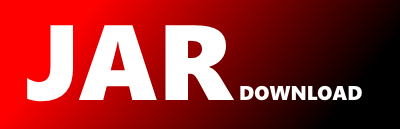
software.amazon.awssdk.services.applicationdiscovery.DefaultApplicationDiscoveryAsyncClient Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.applicationdiscovery;
import java.util.concurrent.CompletableFuture;
import javax.annotation.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.client.AsyncClientHandler;
import software.amazon.awssdk.core.client.ClientExecutionParams;
import software.amazon.awssdk.core.client.SdkAsyncClientHandler;
import software.amazon.awssdk.core.config.AsyncClientConfiguration;
import software.amazon.awssdk.core.exception.SdkServiceException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.protocol.json.JsonClientMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorResponseMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorShapeMetadata;
import software.amazon.awssdk.core.protocol.json.JsonOperationMetadata;
import software.amazon.awssdk.core.protocol.json.SdkJsonProtocolFactory;
import software.amazon.awssdk.services.applicationdiscovery.model.AssociateConfigurationItemsToApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.AssociateConfigurationItemsToApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.AuthorizationErrorException;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateTagsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.CreateTagsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteApplicationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteApplicationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteTagsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DeleteTagsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeAgentsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeConfigurationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeConfigurationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportConfigurationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportConfigurationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeExportTasksResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DescribeTagsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.DisassociateConfigurationItemsFromApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.DisassociateConfigurationItemsFromApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.ExportConfigurationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.ExportConfigurationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.GetDiscoverySummaryRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.GetDiscoverySummaryResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.InvalidParameterException;
import software.amazon.awssdk.services.applicationdiscovery.model.InvalidParameterValueException;
import software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.ListConfigurationsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.ListServerNeighborsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.ListServerNeighborsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.OperationNotPermittedException;
import software.amazon.awssdk.services.applicationdiscovery.model.ResourceNotFoundException;
import software.amazon.awssdk.services.applicationdiscovery.model.ServerInternalErrorException;
import software.amazon.awssdk.services.applicationdiscovery.model.StartDataCollectionByAgentIdsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartDataCollectionByAgentIdsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StartExportTaskRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StartExportTaskResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.StopDataCollectionByAgentIdsRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.StopDataCollectionByAgentIdsResponse;
import software.amazon.awssdk.services.applicationdiscovery.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.applicationdiscovery.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.applicationdiscovery.transform.AssociateConfigurationItemsToApplicationRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.AssociateConfigurationItemsToApplicationResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.CreateApplicationRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.CreateApplicationResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.CreateTagsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.CreateTagsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DeleteApplicationsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DeleteApplicationsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DeleteTagsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DeleteTagsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeAgentsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeAgentsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeConfigurationsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeExportConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeExportConfigurationsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeExportTasksRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeExportTasksResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeTagsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DescribeTagsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DisassociateConfigurationItemsFromApplicationRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.DisassociateConfigurationItemsFromApplicationResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.ExportConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.ExportConfigurationsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.GetDiscoverySummaryRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.GetDiscoverySummaryResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.ListConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.ListConfigurationsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.ListServerNeighborsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.ListServerNeighborsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StartDataCollectionByAgentIdsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StartDataCollectionByAgentIdsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StartExportTaskRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StartExportTaskResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StopDataCollectionByAgentIdsRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.StopDataCollectionByAgentIdsResponseUnmarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.UpdateApplicationRequestMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.transform.UpdateApplicationResponseUnmarshaller;
/**
* Internal implementation of {@link ApplicationDiscoveryAsyncClient}.
*
* @see ApplicationDiscoveryAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultApplicationDiscoveryAsyncClient implements ApplicationDiscoveryAsyncClient {
private final AsyncClientHandler clientHandler;
private final SdkJsonProtocolFactory protocolFactory;
protected DefaultApplicationDiscoveryAsyncClient(AsyncClientConfiguration clientConfiguration) {
this.clientHandler = new SdkAsyncClientHandler(clientConfiguration, null);
this.protocolFactory = init();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Associates one or more configuration items with an application.
*
*
* @param associateConfigurationItemsToApplicationRequest
* @return A Java Future containing the result of the AssociateConfigurationItemsToApplication operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.AssociateConfigurationItemsToApplication
*/
@Override
public CompletableFuture associateConfigurationItemsToApplication(
AssociateConfigurationItemsToApplicationRequest associateConfigurationItemsToApplicationRequest) {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateConfigurationItemsToApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withMarshaller(new AssociateConfigurationItemsToApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(associateConfigurationItemsToApplicationRequest));
}
/**
*
* Creates an application with the given name and description.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.CreateApplication
*/
@Override
public CompletableFuture createApplication(CreateApplicationRequest createApplicationRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new CreateApplicationRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createApplicationRequest));
}
/**
*
* Creates one or more tags for configuration items. Tags are metadata that help you categorize IT assets. This API
* accepts a list of multiple configuration items.
*
*
* @param createTagsRequest
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.CreateTags
*/
@Override
public CompletableFuture createTags(CreateTagsRequest createTagsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateTagsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new CreateTagsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createTagsRequest));
}
/**
*
* Deletes a list of applications and their associations with configuration items.
*
*
* @param deleteApplicationsRequest
* @return A Java Future containing the result of the DeleteApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DeleteApplications
*/
@Override
public CompletableFuture deleteApplications(DeleteApplicationsRequest deleteApplicationsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteApplicationsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new DeleteApplicationsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteApplicationsRequest));
}
/**
*
* Deletes the association between configuration items and one or more tags. This API accepts a list of multiple
* configuration items.
*
*
* @param deleteTagsRequest
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DeleteTags
*/
@Override
public CompletableFuture deleteTags(DeleteTagsRequest deleteTagsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteTagsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new DeleteTagsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteTagsRequest));
}
/**
*
* Lists agents or the Connector by ID or lists all agents/Connectors associated with your user account if you did
* not specify an ID.
*
*
* @param describeAgentsRequest
* @return A Java Future containing the result of the DescribeAgents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeAgents
*/
@Override
public CompletableFuture describeAgents(DescribeAgentsRequest describeAgentsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAgentsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new DescribeAgentsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeAgentsRequest));
}
/**
*
* Retrieves attributes for a list of configuration item IDs. All of the supplied IDs must be for the same asset
* type (server, application, process, or connection). Output fields are specific to the asset type selected. For
* example, the output for a server configuration item includes a list of attributes about the server, such
* as host name, operating system, and number of network cards.
*
*
* For a complete list of outputs for each asset type, see Using the DescribeConfigurations Action.
*
*
* @param describeConfigurationsRequest
* @return A Java Future containing the result of the DescribeConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeConfigurations
*/
@Override
public CompletableFuture describeConfigurations(
DescribeConfigurationsRequest describeConfigurationsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeConfigurationsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new DescribeConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeConfigurationsRequest));
}
/**
*
* Deprecated. Use DescribeExportTasks
instead.
*
*
* Retrieves the status of a given export process. You can retrieve status from a maximum of 100 processes.
*
*
* @param describeExportConfigurationsRequest
* @return A Java Future containing the result of the DescribeExportConfigurations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeExportConfigurations
*/
@Override
public CompletableFuture describeExportConfigurations(
DescribeExportConfigurationsRequest describeExportConfigurationsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeExportConfigurationsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withMarshaller(new DescribeExportConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeExportConfigurationsRequest));
}
/**
*
* Retrieve status of one or more export tasks. You can retrieve the status of up to 100 export tasks.
*
*
* @param describeExportTasksRequest
* @return A Java Future containing the result of the DescribeExportTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeExportTasks
*/
@Override
public CompletableFuture describeExportTasks(
DescribeExportTasksRequest describeExportTasksRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeExportTasksResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new DescribeExportTasksRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeExportTasksRequest));
}
/**
*
* Retrieves a list of configuration items that are tagged with a specific tag. Or retrieves a list of all tags
* assigned to a specific configuration item.
*
*
* @param describeTagsRequest
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DescribeTags
*/
@Override
public CompletableFuture describeTags(DescribeTagsRequest describeTagsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeTagsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new DescribeTagsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeTagsRequest));
}
/**
*
* Disassociates one or more configuration items from an application.
*
*
* @param disassociateConfigurationItemsFromApplicationRequest
* @return A Java Future containing the result of the DisassociateConfigurationItemsFromApplication operation
* returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.DisassociateConfigurationItemsFromApplication
*/
@Override
public CompletableFuture disassociateConfigurationItemsFromApplication(
DisassociateConfigurationItemsFromApplicationRequest disassociateConfigurationItemsFromApplicationRequest) {
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateConfigurationItemsFromApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withMarshaller(new DisassociateConfigurationItemsFromApplicationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(disassociateConfigurationItemsFromApplicationRequest));
}
/**
*
* Deprecated. Use StartExportTask
instead.
*
*
* Exports all discovered configuration data to an Amazon S3 bucket or an application that enables you to view and
* evaluate the data. Data includes tags and tag associations, processes, connections, servers, and system
* performance. This API returns an export ID that you can query using the DescribeExportConfigurations API.
* The system imposes a limit of two configuration exports in six hours.
*
*
* @param exportConfigurationsRequest
* @return A Java Future containing the result of the ExportConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ExportConfigurations
*/
@Override
public CompletableFuture exportConfigurations(
ExportConfigurationsRequest exportConfigurationsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ExportConfigurationsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new ExportConfigurationsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(exportConfigurationsRequest));
}
/**
*
* Retrieves a short summary of discovered assets.
*
*
* @param getDiscoverySummaryRequest
* @return A Java Future containing the result of the GetDiscoverySummary operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.GetDiscoverySummary
*/
@Override
public CompletableFuture getDiscoverySummary(
GetDiscoverySummaryRequest getDiscoverySummaryRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetDiscoverySummaryResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new GetDiscoverySummaryRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getDiscoverySummaryRequest));
}
/**
*
* Retrieves a list of configuration items according to criteria that you specify in a filter. The filter criteria
* identifies the relationship requirements.
*
*
* @param listConfigurationsRequest
* @return A Java Future containing the result of the ListConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - ResourceNotFoundException The specified configuration ID was not located. Verify the configuration ID
* and try again.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListConfigurations
*/
@Override
public CompletableFuture listConfigurations(ListConfigurationsRequest listConfigurationsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListConfigurationsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new ListConfigurationsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listConfigurationsRequest));
}
/**
*
* Retrieves a list of servers that are one network hop away from a specified server.
*
*
* @param listServerNeighborsRequest
* @return A Java Future containing the result of the ListServerNeighbors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.ListServerNeighbors
*/
@Override
public CompletableFuture listServerNeighbors(
ListServerNeighborsRequest listServerNeighborsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListServerNeighborsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new ListServerNeighborsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listServerNeighborsRequest));
}
/**
*
* Instructs the specified agents or connectors to start collecting data.
*
*
* @param startDataCollectionByAgentIdsRequest
* @return A Java Future containing the result of the StartDataCollectionByAgentIds operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartDataCollectionByAgentIds
*/
@Override
public CompletableFuture startDataCollectionByAgentIds(
StartDataCollectionByAgentIdsRequest startDataCollectionByAgentIdsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartDataCollectionByAgentIdsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withMarshaller(new StartDataCollectionByAgentIdsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(startDataCollectionByAgentIdsRequest));
}
/**
*
* Begins the export of discovered data to an S3 bucket.
*
*
* If you specify agentId
in a filter, the task exports up to 72 hours of detailed data collected by
* the identified Application Discovery Agent, including network, process, and performance details. A time range for
* exported agent data may be set by using startTime
and endTime
. Export of detailed agent
* data is limited to five concurrently running exports.
*
*
* If you do not include an agentId
filter, summary data is exported that includes both AWS Agentless
* Discovery Connector data and summary data from AWS Discovery Agents. Export of summary data is limited to two
* exports per day.
*
*
* @param startExportTaskRequest
* @return A Java Future containing the result of the StartExportTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - OperationNotPermittedException This operation is not permitted.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StartExportTask
*/
@Override
public CompletableFuture startExportTask(StartExportTaskRequest startExportTaskRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartExportTaskResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new StartExportTaskRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startExportTaskRequest));
}
/**
*
* Instructs the specified agents or connectors to stop collecting data.
*
*
* @param stopDataCollectionByAgentIdsRequest
* @return A Java Future containing the result of the StopDataCollectionByAgentIds operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.StopDataCollectionByAgentIds
*/
@Override
public CompletableFuture stopDataCollectionByAgentIds(
StopDataCollectionByAgentIdsRequest stopDataCollectionByAgentIdsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopDataCollectionByAgentIdsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withMarshaller(new StopDataCollectionByAgentIdsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(stopDataCollectionByAgentIdsRequest));
}
/**
*
* Updates metadata about an application.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AuthorizationErrorException The AWS user account does not have permission to perform the action.
* Check the IAM policy associated with this account.
* - InvalidParameterException One or more parameters are not valid. Verify the parameters and try again.
* - InvalidParameterValueException The value of one or more parameters are either invalid or out of
* range. Verify the parameter values and try again.
* - ServerInternalErrorException The server experienced an internal error. Try again.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ApplicationDiscoveryException Base class for all service exceptions. Unknown exceptions will be
* thrown as an instance of this type.
*
* @sample ApplicationDiscoveryAsyncClient.UpdateApplication
*/
@Override
public CompletableFuture updateApplication(UpdateApplicationRequest updateApplicationRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new UpdateApplicationRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateApplicationRequest));
}
@Override
public void close() {
clientHandler.close();
}
private software.amazon.awssdk.core.protocol.json.SdkJsonProtocolFactory init() {
return new SdkJsonProtocolFactory(new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withBaseServiceExceptionClass(
software.amazon.awssdk.services.applicationdiscovery.model.ApplicationDiscoveryException.class)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServerInternalErrorException").withModeledClass(
ServerInternalErrorException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterException").withModeledClass(
InvalidParameterException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterValueException").withModeledClass(
InvalidParameterValueException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withModeledClass(
ResourceNotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("OperationNotPermittedException").withModeledClass(
OperationNotPermittedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AuthorizationErrorException").withModeledClass(
AuthorizationErrorException.class)));
}
private HttpResponseHandler createErrorResponseHandler() {
return protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
}
}