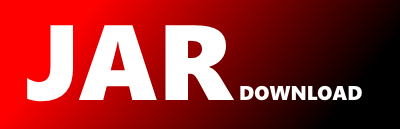
software.amazon.awssdk.services.devicefarm.model.Counters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of devicefarm Show documentation
Show all versions of devicefarm Show documentation
The AWS Java SDK for AWS Device Farm module holds the client classes that are used for communicating
with AWS Device Farm
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents entity counters.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Counters implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TOTAL_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("total")
.getter(getter(Counters::total)).setter(setter(Builder::total))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("total").build()).build();
private static final SdkField PASSED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("passed").getter(getter(Counters::passed)).setter(setter(Builder::passed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("passed").build()).build();
private static final SdkField FAILED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("failed").getter(getter(Counters::failed)).setter(setter(Builder::failed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("failed").build()).build();
private static final SdkField WARNED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("warned").getter(getter(Counters::warned)).setter(setter(Builder::warned))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("warned").build()).build();
private static final SdkField ERRORED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("errored").getter(getter(Counters::errored)).setter(setter(Builder::errored))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("errored").build()).build();
private static final SdkField STOPPED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("stopped").getter(getter(Counters::stopped)).setter(setter(Builder::stopped))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stopped").build()).build();
private static final SdkField SKIPPED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("skipped").getter(getter(Counters::skipped)).setter(setter(Builder::skipped))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("skipped").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TOTAL_FIELD, PASSED_FIELD,
FAILED_FIELD, WARNED_FIELD, ERRORED_FIELD, STOPPED_FIELD, SKIPPED_FIELD));
private static final long serialVersionUID = 1L;
private final Integer total;
private final Integer passed;
private final Integer failed;
private final Integer warned;
private final Integer errored;
private final Integer stopped;
private final Integer skipped;
private Counters(BuilderImpl builder) {
this.total = builder.total;
this.passed = builder.passed;
this.failed = builder.failed;
this.warned = builder.warned;
this.errored = builder.errored;
this.stopped = builder.stopped;
this.skipped = builder.skipped;
}
/**
*
* The total number of entities.
*
*
* @return The total number of entities.
*/
public Integer total() {
return total;
}
/**
*
* The number of passed entities.
*
*
* @return The number of passed entities.
*/
public Integer passed() {
return passed;
}
/**
*
* The number of failed entities.
*
*
* @return The number of failed entities.
*/
public Integer failed() {
return failed;
}
/**
*
* The number of warned entities.
*
*
* @return The number of warned entities.
*/
public Integer warned() {
return warned;
}
/**
*
* The number of errored entities.
*
*
* @return The number of errored entities.
*/
public Integer errored() {
return errored;
}
/**
*
* The number of stopped entities.
*
*
* @return The number of stopped entities.
*/
public Integer stopped() {
return stopped;
}
/**
*
* The number of skipped entities.
*
*
* @return The number of skipped entities.
*/
public Integer skipped() {
return skipped;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(total());
hashCode = 31 * hashCode + Objects.hashCode(passed());
hashCode = 31 * hashCode + Objects.hashCode(failed());
hashCode = 31 * hashCode + Objects.hashCode(warned());
hashCode = 31 * hashCode + Objects.hashCode(errored());
hashCode = 31 * hashCode + Objects.hashCode(stopped());
hashCode = 31 * hashCode + Objects.hashCode(skipped());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Counters)) {
return false;
}
Counters other = (Counters) obj;
return Objects.equals(total(), other.total()) && Objects.equals(passed(), other.passed())
&& Objects.equals(failed(), other.failed()) && Objects.equals(warned(), other.warned())
&& Objects.equals(errored(), other.errored()) && Objects.equals(stopped(), other.stopped())
&& Objects.equals(skipped(), other.skipped());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Counters").add("Total", total()).add("Passed", passed()).add("Failed", failed())
.add("Warned", warned()).add("Errored", errored()).add("Stopped", stopped()).add("Skipped", skipped()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "total":
return Optional.ofNullable(clazz.cast(total()));
case "passed":
return Optional.ofNullable(clazz.cast(passed()));
case "failed":
return Optional.ofNullable(clazz.cast(failed()));
case "warned":
return Optional.ofNullable(clazz.cast(warned()));
case "errored":
return Optional.ofNullable(clazz.cast(errored()));
case "stopped":
return Optional.ofNullable(clazz.cast(stopped()));
case "skipped":
return Optional.ofNullable(clazz.cast(skipped()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy