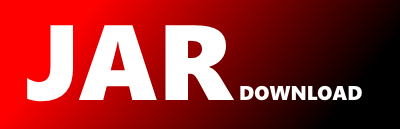
software.amazon.awssdk.services.devopsguru.model.ProactiveOrganizationInsightSummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.devopsguru.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details about a proactive insight. This object is returned by DescribeInsight
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ProactiveOrganizationInsightSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(ProactiveOrganizationInsightSummary::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AccountId").getter(getter(ProactiveOrganizationInsightSummary::accountId))
.setter(setter(Builder::accountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountId").build()).build();
private static final SdkField ORGANIZATIONAL_UNIT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OrganizationalUnitId").getter(getter(ProactiveOrganizationInsightSummary::organizationalUnitId))
.setter(setter(Builder::organizationalUnitId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OrganizationalUnitId").build())
.build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(ProactiveOrganizationInsightSummary::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField SEVERITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Severity").getter(getter(ProactiveOrganizationInsightSummary::severityAsString))
.setter(setter(Builder::severity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Severity").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ProactiveOrganizationInsightSummary::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField INSIGHT_TIME_RANGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InsightTimeRange")
.getter(getter(ProactiveOrganizationInsightSummary::insightTimeRange)).setter(setter(Builder::insightTimeRange))
.constructor(InsightTimeRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InsightTimeRange").build()).build();
private static final SdkField PREDICTION_TIME_RANGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("PredictionTimeRange")
.getter(getter(ProactiveOrganizationInsightSummary::predictionTimeRange))
.setter(setter(Builder::predictionTimeRange)).constructor(PredictionTimeRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PredictionTimeRange").build())
.build();
private static final SdkField RESOURCE_COLLECTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ResourceCollection")
.getter(getter(ProactiveOrganizationInsightSummary::resourceCollection)).setter(setter(Builder::resourceCollection))
.constructor(ResourceCollection::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceCollection").build())
.build();
private static final SdkField SERVICE_COLLECTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ServiceCollection")
.getter(getter(ProactiveOrganizationInsightSummary::serviceCollection)).setter(setter(Builder::serviceCollection))
.constructor(ServiceCollection::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceCollection").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, ACCOUNT_ID_FIELD,
ORGANIZATIONAL_UNIT_ID_FIELD, NAME_FIELD, SEVERITY_FIELD, STATUS_FIELD, INSIGHT_TIME_RANGE_FIELD,
PREDICTION_TIME_RANGE_FIELD, RESOURCE_COLLECTION_FIELD, SERVICE_COLLECTION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Id", ID_FIELD);
put("AccountId", ACCOUNT_ID_FIELD);
put("OrganizationalUnitId", ORGANIZATIONAL_UNIT_ID_FIELD);
put("Name", NAME_FIELD);
put("Severity", SEVERITY_FIELD);
put("Status", STATUS_FIELD);
put("InsightTimeRange", INSIGHT_TIME_RANGE_FIELD);
put("PredictionTimeRange", PREDICTION_TIME_RANGE_FIELD);
put("ResourceCollection", RESOURCE_COLLECTION_FIELD);
put("ServiceCollection", SERVICE_COLLECTION_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String id;
private final String accountId;
private final String organizationalUnitId;
private final String name;
private final String severity;
private final String status;
private final InsightTimeRange insightTimeRange;
private final PredictionTimeRange predictionTimeRange;
private final ResourceCollection resourceCollection;
private final ServiceCollection serviceCollection;
private ProactiveOrganizationInsightSummary(BuilderImpl builder) {
this.id = builder.id;
this.accountId = builder.accountId;
this.organizationalUnitId = builder.organizationalUnitId;
this.name = builder.name;
this.severity = builder.severity;
this.status = builder.status;
this.insightTimeRange = builder.insightTimeRange;
this.predictionTimeRange = builder.predictionTimeRange;
this.resourceCollection = builder.resourceCollection;
this.serviceCollection = builder.serviceCollection;
}
/**
*
* The ID of the insight summary.
*
*
* @return The ID of the insight summary.
*/
public final String id() {
return id;
}
/**
*
* The ID of the Amazon Web Services account.
*
*
* @return The ID of the Amazon Web Services account.
*/
public final String accountId() {
return accountId;
}
/**
*
* The ID of the organizational unit.
*
*
* @return The ID of the organizational unit.
*/
public final String organizationalUnitId() {
return organizationalUnitId;
}
/**
*
* The name of the insight summary.
*
*
* @return The name of the insight summary.
*/
public final String name() {
return name;
}
/**
*
* An array of severity values used to search for insights. For more information, see Understanding insight severities in the Amazon DevOps Guru User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #severity} will
* return {@link InsightSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #severityAsString}.
*
*
* @return An array of severity values used to search for insights. For more information, see Understanding insight severities in the Amazon DevOps Guru User Guide.
* @see InsightSeverity
*/
public final InsightSeverity severity() {
return InsightSeverity.fromValue(severity);
}
/**
*
* An array of severity values used to search for insights. For more information, see Understanding insight severities in the Amazon DevOps Guru User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #severity} will
* return {@link InsightSeverity#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #severityAsString}.
*
*
* @return An array of severity values used to search for insights. For more information, see Understanding insight severities in the Amazon DevOps Guru User Guide.
* @see InsightSeverity
*/
public final String severityAsString() {
return severity;
}
/**
*
* An array of status values used to search for insights.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link InsightStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return An array of status values used to search for insights.
* @see InsightStatus
*/
public final InsightStatus status() {
return InsightStatus.fromValue(status);
}
/**
*
* An array of status values used to search for insights.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link InsightStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return An array of status values used to search for insights.
* @see InsightStatus
*/
public final String statusAsString() {
return status;
}
/**
* Returns the value of the InsightTimeRange property for this object.
*
* @return The value of the InsightTimeRange property for this object.
*/
public final InsightTimeRange insightTimeRange() {
return insightTimeRange;
}
/**
* Returns the value of the PredictionTimeRange property for this object.
*
* @return The value of the PredictionTimeRange property for this object.
*/
public final PredictionTimeRange predictionTimeRange() {
return predictionTimeRange;
}
/**
* Returns the value of the ResourceCollection property for this object.
*
* @return The value of the ResourceCollection property for this object.
*/
public final ResourceCollection resourceCollection() {
return resourceCollection;
}
/**
* Returns the value of the ServiceCollection property for this object.
*
* @return The value of the ServiceCollection property for this object.
*/
public final ServiceCollection serviceCollection() {
return serviceCollection;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(accountId());
hashCode = 31 * hashCode + Objects.hashCode(organizationalUnitId());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(severityAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(insightTimeRange());
hashCode = 31 * hashCode + Objects.hashCode(predictionTimeRange());
hashCode = 31 * hashCode + Objects.hashCode(resourceCollection());
hashCode = 31 * hashCode + Objects.hashCode(serviceCollection());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ProactiveOrganizationInsightSummary)) {
return false;
}
ProactiveOrganizationInsightSummary other = (ProactiveOrganizationInsightSummary) obj;
return Objects.equals(id(), other.id()) && Objects.equals(accountId(), other.accountId())
&& Objects.equals(organizationalUnitId(), other.organizationalUnitId()) && Objects.equals(name(), other.name())
&& Objects.equals(severityAsString(), other.severityAsString())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(insightTimeRange(), other.insightTimeRange())
&& Objects.equals(predictionTimeRange(), other.predictionTimeRange())
&& Objects.equals(resourceCollection(), other.resourceCollection())
&& Objects.equals(serviceCollection(), other.serviceCollection());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ProactiveOrganizationInsightSummary").add("Id", id()).add("AccountId", accountId())
.add("OrganizationalUnitId", organizationalUnitId()).add("Name", name()).add("Severity", severityAsString())
.add("Status", statusAsString()).add("InsightTimeRange", insightTimeRange())
.add("PredictionTimeRange", predictionTimeRange()).add("ResourceCollection", resourceCollection())
.add("ServiceCollection", serviceCollection()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "AccountId":
return Optional.ofNullable(clazz.cast(accountId()));
case "OrganizationalUnitId":
return Optional.ofNullable(clazz.cast(organizationalUnitId()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Severity":
return Optional.ofNullable(clazz.cast(severityAsString()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "InsightTimeRange":
return Optional.ofNullable(clazz.cast(insightTimeRange()));
case "PredictionTimeRange":
return Optional.ofNullable(clazz.cast(predictionTimeRange()));
case "ResourceCollection":
return Optional.ofNullable(clazz.cast(resourceCollection()));
case "ServiceCollection":
return Optional.ofNullable(clazz.cast(serviceCollection()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function