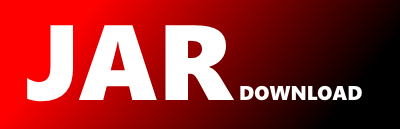
software.amazon.awssdk.services.devicefarm.model.RemoteAccessSession Maven / Gradle / Ivy
Show all versions of devicefarm Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents information about the remote access session.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RemoteAccessSession implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(RemoteAccessSession::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(RemoteAccessSession::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("created").getter(getter(RemoteAccessSession::created)).setter(setter(Builder::created))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("created").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(RemoteAccessSession::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField RESULT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("result")
.getter(getter(RemoteAccessSession::resultAsString)).setter(setter(Builder::result))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("result").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("message")
.getter(getter(RemoteAccessSession::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("message").build()).build();
private static final SdkField STARTED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("started").getter(getter(RemoteAccessSession::started)).setter(setter(Builder::started))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("started").build()).build();
private static final SdkField STOPPED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("stopped").getter(getter(RemoteAccessSession::stopped)).setter(setter(Builder::stopped))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stopped").build()).build();
private static final SdkField DEVICE_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("device")
.getter(getter(RemoteAccessSession::device)).setter(setter(Builder::device)).constructor(Device::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("device").build()).build();
private static final SdkField INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("instanceArn").getter(getter(RemoteAccessSession::instanceArn)).setter(setter(Builder::instanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("instanceArn").build()).build();
private static final SdkField REMOTE_DEBUG_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("remoteDebugEnabled").getter(getter(RemoteAccessSession::remoteDebugEnabled))
.setter(setter(Builder::remoteDebugEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteDebugEnabled").build())
.build();
private static final SdkField REMOTE_RECORD_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("remoteRecordEnabled").getter(getter(RemoteAccessSession::remoteRecordEnabled))
.setter(setter(Builder::remoteRecordEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteRecordEnabled").build())
.build();
private static final SdkField REMOTE_RECORD_APP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("remoteRecordAppArn").getter(getter(RemoteAccessSession::remoteRecordAppArn))
.setter(setter(Builder::remoteRecordAppArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteRecordAppArn").build())
.build();
private static final SdkField HOST_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("hostAddress").getter(getter(RemoteAccessSession::hostAddress)).setter(setter(Builder::hostAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("hostAddress").build()).build();
private static final SdkField CLIENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clientId").getter(getter(RemoteAccessSession::clientId)).setter(setter(Builder::clientId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientId").build()).build();
private static final SdkField BILLING_METHOD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("billingMethod").getter(getter(RemoteAccessSession::billingMethodAsString))
.setter(setter(Builder::billingMethod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("billingMethod").build()).build();
private static final SdkField DEVICE_MINUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deviceMinutes")
.getter(getter(RemoteAccessSession::deviceMinutes)).setter(setter(Builder::deviceMinutes))
.constructor(DeviceMinutes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceMinutes").build()).build();
private static final SdkField ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("endpoint").getter(getter(RemoteAccessSession::endpoint)).setter(setter(Builder::endpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endpoint").build()).build();
private static final SdkField DEVICE_UDID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("deviceUdid").getter(getter(RemoteAccessSession::deviceUdid)).setter(setter(Builder::deviceUdid))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceUdid").build()).build();
private static final SdkField INTERACTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("interactionMode").getter(getter(RemoteAccessSession::interactionModeAsString))
.setter(setter(Builder::interactionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("interactionMode").build()).build();
private static final SdkField SKIP_APP_RESIGN_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("skipAppResign").getter(getter(RemoteAccessSession::skipAppResign))
.setter(setter(Builder::skipAppResign))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("skipAppResign").build()).build();
private static final SdkField VPC_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("vpcConfig").getter(getter(RemoteAccessSession::vpcConfig)).setter(setter(Builder::vpcConfig))
.constructor(VpcConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vpcConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, NAME_FIELD,
CREATED_FIELD, STATUS_FIELD, RESULT_FIELD, MESSAGE_FIELD, STARTED_FIELD, STOPPED_FIELD, DEVICE_FIELD,
INSTANCE_ARN_FIELD, REMOTE_DEBUG_ENABLED_FIELD, REMOTE_RECORD_ENABLED_FIELD, REMOTE_RECORD_APP_ARN_FIELD,
HOST_ADDRESS_FIELD, CLIENT_ID_FIELD, BILLING_METHOD_FIELD, DEVICE_MINUTES_FIELD, ENDPOINT_FIELD, DEVICE_UDID_FIELD,
INTERACTION_MODE_FIELD, SKIP_APP_RESIGN_FIELD, VPC_CONFIG_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String name;
private final Instant created;
private final String status;
private final String result;
private final String message;
private final Instant started;
private final Instant stopped;
private final Device device;
private final String instanceArn;
private final Boolean remoteDebugEnabled;
private final Boolean remoteRecordEnabled;
private final String remoteRecordAppArn;
private final String hostAddress;
private final String clientId;
private final String billingMethod;
private final DeviceMinutes deviceMinutes;
private final String endpoint;
private final String deviceUdid;
private final String interactionMode;
private final Boolean skipAppResign;
private final VpcConfig vpcConfig;
private RemoteAccessSession(BuilderImpl builder) {
this.arn = builder.arn;
this.name = builder.name;
this.created = builder.created;
this.status = builder.status;
this.result = builder.result;
this.message = builder.message;
this.started = builder.started;
this.stopped = builder.stopped;
this.device = builder.device;
this.instanceArn = builder.instanceArn;
this.remoteDebugEnabled = builder.remoteDebugEnabled;
this.remoteRecordEnabled = builder.remoteRecordEnabled;
this.remoteRecordAppArn = builder.remoteRecordAppArn;
this.hostAddress = builder.hostAddress;
this.clientId = builder.clientId;
this.billingMethod = builder.billingMethod;
this.deviceMinutes = builder.deviceMinutes;
this.endpoint = builder.endpoint;
this.deviceUdid = builder.deviceUdid;
this.interactionMode = builder.interactionMode;
this.skipAppResign = builder.skipAppResign;
this.vpcConfig = builder.vpcConfig;
}
/**
*
* The Amazon Resource Name (ARN) of the remote access session.
*
*
* @return The Amazon Resource Name (ARN) of the remote access session.
*/
public final String arn() {
return arn;
}
/**
*
* The name of the remote access session.
*
*
* @return The name of the remote access session.
*/
public final String name() {
return name;
}
/**
*
* The date and time the remote access session was created.
*
*
* @return The date and time the remote access session was created.
*/
public final Instant created() {
return created;
}
/**
*
* The status of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING.
*
*
* -
*
* PENDING_CONCURRENCY.
*
*
* -
*
* PENDING_DEVICE.
*
*
* -
*
* PROCESSING.
*
*
* -
*
* SCHEDULING.
*
*
* -
*
* PREPARING.
*
*
* -
*
* RUNNING.
*
*
* -
*
* COMPLETED.
*
*
* -
*
* STOPPING.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the remote access session. Can be any of the following:
*
* -
*
* PENDING.
*
*
* -
*
* PENDING_CONCURRENCY.
*
*
* -
*
* PENDING_DEVICE.
*
*
* -
*
* PROCESSING.
*
*
* -
*
* SCHEDULING.
*
*
* -
*
* PREPARING.
*
*
* -
*
* RUNNING.
*
*
* -
*
* COMPLETED.
*
*
* -
*
* STOPPING.
*
*
* @see ExecutionStatus
*/
public final ExecutionStatus status() {
return ExecutionStatus.fromValue(status);
}
/**
*
* The status of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING.
*
*
* -
*
* PENDING_CONCURRENCY.
*
*
* -
*
* PENDING_DEVICE.
*
*
* -
*
* PROCESSING.
*
*
* -
*
* SCHEDULING.
*
*
* -
*
* PREPARING.
*
*
* -
*
* RUNNING.
*
*
* -
*
* COMPLETED.
*
*
* -
*
* STOPPING.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the remote access session. Can be any of the following:
*
* -
*
* PENDING.
*
*
* -
*
* PENDING_CONCURRENCY.
*
*
* -
*
* PENDING_DEVICE.
*
*
* -
*
* PROCESSING.
*
*
* -
*
* SCHEDULING.
*
*
* -
*
* PREPARING.
*
*
* -
*
* RUNNING.
*
*
* -
*
* COMPLETED.
*
*
* -
*
* STOPPING.
*
*
* @see ExecutionStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The result of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING.
*
*
* -
*
* PASSED.
*
*
* -
*
* WARNED.
*
*
* -
*
* FAILED.
*
*
* -
*
* SKIPPED.
*
*
* -
*
* ERRORED.
*
*
* -
*
* STOPPED.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The result of the remote access session. Can be any of the following:
*
* -
*
* PENDING.
*
*
* -
*
* PASSED.
*
*
* -
*
* WARNED.
*
*
* -
*
* FAILED.
*
*
* -
*
* SKIPPED.
*
*
* -
*
* ERRORED.
*
*
* -
*
* STOPPED.
*
*
* @see ExecutionResult
*/
public final ExecutionResult result() {
return ExecutionResult.fromValue(result);
}
/**
*
* The result of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING.
*
*
* -
*
* PASSED.
*
*
* -
*
* WARNED.
*
*
* -
*
* FAILED.
*
*
* -
*
* SKIPPED.
*
*
* -
*
* ERRORED.
*
*
* -
*
* STOPPED.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The result of the remote access session. Can be any of the following:
*
* -
*
* PENDING.
*
*
* -
*
* PASSED.
*
*
* -
*
* WARNED.
*
*
* -
*
* FAILED.
*
*
* -
*
* SKIPPED.
*
*
* -
*
* ERRORED.
*
*
* -
*
* STOPPED.
*
*
* @see ExecutionResult
*/
public final String resultAsString() {
return result;
}
/**
*
* A message about the remote access session.
*
*
* @return A message about the remote access session.
*/
public final String message() {
return message;
}
/**
*
* The date and time the remote access session was started.
*
*
* @return The date and time the remote access session was started.
*/
public final Instant started() {
return started;
}
/**
*
* The date and time the remote access session was stopped.
*
*
* @return The date and time the remote access session was stopped.
*/
public final Instant stopped() {
return stopped;
}
/**
*
* The device (phone or tablet) used in the remote access session.
*
*
* @return The device (phone or tablet) used in the remote access session.
*/
public final Device device() {
return device;
}
/**
*
* The ARN of the instance.
*
*
* @return The ARN of the instance.
*/
public final String instanceArn() {
return instanceArn;
}
/**
*
* This flag is set to true
if remote debugging is enabled for the remote access session.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return This flag is set to true
if remote debugging is enabled for the remote access session.
*
* Remote debugging is no longer supported.
*/
public final Boolean remoteDebugEnabled() {
return remoteDebugEnabled;
}
/**
*
* This flag is set to true
if remote recording is enabled for the remote access session.
*
*
* @return This flag is set to true
if remote recording is enabled for the remote access session.
*/
public final Boolean remoteRecordEnabled() {
return remoteRecordEnabled;
}
/**
*
* The ARN for the app to be recorded in the remote access session.
*
*
* @return The ARN for the app to be recorded in the remote access session.
*/
public final String remoteRecordAppArn() {
return remoteRecordAppArn;
}
/**
*
* IP address of the EC2 host where you need to connect to remotely debug devices. Only returned if remote debugging
* is enabled for the remote access session.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return IP address of the EC2 host where you need to connect to remotely debug devices. Only returned if remote
* debugging is enabled for the remote access session.
*
* Remote debugging is no longer supported.
*/
public final String hostAddress() {
return hostAddress;
}
/**
*
* Unique identifier of your client for the remote access session. Only returned if remote debugging is enabled for
* the remote access session.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return Unique identifier of your client for the remote access session. Only returned if remote debugging is
* enabled for the remote access session.
*
* Remote debugging is no longer supported.
*/
public final String clientId() {
return clientId;
}
/**
*
* The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS Device
* Farm terminology.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMethod}
* will return {@link BillingMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingMethodAsString}.
*
*
* @return The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS
* Device Farm terminology.
* @see BillingMethod
*/
public final BillingMethod billingMethod() {
return BillingMethod.fromValue(billingMethod);
}
/**
*
* The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS Device
* Farm terminology.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMethod}
* will return {@link BillingMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingMethodAsString}.
*
*
* @return The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS
* Device Farm terminology.
* @see BillingMethod
*/
public final String billingMethodAsString() {
return billingMethod;
}
/**
*
* The number of minutes a device is used in a remote access session (including setup and teardown minutes).
*
*
* @return The number of minutes a device is used in a remote access session (including setup and teardown minutes).
*/
public final DeviceMinutes deviceMinutes() {
return deviceMinutes;
}
/**
*
* The endpoint for the remote access sesssion.
*
*
* @return The endpoint for the remote access sesssion.
*/
public final String endpoint() {
return endpoint;
}
/**
*
* Unique device identifier for the remote device. Only returned if remote debugging is enabled for the remote
* access session.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return Unique device identifier for the remote device. Only returned if remote debugging is enabled for the
* remote access session.
*
* Remote debugging is no longer supported.
*/
public final String deviceUdid() {
return deviceUdid;
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run
* XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests
* and watch the screen in this mode.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #interactionMode}
* will return {@link InteractionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #interactionModeAsString}.
*
*
* @return The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
* @see InteractionMode
*/
public final InteractionMode interactionMode() {
return InteractionMode.fromValue(interactionMode);
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run
* XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests
* and watch the screen in this mode.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #interactionMode}
* will return {@link InteractionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #interactionModeAsString}.
*
*
* @return The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
* @see InteractionMode
*/
public final String interactionModeAsString() {
return interactionMode;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm FAQs.
*
*
* @return When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information about how Device Farm re-signs your apps, see Do you modify my app? in the AWS Device Farm
* FAQs.
*/
public final Boolean skipAppResign() {
return skipAppResign;
}
/**
*
* The VPC security groups and subnets that are attached to a project.
*
*
* @return The VPC security groups and subnets that are attached to a project.
*/
public final VpcConfig vpcConfig() {
return vpcConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(created());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(resultAsString());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(started());
hashCode = 31 * hashCode + Objects.hashCode(stopped());
hashCode = 31 * hashCode + Objects.hashCode(device());
hashCode = 31 * hashCode + Objects.hashCode(instanceArn());
hashCode = 31 * hashCode + Objects.hashCode(remoteDebugEnabled());
hashCode = 31 * hashCode + Objects.hashCode(remoteRecordEnabled());
hashCode = 31 * hashCode + Objects.hashCode(remoteRecordAppArn());
hashCode = 31 * hashCode + Objects.hashCode(hostAddress());
hashCode = 31 * hashCode + Objects.hashCode(clientId());
hashCode = 31 * hashCode + Objects.hashCode(billingMethodAsString());
hashCode = 31 * hashCode + Objects.hashCode(deviceMinutes());
hashCode = 31 * hashCode + Objects.hashCode(endpoint());
hashCode = 31 * hashCode + Objects.hashCode(deviceUdid());
hashCode = 31 * hashCode + Objects.hashCode(interactionModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(skipAppResign());
hashCode = 31 * hashCode + Objects.hashCode(vpcConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RemoteAccessSession)) {
return false;
}
RemoteAccessSession other = (RemoteAccessSession) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(created(), other.created()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(resultAsString(), other.resultAsString()) && Objects.equals(message(), other.message())
&& Objects.equals(started(), other.started()) && Objects.equals(stopped(), other.stopped())
&& Objects.equals(device(), other.device()) && Objects.equals(instanceArn(), other.instanceArn())
&& Objects.equals(remoteDebugEnabled(), other.remoteDebugEnabled())
&& Objects.equals(remoteRecordEnabled(), other.remoteRecordEnabled())
&& Objects.equals(remoteRecordAppArn(), other.remoteRecordAppArn())
&& Objects.equals(hostAddress(), other.hostAddress()) && Objects.equals(clientId(), other.clientId())
&& Objects.equals(billingMethodAsString(), other.billingMethodAsString())
&& Objects.equals(deviceMinutes(), other.deviceMinutes()) && Objects.equals(endpoint(), other.endpoint())
&& Objects.equals(deviceUdid(), other.deviceUdid())
&& Objects.equals(interactionModeAsString(), other.interactionModeAsString())
&& Objects.equals(skipAppResign(), other.skipAppResign()) && Objects.equals(vpcConfig(), other.vpcConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RemoteAccessSession").add("Arn", arn()).add("Name", name()).add("Created", created())
.add("Status", statusAsString()).add("Result", resultAsString()).add("Message", message())
.add("Started", started()).add("Stopped", stopped()).add("Device", device()).add("InstanceArn", instanceArn())
.add("RemoteDebugEnabled", remoteDebugEnabled()).add("RemoteRecordEnabled", remoteRecordEnabled())
.add("RemoteRecordAppArn", remoteRecordAppArn()).add("HostAddress", hostAddress()).add("ClientId", clientId())
.add("BillingMethod", billingMethodAsString()).add("DeviceMinutes", deviceMinutes()).add("Endpoint", endpoint())
.add("DeviceUdid", deviceUdid()).add("InteractionMode", interactionModeAsString())
.add("SkipAppResign", skipAppResign()).add("VpcConfig", vpcConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "created":
return Optional.ofNullable(clazz.cast(created()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "result":
return Optional.ofNullable(clazz.cast(resultAsString()));
case "message":
return Optional.ofNullable(clazz.cast(message()));
case "started":
return Optional.ofNullable(clazz.cast(started()));
case "stopped":
return Optional.ofNullable(clazz.cast(stopped()));
case "device":
return Optional.ofNullable(clazz.cast(device()));
case "instanceArn":
return Optional.ofNullable(clazz.cast(instanceArn()));
case "remoteDebugEnabled":
return Optional.ofNullable(clazz.cast(remoteDebugEnabled()));
case "remoteRecordEnabled":
return Optional.ofNullable(clazz.cast(remoteRecordEnabled()));
case "remoteRecordAppArn":
return Optional.ofNullable(clazz.cast(remoteRecordAppArn()));
case "hostAddress":
return Optional.ofNullable(clazz.cast(hostAddress()));
case "clientId":
return Optional.ofNullable(clazz.cast(clientId()));
case "billingMethod":
return Optional.ofNullable(clazz.cast(billingMethodAsString()));
case "deviceMinutes":
return Optional.ofNullable(clazz.cast(deviceMinutes()));
case "endpoint":
return Optional.ofNullable(clazz.cast(endpoint()));
case "deviceUdid":
return Optional.ofNullable(clazz.cast(deviceUdid()));
case "interactionMode":
return Optional.ofNullable(clazz.cast(interactionModeAsString()));
case "skipAppResign":
return Optional.ofNullable(clazz.cast(skipAppResign()));
case "vpcConfig":
return Optional.ofNullable(clazz.cast(vpcConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function