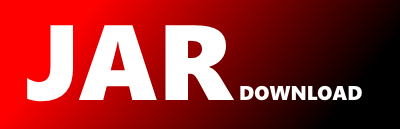
software.amazon.awssdk.services.directconnect.model.DirectConnectGateway Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.directconnect.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a Direct Connect gateway, which enables you to connect virtual interfaces and virtual private
* gateway or transit gateways.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DirectConnectGateway implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DIRECT_CONNECT_GATEWAY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("directConnectGatewayId").getter(getter(DirectConnectGateway::directConnectGatewayId))
.setter(setter(Builder::directConnectGatewayId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("directConnectGatewayId").build())
.build();
private static final SdkField DIRECT_CONNECT_GATEWAY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("directConnectGatewayName").getter(getter(DirectConnectGateway::directConnectGatewayName))
.setter(setter(Builder::directConnectGatewayName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("directConnectGatewayName").build())
.build();
private static final SdkField AMAZON_SIDE_ASN_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("amazonSideAsn").getter(getter(DirectConnectGateway::amazonSideAsn))
.setter(setter(Builder::amazonSideAsn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("amazonSideAsn").build()).build();
private static final SdkField OWNER_ACCOUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ownerAccount").getter(getter(DirectConnectGateway::ownerAccount)).setter(setter(Builder::ownerAccount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ownerAccount").build()).build();
private static final SdkField DIRECT_CONNECT_GATEWAY_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("directConnectGatewayState").getter(getter(DirectConnectGateway::directConnectGatewayStateAsString))
.setter(setter(Builder::directConnectGatewayState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("directConnectGatewayState").build())
.build();
private static final SdkField STATE_CHANGE_ERROR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("stateChangeError").getter(getter(DirectConnectGateway::stateChangeError))
.setter(setter(Builder::stateChangeError))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stateChangeError").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
DIRECT_CONNECT_GATEWAY_ID_FIELD, DIRECT_CONNECT_GATEWAY_NAME_FIELD, AMAZON_SIDE_ASN_FIELD, OWNER_ACCOUNT_FIELD,
DIRECT_CONNECT_GATEWAY_STATE_FIELD, STATE_CHANGE_ERROR_FIELD));
private static final long serialVersionUID = 1L;
private final String directConnectGatewayId;
private final String directConnectGatewayName;
private final Long amazonSideAsn;
private final String ownerAccount;
private final String directConnectGatewayState;
private final String stateChangeError;
private DirectConnectGateway(BuilderImpl builder) {
this.directConnectGatewayId = builder.directConnectGatewayId;
this.directConnectGatewayName = builder.directConnectGatewayName;
this.amazonSideAsn = builder.amazonSideAsn;
this.ownerAccount = builder.ownerAccount;
this.directConnectGatewayState = builder.directConnectGatewayState;
this.stateChangeError = builder.stateChangeError;
}
/**
*
* The ID of the Direct Connect gateway.
*
*
* @return The ID of the Direct Connect gateway.
*/
public final String directConnectGatewayId() {
return directConnectGatewayId;
}
/**
*
* The name of the Direct Connect gateway.
*
*
* @return The name of the Direct Connect gateway.
*/
public final String directConnectGatewayName() {
return directConnectGatewayName;
}
/**
*
* The autonomous system number (ASN) for the Amazon side of the connection.
*
*
* @return The autonomous system number (ASN) for the Amazon side of the connection.
*/
public final Long amazonSideAsn() {
return amazonSideAsn;
}
/**
*
* The ID of the AWS account that owns the Direct Connect gateway.
*
*
* @return The ID of the AWS account that owns the Direct Connect gateway.
*/
public final String ownerAccount() {
return ownerAccount;
}
/**
*
* The state of the Direct Connect gateway. The following are the possible values:
*
*
* -
*
* pending
: The initial state after calling CreateDirectConnectGateway.
*
*
* -
*
* available
: The Direct Connect gateway is ready for use.
*
*
* -
*
* deleting
: The initial state after calling DeleteDirectConnectGateway.
*
*
* -
*
* deleted
: The Direct Connect gateway is deleted and cannot pass traffic.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #directConnectGatewayState} will return {@link DirectConnectGatewayState#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #directConnectGatewayStateAsString}.
*
*
* @return The state of the Direct Connect gateway. The following are the possible values:
*
* -
*
* pending
: The initial state after calling CreateDirectConnectGateway.
*
*
* -
*
* available
: The Direct Connect gateway is ready for use.
*
*
* -
*
* deleting
: The initial state after calling DeleteDirectConnectGateway.
*
*
* -
*
* deleted
: The Direct Connect gateway is deleted and cannot pass traffic.
*
*
* @see DirectConnectGatewayState
*/
public final DirectConnectGatewayState directConnectGatewayState() {
return DirectConnectGatewayState.fromValue(directConnectGatewayState);
}
/**
*
* The state of the Direct Connect gateway. The following are the possible values:
*
*
* -
*
* pending
: The initial state after calling CreateDirectConnectGateway.
*
*
* -
*
* available
: The Direct Connect gateway is ready for use.
*
*
* -
*
* deleting
: The initial state after calling DeleteDirectConnectGateway.
*
*
* -
*
* deleted
: The Direct Connect gateway is deleted and cannot pass traffic.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #directConnectGatewayState} will return {@link DirectConnectGatewayState#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #directConnectGatewayStateAsString}.
*
*
* @return The state of the Direct Connect gateway. The following are the possible values:
*
* -
*
* pending
: The initial state after calling CreateDirectConnectGateway.
*
*
* -
*
* available
: The Direct Connect gateway is ready for use.
*
*
* -
*
* deleting
: The initial state after calling DeleteDirectConnectGateway.
*
*
* -
*
* deleted
: The Direct Connect gateway is deleted and cannot pass traffic.
*
*
* @see DirectConnectGatewayState
*/
public final String directConnectGatewayStateAsString() {
return directConnectGatewayState;
}
/**
*
* The error message if the state of an object failed to advance.
*
*
* @return The error message if the state of an object failed to advance.
*/
public final String stateChangeError() {
return stateChangeError;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(directConnectGatewayId());
hashCode = 31 * hashCode + Objects.hashCode(directConnectGatewayName());
hashCode = 31 * hashCode + Objects.hashCode(amazonSideAsn());
hashCode = 31 * hashCode + Objects.hashCode(ownerAccount());
hashCode = 31 * hashCode + Objects.hashCode(directConnectGatewayStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(stateChangeError());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DirectConnectGateway)) {
return false;
}
DirectConnectGateway other = (DirectConnectGateway) obj;
return Objects.equals(directConnectGatewayId(), other.directConnectGatewayId())
&& Objects.equals(directConnectGatewayName(), other.directConnectGatewayName())
&& Objects.equals(amazonSideAsn(), other.amazonSideAsn()) && Objects.equals(ownerAccount(), other.ownerAccount())
&& Objects.equals(directConnectGatewayStateAsString(), other.directConnectGatewayStateAsString())
&& Objects.equals(stateChangeError(), other.stateChangeError());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DirectConnectGateway").add("DirectConnectGatewayId", directConnectGatewayId())
.add("DirectConnectGatewayName", directConnectGatewayName()).add("AmazonSideAsn", amazonSideAsn())
.add("OwnerAccount", ownerAccount()).add("DirectConnectGatewayState", directConnectGatewayStateAsString())
.add("StateChangeError", stateChangeError()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "directConnectGatewayId":
return Optional.ofNullable(clazz.cast(directConnectGatewayId()));
case "directConnectGatewayName":
return Optional.ofNullable(clazz.cast(directConnectGatewayName()));
case "amazonSideAsn":
return Optional.ofNullable(clazz.cast(amazonSideAsn()));
case "ownerAccount":
return Optional.ofNullable(clazz.cast(ownerAccount()));
case "directConnectGatewayState":
return Optional.ofNullable(clazz.cast(directConnectGatewayStateAsString()));
case "stateChangeError":
return Optional.ofNullable(clazz.cast(stateChangeError()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function