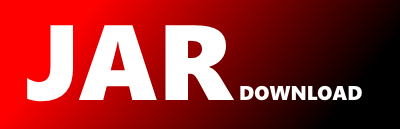
software.amazon.awssdk.services.devicefarm.model.Test Maven / Gradle / Ivy
Show all versions of devicefarm Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a condition that is evaluated.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Test implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).getter(getter(Test::arn))
.setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Test::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Test::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(Test::created)).setter(setter(Builder::created))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("created").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Test::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField RESULT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Test::resultAsString)).setter(setter(Builder::result))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("result").build()).build();
private static final SdkField STARTED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(Test::started)).setter(setter(Builder::started))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("started").build()).build();
private static final SdkField STOPPED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(Test::stopped)).setter(setter(Builder::stopped))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stopped").build()).build();
private static final SdkField COUNTERS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(Test::counters)).setter(setter(Builder::counters)).constructor(Counters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("counters").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Test::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("message").build()).build();
private static final SdkField DEVICE_MINUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Test::deviceMinutes))
.setter(setter(Builder::deviceMinutes)).constructor(DeviceMinutes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceMinutes").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, NAME_FIELD,
TYPE_FIELD, CREATED_FIELD, STATUS_FIELD, RESULT_FIELD, STARTED_FIELD, STOPPED_FIELD, COUNTERS_FIELD, MESSAGE_FIELD,
DEVICE_MINUTES_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String name;
private final String type;
private final Instant created;
private final String status;
private final String result;
private final Instant started;
private final Instant stopped;
private final Counters counters;
private final String message;
private final DeviceMinutes deviceMinutes;
private Test(BuilderImpl builder) {
this.arn = builder.arn;
this.name = builder.name;
this.type = builder.type;
this.created = builder.created;
this.status = builder.status;
this.result = builder.result;
this.started = builder.started;
this.stopped = builder.stopped;
this.counters = builder.counters;
this.message = builder.message;
this.deviceMinutes = builder.deviceMinutes;
}
/**
*
* The test's ARN.
*
*
* @return The test's ARN.
*/
public String arn() {
return arn;
}
/**
*
* The test's name.
*
*
* @return The test's name.
*/
public String name() {
return name;
}
/**
*
* The test's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* For Android, an app explorer that traverses an Android app, interacting with it and capturing screenshots at the
* same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link TestType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The test's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* For Android, an app explorer that traverses an Android app, interacting with it and capturing screenshots
* at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @see TestType
*/
public TestType type() {
return TestType.fromValue(type);
}
/**
*
* The test's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* For Android, an app explorer that traverses an Android app, interacting with it and capturing screenshots at the
* same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link TestType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The test's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* For Android, an app explorer that traverses an Android app, interacting with it and capturing screenshots
* at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @see TestType
*/
public String typeAsString() {
return type;
}
/**
*
* When the test was created.
*
*
* @return When the test was created.
*/
public Instant created() {
return created;
}
/**
*
* The test's status.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The test's status.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
* @see ExecutionStatus
*/
public ExecutionStatus status() {
return ExecutionStatus.fromValue(status);
}
/**
*
* The test's status.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The test's status.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
* @see ExecutionStatus
*/
public String statusAsString() {
return status;
}
/**
*
* The test's result.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The test's result.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
* @see ExecutionResult
*/
public ExecutionResult result() {
return ExecutionResult.fromValue(result);
}
/**
*
* The test's result.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The test's result.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
* @see ExecutionResult
*/
public String resultAsString() {
return result;
}
/**
*
* The test's start time.
*
*
* @return The test's start time.
*/
public Instant started() {
return started;
}
/**
*
* The test's stop time.
*
*
* @return The test's stop time.
*/
public Instant stopped() {
return stopped;
}
/**
*
* The test's result counters.
*
*
* @return The test's result counters.
*/
public Counters counters() {
return counters;
}
/**
*
* A message about the test's result.
*
*
* @return A message about the test's result.
*/
public String message() {
return message;
}
/**
*
* Represents the total (metered or unmetered) minutes used by the test.
*
*
* @return Represents the total (metered or unmetered) minutes used by the test.
*/
public DeviceMinutes deviceMinutes() {
return deviceMinutes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(created());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(resultAsString());
hashCode = 31 * hashCode + Objects.hashCode(started());
hashCode = 31 * hashCode + Objects.hashCode(stopped());
hashCode = 31 * hashCode + Objects.hashCode(counters());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(deviceMinutes());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Test)) {
return false;
}
Test other = (Test) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(created(), other.created())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(resultAsString(), other.resultAsString()) && Objects.equals(started(), other.started())
&& Objects.equals(stopped(), other.stopped()) && Objects.equals(counters(), other.counters())
&& Objects.equals(message(), other.message()) && Objects.equals(deviceMinutes(), other.deviceMinutes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Test").add("Arn", arn()).add("Name", name()).add("Type", typeAsString())
.add("Created", created()).add("Status", statusAsString()).add("Result", resultAsString())
.add("Started", started()).add("Stopped", stopped()).add("Counters", counters()).add("Message", message())
.add("DeviceMinutes", deviceMinutes()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "created":
return Optional.ofNullable(clazz.cast(created()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "result":
return Optional.ofNullable(clazz.cast(resultAsString()));
case "started":
return Optional.ofNullable(clazz.cast(started()));
case "stopped":
return Optional.ofNullable(clazz.cast(stopped()));
case "counters":
return Optional.ofNullable(clazz.cast(counters()));
case "message":
return Optional.ofNullable(clazz.cast(message()));
case "deviceMinutes":
return Optional.ofNullable(clazz.cast(deviceMinutes()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function