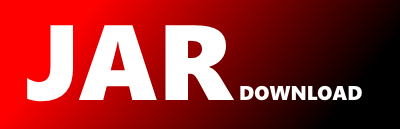
software.amazon.awssdk.services.devicefarm.DeviceFarmClient Maven / Gradle / Ivy
Show all versions of devicefarm Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.devicefarm.model.ArgumentException;
import software.amazon.awssdk.services.devicefarm.model.CannotDeleteException;
import software.amazon.awssdk.services.devicefarm.model.CreateDevicePoolRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateDevicePoolResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateInstanceProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateInstanceProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateNetworkProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateNetworkProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateTestGridProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateTestGridProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateTestGridUrlRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateTestGridUrlResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateUploadRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateUploadResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateVpceConfigurationRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateVpceConfigurationResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteDevicePoolRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteDevicePoolResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteInstanceProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteInstanceProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteNetworkProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteNetworkProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteRunRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteRunResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteTestGridProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteTestGridProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteUploadRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteUploadResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteVpceConfigurationRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteVpceConfigurationResponse;
import software.amazon.awssdk.services.devicefarm.model.DeviceFarmException;
import software.amazon.awssdk.services.devicefarm.model.GetAccountSettingsRequest;
import software.amazon.awssdk.services.devicefarm.model.GetAccountSettingsResponse;
import software.amazon.awssdk.services.devicefarm.model.GetDeviceInstanceRequest;
import software.amazon.awssdk.services.devicefarm.model.GetDeviceInstanceResponse;
import software.amazon.awssdk.services.devicefarm.model.GetDevicePoolCompatibilityRequest;
import software.amazon.awssdk.services.devicefarm.model.GetDevicePoolCompatibilityResponse;
import software.amazon.awssdk.services.devicefarm.model.GetDevicePoolRequest;
import software.amazon.awssdk.services.devicefarm.model.GetDevicePoolResponse;
import software.amazon.awssdk.services.devicefarm.model.GetDeviceRequest;
import software.amazon.awssdk.services.devicefarm.model.GetDeviceResponse;
import software.amazon.awssdk.services.devicefarm.model.GetInstanceProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.GetInstanceProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.GetJobRequest;
import software.amazon.awssdk.services.devicefarm.model.GetJobResponse;
import software.amazon.awssdk.services.devicefarm.model.GetNetworkProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.GetNetworkProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusRequest;
import software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusResponse;
import software.amazon.awssdk.services.devicefarm.model.GetProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.GetProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.GetRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.GetRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.GetRunRequest;
import software.amazon.awssdk.services.devicefarm.model.GetRunResponse;
import software.amazon.awssdk.services.devicefarm.model.GetSuiteRequest;
import software.amazon.awssdk.services.devicefarm.model.GetSuiteResponse;
import software.amazon.awssdk.services.devicefarm.model.GetTestGridProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.GetTestGridProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.GetTestGridSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.GetTestGridSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.GetTestRequest;
import software.amazon.awssdk.services.devicefarm.model.GetTestResponse;
import software.amazon.awssdk.services.devicefarm.model.GetUploadRequest;
import software.amazon.awssdk.services.devicefarm.model.GetUploadResponse;
import software.amazon.awssdk.services.devicefarm.model.GetVpceConfigurationRequest;
import software.amazon.awssdk.services.devicefarm.model.GetVpceConfigurationResponse;
import software.amazon.awssdk.services.devicefarm.model.IdempotencyException;
import software.amazon.awssdk.services.devicefarm.model.InstallToRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.InstallToRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.InternalServiceException;
import software.amazon.awssdk.services.devicefarm.model.InvalidOperationException;
import software.amazon.awssdk.services.devicefarm.model.LimitExceededException;
import software.amazon.awssdk.services.devicefarm.model.ListArtifactsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListArtifactsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListDeviceInstancesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListDeviceInstancesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListDevicesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListDevicesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListInstanceProfilesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListInstanceProfilesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListJobsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListJobsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListNetworkProfilesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListNetworkProfilesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingPromotionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingPromotionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListProjectsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListProjectsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListRemoteAccessSessionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListRemoteAccessSessionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListRunsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListRunsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListSamplesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListSamplesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListSuitesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListSuitesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListUploadsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListUploadsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListVpceConfigurationsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListVpceConfigurationsResponse;
import software.amazon.awssdk.services.devicefarm.model.NotEligibleException;
import software.amazon.awssdk.services.devicefarm.model.NotFoundException;
import software.amazon.awssdk.services.devicefarm.model.PurchaseOfferingRequest;
import software.amazon.awssdk.services.devicefarm.model.PurchaseOfferingResponse;
import software.amazon.awssdk.services.devicefarm.model.RenewOfferingRequest;
import software.amazon.awssdk.services.devicefarm.model.RenewOfferingResponse;
import software.amazon.awssdk.services.devicefarm.model.ScheduleRunRequest;
import software.amazon.awssdk.services.devicefarm.model.ScheduleRunResponse;
import software.amazon.awssdk.services.devicefarm.model.ServiceAccountException;
import software.amazon.awssdk.services.devicefarm.model.StopJobRequest;
import software.amazon.awssdk.services.devicefarm.model.StopJobResponse;
import software.amazon.awssdk.services.devicefarm.model.StopRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.StopRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.StopRunRequest;
import software.amazon.awssdk.services.devicefarm.model.StopRunResponse;
import software.amazon.awssdk.services.devicefarm.model.TagOperationException;
import software.amazon.awssdk.services.devicefarm.model.TagPolicyException;
import software.amazon.awssdk.services.devicefarm.model.TagResourceRequest;
import software.amazon.awssdk.services.devicefarm.model.TagResourceResponse;
import software.amazon.awssdk.services.devicefarm.model.TooManyTagsException;
import software.amazon.awssdk.services.devicefarm.model.UntagResourceRequest;
import software.amazon.awssdk.services.devicefarm.model.UntagResourceResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateDeviceInstanceRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateDeviceInstanceResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateDevicePoolRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateDevicePoolResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateInstanceProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateInstanceProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateNetworkProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateNetworkProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateTestGridProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateTestGridProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateUploadRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateUploadResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateVpceConfigurationRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateVpceConfigurationResponse;
import software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListArtifactsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListDevicePoolsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListJobsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListRunsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListSamplesIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListSuitesIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListTestGridProjectsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionActionsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionArtifactsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListTestsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListUniqueProblemsIterable;
import software.amazon.awssdk.services.devicefarm.paginators.ListUploadsIterable;
/**
* Service client for accessing AWS Device Farm. This can be created using the static {@link #builder()} method.
*
*
* Welcome to the AWS Device Farm API documentation, which contains APIs for:
*
*
* -
*
* Testing on desktop browsers
*
*
* Device Farm makes it possible for you to test your web applications on desktop browsers using Selenium. The APIs for
* desktop browser testing contain TestGrid
in their names. For more information, see Testing Web Applications on Selenium with Device
* Farm.
*
*
* -
*
* Testing on real mobile devices
*
*
* Device Farm makes it possible for you to test apps on physical phones, tablets, and other devices in the cloud. For
* more information, see the Device Farm
* Developer Guide.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface DeviceFarmClient extends SdkClient {
String SERVICE_NAME = "devicefarm";
/**
* Create a {@link DeviceFarmClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static DeviceFarmClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link DeviceFarmClient}.
*/
static DeviceFarmClientBuilder builder() {
return new DefaultDeviceFarmClientBuilder();
}
/**
*
* Creates a device pool.
*
*
* @param createDevicePoolRequest
* Represents a request to the create device pool operation.
* @return Result of the CreateDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateDevicePool
* @see AWS
* API Documentation
*/
default CreateDevicePoolResponse createDevicePool(CreateDevicePoolRequest createDevicePoolRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a device pool.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDevicePoolRequest.Builder} avoiding the need
* to create one manually via {@link CreateDevicePoolRequest#builder()}
*
*
* @param createDevicePoolRequest
* A {@link Consumer} that will call methods on {@link CreateDevicePoolRequest.Builder} to create a request.
* Represents a request to the create device pool operation.
* @return Result of the CreateDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateDevicePool
* @see AWS
* API Documentation
*/
default CreateDevicePoolResponse createDevicePool(Consumer createDevicePoolRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return createDevicePool(CreateDevicePoolRequest.builder().applyMutation(createDevicePoolRequest).build());
}
/**
*
* Creates a profile that can be applied to one or more private fleet device instances.
*
*
* @param createInstanceProfileRequest
* @return Result of the CreateInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateInstanceProfile
* @see AWS API Documentation
*/
default CreateInstanceProfileResponse createInstanceProfile(CreateInstanceProfileRequest createInstanceProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a profile that can be applied to one or more private fleet device instances.
*
*
*
* This is a convenience which creates an instance of the {@link CreateInstanceProfileRequest.Builder} avoiding the
* need to create one manually via {@link CreateInstanceProfileRequest#builder()}
*
*
* @param createInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link CreateInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the CreateInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateInstanceProfile
* @see AWS API Documentation
*/
default CreateInstanceProfileResponse createInstanceProfile(
Consumer createInstanceProfileRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return createInstanceProfile(CreateInstanceProfileRequest.builder().applyMutation(createInstanceProfileRequest).build());
}
/**
*
* Creates a network profile.
*
*
* @param createNetworkProfileRequest
* @return Result of the CreateNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateNetworkProfile
* @see AWS API Documentation
*/
default CreateNetworkProfileResponse createNetworkProfile(CreateNetworkProfileRequest createNetworkProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a network profile.
*
*
*
* This is a convenience which creates an instance of the {@link CreateNetworkProfileRequest.Builder} avoiding the
* need to create one manually via {@link CreateNetworkProfileRequest#builder()}
*
*
* @param createNetworkProfileRequest
* A {@link Consumer} that will call methods on {@link CreateNetworkProfileRequest.Builder} to create a
* request.
* @return Result of the CreateNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateNetworkProfile
* @see AWS API Documentation
*/
default CreateNetworkProfileResponse createNetworkProfile(
Consumer createNetworkProfileRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return createNetworkProfile(CreateNetworkProfileRequest.builder().applyMutation(createNetworkProfileRequest).build());
}
/**
*
* Creates a project.
*
*
* @param createProjectRequest
* Represents a request to the create project operation.
* @return Result of the CreateProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws TagOperationException
* The operation was not successful. Try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateProject
* @see AWS API
* Documentation
*/
default CreateProjectResponse createProject(CreateProjectRequest createProjectRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, TagOperationException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a project.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProjectRequest.Builder} avoiding the need to
* create one manually via {@link CreateProjectRequest#builder()}
*
*
* @param createProjectRequest
* A {@link Consumer} that will call methods on {@link CreateProjectRequest.Builder} to create a request.
* Represents a request to the create project operation.
* @return Result of the CreateProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws TagOperationException
* The operation was not successful. Try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateProject
* @see AWS API
* Documentation
*/
default CreateProjectResponse createProject(Consumer createProjectRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, TagOperationException,
AwsServiceException, SdkClientException, DeviceFarmException {
return createProject(CreateProjectRequest.builder().applyMutation(createProjectRequest).build());
}
/**
*
* Specifies and starts a remote access session.
*
*
* @param createRemoteAccessSessionRequest
* Creates and submits a request to start a remote access session.
* @return Result of the CreateRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateRemoteAccessSession
* @see AWS API Documentation
*/
default CreateRemoteAccessSessionResponse createRemoteAccessSession(
CreateRemoteAccessSessionRequest createRemoteAccessSessionRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Specifies and starts a remote access session.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRemoteAccessSessionRequest.Builder} avoiding
* the need to create one manually via {@link CreateRemoteAccessSessionRequest#builder()}
*
*
* @param createRemoteAccessSessionRequest
* A {@link Consumer} that will call methods on {@link CreateRemoteAccessSessionRequest.Builder} to create a
* request. Creates and submits a request to start a remote access session.
* @return Result of the CreateRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateRemoteAccessSession
* @see AWS API Documentation
*/
default CreateRemoteAccessSessionResponse createRemoteAccessSession(
Consumer createRemoteAccessSessionRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return createRemoteAccessSession(CreateRemoteAccessSessionRequest.builder()
.applyMutation(createRemoteAccessSessionRequest).build());
}
/**
*
* Creates a Selenium testing project. Projects are used to track TestGridSession instances.
*
*
* @param createTestGridProjectRequest
* @return Result of the CreateTestGridProject operation returned by the service.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateTestGridProject
* @see AWS API Documentation
*/
default CreateTestGridProjectResponse createTestGridProject(CreateTestGridProjectRequest createTestGridProjectRequest)
throws InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a Selenium testing project. Projects are used to track TestGridSession instances.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTestGridProjectRequest.Builder} avoiding the
* need to create one manually via {@link CreateTestGridProjectRequest#builder()}
*
*
* @param createTestGridProjectRequest
* A {@link Consumer} that will call methods on {@link CreateTestGridProjectRequest.Builder} to create a
* request.
* @return Result of the CreateTestGridProject operation returned by the service.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateTestGridProject
* @see AWS API Documentation
*/
default CreateTestGridProjectResponse createTestGridProject(
Consumer createTestGridProjectRequest) throws InternalServiceException,
AwsServiceException, SdkClientException, DeviceFarmException {
return createTestGridProject(CreateTestGridProjectRequest.builder().applyMutation(createTestGridProjectRequest).build());
}
/**
*
* Creates a signed, short-term URL that can be passed to a Selenium RemoteWebDriver
constructor.
*
*
* @param createTestGridUrlRequest
* @return Result of the CreateTestGridUrl operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateTestGridUrl
* @see AWS
* API Documentation
*/
default CreateTestGridUrlResponse createTestGridUrl(CreateTestGridUrlRequest createTestGridUrlRequest)
throws NotFoundException, ArgumentException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a signed, short-term URL that can be passed to a Selenium RemoteWebDriver
constructor.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTestGridUrlRequest.Builder} avoiding the need
* to create one manually via {@link CreateTestGridUrlRequest#builder()}
*
*
* @param createTestGridUrlRequest
* A {@link Consumer} that will call methods on {@link CreateTestGridUrlRequest.Builder} to create a request.
* @return Result of the CreateTestGridUrl operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateTestGridUrl
* @see AWS
* API Documentation
*/
default CreateTestGridUrlResponse createTestGridUrl(Consumer createTestGridUrlRequest)
throws NotFoundException, ArgumentException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
return createTestGridUrl(CreateTestGridUrlRequest.builder().applyMutation(createTestGridUrlRequest).build());
}
/**
*
* Uploads an app or test scripts.
*
*
* @param createUploadRequest
* Represents a request to the create upload operation.
* @return Result of the CreateUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateUpload
* @see AWS API
* Documentation
*/
default CreateUploadResponse createUpload(CreateUploadRequest createUploadRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Uploads an app or test scripts.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUploadRequest.Builder} avoiding the need to
* create one manually via {@link CreateUploadRequest#builder()}
*
*
* @param createUploadRequest
* A {@link Consumer} that will call methods on {@link CreateUploadRequest.Builder} to create a request.
* Represents a request to the create upload operation.
* @return Result of the CreateUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateUpload
* @see AWS API
* Documentation
*/
default CreateUploadResponse createUpload(Consumer createUploadRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return createUpload(CreateUploadRequest.builder().applyMutation(createUploadRequest).build());
}
/**
*
* Creates a configuration record in Device Farm for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param createVpceConfigurationRequest
* @return Result of the CreateVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateVPCEConfiguration
* @see AWS API Documentation
*/
default CreateVpceConfigurationResponse createVPCEConfiguration(CreateVpceConfigurationRequest createVpceConfigurationRequest)
throws ArgumentException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a configuration record in Device Farm for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVpceConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link CreateVpceConfigurationRequest#builder()}
*
*
* @param createVpceConfigurationRequest
* A {@link Consumer} that will call methods on {@link CreateVPCEConfigurationRequest.Builder} to create a
* request.
* @return Result of the CreateVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.CreateVPCEConfiguration
* @see AWS API Documentation
*/
default CreateVpceConfigurationResponse createVPCEConfiguration(
Consumer createVpceConfigurationRequest) throws ArgumentException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return createVPCEConfiguration(CreateVpceConfigurationRequest.builder().applyMutation(createVpceConfigurationRequest)
.build());
}
/**
*
* Deletes a device pool given the pool ARN. Does not allow deletion of curated pools owned by the system.
*
*
* @param deleteDevicePoolRequest
* Represents a request to the delete device pool operation.
* @return Result of the DeleteDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteDevicePool
* @see AWS
* API Documentation
*/
default DeleteDevicePoolResponse deleteDevicePool(DeleteDevicePoolRequest deleteDevicePoolRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a device pool given the pool ARN. Does not allow deletion of curated pools owned by the system.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDevicePoolRequest.Builder} avoiding the need
* to create one manually via {@link DeleteDevicePoolRequest#builder()}
*
*
* @param deleteDevicePoolRequest
* A {@link Consumer} that will call methods on {@link DeleteDevicePoolRequest.Builder} to create a request.
* Represents a request to the delete device pool operation.
* @return Result of the DeleteDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteDevicePool
* @see AWS
* API Documentation
*/
default DeleteDevicePoolResponse deleteDevicePool(Consumer deleteDevicePoolRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return deleteDevicePool(DeleteDevicePoolRequest.builder().applyMutation(deleteDevicePoolRequest).build());
}
/**
*
* Deletes a profile that can be applied to one or more private device instances.
*
*
* @param deleteInstanceProfileRequest
* @return Result of the DeleteInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteInstanceProfile
* @see AWS API Documentation
*/
default DeleteInstanceProfileResponse deleteInstanceProfile(DeleteInstanceProfileRequest deleteInstanceProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a profile that can be applied to one or more private device instances.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteInstanceProfileRequest.Builder} avoiding the
* need to create one manually via {@link DeleteInstanceProfileRequest#builder()}
*
*
* @param deleteInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link DeleteInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the DeleteInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteInstanceProfile
* @see AWS API Documentation
*/
default DeleteInstanceProfileResponse deleteInstanceProfile(
Consumer deleteInstanceProfileRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return deleteInstanceProfile(DeleteInstanceProfileRequest.builder().applyMutation(deleteInstanceProfileRequest).build());
}
/**
*
* Deletes a network profile.
*
*
* @param deleteNetworkProfileRequest
* @return Result of the DeleteNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteNetworkProfile
* @see AWS API Documentation
*/
default DeleteNetworkProfileResponse deleteNetworkProfile(DeleteNetworkProfileRequest deleteNetworkProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a network profile.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNetworkProfileRequest.Builder} avoiding the
* need to create one manually via {@link DeleteNetworkProfileRequest#builder()}
*
*
* @param deleteNetworkProfileRequest
* A {@link Consumer} that will call methods on {@link DeleteNetworkProfileRequest.Builder} to create a
* request.
* @return Result of the DeleteNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteNetworkProfile
* @see AWS API Documentation
*/
default DeleteNetworkProfileResponse deleteNetworkProfile(
Consumer deleteNetworkProfileRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return deleteNetworkProfile(DeleteNetworkProfileRequest.builder().applyMutation(deleteNetworkProfileRequest).build());
}
/**
*
* Deletes an AWS Device Farm project, given the project ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteProjectRequest
* Represents a request to the delete project operation.
* @return Result of the DeleteProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteProject
* @see AWS API
* Documentation
*/
default DeleteProjectResponse deleteProject(DeleteProjectRequest deleteProjectRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an AWS Device Farm project, given the project ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProjectRequest.Builder} avoiding the need to
* create one manually via {@link DeleteProjectRequest#builder()}
*
*
* @param deleteProjectRequest
* A {@link Consumer} that will call methods on {@link DeleteProjectRequest.Builder} to create a request.
* Represents a request to the delete project operation.
* @return Result of the DeleteProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteProject
* @see AWS API
* Documentation
*/
default DeleteProjectResponse deleteProject(Consumer deleteProjectRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return deleteProject(DeleteProjectRequest.builder().applyMutation(deleteProjectRequest).build());
}
/**
*
* Deletes a completed remote access session and its results.
*
*
* @param deleteRemoteAccessSessionRequest
* Represents the request to delete the specified remote access session.
* @return Result of the DeleteRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteRemoteAccessSession
* @see AWS API Documentation
*/
default DeleteRemoteAccessSessionResponse deleteRemoteAccessSession(
DeleteRemoteAccessSessionRequest deleteRemoteAccessSessionRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a completed remote access session and its results.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRemoteAccessSessionRequest.Builder} avoiding
* the need to create one manually via {@link DeleteRemoteAccessSessionRequest#builder()}
*
*
* @param deleteRemoteAccessSessionRequest
* A {@link Consumer} that will call methods on {@link DeleteRemoteAccessSessionRequest.Builder} to create a
* request. Represents the request to delete the specified remote access session.
* @return Result of the DeleteRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteRemoteAccessSession
* @see AWS API Documentation
*/
default DeleteRemoteAccessSessionResponse deleteRemoteAccessSession(
Consumer deleteRemoteAccessSessionRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return deleteRemoteAccessSession(DeleteRemoteAccessSessionRequest.builder()
.applyMutation(deleteRemoteAccessSessionRequest).build());
}
/**
*
* Deletes the run, given the run ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteRunRequest
* Represents a request to the delete run operation.
* @return Result of the DeleteRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteRun
* @see AWS API
* Documentation
*/
default DeleteRunResponse deleteRun(DeleteRunRequest deleteRunRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the run, given the run ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRunRequest.Builder} avoiding the need to
* create one manually via {@link DeleteRunRequest#builder()}
*
*
* @param deleteRunRequest
* A {@link Consumer} that will call methods on {@link DeleteRunRequest.Builder} to create a request.
* Represents a request to the delete run operation.
* @return Result of the DeleteRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteRun
* @see AWS API
* Documentation
*/
default DeleteRunResponse deleteRun(Consumer deleteRunRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return deleteRun(DeleteRunRequest.builder().applyMutation(deleteRunRequest).build());
}
/**
*
* Deletes a Selenium testing project and all content generated under it.
*
*
*
* You cannot undo this operation.
*
*
*
* You cannot delete a project if it has active sessions.
*
*
*
* @param deleteTestGridProjectRequest
* @return Result of the DeleteTestGridProject operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws CannotDeleteException
* The requested object could not be deleted.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteTestGridProject
* @see AWS API Documentation
*/
default DeleteTestGridProjectResponse deleteTestGridProject(DeleteTestGridProjectRequest deleteTestGridProjectRequest)
throws NotFoundException, ArgumentException, CannotDeleteException, InternalServiceException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a Selenium testing project and all content generated under it.
*
*
*
* You cannot undo this operation.
*
*
*
* You cannot delete a project if it has active sessions.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTestGridProjectRequest.Builder} avoiding the
* need to create one manually via {@link DeleteTestGridProjectRequest#builder()}
*
*
* @param deleteTestGridProjectRequest
* A {@link Consumer} that will call methods on {@link DeleteTestGridProjectRequest.Builder} to create a
* request.
* @return Result of the DeleteTestGridProject operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws CannotDeleteException
* The requested object could not be deleted.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteTestGridProject
* @see AWS API Documentation
*/
default DeleteTestGridProjectResponse deleteTestGridProject(
Consumer deleteTestGridProjectRequest) throws NotFoundException,
ArgumentException, CannotDeleteException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
return deleteTestGridProject(DeleteTestGridProjectRequest.builder().applyMutation(deleteTestGridProjectRequest).build());
}
/**
*
* Deletes an upload given the upload ARN.
*
*
* @param deleteUploadRequest
* Represents a request to the delete upload operation.
* @return Result of the DeleteUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteUpload
* @see AWS API
* Documentation
*/
default DeleteUploadResponse deleteUpload(DeleteUploadRequest deleteUploadRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an upload given the upload ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUploadRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUploadRequest#builder()}
*
*
* @param deleteUploadRequest
* A {@link Consumer} that will call methods on {@link DeleteUploadRequest.Builder} to create a request.
* Represents a request to the delete upload operation.
* @return Result of the DeleteUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteUpload
* @see AWS API
* Documentation
*/
default DeleteUploadResponse deleteUpload(Consumer deleteUploadRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return deleteUpload(DeleteUploadRequest.builder().applyMutation(deleteUploadRequest).build());
}
/**
*
* Deletes a configuration for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param deleteVpceConfigurationRequest
* @return Result of the DeleteVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws InvalidOperationException
* There was an error with the update request, or you do not have sufficient permissions to update this VPC
* endpoint configuration.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteVPCEConfiguration
* @see AWS API Documentation
*/
default DeleteVpceConfigurationResponse deleteVPCEConfiguration(DeleteVpceConfigurationRequest deleteVpceConfigurationRequest)
throws ArgumentException, NotFoundException, ServiceAccountException, InvalidOperationException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a configuration for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVpceConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link DeleteVpceConfigurationRequest#builder()}
*
*
* @param deleteVpceConfigurationRequest
* A {@link Consumer} that will call methods on {@link DeleteVPCEConfigurationRequest.Builder} to create a
* request.
* @return Result of the DeleteVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws InvalidOperationException
* There was an error with the update request, or you do not have sufficient permissions to update this VPC
* endpoint configuration.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.DeleteVPCEConfiguration
* @see AWS API Documentation
*/
default DeleteVpceConfigurationResponse deleteVPCEConfiguration(
Consumer deleteVpceConfigurationRequest) throws ArgumentException,
NotFoundException, ServiceAccountException, InvalidOperationException, AwsServiceException, SdkClientException,
DeviceFarmException {
return deleteVPCEConfiguration(DeleteVpceConfigurationRequest.builder().applyMutation(deleteVpceConfigurationRequest)
.build());
}
/**
*
* Returns the number of unmetered iOS or unmetered Android devices that have been purchased by the account.
*
*
* @return Result of the GetAccountSettings operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetAccountSettings
* @see #getAccountSettings(GetAccountSettingsRequest)
* @see AWS
* API Documentation
*/
default GetAccountSettingsResponse getAccountSettings() throws ArgumentException, NotFoundException, LimitExceededException,
ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return getAccountSettings(GetAccountSettingsRequest.builder().build());
}
/**
*
* Returns the number of unmetered iOS or unmetered Android devices that have been purchased by the account.
*
*
* @param getAccountSettingsRequest
* Represents the request sent to retrieve the account settings.
* @return Result of the GetAccountSettings operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetAccountSettings
* @see AWS
* API Documentation
*/
default GetAccountSettingsResponse getAccountSettings(GetAccountSettingsRequest getAccountSettingsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the number of unmetered iOS or unmetered Android devices that have been purchased by the account.
*
*
*
* This is a convenience which creates an instance of the {@link GetAccountSettingsRequest.Builder} avoiding the
* need to create one manually via {@link GetAccountSettingsRequest#builder()}
*
*
* @param getAccountSettingsRequest
* A {@link Consumer} that will call methods on {@link GetAccountSettingsRequest.Builder} to create a
* request. Represents the request sent to retrieve the account settings.
* @return Result of the GetAccountSettings operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetAccountSettings
* @see AWS
* API Documentation
*/
default GetAccountSettingsResponse getAccountSettings(Consumer getAccountSettingsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return getAccountSettings(GetAccountSettingsRequest.builder().applyMutation(getAccountSettingsRequest).build());
}
/**
*
* Gets information about a unique device type.
*
*
* @param getDeviceRequest
* Represents a request to the get device request.
* @return Result of the GetDevice operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetDevice
* @see AWS API
* Documentation
*/
default GetDeviceResponse getDevice(GetDeviceRequest getDeviceRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a unique device type.
*
*
*
* This is a convenience which creates an instance of the {@link GetDeviceRequest.Builder} avoiding the need to
* create one manually via {@link GetDeviceRequest#builder()}
*
*
* @param getDeviceRequest
* A {@link Consumer} that will call methods on {@link GetDeviceRequest.Builder} to create a request.
* Represents a request to the get device request.
* @return Result of the GetDevice operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetDevice
* @see AWS API
* Documentation
*/
default GetDeviceResponse getDevice(Consumer getDeviceRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getDevice(GetDeviceRequest.builder().applyMutation(getDeviceRequest).build());
}
/**
*
* Returns information about a device instance that belongs to a private device fleet.
*
*
* @param getDeviceInstanceRequest
* @return Result of the GetDeviceInstance operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetDeviceInstance
* @see AWS
* API Documentation
*/
default GetDeviceInstanceResponse getDeviceInstance(GetDeviceInstanceRequest getDeviceInstanceRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a device instance that belongs to a private device fleet.
*
*
*
* This is a convenience which creates an instance of the {@link GetDeviceInstanceRequest.Builder} avoiding the need
* to create one manually via {@link GetDeviceInstanceRequest#builder()}
*
*
* @param getDeviceInstanceRequest
* A {@link Consumer} that will call methods on {@link GetDeviceInstanceRequest.Builder} to create a request.
* @return Result of the GetDeviceInstance operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetDeviceInstance
* @see AWS
* API Documentation
*/
default GetDeviceInstanceResponse getDeviceInstance(Consumer getDeviceInstanceRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return getDeviceInstance(GetDeviceInstanceRequest.builder().applyMutation(getDeviceInstanceRequest).build());
}
/**
*
* Gets information about a device pool.
*
*
* @param getDevicePoolRequest
* Represents a request to the get device pool operation.
* @return Result of the GetDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetDevicePool
* @see AWS API
* Documentation
*/
default GetDevicePoolResponse getDevicePool(GetDevicePoolRequest getDevicePoolRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a device pool.
*
*
*
* This is a convenience which creates an instance of the {@link GetDevicePoolRequest.Builder} avoiding the need to
* create one manually via {@link GetDevicePoolRequest#builder()}
*
*
* @param getDevicePoolRequest
* A {@link Consumer} that will call methods on {@link GetDevicePoolRequest.Builder} to create a request.
* Represents a request to the get device pool operation.
* @return Result of the GetDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetDevicePool
* @see AWS API
* Documentation
*/
default GetDevicePoolResponse getDevicePool(Consumer getDevicePoolRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return getDevicePool(GetDevicePoolRequest.builder().applyMutation(getDevicePoolRequest).build());
}
/**
*
* Gets information about compatibility with a device pool.
*
*
* @param getDevicePoolCompatibilityRequest
* Represents a request to the get device pool compatibility operation.
* @return Result of the GetDevicePoolCompatibility operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetDevicePoolCompatibility
* @see AWS API Documentation
*/
default GetDevicePoolCompatibilityResponse getDevicePoolCompatibility(
GetDevicePoolCompatibilityRequest getDevicePoolCompatibilityRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about compatibility with a device pool.
*
*
*
* This is a convenience which creates an instance of the {@link GetDevicePoolCompatibilityRequest.Builder} avoiding
* the need to create one manually via {@link GetDevicePoolCompatibilityRequest#builder()}
*
*
* @param getDevicePoolCompatibilityRequest
* A {@link Consumer} that will call methods on {@link GetDevicePoolCompatibilityRequest.Builder} to create a
* request. Represents a request to the get device pool compatibility operation.
* @return Result of the GetDevicePoolCompatibility operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetDevicePoolCompatibility
* @see AWS API Documentation
*/
default GetDevicePoolCompatibilityResponse getDevicePoolCompatibility(
Consumer getDevicePoolCompatibilityRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getDevicePoolCompatibility(GetDevicePoolCompatibilityRequest.builder()
.applyMutation(getDevicePoolCompatibilityRequest).build());
}
/**
*
* Returns information about the specified instance profile.
*
*
* @param getInstanceProfileRequest
* @return Result of the GetInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetInstanceProfile
* @see AWS
* API Documentation
*/
default GetInstanceProfileResponse getInstanceProfile(GetInstanceProfileRequest getInstanceProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the specified instance profile.
*
*
*
* This is a convenience which creates an instance of the {@link GetInstanceProfileRequest.Builder} avoiding the
* need to create one manually via {@link GetInstanceProfileRequest#builder()}
*
*
* @param getInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link GetInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the GetInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetInstanceProfile
* @see AWS
* API Documentation
*/
default GetInstanceProfileResponse getInstanceProfile(Consumer getInstanceProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return getInstanceProfile(GetInstanceProfileRequest.builder().applyMutation(getInstanceProfileRequest).build());
}
/**
*
* Gets information about a job.
*
*
* @param getJobRequest
* Represents a request to the get job operation.
* @return Result of the GetJob operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetJob
* @see AWS API
* Documentation
*/
default GetJobResponse getJob(GetJobRequest getJobRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a job.
*
*
*
* This is a convenience which creates an instance of the {@link GetJobRequest.Builder} avoiding the need to create
* one manually via {@link GetJobRequest#builder()}
*
*
* @param getJobRequest
* A {@link Consumer} that will call methods on {@link GetJobRequest.Builder} to create a request. Represents
* a request to the get job operation.
* @return Result of the GetJob operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetJob
* @see AWS API
* Documentation
*/
default GetJobResponse getJob(Consumer getJobRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return getJob(GetJobRequest.builder().applyMutation(getJobRequest).build());
}
/**
*
* Returns information about a network profile.
*
*
* @param getNetworkProfileRequest
* @return Result of the GetNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetNetworkProfile
* @see AWS
* API Documentation
*/
default GetNetworkProfileResponse getNetworkProfile(GetNetworkProfileRequest getNetworkProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a network profile.
*
*
*
* This is a convenience which creates an instance of the {@link GetNetworkProfileRequest.Builder} avoiding the need
* to create one manually via {@link GetNetworkProfileRequest#builder()}
*
*
* @param getNetworkProfileRequest
* A {@link Consumer} that will call methods on {@link GetNetworkProfileRequest.Builder} to create a request.
* @return Result of the GetNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetNetworkProfile
* @see AWS
* API Documentation
*/
default GetNetworkProfileResponse getNetworkProfile(Consumer getNetworkProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return getNetworkProfile(GetNetworkProfileRequest.builder().applyMutation(getNetworkProfileRequest).build());
}
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
* @return Result of the GetOfferingStatus operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetOfferingStatus
* @see #getOfferingStatus(GetOfferingStatusRequest)
* @see AWS
* API Documentation
*/
default GetOfferingStatusResponse getOfferingStatus() throws ArgumentException, NotFoundException, NotEligibleException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return getOfferingStatus(GetOfferingStatusRequest.builder().build());
}
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
* @param getOfferingStatusRequest
* Represents the request to retrieve the offering status for the specified customer or account.
* @return Result of the GetOfferingStatus operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetOfferingStatus
* @see AWS
* API Documentation
*/
default GetOfferingStatusResponse getOfferingStatus(GetOfferingStatusRequest getOfferingStatusRequest)
throws ArgumentException, NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException,
AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
*
* This is a convenience which creates an instance of the {@link GetOfferingStatusRequest.Builder} avoiding the need
* to create one manually via {@link GetOfferingStatusRequest#builder()}
*
*
* @param getOfferingStatusRequest
* A {@link Consumer} that will call methods on {@link GetOfferingStatusRequest.Builder} to create a request.
* Represents the request to retrieve the offering status for the specified customer or account.
* @return Result of the GetOfferingStatus operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetOfferingStatus
* @see AWS
* API Documentation
*/
default GetOfferingStatusResponse getOfferingStatus(Consumer getOfferingStatusRequest)
throws ArgumentException, NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException,
AwsServiceException, SdkClientException, DeviceFarmException {
return getOfferingStatus(GetOfferingStatusRequest.builder().applyMutation(getOfferingStatusRequest).build());
}
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
*
* This is a variant of
* {@link #getOfferingStatus(software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable responses = client.getOfferingStatusPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable responses = client
* .getOfferingStatusPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable responses = client.getOfferingStatusPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getOfferingStatus(software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetOfferingStatus
* @see #getOfferingStatusPaginator(GetOfferingStatusRequest)
* @see AWS
* API Documentation
*/
default GetOfferingStatusIterable getOfferingStatusPaginator() throws ArgumentException, NotFoundException,
NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getOfferingStatusPaginator(GetOfferingStatusRequest.builder().build());
}
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
*
* This is a variant of
* {@link #getOfferingStatus(software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable responses = client.getOfferingStatusPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable responses = client
* .getOfferingStatusPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable responses = client.getOfferingStatusPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getOfferingStatus(software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusRequest)}
* operation.
*
*
* @param getOfferingStatusRequest
* Represents the request to retrieve the offering status for the specified customer or account.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetOfferingStatus
* @see AWS
* API Documentation
*/
default GetOfferingStatusIterable getOfferingStatusPaginator(GetOfferingStatusRequest getOfferingStatusRequest)
throws ArgumentException, NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException,
AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
*
* This is a variant of
* {@link #getOfferingStatus(software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable responses = client.getOfferingStatusPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable responses = client
* .getOfferingStatusPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.GetOfferingStatusIterable responses = client.getOfferingStatusPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getOfferingStatus(software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetOfferingStatusRequest.Builder} avoiding the need
* to create one manually via {@link GetOfferingStatusRequest#builder()}
*
*
* @param getOfferingStatusRequest
* A {@link Consumer} that will call methods on {@link GetOfferingStatusRequest.Builder} to create a request.
* Represents the request to retrieve the offering status for the specified customer or account.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetOfferingStatus
* @see AWS
* API Documentation
*/
default GetOfferingStatusIterable getOfferingStatusPaginator(
Consumer getOfferingStatusRequest) throws ArgumentException, NotFoundException,
NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getOfferingStatusPaginator(GetOfferingStatusRequest.builder().applyMutation(getOfferingStatusRequest).build());
}
/**
*
* Gets information about a project.
*
*
* @param getProjectRequest
* Represents a request to the get project operation.
* @return Result of the GetProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetProject
* @see AWS API
* Documentation
*/
default GetProjectResponse getProject(GetProjectRequest getProjectRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a project.
*
*
*
* This is a convenience which creates an instance of the {@link GetProjectRequest.Builder} avoiding the need to
* create one manually via {@link GetProjectRequest#builder()}
*
*
* @param getProjectRequest
* A {@link Consumer} that will call methods on {@link GetProjectRequest.Builder} to create a request.
* Represents a request to the get project operation.
* @return Result of the GetProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetProject
* @see AWS API
* Documentation
*/
default GetProjectResponse getProject(Consumer getProjectRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getProject(GetProjectRequest.builder().applyMutation(getProjectRequest).build());
}
/**
*
* Returns a link to a currently running remote access session.
*
*
* @param getRemoteAccessSessionRequest
* Represents the request to get information about the specified remote access session.
* @return Result of the GetRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetRemoteAccessSession
* @see AWS API Documentation
*/
default GetRemoteAccessSessionResponse getRemoteAccessSession(GetRemoteAccessSessionRequest getRemoteAccessSessionRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a link to a currently running remote access session.
*
*
*
* This is a convenience which creates an instance of the {@link GetRemoteAccessSessionRequest.Builder} avoiding the
* need to create one manually via {@link GetRemoteAccessSessionRequest#builder()}
*
*
* @param getRemoteAccessSessionRequest
* A {@link Consumer} that will call methods on {@link GetRemoteAccessSessionRequest.Builder} to create a
* request. Represents the request to get information about the specified remote access session.
* @return Result of the GetRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetRemoteAccessSession
* @see AWS API Documentation
*/
default GetRemoteAccessSessionResponse getRemoteAccessSession(
Consumer getRemoteAccessSessionRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getRemoteAccessSession(GetRemoteAccessSessionRequest.builder().applyMutation(getRemoteAccessSessionRequest)
.build());
}
/**
*
* Gets information about a run.
*
*
* @param getRunRequest
* Represents a request to the get run operation.
* @return Result of the GetRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetRun
* @see AWS API
* Documentation
*/
default GetRunResponse getRun(GetRunRequest getRunRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a run.
*
*
*
* This is a convenience which creates an instance of the {@link GetRunRequest.Builder} avoiding the need to create
* one manually via {@link GetRunRequest#builder()}
*
*
* @param getRunRequest
* A {@link Consumer} that will call methods on {@link GetRunRequest.Builder} to create a request. Represents
* a request to the get run operation.
* @return Result of the GetRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetRun
* @see AWS API
* Documentation
*/
default GetRunResponse getRun(Consumer getRunRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return getRun(GetRunRequest.builder().applyMutation(getRunRequest).build());
}
/**
*
* Gets information about a suite.
*
*
* @param getSuiteRequest
* Represents a request to the get suite operation.
* @return Result of the GetSuite operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetSuite
* @see AWS API
* Documentation
*/
default GetSuiteResponse getSuite(GetSuiteRequest getSuiteRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a suite.
*
*
*
* This is a convenience which creates an instance of the {@link GetSuiteRequest.Builder} avoiding the need to
* create one manually via {@link GetSuiteRequest#builder()}
*
*
* @param getSuiteRequest
* A {@link Consumer} that will call methods on {@link GetSuiteRequest.Builder} to create a request.
* Represents a request to the get suite operation.
* @return Result of the GetSuite operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetSuite
* @see AWS API
* Documentation
*/
default GetSuiteResponse getSuite(Consumer getSuiteRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getSuite(GetSuiteRequest.builder().applyMutation(getSuiteRequest).build());
}
/**
*
* Gets information about a test.
*
*
* @param getTestRequest
* Represents a request to the get test operation.
* @return Result of the GetTest operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetTest
* @see AWS API
* Documentation
*/
default GetTestResponse getTest(GetTestRequest getTestRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a test.
*
*
*
* This is a convenience which creates an instance of the {@link GetTestRequest.Builder} avoiding the need to create
* one manually via {@link GetTestRequest#builder()}
*
*
* @param getTestRequest
* A {@link Consumer} that will call methods on {@link GetTestRequest.Builder} to create a request.
* Represents a request to the get test operation.
* @return Result of the GetTest operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetTest
* @see AWS API
* Documentation
*/
default GetTestResponse getTest(Consumer getTestRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return getTest(GetTestRequest.builder().applyMutation(getTestRequest).build());
}
/**
*
* Retrieves information about a Selenium testing project.
*
*
* @param getTestGridProjectRequest
* @return Result of the GetTestGridProject operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetTestGridProject
* @see AWS
* API Documentation
*/
default GetTestGridProjectResponse getTestGridProject(GetTestGridProjectRequest getTestGridProjectRequest)
throws NotFoundException, ArgumentException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about a Selenium testing project.
*
*
*
* This is a convenience which creates an instance of the {@link GetTestGridProjectRequest.Builder} avoiding the
* need to create one manually via {@link GetTestGridProjectRequest#builder()}
*
*
* @param getTestGridProjectRequest
* A {@link Consumer} that will call methods on {@link GetTestGridProjectRequest.Builder} to create a
* request.
* @return Result of the GetTestGridProject operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetTestGridProject
* @see AWS
* API Documentation
*/
default GetTestGridProjectResponse getTestGridProject(Consumer getTestGridProjectRequest)
throws NotFoundException, ArgumentException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getTestGridProject(GetTestGridProjectRequest.builder().applyMutation(getTestGridProjectRequest).build());
}
/**
*
* A session is an instance of a browser created through a RemoteWebDriver
with the URL from
* CreateTestGridUrlResult$url. You can use the following to look up sessions:
*
*
* -
*
* The session ARN (GetTestGridSessionRequest$sessionArn).
*
*
* -
*
* The project ARN and a session ID (GetTestGridSessionRequest$projectArn and
* GetTestGridSessionRequest$sessionId).
*
*
*
*
*
* @param getTestGridSessionRequest
* @return Result of the GetTestGridSession operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetTestGridSession
* @see AWS
* API Documentation
*/
default GetTestGridSessionResponse getTestGridSession(GetTestGridSessionRequest getTestGridSessionRequest)
throws NotFoundException, ArgumentException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* A session is an instance of a browser created through a RemoteWebDriver
with the URL from
* CreateTestGridUrlResult$url. You can use the following to look up sessions:
*
*
* -
*
* The session ARN (GetTestGridSessionRequest$sessionArn).
*
*
* -
*
* The project ARN and a session ID (GetTestGridSessionRequest$projectArn and
* GetTestGridSessionRequest$sessionId).
*
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetTestGridSessionRequest.Builder} avoiding the
* need to create one manually via {@link GetTestGridSessionRequest#builder()}
*
*
* @param getTestGridSessionRequest
* A {@link Consumer} that will call methods on {@link GetTestGridSessionRequest.Builder} to create a
* request.
* @return Result of the GetTestGridSession operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetTestGridSession
* @see AWS
* API Documentation
*/
default GetTestGridSessionResponse getTestGridSession(Consumer getTestGridSessionRequest)
throws NotFoundException, ArgumentException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getTestGridSession(GetTestGridSessionRequest.builder().applyMutation(getTestGridSessionRequest).build());
}
/**
*
* Gets information about an upload.
*
*
* @param getUploadRequest
* Represents a request to the get upload operation.
* @return Result of the GetUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetUpload
* @see AWS API
* Documentation
*/
default GetUploadResponse getUpload(GetUploadRequest getUploadRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about an upload.
*
*
*
* This is a convenience which creates an instance of the {@link GetUploadRequest.Builder} avoiding the need to
* create one manually via {@link GetUploadRequest#builder()}
*
*
* @param getUploadRequest
* A {@link Consumer} that will call methods on {@link GetUploadRequest.Builder} to create a request.
* Represents a request to the get upload operation.
* @return Result of the GetUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetUpload
* @see AWS API
* Documentation
*/
default GetUploadResponse getUpload(Consumer getUploadRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return getUpload(GetUploadRequest.builder().applyMutation(getUploadRequest).build());
}
/**
*
* Returns information about the configuration settings for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param getVpceConfigurationRequest
* @return Result of the GetVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetVPCEConfiguration
* @see AWS API Documentation
*/
default GetVpceConfigurationResponse getVPCEConfiguration(GetVpceConfigurationRequest getVpceConfigurationRequest)
throws ArgumentException, NotFoundException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the configuration settings for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link GetVpceConfigurationRequest.Builder} avoiding the
* need to create one manually via {@link GetVpceConfigurationRequest#builder()}
*
*
* @param getVpceConfigurationRequest
* A {@link Consumer} that will call methods on {@link GetVPCEConfigurationRequest.Builder} to create a
* request.
* @return Result of the GetVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.GetVPCEConfiguration
* @see AWS API Documentation
*/
default GetVpceConfigurationResponse getVPCEConfiguration(
Consumer getVpceConfigurationRequest) throws ArgumentException,
NotFoundException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return getVPCEConfiguration(GetVpceConfigurationRequest.builder().applyMutation(getVpceConfigurationRequest).build());
}
/**
*
* Installs an application to the device in a remote access session. For Android applications, the file must be in
* .apk format. For iOS applications, the file must be in .ipa format.
*
*
* @param installToRemoteAccessSessionRequest
* Represents the request to install an Android application (in .apk format) or an iOS application (in .ipa
* format) as part of a remote access session.
* @return Result of the InstallToRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.InstallToRemoteAccessSession
* @see AWS API Documentation
*/
default InstallToRemoteAccessSessionResponse installToRemoteAccessSession(
InstallToRemoteAccessSessionRequest installToRemoteAccessSessionRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Installs an application to the device in a remote access session. For Android applications, the file must be in
* .apk format. For iOS applications, the file must be in .ipa format.
*
*
*
* This is a convenience which creates an instance of the {@link InstallToRemoteAccessSessionRequest.Builder}
* avoiding the need to create one manually via {@link InstallToRemoteAccessSessionRequest#builder()}
*
*
* @param installToRemoteAccessSessionRequest
* A {@link Consumer} that will call methods on {@link InstallToRemoteAccessSessionRequest.Builder} to create
* a request. Represents the request to install an Android application (in .apk format) or an iOS application
* (in .ipa format) as part of a remote access session.
* @return Result of the InstallToRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.InstallToRemoteAccessSession
* @see AWS API Documentation
*/
default InstallToRemoteAccessSessionResponse installToRemoteAccessSession(
Consumer installToRemoteAccessSessionRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return installToRemoteAccessSession(InstallToRemoteAccessSessionRequest.builder()
.applyMutation(installToRemoteAccessSessionRequest).build());
}
/**
*
* Gets information about artifacts.
*
*
* @param listArtifactsRequest
* Represents a request to the list artifacts operation.
* @return Result of the ListArtifacts operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListArtifacts
* @see AWS API
* Documentation
*/
default ListArtifactsResponse listArtifacts(ListArtifactsRequest listArtifactsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about artifacts.
*
*
*
* This is a convenience which creates an instance of the {@link ListArtifactsRequest.Builder} avoiding the need to
* create one manually via {@link ListArtifactsRequest#builder()}
*
*
* @param listArtifactsRequest
* A {@link Consumer} that will call methods on {@link ListArtifactsRequest.Builder} to create a request.
* Represents a request to the list artifacts operation.
* @return Result of the ListArtifacts operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListArtifacts
* @see AWS API
* Documentation
*/
default ListArtifactsResponse listArtifacts(Consumer listArtifactsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listArtifacts(ListArtifactsRequest.builder().applyMutation(listArtifactsRequest).build());
}
/**
*
* Gets information about artifacts.
*
*
*
* This is a variant of
* {@link #listArtifacts(software.amazon.awssdk.services.devicefarm.model.ListArtifactsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListArtifactsIterable responses = client.listArtifactsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListArtifactsIterable responses = client
* .listArtifactsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListArtifactsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListArtifactsIterable responses = client.listArtifactsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listArtifacts(software.amazon.awssdk.services.devicefarm.model.ListArtifactsRequest)} operation.
*
*
* @param listArtifactsRequest
* Represents a request to the list artifacts operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListArtifacts
* @see AWS API
* Documentation
*/
default ListArtifactsIterable listArtifactsPaginator(ListArtifactsRequest listArtifactsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about artifacts.
*
*
*
* This is a variant of
* {@link #listArtifacts(software.amazon.awssdk.services.devicefarm.model.ListArtifactsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListArtifactsIterable responses = client.listArtifactsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListArtifactsIterable responses = client
* .listArtifactsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListArtifactsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListArtifactsIterable responses = client.listArtifactsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listArtifacts(software.amazon.awssdk.services.devicefarm.model.ListArtifactsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListArtifactsRequest.Builder} avoiding the need to
* create one manually via {@link ListArtifactsRequest#builder()}
*
*
* @param listArtifactsRequest
* A {@link Consumer} that will call methods on {@link ListArtifactsRequest.Builder} to create a request.
* Represents a request to the list artifacts operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListArtifacts
* @see AWS API
* Documentation
*/
default ListArtifactsIterable listArtifactsPaginator(Consumer listArtifactsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listArtifactsPaginator(ListArtifactsRequest.builder().applyMutation(listArtifactsRequest).build());
}
/**
*
* Returns information about the private device instances associated with one or more AWS accounts.
*
*
* @return Result of the ListDeviceInstances operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDeviceInstances
* @see #listDeviceInstances(ListDeviceInstancesRequest)
* @see AWS
* API Documentation
*/
default ListDeviceInstancesResponse listDeviceInstances() throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listDeviceInstances(ListDeviceInstancesRequest.builder().build());
}
/**
*
* Returns information about the private device instances associated with one or more AWS accounts.
*
*
* @param listDeviceInstancesRequest
* @return Result of the ListDeviceInstances operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDeviceInstances
* @see AWS
* API Documentation
*/
default ListDeviceInstancesResponse listDeviceInstances(ListDeviceInstancesRequest listDeviceInstancesRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the private device instances associated with one or more AWS accounts.
*
*
*
* This is a convenience which creates an instance of the {@link ListDeviceInstancesRequest.Builder} avoiding the
* need to create one manually via {@link ListDeviceInstancesRequest#builder()}
*
*
* @param listDeviceInstancesRequest
* A {@link Consumer} that will call methods on {@link ListDeviceInstancesRequest.Builder} to create a
* request.
* @return Result of the ListDeviceInstances operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDeviceInstances
* @see AWS
* API Documentation
*/
default ListDeviceInstancesResponse listDeviceInstances(
Consumer listDeviceInstancesRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listDeviceInstances(ListDeviceInstancesRequest.builder().applyMutation(listDeviceInstancesRequest).build());
}
/**
*
* Gets information about device pools.
*
*
* @param listDevicePoolsRequest
* Represents the result of a list device pools request.
* @return Result of the ListDevicePools operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevicePools
* @see AWS API
* Documentation
*/
default ListDevicePoolsResponse listDevicePools(ListDevicePoolsRequest listDevicePoolsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about device pools.
*
*
*
* This is a convenience which creates an instance of the {@link ListDevicePoolsRequest.Builder} avoiding the need
* to create one manually via {@link ListDevicePoolsRequest#builder()}
*
*
* @param listDevicePoolsRequest
* A {@link Consumer} that will call methods on {@link ListDevicePoolsRequest.Builder} to create a request.
* Represents the result of a list device pools request.
* @return Result of the ListDevicePools operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevicePools
* @see AWS API
* Documentation
*/
default ListDevicePoolsResponse listDevicePools(Consumer listDevicePoolsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listDevicePools(ListDevicePoolsRequest.builder().applyMutation(listDevicePoolsRequest).build());
}
/**
*
* Gets information about device pools.
*
*
*
* This is a variant of
* {@link #listDevicePools(software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicePoolsIterable responses = client.listDevicePoolsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicePoolsIterable responses = client
* .listDevicePoolsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicePoolsIterable responses = client.listDevicePoolsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevicePools(software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsRequest)} operation.
*
*
* @param listDevicePoolsRequest
* Represents the result of a list device pools request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevicePools
* @see AWS API
* Documentation
*/
default ListDevicePoolsIterable listDevicePoolsPaginator(ListDevicePoolsRequest listDevicePoolsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about device pools.
*
*
*
* This is a variant of
* {@link #listDevicePools(software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicePoolsIterable responses = client.listDevicePoolsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicePoolsIterable responses = client
* .listDevicePoolsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicePoolsIterable responses = client.listDevicePoolsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevicePools(software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListDevicePoolsRequest.Builder} avoiding the need
* to create one manually via {@link ListDevicePoolsRequest#builder()}
*
*
* @param listDevicePoolsRequest
* A {@link Consumer} that will call methods on {@link ListDevicePoolsRequest.Builder} to create a request.
* Represents the result of a list device pools request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevicePools
* @see AWS API
* Documentation
*/
default ListDevicePoolsIterable listDevicePoolsPaginator(Consumer listDevicePoolsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listDevicePoolsPaginator(ListDevicePoolsRequest.builder().applyMutation(listDevicePoolsRequest).build());
}
/**
*
* Gets information about unique device types.
*
*
* @return Result of the ListDevices operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevices
* @see #listDevices(ListDevicesRequest)
* @see AWS API
* Documentation
*/
default ListDevicesResponse listDevices() throws ArgumentException, NotFoundException, LimitExceededException,
ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listDevices(ListDevicesRequest.builder().build());
}
/**
*
* Gets information about unique device types.
*
*
* @param listDevicesRequest
* Represents the result of a list devices request.
* @return Result of the ListDevices operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevices
* @see AWS API
* Documentation
*/
default ListDevicesResponse listDevices(ListDevicesRequest listDevicesRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about unique device types.
*
*
*
* This is a convenience which creates an instance of the {@link ListDevicesRequest.Builder} avoiding the need to
* create one manually via {@link ListDevicesRequest#builder()}
*
*
* @param listDevicesRequest
* A {@link Consumer} that will call methods on {@link ListDevicesRequest.Builder} to create a request.
* Represents the result of a list devices request.
* @return Result of the ListDevices operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevices
* @see AWS API
* Documentation
*/
default ListDevicesResponse listDevices(Consumer listDevicesRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listDevices(ListDevicesRequest.builder().applyMutation(listDevicesRequest).build());
}
/**
*
* Gets information about unique device types.
*
*
*
* This is a variant of {@link #listDevices(software.amazon.awssdk.services.devicefarm.model.ListDevicesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable responses = client.listDevicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable responses = client.listDevicesPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListDevicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable responses = client.listDevicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevices(software.amazon.awssdk.services.devicefarm.model.ListDevicesRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevices
* @see #listDevicesPaginator(ListDevicesRequest)
* @see AWS API
* Documentation
*/
default ListDevicesIterable listDevicesPaginator() throws ArgumentException, NotFoundException, LimitExceededException,
ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listDevicesPaginator(ListDevicesRequest.builder().build());
}
/**
*
* Gets information about unique device types.
*
*
*
* This is a variant of {@link #listDevices(software.amazon.awssdk.services.devicefarm.model.ListDevicesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable responses = client.listDevicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable responses = client.listDevicesPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListDevicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable responses = client.listDevicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevices(software.amazon.awssdk.services.devicefarm.model.ListDevicesRequest)} operation.
*
*
* @param listDevicesRequest
* Represents the result of a list devices request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevices
* @see AWS API
* Documentation
*/
default ListDevicesIterable listDevicesPaginator(ListDevicesRequest listDevicesRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about unique device types.
*
*
*
* This is a variant of {@link #listDevices(software.amazon.awssdk.services.devicefarm.model.ListDevicesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable responses = client.listDevicesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable responses = client.listDevicesPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListDevicesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListDevicesIterable responses = client.listDevicesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDevices(software.amazon.awssdk.services.devicefarm.model.ListDevicesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListDevicesRequest.Builder} avoiding the need to
* create one manually via {@link ListDevicesRequest#builder()}
*
*
* @param listDevicesRequest
* A {@link Consumer} that will call methods on {@link ListDevicesRequest.Builder} to create a request.
* Represents the result of a list devices request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListDevices
* @see AWS API
* Documentation
*/
default ListDevicesIterable listDevicesPaginator(Consumer listDevicesRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listDevicesPaginator(ListDevicesRequest.builder().applyMutation(listDevicesRequest).build());
}
/**
*
* Returns information about all the instance profiles in an AWS account.
*
*
* @return Result of the ListInstanceProfiles operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListInstanceProfiles
* @see #listInstanceProfiles(ListInstanceProfilesRequest)
* @see AWS API Documentation
*/
default ListInstanceProfilesResponse listInstanceProfiles() throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listInstanceProfiles(ListInstanceProfilesRequest.builder().build());
}
/**
*
* Returns information about all the instance profiles in an AWS account.
*
*
* @param listInstanceProfilesRequest
* @return Result of the ListInstanceProfiles operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListInstanceProfiles
* @see AWS API Documentation
*/
default ListInstanceProfilesResponse listInstanceProfiles(ListInstanceProfilesRequest listInstanceProfilesRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about all the instance profiles in an AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListInstanceProfilesRequest.Builder} avoiding the
* need to create one manually via {@link ListInstanceProfilesRequest#builder()}
*
*
* @param listInstanceProfilesRequest
* A {@link Consumer} that will call methods on {@link ListInstanceProfilesRequest.Builder} to create a
* request.
* @return Result of the ListInstanceProfiles operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListInstanceProfiles
* @see AWS API Documentation
*/
default ListInstanceProfilesResponse listInstanceProfiles(
Consumer listInstanceProfilesRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listInstanceProfiles(ListInstanceProfilesRequest.builder().applyMutation(listInstanceProfilesRequest).build());
}
/**
*
* Gets information about jobs for a given test run.
*
*
* @param listJobsRequest
* Represents a request to the list jobs operation.
* @return Result of the ListJobs operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListJobs
* @see AWS API
* Documentation
*/
default ListJobsResponse listJobs(ListJobsRequest listJobsRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about jobs for a given test run.
*
*
*
* This is a convenience which creates an instance of the {@link ListJobsRequest.Builder} avoiding the need to
* create one manually via {@link ListJobsRequest#builder()}
*
*
* @param listJobsRequest
* A {@link Consumer} that will call methods on {@link ListJobsRequest.Builder} to create a request.
* Represents a request to the list jobs operation.
* @return Result of the ListJobs operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListJobs
* @see AWS API
* Documentation
*/
default ListJobsResponse listJobs(Consumer listJobsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listJobs(ListJobsRequest.builder().applyMutation(listJobsRequest).build());
}
/**
*
* Gets information about jobs for a given test run.
*
*
*
* This is a variant of {@link #listJobs(software.amazon.awssdk.services.devicefarm.model.ListJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listJobs(software.amazon.awssdk.services.devicefarm.model.ListJobsRequest)} operation.
*
*
* @param listJobsRequest
* Represents a request to the list jobs operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListJobs
* @see AWS API
* Documentation
*/
default ListJobsIterable listJobsPaginator(ListJobsRequest listJobsRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about jobs for a given test run.
*
*
*
* This is a variant of {@link #listJobs(software.amazon.awssdk.services.devicefarm.model.ListJobsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListJobsIterable responses = client.listJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listJobs(software.amazon.awssdk.services.devicefarm.model.ListJobsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListJobsRequest.Builder} avoiding the need to
* create one manually via {@link ListJobsRequest#builder()}
*
*
* @param listJobsRequest
* A {@link Consumer} that will call methods on {@link ListJobsRequest.Builder} to create a request.
* Represents a request to the list jobs operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListJobs
* @see AWS API
* Documentation
*/
default ListJobsIterable listJobsPaginator(Consumer listJobsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listJobsPaginator(ListJobsRequest.builder().applyMutation(listJobsRequest).build());
}
/**
*
* Returns the list of available network profiles.
*
*
* @param listNetworkProfilesRequest
* @return Result of the ListNetworkProfiles operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListNetworkProfiles
* @see AWS
* API Documentation
*/
default ListNetworkProfilesResponse listNetworkProfiles(ListNetworkProfilesRequest listNetworkProfilesRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the list of available network profiles.
*
*
*
* This is a convenience which creates an instance of the {@link ListNetworkProfilesRequest.Builder} avoiding the
* need to create one manually via {@link ListNetworkProfilesRequest#builder()}
*
*
* @param listNetworkProfilesRequest
* A {@link Consumer} that will call methods on {@link ListNetworkProfilesRequest.Builder} to create a
* request.
* @return Result of the ListNetworkProfiles operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListNetworkProfiles
* @see AWS
* API Documentation
*/
default ListNetworkProfilesResponse listNetworkProfiles(
Consumer listNetworkProfilesRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listNetworkProfiles(ListNetworkProfilesRequest.builder().applyMutation(listNetworkProfilesRequest).build());
}
/**
*
* Returns a list of offering promotions. Each offering promotion record contains the ID and description of the
* promotion. The API returns a NotEligible
error if the caller is not permitted to invoke the
* operation. Contact [email protected] if
* you must be able to invoke this operation.
*
*
* @return Result of the ListOfferingPromotions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferingPromotions
* @see #listOfferingPromotions(ListOfferingPromotionsRequest)
* @see AWS API Documentation
*/
default ListOfferingPromotionsResponse listOfferingPromotions() throws ArgumentException, NotFoundException,
NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listOfferingPromotions(ListOfferingPromotionsRequest.builder().build());
}
/**
*
* Returns a list of offering promotions. Each offering promotion record contains the ID and description of the
* promotion. The API returns a NotEligible
error if the caller is not permitted to invoke the
* operation. Contact [email protected] if
* you must be able to invoke this operation.
*
*
* @param listOfferingPromotionsRequest
* @return Result of the ListOfferingPromotions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferingPromotions
* @see AWS API Documentation
*/
default ListOfferingPromotionsResponse listOfferingPromotions(ListOfferingPromotionsRequest listOfferingPromotionsRequest)
throws ArgumentException, NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException,
AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of offering promotions. Each offering promotion record contains the ID and description of the
* promotion. The API returns a NotEligible
error if the caller is not permitted to invoke the
* operation. Contact [email protected] if
* you must be able to invoke this operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListOfferingPromotionsRequest.Builder} avoiding the
* need to create one manually via {@link ListOfferingPromotionsRequest#builder()}
*
*
* @param listOfferingPromotionsRequest
* A {@link Consumer} that will call methods on {@link ListOfferingPromotionsRequest.Builder} to create a
* request.
* @return Result of the ListOfferingPromotions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferingPromotions
* @see AWS API Documentation
*/
default ListOfferingPromotionsResponse listOfferingPromotions(
Consumer listOfferingPromotionsRequest) throws ArgumentException,
NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listOfferingPromotions(ListOfferingPromotionsRequest.builder().applyMutation(listOfferingPromotionsRequest)
.build());
}
/**
*
* Returns a list of all historical purchases, renewals, and system renewal transactions for an AWS account. The
* list is paginated and ordered by a descending timestamp (most recent transactions are first). The API returns a
* NotEligible
error if the user is not permitted to invoke the operation. If you must be able to
* invoke this operation, contact [email protected].
*
*
* @return Result of the ListOfferingTransactions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferingTransactions
* @see #listOfferingTransactions(ListOfferingTransactionsRequest)
* @see AWS API Documentation
*/
default ListOfferingTransactionsResponse listOfferingTransactions() throws ArgumentException, NotFoundException,
NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listOfferingTransactions(ListOfferingTransactionsRequest.builder().build());
}
/**
*
* Returns a list of all historical purchases, renewals, and system renewal transactions for an AWS account. The
* list is paginated and ordered by a descending timestamp (most recent transactions are first). The API returns a
* NotEligible
error if the user is not permitted to invoke the operation. If you must be able to
* invoke this operation, contact [email protected].
*
*
* @param listOfferingTransactionsRequest
* Represents the request to list the offering transaction history.
* @return Result of the ListOfferingTransactions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferingTransactions
* @see AWS API Documentation
*/
default ListOfferingTransactionsResponse listOfferingTransactions(
ListOfferingTransactionsRequest listOfferingTransactionsRequest) throws ArgumentException, NotFoundException,
NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of all historical purchases, renewals, and system renewal transactions for an AWS account. The
* list is paginated and ordered by a descending timestamp (most recent transactions are first). The API returns a
* NotEligible
error if the user is not permitted to invoke the operation. If you must be able to
* invoke this operation, contact [email protected].
*
*
*
* This is a convenience which creates an instance of the {@link ListOfferingTransactionsRequest.Builder} avoiding
* the need to create one manually via {@link ListOfferingTransactionsRequest#builder()}
*
*
* @param listOfferingTransactionsRequest
* A {@link Consumer} that will call methods on {@link ListOfferingTransactionsRequest.Builder} to create a
* request. Represents the request to list the offering transaction history.
* @return Result of the ListOfferingTransactions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferingTransactions
* @see AWS API Documentation
*/
default ListOfferingTransactionsResponse listOfferingTransactions(
Consumer listOfferingTransactionsRequest) throws ArgumentException,
NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listOfferingTransactions(ListOfferingTransactionsRequest.builder().applyMutation(listOfferingTransactionsRequest)
.build());
}
/**
*
* Returns a list of all historical purchases, renewals, and system renewal transactions for an AWS account. The
* list is paginated and ordered by a descending timestamp (most recent transactions are first). The API returns a
* NotEligible
error if the user is not permitted to invoke the operation. If you must be able to
* invoke this operation, contact [email protected].
*
*
*
* This is a variant of
* {@link #listOfferingTransactions(software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable responses = client.listOfferingTransactionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable responses = client
* .listOfferingTransactionsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable responses = client.listOfferingTransactionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOfferingTransactions(software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsRequest)}
* operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferingTransactions
* @see #listOfferingTransactionsPaginator(ListOfferingTransactionsRequest)
* @see AWS API Documentation
*/
default ListOfferingTransactionsIterable listOfferingTransactionsPaginator() throws ArgumentException, NotFoundException,
NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listOfferingTransactionsPaginator(ListOfferingTransactionsRequest.builder().build());
}
/**
*
* Returns a list of all historical purchases, renewals, and system renewal transactions for an AWS account. The
* list is paginated and ordered by a descending timestamp (most recent transactions are first). The API returns a
* NotEligible
error if the user is not permitted to invoke the operation. If you must be able to
* invoke this operation, contact [email protected].
*
*
*
* This is a variant of
* {@link #listOfferingTransactions(software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable responses = client.listOfferingTransactionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable responses = client
* .listOfferingTransactionsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable responses = client.listOfferingTransactionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOfferingTransactions(software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsRequest)}
* operation.
*
*
* @param listOfferingTransactionsRequest
* Represents the request to list the offering transaction history.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferingTransactions
* @see AWS API Documentation
*/
default ListOfferingTransactionsIterable listOfferingTransactionsPaginator(
ListOfferingTransactionsRequest listOfferingTransactionsRequest) throws ArgumentException, NotFoundException,
NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of all historical purchases, renewals, and system renewal transactions for an AWS account. The
* list is paginated and ordered by a descending timestamp (most recent transactions are first). The API returns a
* NotEligible
error if the user is not permitted to invoke the operation. If you must be able to
* invoke this operation, contact [email protected].
*
*
*
* This is a variant of
* {@link #listOfferingTransactions(software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable responses = client.listOfferingTransactionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable responses = client
* .listOfferingTransactionsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingTransactionsIterable responses = client.listOfferingTransactionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOfferingTransactions(software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListOfferingTransactionsRequest.Builder} avoiding
* the need to create one manually via {@link ListOfferingTransactionsRequest#builder()}
*
*
* @param listOfferingTransactionsRequest
* A {@link Consumer} that will call methods on {@link ListOfferingTransactionsRequest.Builder} to create a
* request. Represents the request to list the offering transaction history.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferingTransactions
* @see AWS API Documentation
*/
default ListOfferingTransactionsIterable listOfferingTransactionsPaginator(
Consumer listOfferingTransactionsRequest) throws ArgumentException,
NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listOfferingTransactionsPaginator(ListOfferingTransactionsRequest.builder()
.applyMutation(listOfferingTransactionsRequest).build());
}
/**
*
* Returns a list of products or offerings that the user can manage through the API. Each offering record indicates
* the recurring price per unit and the frequency for that offering. The API returns a NotEligible
* error if the user is not permitted to invoke the operation. If you must be able to invoke this operation, contact
* [email protected].
*
*
* @return Result of the ListOfferings operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferings
* @see #listOfferings(ListOfferingsRequest)
* @see AWS API
* Documentation
*/
default ListOfferingsResponse listOfferings() throws ArgumentException, NotFoundException, NotEligibleException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listOfferings(ListOfferingsRequest.builder().build());
}
/**
*
* Returns a list of products or offerings that the user can manage through the API. Each offering record indicates
* the recurring price per unit and the frequency for that offering. The API returns a NotEligible
* error if the user is not permitted to invoke the operation. If you must be able to invoke this operation, contact
* [email protected].
*
*
* @param listOfferingsRequest
* Represents the request to list all offerings.
* @return Result of the ListOfferings operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferings
* @see AWS API
* Documentation
*/
default ListOfferingsResponse listOfferings(ListOfferingsRequest listOfferingsRequest) throws ArgumentException,
NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of products or offerings that the user can manage through the API. Each offering record indicates
* the recurring price per unit and the frequency for that offering. The API returns a NotEligible
* error if the user is not permitted to invoke the operation. If you must be able to invoke this operation, contact
* [email protected].
*
*
*
* This is a convenience which creates an instance of the {@link ListOfferingsRequest.Builder} avoiding the need to
* create one manually via {@link ListOfferingsRequest#builder()}
*
*
* @param listOfferingsRequest
* A {@link Consumer} that will call methods on {@link ListOfferingsRequest.Builder} to create a request.
* Represents the request to list all offerings.
* @return Result of the ListOfferings operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferings
* @see AWS API
* Documentation
*/
default ListOfferingsResponse listOfferings(Consumer listOfferingsRequest)
throws ArgumentException, NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException,
AwsServiceException, SdkClientException, DeviceFarmException {
return listOfferings(ListOfferingsRequest.builder().applyMutation(listOfferingsRequest).build());
}
/**
*
* Returns a list of products or offerings that the user can manage through the API. Each offering record indicates
* the recurring price per unit and the frequency for that offering. The API returns a NotEligible
* error if the user is not permitted to invoke the operation. If you must be able to invoke this operation, contact
* [email protected].
*
*
*
* This is a variant of
* {@link #listOfferings(software.amazon.awssdk.services.devicefarm.model.ListOfferingsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable responses = client.listOfferingsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable responses = client
* .listOfferingsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListOfferingsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable responses = client.listOfferingsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOfferings(software.amazon.awssdk.services.devicefarm.model.ListOfferingsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferings
* @see #listOfferingsPaginator(ListOfferingsRequest)
* @see AWS API
* Documentation
*/
default ListOfferingsIterable listOfferingsPaginator() throws ArgumentException, NotFoundException, NotEligibleException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listOfferingsPaginator(ListOfferingsRequest.builder().build());
}
/**
*
* Returns a list of products or offerings that the user can manage through the API. Each offering record indicates
* the recurring price per unit and the frequency for that offering. The API returns a NotEligible
* error if the user is not permitted to invoke the operation. If you must be able to invoke this operation, contact
* [email protected].
*
*
*
* This is a variant of
* {@link #listOfferings(software.amazon.awssdk.services.devicefarm.model.ListOfferingsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable responses = client.listOfferingsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable responses = client
* .listOfferingsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListOfferingsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable responses = client.listOfferingsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOfferings(software.amazon.awssdk.services.devicefarm.model.ListOfferingsRequest)} operation.
*
*
* @param listOfferingsRequest
* Represents the request to list all offerings.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferings
* @see AWS API
* Documentation
*/
default ListOfferingsIterable listOfferingsPaginator(ListOfferingsRequest listOfferingsRequest) throws ArgumentException,
NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of products or offerings that the user can manage through the API. Each offering record indicates
* the recurring price per unit and the frequency for that offering. The API returns a NotEligible
* error if the user is not permitted to invoke the operation. If you must be able to invoke this operation, contact
* [email protected].
*
*
*
* This is a variant of
* {@link #listOfferings(software.amazon.awssdk.services.devicefarm.model.ListOfferingsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable responses = client.listOfferingsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable responses = client
* .listOfferingsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListOfferingsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListOfferingsIterable responses = client.listOfferingsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOfferings(software.amazon.awssdk.services.devicefarm.model.ListOfferingsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListOfferingsRequest.Builder} avoiding the need to
* create one manually via {@link ListOfferingsRequest#builder()}
*
*
* @param listOfferingsRequest
* A {@link Consumer} that will call methods on {@link ListOfferingsRequest.Builder} to create a request.
* Represents the request to list all offerings.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListOfferings
* @see AWS API
* Documentation
*/
default ListOfferingsIterable listOfferingsPaginator(Consumer listOfferingsRequest)
throws ArgumentException, NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException,
AwsServiceException, SdkClientException, DeviceFarmException {
return listOfferingsPaginator(ListOfferingsRequest.builder().applyMutation(listOfferingsRequest).build());
}
/**
*
* Gets information about projects.
*
*
* @return Result of the ListProjects operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListProjects
* @see #listProjects(ListProjectsRequest)
* @see AWS API
* Documentation
*/
default ListProjectsResponse listProjects() throws ArgumentException, NotFoundException, LimitExceededException,
ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listProjects(ListProjectsRequest.builder().build());
}
/**
*
* Gets information about projects.
*
*
* @param listProjectsRequest
* Represents a request to the list projects operation.
* @return Result of the ListProjects operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsResponse listProjects(ListProjectsRequest listProjectsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about projects.
*
*
*
* This is a convenience which creates an instance of the {@link ListProjectsRequest.Builder} avoiding the need to
* create one manually via {@link ListProjectsRequest#builder()}
*
*
* @param listProjectsRequest
* A {@link Consumer} that will call methods on {@link ListProjectsRequest.Builder} to create a request.
* Represents a request to the list projects operation.
* @return Result of the ListProjects operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsResponse listProjects(Consumer listProjectsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listProjects(ListProjectsRequest.builder().applyMutation(listProjectsRequest).build());
}
/**
*
* Gets information about projects.
*
*
*
* This is a variant of {@link #listProjects(software.amazon.awssdk.services.devicefarm.model.ListProjectsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.devicefarm.model.ListProjectsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListProjects
* @see #listProjectsPaginator(ListProjectsRequest)
* @see AWS API
* Documentation
*/
default ListProjectsIterable listProjectsPaginator() throws ArgumentException, NotFoundException, LimitExceededException,
ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listProjectsPaginator(ListProjectsRequest.builder().build());
}
/**
*
* Gets information about projects.
*
*
*
* This is a variant of {@link #listProjects(software.amazon.awssdk.services.devicefarm.model.ListProjectsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.devicefarm.model.ListProjectsRequest)} operation.
*
*
* @param listProjectsRequest
* Represents a request to the list projects operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsIterable listProjectsPaginator(ListProjectsRequest listProjectsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about projects.
*
*
*
* This is a variant of {@link #listProjects(software.amazon.awssdk.services.devicefarm.model.ListProjectsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListProjectsIterable responses = client.listProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProjects(software.amazon.awssdk.services.devicefarm.model.ListProjectsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListProjectsRequest.Builder} avoiding the need to
* create one manually via {@link ListProjectsRequest#builder()}
*
*
* @param listProjectsRequest
* A {@link Consumer} that will call methods on {@link ListProjectsRequest.Builder} to create a request.
* Represents a request to the list projects operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListProjects
* @see AWS API
* Documentation
*/
default ListProjectsIterable listProjectsPaginator(Consumer listProjectsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listProjectsPaginator(ListProjectsRequest.builder().applyMutation(listProjectsRequest).build());
}
/**
*
* Returns a list of all currently running remote access sessions.
*
*
* @param listRemoteAccessSessionsRequest
* Represents the request to return information about the remote access session.
* @return Result of the ListRemoteAccessSessions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListRemoteAccessSessions
* @see AWS API Documentation
*/
default ListRemoteAccessSessionsResponse listRemoteAccessSessions(
ListRemoteAccessSessionsRequest listRemoteAccessSessionsRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of all currently running remote access sessions.
*
*
*
* This is a convenience which creates an instance of the {@link ListRemoteAccessSessionsRequest.Builder} avoiding
* the need to create one manually via {@link ListRemoteAccessSessionsRequest#builder()}
*
*
* @param listRemoteAccessSessionsRequest
* A {@link Consumer} that will call methods on {@link ListRemoteAccessSessionsRequest.Builder} to create a
* request. Represents the request to return information about the remote access session.
* @return Result of the ListRemoteAccessSessions operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListRemoteAccessSessions
* @see AWS API Documentation
*/
default ListRemoteAccessSessionsResponse listRemoteAccessSessions(
Consumer listRemoteAccessSessionsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listRemoteAccessSessions(ListRemoteAccessSessionsRequest.builder().applyMutation(listRemoteAccessSessionsRequest)
.build());
}
/**
*
* Gets information about runs, given an AWS Device Farm project ARN.
*
*
* @param listRunsRequest
* Represents a request to the list runs operation.
* @return Result of the ListRuns operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListRuns
* @see AWS API
* Documentation
*/
default ListRunsResponse listRuns(ListRunsRequest listRunsRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about runs, given an AWS Device Farm project ARN.
*
*
*
* This is a convenience which creates an instance of the {@link ListRunsRequest.Builder} avoiding the need to
* create one manually via {@link ListRunsRequest#builder()}
*
*
* @param listRunsRequest
* A {@link Consumer} that will call methods on {@link ListRunsRequest.Builder} to create a request.
* Represents a request to the list runs operation.
* @return Result of the ListRuns operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListRuns
* @see AWS API
* Documentation
*/
default ListRunsResponse listRuns(Consumer listRunsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listRuns(ListRunsRequest.builder().applyMutation(listRunsRequest).build());
}
/**
*
* Gets information about runs, given an AWS Device Farm project ARN.
*
*
*
* This is a variant of {@link #listRuns(software.amazon.awssdk.services.devicefarm.model.ListRunsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListRunsIterable responses = client.listRunsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListRunsIterable responses = client.listRunsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListRunsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListRunsIterable responses = client.listRunsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRuns(software.amazon.awssdk.services.devicefarm.model.ListRunsRequest)} operation.
*
*
* @param listRunsRequest
* Represents a request to the list runs operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListRuns
* @see AWS API
* Documentation
*/
default ListRunsIterable listRunsPaginator(ListRunsRequest listRunsRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about runs, given an AWS Device Farm project ARN.
*
*
*
* This is a variant of {@link #listRuns(software.amazon.awssdk.services.devicefarm.model.ListRunsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListRunsIterable responses = client.listRunsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListRunsIterable responses = client.listRunsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListRunsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListRunsIterable responses = client.listRunsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRuns(software.amazon.awssdk.services.devicefarm.model.ListRunsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListRunsRequest.Builder} avoiding the need to
* create one manually via {@link ListRunsRequest#builder()}
*
*
* @param listRunsRequest
* A {@link Consumer} that will call methods on {@link ListRunsRequest.Builder} to create a request.
* Represents a request to the list runs operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListRuns
* @see AWS API
* Documentation
*/
default ListRunsIterable listRunsPaginator(Consumer listRunsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listRunsPaginator(ListRunsRequest.builder().applyMutation(listRunsRequest).build());
}
/**
*
* Gets information about samples, given an AWS Device Farm job ARN.
*
*
* @param listSamplesRequest
* Represents a request to the list samples operation.
* @return Result of the ListSamples operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListSamples
* @see AWS API
* Documentation
*/
default ListSamplesResponse listSamples(ListSamplesRequest listSamplesRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about samples, given an AWS Device Farm job ARN.
*
*
*
* This is a convenience which creates an instance of the {@link ListSamplesRequest.Builder} avoiding the need to
* create one manually via {@link ListSamplesRequest#builder()}
*
*
* @param listSamplesRequest
* A {@link Consumer} that will call methods on {@link ListSamplesRequest.Builder} to create a request.
* Represents a request to the list samples operation.
* @return Result of the ListSamples operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListSamples
* @see AWS API
* Documentation
*/
default ListSamplesResponse listSamples(Consumer listSamplesRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listSamples(ListSamplesRequest.builder().applyMutation(listSamplesRequest).build());
}
/**
*
* Gets information about samples, given an AWS Device Farm job ARN.
*
*
*
* This is a variant of {@link #listSamples(software.amazon.awssdk.services.devicefarm.model.ListSamplesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListSamplesIterable responses = client.listSamplesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListSamplesIterable responses = client.listSamplesPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListSamplesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListSamplesIterable responses = client.listSamplesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSamples(software.amazon.awssdk.services.devicefarm.model.ListSamplesRequest)} operation.
*
*
* @param listSamplesRequest
* Represents a request to the list samples operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListSamples
* @see AWS API
* Documentation
*/
default ListSamplesIterable listSamplesPaginator(ListSamplesRequest listSamplesRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about samples, given an AWS Device Farm job ARN.
*
*
*
* This is a variant of {@link #listSamples(software.amazon.awssdk.services.devicefarm.model.ListSamplesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListSamplesIterable responses = client.listSamplesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListSamplesIterable responses = client.listSamplesPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListSamplesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListSamplesIterable responses = client.listSamplesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSamples(software.amazon.awssdk.services.devicefarm.model.ListSamplesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListSamplesRequest.Builder} avoiding the need to
* create one manually via {@link ListSamplesRequest#builder()}
*
*
* @param listSamplesRequest
* A {@link Consumer} that will call methods on {@link ListSamplesRequest.Builder} to create a request.
* Represents a request to the list samples operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListSamples
* @see AWS API
* Documentation
*/
default ListSamplesIterable listSamplesPaginator(Consumer listSamplesRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listSamplesPaginator(ListSamplesRequest.builder().applyMutation(listSamplesRequest).build());
}
/**
*
* Gets information about test suites for a given job.
*
*
* @param listSuitesRequest
* Represents a request to the list suites operation.
* @return Result of the ListSuites operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListSuites
* @see AWS API
* Documentation
*/
default ListSuitesResponse listSuites(ListSuitesRequest listSuitesRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about test suites for a given job.
*
*
*
* This is a convenience which creates an instance of the {@link ListSuitesRequest.Builder} avoiding the need to
* create one manually via {@link ListSuitesRequest#builder()}
*
*
* @param listSuitesRequest
* A {@link Consumer} that will call methods on {@link ListSuitesRequest.Builder} to create a request.
* Represents a request to the list suites operation.
* @return Result of the ListSuites operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListSuites
* @see AWS API
* Documentation
*/
default ListSuitesResponse listSuites(Consumer listSuitesRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listSuites(ListSuitesRequest.builder().applyMutation(listSuitesRequest).build());
}
/**
*
* Gets information about test suites for a given job.
*
*
*
* This is a variant of {@link #listSuites(software.amazon.awssdk.services.devicefarm.model.ListSuitesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListSuitesIterable responses = client.listSuitesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListSuitesIterable responses = client.listSuitesPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListSuitesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListSuitesIterable responses = client.listSuitesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSuites(software.amazon.awssdk.services.devicefarm.model.ListSuitesRequest)} operation.
*
*
* @param listSuitesRequest
* Represents a request to the list suites operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListSuites
* @see AWS API
* Documentation
*/
default ListSuitesIterable listSuitesPaginator(ListSuitesRequest listSuitesRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about test suites for a given job.
*
*
*
* This is a variant of {@link #listSuites(software.amazon.awssdk.services.devicefarm.model.ListSuitesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListSuitesIterable responses = client.listSuitesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListSuitesIterable responses = client.listSuitesPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListSuitesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListSuitesIterable responses = client.listSuitesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSuites(software.amazon.awssdk.services.devicefarm.model.ListSuitesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListSuitesRequest.Builder} avoiding the need to
* create one manually via {@link ListSuitesRequest#builder()}
*
*
* @param listSuitesRequest
* A {@link Consumer} that will call methods on {@link ListSuitesRequest.Builder} to create a request.
* Represents a request to the list suites operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListSuites
* @see AWS API
* Documentation
*/
default ListSuitesIterable listSuitesPaginator(Consumer listSuitesRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listSuitesPaginator(ListSuitesRequest.builder().applyMutation(listSuitesRequest).build());
}
/**
*
* List the tags for an AWS Device Farm resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws TagOperationException
* The operation was not successful. Try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ArgumentException, NotFoundException, TagOperationException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* List the tags for an AWS Device Farm resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws TagOperationException
* The operation was not successful. Try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws ArgumentException, NotFoundException,
TagOperationException, AwsServiceException, SdkClientException, DeviceFarmException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Gets a list of all Selenium testing projects in your account.
*
*
* @param listTestGridProjectsRequest
* @return Result of the ListTestGridProjects operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridProjects
* @see AWS API Documentation
*/
default ListTestGridProjectsResponse listTestGridProjects(ListTestGridProjectsRequest listTestGridProjectsRequest)
throws ArgumentException, InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of all Selenium testing projects in your account.
*
*
*
* This is a convenience which creates an instance of the {@link ListTestGridProjectsRequest.Builder} avoiding the
* need to create one manually via {@link ListTestGridProjectsRequest#builder()}
*
*
* @param listTestGridProjectsRequest
* A {@link Consumer} that will call methods on {@link ListTestGridProjectsRequest.Builder} to create a
* request.
* @return Result of the ListTestGridProjects operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridProjects
* @see AWS API Documentation
*/
default ListTestGridProjectsResponse listTestGridProjects(
Consumer listTestGridProjectsRequest) throws ArgumentException,
InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
return listTestGridProjects(ListTestGridProjectsRequest.builder().applyMutation(listTestGridProjectsRequest).build());
}
/**
*
* Gets a list of all Selenium testing projects in your account.
*
*
*
* This is a variant of
* {@link #listTestGridProjects(software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridProjectsIterable responses = client.listTestGridProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridProjectsIterable responses = client
* .listTestGridProjectsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridProjectsIterable responses = client.listTestGridProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResult won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTestGridProjects(software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsRequest)}
* operation.
*
*
* @param listTestGridProjectsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridProjects
* @see AWS API Documentation
*/
default ListTestGridProjectsIterable listTestGridProjectsPaginator(ListTestGridProjectsRequest listTestGridProjectsRequest)
throws ArgumentException, InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a list of all Selenium testing projects in your account.
*
*
*
* This is a variant of
* {@link #listTestGridProjects(software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridProjectsIterable responses = client.listTestGridProjectsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridProjectsIterable responses = client
* .listTestGridProjectsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridProjectsIterable responses = client.listTestGridProjectsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResult won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTestGridProjects(software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTestGridProjectsRequest.Builder} avoiding the
* need to create one manually via {@link ListTestGridProjectsRequest#builder()}
*
*
* @param listTestGridProjectsRequest
* A {@link Consumer} that will call methods on {@link ListTestGridProjectsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridProjects
* @see AWS API Documentation
*/
default ListTestGridProjectsIterable listTestGridProjectsPaginator(
Consumer listTestGridProjectsRequest) throws ArgumentException,
InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
return listTestGridProjectsPaginator(ListTestGridProjectsRequest.builder().applyMutation(listTestGridProjectsRequest)
.build());
}
/**
*
* Returns a list of the actions taken in a TestGridSession.
*
*
* @param listTestGridSessionActionsRequest
* @return Result of the ListTestGridSessionActions operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessionActions
* @see AWS API Documentation
*/
default ListTestGridSessionActionsResponse listTestGridSessionActions(
ListTestGridSessionActionsRequest listTestGridSessionActionsRequest) throws NotFoundException, ArgumentException,
InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the actions taken in a TestGridSession.
*
*
*
* This is a convenience which creates an instance of the {@link ListTestGridSessionActionsRequest.Builder} avoiding
* the need to create one manually via {@link ListTestGridSessionActionsRequest#builder()}
*
*
* @param listTestGridSessionActionsRequest
* A {@link Consumer} that will call methods on {@link ListTestGridSessionActionsRequest.Builder} to create a
* request.
* @return Result of the ListTestGridSessionActions operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessionActions
* @see AWS API Documentation
*/
default ListTestGridSessionActionsResponse listTestGridSessionActions(
Consumer listTestGridSessionActionsRequest) throws NotFoundException,
ArgumentException, InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
return listTestGridSessionActions(ListTestGridSessionActionsRequest.builder()
.applyMutation(listTestGridSessionActionsRequest).build());
}
/**
*
* Returns a list of the actions taken in a TestGridSession.
*
*
*
* This is a variant of
* {@link #listTestGridSessionActions(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionActionsIterable responses = client.listTestGridSessionActionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionActionsIterable responses = client
* .listTestGridSessionActionsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionActionsIterable responses = client.listTestGridSessionActionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResult won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTestGridSessionActions(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsRequest)}
* operation.
*
*
* @param listTestGridSessionActionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessionActions
* @see AWS API Documentation
*/
default ListTestGridSessionActionsIterable listTestGridSessionActionsPaginator(
ListTestGridSessionActionsRequest listTestGridSessionActionsRequest) throws NotFoundException, ArgumentException,
InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of the actions taken in a TestGridSession.
*
*
*
* This is a variant of
* {@link #listTestGridSessionActions(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionActionsIterable responses = client.listTestGridSessionActionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionActionsIterable responses = client
* .listTestGridSessionActionsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionActionsIterable responses = client.listTestGridSessionActionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResult won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTestGridSessionActions(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTestGridSessionActionsRequest.Builder} avoiding
* the need to create one manually via {@link ListTestGridSessionActionsRequest#builder()}
*
*
* @param listTestGridSessionActionsRequest
* A {@link Consumer} that will call methods on {@link ListTestGridSessionActionsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessionActions
* @see AWS API Documentation
*/
default ListTestGridSessionActionsIterable listTestGridSessionActionsPaginator(
Consumer listTestGridSessionActionsRequest) throws NotFoundException,
ArgumentException, InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
return listTestGridSessionActionsPaginator(ListTestGridSessionActionsRequest.builder()
.applyMutation(listTestGridSessionActionsRequest).build());
}
/**
*
* Retrieves a list of artifacts created during the session.
*
*
* @param listTestGridSessionArtifactsRequest
* @return Result of the ListTestGridSessionArtifacts operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessionArtifacts
* @see AWS API Documentation
*/
default ListTestGridSessionArtifactsResponse listTestGridSessionArtifacts(
ListTestGridSessionArtifactsRequest listTestGridSessionArtifactsRequest) throws NotFoundException, ArgumentException,
InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of artifacts created during the session.
*
*
*
* This is a convenience which creates an instance of the {@link ListTestGridSessionArtifactsRequest.Builder}
* avoiding the need to create one manually via {@link ListTestGridSessionArtifactsRequest#builder()}
*
*
* @param listTestGridSessionArtifactsRequest
* A {@link Consumer} that will call methods on {@link ListTestGridSessionArtifactsRequest.Builder} to create
* a request.
* @return Result of the ListTestGridSessionArtifacts operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessionArtifacts
* @see AWS API Documentation
*/
default ListTestGridSessionArtifactsResponse listTestGridSessionArtifacts(
Consumer listTestGridSessionArtifactsRequest) throws NotFoundException,
ArgumentException, InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
return listTestGridSessionArtifacts(ListTestGridSessionArtifactsRequest.builder()
.applyMutation(listTestGridSessionArtifactsRequest).build());
}
/**
*
* Retrieves a list of artifacts created during the session.
*
*
*
* This is a variant of
* {@link #listTestGridSessionArtifacts(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionArtifactsIterable responses = client.listTestGridSessionArtifactsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionArtifactsIterable responses = client
* .listTestGridSessionArtifactsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionArtifactsIterable responses = client.listTestGridSessionArtifactsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResult won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTestGridSessionArtifacts(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsRequest)}
* operation.
*
*
* @param listTestGridSessionArtifactsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessionArtifacts
* @see AWS API Documentation
*/
default ListTestGridSessionArtifactsIterable listTestGridSessionArtifactsPaginator(
ListTestGridSessionArtifactsRequest listTestGridSessionArtifactsRequest) throws NotFoundException, ArgumentException,
InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of artifacts created during the session.
*
*
*
* This is a variant of
* {@link #listTestGridSessionArtifacts(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionArtifactsIterable responses = client.listTestGridSessionArtifactsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionArtifactsIterable responses = client
* .listTestGridSessionArtifactsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionArtifactsIterable responses = client.listTestGridSessionArtifactsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResult won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTestGridSessionArtifacts(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTestGridSessionArtifactsRequest.Builder}
* avoiding the need to create one manually via {@link ListTestGridSessionArtifactsRequest#builder()}
*
*
* @param listTestGridSessionArtifactsRequest
* A {@link Consumer} that will call methods on {@link ListTestGridSessionArtifactsRequest.Builder} to create
* a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessionArtifacts
* @see AWS API Documentation
*/
default ListTestGridSessionArtifactsIterable listTestGridSessionArtifactsPaginator(
Consumer listTestGridSessionArtifactsRequest) throws NotFoundException,
ArgumentException, InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
return listTestGridSessionArtifactsPaginator(ListTestGridSessionArtifactsRequest.builder()
.applyMutation(listTestGridSessionArtifactsRequest).build());
}
/**
*
* Retrieves a list of sessions for a TestGridProject.
*
*
* @param listTestGridSessionsRequest
* @return Result of the ListTestGridSessions operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessions
* @see AWS API Documentation
*/
default ListTestGridSessionsResponse listTestGridSessions(ListTestGridSessionsRequest listTestGridSessionsRequest)
throws NotFoundException, ArgumentException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of sessions for a TestGridProject.
*
*
*
* This is a convenience which creates an instance of the {@link ListTestGridSessionsRequest.Builder} avoiding the
* need to create one manually via {@link ListTestGridSessionsRequest#builder()}
*
*
* @param listTestGridSessionsRequest
* A {@link Consumer} that will call methods on {@link ListTestGridSessionsRequest.Builder} to create a
* request.
* @return Result of the ListTestGridSessions operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessions
* @see AWS API Documentation
*/
default ListTestGridSessionsResponse listTestGridSessions(
Consumer listTestGridSessionsRequest) throws NotFoundException,
ArgumentException, InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
return listTestGridSessions(ListTestGridSessionsRequest.builder().applyMutation(listTestGridSessionsRequest).build());
}
/**
*
* Retrieves a list of sessions for a TestGridProject.
*
*
*
* This is a variant of
* {@link #listTestGridSessions(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionsIterable responses = client.listTestGridSessionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionsIterable responses = client
* .listTestGridSessionsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionsIterable responses = client.listTestGridSessionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResult won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTestGridSessions(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsRequest)}
* operation.
*
*
* @param listTestGridSessionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessions
* @see AWS API Documentation
*/
default ListTestGridSessionsIterable listTestGridSessionsPaginator(ListTestGridSessionsRequest listTestGridSessionsRequest)
throws NotFoundException, ArgumentException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of sessions for a TestGridProject.
*
*
*
* This is a variant of
* {@link #listTestGridSessions(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionsIterable responses = client.listTestGridSessionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionsIterable responses = client
* .listTestGridSessionsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestGridSessionsIterable responses = client.listTestGridSessionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResult won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTestGridSessions(software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTestGridSessionsRequest.Builder} avoiding the
* need to create one manually via {@link ListTestGridSessionsRequest#builder()}
*
*
* @param listTestGridSessionsRequest
* A {@link Consumer} that will call methods on {@link ListTestGridSessionsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTestGridSessions
* @see AWS API Documentation
*/
default ListTestGridSessionsIterable listTestGridSessionsPaginator(
Consumer listTestGridSessionsRequest) throws NotFoundException,
ArgumentException, InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
return listTestGridSessionsPaginator(ListTestGridSessionsRequest.builder().applyMutation(listTestGridSessionsRequest)
.build());
}
/**
*
* Gets information about tests in a given test suite.
*
*
* @param listTestsRequest
* Represents a request to the list tests operation.
* @return Result of the ListTests operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTests
* @see AWS API
* Documentation
*/
default ListTestsResponse listTests(ListTestsRequest listTestsRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about tests in a given test suite.
*
*
*
* This is a convenience which creates an instance of the {@link ListTestsRequest.Builder} avoiding the need to
* create one manually via {@link ListTestsRequest#builder()}
*
*
* @param listTestsRequest
* A {@link Consumer} that will call methods on {@link ListTestsRequest.Builder} to create a request.
* Represents a request to the list tests operation.
* @return Result of the ListTests operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTests
* @see AWS API
* Documentation
*/
default ListTestsResponse listTests(Consumer listTestsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listTests(ListTestsRequest.builder().applyMutation(listTestsRequest).build());
}
/**
*
* Gets information about tests in a given test suite.
*
*
*
* This is a variant of {@link #listTests(software.amazon.awssdk.services.devicefarm.model.ListTestsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestsIterable responses = client.listTestsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestsIterable responses = client.listTestsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestsIterable responses = client.listTestsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTests(software.amazon.awssdk.services.devicefarm.model.ListTestsRequest)} operation.
*
*
* @param listTestsRequest
* Represents a request to the list tests operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTests
* @see AWS API
* Documentation
*/
default ListTestsIterable listTestsPaginator(ListTestsRequest listTestsRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about tests in a given test suite.
*
*
*
* This is a variant of {@link #listTests(software.amazon.awssdk.services.devicefarm.model.ListTestsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestsIterable responses = client.listTestsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestsIterable responses = client.listTestsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListTestsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListTestsIterable responses = client.listTestsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTests(software.amazon.awssdk.services.devicefarm.model.ListTestsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListTestsRequest.Builder} avoiding the need to
* create one manually via {@link ListTestsRequest#builder()}
*
*
* @param listTestsRequest
* A {@link Consumer} that will call methods on {@link ListTestsRequest.Builder} to create a request.
* Represents a request to the list tests operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListTests
* @see AWS API
* Documentation
*/
default ListTestsIterable listTestsPaginator(Consumer listTestsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listTestsPaginator(ListTestsRequest.builder().applyMutation(listTestsRequest).build());
}
/**
*
* Gets information about unique problems, such as exceptions or crashes.
*
*
* Unique problems are defined as a single instance of an error across a run, job, or suite. For example, if a call
* in your application consistently raises an exception (OutOfBoundsException in MyActivity.java:386
),
* ListUniqueProblems
returns a single entry instead of many individual entries for that exception.
*
*
* @param listUniqueProblemsRequest
* Represents a request to the list unique problems operation.
* @return Result of the ListUniqueProblems operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListUniqueProblems
* @see AWS
* API Documentation
*/
default ListUniqueProblemsResponse listUniqueProblems(ListUniqueProblemsRequest listUniqueProblemsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about unique problems, such as exceptions or crashes.
*
*
* Unique problems are defined as a single instance of an error across a run, job, or suite. For example, if a call
* in your application consistently raises an exception (OutOfBoundsException in MyActivity.java:386
),
* ListUniqueProblems
returns a single entry instead of many individual entries for that exception.
*
*
*
* This is a convenience which creates an instance of the {@link ListUniqueProblemsRequest.Builder} avoiding the
* need to create one manually via {@link ListUniqueProblemsRequest#builder()}
*
*
* @param listUniqueProblemsRequest
* A {@link Consumer} that will call methods on {@link ListUniqueProblemsRequest.Builder} to create a
* request. Represents a request to the list unique problems operation.
* @return Result of the ListUniqueProblems operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListUniqueProblems
* @see AWS
* API Documentation
*/
default ListUniqueProblemsResponse listUniqueProblems(Consumer listUniqueProblemsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listUniqueProblems(ListUniqueProblemsRequest.builder().applyMutation(listUniqueProblemsRequest).build());
}
/**
*
* Gets information about unique problems, such as exceptions or crashes.
*
*
* Unique problems are defined as a single instance of an error across a run, job, or suite. For example, if a call
* in your application consistently raises an exception (OutOfBoundsException in MyActivity.java:386
),
* ListUniqueProblems
returns a single entry instead of many individual entries for that exception.
*
*
*
* This is a variant of
* {@link #listUniqueProblems(software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListUniqueProblemsIterable responses = client.listUniqueProblemsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListUniqueProblemsIterable responses = client
* .listUniqueProblemsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListUniqueProblemsIterable responses = client.listUniqueProblemsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUniqueProblems(software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsRequest)}
* operation.
*
*
* @param listUniqueProblemsRequest
* Represents a request to the list unique problems operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListUniqueProblems
* @see AWS
* API Documentation
*/
default ListUniqueProblemsIterable listUniqueProblemsPaginator(ListUniqueProblemsRequest listUniqueProblemsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about unique problems, such as exceptions or crashes.
*
*
* Unique problems are defined as a single instance of an error across a run, job, or suite. For example, if a call
* in your application consistently raises an exception (OutOfBoundsException in MyActivity.java:386
),
* ListUniqueProblems
returns a single entry instead of many individual entries for that exception.
*
*
*
* This is a variant of
* {@link #listUniqueProblems(software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListUniqueProblemsIterable responses = client.listUniqueProblemsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListUniqueProblemsIterable responses = client
* .listUniqueProblemsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListUniqueProblemsIterable responses = client.listUniqueProblemsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUniqueProblems(software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListUniqueProblemsRequest.Builder} avoiding the
* need to create one manually via {@link ListUniqueProblemsRequest#builder()}
*
*
* @param listUniqueProblemsRequest
* A {@link Consumer} that will call methods on {@link ListUniqueProblemsRequest.Builder} to create a
* request. Represents a request to the list unique problems operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListUniqueProblems
* @see AWS
* API Documentation
*/
default ListUniqueProblemsIterable listUniqueProblemsPaginator(
Consumer listUniqueProblemsRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listUniqueProblemsPaginator(ListUniqueProblemsRequest.builder().applyMutation(listUniqueProblemsRequest).build());
}
/**
*
* Gets information about uploads, given an AWS Device Farm project ARN.
*
*
* @param listUploadsRequest
* Represents a request to the list uploads operation.
* @return Result of the ListUploads operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListUploads
* @see AWS API
* Documentation
*/
default ListUploadsResponse listUploads(ListUploadsRequest listUploadsRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about uploads, given an AWS Device Farm project ARN.
*
*
*
* This is a convenience which creates an instance of the {@link ListUploadsRequest.Builder} avoiding the need to
* create one manually via {@link ListUploadsRequest#builder()}
*
*
* @param listUploadsRequest
* A {@link Consumer} that will call methods on {@link ListUploadsRequest.Builder} to create a request.
* Represents a request to the list uploads operation.
* @return Result of the ListUploads operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListUploads
* @see AWS API
* Documentation
*/
default ListUploadsResponse listUploads(Consumer listUploadsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return listUploads(ListUploadsRequest.builder().applyMutation(listUploadsRequest).build());
}
/**
*
* Gets information about uploads, given an AWS Device Farm project ARN.
*
*
*
* This is a variant of {@link #listUploads(software.amazon.awssdk.services.devicefarm.model.ListUploadsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListUploadsIterable responses = client.listUploadsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListUploadsIterable responses = client.listUploadsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListUploadsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListUploadsIterable responses = client.listUploadsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUploads(software.amazon.awssdk.services.devicefarm.model.ListUploadsRequest)} operation.
*
*
* @param listUploadsRequest
* Represents a request to the list uploads operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListUploads
* @see AWS API
* Documentation
*/
default ListUploadsIterable listUploadsPaginator(ListUploadsRequest listUploadsRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about uploads, given an AWS Device Farm project ARN.
*
*
*
* This is a variant of {@link #listUploads(software.amazon.awssdk.services.devicefarm.model.ListUploadsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListUploadsIterable responses = client.listUploadsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.devicefarm.paginators.ListUploadsIterable responses = client.listUploadsPaginator(request);
* for (software.amazon.awssdk.services.devicefarm.model.ListUploadsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.devicefarm.paginators.ListUploadsIterable responses = client.listUploadsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUploads(software.amazon.awssdk.services.devicefarm.model.ListUploadsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListUploadsRequest.Builder} avoiding the need to
* create one manually via {@link ListUploadsRequest#builder()}
*
*
* @param listUploadsRequest
* A {@link Consumer} that will call methods on {@link ListUploadsRequest.Builder} to create a request.
* Represents a request to the list uploads operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListUploads
* @see AWS API
* Documentation
*/
default ListUploadsIterable listUploadsPaginator(Consumer listUploadsRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return listUploadsPaginator(ListUploadsRequest.builder().applyMutation(listUploadsRequest).build());
}
/**
*
* Returns information about all Amazon Virtual Private Cloud (VPC) endpoint configurations in the AWS account.
*
*
* @param listVpceConfigurationsRequest
* @return Result of the ListVPCEConfigurations operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListVPCEConfigurations
* @see AWS API Documentation
*/
default ListVpceConfigurationsResponse listVPCEConfigurations(ListVpceConfigurationsRequest listVpceConfigurationsRequest)
throws ArgumentException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about all Amazon Virtual Private Cloud (VPC) endpoint configurations in the AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListVpceConfigurationsRequest.Builder} avoiding the
* need to create one manually via {@link ListVpceConfigurationsRequest#builder()}
*
*
* @param listVpceConfigurationsRequest
* A {@link Consumer} that will call methods on {@link ListVPCEConfigurationsRequest.Builder} to create a
* request.
* @return Result of the ListVPCEConfigurations operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ListVPCEConfigurations
* @see AWS API Documentation
*/
default ListVpceConfigurationsResponse listVPCEConfigurations(
Consumer listVpceConfigurationsRequest) throws ArgumentException,
ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return listVPCEConfigurations(ListVpceConfigurationsRequest.builder().applyMutation(listVpceConfigurationsRequest)
.build());
}
/**
*
* Immediately purchases offerings for an AWS account. Offerings renew with the latest total purchased quantity for
* an offering, unless the renewal was overridden. The API returns a NotEligible
error if the user is
* not permitted to invoke the operation. If you must be able to invoke this operation, contact [email protected].
*
*
* @param purchaseOfferingRequest
* Represents a request for a purchase offering.
* @return Result of the PurchaseOffering operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.PurchaseOffering
* @see AWS
* API Documentation
*/
default PurchaseOfferingResponse purchaseOffering(PurchaseOfferingRequest purchaseOfferingRequest) throws ArgumentException,
NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Immediately purchases offerings for an AWS account. Offerings renew with the latest total purchased quantity for
* an offering, unless the renewal was overridden. The API returns a NotEligible
error if the user is
* not permitted to invoke the operation. If you must be able to invoke this operation, contact [email protected].
*
*
*
* This is a convenience which creates an instance of the {@link PurchaseOfferingRequest.Builder} avoiding the need
* to create one manually via {@link PurchaseOfferingRequest#builder()}
*
*
* @param purchaseOfferingRequest
* A {@link Consumer} that will call methods on {@link PurchaseOfferingRequest.Builder} to create a request.
* Represents a request for a purchase offering.
* @return Result of the PurchaseOffering operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.PurchaseOffering
* @see AWS
* API Documentation
*/
default PurchaseOfferingResponse purchaseOffering(Consumer purchaseOfferingRequest)
throws ArgumentException, NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException,
AwsServiceException, SdkClientException, DeviceFarmException {
return purchaseOffering(PurchaseOfferingRequest.builder().applyMutation(purchaseOfferingRequest).build());
}
/**
*
* Explicitly sets the quantity of devices to renew for an offering, starting from the effectiveDate
of
* the next period. The API returns a NotEligible
error if the user is not permitted to invoke the
* operation. If you must be able to invoke this operation, contact [email protected].
*
*
* @param renewOfferingRequest
* A request that represents an offering renewal.
* @return Result of the RenewOffering operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.RenewOffering
* @see AWS API
* Documentation
*/
default RenewOfferingResponse renewOffering(RenewOfferingRequest renewOfferingRequest) throws ArgumentException,
NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Explicitly sets the quantity of devices to renew for an offering, starting from the effectiveDate
of
* the next period. The API returns a NotEligible
error if the user is not permitted to invoke the
* operation. If you must be able to invoke this operation, contact [email protected].
*
*
*
* This is a convenience which creates an instance of the {@link RenewOfferingRequest.Builder} avoiding the need to
* create one manually via {@link RenewOfferingRequest#builder()}
*
*
* @param renewOfferingRequest
* A {@link Consumer} that will call methods on {@link RenewOfferingRequest.Builder} to create a request. A
* request that represents an offering renewal.
* @return Result of the RenewOffering operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws NotEligibleException
* Exception gets thrown when a user is not eligible to perform the specified transaction.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.RenewOffering
* @see AWS API
* Documentation
*/
default RenewOfferingResponse renewOffering(Consumer renewOfferingRequest)
throws ArgumentException, NotFoundException, NotEligibleException, LimitExceededException, ServiceAccountException,
AwsServiceException, SdkClientException, DeviceFarmException {
return renewOffering(RenewOfferingRequest.builder().applyMutation(renewOfferingRequest).build());
}
/**
*
* Schedules a run.
*
*
* @param scheduleRunRequest
* Represents a request to the schedule run operation.
* @return Result of the ScheduleRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws IdempotencyException
* An entity with the same name already exists.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ScheduleRun
* @see AWS API
* Documentation
*/
default ScheduleRunResponse scheduleRun(ScheduleRunRequest scheduleRunRequest) throws ArgumentException, NotFoundException,
LimitExceededException, IdempotencyException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Schedules a run.
*
*
*
* This is a convenience which creates an instance of the {@link ScheduleRunRequest.Builder} avoiding the need to
* create one manually via {@link ScheduleRunRequest#builder()}
*
*
* @param scheduleRunRequest
* A {@link Consumer} that will call methods on {@link ScheduleRunRequest.Builder} to create a request.
* Represents a request to the schedule run operation.
* @return Result of the ScheduleRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws IdempotencyException
* An entity with the same name already exists.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.ScheduleRun
* @see AWS API
* Documentation
*/
default ScheduleRunResponse scheduleRun(Consumer scheduleRunRequest) throws ArgumentException,
NotFoundException, LimitExceededException, IdempotencyException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return scheduleRun(ScheduleRunRequest.builder().applyMutation(scheduleRunRequest).build());
}
/**
*
* Initiates a stop request for the current job. AWS Device Farm immediately stops the job on the device where tests
* have not started. You are not billed for this device. On the device where tests have started, setup suite and
* teardown suite tests run to completion on the device. You are billed for setup, teardown, and any tests that were
* in progress or already completed.
*
*
* @param stopJobRequest
* @return Result of the StopJob operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.StopJob
* @see AWS API
* Documentation
*/
default StopJobResponse stopJob(StopJobRequest stopJobRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Initiates a stop request for the current job. AWS Device Farm immediately stops the job on the device where tests
* have not started. You are not billed for this device. On the device where tests have started, setup suite and
* teardown suite tests run to completion on the device. You are billed for setup, teardown, and any tests that were
* in progress or already completed.
*
*
*
* This is a convenience which creates an instance of the {@link StopJobRequest.Builder} avoiding the need to create
* one manually via {@link StopJobRequest#builder()}
*
*
* @param stopJobRequest
* A {@link Consumer} that will call methods on {@link StopJobRequest.Builder} to create a request.
* @return Result of the StopJob operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.StopJob
* @see AWS API
* Documentation
*/
default StopJobResponse stopJob(Consumer stopJobRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return stopJob(StopJobRequest.builder().applyMutation(stopJobRequest).build());
}
/**
*
* Ends a specified remote access session.
*
*
* @param stopRemoteAccessSessionRequest
* Represents the request to stop the remote access session.
* @return Result of the StopRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.StopRemoteAccessSession
* @see AWS API Documentation
*/
default StopRemoteAccessSessionResponse stopRemoteAccessSession(StopRemoteAccessSessionRequest stopRemoteAccessSessionRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Ends a specified remote access session.
*
*
*
* This is a convenience which creates an instance of the {@link StopRemoteAccessSessionRequest.Builder} avoiding
* the need to create one manually via {@link StopRemoteAccessSessionRequest#builder()}
*
*
* @param stopRemoteAccessSessionRequest
* A {@link Consumer} that will call methods on {@link StopRemoteAccessSessionRequest.Builder} to create a
* request. Represents the request to stop the remote access session.
* @return Result of the StopRemoteAccessSession operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.StopRemoteAccessSession
* @see AWS API Documentation
*/
default StopRemoteAccessSessionResponse stopRemoteAccessSession(
Consumer stopRemoteAccessSessionRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return stopRemoteAccessSession(StopRemoteAccessSessionRequest.builder().applyMutation(stopRemoteAccessSessionRequest)
.build());
}
/**
*
* Initiates a stop request for the current test run. AWS Device Farm immediately stops the run on devices where
* tests have not started. You are not billed for these devices. On devices where tests have started executing,
* setup suite and teardown suite tests run to completion on those devices. You are billed for setup, teardown, and
* any tests that were in progress or already completed.
*
*
* @param stopRunRequest
* Represents the request to stop a specific run.
* @return Result of the StopRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.StopRun
* @see AWS API
* Documentation
*/
default StopRunResponse stopRun(StopRunRequest stopRunRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Initiates a stop request for the current test run. AWS Device Farm immediately stops the run on devices where
* tests have not started. You are not billed for these devices. On devices where tests have started executing,
* setup suite and teardown suite tests run to completion on those devices. You are billed for setup, teardown, and
* any tests that were in progress or already completed.
*
*
*
* This is a convenience which creates an instance of the {@link StopRunRequest.Builder} avoiding the need to create
* one manually via {@link StopRunRequest#builder()}
*
*
* @param stopRunRequest
* A {@link Consumer} that will call methods on {@link StopRunRequest.Builder} to create a request.
* Represents the request to stop a specific run.
* @return Result of the StopRun operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.StopRun
* @see AWS API
* Documentation
*/
default StopRunResponse stopRun(Consumer stopRunRequest) throws ArgumentException, NotFoundException,
LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException, DeviceFarmException {
return stopRun(StopRunRequest.builder().applyMutation(stopRunRequest).build());
}
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource are not specified in the request parameters, they are not changed. When a resource is deleted, the tags
* associated with that resource are also deleted.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws TagOperationException
* The operation was not successful. Try again.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws TagPolicyException
* The request doesn't comply with the AWS Identity and Access Management (IAM) tag policy. Correct your
* request and then retry it.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ArgumentException, NotFoundException,
TagOperationException, TooManyTagsException, TagPolicyException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource are not specified in the request parameters, they are not changed. When a resource is deleted, the tags
* associated with that resource are also deleted.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws TagOperationException
* The operation was not successful. Try again.
* @throws TooManyTagsException
* The list of tags on the repository is over the limit. The maximum number of tags that can be applied to a
* repository is 50.
* @throws TagPolicyException
* The request doesn't comply with the AWS Identity and Access Management (IAM) tag policy. Correct your
* request and then retry it.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest) throws ArgumentException,
NotFoundException, TagOperationException, TooManyTagsException, TagPolicyException, AwsServiceException,
SdkClientException, DeviceFarmException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Deletes the specified tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws TagOperationException
* The operation was not successful. Try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ArgumentException,
NotFoundException, TagOperationException, AwsServiceException, SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified tags from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws TagOperationException
* The operation was not successful. Try again.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws ArgumentException, NotFoundException, TagOperationException, AwsServiceException, SdkClientException,
DeviceFarmException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates information about a private device instance.
*
*
* @param updateDeviceInstanceRequest
* @return Result of the UpdateDeviceInstance operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateDeviceInstance
* @see AWS API Documentation
*/
default UpdateDeviceInstanceResponse updateDeviceInstance(UpdateDeviceInstanceRequest updateDeviceInstanceRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Updates information about a private device instance.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDeviceInstanceRequest.Builder} avoiding the
* need to create one manually via {@link UpdateDeviceInstanceRequest#builder()}
*
*
* @param updateDeviceInstanceRequest
* A {@link Consumer} that will call methods on {@link UpdateDeviceInstanceRequest.Builder} to create a
* request.
* @return Result of the UpdateDeviceInstance operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateDeviceInstance
* @see AWS API Documentation
*/
default UpdateDeviceInstanceResponse updateDeviceInstance(
Consumer updateDeviceInstanceRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return updateDeviceInstance(UpdateDeviceInstanceRequest.builder().applyMutation(updateDeviceInstanceRequest).build());
}
/**
*
* Modifies the name, description, and rules in a device pool given the attributes and the pool ARN. Rule updates
* are all-or-nothing, meaning they can only be updated as a whole (or not at all).
*
*
* @param updateDevicePoolRequest
* Represents a request to the update device pool operation.
* @return Result of the UpdateDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateDevicePool
* @see AWS
* API Documentation
*/
default UpdateDevicePoolResponse updateDevicePool(UpdateDevicePoolRequest updateDevicePoolRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the name, description, and rules in a device pool given the attributes and the pool ARN. Rule updates
* are all-or-nothing, meaning they can only be updated as a whole (or not at all).
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDevicePoolRequest.Builder} avoiding the need
* to create one manually via {@link UpdateDevicePoolRequest#builder()}
*
*
* @param updateDevicePoolRequest
* A {@link Consumer} that will call methods on {@link UpdateDevicePoolRequest.Builder} to create a request.
* Represents a request to the update device pool operation.
* @return Result of the UpdateDevicePool operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateDevicePool
* @see AWS
* API Documentation
*/
default UpdateDevicePoolResponse updateDevicePool(Consumer updateDevicePoolRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return updateDevicePool(UpdateDevicePoolRequest.builder().applyMutation(updateDevicePoolRequest).build());
}
/**
*
* Updates information about an existing private device instance profile.
*
*
* @param updateInstanceProfileRequest
* @return Result of the UpdateInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateInstanceProfile
* @see AWS API Documentation
*/
default UpdateInstanceProfileResponse updateInstanceProfile(UpdateInstanceProfileRequest updateInstanceProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Updates information about an existing private device instance profile.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateInstanceProfileRequest.Builder} avoiding the
* need to create one manually via {@link UpdateInstanceProfileRequest#builder()}
*
*
* @param updateInstanceProfileRequest
* A {@link Consumer} that will call methods on {@link UpdateInstanceProfileRequest.Builder} to create a
* request.
* @return Result of the UpdateInstanceProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateInstanceProfile
* @see AWS API Documentation
*/
default UpdateInstanceProfileResponse updateInstanceProfile(
Consumer updateInstanceProfileRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return updateInstanceProfile(UpdateInstanceProfileRequest.builder().applyMutation(updateInstanceProfileRequest).build());
}
/**
*
* Updates the network profile.
*
*
* @param updateNetworkProfileRequest
* @return Result of the UpdateNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateNetworkProfile
* @see AWS API Documentation
*/
default UpdateNetworkProfileResponse updateNetworkProfile(UpdateNetworkProfileRequest updateNetworkProfileRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the network profile.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateNetworkProfileRequest.Builder} avoiding the
* need to create one manually via {@link UpdateNetworkProfileRequest#builder()}
*
*
* @param updateNetworkProfileRequest
* A {@link Consumer} that will call methods on {@link UpdateNetworkProfileRequest.Builder} to create a
* request.
* @return Result of the UpdateNetworkProfile operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateNetworkProfile
* @see AWS API Documentation
*/
default UpdateNetworkProfileResponse updateNetworkProfile(
Consumer updateNetworkProfileRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
return updateNetworkProfile(UpdateNetworkProfileRequest.builder().applyMutation(updateNetworkProfileRequest).build());
}
/**
*
* Modifies the specified project name, given the project ARN and a new name.
*
*
* @param updateProjectRequest
* Represents a request to the update project operation.
* @return Result of the UpdateProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateProject
* @see AWS API
* Documentation
*/
default UpdateProjectResponse updateProject(UpdateProjectRequest updateProjectRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the specified project name, given the project ARN and a new name.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateProjectRequest.Builder} avoiding the need to
* create one manually via {@link UpdateProjectRequest#builder()}
*
*
* @param updateProjectRequest
* A {@link Consumer} that will call methods on {@link UpdateProjectRequest.Builder} to create a request.
* Represents a request to the update project operation.
* @return Result of the UpdateProject operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateProject
* @see AWS API
* Documentation
*/
default UpdateProjectResponse updateProject(Consumer updateProjectRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return updateProject(UpdateProjectRequest.builder().applyMutation(updateProjectRequest).build());
}
/**
*
* Change details of a project.
*
*
* @param updateTestGridProjectRequest
* @return Result of the UpdateTestGridProject operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateTestGridProject
* @see AWS API Documentation
*/
default UpdateTestGridProjectResponse updateTestGridProject(UpdateTestGridProjectRequest updateTestGridProjectRequest)
throws NotFoundException, ArgumentException, InternalServiceException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Change details of a project.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateTestGridProjectRequest.Builder} avoiding the
* need to create one manually via {@link UpdateTestGridProjectRequest#builder()}
*
*
* @param updateTestGridProjectRequest
* A {@link Consumer} that will call methods on {@link UpdateTestGridProjectRequest.Builder} to create a
* request.
* @return Result of the UpdateTestGridProject operation returned by the service.
* @throws NotFoundException
* The specified entity was not found.
* @throws ArgumentException
* An invalid argument was specified.
* @throws InternalServiceException
* An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateTestGridProject
* @see AWS API Documentation
*/
default UpdateTestGridProjectResponse updateTestGridProject(
Consumer updateTestGridProjectRequest) throws NotFoundException,
ArgumentException, InternalServiceException, AwsServiceException, SdkClientException, DeviceFarmException {
return updateTestGridProject(UpdateTestGridProjectRequest.builder().applyMutation(updateTestGridProjectRequest).build());
}
/**
*
* Updates an uploaded test spec.
*
*
* @param updateUploadRequest
* @return Result of the UpdateUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateUpload
* @see AWS API
* Documentation
*/
default UpdateUploadResponse updateUpload(UpdateUploadRequest updateUploadRequest) throws ArgumentException,
NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException, SdkClientException,
DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an uploaded test spec.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUploadRequest.Builder} avoiding the need to
* create one manually via {@link UpdateUploadRequest#builder()}
*
*
* @param updateUploadRequest
* A {@link Consumer} that will call methods on {@link UpdateUploadRequest.Builder} to create a request.
* @return Result of the UpdateUpload operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws LimitExceededException
* A limit was exceeded.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateUpload
* @see AWS API
* Documentation
*/
default UpdateUploadResponse updateUpload(Consumer updateUploadRequest)
throws ArgumentException, NotFoundException, LimitExceededException, ServiceAccountException, AwsServiceException,
SdkClientException, DeviceFarmException {
return updateUpload(UpdateUploadRequest.builder().applyMutation(updateUploadRequest).build());
}
/**
*
* Updates information about an Amazon Virtual Private Cloud (VPC) endpoint configuration.
*
*
* @param updateVpceConfigurationRequest
* @return Result of the UpdateVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws InvalidOperationException
* There was an error with the update request, or you do not have sufficient permissions to update this VPC
* endpoint configuration.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateVPCEConfiguration
* @see AWS API Documentation
*/
default UpdateVpceConfigurationResponse updateVPCEConfiguration(UpdateVpceConfigurationRequest updateVpceConfigurationRequest)
throws ArgumentException, NotFoundException, ServiceAccountException, InvalidOperationException, AwsServiceException,
SdkClientException, DeviceFarmException {
throw new UnsupportedOperationException();
}
/**
*
* Updates information about an Amazon Virtual Private Cloud (VPC) endpoint configuration.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateVpceConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link UpdateVpceConfigurationRequest#builder()}
*
*
* @param updateVpceConfigurationRequest
* A {@link Consumer} that will call methods on {@link UpdateVPCEConfigurationRequest.Builder} to create a
* request.
* @return Result of the UpdateVPCEConfiguration operation returned by the service.
* @throws ArgumentException
* An invalid argument was specified.
* @throws NotFoundException
* The specified entity was not found.
* @throws ServiceAccountException
* There was a problem with the service account.
* @throws InvalidOperationException
* There was an error with the update request, or you do not have sufficient permissions to update this VPC
* endpoint configuration.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DeviceFarmException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DeviceFarmClient.UpdateVPCEConfiguration
* @see AWS API Documentation
*/
default UpdateVpceConfigurationResponse updateVPCEConfiguration(
Consumer updateVpceConfigurationRequest) throws ArgumentException,
NotFoundException, ServiceAccountException, InvalidOperationException, AwsServiceException, SdkClientException,
DeviceFarmException {
return updateVPCEConfiguration(UpdateVpceConfigurationRequest.builder().applyMutation(updateVpceConfigurationRequest)
.build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("devicefarm");
}
}