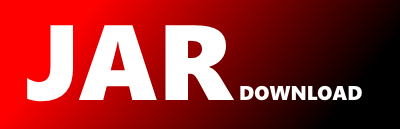
software.amazon.awssdk.services.devicefarm.model.RemoteAccessSession Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devicefarm.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents information about the remote access session.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RemoteAccessSession implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField CREATED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(RemoteAccessSession::created)).setter(setter(Builder::created))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("created").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField RESULT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::resultAsString)).setter(setter(Builder::result))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("result").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("message").build()).build();
private static final SdkField STARTED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(RemoteAccessSession::started)).setter(setter(Builder::started))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("started").build()).build();
private static final SdkField STOPPED_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(RemoteAccessSession::stopped)).setter(setter(Builder::stopped))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stopped").build()).build();
private static final SdkField DEVICE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(RemoteAccessSession::device)).setter(setter(Builder::device)).constructor(Device::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("device").build()).build();
private static final SdkField INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::instanceArn)).setter(setter(Builder::instanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("instanceArn").build()).build();
private static final SdkField REMOTE_DEBUG_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RemoteAccessSession::remoteDebugEnabled)).setter(setter(Builder::remoteDebugEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteDebugEnabled").build())
.build();
private static final SdkField REMOTE_RECORD_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RemoteAccessSession::remoteRecordEnabled)).setter(setter(Builder::remoteRecordEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteRecordEnabled").build())
.build();
private static final SdkField REMOTE_RECORD_APP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::remoteRecordAppArn)).setter(setter(Builder::remoteRecordAppArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remoteRecordAppArn").build())
.build();
private static final SdkField HOST_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::hostAddress)).setter(setter(Builder::hostAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("hostAddress").build()).build();
private static final SdkField CLIENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::clientId)).setter(setter(Builder::clientId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientId").build()).build();
private static final SdkField BILLING_METHOD_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::billingMethodAsString)).setter(setter(Builder::billingMethod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("billingMethod").build()).build();
private static final SdkField DEVICE_MINUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(RemoteAccessSession::deviceMinutes))
.setter(setter(Builder::deviceMinutes)).constructor(DeviceMinutes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceMinutes").build()).build();
private static final SdkField ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::endpoint)).setter(setter(Builder::endpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endpoint").build()).build();
private static final SdkField DEVICE_UDID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::deviceUdid)).setter(setter(Builder::deviceUdid))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceUdid").build()).build();
private static final SdkField INTERACTION_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RemoteAccessSession::interactionModeAsString)).setter(setter(Builder::interactionMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("interactionMode").build()).build();
private static final SdkField SKIP_APP_RESIGN_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RemoteAccessSession::skipAppResign)).setter(setter(Builder::skipAppResign))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("skipAppResign").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, NAME_FIELD,
CREATED_FIELD, STATUS_FIELD, RESULT_FIELD, MESSAGE_FIELD, STARTED_FIELD, STOPPED_FIELD, DEVICE_FIELD,
INSTANCE_ARN_FIELD, REMOTE_DEBUG_ENABLED_FIELD, REMOTE_RECORD_ENABLED_FIELD, REMOTE_RECORD_APP_ARN_FIELD,
HOST_ADDRESS_FIELD, CLIENT_ID_FIELD, BILLING_METHOD_FIELD, DEVICE_MINUTES_FIELD, ENDPOINT_FIELD, DEVICE_UDID_FIELD,
INTERACTION_MODE_FIELD, SKIP_APP_RESIGN_FIELD));
private static final long serialVersionUID = 1L;
private final String arn;
private final String name;
private final Instant created;
private final String status;
private final String result;
private final String message;
private final Instant started;
private final Instant stopped;
private final Device device;
private final String instanceArn;
private final Boolean remoteDebugEnabled;
private final Boolean remoteRecordEnabled;
private final String remoteRecordAppArn;
private final String hostAddress;
private final String clientId;
private final String billingMethod;
private final DeviceMinutes deviceMinutes;
private final String endpoint;
private final String deviceUdid;
private final String interactionMode;
private final Boolean skipAppResign;
private RemoteAccessSession(BuilderImpl builder) {
this.arn = builder.arn;
this.name = builder.name;
this.created = builder.created;
this.status = builder.status;
this.result = builder.result;
this.message = builder.message;
this.started = builder.started;
this.stopped = builder.stopped;
this.device = builder.device;
this.instanceArn = builder.instanceArn;
this.remoteDebugEnabled = builder.remoteDebugEnabled;
this.remoteRecordEnabled = builder.remoteRecordEnabled;
this.remoteRecordAppArn = builder.remoteRecordAppArn;
this.hostAddress = builder.hostAddress;
this.clientId = builder.clientId;
this.billingMethod = builder.billingMethod;
this.deviceMinutes = builder.deviceMinutes;
this.endpoint = builder.endpoint;
this.deviceUdid = builder.deviceUdid;
this.interactionMode = builder.interactionMode;
this.skipAppResign = builder.skipAppResign;
}
/**
*
* The Amazon Resource Name (ARN) of the remote access session.
*
*
* @return The Amazon Resource Name (ARN) of the remote access session.
*/
public String arn() {
return arn;
}
/**
*
* The name of the remote access session.
*
*
* @return The name of the remote access session.
*/
public String name() {
return name;
}
/**
*
* The date and time the remote access session was created.
*
*
* @return The date and time the remote access session was created.
*/
public Instant created() {
return created;
}
/**
*
* The status of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING: A pending status.
*
*
* -
*
* PENDING_CONCURRENCY: A pending concurrency status.
*
*
* -
*
* PENDING_DEVICE: A pending device status.
*
*
* -
*
* PROCESSING: A processing status.
*
*
* -
*
* SCHEDULING: A scheduling status.
*
*
* -
*
* PREPARING: A preparing status.
*
*
* -
*
* RUNNING: A running status.
*
*
* -
*
* COMPLETED: A completed status.
*
*
* -
*
* STOPPING: A stopping status.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the remote access session. Can be any of the following:
*
* -
*
* PENDING: A pending status.
*
*
* -
*
* PENDING_CONCURRENCY: A pending concurrency status.
*
*
* -
*
* PENDING_DEVICE: A pending device status.
*
*
* -
*
* PROCESSING: A processing status.
*
*
* -
*
* SCHEDULING: A scheduling status.
*
*
* -
*
* PREPARING: A preparing status.
*
*
* -
*
* RUNNING: A running status.
*
*
* -
*
* COMPLETED: A completed status.
*
*
* -
*
* STOPPING: A stopping status.
*
*
* @see ExecutionStatus
*/
public ExecutionStatus status() {
return ExecutionStatus.fromValue(status);
}
/**
*
* The status of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING: A pending status.
*
*
* -
*
* PENDING_CONCURRENCY: A pending concurrency status.
*
*
* -
*
* PENDING_DEVICE: A pending device status.
*
*
* -
*
* PROCESSING: A processing status.
*
*
* -
*
* SCHEDULING: A scheduling status.
*
*
* -
*
* PREPARING: A preparing status.
*
*
* -
*
* RUNNING: A running status.
*
*
* -
*
* COMPLETED: A completed status.
*
*
* -
*
* STOPPING: A stopping status.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the remote access session. Can be any of the following:
*
* -
*
* PENDING: A pending status.
*
*
* -
*
* PENDING_CONCURRENCY: A pending concurrency status.
*
*
* -
*
* PENDING_DEVICE: A pending device status.
*
*
* -
*
* PROCESSING: A processing status.
*
*
* -
*
* SCHEDULING: A scheduling status.
*
*
* -
*
* PREPARING: A preparing status.
*
*
* -
*
* RUNNING: A running status.
*
*
* -
*
* COMPLETED: A completed status.
*
*
* -
*
* STOPPING: A stopping status.
*
*
* @see ExecutionStatus
*/
public String statusAsString() {
return status;
}
/**
*
* The result of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING: A pending condition.
*
*
* -
*
* PASSED: A passing condition.
*
*
* -
*
* WARNED: A warning condition.
*
*
* -
*
* FAILED: A failed condition.
*
*
* -
*
* SKIPPED: A skipped condition.
*
*
* -
*
* ERRORED: An error condition.
*
*
* -
*
* STOPPED: A stopped condition.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The result of the remote access session. Can be any of the following:
*
* -
*
* PENDING: A pending condition.
*
*
* -
*
* PASSED: A passing condition.
*
*
* -
*
* WARNED: A warning condition.
*
*
* -
*
* FAILED: A failed condition.
*
*
* -
*
* SKIPPED: A skipped condition.
*
*
* -
*
* ERRORED: An error condition.
*
*
* -
*
* STOPPED: A stopped condition.
*
*
* @see ExecutionResult
*/
public ExecutionResult result() {
return ExecutionResult.fromValue(result);
}
/**
*
* The result of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING: A pending condition.
*
*
* -
*
* PASSED: A passing condition.
*
*
* -
*
* WARNED: A warning condition.
*
*
* -
*
* FAILED: A failed condition.
*
*
* -
*
* SKIPPED: A skipped condition.
*
*
* -
*
* ERRORED: An error condition.
*
*
* -
*
* STOPPED: A stopped condition.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #result} will
* return {@link ExecutionResult#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resultAsString}.
*
*
* @return The result of the remote access session. Can be any of the following:
*
* -
*
* PENDING: A pending condition.
*
*
* -
*
* PASSED: A passing condition.
*
*
* -
*
* WARNED: A warning condition.
*
*
* -
*
* FAILED: A failed condition.
*
*
* -
*
* SKIPPED: A skipped condition.
*
*
* -
*
* ERRORED: An error condition.
*
*
* -
*
* STOPPED: A stopped condition.
*
*
* @see ExecutionResult
*/
public String resultAsString() {
return result;
}
/**
*
* A message about the remote access session.
*
*
* @return A message about the remote access session.
*/
public String message() {
return message;
}
/**
*
* The date and time the remote access session was started.
*
*
* @return The date and time the remote access session was started.
*/
public Instant started() {
return started;
}
/**
*
* The date and time the remote access session was stopped.
*
*
* @return The date and time the remote access session was stopped.
*/
public Instant stopped() {
return stopped;
}
/**
*
* The device (phone or tablet) used in the remote access session.
*
*
* @return The device (phone or tablet) used in the remote access session.
*/
public Device device() {
return device;
}
/**
*
* The Amazon Resource Name (ARN) of the instance.
*
*
* @return The Amazon Resource Name (ARN) of the instance.
*/
public String instanceArn() {
return instanceArn;
}
/**
*
* This flag is set to true
if remote debugging is enabled for the remote access session.
*
*
* @return This flag is set to true
if remote debugging is enabled for the remote access session.
*/
public Boolean remoteDebugEnabled() {
return remoteDebugEnabled;
}
/**
*
* This flag is set to true
if remote recording is enabled for the remote access session.
*
*
* @return This flag is set to true
if remote recording is enabled for the remote access session.
*/
public Boolean remoteRecordEnabled() {
return remoteRecordEnabled;
}
/**
*
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*
*
* @return The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*/
public String remoteRecordAppArn() {
return remoteRecordAppArn;
}
/**
*
* IP address of the EC2 host where you need to connect to remotely debug devices. Only returned if remote debugging
* is enabled for the remote access session.
*
*
* @return IP address of the EC2 host where you need to connect to remotely debug devices. Only returned if remote
* debugging is enabled for the remote access session.
*/
public String hostAddress() {
return hostAddress;
}
/**
*
* Unique identifier of your client for the remote access session. Only returned if remote debugging is enabled for
* the remote access session.
*
*
* @return Unique identifier of your client for the remote access session. Only returned if remote debugging is
* enabled for the remote access session.
*/
public String clientId() {
return clientId;
}
/**
*
* The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS Device
* Farm terminology."
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMethod}
* will return {@link BillingMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingMethodAsString}.
*
*
* @return The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS
* Device Farm terminology."
* @see BillingMethod
*/
public BillingMethod billingMethod() {
return BillingMethod.fromValue(billingMethod);
}
/**
*
* The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS Device
* Farm terminology."
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMethod}
* will return {@link BillingMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingMethodAsString}.
*
*
* @return The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS
* Device Farm terminology."
* @see BillingMethod
*/
public String billingMethodAsString() {
return billingMethod;
}
/**
*
* The number of minutes a device is used in a remote access session (including setup and teardown minutes).
*
*
* @return The number of minutes a device is used in a remote access session (including setup and teardown minutes).
*/
public DeviceMinutes deviceMinutes() {
return deviceMinutes;
}
/**
*
* The endpoint for the remote access sesssion.
*
*
* @return The endpoint for the remote access sesssion.
*/
public String endpoint() {
return endpoint;
}
/**
*
* Unique device identifier for the remote device. Only returned if remote debugging is enabled for the remote
* access session.
*
*
* @return Unique device identifier for the remote device. Only returned if remote debugging is enabled for the
* remote access session.
*/
public String deviceUdid() {
return deviceUdid;
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #interactionMode}
* will return {@link InteractionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #interactionModeAsString}.
*
*
* @return The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen but cannot touch or rotate it. You can run XCUITest
* framework-based tests and watch the screen in this mode.
*
*
* @see InteractionMode
*/
public InteractionMode interactionMode() {
return InteractionMode.fromValue(interactionMode);
}
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen but cannot touch or rotate it. You can run XCUITest framework-based
* tests and watch the screen in this mode.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #interactionMode}
* will return {@link InteractionMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #interactionModeAsString}.
*
*
* @return The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device but cannot interact with it or view the screen. This mode has
* the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen but cannot touch or rotate it. You can run XCUITest
* framework-based tests and watch the screen in this mode.
*
*
* @see InteractionMode
*/
public String interactionModeAsString() {
return interactionMode;
}
/**
*
* When set to true
, for private devices, Device Farm will not sign your app again. For public devices,
* Device Farm always signs your apps again and this parameter has no effect.
*
*
* For more information about how Device Farm re-signs your app(s), see Do you modify my app? in the AWS Device Farm FAQs.
*
*
* @return When set to true
, for private devices, Device Farm will not sign your app again. For public
* devices, Device Farm always signs your apps again and this parameter has no effect.
*
* For more information about how Device Farm re-signs your app(s), see Do you modify my app? in the AWS Device Farm
* FAQs.
*/
public Boolean skipAppResign() {
return skipAppResign;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(created());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(resultAsString());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(started());
hashCode = 31 * hashCode + Objects.hashCode(stopped());
hashCode = 31 * hashCode + Objects.hashCode(device());
hashCode = 31 * hashCode + Objects.hashCode(instanceArn());
hashCode = 31 * hashCode + Objects.hashCode(remoteDebugEnabled());
hashCode = 31 * hashCode + Objects.hashCode(remoteRecordEnabled());
hashCode = 31 * hashCode + Objects.hashCode(remoteRecordAppArn());
hashCode = 31 * hashCode + Objects.hashCode(hostAddress());
hashCode = 31 * hashCode + Objects.hashCode(clientId());
hashCode = 31 * hashCode + Objects.hashCode(billingMethodAsString());
hashCode = 31 * hashCode + Objects.hashCode(deviceMinutes());
hashCode = 31 * hashCode + Objects.hashCode(endpoint());
hashCode = 31 * hashCode + Objects.hashCode(deviceUdid());
hashCode = 31 * hashCode + Objects.hashCode(interactionModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(skipAppResign());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RemoteAccessSession)) {
return false;
}
RemoteAccessSession other = (RemoteAccessSession) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(created(), other.created()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(resultAsString(), other.resultAsString()) && Objects.equals(message(), other.message())
&& Objects.equals(started(), other.started()) && Objects.equals(stopped(), other.stopped())
&& Objects.equals(device(), other.device()) && Objects.equals(instanceArn(), other.instanceArn())
&& Objects.equals(remoteDebugEnabled(), other.remoteDebugEnabled())
&& Objects.equals(remoteRecordEnabled(), other.remoteRecordEnabled())
&& Objects.equals(remoteRecordAppArn(), other.remoteRecordAppArn())
&& Objects.equals(hostAddress(), other.hostAddress()) && Objects.equals(clientId(), other.clientId())
&& Objects.equals(billingMethodAsString(), other.billingMethodAsString())
&& Objects.equals(deviceMinutes(), other.deviceMinutes()) && Objects.equals(endpoint(), other.endpoint())
&& Objects.equals(deviceUdid(), other.deviceUdid())
&& Objects.equals(interactionModeAsString(), other.interactionModeAsString())
&& Objects.equals(skipAppResign(), other.skipAppResign());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("RemoteAccessSession").add("Arn", arn()).add("Name", name()).add("Created", created())
.add("Status", statusAsString()).add("Result", resultAsString()).add("Message", message())
.add("Started", started()).add("Stopped", stopped()).add("Device", device()).add("InstanceArn", instanceArn())
.add("RemoteDebugEnabled", remoteDebugEnabled()).add("RemoteRecordEnabled", remoteRecordEnabled())
.add("RemoteRecordAppArn", remoteRecordAppArn()).add("HostAddress", hostAddress()).add("ClientId", clientId())
.add("BillingMethod", billingMethodAsString()).add("DeviceMinutes", deviceMinutes()).add("Endpoint", endpoint())
.add("DeviceUdid", deviceUdid()).add("InteractionMode", interactionModeAsString())
.add("SkipAppResign", skipAppResign()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "created":
return Optional.ofNullable(clazz.cast(created()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "result":
return Optional.ofNullable(clazz.cast(resultAsString()));
case "message":
return Optional.ofNullable(clazz.cast(message()));
case "started":
return Optional.ofNullable(clazz.cast(started()));
case "stopped":
return Optional.ofNullable(clazz.cast(stopped()));
case "device":
return Optional.ofNullable(clazz.cast(device()));
case "instanceArn":
return Optional.ofNullable(clazz.cast(instanceArn()));
case "remoteDebugEnabled":
return Optional.ofNullable(clazz.cast(remoteDebugEnabled()));
case "remoteRecordEnabled":
return Optional.ofNullable(clazz.cast(remoteRecordEnabled()));
case "remoteRecordAppArn":
return Optional.ofNullable(clazz.cast(remoteRecordAppArn()));
case "hostAddress":
return Optional.ofNullable(clazz.cast(hostAddress()));
case "clientId":
return Optional.ofNullable(clazz.cast(clientId()));
case "billingMethod":
return Optional.ofNullable(clazz.cast(billingMethodAsString()));
case "deviceMinutes":
return Optional.ofNullable(clazz.cast(deviceMinutes()));
case "endpoint":
return Optional.ofNullable(clazz.cast(endpoint()));
case "deviceUdid":
return Optional.ofNullable(clazz.cast(deviceUdid()));
case "interactionMode":
return Optional.ofNullable(clazz.cast(interactionModeAsString()));
case "skipAppResign":
return Optional.ofNullable(clazz.cast(skipAppResign()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* PENDING: A pending status.
*
*
* -
*
* PENDING_CONCURRENCY: A pending concurrency status.
*
*
* -
*
* PENDING_DEVICE: A pending device status.
*
*
* -
*
* PROCESSING: A processing status.
*
*
* -
*
* SCHEDULING: A scheduling status.
*
*
* -
*
* PREPARING: A preparing status.
*
*
* -
*
* RUNNING: A running status.
*
*
* -
*
* COMPLETED: A completed status.
*
*
* -
*
* STOPPING: A stopping status.
*
*
* @see ExecutionStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionStatus
*/
Builder status(String status);
/**
*
* The status of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING: A pending status.
*
*
* -
*
* PENDING_CONCURRENCY: A pending concurrency status.
*
*
* -
*
* PENDING_DEVICE: A pending device status.
*
*
* -
*
* PROCESSING: A processing status.
*
*
* -
*
* SCHEDULING: A scheduling status.
*
*
* -
*
* PREPARING: A preparing status.
*
*
* -
*
* RUNNING: A running status.
*
*
* -
*
* COMPLETED: A completed status.
*
*
* -
*
* STOPPING: A stopping status.
*
*
*
*
* @param status
* The status of the remote access session. Can be any of the following:
*
* -
*
* PENDING: A pending status.
*
*
* -
*
* PENDING_CONCURRENCY: A pending concurrency status.
*
*
* -
*
* PENDING_DEVICE: A pending device status.
*
*
* -
*
* PROCESSING: A processing status.
*
*
* -
*
* SCHEDULING: A scheduling status.
*
*
* -
*
* PREPARING: A preparing status.
*
*
* -
*
* RUNNING: A running status.
*
*
* -
*
* COMPLETED: A completed status.
*
*
* -
*
* STOPPING: A stopping status.
*
*
* @see ExecutionStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionStatus
*/
Builder status(ExecutionStatus status);
/**
*
* The result of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING: A pending condition.
*
*
* -
*
* PASSED: A passing condition.
*
*
* -
*
* WARNED: A warning condition.
*
*
* -
*
* FAILED: A failed condition.
*
*
* -
*
* SKIPPED: A skipped condition.
*
*
* -
*
* ERRORED: An error condition.
*
*
* -
*
* STOPPED: A stopped condition.
*
*
*
*
* @param result
* The result of the remote access session. Can be any of the following:
*
* -
*
* PENDING: A pending condition.
*
*
* -
*
* PASSED: A passing condition.
*
*
* -
*
* WARNED: A warning condition.
*
*
* -
*
* FAILED: A failed condition.
*
*
* -
*
* SKIPPED: A skipped condition.
*
*
* -
*
* ERRORED: An error condition.
*
*
* -
*
* STOPPED: A stopped condition.
*
*
* @see ExecutionResult
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionResult
*/
Builder result(String result);
/**
*
* The result of the remote access session. Can be any of the following:
*
*
* -
*
* PENDING: A pending condition.
*
*
* -
*
* PASSED: A passing condition.
*
*
* -
*
* WARNED: A warning condition.
*
*
* -
*
* FAILED: A failed condition.
*
*
* -
*
* SKIPPED: A skipped condition.
*
*
* -
*
* ERRORED: An error condition.
*
*
* -
*
* STOPPED: A stopped condition.
*
*
*
*
* @param result
* The result of the remote access session. Can be any of the following:
*
* -
*
* PENDING: A pending condition.
*
*
* -
*
* PASSED: A passing condition.
*
*
* -
*
* WARNED: A warning condition.
*
*
* -
*
* FAILED: A failed condition.
*
*
* -
*
* SKIPPED: A skipped condition.
*
*
* -
*
* ERRORED: An error condition.
*
*
* -
*
* STOPPED: A stopped condition.
*
*
* @see ExecutionResult
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExecutionResult
*/
Builder result(ExecutionResult result);
/**
*
* A message about the remote access session.
*
*
* @param message
* A message about the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder message(String message);
/**
*
* The date and time the remote access session was started.
*
*
* @param started
* The date and time the remote access session was started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder started(Instant started);
/**
*
* The date and time the remote access session was stopped.
*
*
* @param stopped
* The date and time the remote access session was stopped.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder stopped(Instant stopped);
/**
*
* The device (phone or tablet) used in the remote access session.
*
*
* @param device
* The device (phone or tablet) used in the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder device(Device device);
/**
*
* The device (phone or tablet) used in the remote access session.
*
* This is a convenience that creates an instance of the {@link Device.Builder} avoiding the need to create one
* manually via {@link Device#builder()}.
*
* When the {@link Consumer} completes, {@link Device.Builder#build()} is called immediately and its result is
* passed to {@link #device(Device)}.
*
* @param device
* a consumer that will call methods on {@link Device.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #device(Device)
*/
default Builder device(Consumer device) {
return device(Device.builder().applyMutation(device).build());
}
/**
*
* The Amazon Resource Name (ARN) of the instance.
*
*
* @param instanceArn
* The Amazon Resource Name (ARN) of the instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder instanceArn(String instanceArn);
/**
*
* This flag is set to true
if remote debugging is enabled for the remote access session.
*
*
* @param remoteDebugEnabled
* This flag is set to true
if remote debugging is enabled for the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder remoteDebugEnabled(Boolean remoteDebugEnabled);
/**
*
* This flag is set to true
if remote recording is enabled for the remote access session.
*
*
* @param remoteRecordEnabled
* This flag is set to true
if remote recording is enabled for the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder remoteRecordEnabled(Boolean remoteRecordEnabled);
/**
*
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*
*
* @param remoteRecordAppArn
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder remoteRecordAppArn(String remoteRecordAppArn);
/**
*
* IP address of the EC2 host where you need to connect to remotely debug devices. Only returned if remote
* debugging is enabled for the remote access session.
*
*
* @param hostAddress
* IP address of the EC2 host where you need to connect to remotely debug devices. Only returned if
* remote debugging is enabled for the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder hostAddress(String hostAddress);
/**
*
* Unique identifier of your client for the remote access session. Only returned if remote debugging is enabled
* for the remote access session.
*
*
* @param clientId
* Unique identifier of your client for the remote access session. Only returned if remote debugging is
* enabled for the remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientId(String clientId);
/**
*
* The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS
* Device Farm terminology."
*
*
* @param billingMethod
* The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS Device Farm terminology."
* @see BillingMethod
* @return Returns a reference to this object so that method calls can be chained together.
* @see BillingMethod
*/
Builder billingMethod(String billingMethod);
/**
*
* The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS
* Device Farm terminology."
*
*
* @param billingMethod
* The billing method of the remote access session. Possible values include METERED
or
* UNMETERED
. For more information about metered devices, see AWS Device Farm terminology."
* @see BillingMethod
* @return Returns a reference to this object so that method calls can be chained together.
* @see BillingMethod
*/
Builder billingMethod(BillingMethod billingMethod);
/**
*
* The number of minutes a device is used in a remote access session (including setup and teardown minutes).
*
*
* @param deviceMinutes
* The number of minutes a device is used in a remote access session (including setup and teardown
* minutes).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder deviceMinutes(DeviceMinutes deviceMinutes);
/**
*
* The number of minutes a device is used in a remote access session (including setup and teardown minutes).
*
* This is a convenience that creates an instance of the {@link DeviceMinutes.Builder} avoiding the need to
* create one manually via {@link DeviceMinutes#builder()}.
*
* When the {@link Consumer} completes, {@link DeviceMinutes.Builder#build()} is called immediately and its
* result is passed to {@link #deviceMinutes(DeviceMinutes)}.
*
* @param deviceMinutes
* a consumer that will call methods on {@link DeviceMinutes.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #deviceMinutes(DeviceMinutes)
*/
default Builder deviceMinutes(Consumer deviceMinutes) {
return deviceMinutes(DeviceMinutes.builder().applyMutation(deviceMinutes).build());
}
/**
*
* The endpoint for the remote access sesssion.
*
*
* @param endpoint
* The endpoint for the remote access sesssion.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder endpoint(String endpoint);
/**
*
* Unique device identifier for the remote device. Only returned if remote debugging is enabled for the remote
* access session.
*
*
* @param deviceUdid
* Unique device identifier for the remote device. Only returned if remote debugging is enabled for the
* remote access session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder deviceUdid(String deviceUdid);
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen but cannot touch or rotate it. You can run XCUITest
* framework-based tests and watch the screen in this mode.
*
*
*
*
* @param interactionMode
* The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device but cannot interact with it or view the screen. This mode
* has the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen but cannot touch or rotate it. You can run XCUITest
* framework-based tests and watch the screen in this mode.
*
*
* @see InteractionMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see InteractionMode
*/
Builder interactionMode(String interactionMode);
/**
*
* The interaction mode of the remote access session. Valid values are:
*
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device but cannot interact with it or view the screen. This mode has the
* fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen but cannot touch or rotate it. You can run XCUITest
* framework-based tests and watch the screen in this mode.
*
*
*
*
* @param interactionMode
* The interaction mode of the remote access session. Valid values are:
*
* -
*
* INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You
* cannot run XCUITest framework-based tests in this mode.
*
*
* -
*
* NO_VIDEO: You are connected to the device but cannot interact with it or view the screen. This mode
* has the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
*
*
* -
*
* VIDEO_ONLY: You can view the screen but cannot touch or rotate it. You can run XCUITest
* framework-based tests and watch the screen in this mode.
*
*
* @see InteractionMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see InteractionMode
*/
Builder interactionMode(InteractionMode interactionMode);
/**
*
* When set to true
, for private devices, Device Farm will not sign your app again. For public
* devices, Device Farm always signs your apps again and this parameter has no effect.
*
*
* For more information about how Device Farm re-signs your app(s), see Do you modify my app? in the AWS Device Farm FAQs.
*
*
* @param skipAppResign
* When set to true
, for private devices, Device Farm will not sign your app again. For
* public devices, Device Farm always signs your apps again and this parameter has no effect.
*
* For more information about how Device Farm re-signs your app(s), see Do you modify my app? in the AWS Device Farm
* FAQs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder skipAppResign(Boolean skipAppResign);
}
static final class BuilderImpl implements Builder {
private String arn;
private String name;
private Instant created;
private String status;
private String result;
private String message;
private Instant started;
private Instant stopped;
private Device device;
private String instanceArn;
private Boolean remoteDebugEnabled;
private Boolean remoteRecordEnabled;
private String remoteRecordAppArn;
private String hostAddress;
private String clientId;
private String billingMethod;
private DeviceMinutes deviceMinutes;
private String endpoint;
private String deviceUdid;
private String interactionMode;
private Boolean skipAppResign;
private BuilderImpl() {
}
private BuilderImpl(RemoteAccessSession model) {
arn(model.arn);
name(model.name);
created(model.created);
status(model.status);
result(model.result);
message(model.message);
started(model.started);
stopped(model.stopped);
device(model.device);
instanceArn(model.instanceArn);
remoteDebugEnabled(model.remoteDebugEnabled);
remoteRecordEnabled(model.remoteRecordEnabled);
remoteRecordAppArn(model.remoteRecordAppArn);
hostAddress(model.hostAddress);
clientId(model.clientId);
billingMethod(model.billingMethod);
deviceMinutes(model.deviceMinutes);
endpoint(model.endpoint);
deviceUdid(model.deviceUdid);
interactionMode(model.interactionMode);
skipAppResign(model.skipAppResign);
}
public final String getArn() {
return arn;
}
@Override
public final Builder arn(String arn) {
this.arn = arn;
return this;
}
public final void setArn(String arn) {
this.arn = arn;
}
public final String getName() {
return name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final void setName(String name) {
this.name = name;
}
public final Instant getCreated() {
return created;
}
@Override
public final Builder created(Instant created) {
this.created = created;
return this;
}
public final void setCreated(Instant created) {
this.created = created;
}
public final String getStatusAsString() {
return status;
}
@Override
public final Builder status(String status) {
this.status = status;
return this;
}
@Override
public final Builder status(ExecutionStatus status) {
this.status(status == null ? null : status.toString());
return this;
}
public final void setStatus(String status) {
this.status = status;
}
public final String getResultAsString() {
return result;
}
@Override
public final Builder result(String result) {
this.result = result;
return this;
}
@Override
public final Builder result(ExecutionResult result) {
this.result(result == null ? null : result.toString());
return this;
}
public final void setResult(String result) {
this.result = result;
}
public final String getMessage() {
return message;
}
@Override
public final Builder message(String message) {
this.message = message;
return this;
}
public final void setMessage(String message) {
this.message = message;
}
public final Instant getStarted() {
return started;
}
@Override
public final Builder started(Instant started) {
this.started = started;
return this;
}
public final void setStarted(Instant started) {
this.started = started;
}
public final Instant getStopped() {
return stopped;
}
@Override
public final Builder stopped(Instant stopped) {
this.stopped = stopped;
return this;
}
public final void setStopped(Instant stopped) {
this.stopped = stopped;
}
public final Device.Builder getDevice() {
return device != null ? device.toBuilder() : null;
}
@Override
public final Builder device(Device device) {
this.device = device;
return this;
}
public final void setDevice(Device.BuilderImpl device) {
this.device = device != null ? device.build() : null;
}
public final String getInstanceArn() {
return instanceArn;
}
@Override
public final Builder instanceArn(String instanceArn) {
this.instanceArn = instanceArn;
return this;
}
public final void setInstanceArn(String instanceArn) {
this.instanceArn = instanceArn;
}
public final Boolean getRemoteDebugEnabled() {
return remoteDebugEnabled;
}
@Override
public final Builder remoteDebugEnabled(Boolean remoteDebugEnabled) {
this.remoteDebugEnabled = remoteDebugEnabled;
return this;
}
public final void setRemoteDebugEnabled(Boolean remoteDebugEnabled) {
this.remoteDebugEnabled = remoteDebugEnabled;
}
public final Boolean getRemoteRecordEnabled() {
return remoteRecordEnabled;
}
@Override
public final Builder remoteRecordEnabled(Boolean remoteRecordEnabled) {
this.remoteRecordEnabled = remoteRecordEnabled;
return this;
}
public final void setRemoteRecordEnabled(Boolean remoteRecordEnabled) {
this.remoteRecordEnabled = remoteRecordEnabled;
}
public final String getRemoteRecordAppArn() {
return remoteRecordAppArn;
}
@Override
public final Builder remoteRecordAppArn(String remoteRecordAppArn) {
this.remoteRecordAppArn = remoteRecordAppArn;
return this;
}
public final void setRemoteRecordAppArn(String remoteRecordAppArn) {
this.remoteRecordAppArn = remoteRecordAppArn;
}
public final String getHostAddress() {
return hostAddress;
}
@Override
public final Builder hostAddress(String hostAddress) {
this.hostAddress = hostAddress;
return this;
}
public final void setHostAddress(String hostAddress) {
this.hostAddress = hostAddress;
}
public final String getClientId() {
return clientId;
}
@Override
public final Builder clientId(String clientId) {
this.clientId = clientId;
return this;
}
public final void setClientId(String clientId) {
this.clientId = clientId;
}
public final String getBillingMethodAsString() {
return billingMethod;
}
@Override
public final Builder billingMethod(String billingMethod) {
this.billingMethod = billingMethod;
return this;
}
@Override
public final Builder billingMethod(BillingMethod billingMethod) {
this.billingMethod(billingMethod == null ? null : billingMethod.toString());
return this;
}
public final void setBillingMethod(String billingMethod) {
this.billingMethod = billingMethod;
}
public final DeviceMinutes.Builder getDeviceMinutes() {
return deviceMinutes != null ? deviceMinutes.toBuilder() : null;
}
@Override
public final Builder deviceMinutes(DeviceMinutes deviceMinutes) {
this.deviceMinutes = deviceMinutes;
return this;
}
public final void setDeviceMinutes(DeviceMinutes.BuilderImpl deviceMinutes) {
this.deviceMinutes = deviceMinutes != null ? deviceMinutes.build() : null;
}
public final String getEndpoint() {
return endpoint;
}
@Override
public final Builder endpoint(String endpoint) {
this.endpoint = endpoint;
return this;
}
public final void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
public final String getDeviceUdid() {
return deviceUdid;
}
@Override
public final Builder deviceUdid(String deviceUdid) {
this.deviceUdid = deviceUdid;
return this;
}
public final void setDeviceUdid(String deviceUdid) {
this.deviceUdid = deviceUdid;
}
public final String getInteractionModeAsString() {
return interactionMode;
}
@Override
public final Builder interactionMode(String interactionMode) {
this.interactionMode = interactionMode;
return this;
}
@Override
public final Builder interactionMode(InteractionMode interactionMode) {
this.interactionMode(interactionMode == null ? null : interactionMode.toString());
return this;
}
public final void setInteractionMode(String interactionMode) {
this.interactionMode = interactionMode;
}
public final Boolean getSkipAppResign() {
return skipAppResign;
}
@Override
public final Builder skipAppResign(Boolean skipAppResign) {
this.skipAppResign = skipAppResign;
return this;
}
public final void setSkipAppResign(Boolean skipAppResign) {
this.skipAppResign = skipAppResign;
}
@Override
public RemoteAccessSession build() {
return new RemoteAccessSession(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}