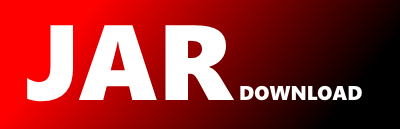
software.amazon.awssdk.services.directconnect.model.DeleteVirtualInterfaceResponse Maven / Gradle / Ivy
Show all versions of directconnect Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.directconnect.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeleteVirtualInterfaceResponse extends DirectConnectResponse implements
ToCopyableBuilder {
private static final SdkField VIRTUAL_INTERFACE_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("virtualInterfaceState").getter(getter(DeleteVirtualInterfaceResponse::virtualInterfaceStateAsString))
.setter(setter(Builder::virtualInterfaceState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("virtualInterfaceState").build())
.build();
private static final List> SDK_FIELDS = Collections
.unmodifiableList(Arrays.asList(VIRTUAL_INTERFACE_STATE_FIELD));
private final String virtualInterfaceState;
private DeleteVirtualInterfaceResponse(BuilderImpl builder) {
super(builder);
this.virtualInterfaceState = builder.virtualInterfaceState;
}
/**
*
* The state of the virtual interface. The following are the possible values:
*
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual interface
* owner. If the owner of the virtual interface is different from the owner of the connection on which it is
* provisioned, then the virtual interface will remain in this state until it is confirmed by the virtual interface
* owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual interface needs
* validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the virtual
* interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a virtual
* interface in the Confirming
state is deleted by the virtual interface owner, the virtual interface
* enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #virtualInterfaceState} will return {@link VirtualInterfaceState#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #virtualInterfaceStateAsString}.
*
*
* @return The state of the virtual interface. The following are the possible values:
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual
* interface owner. If the owner of the virtual interface is different from the owner of the connection on
* which it is provisioned, then the virtual interface will remain in this state until it is confirmed by
* the virtual interface owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual
* interface needs validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the
* virtual interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a
* virtual interface in the Confirming
state is deleted by the virtual interface owner, the
* virtual interface enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
* @see VirtualInterfaceState
*/
public VirtualInterfaceState virtualInterfaceState() {
return VirtualInterfaceState.fromValue(virtualInterfaceState);
}
/**
*
* The state of the virtual interface. The following are the possible values:
*
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual interface
* owner. If the owner of the virtual interface is different from the owner of the connection on which it is
* provisioned, then the virtual interface will remain in this state until it is confirmed by the virtual interface
* owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual interface needs
* validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the virtual
* interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a virtual
* interface in the Confirming
state is deleted by the virtual interface owner, the virtual interface
* enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #virtualInterfaceState} will return {@link VirtualInterfaceState#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #virtualInterfaceStateAsString}.
*
*
* @return The state of the virtual interface. The following are the possible values:
*
* -
*
* confirming
: The creation of the virtual interface is pending confirmation from the virtual
* interface owner. If the owner of the virtual interface is different from the owner of the connection on
* which it is provisioned, then the virtual interface will remain in this state until it is confirmed by
* the virtual interface owner.
*
*
* -
*
* verifying
: This state only applies to public virtual interfaces. Each public virtual
* interface needs validation before the virtual interface can be created.
*
*
* -
*
* pending
: A virtual interface is in this state from the time that it is created until the
* virtual interface is ready to forward traffic.
*
*
* -
*
* available
: A virtual interface that is able to forward traffic.
*
*
* -
*
* down
: A virtual interface that is BGP down.
*
*
* -
*
* deleting
: A virtual interface is in this state immediately after calling
* DeleteVirtualInterface until it can no longer forward traffic.
*
*
* -
*
* deleted
: A virtual interface that cannot forward traffic.
*
*
* -
*
* rejected
: The virtual interface owner has declined creation of the virtual interface. If a
* virtual interface in the Confirming
state is deleted by the virtual interface owner, the
* virtual interface enters the Rejected
state.
*
*
* -
*
* unknown
: The state of the virtual interface is not available.
*
*
* @see VirtualInterfaceState
*/
public String virtualInterfaceStateAsString() {
return virtualInterfaceState;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(virtualInterfaceStateAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeleteVirtualInterfaceResponse)) {
return false;
}
DeleteVirtualInterfaceResponse other = (DeleteVirtualInterfaceResponse) obj;
return Objects.equals(virtualInterfaceStateAsString(), other.virtualInterfaceStateAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DeleteVirtualInterfaceResponse").add("VirtualInterfaceState", virtualInterfaceStateAsString())
.build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "virtualInterfaceState":
return Optional.ofNullable(clazz.cast(virtualInterfaceStateAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function