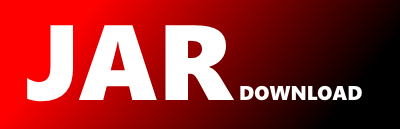
software.amazon.awssdk.services.devicefarm.model.Suite Maven / Gradle / Ivy
Show all versions of devicefarm Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a collection of one or more tests.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Suite implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(Suite::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(Suite::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(Suite::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("created").getter(getter(Suite::created)).setter(setter(Builder::created))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("created").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(Suite::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField RESULT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("result")
.getter(getter(Suite::resultAsString)).setter(setter(Builder::result))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("result").build()).build();
private static final SdkField STARTED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("started").getter(getter(Suite::started)).setter(setter(Builder::started))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("started").build()).build();
private static final SdkField STOPPED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("stopped").getter(getter(Suite::stopped)).setter(setter(Builder::stopped))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stopped").build()).build();
private static final SdkField COUNTERS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("counters").getter(getter(Suite::counters)).setter(setter(Builder::counters))
.constructor(Counters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("counters").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("message")
.getter(getter(Suite::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("message").build()).build();
private static final SdkField DEVICE_MINUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deviceMinutes").getter(getter(Suite::deviceMinutes))
.setter(setter(Builder::deviceMinutes)).constructor(DeviceMinutes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceMinutes").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, NAME_FIELD,
TYPE_FIELD, CREATED_FIELD, STATUS_FIELD, RESULT_FIELD, STARTED_FIELD, STOPPED_FIELD, COUNTERS_FIELD, MESSAGE_FIELD,
DEVICE_MINUTES_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String name;
private final String type;
private final Instant created;
private final String status;
private final String result;
private final Instant started;
private final Instant stopped;
private final Counters counters;
private final String message;
private final DeviceMinutes deviceMinutes;
private Suite(BuilderImpl builder) {
this.arn = builder.arn;
this.name = builder.name;
this.type = builder.type;
this.created = builder.created;
this.status = builder.status;
this.result = builder.result;
this.started = builder.started;
this.stopped = builder.stopped;
this.counters = builder.counters;
this.message = builder.message;
this.deviceMinutes = builder.deviceMinutes;
}
/**
*
* The suite's ARN.
*
*
* @return The suite's ARN.
*/
public final String arn() {
return arn;
}
/**
*
* The suite's name.
*
*
* @return The suite's name.
*/
public final String name() {
return name;
}
/**
*
* The suite's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* Only available for Android; an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link TestType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The suite's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* Only available for Android; an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @see TestType
*/
public final TestType type() {
return TestType.fromValue(type);
}
/**
*
* The suite's type.
*
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* Only available for Android; an app explorer that traverses an Android app, interacting with it and capturing
* screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link TestType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The suite's type.
*
* Must be one of the following values:
*
*
* -
*
* BUILTIN_FUZZ
*
*
* -
*
* BUILTIN_EXPLORER
*
*
*
* Only available for Android; an app explorer that traverses an Android app, interacting with it and
* capturing screenshots at the same time.
*
*
* -
*
* APPIUM_JAVA_JUNIT
*
*
* -
*
* APPIUM_JAVA_TESTNG
*
*
* -
*
* APPIUM_PYTHON
*
*
* -
*
* APPIUM_NODE
*
*
* -
*
* APPIUM_RUBY
*
*
* -
*
* APPIUM_WEB_JAVA_JUNIT
*
*
* -
*
* APPIUM_WEB_JAVA_TESTNG
*
*
* -
*
* APPIUM_WEB_PYTHON
*
*
* -
*
* APPIUM_WEB_NODE
*
*
* -
*
* APPIUM_WEB_RUBY
*
*
* -
*
* CALABASH
*
*
* -
*
* INSTRUMENTATION
*
*
* -
*
* UIAUTOMATION
*
*
* -
*
* UIAUTOMATOR
*
*
* -
*
* XCTEST
*
*
* -
*
* XCTEST_UI
*
*
* @see TestType
*/
public final String typeAsString() {
return type;
}
/**
*
* When the suite was created.
*
*
* @return When the suite was created.
*/
public final Instant created() {
return created;
}
/**
*
* The suite's status.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The suite's status.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
* @see ExecutionStatus
*/
public final ExecutionStatus status() {
return ExecutionStatus.fromValue(status);
}
/**
*
* The suite's status.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The suite's status.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PENDING_CONCURRENCY
*
*
* -
*
* PENDING_DEVICE
*
*
* -
*
* PROCESSING
*
*
* -
*
* SCHEDULING
*
*
* -
*
* PREPARING
*
*
* -
*
* RUNNING
*
*
* -
*
* COMPLETED
*
*
* -
*
* STOPPING
*
*
* @see ExecutionStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The suite's result.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The suite's result.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
* @see ExecutionResult
*/
public final ExecutionResult result() {
return ExecutionResult.fromValue(result);
}
/**
*
* The suite's result.
*
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The suite's result.
*
* Allowed values include:
*
*
* -
*
* PENDING
*
*
* -
*
* PASSED
*
*
* -
*
* WARNED
*
*
* -
*
* FAILED
*
*
* -
*
* SKIPPED
*
*
* -
*
* ERRORED
*
*
* -
*
* STOPPED
*
*
* @see ExecutionResult
*/
public final String resultAsString() {
return result;
}
/**
*
* The suite's start time.
*
*
* @return The suite's start time.
*/
public final Instant started() {
return started;
}
/**
*
* The suite's stop time.
*
*
* @return The suite's stop time.
*/
public final Instant stopped() {
return stopped;
}
/**
*
* The suite's result counters.
*
*
* @return The suite's result counters.
*/
public final Counters counters() {
return counters;
}
/**
*
* A message about the suite's result.
*
*
* @return A message about the suite's result.
*/
public final String message() {
return message;
}
/**
*
* Represents the total (metered or unmetered) minutes used by the test suite.
*
*
* @return Represents the total (metered or unmetered) minutes used by the test suite.
*/
public final DeviceMinutes deviceMinutes() {
return deviceMinutes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(created());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(resultAsString());
hashCode = 31 * hashCode + Objects.hashCode(started());
hashCode = 31 * hashCode + Objects.hashCode(stopped());
hashCode = 31 * hashCode + Objects.hashCode(counters());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(deviceMinutes());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Suite)) {
return false;
}
Suite other = (Suite) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(created(), other.created())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(resultAsString(), other.resultAsString()) && Objects.equals(started(), other.started())
&& Objects.equals(stopped(), other.stopped()) && Objects.equals(counters(), other.counters())
&& Objects.equals(message(), other.message()) && Objects.equals(deviceMinutes(), other.deviceMinutes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Suite").add("Arn", arn()).add("Name", name()).add("Type", typeAsString())
.add("Created", created()).add("Status", statusAsString()).add("Result", resultAsString())
.add("Started", started()).add("Stopped", stopped()).add("Counters", counters()).add("Message", message())
.add("DeviceMinutes", deviceMinutes()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "created":
return Optional.ofNullable(clazz.cast(created()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "result":
return Optional.ofNullable(clazz.cast(resultAsString()));
case "started":
return Optional.ofNullable(clazz.cast(started()));
case "stopped":
return Optional.ofNullable(clazz.cast(stopped()));
case "counters":
return Optional.ofNullable(clazz.cast(counters()));
case "message":
return Optional.ofNullable(clazz.cast(message()));
case "deviceMinutes":
return Optional.ofNullable(clazz.cast(deviceMinutes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function