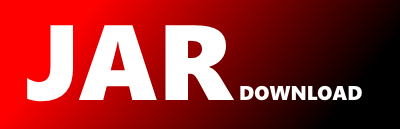
software.amazon.awssdk.services.detective.DefaultDetectiveClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.detective;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.detective.model.AcceptInvitationRequest;
import software.amazon.awssdk.services.detective.model.AcceptInvitationResponse;
import software.amazon.awssdk.services.detective.model.ConflictException;
import software.amazon.awssdk.services.detective.model.CreateGraphRequest;
import software.amazon.awssdk.services.detective.model.CreateGraphResponse;
import software.amazon.awssdk.services.detective.model.CreateMembersRequest;
import software.amazon.awssdk.services.detective.model.CreateMembersResponse;
import software.amazon.awssdk.services.detective.model.DeleteGraphRequest;
import software.amazon.awssdk.services.detective.model.DeleteGraphResponse;
import software.amazon.awssdk.services.detective.model.DeleteMembersRequest;
import software.amazon.awssdk.services.detective.model.DeleteMembersResponse;
import software.amazon.awssdk.services.detective.model.DescribeOrganizationConfigurationRequest;
import software.amazon.awssdk.services.detective.model.DescribeOrganizationConfigurationResponse;
import software.amazon.awssdk.services.detective.model.DetectiveException;
import software.amazon.awssdk.services.detective.model.DetectiveRequest;
import software.amazon.awssdk.services.detective.model.DisableOrganizationAdminAccountRequest;
import software.amazon.awssdk.services.detective.model.DisableOrganizationAdminAccountResponse;
import software.amazon.awssdk.services.detective.model.DisassociateMembershipRequest;
import software.amazon.awssdk.services.detective.model.DisassociateMembershipResponse;
import software.amazon.awssdk.services.detective.model.EnableOrganizationAdminAccountRequest;
import software.amazon.awssdk.services.detective.model.EnableOrganizationAdminAccountResponse;
import software.amazon.awssdk.services.detective.model.GetMembersRequest;
import software.amazon.awssdk.services.detective.model.GetMembersResponse;
import software.amazon.awssdk.services.detective.model.InternalServerException;
import software.amazon.awssdk.services.detective.model.ListGraphsRequest;
import software.amazon.awssdk.services.detective.model.ListGraphsResponse;
import software.amazon.awssdk.services.detective.model.ListInvitationsRequest;
import software.amazon.awssdk.services.detective.model.ListInvitationsResponse;
import software.amazon.awssdk.services.detective.model.ListMembersRequest;
import software.amazon.awssdk.services.detective.model.ListMembersResponse;
import software.amazon.awssdk.services.detective.model.ListOrganizationAdminAccountsRequest;
import software.amazon.awssdk.services.detective.model.ListOrganizationAdminAccountsResponse;
import software.amazon.awssdk.services.detective.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.detective.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.detective.model.RejectInvitationRequest;
import software.amazon.awssdk.services.detective.model.RejectInvitationResponse;
import software.amazon.awssdk.services.detective.model.ResourceNotFoundException;
import software.amazon.awssdk.services.detective.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.detective.model.StartMonitoringMemberRequest;
import software.amazon.awssdk.services.detective.model.StartMonitoringMemberResponse;
import software.amazon.awssdk.services.detective.model.TagResourceRequest;
import software.amazon.awssdk.services.detective.model.TagResourceResponse;
import software.amazon.awssdk.services.detective.model.TooManyRequestsException;
import software.amazon.awssdk.services.detective.model.UntagResourceRequest;
import software.amazon.awssdk.services.detective.model.UntagResourceResponse;
import software.amazon.awssdk.services.detective.model.UpdateOrganizationConfigurationRequest;
import software.amazon.awssdk.services.detective.model.UpdateOrganizationConfigurationResponse;
import software.amazon.awssdk.services.detective.model.ValidationException;
import software.amazon.awssdk.services.detective.paginators.ListGraphsIterable;
import software.amazon.awssdk.services.detective.paginators.ListInvitationsIterable;
import software.amazon.awssdk.services.detective.paginators.ListMembersIterable;
import software.amazon.awssdk.services.detective.paginators.ListOrganizationAdminAccountsIterable;
import software.amazon.awssdk.services.detective.transform.AcceptInvitationRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.CreateGraphRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.CreateMembersRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.DeleteGraphRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.DeleteMembersRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.DescribeOrganizationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.DisableOrganizationAdminAccountRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.DisassociateMembershipRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.EnableOrganizationAdminAccountRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.GetMembersRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.ListGraphsRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.ListInvitationsRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.ListMembersRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.ListOrganizationAdminAccountsRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.RejectInvitationRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.StartMonitoringMemberRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.detective.transform.UpdateOrganizationConfigurationRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link DetectiveClient}.
*
* @see DetectiveClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultDetectiveClient implements DetectiveClient {
private static final Logger log = Logger.loggerFor(DefaultDetectiveClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultDetectiveClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Accepts an invitation for the member account to contribute data to a behavior graph. This operation can only be
* called by an invited member account.
*
*
* The request provides the ARN of behavior graph.
*
*
* The member account status in the graph must be INVITED
.
*
*
* @param acceptInvitationRequest
* @return Result of the AcceptInvitation operation returned by the service.
* @throws ConflictException
* The request attempted an invalid action.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.AcceptInvitation
* @see AWS
* API Documentation
*/
@Override
public AcceptInvitationResponse acceptInvitation(AcceptInvitationRequest acceptInvitationRequest) throws ConflictException,
InternalServerException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AcceptInvitationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, acceptInvitationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AcceptInvitation");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AcceptInvitation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(acceptInvitationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AcceptInvitationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new behavior graph for the calling account, and sets that account as the administrator account. This
* operation is called by the account that is enabling Detective.
*
*
* Before you try to enable Detective, make sure that your account has been enrolled in Amazon GuardDuty for at
* least 48 hours. If you do not meet this requirement, you cannot enable Detective. If you do meet the GuardDuty
* prerequisite, then when you make the request to enable Detective, it checks whether your data volume is within
* the Detective quota. If it exceeds the quota, then you cannot enable Detective.
*
*
* The operation also enables Detective for the calling account in the currently selected Region. It returns the ARN
* of the new behavior graph.
*
*
* CreateGraph
triggers a process to create the corresponding data tables for the new behavior graph.
*
*
* An account can only be the administrator account for one behavior graph within a Region. If the same account
* calls CreateGraph
with the same administrator account, it always returns the same behavior graph
* ARN. It does not create a new behavior graph.
*
*
* @param createGraphRequest
* @return Result of the CreateGraph operation returned by the service.
* @throws ConflictException
* The request attempted an invalid action.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ServiceQuotaExceededException
* This request cannot be completed for one of the following reasons.
*
* -
*
* The request would cause the number of member accounts in the behavior graph to exceed the maximum
* allowed. A behavior graph cannot have more than 1200 member accounts.
*
*
* -
*
* The request would cause the data rate for the behavior graph to exceed the maximum allowed.
*
*
* -
*
* Detective is unable to verify the data rate for the member account. This is usually because the member
* account is not enrolled in Amazon GuardDuty.
*
*
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.CreateGraph
* @see AWS API
* Documentation
*/
@Override
public CreateGraphResponse createGraph(CreateGraphRequest createGraphRequest) throws ConflictException,
InternalServerException, ServiceQuotaExceededException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateGraphResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createGraphRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateGraph");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateGraph").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createGraphRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateGraphRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* CreateMembers
is used to send invitations to accounts. For the organization behavior graph, the
* Detective administrator account uses CreateMembers
to enable organization accounts as member
* accounts.
*
*
* For invited accounts, CreateMembers
sends a request to invite the specified Amazon Web Services
* accounts to be member accounts in the behavior graph. This operation can only be called by the administrator
* account for a behavior graph.
*
*
* CreateMembers
verifies the accounts and then invites the verified accounts. The administrator can
* optionally specify to not send invitation emails to the member accounts. This would be used when the
* administrator manages their member accounts centrally.
*
*
* For organization accounts in the organization behavior graph, CreateMembers
attempts to enable the
* accounts. The organization accounts do not receive invitations.
*
*
* The request provides the behavior graph ARN and the list of accounts to invite or to enable.
*
*
* The response separates the requested accounts into two lists:
*
*
* -
*
* The accounts that CreateMembers
was able to process. For invited accounts, includes member accounts
* that are being verified, that have passed verification and are to be invited, and that have failed verification.
* For organization accounts in the organization behavior graph, includes accounts that can be enabled and that
* cannot be enabled.
*
*
* -
*
* The accounts that CreateMembers
was unable to process. This list includes accounts that were already
* invited to be member accounts in the behavior graph.
*
*
*
*
* @param createMembersRequest
* @return Result of the CreateMembers operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ValidationException
* The request parameters are invalid.
* @throws ServiceQuotaExceededException
* This request cannot be completed for one of the following reasons.
*
* -
*
* The request would cause the number of member accounts in the behavior graph to exceed the maximum
* allowed. A behavior graph cannot have more than 1200 member accounts.
*
*
* -
*
* The request would cause the data rate for the behavior graph to exceed the maximum allowed.
*
*
* -
*
* Detective is unable to verify the data rate for the member account. This is usually because the member
* account is not enrolled in Amazon GuardDuty.
*
*
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.CreateMembers
* @see AWS API
* Documentation
*/
@Override
public CreateMembersResponse createMembers(CreateMembersRequest createMembersRequest) throws InternalServerException,
ResourceNotFoundException, ValidationException, ServiceQuotaExceededException, AwsServiceException,
SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateMembersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createMembersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateMembers");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateMembers").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createMembersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateMembersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disables the specified behavior graph and queues it to be deleted. This operation removes the behavior graph from
* each member account's list of behavior graphs.
*
*
* DeleteGraph
can only be called by the administrator account for a behavior graph.
*
*
* @param deleteGraphRequest
* @return Result of the DeleteGraph operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.DeleteGraph
* @see AWS API
* Documentation
*/
@Override
public DeleteGraphResponse deleteGraph(DeleteGraphRequest deleteGraphRequest) throws InternalServerException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteGraphResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteGraphRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteGraph");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteGraph").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteGraphRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteGraphRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the specified member accounts from the behavior graph. The removed accounts no longer contribute data to
* the behavior graph. This operation can only be called by the administrator account for the behavior graph.
*
*
* For invited accounts, the removed accounts are deleted from the list of accounts in the behavior graph. To
* restore the account, the administrator account must send another invitation.
*
*
* For organization accounts in the organization behavior graph, the Detective administrator account can always
* enable the organization account again. Organization accounts that are not enabled as member accounts are not
* included in the ListMembers
results for the organization behavior graph.
*
*
* An administrator account cannot use DeleteMembers
to remove their own account from the behavior
* graph. To disable a behavior graph, the administrator account uses the DeleteGraph
API method.
*
*
* @param deleteMembersRequest
* @return Result of the DeleteMembers operation returned by the service.
* @throws ConflictException
* The request attempted an invalid action.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.DeleteMembers
* @see AWS API
* Documentation
*/
@Override
public DeleteMembersResponse deleteMembers(DeleteMembersRequest deleteMembersRequest) throws ConflictException,
InternalServerException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteMembersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteMembersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMembers");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteMembers").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteMembersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteMembersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about the configuration for the organization behavior graph. Currently indicates whether to
* automatically enable new organization accounts as member accounts.
*
*
* Can only be called by the Detective administrator account for the organization.
*
*
* @param describeOrganizationConfigurationRequest
* @return Result of the DescribeOrganizationConfiguration operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws TooManyRequestsException
* The request cannot be completed because too many other requests are occurring at the same time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.DescribeOrganizationConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeOrganizationConfigurationResponse describeOrganizationConfiguration(
DescribeOrganizationConfigurationRequest describeOrganizationConfigurationRequest) throws InternalServerException,
ValidationException, TooManyRequestsException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeOrganizationConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeOrganizationConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeOrganizationConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeOrganizationConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeOrganizationConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeOrganizationConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the Detective administrator account for the organization in the current Region. Deletes the behavior
* graph for that account.
*
*
* Can only be called by the organization management account. Before you can select a different Detective
* administrator account, you must remove the Detective administrator account in all Regions.
*
*
* @param disableOrganizationAdminAccountRequest
* @return Result of the DisableOrganizationAdminAccount operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws TooManyRequestsException
* The request cannot be completed because too many other requests are occurring at the same time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.DisableOrganizationAdminAccount
* @see AWS API Documentation
*/
@Override
public DisableOrganizationAdminAccountResponse disableOrganizationAdminAccount(
DisableOrganizationAdminAccountRequest disableOrganizationAdminAccountRequest) throws InternalServerException,
ValidationException, TooManyRequestsException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisableOrganizationAdminAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disableOrganizationAdminAccountRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisableOrganizationAdminAccount");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisableOrganizationAdminAccount").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disableOrganizationAdminAccountRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisableOrganizationAdminAccountRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the member account from the specified behavior graph. This operation can only be called by an invited
* member account that has the ENABLED
status.
*
*
* DisassociateMembership
cannot be called by an organization account in the organization behavior
* graph. For the organization behavior graph, the Detective administrator account determines which organization
* accounts to enable or disable as member accounts.
*
*
* @param disassociateMembershipRequest
* @return Result of the DisassociateMembership operation returned by the service.
* @throws ConflictException
* The request attempted an invalid action.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.DisassociateMembership
* @see AWS API Documentation
*/
@Override
public DisassociateMembershipResponse disassociateMembership(DisassociateMembershipRequest disassociateMembershipRequest)
throws ConflictException, InternalServerException, ResourceNotFoundException, ValidationException,
AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateMembershipResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateMembershipRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateMembership");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateMembership").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateMembershipRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateMembershipRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Designates the Detective administrator account for the organization in the current Region.
*
*
* If the account does not have Detective enabled, then enables Detective for that account and creates a new
* behavior graph.
*
*
* Can only be called by the organization management account.
*
*
* The Detective administrator account for an organization must be the same in all Regions. If you already
* designated a Detective administrator account in another Region, then you must designate the same account.
*
*
* @param enableOrganizationAdminAccountRequest
* @return Result of the EnableOrganizationAdminAccount operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws TooManyRequestsException
* The request cannot be completed because too many other requests are occurring at the same time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.EnableOrganizationAdminAccount
* @see AWS API Documentation
*/
@Override
public EnableOrganizationAdminAccountResponse enableOrganizationAdminAccount(
EnableOrganizationAdminAccountRequest enableOrganizationAdminAccountRequest) throws InternalServerException,
ValidationException, TooManyRequestsException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, EnableOrganizationAdminAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
enableOrganizationAdminAccountRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "EnableOrganizationAdminAccount");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("EnableOrganizationAdminAccount").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(enableOrganizationAdminAccountRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new EnableOrganizationAdminAccountRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the membership details for specified member accounts for a behavior graph.
*
*
* @param getMembersRequest
* @return Result of the GetMembers operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.GetMembers
* @see AWS API
* Documentation
*/
@Override
public GetMembersResponse getMembers(GetMembersRequest getMembersRequest) throws InternalServerException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetMembersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMembersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMembers");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("GetMembers")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getMembersRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetMembersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the list of behavior graphs that the calling account is an administrator account of. This operation can
* only be called by an administrator account.
*
*
* Because an account can currently only be the administrator of one behavior graph within a Region, the results
* always contain a single behavior graph.
*
*
* @param listGraphsRequest
* @return Result of the ListGraphs operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.ListGraphs
* @see AWS API
* Documentation
*/
@Override
public ListGraphsResponse listGraphs(ListGraphsRequest listGraphsRequest) throws InternalServerException,
ValidationException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListGraphsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listGraphsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListGraphs");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("ListGraphs")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listGraphsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListGraphsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the list of behavior graphs that the calling account is an administrator account of. This operation can
* only be called by an administrator account.
*
*
* Because an account can currently only be the administrator of one behavior graph within a Region, the results
* always contain a single behavior graph.
*
*
*
* This is a variant of {@link #listGraphs(software.amazon.awssdk.services.detective.model.ListGraphsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.detective.paginators.ListGraphsIterable responses = client.listGraphsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.detective.paginators.ListGraphsIterable responses = client.listGraphsPaginator(request);
* for (software.amazon.awssdk.services.detective.model.ListGraphsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.detective.paginators.ListGraphsIterable responses = client.listGraphsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGraphs(software.amazon.awssdk.services.detective.model.ListGraphsRequest)} operation.
*
*
* @param listGraphsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.ListGraphs
* @see AWS API
* Documentation
*/
@Override
public ListGraphsIterable listGraphsPaginator(ListGraphsRequest listGraphsRequest) throws InternalServerException,
ValidationException, AwsServiceException, SdkClientException, DetectiveException {
return new ListGraphsIterable(this, applyPaginatorUserAgent(listGraphsRequest));
}
/**
*
* Retrieves the list of open and accepted behavior graph invitations for the member account. This operation can
* only be called by an invited member account.
*
*
* Open invitations are invitations that the member account has not responded to.
*
*
* The results do not include behavior graphs for which the member account declined the invitation. The results also
* do not include behavior graphs that the member account resigned from or was removed from.
*
*
* @param listInvitationsRequest
* @return Result of the ListInvitations operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.ListInvitations
* @see AWS API
* Documentation
*/
@Override
public ListInvitationsResponse listInvitations(ListInvitationsRequest listInvitationsRequest) throws InternalServerException,
ValidationException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListInvitationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listInvitationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListInvitations");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListInvitations").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listInvitationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListInvitationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the list of open and accepted behavior graph invitations for the member account. This operation can
* only be called by an invited member account.
*
*
* Open invitations are invitations that the member account has not responded to.
*
*
* The results do not include behavior graphs for which the member account declined the invitation. The results also
* do not include behavior graphs that the member account resigned from or was removed from.
*
*
*
* This is a variant of
* {@link #listInvitations(software.amazon.awssdk.services.detective.model.ListInvitationsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.detective.paginators.ListInvitationsIterable responses = client.listInvitationsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.detective.paginators.ListInvitationsIterable responses = client
* .listInvitationsPaginator(request);
* for (software.amazon.awssdk.services.detective.model.ListInvitationsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.detective.paginators.ListInvitationsIterable responses = client.listInvitationsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInvitations(software.amazon.awssdk.services.detective.model.ListInvitationsRequest)} operation.
*
*
* @param listInvitationsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.ListInvitations
* @see AWS API
* Documentation
*/
@Override
public ListInvitationsIterable listInvitationsPaginator(ListInvitationsRequest listInvitationsRequest)
throws InternalServerException, ValidationException, AwsServiceException, SdkClientException, DetectiveException {
return new ListInvitationsIterable(this, applyPaginatorUserAgent(listInvitationsRequest));
}
/**
*
* Retrieves the list of member accounts for a behavior graph.
*
*
* For invited accounts, the results do not include member accounts that were removed from the behavior graph.
*
*
* For the organization behavior graph, the results do not include organization accounts that the Detective
* administrator account has not enabled as member accounts.
*
*
* @param listMembersRequest
* @return Result of the ListMembers operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.ListMembers
* @see AWS API
* Documentation
*/
@Override
public ListMembersResponse listMembers(ListMembersRequest listMembersRequest) throws InternalServerException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListMembersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMembersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMembers");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListMembers").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listMembersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListMembersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the list of member accounts for a behavior graph.
*
*
* For invited accounts, the results do not include member accounts that were removed from the behavior graph.
*
*
* For the organization behavior graph, the results do not include organization accounts that the Detective
* administrator account has not enabled as member accounts.
*
*
*
* This is a variant of {@link #listMembers(software.amazon.awssdk.services.detective.model.ListMembersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.detective.paginators.ListMembersIterable responses = client.listMembersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.detective.paginators.ListMembersIterable responses = client.listMembersPaginator(request);
* for (software.amazon.awssdk.services.detective.model.ListMembersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.detective.paginators.ListMembersIterable responses = client.listMembersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMembers(software.amazon.awssdk.services.detective.model.ListMembersRequest)} operation.
*
*
* @param listMembersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.ListMembers
* @see AWS API
* Documentation
*/
@Override
public ListMembersIterable listMembersPaginator(ListMembersRequest listMembersRequest) throws InternalServerException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, DetectiveException {
return new ListMembersIterable(this, applyPaginatorUserAgent(listMembersRequest));
}
/**
*
* Returns information about the Detective administrator account for an organization. Can only be called by the
* organization management account.
*
*
* @param listOrganizationAdminAccountsRequest
* @return Result of the ListOrganizationAdminAccounts operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws TooManyRequestsException
* The request cannot be completed because too many other requests are occurring at the same time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.ListOrganizationAdminAccounts
* @see AWS API Documentation
*/
@Override
public ListOrganizationAdminAccountsResponse listOrganizationAdminAccounts(
ListOrganizationAdminAccountsRequest listOrganizationAdminAccountsRequest) throws InternalServerException,
ValidationException, TooManyRequestsException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListOrganizationAdminAccountsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listOrganizationAdminAccountsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListOrganizationAdminAccounts");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListOrganizationAdminAccounts").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listOrganizationAdminAccountsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListOrganizationAdminAccountsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about the Detective administrator account for an organization. Can only be called by the
* organization management account.
*
*
*
* This is a variant of
* {@link #listOrganizationAdminAccounts(software.amazon.awssdk.services.detective.model.ListOrganizationAdminAccountsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.detective.paginators.ListOrganizationAdminAccountsIterable responses = client.listOrganizationAdminAccountsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.detective.paginators.ListOrganizationAdminAccountsIterable responses = client
* .listOrganizationAdminAccountsPaginator(request);
* for (software.amazon.awssdk.services.detective.model.ListOrganizationAdminAccountsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.detective.paginators.ListOrganizationAdminAccountsIterable responses = client.listOrganizationAdminAccountsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOrganizationAdminAccounts(software.amazon.awssdk.services.detective.model.ListOrganizationAdminAccountsRequest)}
* operation.
*
*
* @param listOrganizationAdminAccountsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws TooManyRequestsException
* The request cannot be completed because too many other requests are occurring at the same time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.ListOrganizationAdminAccounts
* @see AWS API Documentation
*/
@Override
public ListOrganizationAdminAccountsIterable listOrganizationAdminAccountsPaginator(
ListOrganizationAdminAccountsRequest listOrganizationAdminAccountsRequest) throws InternalServerException,
ValidationException, TooManyRequestsException, AwsServiceException, SdkClientException, DetectiveException {
return new ListOrganizationAdminAccountsIterable(this, applyPaginatorUserAgent(listOrganizationAdminAccountsRequest));
}
/**
*
* Returns the tag values that are assigned to a behavior graph.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InternalServerException, ValidationException, ResourceNotFoundException, AwsServiceException,
SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Rejects an invitation to contribute the account data to a behavior graph. This operation must be called by an
* invited member account that has the INVITED
status.
*
*
* RejectInvitation
cannot be called by an organization account in the organization behavior graph. In
* the organization behavior graph, organization accounts do not receive an invitation.
*
*
* @param rejectInvitationRequest
* @return Result of the RejectInvitation operation returned by the service.
* @throws ConflictException
* The request attempted an invalid action.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.RejectInvitation
* @see AWS
* API Documentation
*/
@Override
public RejectInvitationResponse rejectInvitation(RejectInvitationRequest rejectInvitationRequest) throws ConflictException,
InternalServerException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RejectInvitationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, rejectInvitationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RejectInvitation");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RejectInvitation").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(rejectInvitationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RejectInvitationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Sends a request to enable data ingest for a member account that has a status of
* ACCEPTED_BUT_DISABLED
.
*
*
* For valid member accounts, the status is updated as follows.
*
*
* -
*
* If Detective enabled the member account, then the new status is ENABLED
.
*
*
* -
*
* If Detective cannot enable the member account, the status remains ACCEPTED_BUT_DISABLED
.
*
*
*
*
* @param startMonitoringMemberRequest
* @return Result of the StartMonitoringMember operation returned by the service.
* @throws ConflictException
* The request attempted an invalid action.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws ServiceQuotaExceededException
* This request cannot be completed for one of the following reasons.
*
* -
*
* The request would cause the number of member accounts in the behavior graph to exceed the maximum
* allowed. A behavior graph cannot have more than 1200 member accounts.
*
*
* -
*
* The request would cause the data rate for the behavior graph to exceed the maximum allowed.
*
*
* -
*
* Detective is unable to verify the data rate for the member account. This is usually because the member
* account is not enrolled in Amazon GuardDuty.
*
*
* @throws ValidationException
* The request parameters are invalid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.StartMonitoringMember
* @see AWS API Documentation
*/
@Override
public StartMonitoringMemberResponse startMonitoringMember(StartMonitoringMemberRequest startMonitoringMemberRequest)
throws ConflictException, InternalServerException, ResourceNotFoundException, ServiceQuotaExceededException,
ValidationException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartMonitoringMemberResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startMonitoringMemberRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartMonitoringMember");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StartMonitoringMember").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startMonitoringMemberRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartMonitoringMemberRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Applies tag values to a behavior graph.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InternalServerException,
ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes tags from a behavior graph.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws ResourceNotFoundException
* The request refers to a nonexistent resource.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InternalServerException,
ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the configuration for the Organizations integration in the current Region. Can only be called by the
* Detective administrator account for the organization.
*
*
* @param updateOrganizationConfigurationRequest
* @return Result of the UpdateOrganizationConfiguration operation returned by the service.
* @throws InternalServerException
* The request was valid but failed because of a problem with the service.
* @throws ValidationException
* The request parameters are invalid.
* @throws TooManyRequestsException
* The request cannot be completed because too many other requests are occurring at the same time.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DetectiveException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DetectiveClient.UpdateOrganizationConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateOrganizationConfigurationResponse updateOrganizationConfiguration(
UpdateOrganizationConfigurationRequest updateOrganizationConfigurationRequest) throws InternalServerException,
ValidationException, TooManyRequestsException, AwsServiceException, SdkClientException, DetectiveException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateOrganizationConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
updateOrganizationConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Detective");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateOrganizationConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateOrganizationConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateOrganizationConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateOrganizationConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(DetectiveException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRequestsException")
.exceptionBuilderSupplier(TooManyRequestsException::builder).httpStatusCode(429).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
}