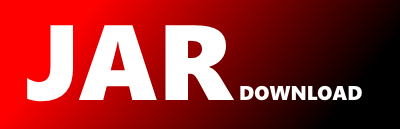
software.amazon.awssdk.services.devicefarm.DefaultDeviceFarmAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.devicefarm.model.ArgumentException;
import software.amazon.awssdk.services.devicefarm.model.CannotDeleteException;
import software.amazon.awssdk.services.devicefarm.model.CreateDevicePoolRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateDevicePoolResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateInstanceProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateInstanceProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateNetworkProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateNetworkProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateTestGridProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateTestGridProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateTestGridUrlRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateTestGridUrlResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateUploadRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateUploadResponse;
import software.amazon.awssdk.services.devicefarm.model.CreateVpceConfigurationRequest;
import software.amazon.awssdk.services.devicefarm.model.CreateVpceConfigurationResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteDevicePoolRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteDevicePoolResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteInstanceProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteInstanceProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteNetworkProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteNetworkProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteRunRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteRunResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteTestGridProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteTestGridProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteUploadRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteUploadResponse;
import software.amazon.awssdk.services.devicefarm.model.DeleteVpceConfigurationRequest;
import software.amazon.awssdk.services.devicefarm.model.DeleteVpceConfigurationResponse;
import software.amazon.awssdk.services.devicefarm.model.DeviceFarmException;
import software.amazon.awssdk.services.devicefarm.model.GetAccountSettingsRequest;
import software.amazon.awssdk.services.devicefarm.model.GetAccountSettingsResponse;
import software.amazon.awssdk.services.devicefarm.model.GetDeviceInstanceRequest;
import software.amazon.awssdk.services.devicefarm.model.GetDeviceInstanceResponse;
import software.amazon.awssdk.services.devicefarm.model.GetDevicePoolCompatibilityRequest;
import software.amazon.awssdk.services.devicefarm.model.GetDevicePoolCompatibilityResponse;
import software.amazon.awssdk.services.devicefarm.model.GetDevicePoolRequest;
import software.amazon.awssdk.services.devicefarm.model.GetDevicePoolResponse;
import software.amazon.awssdk.services.devicefarm.model.GetDeviceRequest;
import software.amazon.awssdk.services.devicefarm.model.GetDeviceResponse;
import software.amazon.awssdk.services.devicefarm.model.GetInstanceProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.GetInstanceProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.GetJobRequest;
import software.amazon.awssdk.services.devicefarm.model.GetJobResponse;
import software.amazon.awssdk.services.devicefarm.model.GetNetworkProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.GetNetworkProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusRequest;
import software.amazon.awssdk.services.devicefarm.model.GetOfferingStatusResponse;
import software.amazon.awssdk.services.devicefarm.model.GetProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.GetProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.GetRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.GetRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.GetRunRequest;
import software.amazon.awssdk.services.devicefarm.model.GetRunResponse;
import software.amazon.awssdk.services.devicefarm.model.GetSuiteRequest;
import software.amazon.awssdk.services.devicefarm.model.GetSuiteResponse;
import software.amazon.awssdk.services.devicefarm.model.GetTestGridProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.GetTestGridProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.GetTestGridSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.GetTestGridSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.GetTestRequest;
import software.amazon.awssdk.services.devicefarm.model.GetTestResponse;
import software.amazon.awssdk.services.devicefarm.model.GetUploadRequest;
import software.amazon.awssdk.services.devicefarm.model.GetUploadResponse;
import software.amazon.awssdk.services.devicefarm.model.GetVpceConfigurationRequest;
import software.amazon.awssdk.services.devicefarm.model.GetVpceConfigurationResponse;
import software.amazon.awssdk.services.devicefarm.model.IdempotencyException;
import software.amazon.awssdk.services.devicefarm.model.InstallToRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.InstallToRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.InternalServiceException;
import software.amazon.awssdk.services.devicefarm.model.InvalidOperationException;
import software.amazon.awssdk.services.devicefarm.model.LimitExceededException;
import software.amazon.awssdk.services.devicefarm.model.ListArtifactsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListArtifactsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListDeviceInstancesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListDeviceInstancesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListDevicePoolsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListDevicesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListDevicesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListInstanceProfilesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListInstanceProfilesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListJobsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListJobsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListNetworkProfilesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListNetworkProfilesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingPromotionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingPromotionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingTransactionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListOfferingsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListProjectsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListProjectsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListRemoteAccessSessionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListRemoteAccessSessionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListRunsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListRunsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListSamplesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListSamplesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListSuitesRequest;
import software.amazon.awssdk.services.devicefarm.model.ListSuitesResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridProjectsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionActionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionArtifactsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestGridSessionsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListTestsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListTestsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListUniqueProblemsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListUploadsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListUploadsResponse;
import software.amazon.awssdk.services.devicefarm.model.ListVpceConfigurationsRequest;
import software.amazon.awssdk.services.devicefarm.model.ListVpceConfigurationsResponse;
import software.amazon.awssdk.services.devicefarm.model.NotEligibleException;
import software.amazon.awssdk.services.devicefarm.model.NotFoundException;
import software.amazon.awssdk.services.devicefarm.model.PurchaseOfferingRequest;
import software.amazon.awssdk.services.devicefarm.model.PurchaseOfferingResponse;
import software.amazon.awssdk.services.devicefarm.model.RenewOfferingRequest;
import software.amazon.awssdk.services.devicefarm.model.RenewOfferingResponse;
import software.amazon.awssdk.services.devicefarm.model.ScheduleRunRequest;
import software.amazon.awssdk.services.devicefarm.model.ScheduleRunResponse;
import software.amazon.awssdk.services.devicefarm.model.ServiceAccountException;
import software.amazon.awssdk.services.devicefarm.model.StopJobRequest;
import software.amazon.awssdk.services.devicefarm.model.StopJobResponse;
import software.amazon.awssdk.services.devicefarm.model.StopRemoteAccessSessionRequest;
import software.amazon.awssdk.services.devicefarm.model.StopRemoteAccessSessionResponse;
import software.amazon.awssdk.services.devicefarm.model.StopRunRequest;
import software.amazon.awssdk.services.devicefarm.model.StopRunResponse;
import software.amazon.awssdk.services.devicefarm.model.TagOperationException;
import software.amazon.awssdk.services.devicefarm.model.TagPolicyException;
import software.amazon.awssdk.services.devicefarm.model.TagResourceRequest;
import software.amazon.awssdk.services.devicefarm.model.TagResourceResponse;
import software.amazon.awssdk.services.devicefarm.model.TooManyTagsException;
import software.amazon.awssdk.services.devicefarm.model.UntagResourceRequest;
import software.amazon.awssdk.services.devicefarm.model.UntagResourceResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateDeviceInstanceRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateDeviceInstanceResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateDevicePoolRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateDevicePoolResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateInstanceProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateInstanceProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateNetworkProfileRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateNetworkProfileResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateTestGridProjectRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateTestGridProjectResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateUploadRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateUploadResponse;
import software.amazon.awssdk.services.devicefarm.model.UpdateVpceConfigurationRequest;
import software.amazon.awssdk.services.devicefarm.model.UpdateVpceConfigurationResponse;
import software.amazon.awssdk.services.devicefarm.transform.CreateDevicePoolRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.CreateInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.CreateNetworkProfileRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.CreateProjectRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.CreateRemoteAccessSessionRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.CreateTestGridProjectRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.CreateTestGridUrlRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.CreateUploadRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.CreateVpceConfigurationRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.DeleteDevicePoolRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.DeleteInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.DeleteNetworkProfileRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.DeleteProjectRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.DeleteRemoteAccessSessionRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.DeleteRunRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.DeleteTestGridProjectRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.DeleteUploadRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.DeleteVpceConfigurationRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetAccountSettingsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetDeviceInstanceRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetDevicePoolCompatibilityRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetDevicePoolRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetDeviceRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetJobRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetNetworkProfileRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetOfferingStatusRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetProjectRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetRemoteAccessSessionRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetRunRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetSuiteRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetTestGridProjectRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetTestGridSessionRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetTestRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetUploadRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.GetVpceConfigurationRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.InstallToRemoteAccessSessionRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListArtifactsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListDeviceInstancesRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListDevicePoolsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListDevicesRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListInstanceProfilesRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListJobsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListNetworkProfilesRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListOfferingPromotionsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListOfferingTransactionsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListOfferingsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListProjectsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListRemoteAccessSessionsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListRunsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListSamplesRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListSuitesRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListTestGridProjectsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListTestGridSessionActionsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListTestGridSessionArtifactsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListTestGridSessionsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListTestsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListUniqueProblemsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListUploadsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ListVpceConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.PurchaseOfferingRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.RenewOfferingRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.ScheduleRunRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.StopJobRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.StopRemoteAccessSessionRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.StopRunRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.UpdateDeviceInstanceRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.UpdateDevicePoolRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.UpdateInstanceProfileRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.UpdateNetworkProfileRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.UpdateProjectRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.UpdateTestGridProjectRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.UpdateUploadRequestMarshaller;
import software.amazon.awssdk.services.devicefarm.transform.UpdateVpceConfigurationRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link DeviceFarmAsyncClient}.
*
* @see DeviceFarmAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultDeviceFarmAsyncClient implements DeviceFarmAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultDeviceFarmAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final DeviceFarmServiceClientConfiguration serviceClientConfiguration;
protected DefaultDeviceFarmAsyncClient(DeviceFarmServiceClientConfiguration serviceClientConfiguration,
SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.serviceClientConfiguration = serviceClientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Creates a device pool.
*
*
* @param createDevicePoolRequest
* Represents a request to the create device pool operation.
* @return A Java Future containing the result of the CreateDevicePool operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.CreateDevicePool
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createDevicePool(CreateDevicePoolRequest createDevicePoolRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDevicePoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDevicePool");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDevicePoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDevicePool")
.withMarshaller(new CreateDevicePoolRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createDevicePoolRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a profile that can be applied to one or more private fleet device instances.
*
*
* @param createInstanceProfileRequest
* @return A Java Future containing the result of the CreateInstanceProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.CreateInstanceProfile
* @see AWS API Documentation
*/
@Override
public CompletableFuture createInstanceProfile(
CreateInstanceProfileRequest createInstanceProfileRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createInstanceProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateInstanceProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateInstanceProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateInstanceProfile")
.withMarshaller(new CreateInstanceProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createInstanceProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a network profile.
*
*
* @param createNetworkProfileRequest
* @return A Java Future containing the result of the CreateNetworkProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.CreateNetworkProfile
* @see AWS API Documentation
*/
@Override
public CompletableFuture createNetworkProfile(
CreateNetworkProfileRequest createNetworkProfileRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createNetworkProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateNetworkProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateNetworkProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateNetworkProfile")
.withMarshaller(new CreateNetworkProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createNetworkProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a project.
*
*
* @param createProjectRequest
* Represents a request to the create project operation.
* @return A Java Future containing the result of the CreateProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - TagOperationException The operation was not successful. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.CreateProject
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createProject(CreateProjectRequest createProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateProject")
.withMarshaller(new CreateProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createProjectRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Specifies and starts a remote access session.
*
*
* @param createRemoteAccessSessionRequest
* Creates and submits a request to start a remote access session.
* @return A Java Future containing the result of the CreateRemoteAccessSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.CreateRemoteAccessSession
* @see AWS API Documentation
*/
@Override
public CompletableFuture createRemoteAccessSession(
CreateRemoteAccessSessionRequest createRemoteAccessSessionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRemoteAccessSessionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRemoteAccessSession");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateRemoteAccessSessionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateRemoteAccessSession")
.withMarshaller(new CreateRemoteAccessSessionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createRemoteAccessSessionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a Selenium testing project. Projects are used to track TestGridSession instances.
*
*
* @param createTestGridProjectRequest
* @return A Java Future containing the result of the CreateTestGridProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - LimitExceededException A limit was exceeded.
* - InternalServiceException An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.CreateTestGridProject
* @see AWS API Documentation
*/
@Override
public CompletableFuture createTestGridProject(
CreateTestGridProjectRequest createTestGridProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createTestGridProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateTestGridProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateTestGridProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateTestGridProject")
.withMarshaller(new CreateTestGridProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createTestGridProjectRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a signed, short-term URL that can be passed to a Selenium RemoteWebDriver
constructor.
*
*
* @param createTestGridUrlRequest
* @return A Java Future containing the result of the CreateTestGridUrl operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The specified entity was not found.
* - ArgumentException An invalid argument was specified.
* - InternalServiceException An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.CreateTestGridUrl
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createTestGridUrl(CreateTestGridUrlRequest createTestGridUrlRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createTestGridUrlRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateTestGridUrl");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateTestGridUrlResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateTestGridUrl")
.withMarshaller(new CreateTestGridUrlRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createTestGridUrlRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Uploads an app or test scripts.
*
*
* @param createUploadRequest
* Represents a request to the create upload operation.
* @return A Java Future containing the result of the CreateUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.CreateUpload
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createUpload(CreateUploadRequest createUploadRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUploadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUpload");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateUploadResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateUpload").withMarshaller(new CreateUploadRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createUploadRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a configuration record in Device Farm for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param createVpceConfigurationRequest
* @return A Java Future containing the result of the CreateVPCEConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.CreateVPCEConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture createVPCEConfiguration(
CreateVpceConfigurationRequest createVpceConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVpceConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVPCEConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateVpceConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateVPCEConfiguration")
.withMarshaller(new CreateVpceConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createVpceConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a device pool given the pool ARN. Does not allow deletion of curated pools owned by the system.
*
*
* @param deleteDevicePoolRequest
* Represents a request to the delete device pool operation.
* @return A Java Future containing the result of the DeleteDevicePool operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.DeleteDevicePool
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteDevicePool(DeleteDevicePoolRequest deleteDevicePoolRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDevicePoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDevicePool");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteDevicePoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteDevicePool")
.withMarshaller(new DeleteDevicePoolRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteDevicePoolRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a profile that can be applied to one or more private device instances.
*
*
* @param deleteInstanceProfileRequest
* @return A Java Future containing the result of the DeleteInstanceProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.DeleteInstanceProfile
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteInstanceProfile(
DeleteInstanceProfileRequest deleteInstanceProfileRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteInstanceProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteInstanceProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteInstanceProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteInstanceProfile")
.withMarshaller(new DeleteInstanceProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteInstanceProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a network profile.
*
*
* @param deleteNetworkProfileRequest
* @return A Java Future containing the result of the DeleteNetworkProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.DeleteNetworkProfile
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteNetworkProfile(
DeleteNetworkProfileRequest deleteNetworkProfileRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteNetworkProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteNetworkProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteNetworkProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteNetworkProfile")
.withMarshaller(new DeleteNetworkProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteNetworkProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an AWS Device Farm project, given the project ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteProjectRequest
* Represents a request to the delete project operation.
* @return A Java Future containing the result of the DeleteProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.DeleteProject
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteProject(DeleteProjectRequest deleteProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteProject")
.withMarshaller(new DeleteProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteProjectRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a completed remote access session and its results.
*
*
* @param deleteRemoteAccessSessionRequest
* Represents the request to delete the specified remote access session.
* @return A Java Future containing the result of the DeleteRemoteAccessSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.DeleteRemoteAccessSession
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteRemoteAccessSession(
DeleteRemoteAccessSessionRequest deleteRemoteAccessSessionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRemoteAccessSessionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRemoteAccessSession");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteRemoteAccessSessionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteRemoteAccessSession")
.withMarshaller(new DeleteRemoteAccessSessionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteRemoteAccessSessionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the run, given the run ARN.
*
*
* Deleting this resource does not stop an in-progress run.
*
*
* @param deleteRunRequest
* Represents a request to the delete run operation.
* @return A Java Future containing the result of the DeleteRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.DeleteRun
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteRun(DeleteRunRequest deleteRunRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRunRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRun");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteRunResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteRun")
.withMarshaller(new DeleteRunRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(deleteRunRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a Selenium testing project and all content generated under it.
*
*
*
* You cannot undo this operation.
*
*
*
* You cannot delete a project if it has active sessions.
*
*
*
* @param deleteTestGridProjectRequest
* @return A Java Future containing the result of the DeleteTestGridProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The specified entity was not found.
* - ArgumentException An invalid argument was specified.
* - CannotDeleteException The requested object could not be deleted.
* - InternalServiceException An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.DeleteTestGridProject
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteTestGridProject(
DeleteTestGridProjectRequest deleteTestGridProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteTestGridProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteTestGridProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteTestGridProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteTestGridProject")
.withMarshaller(new DeleteTestGridProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteTestGridProjectRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an upload given the upload ARN.
*
*
* @param deleteUploadRequest
* Represents a request to the delete upload operation.
* @return A Java Future containing the result of the DeleteUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.DeleteUpload
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteUpload(DeleteUploadRequest deleteUploadRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUploadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUpload");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteUploadResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteUpload").withMarshaller(new DeleteUploadRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteUploadRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a configuration for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param deleteVpceConfigurationRequest
* @return A Java Future containing the result of the DeleteVPCEConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - ServiceAccountException There was a problem with the service account.
* - InvalidOperationException There was an error with the update request, or you do not have sufficient
* permissions to update this VPC endpoint configuration.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.DeleteVPCEConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteVPCEConfiguration(
DeleteVpceConfigurationRequest deleteVpceConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVpceConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVPCEConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteVpceConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteVPCEConfiguration")
.withMarshaller(new DeleteVpceConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteVpceConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the number of unmetered iOS or unmetered Android devices that have been purchased by the account.
*
*
* @param getAccountSettingsRequest
* Represents the request sent to retrieve the account settings.
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetAccountSettings
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getAccountSettings(GetAccountSettingsRequest getAccountSettingsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAccountSettingsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccountSettings");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAccountSettingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAccountSettings")
.withMarshaller(new GetAccountSettingsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getAccountSettingsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about a unique device type.
*
*
* @param getDeviceRequest
* Represents a request to the get device request.
* @return A Java Future containing the result of the GetDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetDevice
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getDevice(GetDeviceRequest getDeviceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeviceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDevice");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetDeviceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetDevice")
.withMarshaller(new GetDeviceRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getDeviceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about a device instance that belongs to a private device fleet.
*
*
* @param getDeviceInstanceRequest
* @return A Java Future containing the result of the GetDeviceInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetDeviceInstance
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getDeviceInstance(GetDeviceInstanceRequest getDeviceInstanceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDeviceInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDeviceInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDeviceInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDeviceInstance")
.withMarshaller(new GetDeviceInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getDeviceInstanceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about a device pool.
*
*
* @param getDevicePoolRequest
* Represents a request to the get device pool operation.
* @return A Java Future containing the result of the GetDevicePool operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetDevicePool
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getDevicePool(GetDevicePoolRequest getDevicePoolRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDevicePoolRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDevicePool");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetDevicePoolResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDevicePool")
.withMarshaller(new GetDevicePoolRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getDevicePoolRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about compatibility with a device pool.
*
*
* @param getDevicePoolCompatibilityRequest
* Represents a request to the get device pool compatibility operation.
* @return A Java Future containing the result of the GetDevicePoolCompatibility operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetDevicePoolCompatibility
* @see AWS API Documentation
*/
@Override
public CompletableFuture getDevicePoolCompatibility(
GetDevicePoolCompatibilityRequest getDevicePoolCompatibilityRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDevicePoolCompatibilityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDevicePoolCompatibility");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDevicePoolCompatibilityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDevicePoolCompatibility")
.withMarshaller(new GetDevicePoolCompatibilityRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getDevicePoolCompatibilityRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about the specified instance profile.
*
*
* @param getInstanceProfileRequest
* @return A Java Future containing the result of the GetInstanceProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetInstanceProfile
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getInstanceProfile(GetInstanceProfileRequest getInstanceProfileRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getInstanceProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetInstanceProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetInstanceProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetInstanceProfile")
.withMarshaller(new GetInstanceProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getInstanceProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about a job.
*
*
* @param getJobRequest
* Represents a request to the get job operation.
* @return A Java Future containing the result of the GetJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetJob
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getJob(GetJobRequest getJobRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetJob")
.withMarshaller(new GetJobRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about a network profile.
*
*
* @param getNetworkProfileRequest
* @return A Java Future containing the result of the GetNetworkProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetNetworkProfile
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getNetworkProfile(GetNetworkProfileRequest getNetworkProfileRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getNetworkProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetNetworkProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetNetworkProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetNetworkProfile")
.withMarshaller(new GetNetworkProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getNetworkProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the current status and future status of all offerings purchased by an AWS account. The response indicates
* how many offerings are currently available and the offerings that will be available in the next period. The API
* returns a NotEligible
error if the user is not permitted to invoke the operation. If you must be
* able to invoke this operation, contact [email protected].
*
*
* @param getOfferingStatusRequest
* Represents the request to retrieve the offering status for the specified customer or account.
* @return A Java Future containing the result of the GetOfferingStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - NotEligibleException Exception gets thrown when a user is not eligible to perform the specified
* transaction.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetOfferingStatus
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getOfferingStatus(GetOfferingStatusRequest getOfferingStatusRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getOfferingStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetOfferingStatus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetOfferingStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetOfferingStatus")
.withMarshaller(new GetOfferingStatusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getOfferingStatusRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about a project.
*
*
* @param getProjectRequest
* Represents a request to the get project operation.
* @return A Java Future containing the result of the GetProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetProject
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getProject(GetProjectRequest getProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetProject")
.withMarshaller(new GetProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getProjectRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a link to a currently running remote access session.
*
*
* @param getRemoteAccessSessionRequest
* Represents the request to get information about the specified remote access session.
* @return A Java Future containing the result of the GetRemoteAccessSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetRemoteAccessSession
* @see AWS API Documentation
*/
@Override
public CompletableFuture getRemoteAccessSession(
GetRemoteAccessSessionRequest getRemoteAccessSessionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRemoteAccessSessionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRemoteAccessSession");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRemoteAccessSessionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetRemoteAccessSession")
.withMarshaller(new GetRemoteAccessSessionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getRemoteAccessSessionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about a run.
*
*
* @param getRunRequest
* Represents a request to the get run operation.
* @return A Java Future containing the result of the GetRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetRun
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getRun(GetRunRequest getRunRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRunRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRun");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetRunResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetRun")
.withMarshaller(new GetRunRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getRunRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about a suite.
*
*
* @param getSuiteRequest
* Represents a request to the get suite operation.
* @return A Java Future containing the result of the GetSuite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetSuite
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getSuite(GetSuiteRequest getSuiteRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSuiteRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSuite");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetSuiteResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetSuite")
.withMarshaller(new GetSuiteRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getSuiteRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about a test.
*
*
* @param getTestRequest
* Represents a request to the get test operation.
* @return A Java Future containing the result of the GetTest operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetTest
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getTest(GetTestRequest getTestRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getTestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetTest");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetTestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetTest")
.withMarshaller(new GetTestRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getTestRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about a Selenium testing project.
*
*
* @param getTestGridProjectRequest
* @return A Java Future containing the result of the GetTestGridProject operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The specified entity was not found.
* - ArgumentException An invalid argument was specified.
* - InternalServiceException An internal exception was raised in the service. Contact [email protected] if you see this
* error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetTestGridProject
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getTestGridProject(GetTestGridProjectRequest getTestGridProjectRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getTestGridProjectRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetTestGridProject");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetTestGridProjectResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetTestGridProject")
.withMarshaller(new GetTestGridProjectRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getTestGridProjectRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* A session is an instance of a browser created through a RemoteWebDriver
with the URL from
* CreateTestGridUrlResult$url. You can use the following to look up sessions:
*
*
* -
*
* The session ARN (GetTestGridSessionRequest$sessionArn).
*
*
* -
*
* The project ARN and a session ID (GetTestGridSessionRequest$projectArn and
* GetTestGridSessionRequest$sessionId).
*
*
*
*
*
* @param getTestGridSessionRequest
* @return A Java Future containing the result of the GetTestGridSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException The specified entity was not found.
- ArgumentException An invalid argument
* was specified.
- InternalServiceException An internal exception was raised in the service. Contact
* [email protected] if you see this
* error.
- SdkException Base class for all exceptions that can be thrown by the SDK (both service
* and client). Can be used for catch all scenarios.
- SdkClientException If any client side error
* occurs such as an IO related failure, failure to get credentials, etc.
- DeviceFarmException Base
* class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
*
* @sample DeviceFarmAsyncClient.GetTestGridSession
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getTestGridSession(GetTestGridSessionRequest getTestGridSessionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getTestGridSessionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetTestGridSession");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetTestGridSessionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetTestGridSession")
.withMarshaller(new GetTestGridSessionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getTestGridSessionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about an upload.
*
*
* @param getUploadRequest
* Represents a request to the get upload operation.
* @return A Java Future containing the result of the GetUpload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - LimitExceededException A limit was exceeded.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetUpload
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getUpload(GetUploadRequest getUploadRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getUploadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetUpload");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetUploadResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetUpload")
.withMarshaller(new GetUploadRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(getUploadRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about the configuration settings for your Amazon Virtual Private Cloud (VPC) endpoint.
*
*
* @param getVpceConfigurationRequest
* @return A Java Future containing the result of the GetVPCEConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ArgumentException An invalid argument was specified.
* - NotFoundException The specified entity was not found.
* - ServiceAccountException There was a problem with the service account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - DeviceFarmException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample DeviceFarmAsyncClient.GetVPCEConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture getVPCEConfiguration(
GetVpceConfigurationRequest getVpceConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getVpceConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Device Farm");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetVPCEConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetVpceConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams