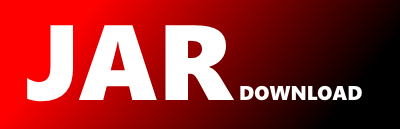
software.amazon.awssdk.services.devicefarm.model.ScheduleRunConfiguration Maven / Gradle / Ivy
Show all versions of devicefarm Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the settings for a run. Includes things like location, radio states, auxiliary apps, and network profiles.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ScheduleRunConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField EXTRA_DATA_PACKAGE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ScheduleRunConfiguration::extraDataPackageArn)).setter(setter(Builder::extraDataPackageArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("extraDataPackageArn").build())
.build();
private static final SdkField NETWORK_PROFILE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ScheduleRunConfiguration::networkProfileArn)).setter(setter(Builder::networkProfileArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkProfileArn").build()).build();
private static final SdkField LOCALE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ScheduleRunConfiguration::locale)).setter(setter(Builder::locale))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("locale").build()).build();
private static final SdkField LOCATION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(ScheduleRunConfiguration::location)).setter(setter(Builder::location)).constructor(Location::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("location").build()).build();
private static final SdkField> VPCE_CONFIGURATION_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ScheduleRunConfiguration::vpceConfigurationArns))
.setter(setter(Builder::vpceConfigurationArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vpceConfigurationArns").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CUSTOMER_ARTIFACT_PATHS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(ScheduleRunConfiguration::customerArtifactPaths)).setter(setter(Builder::customerArtifactPaths))
.constructor(CustomerArtifactPaths::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("customerArtifactPaths").build())
.build();
private static final SdkField RADIOS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(ScheduleRunConfiguration::radios)).setter(setter(Builder::radios)).constructor(Radios::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("radios").build()).build();
private static final SdkField> AUXILIARY_APPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ScheduleRunConfiguration::auxiliaryApps))
.setter(setter(Builder::auxiliaryApps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("auxiliaryApps").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField BILLING_METHOD_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ScheduleRunConfiguration::billingMethodAsString)).setter(setter(Builder::billingMethod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("billingMethod").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EXTRA_DATA_PACKAGE_ARN_FIELD,
NETWORK_PROFILE_ARN_FIELD, LOCALE_FIELD, LOCATION_FIELD, VPCE_CONFIGURATION_ARNS_FIELD,
CUSTOMER_ARTIFACT_PATHS_FIELD, RADIOS_FIELD, AUXILIARY_APPS_FIELD, BILLING_METHOD_FIELD));
private static final long serialVersionUID = 1L;
private final String extraDataPackageArn;
private final String networkProfileArn;
private final String locale;
private final Location location;
private final List vpceConfigurationArns;
private final CustomerArtifactPaths customerArtifactPaths;
private final Radios radios;
private final List auxiliaryApps;
private final String billingMethod;
private ScheduleRunConfiguration(BuilderImpl builder) {
this.extraDataPackageArn = builder.extraDataPackageArn;
this.networkProfileArn = builder.networkProfileArn;
this.locale = builder.locale;
this.location = builder.location;
this.vpceConfigurationArns = builder.vpceConfigurationArns;
this.customerArtifactPaths = builder.customerArtifactPaths;
this.radios = builder.radios;
this.auxiliaryApps = builder.auxiliaryApps;
this.billingMethod = builder.billingMethod;
}
/**
*
* The ARN of the extra data for the run. The extra data is a .zip file that AWS Device Farm will extract to
* external data for Android or the app's sandbox for iOS.
*
*
* @return The ARN of the extra data for the run. The extra data is a .zip file that AWS Device Farm will extract to
* external data for Android or the app's sandbox for iOS.
*/
public String extraDataPackageArn() {
return extraDataPackageArn;
}
/**
*
* Reserved for internal use.
*
*
* @return Reserved for internal use.
*/
public String networkProfileArn() {
return networkProfileArn;
}
/**
*
* Information about the locale that is used for the run.
*
*
* @return Information about the locale that is used for the run.
*/
public String locale() {
return locale;
}
/**
*
* Information about the location that is used for the run.
*
*
* @return Information about the location that is used for the run.
*/
public Location location() {
return location;
}
/**
*
* An array of Amazon Resource Names (ARNs) for your VPC endpoint configurations.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An array of Amazon Resource Names (ARNs) for your VPC endpoint configurations.
*/
public List vpceConfigurationArns() {
return vpceConfigurationArns;
}
/**
*
* Input CustomerArtifactPaths
object for the scheduled run configuration.
*
*
* @return Input CustomerArtifactPaths
object for the scheduled run configuration.
*/
public CustomerArtifactPaths customerArtifactPaths() {
return customerArtifactPaths;
}
/**
*
* Information about the radio states for the run.
*
*
* @return Information about the radio states for the run.
*/
public Radios radios() {
return radios;
}
/**
*
* A list of auxiliary apps for the run.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of auxiliary apps for the run.
*/
public List auxiliaryApps() {
return auxiliaryApps;
}
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMethod}
* will return {@link BillingMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingMethodAsString}.
*
*
* @return Specifies the billing method for a test run: metered
or unmetered
. If the
* parameter is not specified, the default value is metered
.
* @see BillingMethod
*/
public BillingMethod billingMethod() {
return BillingMethod.fromValue(billingMethod);
}
/**
*
* Specifies the billing method for a test run: metered
or unmetered
. If the parameter is
* not specified, the default value is metered
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMethod}
* will return {@link BillingMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingMethodAsString}.
*
*
* @return Specifies the billing method for a test run: metered
or unmetered
. If the
* parameter is not specified, the default value is metered
.
* @see BillingMethod
*/
public String billingMethodAsString() {
return billingMethod;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(extraDataPackageArn());
hashCode = 31 * hashCode + Objects.hashCode(networkProfileArn());
hashCode = 31 * hashCode + Objects.hashCode(locale());
hashCode = 31 * hashCode + Objects.hashCode(location());
hashCode = 31 * hashCode + Objects.hashCode(vpceConfigurationArns());
hashCode = 31 * hashCode + Objects.hashCode(customerArtifactPaths());
hashCode = 31 * hashCode + Objects.hashCode(radios());
hashCode = 31 * hashCode + Objects.hashCode(auxiliaryApps());
hashCode = 31 * hashCode + Objects.hashCode(billingMethodAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ScheduleRunConfiguration)) {
return false;
}
ScheduleRunConfiguration other = (ScheduleRunConfiguration) obj;
return Objects.equals(extraDataPackageArn(), other.extraDataPackageArn())
&& Objects.equals(networkProfileArn(), other.networkProfileArn()) && Objects.equals(locale(), other.locale())
&& Objects.equals(location(), other.location())
&& Objects.equals(vpceConfigurationArns(), other.vpceConfigurationArns())
&& Objects.equals(customerArtifactPaths(), other.customerArtifactPaths())
&& Objects.equals(radios(), other.radios()) && Objects.equals(auxiliaryApps(), other.auxiliaryApps())
&& Objects.equals(billingMethodAsString(), other.billingMethodAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ScheduleRunConfiguration").add("ExtraDataPackageArn", extraDataPackageArn())
.add("NetworkProfileArn", networkProfileArn()).add("Locale", locale()).add("Location", location())
.add("VpceConfigurationArns", vpceConfigurationArns()).add("CustomerArtifactPaths", customerArtifactPaths())
.add("Radios", radios()).add("AuxiliaryApps", auxiliaryApps()).add("BillingMethod", billingMethodAsString())
.build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "extraDataPackageArn":
return Optional.ofNullable(clazz.cast(extraDataPackageArn()));
case "networkProfileArn":
return Optional.ofNullable(clazz.cast(networkProfileArn()));
case "locale":
return Optional.ofNullable(clazz.cast(locale()));
case "location":
return Optional.ofNullable(clazz.cast(location()));
case "vpceConfigurationArns":
return Optional.ofNullable(clazz.cast(vpceConfigurationArns()));
case "customerArtifactPaths":
return Optional.ofNullable(clazz.cast(customerArtifactPaths()));
case "radios":
return Optional.ofNullable(clazz.cast(radios()));
case "auxiliaryApps":
return Optional.ofNullable(clazz.cast(auxiliaryApps()));
case "billingMethod":
return Optional.ofNullable(clazz.cast(billingMethodAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function