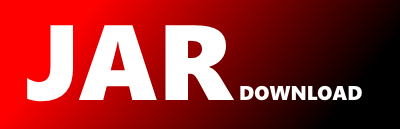
software.amazon.awssdk.services.devicefarm.model.CreateRemoteAccessSessionRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Creates and submits a request to start a remote access session.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateRemoteAccessSessionRequest extends DeviceFarmRequest implements
ToCopyableBuilder {
private static final SdkField PROJECT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("projectArn").getter(getter(CreateRemoteAccessSessionRequest::projectArn))
.setter(setter(Builder::projectArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("projectArn").build()).build();
private static final SdkField DEVICE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("deviceArn").getter(getter(CreateRemoteAccessSessionRequest::deviceArn))
.setter(setter(Builder::deviceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceArn").build()).build();
private static final SdkField INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("instanceArn").getter(getter(CreateRemoteAccessSessionRequest::instanceArn))
.setter(setter(Builder::instanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("instanceArn").build()).build();
private static final SdkField SSH_PUBLIC_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sshPublicKey").getter(getter(CreateRemoteAccessSessionRequest::sshPublicKey))
.setter(setter(Builder::sshPublicKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sshPublicKey").build()).build();
private static final SdkField REMOTE_DEBUG_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("remoteDebugEnabled").getter(getter(CreateRemoteAccessSessionRequest::remoteDebugEnabled))
.setter(setter(Builder::remoteDebugEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteDebugEnabled").build())
.build();
private static final SdkField REMOTE_RECORD_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("remoteRecordEnabled").getter(getter(CreateRemoteAccessSessionRequest::remoteRecordEnabled))
.setter(setter(Builder::remoteRecordEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteRecordEnabled").build())
.build();
private static final SdkField REMOTE_RECORD_APP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("remoteRecordAppArn").getter(getter(CreateRemoteAccessSessionRequest::remoteRecordAppArn))
.setter(setter(Builder::remoteRecordAppArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteRecordAppArn").build())
.build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(CreateRemoteAccessSessionRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField CLIENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clientId").getter(getter(CreateRemoteAccessSessionRequest::clientId)).setter(setter(Builder::clientId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientId").build()).build();
private static final SdkField CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("configuration")
.getter(getter(CreateRemoteAccessSessionRequest::configuration)).setter(setter(Builder::configuration))
.constructor(CreateRemoteAccessSessionConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("configuration").build()).build();
private static final SdkField INTERACTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("interactionMode").getter(getter(CreateRemoteAccessSessionRequest::interactionModeAsString))
.setter(setter(Builder::interactionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("interactionMode").build()).build();
private static final SdkField SKIP_APP_RESIGN_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("skipAppResign").getter(getter(CreateRemoteAccessSessionRequest::skipAppResign))
.setter(setter(Builder::skipAppResign))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("skipAppResign").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PROJECT_ARN_FIELD,
DEVICE_ARN_FIELD, INSTANCE_ARN_FIELD, SSH_PUBLIC_KEY_FIELD, REMOTE_DEBUG_ENABLED_FIELD, REMOTE_RECORD_ENABLED_FIELD,
REMOTE_RECORD_APP_ARN_FIELD, NAME_FIELD, CLIENT_ID_FIELD, CONFIGURATION_FIELD, INTERACTION_MODE_FIELD,
SKIP_APP_RESIGN_FIELD));
private final String projectArn;
private final String deviceArn;
private final String instanceArn;
private final String sshPublicKey;
private final Boolean remoteDebugEnabled;
private final Boolean remoteRecordEnabled;
private final String remoteRecordAppArn;
private final String name;
private final String clientId;
private final CreateRemoteAccessSessionConfiguration configuration;
private final String interactionMode;
private final Boolean skipAppResign;
private CreateRemoteAccessSessionRequest(BuilderImpl builder) {
super(builder);
this.projectArn = builder.projectArn;
this.deviceArn = builder.deviceArn;
this.instanceArn = builder.instanceArn;
this.sshPublicKey = builder.sshPublicKey;
this.remoteDebugEnabled = builder.remoteDebugEnabled;
this.remoteRecordEnabled = builder.remoteRecordEnabled;
this.remoteRecordAppArn = builder.remoteRecordAppArn;
this.name = builder.name;
this.clientId = builder.clientId;
this.configuration = builder.configuration;
this.interactionMode = builder.interactionMode;
this.skipAppResign = builder.skipAppResign;
}
/**
*
* The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*
*
* @return The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*/
public final String projectArn() {
return projectArn;
}
/**
*
* The ARN of the device for which you want to create a remote access session.
*
*
* @return The ARN of the device for which you want to create a remote access session.
*/
public final String deviceArn() {
return deviceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access session.
*
*
* @return The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access
* session.
*/
public final String instanceArn() {
return instanceArn;
}
/**
*
* Ignored. The public key of the ssh
key pair you want to use for connecting to remote devices in your
* remote debugging session. This key is required only if remoteDebugEnabled
is set to
* true
.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return Ignored. The public key of the ssh
key pair you want to use for connecting to remote devices
* in your remote debugging session. This key is required only if remoteDebugEnabled
is set to
* true
.
*
* Remote debugging is no longer supported.
*/
public final String sshPublicKey() {
return sshPublicKey;
}
/**
*
* Set to true
if you want to access devices remotely for debugging in your remote access session.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return Set to true
if you want to access devices remotely for debugging in your remote access
* session.
*
* Remote debugging is no longer supported.
*/
public final Boolean remoteDebugEnabled() {
return remoteDebugEnabled;
}
/**
*
* Set to true
to enable remote recording for the remote access session.
*
*
* @return Set to true
to enable remote recording for the remote access session.
*/
public final Boolean remoteRecordEnabled() {
return remoteRecordEnabled;
}
/**
*
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*
*
* @return The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*/
public final String remoteRecordAppArn() {
return remoteRecordAppArn;
}
/**
*
* The name of the remote access session to create.
*
*
* @return The name of the remote access session to create.
*/
public final String name() {
return name;
}
/**
*
* Unique identifier for the client. If you want access to multiple devices on the same client, you should pass the
* same clientId
value in each call to CreateRemoteAccessSession
. This identifier is
* required only if remoteDebugEnabled
is set to true
.
*
*
* Remote debugging is no longer
* supported.
*
*
* @return Unique identifier for the client. If you want access to multiple devices on the same client, you should
* pass the same clientId
value in each call to CreateRemoteAccessSession
. This
* identifier is required only if remoteDebugEnabled
is set to true
.
*
* Remote debugging is no longer supported.
*/
public final String clientId() {
return clientId;
}
/**
*
* The configuration information for the remote access session request.
*
*
* @return The configuration information for the remote access session request.
*/
public final CreateRemoteAccessSessionConfiguration configuration() {
return configuration;
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run
* XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests
* and watch the screen in this mode.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #interactionMode}
* will return {@link InteractionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #interactionModeAsString}.
*
*
* @return The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
* @see InteractionMode
*/
public final InteractionMode interactionMode() {
return InteractionMode.fromValue(interactionMode);
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run
* XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests
* and watch the screen in this mode.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #interactionMode}
* will return {@link InteractionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #interactionModeAsString}.
*
*
* @return The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
* @see InteractionMode
*/
public final String interactionModeAsString() {
return interactionMode;
}
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public devices,
* Device Farm always signs your apps again.
*
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*
*
* @return When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*/
public final Boolean skipAppResign() {
return skipAppResign;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(projectArn());
hashCode = 31 * hashCode + Objects.hashCode(deviceArn());
hashCode = 31 * hashCode + Objects.hashCode(instanceArn());
hashCode = 31 * hashCode + Objects.hashCode(sshPublicKey());
hashCode = 31 * hashCode + Objects.hashCode(remoteDebugEnabled());
hashCode = 31 * hashCode + Objects.hashCode(remoteRecordEnabled());
hashCode = 31 * hashCode + Objects.hashCode(remoteRecordAppArn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(clientId());
hashCode = 31 * hashCode + Objects.hashCode(configuration());
hashCode = 31 * hashCode + Objects.hashCode(interactionModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(skipAppResign());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateRemoteAccessSessionRequest)) {
return false;
}
CreateRemoteAccessSessionRequest other = (CreateRemoteAccessSessionRequest) obj;
return Objects.equals(projectArn(), other.projectArn()) && Objects.equals(deviceArn(), other.deviceArn())
&& Objects.equals(instanceArn(), other.instanceArn()) && Objects.equals(sshPublicKey(), other.sshPublicKey())
&& Objects.equals(remoteDebugEnabled(), other.remoteDebugEnabled())
&& Objects.equals(remoteRecordEnabled(), other.remoteRecordEnabled())
&& Objects.equals(remoteRecordAppArn(), other.remoteRecordAppArn()) && Objects.equals(name(), other.name())
&& Objects.equals(clientId(), other.clientId()) && Objects.equals(configuration(), other.configuration())
&& Objects.equals(interactionModeAsString(), other.interactionModeAsString())
&& Objects.equals(skipAppResign(), other.skipAppResign());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateRemoteAccessSessionRequest").add("ProjectArn", projectArn()).add("DeviceArn", deviceArn())
.add("InstanceArn", instanceArn()).add("SshPublicKey", sshPublicKey())
.add("RemoteDebugEnabled", remoteDebugEnabled()).add("RemoteRecordEnabled", remoteRecordEnabled())
.add("RemoteRecordAppArn", remoteRecordAppArn()).add("Name", name()).add("ClientId", clientId())
.add("Configuration", configuration()).add("InteractionMode", interactionModeAsString())
.add("SkipAppResign", skipAppResign()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "projectArn":
return Optional.ofNullable(clazz.cast(projectArn()));
case "deviceArn":
return Optional.ofNullable(clazz.cast(deviceArn()));
case "instanceArn":
return Optional.ofNullable(clazz.cast(instanceArn()));
case "sshPublicKey":
return Optional.ofNullable(clazz.cast(sshPublicKey()));
case "remoteDebugEnabled":
return Optional.ofNullable(clazz.cast(remoteDebugEnabled()));
case "remoteRecordEnabled":
return Optional.ofNullable(clazz.cast(remoteRecordEnabled()));
case "remoteRecordAppArn":
return Optional.ofNullable(clazz.cast(remoteRecordAppArn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "clientId":
return Optional.ofNullable(clazz.cast(clientId()));
case "configuration":
return Optional.ofNullable(clazz.cast(configuration()));
case "interactionMode":
return Optional.ofNullable(clazz.cast(interactionModeAsString()));
case "skipAppResign":
return Optional.ofNullable(clazz.cast(skipAppResign()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Remote debugging is no longer
* supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sshPublicKey(String sshPublicKey);
/**
*
* Set to true
if you want to access devices remotely for debugging in your remote access session.
*
*
* Remote debugging is no
* longer supported.
*
*
* @param remoteDebugEnabled
* Set to true
if you want to access devices remotely for debugging in your remote access
* session.
*
* Remote debugging is no longer
* supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder remoteDebugEnabled(Boolean remoteDebugEnabled);
/**
*
* Set to true
to enable remote recording for the remote access session.
*
*
* @param remoteRecordEnabled
* Set to true
to enable remote recording for the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder remoteRecordEnabled(Boolean remoteRecordEnabled);
/**
*
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*
*
* @param remoteRecordAppArn
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder remoteRecordAppArn(String remoteRecordAppArn);
/**
*
* The name of the remote access session to create.
*
*
* @param name
* The name of the remote access session to create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder name(String name);
/**
*
* Unique identifier for the client. If you want access to multiple devices on the same client, you should pass
* the same clientId
value in each call to CreateRemoteAccessSession
. This identifier
* is required only if remoteDebugEnabled
is set to true
.
*
*
* Remote debugging is no
* longer supported.
*
*
* @param clientId
* Unique identifier for the client. If you want access to multiple devices on the same client, you
* should pass the same clientId
value in each call to
* CreateRemoteAccessSession
. This identifier is required only if
* remoteDebugEnabled
is set to true
.
*
* Remote debugging is no longer
* supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientId(String clientId);
/**
*
* The configuration information for the remote access session request.
*
*
* @param configuration
* The configuration information for the remote access session request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder configuration(CreateRemoteAccessSessionConfiguration configuration);
/**
*
* The configuration information for the remote access session request.
*
* This is a convenience method that creates an instance of the
* {@link CreateRemoteAccessSessionConfiguration.Builder} avoiding the need to create one manually via
* {@link CreateRemoteAccessSessionConfiguration#builder()}.
*
*
* When the {@link Consumer} completes, {@link CreateRemoteAccessSessionConfiguration.Builder#build()} is called
* immediately and its result is passed to {@link #configuration(CreateRemoteAccessSessionConfiguration)}.
*
* @param configuration
* a consumer that will call methods on {@link CreateRemoteAccessSessionConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #configuration(CreateRemoteAccessSessionConfiguration)
*/
default Builder configuration(Consumer configuration) {
return configuration(CreateRemoteAccessSessionConfiguration.builder().applyMutation(configuration).build());
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot
* run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
*
*
* @param interactionMode
* The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode
* has the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest
* framework-based tests and watch the screen in this mode.
*
*
* @see InteractionMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see InteractionMode
*/
Builder interactionMode(String interactionMode);
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot
* run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
*
*
* @param interactionMode
* The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode
* has the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest
* framework-based tests and watch the screen in this mode.
*
*
* @see InteractionMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see InteractionMode
*/
Builder interactionMode(InteractionMode interactionMode);
/**
*
* When set to true
, for private devices, Device Farm does not sign your app again. For public
* devices, Device Farm always signs your apps again.
*
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
*
*
* @param skipAppResign
* When set to true
, for private devices, Device Farm does not sign your app again. For
* public devices, Device Farm always signs your apps again.
*
* For more information on how Device Farm modifies your uploads during tests, see Do you modify my app?
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder skipAppResign(Boolean skipAppResign);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends DeviceFarmRequest.BuilderImpl implements Builder {
private String projectArn;
private String deviceArn;
private String instanceArn;
private String sshPublicKey;
private Boolean remoteDebugEnabled;
private Boolean remoteRecordEnabled;
private String remoteRecordAppArn;
private String name;
private String clientId;
private CreateRemoteAccessSessionConfiguration configuration;
private String interactionMode;
private Boolean skipAppResign;
private BuilderImpl() {
}
private BuilderImpl(CreateRemoteAccessSessionRequest model) {
super(model);
projectArn(model.projectArn);
deviceArn(model.deviceArn);
instanceArn(model.instanceArn);
sshPublicKey(model.sshPublicKey);
remoteDebugEnabled(model.remoteDebugEnabled);
remoteRecordEnabled(model.remoteRecordEnabled);
remoteRecordAppArn(model.remoteRecordAppArn);
name(model.name);
clientId(model.clientId);
configuration(model.configuration);
interactionMode(model.interactionMode);
skipAppResign(model.skipAppResign);
}
public final String getProjectArn() {
return projectArn;
}
public final void setProjectArn(String projectArn) {
this.projectArn = projectArn;
}
@Override
public final Builder projectArn(String projectArn) {
this.projectArn = projectArn;
return this;
}
public final String getDeviceArn() {
return deviceArn;
}
public final void setDeviceArn(String deviceArn) {
this.deviceArn = deviceArn;
}
@Override
public final Builder deviceArn(String deviceArn) {
this.deviceArn = deviceArn;
return this;
}
public final String getInstanceArn() {
return instanceArn;
}
public final void setInstanceArn(String instanceArn) {
this.instanceArn = instanceArn;
}
@Override
public final Builder instanceArn(String instanceArn) {
this.instanceArn = instanceArn;
return this;
}
public final String getSshPublicKey() {
return sshPublicKey;
}
public final void setSshPublicKey(String sshPublicKey) {
this.sshPublicKey = sshPublicKey;
}
@Override
public final Builder sshPublicKey(String sshPublicKey) {
this.sshPublicKey = sshPublicKey;
return this;
}
public final Boolean getRemoteDebugEnabled() {
return remoteDebugEnabled;
}
public final void setRemoteDebugEnabled(Boolean remoteDebugEnabled) {
this.remoteDebugEnabled = remoteDebugEnabled;
}
@Override
public final Builder remoteDebugEnabled(Boolean remoteDebugEnabled) {
this.remoteDebugEnabled = remoteDebugEnabled;
return this;
}
public final Boolean getRemoteRecordEnabled() {
return remoteRecordEnabled;
}
public final void setRemoteRecordEnabled(Boolean remoteRecordEnabled) {
this.remoteRecordEnabled = remoteRecordEnabled;
}
@Override
public final Builder remoteRecordEnabled(Boolean remoteRecordEnabled) {
this.remoteRecordEnabled = remoteRecordEnabled;
return this;
}
public final String getRemoteRecordAppArn() {
return remoteRecordAppArn;
}
public final void setRemoteRecordAppArn(String remoteRecordAppArn) {
this.remoteRecordAppArn = remoteRecordAppArn;
}
@Override
public final Builder remoteRecordAppArn(String remoteRecordAppArn) {
this.remoteRecordAppArn = remoteRecordAppArn;
return this;
}
public final String getName() {
return name;
}
public final void setName(String name) {
this.name = name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final String getClientId() {
return clientId;
}
public final void setClientId(String clientId) {
this.clientId = clientId;
}
@Override
public final Builder clientId(String clientId) {
this.clientId = clientId;
return this;
}
public final CreateRemoteAccessSessionConfiguration.Builder getConfiguration() {
return configuration != null ? configuration.toBuilder() : null;
}
public final void setConfiguration(CreateRemoteAccessSessionConfiguration.BuilderImpl configuration) {
this.configuration = configuration != null ? configuration.build() : null;
}
@Override
public final Builder configuration(CreateRemoteAccessSessionConfiguration configuration) {
this.configuration = configuration;
return this;
}
public final String getInteractionMode() {
return interactionMode;
}
public final void setInteractionMode(String interactionMode) {
this.interactionMode = interactionMode;
}
@Override
public final Builder interactionMode(String interactionMode) {
this.interactionMode = interactionMode;
return this;
}
@Override
public final Builder interactionMode(InteractionMode interactionMode) {
this.interactionMode(interactionMode == null ? null : interactionMode.toString());
return this;
}
public final Boolean getSkipAppResign() {
return skipAppResign;
}
public final void setSkipAppResign(Boolean skipAppResign) {
this.skipAppResign = skipAppResign;
}
@Override
public final Builder skipAppResign(Boolean skipAppResign) {
this.skipAppResign = skipAppResign;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateRemoteAccessSessionRequest build() {
return new CreateRemoteAccessSessionRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}