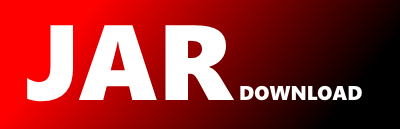
software.amazon.awssdk.services.applicationdiscovery.transform.ExportInfoMarshaller Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.applicationdiscovery.transform;
import java.time.Instant;
import javax.annotation.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingInfo;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.services.applicationdiscovery.model.ExportInfo;
import software.amazon.awssdk.utils.Validate;
/**
* {@link ExportInfo} Marshaller
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
public class ExportInfoMarshaller {
private static final MarshallingInfo EXPORTID_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("exportId").isBinary(false).build();
private static final MarshallingInfo EXPORTSTATUS_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("exportStatus").isBinary(false).build();
private static final MarshallingInfo STATUSMESSAGE_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("statusMessage").isBinary(false).build();
private static final MarshallingInfo CONFIGURATIONSDOWNLOADURL_BINDING = MarshallingInfo
.builder(MarshallingType.STRING).marshallLocation(MarshallLocation.PAYLOAD)
.marshallLocationName("configurationsDownloadUrl").isBinary(false).build();
private static final MarshallingInfo EXPORTREQUESTTIME_BINDING = MarshallingInfo.builder(MarshallingType.INSTANT)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("exportRequestTime").isBinary(false).build();
private static final MarshallingInfo ISTRUNCATED_BINDING = MarshallingInfo.builder(MarshallingType.BOOLEAN)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("isTruncated").isBinary(false).build();
private static final MarshallingInfo REQUESTEDSTARTTIME_BINDING = MarshallingInfo.builder(MarshallingType.INSTANT)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("requestedStartTime").isBinary(false).build();
private static final MarshallingInfo REQUESTEDENDTIME_BINDING = MarshallingInfo.builder(MarshallingType.INSTANT)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("requestedEndTime").isBinary(false).build();
private static final ExportInfoMarshaller INSTANCE = new ExportInfoMarshaller();
private ExportInfoMarshaller() {
}
public static ExportInfoMarshaller getInstance() {
return INSTANCE;
}
/**
* Marshall the given parameter object
*/
public void marshall(ExportInfo exportInfo, ProtocolMarshaller protocolMarshaller) {
Validate.paramNotNull(exportInfo, "exportInfo");
Validate.paramNotNull(protocolMarshaller, "protocolMarshaller");
try {
protocolMarshaller.marshall(exportInfo.exportId(), EXPORTID_BINDING);
protocolMarshaller.marshall(exportInfo.exportStatusString(), EXPORTSTATUS_BINDING);
protocolMarshaller.marshall(exportInfo.statusMessage(), STATUSMESSAGE_BINDING);
protocolMarshaller.marshall(exportInfo.configurationsDownloadUrl(), CONFIGURATIONSDOWNLOADURL_BINDING);
protocolMarshaller.marshall(exportInfo.exportRequestTime(), EXPORTREQUESTTIME_BINDING);
protocolMarshaller.marshall(exportInfo.isTruncated(), ISTRUNCATED_BINDING);
protocolMarshaller.marshall(exportInfo.requestedStartTime(), REQUESTEDSTARTTIME_BINDING);
protocolMarshaller.marshall(exportInfo.requestedEndTime(), REQUESTEDENDTIME_BINDING);
} catch (Exception e) {
throw new SdkClientException("Unable to marshall request to JSON: " + e.getMessage(), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy