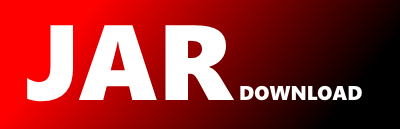
software.amazon.awssdk.services.devicefarm.model.ScheduleRunRequest Maven / Gradle / Ivy
Show all versions of devicefarm Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a request to the schedule run operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ScheduleRunRequest extends DeviceFarmRequest implements
ToCopyableBuilder {
private static final SdkField PROJECT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("projectArn").getter(getter(ScheduleRunRequest::projectArn)).setter(setter(Builder::projectArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("projectArn").build()).build();
private static final SdkField APP_ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("appArn")
.getter(getter(ScheduleRunRequest::appArn)).setter(setter(Builder::appArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("appArn").build()).build();
private static final SdkField DEVICE_POOL_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("devicePoolArn").getter(getter(ScheduleRunRequest::devicePoolArn)).setter(setter(Builder::devicePoolArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("devicePoolArn").build()).build();
private static final SdkField DEVICE_SELECTION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("deviceSelectionConfiguration")
.getter(getter(ScheduleRunRequest::deviceSelectionConfiguration))
.setter(setter(Builder::deviceSelectionConfiguration))
.constructor(DeviceSelectionConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceSelectionConfiguration")
.build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(ScheduleRunRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField TEST_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("test").getter(getter(ScheduleRunRequest::test)).setter(setter(Builder::test))
.constructor(ScheduleRunTest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("test").build()).build();
private static final SdkField CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("configuration")
.getter(getter(ScheduleRunRequest::configuration)).setter(setter(Builder::configuration))
.constructor(ScheduleRunConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("configuration").build()).build();
private static final SdkField EXECUTION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("executionConfiguration")
.getter(getter(ScheduleRunRequest::executionConfiguration)).setter(setter(Builder::executionConfiguration))
.constructor(ExecutionConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("executionConfiguration").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PROJECT_ARN_FIELD,
APP_ARN_FIELD, DEVICE_POOL_ARN_FIELD, DEVICE_SELECTION_CONFIGURATION_FIELD, NAME_FIELD, TEST_FIELD,
CONFIGURATION_FIELD, EXECUTION_CONFIGURATION_FIELD));
private final String projectArn;
private final String appArn;
private final String devicePoolArn;
private final DeviceSelectionConfiguration deviceSelectionConfiguration;
private final String name;
private final ScheduleRunTest test;
private final ScheduleRunConfiguration configuration;
private final ExecutionConfiguration executionConfiguration;
private ScheduleRunRequest(BuilderImpl builder) {
super(builder);
this.projectArn = builder.projectArn;
this.appArn = builder.appArn;
this.devicePoolArn = builder.devicePoolArn;
this.deviceSelectionConfiguration = builder.deviceSelectionConfiguration;
this.name = builder.name;
this.test = builder.test;
this.configuration = builder.configuration;
this.executionConfiguration = builder.executionConfiguration;
}
/**
*
* The ARN of the project for the run to be scheduled.
*
*
* @return The ARN of the project for the run to be scheduled.
*/
public final String projectArn() {
return projectArn;
}
/**
*
* The ARN of an application package to run tests against, created with CreateUpload. See ListUploads.
*
*
* @return The ARN of an application package to run tests against, created with CreateUpload. See
* ListUploads.
*/
public final String appArn() {
return appArn;
}
/**
*
* The ARN of the device pool for the run to be scheduled.
*
*
* @return The ARN of the device pool for the run to be scheduled.
*/
public final String devicePoolArn() {
return devicePoolArn;
}
/**
*
* The filter criteria used to dynamically select a set of devices for a test run and the maximum number of devices
* to be included in the run.
*
*
* Either devicePoolArn
or deviceSelectionConfiguration
is required in a
* request.
*
*
* @return The filter criteria used to dynamically select a set of devices for a test run and the maximum number of
* devices to be included in the run.
*
* Either devicePoolArn
or deviceSelectionConfiguration
is
* required in a request.
*/
public final DeviceSelectionConfiguration deviceSelectionConfiguration() {
return deviceSelectionConfiguration;
}
/**
*
* The name for the run to be scheduled.
*
*
* @return The name for the run to be scheduled.
*/
public final String name() {
return name;
}
/**
*
* Information about the test for the run to be scheduled.
*
*
* @return Information about the test for the run to be scheduled.
*/
public final ScheduleRunTest test() {
return test;
}
/**
*
* Information about the settings for the run to be scheduled.
*
*
* @return Information about the settings for the run to be scheduled.
*/
public final ScheduleRunConfiguration configuration() {
return configuration;
}
/**
*
* Specifies configuration information about a test run, such as the execution timeout (in minutes).
*
*
* @return Specifies configuration information about a test run, such as the execution timeout (in minutes).
*/
public final ExecutionConfiguration executionConfiguration() {
return executionConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(projectArn());
hashCode = 31 * hashCode + Objects.hashCode(appArn());
hashCode = 31 * hashCode + Objects.hashCode(devicePoolArn());
hashCode = 31 * hashCode + Objects.hashCode(deviceSelectionConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(test());
hashCode = 31 * hashCode + Objects.hashCode(configuration());
hashCode = 31 * hashCode + Objects.hashCode(executionConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ScheduleRunRequest)) {
return false;
}
ScheduleRunRequest other = (ScheduleRunRequest) obj;
return Objects.equals(projectArn(), other.projectArn()) && Objects.equals(appArn(), other.appArn())
&& Objects.equals(devicePoolArn(), other.devicePoolArn())
&& Objects.equals(deviceSelectionConfiguration(), other.deviceSelectionConfiguration())
&& Objects.equals(name(), other.name()) && Objects.equals(test(), other.test())
&& Objects.equals(configuration(), other.configuration())
&& Objects.equals(executionConfiguration(), other.executionConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ScheduleRunRequest").add("ProjectArn", projectArn()).add("AppArn", appArn())
.add("DevicePoolArn", devicePoolArn()).add("DeviceSelectionConfiguration", deviceSelectionConfiguration())
.add("Name", name()).add("Test", test()).add("Configuration", configuration())
.add("ExecutionConfiguration", executionConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "projectArn":
return Optional.ofNullable(clazz.cast(projectArn()));
case "appArn":
return Optional.ofNullable(clazz.cast(appArn()));
case "devicePoolArn":
return Optional.ofNullable(clazz.cast(devicePoolArn()));
case "deviceSelectionConfiguration":
return Optional.ofNullable(clazz.cast(deviceSelectionConfiguration()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "test":
return Optional.ofNullable(clazz.cast(test()));
case "configuration":
return Optional.ofNullable(clazz.cast(configuration()));
case "executionConfiguration":
return Optional.ofNullable(clazz.cast(executionConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function