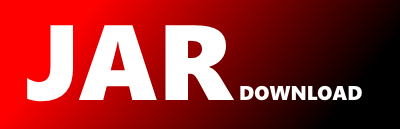
software.amazon.awssdk.services.applicationdiscovery.model.StartExportTaskRequest Maven / Gradle / Ivy
Show all versions of discovery Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.applicationdiscovery.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import javax.annotation.Generated;
import software.amazon.awssdk.core.AwsRequestOverrideConfig;
import software.amazon.awssdk.core.runtime.TypeConverter;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public class StartExportTaskRequest extends ApplicationDiscoveryRequest implements
ToCopyableBuilder {
private final List exportDataFormat;
private final List filters;
private final Instant startTime;
private final Instant endTime;
private StartExportTaskRequest(BuilderImpl builder) {
super(builder);
this.exportDataFormat = builder.exportDataFormat;
this.filters = builder.filters;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
}
/**
*
* The file format for the returned export data. Default value is CSV
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The file format for the returned export data. Default value is CSV
.
*/
public List exportDataFormat() {
return TypeConverter.convert(exportDataFormat, ExportDataFormat::fromValue);
}
/**
*
* The file format for the returned export data. Default value is CSV
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The file format for the returned export data. Default value is CSV
.
*/
public List exportDataFormatStrings() {
return exportDataFormat;
}
/**
*
* If a filter is present, it selects the single agentId
of the Application Discovery Agent for which
* data is exported. The agentId
can be found in the results of the DescribeAgents
API or
* CLI. If no filter is present, startTime
and endTime
are ignored and exported data
* includes both Agentless Discovery Connector data and summary data from Application Discovery agents.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return If a filter is present, it selects the single agentId
of the Application Discovery Agent for
* which data is exported. The agentId
can be found in the results of the
* DescribeAgents
API or CLI. If no filter is present, startTime
and
* endTime
are ignored and exported data includes both Agentless Discovery Connector data and
* summary data from Application Discovery agents.
*/
public List filters() {
return filters;
}
/**
*
* The start timestamp for exported data from the single Application Discovery Agent selected in the filters. If no
* value is specified, data is exported starting from the first data collected by the agent.
*
*
* @return The start timestamp for exported data from the single Application Discovery Agent selected in the
* filters. If no value is specified, data is exported starting from the first data collected by the agent.
*/
public Instant startTime() {
return startTime;
}
/**
*
* The end timestamp for exported data from the single Application Discovery Agent selected in the filters. If no
* value is specified, exported data includes the most recent data collected by the agent.
*
*
* @return The end timestamp for exported data from the single Application Discovery Agent selected in the filters.
* If no value is specified, exported data includes the most recent data collected by the agent.
*/
public Instant endTime() {
return endTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(exportDataFormatStrings());
hashCode = 31 * hashCode + Objects.hashCode(filters());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartExportTaskRequest)) {
return false;
}
StartExportTaskRequest other = (StartExportTaskRequest) obj;
return Objects.equals(exportDataFormatStrings(), other.exportDataFormatStrings())
&& Objects.equals(filters(), other.filters()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(endTime(), other.endTime());
}
@Override
public String toString() {
return ToString.builder("StartExportTaskRequest").add("ExportDataFormat", exportDataFormatStrings())
.add("Filters", filters()).add("StartTime", startTime()).add("EndTime", endTime()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "exportDataFormat":
return Optional.of(clazz.cast(exportDataFormatStrings()));
case "filters":
return Optional.of(clazz.cast(filters()));
case "startTime":
return Optional.of(clazz.cast(startTime()));
case "endTime":
return Optional.of(clazz.cast(endTime()));
default:
return Optional.empty();
}
}
public interface Builder extends ApplicationDiscoveryRequest.Builder, CopyableBuilder {
/**
*
* The file format for the returned export data. Default value is CSV
.
*
*
* @param exportDataFormat
* The file format for the returned export data. Default value is CSV
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder exportDataFormat(Collection exportDataFormat);
/**
*
* The file format for the returned export data. Default value is CSV
.
*
*
* @param exportDataFormat
* The file format for the returned export data. Default value is CSV
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder exportDataFormat(String... exportDataFormat);
/**
*
* If a filter is present, it selects the single agentId
of the Application Discovery Agent for
* which data is exported. The agentId
can be found in the results of the
* DescribeAgents
API or CLI. If no filter is present, startTime
and
* endTime
are ignored and exported data includes both Agentless Discovery Connector data and
* summary data from Application Discovery agents.
*
*
* @param filters
* If a filter is present, it selects the single agentId
of the Application Discovery Agent
* for which data is exported. The agentId
can be found in the results of the
* DescribeAgents
API or CLI. If no filter is present, startTime
and
* endTime
are ignored and exported data includes both Agentless Discovery Connector data
* and summary data from Application Discovery agents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder filters(Collection filters);
/**
*
* If a filter is present, it selects the single agentId
of the Application Discovery Agent for
* which data is exported. The agentId
can be found in the results of the
* DescribeAgents
API or CLI. If no filter is present, startTime
and
* endTime
are ignored and exported data includes both Agentless Discovery Connector data and
* summary data from Application Discovery agents.
*
*
* @param filters
* If a filter is present, it selects the single agentId
of the Application Discovery Agent
* for which data is exported. The agentId
can be found in the results of the
* DescribeAgents
API or CLI. If no filter is present, startTime
and
* endTime
are ignored and exported data includes both Agentless Discovery Connector data
* and summary data from Application Discovery agents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder filters(ExportFilter... filters);
/**
*
* The start timestamp for exported data from the single Application Discovery Agent selected in the filters. If
* no value is specified, data is exported starting from the first data collected by the agent.
*
*
* @param startTime
* The start timestamp for exported data from the single Application Discovery Agent selected in the
* filters. If no value is specified, data is exported starting from the first data collected by the
* agent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder startTime(Instant startTime);
/**
*
* The end timestamp for exported data from the single Application Discovery Agent selected in the filters. If
* no value is specified, exported data includes the most recent data collected by the agent.
*
*
* @param endTime
* The end timestamp for exported data from the single Application Discovery Agent selected in the
* filters. If no value is specified, exported data includes the most recent data collected by the agent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder endTime(Instant endTime);
@Override
Builder requestOverrideConfig(AwsRequestOverrideConfig awsRequestOverrideConfig);
}
static final class BuilderImpl extends ApplicationDiscoveryRequest.BuilderImpl implements Builder {
private List exportDataFormat;
private List filters;
private Instant startTime;
private Instant endTime;
private BuilderImpl() {
}
private BuilderImpl(StartExportTaskRequest model) {
exportDataFormat(model.exportDataFormat);
filters(model.filters);
startTime(model.startTime);
endTime(model.endTime);
}
public final Collection getExportDataFormat() {
return exportDataFormat;
}
@Override
public final Builder exportDataFormat(Collection exportDataFormat) {
this.exportDataFormat = ExportDataFormatsCopier.copy(exportDataFormat);
return this;
}
@Override
@SafeVarargs
public final Builder exportDataFormat(String... exportDataFormat) {
exportDataFormat(Arrays.asList(exportDataFormat));
return this;
}
public final void setExportDataFormat(Collection exportDataFormat) {
this.exportDataFormat = ExportDataFormatsCopier.copy(exportDataFormat);
}
public final Collection getFilters() {
return filters != null ? filters.stream().map(ExportFilter::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder filters(Collection filters) {
this.filters = ExportFiltersCopier.copy(filters);
return this;
}
@Override
@SafeVarargs
public final Builder filters(ExportFilter... filters) {
filters(Arrays.asList(filters));
return this;
}
public final void setFilters(Collection filters) {
this.filters = ExportFiltersCopier.copyFromBuilder(filters);
}
public final Instant getStartTime() {
return startTime;
}
@Override
public final Builder startTime(Instant startTime) {
this.startTime = startTime;
return this;
}
public final void setStartTime(Instant startTime) {
this.startTime = startTime;
}
public final Instant getEndTime() {
return endTime;
}
@Override
public final Builder endTime(Instant endTime) {
this.endTime = endTime;
return this;
}
public final void setEndTime(Instant endTime) {
this.endTime = endTime;
}
@Override
public Builder requestOverrideConfig(AwsRequestOverrideConfig awsRequestOverrideConfig) {
super.requestOverrideConfig(awsRequestOverrideConfig);
return this;
}
@Override
public Builder requestOverrideConfig(Consumer builderConsumer) {
super.requestOverrideConfig(builderConsumer);
return this;
}
@Override
public StartExportTaskRequest build() {
return new StartExportTaskRequest(this);
}
}
}