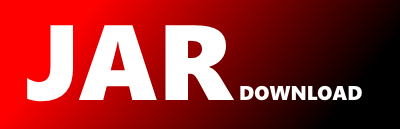
software.amazon.awssdk.services.devicefarm.model.Device Maven / Gradle / Ivy
Show all versions of devicefarm Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a device type that an app is tested against.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Device implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(Device::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(Device::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField MANUFACTURER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("manufacturer").getter(getter(Device::manufacturer)).setter(setter(Builder::manufacturer))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("manufacturer").build()).build();
private static final SdkField MODEL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("model")
.getter(getter(Device::model)).setter(setter(Builder::model))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("model").build()).build();
private static final SdkField MODEL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("modelId").getter(getter(Device::modelId)).setter(setter(Builder::modelId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("modelId").build()).build();
private static final SdkField FORM_FACTOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("formFactor").getter(getter(Device::formFactorAsString)).setter(setter(Builder::formFactor))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("formFactor").build()).build();
private static final SdkField PLATFORM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platform").getter(getter(Device::platformAsString)).setter(setter(Builder::platform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platform").build()).build();
private static final SdkField OS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("os")
.getter(getter(Device::os)).setter(setter(Builder::os))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("os").build()).build();
private static final SdkField CPU_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("cpu")
.getter(getter(Device::cpu)).setter(setter(Builder::cpu)).constructor(CPU::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cpu").build()).build();
private static final SdkField RESOLUTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("resolution").getter(getter(Device::resolution)).setter(setter(Builder::resolution))
.constructor(Resolution::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resolution").build()).build();
private static final SdkField HEAP_SIZE_FIELD = SdkField. builder(MarshallingType.LONG).memberName("heapSize")
.getter(getter(Device::heapSize)).setter(setter(Builder::heapSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("heapSize").build()).build();
private static final SdkField MEMORY_FIELD = SdkField. builder(MarshallingType.LONG).memberName("memory")
.getter(getter(Device::memory)).setter(setter(Builder::memory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memory").build()).build();
private static final SdkField IMAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("image")
.getter(getter(Device::image)).setter(setter(Builder::image))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("image").build()).build();
private static final SdkField CARRIER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("carrier")
.getter(getter(Device::carrier)).setter(setter(Builder::carrier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("carrier").build()).build();
private static final SdkField RADIO_FIELD = SdkField. builder(MarshallingType.STRING).memberName("radio")
.getter(getter(Device::radio)).setter(setter(Builder::radio))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("radio").build()).build();
private static final SdkField REMOTE_ACCESS_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("remoteAccessEnabled").getter(getter(Device::remoteAccessEnabled))
.setter(setter(Builder::remoteAccessEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteAccessEnabled").build())
.build();
private static final SdkField REMOTE_DEBUG_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("remoteDebugEnabled").getter(getter(Device::remoteDebugEnabled))
.setter(setter(Builder::remoteDebugEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteDebugEnabled").build())
.build();
private static final SdkField FLEET_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("fleetType").getter(getter(Device::fleetType)).setter(setter(Builder::fleetType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fleetType").build()).build();
private static final SdkField FLEET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("fleetName").getter(getter(Device::fleetName)).setter(setter(Builder::fleetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fleetName").build()).build();
private static final SdkField> INSTANCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("instances")
.getter(getter(Device::instances))
.setter(setter(Builder::instances))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("instances").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DeviceInstance::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField AVAILABILITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("availability").getter(getter(Device::availabilityAsString)).setter(setter(Builder::availability))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("availability").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, NAME_FIELD,
MANUFACTURER_FIELD, MODEL_FIELD, MODEL_ID_FIELD, FORM_FACTOR_FIELD, PLATFORM_FIELD, OS_FIELD, CPU_FIELD,
RESOLUTION_FIELD, HEAP_SIZE_FIELD, MEMORY_FIELD, IMAGE_FIELD, CARRIER_FIELD, RADIO_FIELD,
REMOTE_ACCESS_ENABLED_FIELD, REMOTE_DEBUG_ENABLED_FIELD, FLEET_TYPE_FIELD, FLEET_NAME_FIELD, INSTANCES_FIELD,
AVAILABILITY_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String name;
private final String manufacturer;
private final String model;
private final String modelId;
private final String formFactor;
private final String platform;
private final String os;
private final CPU cpu;
private final Resolution resolution;
private final Long heapSize;
private final Long memory;
private final String image;
private final String carrier;
private final String radio;
private final Boolean remoteAccessEnabled;
private final Boolean remoteDebugEnabled;
private final String fleetType;
private final String fleetName;
private final List instances;
private final String availability;
private Device(BuilderImpl builder) {
this.arn = builder.arn;
this.name = builder.name;
this.manufacturer = builder.manufacturer;
this.model = builder.model;
this.modelId = builder.modelId;
this.formFactor = builder.formFactor;
this.platform = builder.platform;
this.os = builder.os;
this.cpu = builder.cpu;
this.resolution = builder.resolution;
this.heapSize = builder.heapSize;
this.memory = builder.memory;
this.image = builder.image;
this.carrier = builder.carrier;
this.radio = builder.radio;
this.remoteAccessEnabled = builder.remoteAccessEnabled;
this.remoteDebugEnabled = builder.remoteDebugEnabled;
this.fleetType = builder.fleetType;
this.fleetName = builder.fleetName;
this.instances = builder.instances;
this.availability = builder.availability;
}
/**
*
* The device's ARN.
*
*
* @return The device's ARN.
*/
public final String arn() {
return arn;
}
/**
*
* The device's display name.
*
*
* @return The device's display name.
*/
public final String name() {
return name;
}
/**
*
* The device's manufacturer name.
*
*
* @return The device's manufacturer name.
*/
public final String manufacturer() {
return manufacturer;
}
/**
*
* The device's model name.
*
*
* @return The device's model name.
*/
public final String model() {
return model;
}
/**
*
* The device's model ID.
*
*
* @return The device's model ID.
*/
public final String modelId() {
return modelId;
}
/**
*
* The device's form factor.
*
*
* Allowed values include:
*
*
* -
*
* PHONE
*
*
* -
*
* TABLET
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #formFactor} will
* return {@link DeviceFormFactor#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #formFactorAsString}.
*
*
* @return The device's form factor.
*
* Allowed values include:
*
*
* -
*
* PHONE
*
*
* -
*
* TABLET
*
*
* @see DeviceFormFactor
*/
public final DeviceFormFactor formFactor() {
return DeviceFormFactor.fromValue(formFactor);
}
/**
*
* The device's form factor.
*
*
* Allowed values include:
*
*
* -
*
* PHONE
*
*
* -
*
* TABLET
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #formFactor} will
* return {@link DeviceFormFactor#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #formFactorAsString}.
*
*
* @return The device's form factor.
*
* Allowed values include:
*
*
* -
*
* PHONE
*
*
* -
*
* TABLET
*
*
* @see DeviceFormFactor
*/
public final String formFactorAsString() {
return formFactor;
}
/**
*
* The device's platform.
*
*
* Allowed values include:
*
*
* -
*
* ANDROID
*
*
* -
*
* IOS
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #platform} will
* return {@link DevicePlatform#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #platformAsString}.
*
*
* @return The device's platform.
*
* Allowed values include:
*
*
* -
*
* ANDROID
*
*
* -
*
* IOS
*
*
* @see DevicePlatform
*/
public final DevicePlatform platform() {
return DevicePlatform.fromValue(platform);
}
/**
*
* The device's platform.
*
*
* Allowed values include:
*
*
* -
*
* ANDROID
*
*
* -
*
* IOS
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #platform} will
* return {@link DevicePlatform#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #platformAsString}.
*
*
* @return The device's platform.
*
* Allowed values include:
*
*
* -
*
* ANDROID
*
*
* -
*
* IOS
*
*
* @see DevicePlatform
*/
public final String platformAsString() {
return platform;
}
/**
*
* The device's operating system type.
*
*
* @return The device's operating system type.
*/
public final String os() {
return os;
}
/**
*
* Information about the device's CPU.
*
*
* @return Information about the device's CPU.
*/
public final CPU cpu() {
return cpu;
}
/**
*
* The resolution of the device.
*
*
* @return The resolution of the device.
*/
public final Resolution resolution() {
return resolution;
}
/**
*
* The device's heap size, expressed in bytes.
*
*
* @return The device's heap size, expressed in bytes.
*/
public final Long heapSize() {
return heapSize;
}
/**
*
* The device's total memory size, expressed in bytes.
*
*
* @return The device's total memory size, expressed in bytes.
*/
public final Long memory() {
return memory;
}
/**
*
* The device's image name.
*
*
* @return The device's image name.
*/
public final String image() {
return image;
}
/**
*
* The device's carrier.
*
*
* @return The device's carrier.
*/
public final String carrier() {
return carrier;
}
/**
*
* The device's radio.
*
*
* @return The device's radio.
*/
public final String radio() {
return radio;
}
/**
*
* Specifies whether remote access has been enabled for the specified device.
*
*
* @return Specifies whether remote access has been enabled for the specified device.
*/
public final Boolean remoteAccessEnabled() {
return remoteAccessEnabled;
}
/**
*
* This flag is set to true
if remote debugging is enabled for the device.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return This flag is set to true
if remote debugging is enabled for the device.
*
* Remote debugging is no longer supported.
*/
public final Boolean remoteDebugEnabled() {
return remoteDebugEnabled;
}
/**
*
* The type of fleet to which this device belongs. Possible values are PRIVATE and PUBLIC.
*
*
* @return The type of fleet to which this device belongs. Possible values are PRIVATE and PUBLIC.
*/
public final String fleetType() {
return fleetType;
}
/**
*
* The name of the fleet to which this device belongs.
*
*
* @return The name of the fleet to which this device belongs.
*/
public final String fleetName() {
return fleetName;
}
/**
* For responses, this returns true if the service returned a value for the Instances property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasInstances() {
return instances != null && !(instances instanceof SdkAutoConstructList);
}
/**
*
* The instances that belong to this device.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInstances} method.
*
*
* @return The instances that belong to this device.
*/
public final List instances() {
return instances;
}
/**
*
* Indicates how likely a device is available for a test run. Currently available in the ListDevices and
* GetDevice API methods.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #availability} will
* return {@link DeviceAvailability#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #availabilityAsString}.
*
*
* @return Indicates how likely a device is available for a test run. Currently available in the ListDevices
* and GetDevice API methods.
* @see DeviceAvailability
*/
public final DeviceAvailability availability() {
return DeviceAvailability.fromValue(availability);
}
/**
*
* Indicates how likely a device is available for a test run. Currently available in the ListDevices and
* GetDevice API methods.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #availability} will
* return {@link DeviceAvailability#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #availabilityAsString}.
*
*
* @return Indicates how likely a device is available for a test run. Currently available in the ListDevices
* and GetDevice API methods.
* @see DeviceAvailability
*/
public final String availabilityAsString() {
return availability;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(manufacturer());
hashCode = 31 * hashCode + Objects.hashCode(model());
hashCode = 31 * hashCode + Objects.hashCode(modelId());
hashCode = 31 * hashCode + Objects.hashCode(formFactorAsString());
hashCode = 31 * hashCode + Objects.hashCode(platformAsString());
hashCode = 31 * hashCode + Objects.hashCode(os());
hashCode = 31 * hashCode + Objects.hashCode(cpu());
hashCode = 31 * hashCode + Objects.hashCode(resolution());
hashCode = 31 * hashCode + Objects.hashCode(heapSize());
hashCode = 31 * hashCode + Objects.hashCode(memory());
hashCode = 31 * hashCode + Objects.hashCode(image());
hashCode = 31 * hashCode + Objects.hashCode(carrier());
hashCode = 31 * hashCode + Objects.hashCode(radio());
hashCode = 31 * hashCode + Objects.hashCode(remoteAccessEnabled());
hashCode = 31 * hashCode + Objects.hashCode(remoteDebugEnabled());
hashCode = 31 * hashCode + Objects.hashCode(fleetType());
hashCode = 31 * hashCode + Objects.hashCode(fleetName());
hashCode = 31 * hashCode + Objects.hashCode(hasInstances() ? instances() : null);
hashCode = 31 * hashCode + Objects.hashCode(availabilityAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Device)) {
return false;
}
Device other = (Device) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(manufacturer(), other.manufacturer()) && Objects.equals(model(), other.model())
&& Objects.equals(modelId(), other.modelId()) && Objects.equals(formFactorAsString(), other.formFactorAsString())
&& Objects.equals(platformAsString(), other.platformAsString()) && Objects.equals(os(), other.os())
&& Objects.equals(cpu(), other.cpu()) && Objects.equals(resolution(), other.resolution())
&& Objects.equals(heapSize(), other.heapSize()) && Objects.equals(memory(), other.memory())
&& Objects.equals(image(), other.image()) && Objects.equals(carrier(), other.carrier())
&& Objects.equals(radio(), other.radio()) && Objects.equals(remoteAccessEnabled(), other.remoteAccessEnabled())
&& Objects.equals(remoteDebugEnabled(), other.remoteDebugEnabled())
&& Objects.equals(fleetType(), other.fleetType()) && Objects.equals(fleetName(), other.fleetName())
&& hasInstances() == other.hasInstances() && Objects.equals(instances(), other.instances())
&& Objects.equals(availabilityAsString(), other.availabilityAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Device").add("Arn", arn()).add("Name", name()).add("Manufacturer", manufacturer())
.add("Model", model()).add("ModelId", modelId()).add("FormFactor", formFactorAsString())
.add("Platform", platformAsString()).add("Os", os()).add("Cpu", cpu()).add("Resolution", resolution())
.add("HeapSize", heapSize()).add("Memory", memory()).add("Image", image()).add("Carrier", carrier())
.add("Radio", radio()).add("RemoteAccessEnabled", remoteAccessEnabled())
.add("RemoteDebugEnabled", remoteDebugEnabled()).add("FleetType", fleetType()).add("FleetName", fleetName())
.add("Instances", hasInstances() ? instances() : null).add("Availability", availabilityAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "manufacturer":
return Optional.ofNullable(clazz.cast(manufacturer()));
case "model":
return Optional.ofNullable(clazz.cast(model()));
case "modelId":
return Optional.ofNullable(clazz.cast(modelId()));
case "formFactor":
return Optional.ofNullable(clazz.cast(formFactorAsString()));
case "platform":
return Optional.ofNullable(clazz.cast(platformAsString()));
case "os":
return Optional.ofNullable(clazz.cast(os()));
case "cpu":
return Optional.ofNullable(clazz.cast(cpu()));
case "resolution":
return Optional.ofNullable(clazz.cast(resolution()));
case "heapSize":
return Optional.ofNullable(clazz.cast(heapSize()));
case "memory":
return Optional.ofNullable(clazz.cast(memory()));
case "image":
return Optional.ofNullable(clazz.cast(image()));
case "carrier":
return Optional.ofNullable(clazz.cast(carrier()));
case "radio":
return Optional.ofNullable(clazz.cast(radio()));
case "remoteAccessEnabled":
return Optional.ofNullable(clazz.cast(remoteAccessEnabled()));
case "remoteDebugEnabled":
return Optional.ofNullable(clazz.cast(remoteDebugEnabled()));
case "fleetType":
return Optional.ofNullable(clazz.cast(fleetType()));
case "fleetName":
return Optional.ofNullable(clazz.cast(fleetName()));
case "instances":
return Optional.ofNullable(clazz.cast(instances()));
case "availability":
return Optional.ofNullable(clazz.cast(availabilityAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function