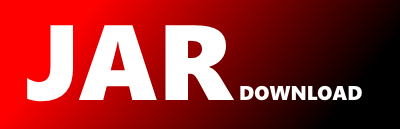
software.amazon.awssdk.services.directoryservicedata.DirectoryServiceDataClient Maven / Gradle / Ivy
Show all versions of directoryservicedata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.directoryservicedata;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.directoryservicedata.model.AccessDeniedException;
import software.amazon.awssdk.services.directoryservicedata.model.AddGroupMemberRequest;
import software.amazon.awssdk.services.directoryservicedata.model.AddGroupMemberResponse;
import software.amazon.awssdk.services.directoryservicedata.model.ConflictException;
import software.amazon.awssdk.services.directoryservicedata.model.CreateGroupRequest;
import software.amazon.awssdk.services.directoryservicedata.model.CreateGroupResponse;
import software.amazon.awssdk.services.directoryservicedata.model.CreateUserRequest;
import software.amazon.awssdk.services.directoryservicedata.model.CreateUserResponse;
import software.amazon.awssdk.services.directoryservicedata.model.DeleteGroupRequest;
import software.amazon.awssdk.services.directoryservicedata.model.DeleteGroupResponse;
import software.amazon.awssdk.services.directoryservicedata.model.DeleteUserRequest;
import software.amazon.awssdk.services.directoryservicedata.model.DeleteUserResponse;
import software.amazon.awssdk.services.directoryservicedata.model.DescribeGroupRequest;
import software.amazon.awssdk.services.directoryservicedata.model.DescribeGroupResponse;
import software.amazon.awssdk.services.directoryservicedata.model.DescribeUserRequest;
import software.amazon.awssdk.services.directoryservicedata.model.DescribeUserResponse;
import software.amazon.awssdk.services.directoryservicedata.model.DirectoryServiceDataException;
import software.amazon.awssdk.services.directoryservicedata.model.DirectoryUnavailableException;
import software.amazon.awssdk.services.directoryservicedata.model.DisableUserRequest;
import software.amazon.awssdk.services.directoryservicedata.model.DisableUserResponse;
import software.amazon.awssdk.services.directoryservicedata.model.InternalServerException;
import software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersRequest;
import software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersResponse;
import software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberRequest;
import software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberResponse;
import software.amazon.awssdk.services.directoryservicedata.model.ListGroupsRequest;
import software.amazon.awssdk.services.directoryservicedata.model.ListGroupsResponse;
import software.amazon.awssdk.services.directoryservicedata.model.ListUsersRequest;
import software.amazon.awssdk.services.directoryservicedata.model.ListUsersResponse;
import software.amazon.awssdk.services.directoryservicedata.model.RemoveGroupMemberRequest;
import software.amazon.awssdk.services.directoryservicedata.model.RemoveGroupMemberResponse;
import software.amazon.awssdk.services.directoryservicedata.model.ResourceNotFoundException;
import software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsRequest;
import software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsResponse;
import software.amazon.awssdk.services.directoryservicedata.model.SearchUsersRequest;
import software.amazon.awssdk.services.directoryservicedata.model.SearchUsersResponse;
import software.amazon.awssdk.services.directoryservicedata.model.ThrottlingException;
import software.amazon.awssdk.services.directoryservicedata.model.UpdateGroupRequest;
import software.amazon.awssdk.services.directoryservicedata.model.UpdateGroupResponse;
import software.amazon.awssdk.services.directoryservicedata.model.UpdateUserRequest;
import software.amazon.awssdk.services.directoryservicedata.model.UpdateUserResponse;
import software.amazon.awssdk.services.directoryservicedata.model.ValidationException;
import software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupMembersIterable;
import software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsForMemberIterable;
import software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsIterable;
import software.amazon.awssdk.services.directoryservicedata.paginators.ListUsersIterable;
import software.amazon.awssdk.services.directoryservicedata.paginators.SearchGroupsIterable;
import software.amazon.awssdk.services.directoryservicedata.paginators.SearchUsersIterable;
/**
* Service client for accessing AWS Directory Service Data. This can be created using the static {@link #builder()}
* method.
*
*
* Amazon Web Services Directory Service Data is an extension of Directory Service. This API reference provides detailed
* information about Directory Service Data operations and object types.
*
*
* With Directory Service Data, you can create, read, update, and delete users, groups, and memberships from your
* Managed Microsoft AD without additional costs and without deploying dedicated management instances. You can also
* perform built-in object management tasks across directories without direct network connectivity, which simplifies
* provisioning and access management to achieve fully automated deployments. Directory Service Data supports user and
* group write operations, such as CreateUser
and CreateGroup
, within the organizational unit
* (OU) of your Managed Microsoft AD. Directory Service Data supports read operations, such as ListUsers
* and ListGroups
, on all users, groups, and group memberships within your Managed Microsoft AD and across
* trusted realms. Directory Service Data supports adding and removing group members in your OU and the Amazon Web
* Services Delegated Groups OU, so you can grant and deny access to specific roles and permissions. For more
* information, see Manage users
* and groups in the Directory Service Administration Guide.
*
*
*
* Directory management operations and configuration changes made against the Directory Service API will also reflect in
* Directory Service Data API with eventual consistency. You can expect a short delay between management changes, such
* as adding a new directory trust and calling the Directory Service Data API for the newly created trusted realm.
*
*
*
* Directory Service Data connects to your Managed Microsoft AD domain controllers and performs operations on underlying
* directory objects. When you create your Managed Microsoft AD, you choose subnets for domain controllers that
* Directory Service creates on your behalf. If a domain controller is unavailable, Directory Service Data uses an
* available domain controller. As a result, you might notice eventual consistency while objects replicate from one
* domain controller to another domain controller. For more information, see What gets created in the Directory Service Administration Guide. Directory limits vary by Managed
* Microsoft AD edition:
*
*
* -
*
* Standard edition – Supports 8 transactions per second (TPS) for read operations and 4 TPS for write operations
* per directory. There's a concurrency limit of 10 concurrent requests.
*
*
* -
*
* Enterprise edition – Supports 16 transactions per second (TPS) for read operations and 8 TPS for write
* operations per directory. There's a concurrency limit of 10 concurrent requests.
*
*
* -
*
* Amazon Web Services Account - Supports a total of 100 TPS for Directory Service Data operations across all
* directories.
*
*
*
*
* Directory Service Data only supports the Managed Microsoft AD directory type and is only available in the primary
* Amazon Web Services Region. For more information, see Managed Microsoft
* AD and Primary vs additional Regions in the Directory Service Administration Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface DirectoryServiceDataClient extends AwsClient {
String SERVICE_NAME = "ds-data";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "ds-data";
/**
*
* Adds an existing user, group, or computer as a group member.
*
*
* @param addGroupMemberRequest
* @return Result of the AddGroupMember operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.AddGroupMember
* @see AWS API Documentation
*/
default AddGroupMemberResponse addGroupMember(AddGroupMemberRequest addGroupMemberRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ConflictException, ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Adds an existing user, group, or computer as a group member.
*
*
*
* This is a convenience which creates an instance of the {@link AddGroupMemberRequest.Builder} avoiding the need to
* create one manually via {@link AddGroupMemberRequest#builder()}
*
*
* @param addGroupMemberRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.AddGroupMemberRequest.Builder} to create
* a request.
* @return Result of the AddGroupMember operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.AddGroupMember
* @see AWS API Documentation
*/
default AddGroupMemberResponse addGroupMember(Consumer addGroupMemberRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ConflictException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return addGroupMember(AddGroupMemberRequest.builder().applyMutation(addGroupMemberRequest).build());
}
/**
*
* Creates a new group.
*
*
* @param createGroupRequest
* @return Result of the CreateGroup operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.CreateGroup
* @see AWS API Documentation
*/
default CreateGroupResponse createGroup(CreateGroupRequest createGroupRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ConflictException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new group.
*
*
*
* This is a convenience which creates an instance of the {@link CreateGroupRequest.Builder} avoiding the need to
* create one manually via {@link CreateGroupRequest#builder()}
*
*
* @param createGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.CreateGroupRequest.Builder} to create a
* request.
* @return Result of the CreateGroup operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.CreateGroup
* @see AWS API Documentation
*/
default CreateGroupResponse createGroup(Consumer createGroupRequest)
throws AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ConflictException, ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
return createGroup(CreateGroupRequest.builder().applyMutation(createGroupRequest).build());
}
/**
*
* Creates a new user.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.CreateUser
* @see AWS API Documentation
*/
default CreateUserResponse createUser(CreateUserRequest createUserRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ConflictException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new user.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.CreateUserRequest.Builder} to create a
* request.
* @return Result of the CreateUser operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.CreateUser
* @see AWS API Documentation
*/
default CreateUserResponse createUser(Consumer createUserRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ConflictException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Deletes a group.
*
*
* @param deleteGroupRequest
* @return Result of the DeleteGroup operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DeleteGroup
* @see AWS API Documentation
*/
default DeleteGroupResponse deleteGroup(DeleteGroupRequest deleteGroupRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ConflictException, ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a group.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGroupRequest.Builder} avoiding the need to
* create one manually via {@link DeleteGroupRequest#builder()}
*
*
* @param deleteGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.DeleteGroupRequest.Builder} to create a
* request.
* @return Result of the DeleteGroup operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DeleteGroup
* @see AWS API Documentation
*/
default DeleteGroupResponse deleteGroup(Consumer deleteGroupRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ConflictException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return deleteGroup(DeleteGroupRequest.builder().applyMutation(deleteGroupRequest).build());
}
/**
*
* Deletes a user.
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DeleteUser
* @see AWS API Documentation
*/
default DeleteUserResponse deleteUser(DeleteUserRequest deleteUserRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ConflictException, ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a user.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserRequest.Builder} avoiding the need to
* create one manually via {@link DeleteUserRequest#builder()}
*
*
* @param deleteUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.DeleteUserRequest.Builder} to create a
* request.
* @return Result of the DeleteUser operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DeleteUser
* @see AWS API Documentation
*/
default DeleteUserResponse deleteUser(Consumer deleteUserRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ConflictException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return deleteUser(DeleteUserRequest.builder().applyMutation(deleteUserRequest).build());
}
/**
*
* Returns information about a specific group.
*
*
* @param describeGroupRequest
* @return Result of the DescribeGroup operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DescribeGroup
* @see AWS API Documentation
*/
default DescribeGroupResponse describeGroup(DescribeGroupRequest describeGroupRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a specific group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeGroupRequest.Builder} avoiding the need to
* create one manually via {@link DescribeGroupRequest#builder()}
*
*
* @param describeGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.DescribeGroupRequest.Builder} to create
* a request.
* @return Result of the DescribeGroup operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DescribeGroup
* @see AWS API Documentation
*/
default DescribeGroupResponse describeGroup(Consumer describeGroupRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return describeGroup(DescribeGroupRequest.builder().applyMutation(describeGroupRequest).build());
}
/**
*
* Returns information about a specific user.
*
*
* @param describeUserRequest
* @return Result of the DescribeUser operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DescribeUser
* @see AWS API Documentation
*/
default DescribeUserResponse describeUser(DescribeUserRequest describeUserRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a specific user.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserRequest.Builder} avoiding the need to
* create one manually via {@link DescribeUserRequest#builder()}
*
*
* @param describeUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.DescribeUserRequest.Builder} to create a
* request.
* @return Result of the DescribeUser operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DescribeUser
* @see AWS API Documentation
*/
default DescribeUserResponse describeUser(Consumer describeUserRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return describeUser(DescribeUserRequest.builder().applyMutation(describeUserRequest).build());
}
/**
*
* Deactivates an active user account. For information about how to enable an inactive user account, see ResetUserPassword in the Directory Service API Reference.
*
*
* @param disableUserRequest
* @return Result of the DisableUser operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DisableUser
* @see AWS API Documentation
*/
default DisableUserResponse disableUser(DisableUserRequest disableUserRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ConflictException, ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Deactivates an active user account. For information about how to enable an inactive user account, see ResetUserPassword in the Directory Service API Reference.
*
*
*
* This is a convenience which creates an instance of the {@link DisableUserRequest.Builder} avoiding the need to
* create one manually via {@link DisableUserRequest#builder()}
*
*
* @param disableUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.DisableUserRequest.Builder} to create a
* request.
* @return Result of the DisableUser operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.DisableUser
* @see AWS API Documentation
*/
default DisableUserResponse disableUser(Consumer disableUserRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ConflictException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return disableUser(DisableUserRequest.builder().applyMutation(disableUserRequest).build());
}
/**
*
* Returns member information for the specified group.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the ListGroupMembers.NextToken
member contains a token that you pass in
* the next call to ListGroupMembers
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
* @param listGroupMembersRequest
* @return Result of the ListGroupMembers operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroupMembers
* @see AWS API Documentation
*/
default ListGroupMembersResponse listGroupMembers(ListGroupMembersRequest listGroupMembersRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Returns member information for the specified group.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the ListGroupMembers.NextToken
member contains a token that you pass in
* the next call to ListGroupMembers
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupMembersRequest.Builder} avoiding the need
* to create one manually via {@link ListGroupMembersRequest#builder()}
*
*
* @param listGroupMembersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersRequest.Builder} to
* create a request.
* @return Result of the ListGroupMembers operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroupMembers
* @see AWS API Documentation
*/
default ListGroupMembersResponse listGroupMembers(Consumer listGroupMembersRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return listGroupMembers(ListGroupMembersRequest.builder().applyMutation(listGroupMembersRequest).build());
}
/**
*
* This is a variant of
* {@link #listGroupMembers(software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupMembersIterable responses = client.listGroupMembersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupMembersIterable responses = client
* .listGroupMembersPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupMembersIterable responses = client.listGroupMembersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupMembers(software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersRequest)}
* operation.
*
*
* @param listGroupMembersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroupMembers
* @see AWS API Documentation
*/
default ListGroupMembersIterable listGroupMembersPaginator(ListGroupMembersRequest listGroupMembersRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return new ListGroupMembersIterable(this, listGroupMembersRequest);
}
/**
*
* This is a variant of
* {@link #listGroupMembers(software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupMembersIterable responses = client.listGroupMembersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupMembersIterable responses = client
* .listGroupMembersPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupMembersIterable responses = client.listGroupMembersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupMembers(software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupMembersRequest.Builder} avoiding the need
* to create one manually via {@link ListGroupMembersRequest#builder()}
*
*
* @param listGroupMembersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.ListGroupMembersRequest.Builder} to
* create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroupMembers
* @see AWS API Documentation
*/
default ListGroupMembersIterable listGroupMembersPaginator(Consumer listGroupMembersRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return listGroupMembersPaginator(ListGroupMembersRequest.builder().applyMutation(listGroupMembersRequest).build());
}
/**
*
* Returns group information for the specified directory.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the ListGroups.NextToken
member contains a token that you pass in the
* next call to ListGroups
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
* @param listGroupsRequest
* @return Result of the ListGroups operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroups
* @see AWS API Documentation
*/
default ListGroupsResponse listGroups(ListGroupsRequest listGroupsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Returns group information for the specified directory.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the ListGroups.NextToken
member contains a token that you pass in the
* next call to ListGroups
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListGroupsRequest#builder()}
*
*
* @param listGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.ListGroupsRequest.Builder} to create a
* request.
* @return Result of the ListGroups operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroups
* @see AWS API Documentation
*/
default ListGroupsResponse listGroups(Consumer listGroupsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
return listGroups(ListGroupsRequest.builder().applyMutation(listGroupsRequest).build());
}
/**
*
* This is a variant of
* {@link #listGroups(software.amazon.awssdk.services.directoryservicedata.model.ListGroupsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsIterable responses = client
* .listGroupsPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.ListGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.directoryservicedata.model.ListGroupsRequest)} operation.
*
*
* @param listGroupsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroups
* @see AWS API Documentation
*/
default ListGroupsIterable listGroupsPaginator(ListGroupsRequest listGroupsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
return new ListGroupsIterable(this, listGroupsRequest);
}
/**
*
* This is a variant of
* {@link #listGroups(software.amazon.awssdk.services.directoryservicedata.model.ListGroupsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsIterable responses = client
* .listGroupsPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.ListGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsIterable responses = client.listGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroups(software.amazon.awssdk.services.directoryservicedata.model.ListGroupsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupsRequest.Builder} avoiding the need to
* create one manually via {@link ListGroupsRequest#builder()}
*
*
* @param listGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.ListGroupsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroups
* @see AWS API Documentation
*/
default ListGroupsIterable listGroupsPaginator(Consumer listGroupsRequest)
throws AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
return listGroupsPaginator(ListGroupsRequest.builder().applyMutation(listGroupsRequest).build());
}
/**
*
* Returns group information for the specified member.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the ListGroupsForMember.NextToken
member contains a token that you pass
* in the next call to ListGroupsForMember
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
* @param listGroupsForMemberRequest
* @return Result of the ListGroupsForMember operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroupsForMember
* @see AWS API Documentation
*/
default ListGroupsForMemberResponse listGroupsForMember(ListGroupsForMemberRequest listGroupsForMemberRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Returns group information for the specified member.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the ListGroupsForMember.NextToken
member contains a token that you pass
* in the next call to ListGroupsForMember
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupsForMemberRequest.Builder} avoiding the
* need to create one manually via {@link ListGroupsForMemberRequest#builder()}
*
*
* @param listGroupsForMemberRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberRequest.Builder} to
* create a request.
* @return Result of the ListGroupsForMember operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroupsForMember
* @see AWS API Documentation
*/
default ListGroupsForMemberResponse listGroupsForMember(
Consumer listGroupsForMemberRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
return listGroupsForMember(ListGroupsForMemberRequest.builder().applyMutation(listGroupsForMemberRequest).build());
}
/**
*
* This is a variant of
* {@link #listGroupsForMember(software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsForMemberIterable responses = client.listGroupsForMemberPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsForMemberIterable responses = client
* .listGroupsForMemberPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsForMemberIterable responses = client.listGroupsForMemberPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupsForMember(software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberRequest)}
* operation.
*
*
* @param listGroupsForMemberRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroupsForMember
* @see AWS API Documentation
*/
default ListGroupsForMemberIterable listGroupsForMemberPaginator(ListGroupsForMemberRequest listGroupsForMemberRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return new ListGroupsForMemberIterable(this, listGroupsForMemberRequest);
}
/**
*
* This is a variant of
* {@link #listGroupsForMember(software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsForMemberIterable responses = client.listGroupsForMemberPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsForMemberIterable responses = client
* .listGroupsForMemberPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListGroupsForMemberIterable responses = client.listGroupsForMemberPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listGroupsForMember(software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListGroupsForMemberRequest.Builder} avoiding the
* need to create one manually via {@link ListGroupsForMemberRequest#builder()}
*
*
* @param listGroupsForMemberRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.ListGroupsForMemberRequest.Builder} to
* create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListGroupsForMember
* @see AWS API Documentation
*/
default ListGroupsForMemberIterable listGroupsForMemberPaginator(
Consumer listGroupsForMemberRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
return listGroupsForMemberPaginator(ListGroupsForMemberRequest.builder().applyMutation(listGroupsForMemberRequest)
.build());
}
/**
*
* Returns user information for the specified directory.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the ListUsers.NextToken
member contains a token that you pass in the
* next call to ListUsers
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
* @param listUsersRequest
* @return Result of the ListUsers operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListUsers
* @see AWS API Documentation
*/
default ListUsersResponse listUsers(ListUsersRequest listUsersRequest) throws AccessDeniedException, InternalServerException,
ValidationException, DirectoryUnavailableException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Returns user information for the specified directory.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the ListUsers.NextToken
member contains a token that you pass in the
* next call to ListUsers
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.ListUsersRequest.Builder} to create a
* request.
* @return Result of the ListUsers operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListUsers
* @see AWS API Documentation
*/
default ListUsersResponse listUsers(Consumer listUsersRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
return listUsers(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* This is a variant of
* {@link #listUsers(software.amazon.awssdk.services.directoryservicedata.model.ListUsersRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListUsersIterable responses = client
* .listUsersPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.ListUsersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.directoryservicedata.model.ListUsersRequest)} operation.
*
*
* @param listUsersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListUsers
* @see AWS API Documentation
*/
default ListUsersIterable listUsersPaginator(ListUsersRequest listUsersRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
return new ListUsersIterable(this, listUsersRequest);
}
/**
*
* This is a variant of
* {@link #listUsers(software.amazon.awssdk.services.directoryservicedata.model.ListUsersRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListUsersIterable responses = client
* .listUsersPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.ListUsersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.ListUsersIterable responses = client.listUsersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.directoryservicedata.model.ListUsersRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.ListUsersRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.ListUsers
* @see AWS API Documentation
*/
default ListUsersIterable listUsersPaginator(Consumer listUsersRequest)
throws AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
return listUsersPaginator(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* Removes a member from a group.
*
*
* @param removeGroupMemberRequest
* @return Result of the RemoveGroupMember operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.RemoveGroupMember
* @see AWS API Documentation
*/
default RemoveGroupMemberResponse removeGroupMember(RemoveGroupMemberRequest removeGroupMemberRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ConflictException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Removes a member from a group.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveGroupMemberRequest.Builder} avoiding the need
* to create one manually via {@link RemoveGroupMemberRequest#builder()}
*
*
* @param removeGroupMemberRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.RemoveGroupMemberRequest.Builder} to
* create a request.
* @return Result of the RemoveGroupMember operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.RemoveGroupMember
* @see AWS API Documentation
*/
default RemoveGroupMemberResponse removeGroupMember(Consumer removeGroupMemberRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ConflictException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return removeGroupMember(RemoveGroupMemberRequest.builder().applyMutation(removeGroupMemberRequest).build());
}
/**
*
* Searches the specified directory for a group. You can find groups that match the SearchString
* parameter with the value of their attributes included in the SearchString
parameter.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the SearchGroups.NextToken
member contains a token that you pass in the
* next call to SearchGroups
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
* @param searchGroupsRequest
* @return Result of the SearchGroups operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.SearchGroups
* @see AWS API Documentation
*/
default SearchGroupsResponse searchGroups(SearchGroupsRequest searchGroupsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Searches the specified directory for a group. You can find groups that match the SearchString
* parameter with the value of their attributes included in the SearchString
parameter.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the SearchGroups.NextToken
member contains a token that you pass in the
* next call to SearchGroups
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link SearchGroupsRequest.Builder} avoiding the need to
* create one manually via {@link SearchGroupsRequest#builder()}
*
*
* @param searchGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsRequest.Builder} to create a
* request.
* @return Result of the SearchGroups operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.SearchGroups
* @see AWS API Documentation
*/
default SearchGroupsResponse searchGroups(Consumer searchGroupsRequest)
throws AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
return searchGroups(SearchGroupsRequest.builder().applyMutation(searchGroupsRequest).build());
}
/**
*
* This is a variant of
* {@link #searchGroups(software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchGroupsIterable responses = client.searchGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchGroupsIterable responses = client
* .searchGroupsPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchGroupsIterable responses = client.searchGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchGroups(software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsRequest)}
* operation.
*
*
* @param searchGroupsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.SearchGroups
* @see AWS API Documentation
*/
default SearchGroupsIterable searchGroupsPaginator(SearchGroupsRequest searchGroupsRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
return new SearchGroupsIterable(this, searchGroupsRequest);
}
/**
*
* This is a variant of
* {@link #searchGroups(software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchGroupsIterable responses = client.searchGroupsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchGroupsIterable responses = client
* .searchGroupsPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchGroupsIterable responses = client.searchGroupsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchGroups(software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link SearchGroupsRequest.Builder} avoiding the need to
* create one manually via {@link SearchGroupsRequest#builder()}
*
*
* @param searchGroupsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.SearchGroupsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.SearchGroups
* @see AWS API Documentation
*/
default SearchGroupsIterable searchGroupsPaginator(Consumer searchGroupsRequest)
throws AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
return searchGroupsPaginator(SearchGroupsRequest.builder().applyMutation(searchGroupsRequest).build());
}
/**
*
* Searches the specified directory for a user. You can find users that match the SearchString
* parameter with the value of their attributes included in the SearchString
parameter.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the SearchUsers.NextToken
member contains a token that you pass in the
* next call to SearchUsers
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
* @param searchUsersRequest
* @return Result of the SearchUsers operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.SearchUsers
* @see AWS API Documentation
*/
default SearchUsersResponse searchUsers(SearchUsersRequest searchUsersRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Searches the specified directory for a user. You can find users that match the SearchString
* parameter with the value of their attributes included in the SearchString
parameter.
*
*
* This operation supports pagination with the use of the NextToken
request and response parameters. If
* more results are available, the SearchUsers.NextToken
member contains a token that you pass in the
* next call to SearchUsers
. This retrieves the next set of items.
*
*
* You can also specify a maximum number of return results with the MaxResults
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link SearchUsersRequest.Builder} avoiding the need to
* create one manually via {@link SearchUsersRequest#builder()}
*
*
* @param searchUsersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.SearchUsersRequest.Builder} to create a
* request.
* @return Result of the SearchUsers operation returned by the service.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.SearchUsers
* @see AWS API Documentation
*/
default SearchUsersResponse searchUsers(Consumer searchUsersRequest)
throws AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
return searchUsers(SearchUsersRequest.builder().applyMutation(searchUsersRequest).build());
}
/**
*
* This is a variant of
* {@link #searchUsers(software.amazon.awssdk.services.directoryservicedata.model.SearchUsersRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchUsersIterable responses = client.searchUsersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchUsersIterable responses = client
* .searchUsersPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.SearchUsersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchUsersIterable responses = client.searchUsersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchUsers(software.amazon.awssdk.services.directoryservicedata.model.SearchUsersRequest)}
* operation.
*
*
* @param searchUsersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.SearchUsers
* @see AWS API Documentation
*/
default SearchUsersIterable searchUsersPaginator(SearchUsersRequest searchUsersRequest) throws AccessDeniedException,
InternalServerException, ValidationException, DirectoryUnavailableException, ThrottlingException,
AwsServiceException, SdkClientException, DirectoryServiceDataException {
return new SearchUsersIterable(this, searchUsersRequest);
}
/**
*
* This is a variant of
* {@link #searchUsers(software.amazon.awssdk.services.directoryservicedata.model.SearchUsersRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchUsersIterable responses = client.searchUsersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchUsersIterable responses = client
* .searchUsersPaginator(request);
* for (software.amazon.awssdk.services.directoryservicedata.model.SearchUsersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.directoryservicedata.paginators.SearchUsersIterable responses = client.searchUsersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #searchUsers(software.amazon.awssdk.services.directoryservicedata.model.SearchUsersRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link SearchUsersRequest.Builder} avoiding the need to
* create one manually via {@link SearchUsersRequest#builder()}
*
*
* @param searchUsersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.SearchUsersRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.SearchUsers
* @see AWS API Documentation
*/
default SearchUsersIterable searchUsersPaginator(Consumer searchUsersRequest)
throws AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
return searchUsersPaginator(SearchUsersRequest.builder().applyMutation(searchUsersRequest).build());
}
/**
*
* Updates group information.
*
*
* @param updateGroupRequest
* @return Result of the UpdateGroup operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.UpdateGroup
* @see AWS API Documentation
*/
default UpdateGroupResponse updateGroup(UpdateGroupRequest updateGroupRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ConflictException, ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Updates group information.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateGroupRequest.Builder} avoiding the need to
* create one manually via {@link UpdateGroupRequest#builder()}
*
*
* @param updateGroupRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.UpdateGroupRequest.Builder} to create a
* request.
* @return Result of the UpdateGroup operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.UpdateGroup
* @see AWS API Documentation
*/
default UpdateGroupResponse updateGroup(Consumer updateGroupRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ConflictException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return updateGroup(UpdateGroupRequest.builder().applyMutation(updateGroupRequest).build());
}
/**
*
* Updates user information.
*
*
* @param updateUserRequest
* @return Result of the UpdateUser operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.UpdateUser
* @see AWS API Documentation
*/
default UpdateUserResponse updateUser(UpdateUserRequest updateUserRequest) throws ResourceNotFoundException,
AccessDeniedException, InternalServerException, ValidationException, DirectoryUnavailableException,
ConflictException, ThrottlingException, AwsServiceException, SdkClientException, DirectoryServiceDataException {
throw new UnsupportedOperationException();
}
/**
*
* Updates user information.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserRequest.Builder} avoiding the need to
* create one manually via {@link UpdateUserRequest#builder()}
*
*
* @param updateUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.directoryservicedata.model.UpdateUserRequest.Builder} to create a
* request.
* @return Result of the UpdateUser operation returned by the service.
* @throws ResourceNotFoundException
* The resource couldn't be found.
* @throws AccessDeniedException
* You don't have permission to perform the request or access the directory. It can also occur when the
* DirectoryId
doesn't exist or the user, member, or group might be outside of your
* organizational unit (OU).
*
* Make sure that you have the authentication and authorization to perform the action. Review the directory
* information in the request, and make sure that the object isn't outside of your OU.
* @throws InternalServerException
* The operation didn't succeed because an internal error occurred. Try again later.
* @throws ValidationException
* The request isn't valid. Review the details in the error message to update the invalid parameters or
* values in your request.
* @throws DirectoryUnavailableException
* The request could not be completed due to a problem in the configuration or current state of the
* specified directory.
* @throws ConflictException
* This error will occur when you try to create a resource that conflicts with an existing object. It can
* also occur when adding a member to a group that the member is already in.
*
*
* This error can be caused by a request sent within the 8-hour idempotency window with the same client
* token but different input parameters. Client tokens should not be re-used across different requests.
* After 8 hours, any request with the same client token is treated as a new request.
* @throws ThrottlingException
* The limit on the number of requests per second has been exceeded.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws DirectoryServiceDataException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample DirectoryServiceDataClient.UpdateUser
* @see AWS API Documentation
*/
default UpdateUserResponse updateUser(Consumer updateUserRequest)
throws ResourceNotFoundException, AccessDeniedException, InternalServerException, ValidationException,
DirectoryUnavailableException, ConflictException, ThrottlingException, AwsServiceException, SdkClientException,
DirectoryServiceDataException {
return updateUser(UpdateUserRequest.builder().applyMutation(updateUserRequest).build());
}
/**
* Create a {@link DirectoryServiceDataClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static DirectoryServiceDataClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link DirectoryServiceDataClient}.
*/
static DirectoryServiceDataClientBuilder builder() {
return new DefaultDirectoryServiceDataClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default DirectoryServiceDataServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}