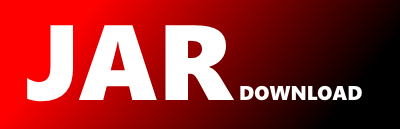
software.amazon.awssdk.services.detective.model.MemberDetail Maven / Gradle / Ivy
Show all versions of detective Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.detective.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details about a member account in a behavior graph.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MemberDetail implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccountId").getter(getter(MemberDetail::accountId)).setter(setter(Builder::accountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountId").build()).build();
private static final SdkField EMAIL_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EmailAddress").getter(getter(MemberDetail::emailAddress)).setter(setter(Builder::emailAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EmailAddress").build()).build();
private static final SdkField GRAPH_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GraphArn").getter(getter(MemberDetail::graphArn)).setter(setter(Builder::graphArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GraphArn").build()).build();
private static final SdkField MASTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterId").getter(getter(MemberDetail::masterId)).setter(setter(Builder::masterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterId").build()).build();
private static final SdkField ADMINISTRATOR_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdministratorId").getter(getter(MemberDetail::administratorId)).setter(setter(Builder::administratorId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdministratorId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(MemberDetail::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField DISABLED_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DisabledReason").getter(getter(MemberDetail::disabledReasonAsString))
.setter(setter(Builder::disabledReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DisabledReason").build()).build();
private static final SdkField INVITED_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("InvitedTime")
.getter(getter(MemberDetail::invitedTime))
.setter(setter(Builder::invitedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InvitedTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField UPDATED_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("UpdatedTime")
.getter(getter(MemberDetail::updatedTime))
.setter(setter(Builder::updatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdatedTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField VOLUME_USAGE_IN_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("VolumeUsageInBytes").getter(getter(MemberDetail::volumeUsageInBytes))
.setter(setter(Builder::volumeUsageInBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VolumeUsageInBytes").build())
.build();
private static final SdkField VOLUME_USAGE_UPDATED_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("VolumeUsageUpdatedTime")
.getter(getter(MemberDetail::volumeUsageUpdatedTime))
.setter(setter(Builder::volumeUsageUpdatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VolumeUsageUpdatedTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField PERCENT_OF_GRAPH_UTILIZATION_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("PercentOfGraphUtilization").getter(getter(MemberDetail::percentOfGraphUtilization))
.setter(setter(Builder::percentOfGraphUtilization))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PercentOfGraphUtilization").build())
.build();
private static final SdkField PERCENT_OF_GRAPH_UTILIZATION_UPDATED_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("PercentOfGraphUtilizationUpdatedTime")
.getter(getter(MemberDetail::percentOfGraphUtilizationUpdatedTime))
.setter(setter(Builder::percentOfGraphUtilizationUpdatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("PercentOfGraphUtilizationUpdatedTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField INVITATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InvitationType").getter(getter(MemberDetail::invitationTypeAsString))
.setter(setter(Builder::invitationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InvitationType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCOUNT_ID_FIELD,
EMAIL_ADDRESS_FIELD, GRAPH_ARN_FIELD, MASTER_ID_FIELD, ADMINISTRATOR_ID_FIELD, STATUS_FIELD, DISABLED_REASON_FIELD,
INVITED_TIME_FIELD, UPDATED_TIME_FIELD, VOLUME_USAGE_IN_BYTES_FIELD, VOLUME_USAGE_UPDATED_TIME_FIELD,
PERCENT_OF_GRAPH_UTILIZATION_FIELD, PERCENT_OF_GRAPH_UTILIZATION_UPDATED_TIME_FIELD, INVITATION_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String accountId;
private final String emailAddress;
private final String graphArn;
private final String masterId;
private final String administratorId;
private final String status;
private final String disabledReason;
private final Instant invitedTime;
private final Instant updatedTime;
private final Long volumeUsageInBytes;
private final Instant volumeUsageUpdatedTime;
private final Double percentOfGraphUtilization;
private final Instant percentOfGraphUtilizationUpdatedTime;
private final String invitationType;
private MemberDetail(BuilderImpl builder) {
this.accountId = builder.accountId;
this.emailAddress = builder.emailAddress;
this.graphArn = builder.graphArn;
this.masterId = builder.masterId;
this.administratorId = builder.administratorId;
this.status = builder.status;
this.disabledReason = builder.disabledReason;
this.invitedTime = builder.invitedTime;
this.updatedTime = builder.updatedTime;
this.volumeUsageInBytes = builder.volumeUsageInBytes;
this.volumeUsageUpdatedTime = builder.volumeUsageUpdatedTime;
this.percentOfGraphUtilization = builder.percentOfGraphUtilization;
this.percentOfGraphUtilizationUpdatedTime = builder.percentOfGraphUtilizationUpdatedTime;
this.invitationType = builder.invitationType;
}
/**
*
* The Amazon Web Services account identifier for the member account.
*
*
* @return The Amazon Web Services account identifier for the member account.
*/
public final String accountId() {
return accountId;
}
/**
*
* The Amazon Web Services account root user email address for the member account.
*
*
* @return The Amazon Web Services account root user email address for the member account.
*/
public final String emailAddress() {
return emailAddress;
}
/**
*
* The ARN of the behavior graph.
*
*
* @return The ARN of the behavior graph.
*/
public final String graphArn() {
return graphArn;
}
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*
* @return The Amazon Web Services account identifier of the administrator account for the behavior graph.
* @deprecated This property is deprecated. Use AdministratorId instead.
*/
@Deprecated
public final String masterId() {
return masterId;
}
/**
*
* The Amazon Web Services account identifier of the administrator account for the behavior graph.
*
*
* @return The Amazon Web Services account identifier of the administrator account for the behavior graph.
*/
public final String administratorId() {
return administratorId;
}
/**
*
* The current membership status of the member account. The status can have one of the following values:
*
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has not
* yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying that the
* account identifier and email address provided for the member account match. If they do match, then Detective
* sends the invitation. If the email address and account identifier don't match, then the member cannot be added to
* the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that the
* account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph. For
* invited accounts, the member account accepted the invitation. For organization accounts in the organization
* behavior graph, the Detective administrator account enabled the organization account as a member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph. DisabledReason
* provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not included. In
* the organization behavior graph, organization accounts that the Detective administrator account did not enable
* are not included.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link MemberStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current membership status of the member account. The status can have one of the following values:
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but
* has not yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying
* that the account identifier and email address provided for the member account match. If they do match,
* then Detective sends the invitation. If the email address and account identifier don't match, then the
* member cannot be added to the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that
* the account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email
* address provided for the member account do not match, and Detective did not send an invitation to the
* account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior
* graph. For invited accounts, the member account accepted the invitation. For organization accounts in the
* organization behavior graph, the Detective administrator account enabled the organization account as a
* member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph.
* DisabledReason
provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not
* included. In the organization behavior graph, organization accounts that the Detective administrator
* account did not enable are not included.
* @see MemberStatus
*/
public final MemberStatus status() {
return MemberStatus.fromValue(status);
}
/**
*
* The current membership status of the member account. The status can have one of the following values:
*
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has not
* yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying that the
* account identifier and email address provided for the member account match. If they do match, then Detective
* sends the invitation. If the email address and account identifier don't match, then the member cannot be added to
* the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that the
* account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph. For
* invited accounts, the member account accepted the invitation. For organization accounts in the organization
* behavior graph, the Detective administrator account enabled the organization account as a member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph. DisabledReason
* provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not included. In
* the organization behavior graph, organization accounts that the Detective administrator account did not enable
* are not included.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link MemberStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current membership status of the member account. The status can have one of the following values:
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but
* has not yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying
* that the account identifier and email address provided for the member account match. If they do match,
* then Detective sends the invitation. If the email address and account identifier don't match, then the
* member cannot be added to the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that
* the account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email
* address provided for the member account do not match, and Detective did not send an invitation to the
* account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior
* graph. For invited accounts, the member account accepted the invitation. For organization accounts in the
* organization behavior graph, the Detective administrator account enabled the organization account as a
* member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph.
* DisabledReason
provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not
* included. In the organization behavior graph, organization accounts that the Detective administrator
* account did not enable are not included.
* @see MemberStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member account is
* not enabled.
*
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for the
* behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #disabledReason}
* will return {@link MemberDisabledReason#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #disabledReasonAsString}.
*
*
* @return For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member
* account is not enabled.
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for
* the behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
* @see MemberDisabledReason
*/
public final MemberDisabledReason disabledReason() {
return MemberDisabledReason.fromValue(disabledReason);
}
/**
*
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member account is
* not enabled.
*
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for the
* behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #disabledReason}
* will return {@link MemberDisabledReason#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #disabledReasonAsString}.
*
*
* @return For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member
* account is not enabled.
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for
* the behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
* @see MemberDisabledReason
*/
public final String disabledReasonAsString() {
return disabledReason;
}
/**
*
* For invited accounts, the date and time that Detective sent the invitation to the account. The value is an
* ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @return For invited accounts, the date and time that Detective sent the invitation to the account. The value is
* an ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
*/
public final Instant invitedTime() {
return invitedTime;
}
/**
*
* The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
*
*
* @return The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
*/
public final Instant updatedTime() {
return updatedTime;
}
/**
*
* The data volume in bytes per day for the member account.
*
*
* @return The data volume in bytes per day for the member account.
*/
public final Long volumeUsageInBytes() {
return volumeUsageInBytes;
}
/**
*
* The data and time when the member account data volume was last updated. The value is an ISO8601 formatted string.
* For example, 2021-08-18T16:35:56.284Z
.
*
*
* @return The data and time when the member account data volume was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*/
public final Instant volumeUsageUpdatedTime() {
return volumeUsageUpdatedTime;
}
/**
*
* The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent, and 100
* indicates 100 percent.
*
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB per day.
* If the data volume for the member account is 40 GB per day, then PercentOfGraphUtilization
is 25. It
* represents 25% of the maximum allowed data volume.
*
*
* @return The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent,
* and 100 indicates 100 percent.
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB
* per day. If the data volume for the member account is 40 GB per day, then
* PercentOfGraphUtilization
is 25. It represents 25% of the maximum allowed data volume.
* @deprecated This property is deprecated. Use VolumeUsageInBytes instead.
*/
@Deprecated
public final Double percentOfGraphUtilization() {
return percentOfGraphUtilization;
}
/**
*
* The date and time when the graph utilization percentage was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @return The date and time when the graph utilization percentage was last updated. The value is an ISO8601
* formatted string. For example, 2021-08-18T16:35:56.284Z
.
* @deprecated This property is deprecated. Use VolumeUsageUpdatedTime instead.
*/
@Deprecated
public final Instant percentOfGraphUtilizationUpdatedTime() {
return percentOfGraphUtilizationUpdatedTime;
}
/**
*
* The type of behavior graph membership.
*
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #invitationType}
* will return {@link InvitationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #invitationTypeAsString}.
*
*
* @return The type of behavior graph membership.
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
* @see InvitationType
*/
public final InvitationType invitationType() {
return InvitationType.fromValue(invitationType);
}
/**
*
* The type of behavior graph membership.
*
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #invitationType}
* will return {@link InvitationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #invitationTypeAsString}.
*
*
* @return The type of behavior graph membership.
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
* @see InvitationType
*/
public final String invitationTypeAsString() {
return invitationType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(accountId());
hashCode = 31 * hashCode + Objects.hashCode(emailAddress());
hashCode = 31 * hashCode + Objects.hashCode(graphArn());
hashCode = 31 * hashCode + Objects.hashCode(masterId());
hashCode = 31 * hashCode + Objects.hashCode(administratorId());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(disabledReasonAsString());
hashCode = 31 * hashCode + Objects.hashCode(invitedTime());
hashCode = 31 * hashCode + Objects.hashCode(updatedTime());
hashCode = 31 * hashCode + Objects.hashCode(volumeUsageInBytes());
hashCode = 31 * hashCode + Objects.hashCode(volumeUsageUpdatedTime());
hashCode = 31 * hashCode + Objects.hashCode(percentOfGraphUtilization());
hashCode = 31 * hashCode + Objects.hashCode(percentOfGraphUtilizationUpdatedTime());
hashCode = 31 * hashCode + Objects.hashCode(invitationTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MemberDetail)) {
return false;
}
MemberDetail other = (MemberDetail) obj;
return Objects.equals(accountId(), other.accountId()) && Objects.equals(emailAddress(), other.emailAddress())
&& Objects.equals(graphArn(), other.graphArn()) && Objects.equals(masterId(), other.masterId())
&& Objects.equals(administratorId(), other.administratorId())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(disabledReasonAsString(), other.disabledReasonAsString())
&& Objects.equals(invitedTime(), other.invitedTime()) && Objects.equals(updatedTime(), other.updatedTime())
&& Objects.equals(volumeUsageInBytes(), other.volumeUsageInBytes())
&& Objects.equals(volumeUsageUpdatedTime(), other.volumeUsageUpdatedTime())
&& Objects.equals(percentOfGraphUtilization(), other.percentOfGraphUtilization())
&& Objects.equals(percentOfGraphUtilizationUpdatedTime(), other.percentOfGraphUtilizationUpdatedTime())
&& Objects.equals(invitationTypeAsString(), other.invitationTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MemberDetail").add("AccountId", accountId()).add("EmailAddress", emailAddress())
.add("GraphArn", graphArn()).add("MasterId", masterId()).add("AdministratorId", administratorId())
.add("Status", statusAsString()).add("DisabledReason", disabledReasonAsString())
.add("InvitedTime", invitedTime()).add("UpdatedTime", updatedTime())
.add("VolumeUsageInBytes", volumeUsageInBytes()).add("VolumeUsageUpdatedTime", volumeUsageUpdatedTime())
.add("PercentOfGraphUtilization", percentOfGraphUtilization())
.add("PercentOfGraphUtilizationUpdatedTime", percentOfGraphUtilizationUpdatedTime())
.add("InvitationType", invitationTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AccountId":
return Optional.ofNullable(clazz.cast(accountId()));
case "EmailAddress":
return Optional.ofNullable(clazz.cast(emailAddress()));
case "GraphArn":
return Optional.ofNullable(clazz.cast(graphArn()));
case "MasterId":
return Optional.ofNullable(clazz.cast(masterId()));
case "AdministratorId":
return Optional.ofNullable(clazz.cast(administratorId()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "DisabledReason":
return Optional.ofNullable(clazz.cast(disabledReasonAsString()));
case "InvitedTime":
return Optional.ofNullable(clazz.cast(invitedTime()));
case "UpdatedTime":
return Optional.ofNullable(clazz.cast(updatedTime()));
case "VolumeUsageInBytes":
return Optional.ofNullable(clazz.cast(volumeUsageInBytes()));
case "VolumeUsageUpdatedTime":
return Optional.ofNullable(clazz.cast(volumeUsageUpdatedTime()));
case "PercentOfGraphUtilization":
return Optional.ofNullable(clazz.cast(percentOfGraphUtilization()));
case "PercentOfGraphUtilizationUpdatedTime":
return Optional.ofNullable(clazz.cast(percentOfGraphUtilizationUpdatedTime()));
case "InvitationType":
return Optional.ofNullable(clazz.cast(invitationTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but
* has not yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is
* verifying that the account identifier and email address provided for the member account match. If they
* do match, then Detective sends the invitation. If the email address and account identifier don't
* match, then the member cannot be added to the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying
* that the account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email
* address provided for the member account do not match, and Detective did not send an invitation to the
* account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior
* graph. For invited accounts, the member account accepted the invitation. For organization accounts in
* the organization behavior graph, the Detective administrator account enabled the organization account
* as a member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the
* Detective administrator account, but is prevented from contributing data to the behavior graph.
* DisabledReason
provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not
* included. In the organization behavior graph, organization accounts that the Detective administrator
* account did not enable are not included.
* @see MemberStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see MemberStatus
*/
Builder status(String status);
/**
*
* The current membership status of the member account. The status can have one of the following values:
*
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but has
* not yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is verifying that
* the account identifier and email address provided for the member account match. If they do match, then
* Detective sends the invitation. If the email address and account identifier don't match, then the member
* cannot be added to the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying that the
* account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email address
* provided for the member account do not match, and Detective did not send an invitation to the account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior graph.
* For invited accounts, the member account accepted the invitation. For organization accounts in the
* organization behavior graph, the Detective administrator account enabled the organization account as a member
* account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the Detective
* administrator account, but is prevented from contributing data to the behavior graph.
* DisabledReason
provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not included.
* In the organization behavior graph, organization accounts that the Detective administrator account did not
* enable are not included.
*
*
* @param status
* The current membership status of the member account. The status can have one of the following
* values:
*
* -
*
* INVITED
- For invited accounts only. Indicates that the member was sent an invitation but
* has not yet responded.
*
*
* -
*
* VERIFICATION_IN_PROGRESS
- For invited accounts only, indicates that Detective is
* verifying that the account identifier and email address provided for the member account match. If they
* do match, then Detective sends the invitation. If the email address and account identifier don't
* match, then the member cannot be added to the behavior graph.
*
*
* For organization accounts in the organization behavior graph, indicates that Detective is verifying
* that the account belongs to the organization.
*
*
* -
*
* VERIFICATION_FAILED
- For invited accounts only. Indicates that the account and email
* address provided for the member account do not match, and Detective did not send an invitation to the
* account.
*
*
* -
*
* ENABLED
- Indicates that the member account currently contributes data to the behavior
* graph. For invited accounts, the member account accepted the invitation. For organization accounts in
* the organization behavior graph, the Detective administrator account enabled the organization account
* as a member account.
*
*
* -
*
* ACCEPTED_BUT_DISABLED
- The account accepted the invitation, or was enabled by the
* Detective administrator account, but is prevented from contributing data to the behavior graph.
* DisabledReason
provides the reason why the member account is not enabled.
*
*
*
*
* Invited accounts that declined an invitation or that were removed from the behavior graph are not
* included. In the organization behavior graph, organization accounts that the Detective administrator
* account did not enable are not included.
* @see MemberStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see MemberStatus
*/
Builder status(MemberStatus status);
/**
*
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member account
* is not enabled.
*
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for the
* behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
*
*
* @param disabledReason
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member
* account is not enabled.
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume
* for the behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the
* member account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
* @see MemberDisabledReason
* @return Returns a reference to this object so that method calls can be chained together.
* @see MemberDisabledReason
*/
Builder disabledReason(String disabledReason);
/**
*
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member account
* is not enabled.
*
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume for the
* behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the member
* account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
*
*
* @param disabledReason
* For member accounts with a status of ACCEPTED_BUT_DISABLED
, the reason that the member
* account is not enabled.
*
* The reason can have one of the following values:
*
*
* -
*
* VOLUME_TOO_HIGH
- Indicates that adding the member account would cause the data volume
* for the behavior graph to be too high.
*
*
* -
*
* VOLUME_UNKNOWN
- Indicates that Detective is unable to verify the data volume for the
* member account. This is usually because the member account is not enrolled in Amazon GuardDuty.
*
*
* @see MemberDisabledReason
* @return Returns a reference to this object so that method calls can be chained together.
* @see MemberDisabledReason
*/
Builder disabledReason(MemberDisabledReason disabledReason);
/**
*
* For invited accounts, the date and time that Detective sent the invitation to the account. The value is an
* ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @param invitedTime
* For invited accounts, the date and time that Detective sent the invitation to the account. The value
* is an ISO8601 formatted string. For example, 2021-08-18T16:35:56.284Z
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder invitedTime(Instant invitedTime);
/**
*
* The date and time that the member account was last updated. The value is an ISO8601 formatted string. For
* example, 2021-08-18T16:35:56.284Z
.
*
*
* @param updatedTime
* The date and time that the member account was last updated. The value is an ISO8601 formatted string.
* For example, 2021-08-18T16:35:56.284Z
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder updatedTime(Instant updatedTime);
/**
*
* The data volume in bytes per day for the member account.
*
*
* @param volumeUsageInBytes
* The data volume in bytes per day for the member account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder volumeUsageInBytes(Long volumeUsageInBytes);
/**
*
* The data and time when the member account data volume was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @param volumeUsageUpdatedTime
* The data and time when the member account data volume was last updated. The value is an ISO8601
* formatted string. For example, 2021-08-18T16:35:56.284Z
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder volumeUsageUpdatedTime(Instant volumeUsageUpdatedTime);
/**
*
* The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0 percent, and
* 100 indicates 100 percent.
*
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160 GB per
* day. If the data volume for the member account is 40 GB per day, then PercentOfGraphUtilization
* is 25. It represents 25% of the maximum allowed data volume.
*
*
* @param percentOfGraphUtilization
* The member account data volume as a percentage of the maximum allowed data volume. 0 indicates 0
* percent, and 100 indicates 100 percent.
*
* Note that this is not the percentage of the behavior graph data volume.
*
*
* For example, the data volume for the behavior graph is 80 GB per day. The maximum data volume is 160
* GB per day. If the data volume for the member account is 40 GB per day, then
* PercentOfGraphUtilization
is 25. It represents 25% of the maximum allowed data volume.
* @return Returns a reference to this object so that method calls can be chained together.
* @deprecated This property is deprecated. Use VolumeUsageInBytes instead.
*/
@Deprecated
Builder percentOfGraphUtilization(Double percentOfGraphUtilization);
/**
*
* The date and time when the graph utilization percentage was last updated. The value is an ISO8601 formatted
* string. For example, 2021-08-18T16:35:56.284Z
.
*
*
* @param percentOfGraphUtilizationUpdatedTime
* The date and time when the graph utilization percentage was last updated. The value is an ISO8601
* formatted string. For example, 2021-08-18T16:35:56.284Z
.
* @return Returns a reference to this object so that method calls can be chained together.
* @deprecated This property is deprecated. Use VolumeUsageUpdatedTime instead.
*/
@Deprecated
Builder percentOfGraphUtilizationUpdatedTime(Instant percentOfGraphUtilizationUpdatedTime);
/**
*
* The type of behavior graph membership.
*
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
*
*
* @param invitationType
* The type of behavior graph membership.
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
* @see InvitationType
* @return Returns a reference to this object so that method calls can be chained together.
* @see InvitationType
*/
Builder invitationType(String invitationType);
/**
*
* The type of behavior graph membership.
*
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
*
*
* @param invitationType
* The type of behavior graph membership.
*
* For an organization account in the organization behavior graph, the type is ORGANIZATION
.
*
*
* For an account that was invited to a behavior graph, the type is INVITATION
.
* @see InvitationType
* @return Returns a reference to this object so that method calls can be chained together.
* @see InvitationType
*/
Builder invitationType(InvitationType invitationType);
}
static final class BuilderImpl implements Builder {
private String accountId;
private String emailAddress;
private String graphArn;
private String masterId;
private String administratorId;
private String status;
private String disabledReason;
private Instant invitedTime;
private Instant updatedTime;
private Long volumeUsageInBytes;
private Instant volumeUsageUpdatedTime;
private Double percentOfGraphUtilization;
private Instant percentOfGraphUtilizationUpdatedTime;
private String invitationType;
private BuilderImpl() {
}
private BuilderImpl(MemberDetail model) {
accountId(model.accountId);
emailAddress(model.emailAddress);
graphArn(model.graphArn);
masterId(model.masterId);
administratorId(model.administratorId);
status(model.status);
disabledReason(model.disabledReason);
invitedTime(model.invitedTime);
updatedTime(model.updatedTime);
volumeUsageInBytes(model.volumeUsageInBytes);
volumeUsageUpdatedTime(model.volumeUsageUpdatedTime);
percentOfGraphUtilization(model.percentOfGraphUtilization);
percentOfGraphUtilizationUpdatedTime(model.percentOfGraphUtilizationUpdatedTime);
invitationType(model.invitationType);
}
public final String getAccountId() {
return accountId;
}
public final void setAccountId(String accountId) {
this.accountId = accountId;
}
@Override
public final Builder accountId(String accountId) {
this.accountId = accountId;
return this;
}
public final String getEmailAddress() {
return emailAddress;
}
public final void setEmailAddress(String emailAddress) {
this.emailAddress = emailAddress;
}
@Override
public final Builder emailAddress(String emailAddress) {
this.emailAddress = emailAddress;
return this;
}
public final String getGraphArn() {
return graphArn;
}
public final void setGraphArn(String graphArn) {
this.graphArn = graphArn;
}
@Override
public final Builder graphArn(String graphArn) {
this.graphArn = graphArn;
return this;
}
@Deprecated
public final String getMasterId() {
return masterId;
}
@Deprecated
public final void setMasterId(String masterId) {
this.masterId = masterId;
}
@Override
@Deprecated
public final Builder masterId(String masterId) {
this.masterId = masterId;
return this;
}
public final String getAdministratorId() {
return administratorId;
}
public final void setAdministratorId(String administratorId) {
this.administratorId = administratorId;
}
@Override
public final Builder administratorId(String administratorId) {
this.administratorId = administratorId;
return this;
}
public final String getStatus() {
return status;
}
public final void setStatus(String status) {
this.status = status;
}
@Override
public final Builder status(String status) {
this.status = status;
return this;
}
@Override
public final Builder status(MemberStatus status) {
this.status(status == null ? null : status.toString());
return this;
}
public final String getDisabledReason() {
return disabledReason;
}
public final void setDisabledReason(String disabledReason) {
this.disabledReason = disabledReason;
}
@Override
public final Builder disabledReason(String disabledReason) {
this.disabledReason = disabledReason;
return this;
}
@Override
public final Builder disabledReason(MemberDisabledReason disabledReason) {
this.disabledReason(disabledReason == null ? null : disabledReason.toString());
return this;
}
public final Instant getInvitedTime() {
return invitedTime;
}
public final void setInvitedTime(Instant invitedTime) {
this.invitedTime = invitedTime;
}
@Override
public final Builder invitedTime(Instant invitedTime) {
this.invitedTime = invitedTime;
return this;
}
public final Instant getUpdatedTime() {
return updatedTime;
}
public final void setUpdatedTime(Instant updatedTime) {
this.updatedTime = updatedTime;
}
@Override
public final Builder updatedTime(Instant updatedTime) {
this.updatedTime = updatedTime;
return this;
}
public final Long getVolumeUsageInBytes() {
return volumeUsageInBytes;
}
public final void setVolumeUsageInBytes(Long volumeUsageInBytes) {
this.volumeUsageInBytes = volumeUsageInBytes;
}
@Override
public final Builder volumeUsageInBytes(Long volumeUsageInBytes) {
this.volumeUsageInBytes = volumeUsageInBytes;
return this;
}
public final Instant getVolumeUsageUpdatedTime() {
return volumeUsageUpdatedTime;
}
public final void setVolumeUsageUpdatedTime(Instant volumeUsageUpdatedTime) {
this.volumeUsageUpdatedTime = volumeUsageUpdatedTime;
}
@Override
public final Builder volumeUsageUpdatedTime(Instant volumeUsageUpdatedTime) {
this.volumeUsageUpdatedTime = volumeUsageUpdatedTime;
return this;
}
@Deprecated
public final Double getPercentOfGraphUtilization() {
return percentOfGraphUtilization;
}
@Deprecated
public final void setPercentOfGraphUtilization(Double percentOfGraphUtilization) {
this.percentOfGraphUtilization = percentOfGraphUtilization;
}
@Override
@Deprecated
public final Builder percentOfGraphUtilization(Double percentOfGraphUtilization) {
this.percentOfGraphUtilization = percentOfGraphUtilization;
return this;
}
@Deprecated
public final Instant getPercentOfGraphUtilizationUpdatedTime() {
return percentOfGraphUtilizationUpdatedTime;
}
@Deprecated
public final void setPercentOfGraphUtilizationUpdatedTime(Instant percentOfGraphUtilizationUpdatedTime) {
this.percentOfGraphUtilizationUpdatedTime = percentOfGraphUtilizationUpdatedTime;
}
@Override
@Deprecated
public final Builder percentOfGraphUtilizationUpdatedTime(Instant percentOfGraphUtilizationUpdatedTime) {
this.percentOfGraphUtilizationUpdatedTime = percentOfGraphUtilizationUpdatedTime;
return this;
}
public final String getInvitationType() {
return invitationType;
}
public final void setInvitationType(String invitationType) {
this.invitationType = invitationType;
}
@Override
public final Builder invitationType(String invitationType) {
this.invitationType = invitationType;
return this;
}
@Override
public final Builder invitationType(InvitationType invitationType) {
this.invitationType(invitationType == null ? null : invitationType.toString());
return this;
}
@Override
public MemberDetail build() {
return new MemberDetail(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}