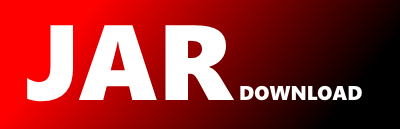
software.amazon.awssdk.services.deadline.model.JobEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deadline Show documentation
Show all versions of deadline Show documentation
The AWS Java SDK for Deadline module holds the client classes that are used for
communicating with Deadline.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.deadline.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.EnumSet;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructMap;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of a job entity.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class JobEntity implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField JOB_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("jobDetails").getter(getter(JobEntity::jobDetails))
.setter(setter(Builder::jobDetails)).constructor(JobDetailsEntity::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("jobDetails").build()).build();
private static final SdkField JOB_ATTACHMENT_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("jobAttachmentDetails")
.getter(getter(JobEntity::jobAttachmentDetails)).setter(setter(Builder::jobAttachmentDetails))
.constructor(JobAttachmentDetailsEntity::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("jobAttachmentDetails").build())
.build();
private static final SdkField STEP_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("stepDetails")
.getter(getter(JobEntity::stepDetails)).setter(setter(Builder::stepDetails)).constructor(StepDetailsEntity::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stepDetails").build()).build();
private static final SdkField ENVIRONMENT_DETAILS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("environmentDetails")
.getter(getter(JobEntity::environmentDetails)).setter(setter(Builder::environmentDetails))
.constructor(EnvironmentDetailsEntity::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("environmentDetails").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(JOB_DETAILS_FIELD,
JOB_ATTACHMENT_DETAILS_FIELD, STEP_DETAILS_FIELD, ENVIRONMENT_DETAILS_FIELD));
private static final long serialVersionUID = 1L;
private final JobDetailsEntity jobDetails;
private final JobAttachmentDetailsEntity jobAttachmentDetails;
private final StepDetailsEntity stepDetails;
private final EnvironmentDetailsEntity environmentDetails;
private final Type type;
private JobEntity(BuilderImpl builder) {
this.jobDetails = builder.jobDetails;
this.jobAttachmentDetails = builder.jobAttachmentDetails;
this.stepDetails = builder.stepDetails;
this.environmentDetails = builder.environmentDetails;
this.type = builder.type;
}
/**
*
* The job details.
*
*
* @return The job details.
*/
public final JobDetailsEntity jobDetails() {
return jobDetails;
}
/**
*
* The job attachment details.
*
*
* @return The job attachment details.
*/
public final JobAttachmentDetailsEntity jobAttachmentDetails() {
return jobAttachmentDetails;
}
/**
*
* The step details.
*
*
* @return The step details.
*/
public final StepDetailsEntity stepDetails() {
return stepDetails;
}
/**
*
* The environment details for the job entity.
*
*
* @return The environment details for the job entity.
*/
public final EnvironmentDetailsEntity environmentDetails() {
return environmentDetails;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(jobDetails());
hashCode = 31 * hashCode + Objects.hashCode(jobAttachmentDetails());
hashCode = 31 * hashCode + Objects.hashCode(stepDetails());
hashCode = 31 * hashCode + Objects.hashCode(environmentDetails());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof JobEntity)) {
return false;
}
JobEntity other = (JobEntity) obj;
return Objects.equals(jobDetails(), other.jobDetails())
&& Objects.equals(jobAttachmentDetails(), other.jobAttachmentDetails())
&& Objects.equals(stepDetails(), other.stepDetails())
&& Objects.equals(environmentDetails(), other.environmentDetails());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("JobEntity").add("JobDetails", jobDetails()).add("JobAttachmentDetails", jobAttachmentDetails())
.add("StepDetails", stepDetails()).add("EnvironmentDetails", environmentDetails()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "jobDetails":
return Optional.ofNullable(clazz.cast(jobDetails()));
case "jobAttachmentDetails":
return Optional.ofNullable(clazz.cast(jobAttachmentDetails()));
case "stepDetails":
return Optional.ofNullable(clazz.cast(stepDetails()));
case "environmentDetails":
return Optional.ofNullable(clazz.cast(environmentDetails()));
default:
return Optional.empty();
}
}
/**
* Create an instance of this class with {@link #jobDetails()} initialized to the given value.
*
*
* The job details.
*
*
* @param jobDetails
* The job details.
*/
public static JobEntity fromJobDetails(JobDetailsEntity jobDetails) {
return builder().jobDetails(jobDetails).build();
}
/**
* Create an instance of this class with {@link #jobDetails()} initialized to the given value.
*
*
* The job details.
*
*
* @param jobDetails
* The job details.
*/
public static JobEntity fromJobDetails(Consumer jobDetails) {
JobDetailsEntity.Builder builder = JobDetailsEntity.builder();
jobDetails.accept(builder);
return fromJobDetails(builder.build());
}
/**
* Create an instance of this class with {@link #jobAttachmentDetails()} initialized to the given value.
*
*
* The job attachment details.
*
*
* @param jobAttachmentDetails
* The job attachment details.
*/
public static JobEntity fromJobAttachmentDetails(JobAttachmentDetailsEntity jobAttachmentDetails) {
return builder().jobAttachmentDetails(jobAttachmentDetails).build();
}
/**
* Create an instance of this class with {@link #jobAttachmentDetails()} initialized to the given value.
*
*
* The job attachment details.
*
*
* @param jobAttachmentDetails
* The job attachment details.
*/
public static JobEntity fromJobAttachmentDetails(Consumer jobAttachmentDetails) {
JobAttachmentDetailsEntity.Builder builder = JobAttachmentDetailsEntity.builder();
jobAttachmentDetails.accept(builder);
return fromJobAttachmentDetails(builder.build());
}
/**
* Create an instance of this class with {@link #stepDetails()} initialized to the given value.
*
*
* The step details.
*
*
* @param stepDetails
* The step details.
*/
public static JobEntity fromStepDetails(StepDetailsEntity stepDetails) {
return builder().stepDetails(stepDetails).build();
}
/**
* Create an instance of this class with {@link #stepDetails()} initialized to the given value.
*
*
* The step details.
*
*
* @param stepDetails
* The step details.
*/
public static JobEntity fromStepDetails(Consumer stepDetails) {
StepDetailsEntity.Builder builder = StepDetailsEntity.builder();
stepDetails.accept(builder);
return fromStepDetails(builder.build());
}
/**
* Create an instance of this class with {@link #environmentDetails()} initialized to the given value.
*
*
* The environment details for the job entity.
*
*
* @param environmentDetails
* The environment details for the job entity.
*/
public static JobEntity fromEnvironmentDetails(EnvironmentDetailsEntity environmentDetails) {
return builder().environmentDetails(environmentDetails).build();
}
/**
* Create an instance of this class with {@link #environmentDetails()} initialized to the given value.
*
*
* The environment details for the job entity.
*
*
* @param environmentDetails
* The environment details for the job entity.
*/
public static JobEntity fromEnvironmentDetails(Consumer environmentDetails) {
EnvironmentDetailsEntity.Builder builder = EnvironmentDetailsEntity.builder();
environmentDetails.accept(builder);
return fromEnvironmentDetails(builder.build());
}
/**
* Retrieve an enum value representing which member of this object is populated.
*
* When this class is returned in a service response, this will be {@link Type#UNKNOWN_TO_SDK_VERSION} if the
* service returned a member that is only known to a newer SDK version.
*
* When this class is created directly in your code, this will be {@link Type#UNKNOWN_TO_SDK_VERSION} if zero
* members are set, and {@code null} if more than one member is set.
*/
public Type type() {
return type;
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy