResponse updateCluster(UpdateClusterRequest updateClusterRequest) throws InvalidClusterStateException,
ClusterNotFoundException, InvalidParameterGroupStateException, ParameterGroupNotFoundException,
ServiceLinkedRoleNotFoundException, InvalidParameterValueException, InvalidParameterCombinationException,
AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateCluster").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the parameters of a parameter group. You can modify up to 20 parameters in a single request by
* submitting a list parameter name and value pairs.
*
*
* @param updateParameterGroupRequest
* @return Result of the UpdateParameterGroup operation returned by the service.
* @throws InvalidParameterGroupStateException
* One or more parameters in a parameter group are in an invalid state.
* @throws ParameterGroupNotFoundException
* The specified parameter group does not exist.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws InvalidParameterValueException
* The value for a parameter is invalid.
* @throws InvalidParameterCombinationException
* Two or more incompatible parameters were specified.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.UpdateParameterGroup
* @see AWS API
* Documentation
*/
@Override
public UpdateParameterGroupResponse updateParameterGroup(UpdateParameterGroupRequest updateParameterGroupRequest)
throws InvalidParameterGroupStateException, ParameterGroupNotFoundException, ServiceLinkedRoleNotFoundException,
InvalidParameterValueException, InvalidParameterCombinationException, AwsServiceException, SdkClientException,
DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateParameterGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateParameterGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateParameterGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateParameterGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateParameterGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateParameterGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies an existing subnet group.
*
*
* @param updateSubnetGroupRequest
* @return Result of the UpdateSubnetGroup operation returned by the service.
* @throws SubnetGroupNotFoundException
* The requested subnet group name does not refer to an existing subnet group.
* @throws SubnetQuotaExceededException
* The request cannot be processed because it would exceed the allowed number of subnets in a subnet group.
* @throws SubnetInUseException
* The requested subnet is being used by another subnet group.
* @throws InvalidSubnetException
* An invalid subnet identifier was specified.
* @throws ServiceLinkedRoleNotFoundException
* The specified service linked role (SLR) was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DaxException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DaxClient.UpdateSubnetGroup
* @see AWS API
* Documentation
*/
@Override
public UpdateSubnetGroupResponse updateSubnetGroup(UpdateSubnetGroupRequest updateSubnetGroupRequest)
throws SubnetGroupNotFoundException, SubnetQuotaExceededException, SubnetInUseException, InvalidSubnetException,
ServiceLinkedRoleNotFoundException, AwsServiceException, SdkClientException, DaxException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateSubnetGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateSubnetGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "DAX");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateSubnetGroup");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateSubnetGroup").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateSubnetGroupRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateSubnetGroupRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(DaxException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("SubnetQuotaExceededFault")
.exceptionBuilderSupplier(SubnetQuotaExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SubnetGroupNotFoundFault")
.exceptionBuilderSupplier(SubnetGroupNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SubnetGroupQuotaExceededFault")
.exceptionBuilderSupplier(SubnetGroupQuotaExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ParameterGroupAlreadyExistsFault")
.exceptionBuilderSupplier(ParameterGroupAlreadyExistsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SubnetInUse")
.exceptionBuilderSupplier(SubnetInUseException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ClusterNotFoundFault")
.exceptionBuilderSupplier(ClusterNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterValueException")
.exceptionBuilderSupplier(InvalidParameterValueException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NodeNotFoundFault")
.exceptionBuilderSupplier(NodeNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidARNFault")
.exceptionBuilderSupplier(InvalidArnException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterCombinationException")
.exceptionBuilderSupplier(InvalidParameterCombinationException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NodeQuotaForCustomerExceededFault")
.exceptionBuilderSupplier(NodeQuotaForCustomerExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidClusterStateFault")
.exceptionBuilderSupplier(InvalidClusterStateException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InsufficientClusterCapacityFault")
.exceptionBuilderSupplier(InsufficientClusterCapacityException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TagNotFoundFault")
.exceptionBuilderSupplier(TagNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidVPCNetworkStateFault")
.exceptionBuilderSupplier(InvalidVpcNetworkStateException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TagQuotaPerResourceExceeded")
.exceptionBuilderSupplier(TagQuotaPerResourceExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ClusterQuotaForCustomerExceededFault")
.exceptionBuilderSupplier(ClusterQuotaForCustomerExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SubnetGroupInUseFault")
.exceptionBuilderSupplier(SubnetGroupInUseException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterGroupStateFault")
.exceptionBuilderSupplier(InvalidParameterGroupStateException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ParameterGroupNotFoundFault")
.exceptionBuilderSupplier(ParameterGroupNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSubnet")
.exceptionBuilderSupplier(InvalidSubnetException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ClusterAlreadyExistsFault")
.exceptionBuilderSupplier(ClusterAlreadyExistsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceLinkedRoleNotFoundFault")
.exceptionBuilderSupplier(ServiceLinkedRoleNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NodeQuotaForClusterExceededFault")
.exceptionBuilderSupplier(NodeQuotaForClusterExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ParameterGroupQuotaExceededFault")
.exceptionBuilderSupplier(ParameterGroupQuotaExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("SubnetGroupAlreadyExistsFault")
.exceptionBuilderSupplier(SubnetGroupAlreadyExistsException::builder).build());
}
@Override
public void close() {
clientHandler.close();
}
}