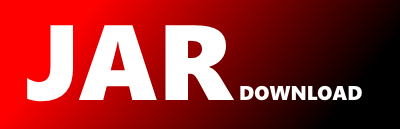
software.amazon.awssdk.services.devicefarm.model.Run Maven / Gradle / Ivy
Show all versions of devicefarm Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.beans.Transient;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a test run on a set of devices with a given app package, test parameters, and so on.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Run implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(Run::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(Run::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(Run::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField PLATFORM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platform").getter(getter(Run::platformAsString)).setter(setter(Builder::platform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platform").build()).build();
private static final SdkField CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("created").getter(getter(Run::created)).setter(setter(Builder::created))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("created").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(Run::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField RESULT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("result")
.getter(getter(Run::resultAsString)).setter(setter(Builder::result))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("result").build()).build();
private static final SdkField STARTED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("started").getter(getter(Run::started)).setter(setter(Builder::started))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("started").build()).build();
private static final SdkField STOPPED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("stopped").getter(getter(Run::stopped)).setter(setter(Builder::stopped))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stopped").build()).build();
private static final SdkField COUNTERS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("counters").getter(getter(Run::counters)).setter(setter(Builder::counters))
.constructor(Counters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("counters").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("message")
.getter(getter(Run::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("message").build()).build();
private static final SdkField TOTAL_JOBS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("totalJobs").getter(getter(Run::totalJobs)).setter(setter(Builder::totalJobs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalJobs").build()).build();
private static final SdkField COMPLETED_JOBS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("completedJobs").getter(getter(Run::completedJobs)).setter(setter(Builder::completedJobs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("completedJobs").build()).build();
private static final SdkField BILLING_METHOD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("billingMethod").getter(getter(Run::billingMethodAsString)).setter(setter(Builder::billingMethod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("billingMethod").build()).build();
private static final SdkField DEVICE_MINUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deviceMinutes").getter(getter(Run::deviceMinutes))
.setter(setter(Builder::deviceMinutes)).constructor(DeviceMinutes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceMinutes").build()).build();
private static final SdkField NETWORK_PROFILE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkProfile").getter(getter(Run::networkProfile))
.setter(setter(Builder::networkProfile)).constructor(NetworkProfile::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkProfile").build()).build();
private static final SdkField PARSING_RESULT_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("parsingResultUrl").getter(getter(Run::parsingResultUrl)).setter(setter(Builder::parsingResultUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("parsingResultUrl").build()).build();
private static final SdkField RESULT_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resultCode").getter(getter(Run::resultCodeAsString)).setter(setter(Builder::resultCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resultCode").build()).build();
private static final SdkField SEED_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("seed")
.getter(getter(Run::seed)).setter(setter(Builder::seed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("seed").build()).build();
private static final SdkField APP_UPLOAD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("appUpload").getter(getter(Run::appUpload)).setter(setter(Builder::appUpload))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("appUpload").build()).build();
private static final SdkField EVENT_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("eventCount").getter(getter(Run::eventCount)).setter(setter(Builder::eventCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventCount").build()).build();
private static final SdkField JOB_TIMEOUT_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("jobTimeoutMinutes").getter(getter(Run::jobTimeoutMinutes)).setter(setter(Builder::jobTimeoutMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("jobTimeoutMinutes").build()).build();
private static final SdkField DEVICE_POOL_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("devicePoolArn").getter(getter(Run::devicePoolArn)).setter(setter(Builder::devicePoolArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("devicePoolArn").build()).build();
private static final SdkField LOCALE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("locale")
.getter(getter(Run::locale)).setter(setter(Builder::locale))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("locale").build()).build();
private static final SdkField RADIOS_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("radios")
.getter(getter(Run::radios)).setter(setter(Builder::radios)).constructor(Radios::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("radios").build()).build();
private static final SdkField LOCATION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("location").getter(getter(Run::location)).setter(setter(Builder::location))
.constructor(Location::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("location").build()).build();
private static final SdkField CUSTOMER_ARTIFACT_PATHS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("customerArtifactPaths")
.getter(getter(Run::customerArtifactPaths)).setter(setter(Builder::customerArtifactPaths))
.constructor(CustomerArtifactPaths::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("customerArtifactPaths").build())
.build();
private static final SdkField WEB_URL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("webUrl")
.getter(getter(Run::webUrl)).setter(setter(Builder::webUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("webUrl").build()).build();
private static final SdkField SKIP_APP_RESIGN_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("skipAppResign").getter(getter(Run::skipAppResign)).setter(setter(Builder::skipAppResign))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("skipAppResign").build()).build();
private static final SdkField TEST_SPEC_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("testSpecArn").getter(getter(Run::testSpecArn)).setter(setter(Builder::testSpecArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("testSpecArn").build()).build();
private static final SdkField DEVICE_SELECTION_RESULT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deviceSelectionResult")
.getter(getter(Run::deviceSelectionResult)).setter(setter(Builder::deviceSelectionResult))
.constructor(DeviceSelectionResult::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceSelectionResult").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, NAME_FIELD,
TYPE_FIELD, PLATFORM_FIELD, CREATED_FIELD, STATUS_FIELD, RESULT_FIELD, STARTED_FIELD, STOPPED_FIELD, COUNTERS_FIELD,
MESSAGE_FIELD, TOTAL_JOBS_FIELD, COMPLETED_JOBS_FIELD, BILLING_METHOD_FIELD, DEVICE_MINUTES_FIELD,
NETWORK_PROFILE_FIELD, PARSING_RESULT_URL_FIELD, RESULT_CODE_FIELD, SEED_FIELD, APP_UPLOAD_FIELD, EVENT_COUNT_FIELD,
JOB_TIMEOUT_MINUTES_FIELD, DEVICE_POOL_ARN_FIELD, LOCALE_FIELD, RADIOS_FIELD, LOCATION_FIELD,
CUSTOMER_ARTIFACT_PATHS_FIELD, WEB_URL_FIELD, SKIP_APP_RESIGN_FIELD, TEST_SPEC_ARN_FIELD,
DEVICE_SELECTION_RESULT_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String name;
private final String type;
private final String platform;
private final Instant created;
private final String status;
private final String result;
private final Instant started;
private final Instant stopped;
private final Counters counters;
private final String message;
private final Integer totalJobs;
private final Integer completedJobs;
private final String billingMethod;
private final DeviceMinutes deviceMinutes;
private final NetworkProfile networkProfile;
private final String parsingResultUrl;
private final String resultCode;
private final Integer seed;
private final String appUpload;
private final Integer eventCount;
private final Integer jobTimeoutMinutes;
private final String devicePoolArn;
private final String locale;
private final Radios radios;
private final Location location;
private final CustomerArtifactPaths customerArtifactPaths;
private final String webUrl;
private final Boolean skipAppResign;
private final String testSpecArn;
private final DeviceSelectionResult deviceSelectionResult;
private Run(BuilderImpl builder) {
this.arn = builder.arn;
this.name = builder.name;
this.type = builder.type;
this.platform = builder.platform;
this.created = builder.created;
this.status = builder.status;
this.result = builder.result;
this.started = builder.started;
this.stopped = builder.stopped;
this.counters = builder.counters;
this.message = builder.message;
this.totalJobs = builder.totalJobs;
this.completedJobs = builder.completedJobs;
this.billingMethod = builder.billingMethod;
this.deviceMinutes = builder.deviceMinutes;
this.networkProfile = builder.networkProfile;
this.parsingResultUrl = builder.parsingResultUrl;
this.resultCode = builder.resultCode;
this.seed = builder.seed;
this.appUpload = builder.appUpload;
this.eventCount = builder.eventCount;
this.jobTimeoutMinutes = builder.jobTimeoutMinutes;
this.devicePoolArn = builder.devicePoolArn;
this.locale = builder.locale;
this.radios = builder.radios;
this.location = builder.location;
this.customerArtifactPaths = builder.customerArtifactPaths;
this.webUrl = builder.webUrl;
this.skipAppResign = builder.skipAppResign;
this.testSpecArn = builder.testSpecArn;
this.deviceSelectionResult = builder.deviceSelectionResult;
}
/**
*
* The run's ARN.
*
*
* @return The run's ARN.
*/
public final String arn() {
return arn;
}
/**
*
* The run's name.
*
*
* @return The run's name.
*/
public final String name() {
return name;
}
/**
*
* The run's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* For Android, an app explorer that traverses an Android app, interacting with it and capturing screenshots at the
* same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link TestType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The run's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* For Android, an app explorer that traverses an Android app, interacting with it and capturing screenshots
* at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @see TestType
*/
public final TestType type() {
return TestType.fromValue(type);
}
/**
*
* The run's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* For Android, an app explorer that traverses an Android app, interacting with it and capturing screenshots at the
* same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link TestType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The run's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* For Android, an app explorer that traverses an Android app, interacting with it and capturing screenshots
* at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @see TestType
*/
public final String typeAsString() {
return type;
}
/**
*
* The run's platform.
*
*
* Allowed values include:
*
*
* -
*
* ANDROID
*
*
* -
*
* IOS
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #platform} will
* return {@link DevicePlatform#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #platformAsString}.
*
*
* @return The run's platform.
*
* Allowed values include:
*
*
* -
*
* ANDROID
*
*
* -
*
* IOS
*
*
* @see DevicePlatform
*/
public final DevicePlatform platform() {
return DevicePlatform.fromValue(platform);
}
/**
*
* The run's platform.
*
*
* Allowed values include:
*
*
* -
*
* ANDROID
*
*
* -
*
* IOS
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #platform} will
* return {@link DevicePlatform#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #platformAsString}.
*
*
* @return The run's platform.
*
* Allowed values include:
*
*
* -
*
* ANDROID
*
*
* -
*
* IOS
*
*
* @see DevicePlatform
*/
public final String platformAsString() {
return platform;
}
/**
*
* When the run was created.
*
*
* @return When the run was created.
*/
public final Instant created() {
return created;
}
/**
*
* The run's status.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The run's status.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
* @see ExecutionStatus
*/
public final ExecutionStatus status() {
return ExecutionStatus.fromValue(status);
}
/**
*
* The run's status.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The run's status.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
* @see ExecutionStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The run's result.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The run's result.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
* @see ExecutionResult
*/
public final ExecutionResult result() {
return ExecutionResult.fromValue(result);
}
/**
*
* The run's result.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The run's result.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
* @see ExecutionResult
*/
public final String resultAsString() {
return result;
}
/**
*
* The run's start time.
*
*
* @return The run's start time.
*/
public final Instant started() {
return started;
}
/**
*
* The run's stop time.
*
*
* @return The run's stop time.
*/
public final Instant stopped() {
return stopped;
}
/**
*
* The run's result counters.
*
*
* @return The run's result counters.
*/
public final Counters counters() {
return counters;
}
/**
*
* A message about the run's result.
*
*
* @return A message about the run's result.
*/
public final String message() {
return message;
}
/**
*
* The total number of jobs for the run.
*
*
* @return The total number of jobs for the run.
*/
public final Integer totalJobs() {
return totalJobs;
}
/**
*
* The total number of completed jobs.
*
*
* @return The total number of completed jobs.
*/
public final Integer completedJobs() {
return completedJobs;
}
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
*
* If you have unmetered device slots, you must set this to unmetered
to use them. Otherwise, the run
* is counted toward metered device minutes.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMethod}
* will return {@link BillingMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingMethodAsString}.
*
*
* @return Specifies the billing method for a test run: metered
or unmetered
. If the
* parameter is not specified, the default value is metered
.
*
* If you have unmetered device slots, you must set this to unmetered
to use them. Otherwise,
* the run is counted toward metered device minutes.
*
* @see BillingMethod
*/
public final BillingMethod billingMethod() {
return BillingMethod.fromValue(billingMethod);
}
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
*
* If you have unmetered device slots, you must set this to unmetered
to use them. Otherwise, the run
* is counted toward metered device minutes.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMethod}
* will return {@link BillingMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingMethodAsString}.
*
*
* @return Specifies the billing method for a test run: metered
or unmetered
. If the
* parameter is not specified, the default value is metered
.
*
* If you have unmetered device slots, you must set this to unmetered
to use them. Otherwise,
* the run is counted toward metered device minutes.
*
* @see BillingMethod
*/
public final String billingMethodAsString() {
return billingMethod;
}
/**
*
* Represents the total (metered or unmetered) minutes used by the test run.
*
*
* @return Represents the total (metered or unmetered) minutes used by the test run.
*/
public final DeviceMinutes deviceMinutes() {
return deviceMinutes;
}
/**
*
* The network profile being used for a test run.
*
*
* @return The network profile being used for a test run.
*/
public final NetworkProfile networkProfile() {
return networkProfile;
}
/**
*
* Read-only URL for an object in an S3 bucket where you can get the parsing results of the test package. If the
* test package doesn't parse, the reason why it doesn't parse appears in the file that this URL points to.
*
*
* @return Read-only URL for an object in an S3 bucket where you can get the parsing results of the test package. If
* the test package doesn't parse, the reason why it doesn't parse appears in the file that this URL points
* to.
*/
public final String parsingResultUrl() {
return parsingResultUrl;
}
/**
*
* Supporting field for the result field. Set only if result
is SKIPPED
.
* PARSING_FAILED
if the result is skipped because of test package parsing failure.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resultCode} will
* return {@link ExecutionResultCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resultCodeAsString}.
*
*
* @return Supporting field for the result field. Set only if result
is SKIPPED
.
* PARSING_FAILED
if the result is skipped because of test package parsing failure.
* @see ExecutionResultCode
*/
public final ExecutionResultCode resultCode() {
return ExecutionResultCode.fromValue(resultCode);
}
/**
*
* Supporting field for the result field. Set only if result
is SKIPPED
.
* PARSING_FAILED
if the result is skipped because of test package parsing failure.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resultCode} will
* return {@link ExecutionResultCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resultCodeAsString}.
*
*
* @return Supporting field for the result field. Set only if result
is SKIPPED
.
* PARSING_FAILED
if the result is skipped because of test package parsing failure.
* @see ExecutionResultCode
*/
public final String resultCodeAsString() {
return resultCode;
}
/**
*
* For fuzz tests, this is a seed to use for randomizing the UI fuzz test. Using the same seed value between tests
* ensures identical event sequences.
*
*
* @return For fuzz tests, this is a seed to use for randomizing the UI fuzz test. Using the same seed value between
* tests ensures identical event sequences.
*/
public final Integer seed() {
return seed;
}
/**
*
* An app to upload or that has been uploaded.
*
*
* @return An app to upload or that has been uploaded.
*/
public final String appUpload() {
return appUpload;
}
/**
*
* For fuzz tests, this is the number of events, between 1 and 10000, that the UI fuzz test should perform.
*
*
* @return For fuzz tests, this is the number of events, between 1 and 10000, that the UI fuzz test should perform.
*/
public final Integer eventCount() {
return eventCount;
}
/**
*
* The number of minutes the job executes before it times out.
*
*
* @return The number of minutes the job executes before it times out.
*/
public final Integer jobTimeoutMinutes() {
return jobTimeoutMinutes;
}
/**
*
* The ARN of the device pool for the run.
*
*
* @return The ARN of the device pool for the run.
*/
public final String devicePoolArn() {
return devicePoolArn;
}
/**
*
* Information about the locale that is used for the run.
*
*
* @return Information about the locale that is used for the run.
*/
public final String locale() {
return locale;
}
/**
*
* Information about the radio states for the run.
*
*
* @return Information about the radio states for the run.
*/
public final Radios radios() {
return radios;
}
/**
*
* Information about the location that is used for the run.
*
*
* @return Information about the location that is used for the run.
*/
public final Location location() {
return location;
}
/**
*
* Output CustomerArtifactPaths
object for the test run.
*
*
* @return Output CustomerArtifactPaths
object for the test run.
*/
public final CustomerArtifactPaths customerArtifactPaths() {
return customerArtifactPaths;
}
/**
*
* The Device Farm console URL for the recording of the run.
*
*
* @return The Device Farm console URL for the recording of the run.
*/
public final String webUrl() {
return webUrl;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm FAQs.
*
*
* @return When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm
* FAQs.
*/
public final Boolean skipAppResign() {
return skipAppResign;
}
/**
*
* The ARN of the YAML-formatted test specification for the run.
*
*
* @return The ARN of the YAML-formatted test specification for the run.
*/
public final String testSpecArn() {
return testSpecArn;
}
/**
*
* The results of a device filter used to select the devices for a test run.
*
*
* @return The results of a device filter used to select the devices for a test run.
*/
public final DeviceSelectionResult deviceSelectionResult() {
return deviceSelectionResult;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(platformAsString());
hashCode = 31 * hashCode + Objects.hashCode(created());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(resultAsString());
hashCode = 31 * hashCode + Objects.hashCode(started());
hashCode = 31 * hashCode + Objects.hashCode(stopped());
hashCode = 31 * hashCode + Objects.hashCode(counters());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(totalJobs());
hashCode = 31 * hashCode + Objects.hashCode(completedJobs());
hashCode = 31 * hashCode + Objects.hashCode(billingMethodAsString());
hashCode = 31 * hashCode + Objects.hashCode(deviceMinutes());
hashCode = 31 * hashCode + Objects.hashCode(networkProfile());
hashCode = 31 * hashCode + Objects.hashCode(parsingResultUrl());
hashCode = 31 * hashCode + Objects.hashCode(resultCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(seed());
hashCode = 31 * hashCode + Objects.hashCode(appUpload());
hashCode = 31 * hashCode + Objects.hashCode(eventCount());
hashCode = 31 * hashCode + Objects.hashCode(jobTimeoutMinutes());
hashCode = 31 * hashCode + Objects.hashCode(devicePoolArn());
hashCode = 31 * hashCode + Objects.hashCode(locale());
hashCode = 31 * hashCode + Objects.hashCode(radios());
hashCode = 31 * hashCode + Objects.hashCode(location());
hashCode = 31 * hashCode + Objects.hashCode(customerArtifactPaths());
hashCode = 31 * hashCode + Objects.hashCode(webUrl());
hashCode = 31 * hashCode + Objects.hashCode(skipAppResign());
hashCode = 31 * hashCode + Objects.hashCode(testSpecArn());
hashCode = 31 * hashCode + Objects.hashCode(deviceSelectionResult());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Run)) {
return false;
}
Run other = (Run) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(platformAsString(), other.platformAsString()) && Objects.equals(created(), other.created())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(resultAsString(), other.resultAsString()) && Objects.equals(started(), other.started())
&& Objects.equals(stopped(), other.stopped()) && Objects.equals(counters(), other.counters())
&& Objects.equals(message(), other.message()) && Objects.equals(totalJobs(), other.totalJobs())
&& Objects.equals(completedJobs(), other.completedJobs())
&& Objects.equals(billingMethodAsString(), other.billingMethodAsString())
&& Objects.equals(deviceMinutes(), other.deviceMinutes())
&& Objects.equals(networkProfile(), other.networkProfile())
&& Objects.equals(parsingResultUrl(), other.parsingResultUrl())
&& Objects.equals(resultCodeAsString(), other.resultCodeAsString()) && Objects.equals(seed(), other.seed())
&& Objects.equals(appUpload(), other.appUpload()) && Objects.equals(eventCount(), other.eventCount())
&& Objects.equals(jobTimeoutMinutes(), other.jobTimeoutMinutes())
&& Objects.equals(devicePoolArn(), other.devicePoolArn()) && Objects.equals(locale(), other.locale())
&& Objects.equals(radios(), other.radios()) && Objects.equals(location(), other.location())
&& Objects.equals(customerArtifactPaths(), other.customerArtifactPaths())
&& Objects.equals(webUrl(), other.webUrl()) && Objects.equals(skipAppResign(), other.skipAppResign())
&& Objects.equals(testSpecArn(), other.testSpecArn())
&& Objects.equals(deviceSelectionResult(), other.deviceSelectionResult());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Run").add("Arn", arn()).add("Name", name()).add("Type", typeAsString())
.add("Platform", platformAsString()).add("Created", created()).add("Status", statusAsString())
.add("Result", resultAsString()).add("Started", started()).add("Stopped", stopped()).add("Counters", counters())
.add("Message", message()).add("TotalJobs", totalJobs()).add("CompletedJobs", completedJobs())
.add("BillingMethod", billingMethodAsString()).add("DeviceMinutes", deviceMinutes())
.add("NetworkProfile", networkProfile()).add("ParsingResultUrl", parsingResultUrl())
.add("ResultCode", resultCodeAsString()).add("Seed", seed()).add("AppUpload", appUpload())
.add("EventCount", eventCount()).add("JobTimeoutMinutes", jobTimeoutMinutes())
.add("DevicePoolArn", devicePoolArn()).add("Locale", locale()).add("Radios", radios())
.add("Location", location()).add("CustomerArtifactPaths", customerArtifactPaths()).add("WebUrl", webUrl())
.add("SkipAppResign", skipAppResign()).add("TestSpecArn", testSpecArn())
.add("DeviceSelectionResult", deviceSelectionResult()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "platform":
return Optional.ofNullable(clazz.cast(platformAsString()));
case "created":
return Optional.ofNullable(clazz.cast(created()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "result":
return Optional.ofNullable(clazz.cast(resultAsString()));
case "started":
return Optional.ofNullable(clazz.cast(started()));
case "stopped":
return Optional.ofNullable(clazz.cast(stopped()));
case "counters":
return Optional.ofNullable(clazz.cast(counters()));
case "message":
return Optional.ofNullable(clazz.cast(message()));
case "totalJobs":
return Optional.ofNullable(clazz.cast(totalJobs()));
case "completedJobs":
return Optional.ofNullable(clazz.cast(completedJobs()));
case "billingMethod":
return Optional.ofNullable(clazz.cast(billingMethodAsString()));
case "deviceMinutes":
return Optional.ofNullable(clazz.cast(deviceMinutes()));
case "networkProfile":
return Optional.ofNullable(clazz.cast(networkProfile()));
case "parsingResultUrl":
return Optional.ofNullable(clazz.cast(parsingResultUrl()));
case "resultCode":
return Optional.ofNullable(clazz.cast(resultCodeAsString()));
case "seed":
return Optional.ofNullable(clazz.cast(seed()));
case "appUpload":
return Optional.ofNullable(clazz.cast(appUpload()));
case "eventCount":
return Optional.ofNullable(clazz.cast(eventCount()));
case "jobTimeoutMinutes":
return Optional.ofNullable(clazz.cast(jobTimeoutMinutes()));
case "devicePoolArn":
return Optional.ofNullable(clazz.cast(devicePoolArn()));
case "locale":
return Optional.ofNullable(clazz.cast(locale()));
case "radios":
return Optional.ofNullable(clazz.cast(radios()));
case "location":
return Optional.ofNullable(clazz.cast(location()));
case "customerArtifactPaths":
return Optional.ofNullable(clazz.cast(customerArtifactPaths()));
case "webUrl":
return Optional.ofNullable(clazz.cast(webUrl()));
case "skipAppResign":
return Optional.ofNullable(clazz.cast(skipAppResign()));
case "testSpecArn":
return Optional.ofNullable(clazz.cast(testSpecArn()));
case "deviceSelectionResult":
return Optional.ofNullable(clazz.cast(deviceSelectionResult()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function