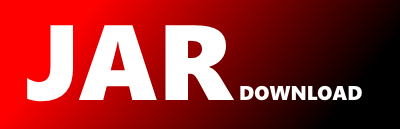
software.amazon.awssdk.services.directconnect.model.DirectConnectGatewayAttachment Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.directconnect.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about an attachment between a Direct Connect gateway and a virtual interface.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DirectConnectGatewayAttachment implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DIRECT_CONNECT_GATEWAY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DirectConnectGatewayAttachment::directConnectGatewayId))
.setter(setter(Builder::directConnectGatewayId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("directConnectGatewayId").build())
.build();
private static final SdkField VIRTUAL_INTERFACE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DirectConnectGatewayAttachment::virtualInterfaceId)).setter(setter(Builder::virtualInterfaceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("virtualInterfaceId").build())
.build();
private static final SdkField VIRTUAL_INTERFACE_REGION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DirectConnectGatewayAttachment::virtualInterfaceRegion))
.setter(setter(Builder::virtualInterfaceRegion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("virtualInterfaceRegion").build())
.build();
private static final SdkField VIRTUAL_INTERFACE_OWNER_ACCOUNT_FIELD = SdkField
. builder(MarshallingType.STRING)
.getter(getter(DirectConnectGatewayAttachment::virtualInterfaceOwnerAccount))
.setter(setter(Builder::virtualInterfaceOwnerAccount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("virtualInterfaceOwnerAccount")
.build()).build();
private static final SdkField ATTACHMENT_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DirectConnectGatewayAttachment::attachmentStateAsString)).setter(setter(Builder::attachmentState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attachmentState").build()).build();
private static final SdkField ATTACHMENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DirectConnectGatewayAttachment::attachmentTypeAsString)).setter(setter(Builder::attachmentType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attachmentType").build()).build();
private static final SdkField STATE_CHANGE_ERROR_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(DirectConnectGatewayAttachment::stateChangeError)).setter(setter(Builder::stateChangeError))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stateChangeError").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
DIRECT_CONNECT_GATEWAY_ID_FIELD, VIRTUAL_INTERFACE_ID_FIELD, VIRTUAL_INTERFACE_REGION_FIELD,
VIRTUAL_INTERFACE_OWNER_ACCOUNT_FIELD, ATTACHMENT_STATE_FIELD, ATTACHMENT_TYPE_FIELD, STATE_CHANGE_ERROR_FIELD));
private static final long serialVersionUID = 1L;
private final String directConnectGatewayId;
private final String virtualInterfaceId;
private final String virtualInterfaceRegion;
private final String virtualInterfaceOwnerAccount;
private final String attachmentState;
private final String attachmentType;
private final String stateChangeError;
private DirectConnectGatewayAttachment(BuilderImpl builder) {
this.directConnectGatewayId = builder.directConnectGatewayId;
this.virtualInterfaceId = builder.virtualInterfaceId;
this.virtualInterfaceRegion = builder.virtualInterfaceRegion;
this.virtualInterfaceOwnerAccount = builder.virtualInterfaceOwnerAccount;
this.attachmentState = builder.attachmentState;
this.attachmentType = builder.attachmentType;
this.stateChangeError = builder.stateChangeError;
}
/**
*
* The ID of the Direct Connect gateway.
*
*
* @return The ID of the Direct Connect gateway.
*/
public String directConnectGatewayId() {
return directConnectGatewayId;
}
/**
*
* The ID of the virtual interface.
*
*
* @return The ID of the virtual interface.
*/
public String virtualInterfaceId() {
return virtualInterfaceId;
}
/**
*
* The AWS Region where the virtual interface is located.
*
*
* @return The AWS Region where the virtual interface is located.
*/
public String virtualInterfaceRegion() {
return virtualInterfaceRegion;
}
/**
*
* The ID of the AWS account that owns the virtual interface.
*
*
* @return The ID of the AWS account that owns the virtual interface.
*/
public String virtualInterfaceOwnerAccount() {
return virtualInterfaceOwnerAccount;
}
/**
*
* The state of the attachment. The following are the possible values:
*
*
* -
*
* attaching
: The initial state after a virtual interface is created using the Direct Connect gateway.
*
*
* -
*
* attached
: The Direct Connect gateway and virtual interface are attached and ready to pass traffic.
*
*
* -
*
* detaching
: The initial state after calling DeleteVirtualInterface.
*
*
* -
*
* detached
: The virtual interface is detached from the Direct Connect gateway. Traffic flow between
* the Direct Connect gateway and virtual interface is stopped.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #attachmentState}
* will return {@link DirectConnectGatewayAttachmentState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #attachmentStateAsString}.
*
*
* @return The state of the attachment. The following are the possible values:
*
* -
*
* attaching
: The initial state after a virtual interface is created using the Direct Connect
* gateway.
*
*
* -
*
* attached
: The Direct Connect gateway and virtual interface are attached and ready to pass
* traffic.
*
*
* -
*
* detaching
: The initial state after calling DeleteVirtualInterface.
*
*
* -
*
* detached
: The virtual interface is detached from the Direct Connect gateway. Traffic flow
* between the Direct Connect gateway and virtual interface is stopped.
*
*
* @see DirectConnectGatewayAttachmentState
*/
public DirectConnectGatewayAttachmentState attachmentState() {
return DirectConnectGatewayAttachmentState.fromValue(attachmentState);
}
/**
*
* The state of the attachment. The following are the possible values:
*
*
* -
*
* attaching
: The initial state after a virtual interface is created using the Direct Connect gateway.
*
*
* -
*
* attached
: The Direct Connect gateway and virtual interface are attached and ready to pass traffic.
*
*
* -
*
* detaching
: The initial state after calling DeleteVirtualInterface.
*
*
* -
*
* detached
: The virtual interface is detached from the Direct Connect gateway. Traffic flow between
* the Direct Connect gateway and virtual interface is stopped.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #attachmentState}
* will return {@link DirectConnectGatewayAttachmentState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #attachmentStateAsString}.
*
*
* @return The state of the attachment. The following are the possible values:
*
* -
*
* attaching
: The initial state after a virtual interface is created using the Direct Connect
* gateway.
*
*
* -
*
* attached
: The Direct Connect gateway and virtual interface are attached and ready to pass
* traffic.
*
*
* -
*
* detaching
: The initial state after calling DeleteVirtualInterface.
*
*
* -
*
* detached
: The virtual interface is detached from the Direct Connect gateway. Traffic flow
* between the Direct Connect gateway and virtual interface is stopped.
*
*
* @see DirectConnectGatewayAttachmentState
*/
public String attachmentStateAsString() {
return attachmentState;
}
/**
*
* The type of attachment.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #attachmentType}
* will return {@link DirectConnectGatewayAttachmentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #attachmentTypeAsString}.
*
*
* @return The type of attachment.
* @see DirectConnectGatewayAttachmentType
*/
public DirectConnectGatewayAttachmentType attachmentType() {
return DirectConnectGatewayAttachmentType.fromValue(attachmentType);
}
/**
*
* The type of attachment.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #attachmentType}
* will return {@link DirectConnectGatewayAttachmentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #attachmentTypeAsString}.
*
*
* @return The type of attachment.
* @see DirectConnectGatewayAttachmentType
*/
public String attachmentTypeAsString() {
return attachmentType;
}
/**
*
* The error message if the state of an object failed to advance.
*
*
* @return The error message if the state of an object failed to advance.
*/
public String stateChangeError() {
return stateChangeError;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(directConnectGatewayId());
hashCode = 31 * hashCode + Objects.hashCode(virtualInterfaceId());
hashCode = 31 * hashCode + Objects.hashCode(virtualInterfaceRegion());
hashCode = 31 * hashCode + Objects.hashCode(virtualInterfaceOwnerAccount());
hashCode = 31 * hashCode + Objects.hashCode(attachmentStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(attachmentTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(stateChangeError());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DirectConnectGatewayAttachment)) {
return false;
}
DirectConnectGatewayAttachment other = (DirectConnectGatewayAttachment) obj;
return Objects.equals(directConnectGatewayId(), other.directConnectGatewayId())
&& Objects.equals(virtualInterfaceId(), other.virtualInterfaceId())
&& Objects.equals(virtualInterfaceRegion(), other.virtualInterfaceRegion())
&& Objects.equals(virtualInterfaceOwnerAccount(), other.virtualInterfaceOwnerAccount())
&& Objects.equals(attachmentStateAsString(), other.attachmentStateAsString())
&& Objects.equals(attachmentTypeAsString(), other.attachmentTypeAsString())
&& Objects.equals(stateChangeError(), other.stateChangeError());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DirectConnectGatewayAttachment").add("DirectConnectGatewayId", directConnectGatewayId())
.add("VirtualInterfaceId", virtualInterfaceId()).add("VirtualInterfaceRegion", virtualInterfaceRegion())
.add("VirtualInterfaceOwnerAccount", virtualInterfaceOwnerAccount())
.add("AttachmentState", attachmentStateAsString()).add("AttachmentType", attachmentTypeAsString())
.add("StateChangeError", stateChangeError()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "directConnectGatewayId":
return Optional.ofNullable(clazz.cast(directConnectGatewayId()));
case "virtualInterfaceId":
return Optional.ofNullable(clazz.cast(virtualInterfaceId()));
case "virtualInterfaceRegion":
return Optional.ofNullable(clazz.cast(virtualInterfaceRegion()));
case "virtualInterfaceOwnerAccount":
return Optional.ofNullable(clazz.cast(virtualInterfaceOwnerAccount()));
case "attachmentState":
return Optional.ofNullable(clazz.cast(attachmentStateAsString()));
case "attachmentType":
return Optional.ofNullable(clazz.cast(attachmentTypeAsString()));
case "stateChangeError":
return Optional.ofNullable(clazz.cast(stateChangeError()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function