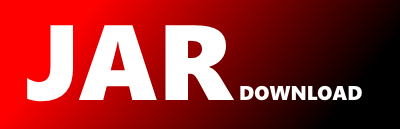
software.amazon.awssdk.services.devicefarm.model.Upload Maven / Gradle / Ivy
Show all versions of devicefarm Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.beans.Transient;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An app or a set of one or more tests to upload or that have been uploaded.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Upload implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(Upload::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(Upload::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("created").getter(getter(Upload::created)).setter(setter(Builder::created))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("created").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(Upload::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(Upload::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField URL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("url")
.getter(getter(Upload::url)).setter(setter(Builder::url))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("url").build()).build();
private static final SdkField METADATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("metadata").getter(getter(Upload::metadata)).setter(setter(Builder::metadata))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("metadata").build()).build();
private static final SdkField CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("contentType").getter(getter(Upload::contentType)).setter(setter(Builder::contentType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("contentType").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("message")
.getter(getter(Upload::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("message").build()).build();
private static final SdkField CATEGORY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("category").getter(getter(Upload::categoryAsString)).setter(setter(Builder::category))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("category").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays
.asList(ARN_FIELD, NAME_FIELD, CREATED_FIELD, TYPE_FIELD, STATUS_FIELD, URL_FIELD, METADATA_FIELD,
CONTENT_TYPE_FIELD, MESSAGE_FIELD, CATEGORY_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String name;
private final Instant created;
private final String type;
private final String status;
private final String url;
private final String metadata;
private final String contentType;
private final String message;
private final String category;
private Upload(BuilderImpl builder) {
this.arn = builder.arn;
this.name = builder.name;
this.created = builder.created;
this.type = builder.type;
this.status = builder.status;
this.url = builder.url;
this.metadata = builder.metadata;
this.contentType = builder.contentType;
this.message = builder.message;
this.category = builder.category;
}
/**
*
* The upload's ARN.
*
*
* @return The upload's ARN.
*/
public final String arn() {
return arn;
}
/**
*
* The upload's file name.
*
*
* @return The upload's file name.
*/
public final String name() {
return name;
}
/**
*
* When the upload was created.
*
*
* @return When the upload was created.
*/
public final Instant created() {
return created;
}
/**
*
* The upload's type.
*
*
* Must be one of the following values:
*
*
* -
*
* ANDROID_APP
*
*
* -
*
* IOS_APP
*
*
* -
*
* WEB_APP
*
*
* -
*
* EXTERNAL_DATA
*
*
* -
*
* APPIUM_JAVA_JUNIT_TEST_PACKAGE
*
*
* -
*
* APPIUM_JAVA_TESTNG_TEST_PACKAGE
*
*
* -
*
* APPIUM_PYTHON_TEST_PACKAGE
*
*
* -
*
* APPIUM_NODE_TEST_PACKAGE
*
*
* -
*
* APPIUM_RUBY_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_PYTHON_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_NODE_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_RUBY_TEST_PACKAGE
*
*
* -
*
* CALABASH_TEST_PACKAGE
*
*
* -
*
* INSTRUMENTATION_TEST_PACKAGE
*
*
* -
*
* UIAUTOMATION_TEST_PACKAGE
*
*
* -
*
* UIAUTOMATOR_TEST_PACKAGE
*
*
* -
*
* XCTEST_TEST_PACKAGE
*
*
* -
*
* XCTEST_UI_TEST_PACKAGE
*
*
* -
*
* APPIUM_JAVA_JUNIT_TEST_SPEC
*
*
* -
*
* APPIUM_JAVA_TESTNG_TEST_SPEC
*
*
* -
*
* APPIUM_PYTHON_TEST_SPEC
*
*
* -
*
* APPIUM_NODE_TEST_SPEC
*
*
* -
*
* APPIUM_RUBY_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_PYTHON_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_NODE_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_RUBY_TEST_SPEC
*
*
* -
*
* INSTRUMENTATION_TEST_SPEC
*
*
* -
*
* XCTEST_UI_TEST_SPEC
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link UploadType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The upload's type.
*
* Must be one of the following values:
*
*
* -
*
* ANDROID_APP
*
*
* -
*
* IOS_APP
*
*
* -
*
* WEB_APP
*
*
* -
*
* EXTERNAL_DATA
*
*
* -
*
* APPIUM_JAVA_JUNIT_TEST_PACKAGE
*
*
* -
*
* APPIUM_JAVA_TESTNG_TEST_PACKAGE
*
*
* -
*
* APPIUM_PYTHON_TEST_PACKAGE
*
*
* -
*
* APPIUM_NODE_TEST_PACKAGE
*
*
* -
*
* APPIUM_RUBY_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_PYTHON_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_NODE_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_RUBY_TEST_PACKAGE
*
*
* -
*
* CALABASH_TEST_PACKAGE
*
*
* -
*
* INSTRUMENTATION_TEST_PACKAGE
*
*
* -
*
* UIAUTOMATION_TEST_PACKAGE
*
*
* -
*
* UIAUTOMATOR_TEST_PACKAGE
*
*
* -
*
* XCTEST_TEST_PACKAGE
*
*
* -
*
* XCTEST_UI_TEST_PACKAGE
*
*
* -
*
* APPIUM_JAVA_JUNIT_TEST_SPEC
*
*
* -
*
* APPIUM_JAVA_TESTNG_TEST_SPEC
*
*
* -
*
* APPIUM_PYTHON_TEST_SPEC
*
*
* -
*
* APPIUM_NODE_TEST_SPEC
*
*
* -
*
* APPIUM_RUBY_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_PYTHON_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_NODE_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_RUBY_TEST_SPEC
*
*
* -
*
* INSTRUMENTATION_TEST_SPEC
*
*
* -
*
* XCTEST_UI_TEST_SPEC
*
*
* @see UploadType
*/
public final UploadType type() {
return UploadType.fromValue(type);
}
/**
*
* The upload's type.
*
*
* Must be one of the following values:
*
*
* -
*
* ANDROID_APP
*
*
* -
*
* IOS_APP
*
*
* -
*
* WEB_APP
*
*
* -
*
* EXTERNAL_DATA
*
*
* -
*
* APPIUM_JAVA_JUNIT_TEST_PACKAGE
*
*
* -
*
* APPIUM_JAVA_TESTNG_TEST_PACKAGE
*
*
* -
*
* APPIUM_PYTHON_TEST_PACKAGE
*
*
* -
*
* APPIUM_NODE_TEST_PACKAGE
*
*
* -
*
* APPIUM_RUBY_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_PYTHON_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_NODE_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_RUBY_TEST_PACKAGE
*
*
* -
*
* CALABASH_TEST_PACKAGE
*
*
* -
*
* INSTRUMENTATION_TEST_PACKAGE
*
*
* -
*
* UIAUTOMATION_TEST_PACKAGE
*
*
* -
*
* UIAUTOMATOR_TEST_PACKAGE
*
*
* -
*
* XCTEST_TEST_PACKAGE
*
*
* -
*
* XCTEST_UI_TEST_PACKAGE
*
*
* -
*
* APPIUM_JAVA_JUNIT_TEST_SPEC
*
*
* -
*
* APPIUM_JAVA_TESTNG_TEST_SPEC
*
*
* -
*
* APPIUM_PYTHON_TEST_SPEC
*
*
* -
*
* APPIUM_NODE_TEST_SPEC
*
*
* -
*
* APPIUM_RUBY_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_PYTHON_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_NODE_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_RUBY_TEST_SPEC
*
*
* -
*
* INSTRUMENTATION_TEST_SPEC
*
*
* -
*
* XCTEST_UI_TEST_SPEC
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link UploadType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The upload's type.
*
* Must be one of the following values:
*
*
* -
*
* ANDROID_APP
*
*
* -
*
* IOS_APP
*
*
* -
*
* WEB_APP
*
*
* -
*
* EXTERNAL_DATA
*
*
* -
*
* APPIUM_JAVA_JUNIT_TEST_PACKAGE
*
*
* -
*
* APPIUM_JAVA_TESTNG_TEST_PACKAGE
*
*
* -
*
* APPIUM_PYTHON_TEST_PACKAGE
*
*
* -
*
* APPIUM_NODE_TEST_PACKAGE
*
*
* -
*
* APPIUM_RUBY_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_PYTHON_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_NODE_TEST_PACKAGE
*
*
* -
*
* APPIUM_WEB_RUBY_TEST_PACKAGE
*
*
* -
*
* CALABASH_TEST_PACKAGE
*
*
* -
*
* INSTRUMENTATION_TEST_PACKAGE
*
*
* -
*
* UIAUTOMATION_TEST_PACKAGE
*
*
* -
*
* UIAUTOMATOR_TEST_PACKAGE
*
*
* -
*
* XCTEST_TEST_PACKAGE
*
*
* -
*
* XCTEST_UI_TEST_PACKAGE
*
*
* -
*
* APPIUM_JAVA_JUNIT_TEST_SPEC
*
*
* -
*
* APPIUM_JAVA_TESTNG_TEST_SPEC
*
*
* -
*
* APPIUM_PYTHON_TEST_SPEC
*
*
* -
*
* APPIUM_NODE_TEST_SPEC
*
*
* -
*
* APPIUM_RUBY_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_PYTHON_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_NODE_TEST_SPEC
*
*
* -
*
* APPIUM_WEB_RUBY_TEST_SPEC
*
*
* -
*
* INSTRUMENTATION_TEST_SPEC
*
*
* -
*
* XCTEST_UI_TEST_SPEC
*
*
* @see UploadType
*/
public final String typeAsString() {
return type;
}
/**
*
* The upload's status.
*
*
* Must be one of the following values:
*
*
* -
*
* FAILED
*
*
* -
*
* INITIALIZED
*
*
* -
*
* PROCESSING
*
*
* -
*
* SUCCEEDED
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link UploadStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The upload's status.
*
* Must be one of the following values:
*
*
* -
*
* FAILED
*
*
* -
*
* INITIALIZED
*
*
* -
*
* PROCESSING
*
*
* -
*
* SUCCEEDED
*
*
* @see UploadStatus
*/
public final UploadStatus status() {
return UploadStatus.fromValue(status);
}
/**
*
* The upload's status.
*
*
* Must be one of the following values:
*
*
* -
*
* FAILED
*
*
* -
*
* INITIALIZED
*
*
* -
*
* PROCESSING
*
*
* -
*
* SUCCEEDED
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link UploadStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The upload's status.
*
* Must be one of the following values:
*
*
* -
*
* FAILED
*
*
* -
*
* INITIALIZED
*
*
* -
*
* PROCESSING
*
*
* -
*
* SUCCEEDED
*
*
* @see UploadStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The presigned Amazon S3 URL that was used to store a file using a PUT request.
*
*
* @return The presigned Amazon S3 URL that was used to store a file using a PUT request.
*/
public final String url() {
return url;
}
/**
*
* The upload's metadata. For example, for Android, this contains information that is parsed from the manifest and
* is displayed in the AWS Device Farm console after the associated app is uploaded.
*
*
* @return The upload's metadata. For example, for Android, this contains information that is parsed from the
* manifest and is displayed in the AWS Device Farm console after the associated app is uploaded.
*/
public final String metadata() {
return metadata;
}
/**
*
* The upload's content type (for example, application/octet-stream
).
*
*
* @return The upload's content type (for example, application/octet-stream
).
*/
public final String contentType() {
return contentType;
}
/**
*
* A message about the upload's result.
*
*
* @return A message about the upload's result.
*/
public final String message() {
return message;
}
/**
*
* The upload's category. Allowed values include:
*
*
* -
*
* CURATED: An upload managed by AWS Device Farm.
*
*
* -
*
* PRIVATE: An upload managed by the AWS Device Farm customer.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #category} will
* return {@link UploadCategory#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #categoryAsString}.
*
*
* @return The upload's category. Allowed values include:
*
* -
*
* CURATED: An upload managed by AWS Device Farm.
*
*
* -
*
* PRIVATE: An upload managed by the AWS Device Farm customer.
*
*
* @see UploadCategory
*/
public final UploadCategory category() {
return UploadCategory.fromValue(category);
}
/**
*
* The upload's category. Allowed values include:
*
*
* -
*
* CURATED: An upload managed by AWS Device Farm.
*
*
* -
*
* PRIVATE: An upload managed by the AWS Device Farm customer.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #category} will
* return {@link UploadCategory#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #categoryAsString}.
*
*
* @return The upload's category. Allowed values include:
*
* -
*
* CURATED: An upload managed by AWS Device Farm.
*
*
* -
*
* PRIVATE: An upload managed by the AWS Device Farm customer.
*
*
* @see UploadCategory
*/
public final String categoryAsString() {
return category;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(created());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(url());
hashCode = 31 * hashCode + Objects.hashCode(metadata());
hashCode = 31 * hashCode + Objects.hashCode(contentType());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(categoryAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Upload)) {
return false;
}
Upload other = (Upload) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(created(), other.created()) && Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(url(), other.url())
&& Objects.equals(metadata(), other.metadata()) && Objects.equals(contentType(), other.contentType())
&& Objects.equals(message(), other.message()) && Objects.equals(categoryAsString(), other.categoryAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Upload").add("Arn", arn()).add("Name", name()).add("Created", created())
.add("Type", typeAsString()).add("Status", statusAsString())
.add("Url", url() == null ? null : "*** Sensitive Data Redacted ***").add("Metadata", metadata())
.add("ContentType", contentType()).add("Message", message()).add("Category", categoryAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "created":
return Optional.ofNullable(clazz.cast(created()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "url":
return Optional.ofNullable(clazz.cast(url()));
case "metadata":
return Optional.ofNullable(clazz.cast(metadata()));
case "contentType":
return Optional.ofNullable(clazz.cast(contentType()));
case "message":
return Optional.ofNullable(clazz.cast(message()));
case "category":
return Optional.ofNullable(clazz.cast(categoryAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function