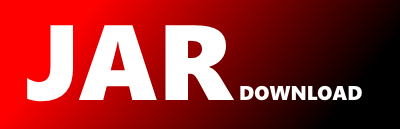
software.amazon.awssdk.services.deadline.model.LogConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deadline Show documentation
Show all versions of deadline Show documentation
The AWS Java SDK for Deadline module holds the client classes that are used for
communicating with Deadline.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.deadline.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.MapTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructMap;
import software.amazon.awssdk.core.util.SdkAutoConstructMap;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Log configuration details.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LogConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField LOG_DRIVER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("logDriver").getter(getter(LogConfiguration::logDriver)).setter(setter(Builder::logDriver))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logDriver").build()).build();
private static final SdkField
© 2015 - 2025 Weber Informatics LLC | Privacy Policy