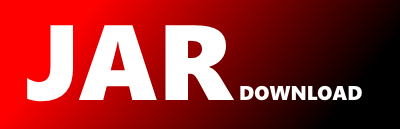
software.amazon.awssdk.services.applicationdiscovery.model.NeighborConnectionDetail Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.applicationdiscovery.model;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.core.protocol.StructuredPojo;
import software.amazon.awssdk.services.applicationdiscovery.transform.NeighborConnectionDetailMarshaller;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details about neighboring servers.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class NeighborConnectionDetail implements StructuredPojo,
ToCopyableBuilder {
private final String sourceServerId;
private final String destinationServerId;
private final Integer destinationPort;
private final String transportProtocol;
private final Long connectionsCount;
private NeighborConnectionDetail(BuilderImpl builder) {
this.sourceServerId = builder.sourceServerId;
this.destinationServerId = builder.destinationServerId;
this.destinationPort = builder.destinationPort;
this.transportProtocol = builder.transportProtocol;
this.connectionsCount = builder.connectionsCount;
}
/**
*
* The ID of the server that opened the network connection.
*
*
* @return The ID of the server that opened the network connection.
*/
public String sourceServerId() {
return sourceServerId;
}
/**
*
* The ID of the server that accepted the network connection.
*
*
* @return The ID of the server that accepted the network connection.
*/
public String destinationServerId() {
return destinationServerId;
}
/**
*
* The destination network port for the connection.
*
*
* @return The destination network port for the connection.
*/
public Integer destinationPort() {
return destinationPort;
}
/**
*
* The network protocol used for the connection.
*
*
* @return The network protocol used for the connection.
*/
public String transportProtocol() {
return transportProtocol;
}
/**
*
* The number of open network connections with the neighboring server.
*
*
* @return The number of open network connections with the neighboring server.
*/
public Long connectionsCount() {
return connectionsCount;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(sourceServerId());
hashCode = 31 * hashCode + Objects.hashCode(destinationServerId());
hashCode = 31 * hashCode + Objects.hashCode(destinationPort());
hashCode = 31 * hashCode + Objects.hashCode(transportProtocol());
hashCode = 31 * hashCode + Objects.hashCode(connectionsCount());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NeighborConnectionDetail)) {
return false;
}
NeighborConnectionDetail other = (NeighborConnectionDetail) obj;
return Objects.equals(sourceServerId(), other.sourceServerId())
&& Objects.equals(destinationServerId(), other.destinationServerId())
&& Objects.equals(destinationPort(), other.destinationPort())
&& Objects.equals(transportProtocol(), other.transportProtocol())
&& Objects.equals(connectionsCount(), other.connectionsCount());
}
@Override
public String toString() {
return ToString.builder("NeighborConnectionDetail").add("SourceServerId", sourceServerId())
.add("DestinationServerId", destinationServerId()).add("DestinationPort", destinationPort())
.add("TransportProtocol", transportProtocol()).add("ConnectionsCount", connectionsCount()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "sourceServerId":
return Optional.of(clazz.cast(sourceServerId()));
case "destinationServerId":
return Optional.of(clazz.cast(destinationServerId()));
case "destinationPort":
return Optional.of(clazz.cast(destinationPort()));
case "transportProtocol":
return Optional.of(clazz.cast(transportProtocol()));
case "connectionsCount":
return Optional.of(clazz.cast(connectionsCount()));
default:
return Optional.empty();
}
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
NeighborConnectionDetailMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
*
* The ID of the server that opened the network connection.
*
*
* @param sourceServerId
* The ID of the server that opened the network connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sourceServerId(String sourceServerId);
/**
*
* The ID of the server that accepted the network connection.
*
*
* @param destinationServerId
* The ID of the server that accepted the network connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder destinationServerId(String destinationServerId);
/**
*
* The destination network port for the connection.
*
*
* @param destinationPort
* The destination network port for the connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder destinationPort(Integer destinationPort);
/**
*
* The network protocol used for the connection.
*
*
* @param transportProtocol
* The network protocol used for the connection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder transportProtocol(String transportProtocol);
/**
*
* The number of open network connections with the neighboring server.
*
*
* @param connectionsCount
* The number of open network connections with the neighboring server.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder connectionsCount(Long connectionsCount);
}
static final class BuilderImpl implements Builder {
private String sourceServerId;
private String destinationServerId;
private Integer destinationPort;
private String transportProtocol;
private Long connectionsCount;
private BuilderImpl() {
}
private BuilderImpl(NeighborConnectionDetail model) {
sourceServerId(model.sourceServerId);
destinationServerId(model.destinationServerId);
destinationPort(model.destinationPort);
transportProtocol(model.transportProtocol);
connectionsCount(model.connectionsCount);
}
public final String getSourceServerId() {
return sourceServerId;
}
@Override
public final Builder sourceServerId(String sourceServerId) {
this.sourceServerId = sourceServerId;
return this;
}
public final void setSourceServerId(String sourceServerId) {
this.sourceServerId = sourceServerId;
}
public final String getDestinationServerId() {
return destinationServerId;
}
@Override
public final Builder destinationServerId(String destinationServerId) {
this.destinationServerId = destinationServerId;
return this;
}
public final void setDestinationServerId(String destinationServerId) {
this.destinationServerId = destinationServerId;
}
public final Integer getDestinationPort() {
return destinationPort;
}
@Override
public final Builder destinationPort(Integer destinationPort) {
this.destinationPort = destinationPort;
return this;
}
public final void setDestinationPort(Integer destinationPort) {
this.destinationPort = destinationPort;
}
public final String getTransportProtocol() {
return transportProtocol;
}
@Override
public final Builder transportProtocol(String transportProtocol) {
this.transportProtocol = transportProtocol;
return this;
}
public final void setTransportProtocol(String transportProtocol) {
this.transportProtocol = transportProtocol;
}
public final Long getConnectionsCount() {
return connectionsCount;
}
@Override
public final Builder connectionsCount(Long connectionsCount) {
this.connectionsCount = connectionsCount;
return this;
}
public final void setConnectionsCount(Long connectionsCount) {
this.connectionsCount = connectionsCount;
}
@Override
public NeighborConnectionDetail build() {
return new NeighborConnectionDetail(this);
}
}
}