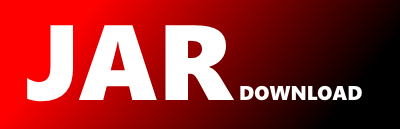
software.amazon.awssdk.services.databasemigration.model.CreateReplicationInstanceRequest Maven / Gradle / Ivy
Show all versions of dms Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateReplicationInstanceRequest extends DatabaseMigrationRequest implements
ToCopyableBuilder {
private final String replicationInstanceIdentifier;
private final Integer allocatedStorage;
private final String replicationInstanceClass;
private final List vpcSecurityGroupIds;
private final String availabilityZone;
private final String replicationSubnetGroupIdentifier;
private final String preferredMaintenanceWindow;
private final Boolean multiAZ;
private final String engineVersion;
private final Boolean autoMinorVersionUpgrade;
private final List tags;
private final String kmsKeyId;
private final Boolean publiclyAccessible;
private CreateReplicationInstanceRequest(BuilderImpl builder) {
super(builder);
this.replicationInstanceIdentifier = builder.replicationInstanceIdentifier;
this.allocatedStorage = builder.allocatedStorage;
this.replicationInstanceClass = builder.replicationInstanceClass;
this.vpcSecurityGroupIds = builder.vpcSecurityGroupIds;
this.availabilityZone = builder.availabilityZone;
this.replicationSubnetGroupIdentifier = builder.replicationSubnetGroupIdentifier;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.multiAZ = builder.multiAZ;
this.engineVersion = builder.engineVersion;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.tags = builder.tags;
this.kmsKeyId = builder.kmsKeyId;
this.publiclyAccessible = builder.publiclyAccessible;
}
/**
*
* The replication instance identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
*
*
* @return The replication instance identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
*/
public String replicationInstanceIdentifier() {
return replicationInstanceIdentifier;
}
/**
*
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*
*
* @return The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*/
public Integer allocatedStorage() {
return allocatedStorage;
}
/**
*
* The compute and memory capacity of the replication instance as specified by the replication instance class.
*
*
* Valid Values:
* dms.t2.micro | dms.t2.small | dms.t2.medium | dms.t2.large | dms.c4.large | dms.c4.xlarge | dms.c4.2xlarge | dms.c4.4xlarge
*
*
* @return The compute and memory capacity of the replication instance as specified by the replication instance
* class.
*
* Valid Values:
* dms.t2.micro | dms.t2.small | dms.t2.medium | dms.t2.large | dms.c4.large | dms.c4.xlarge | dms.c4.2xlarge | dms.c4.4xlarge
*/
public String replicationInstanceClass() {
return replicationInstanceClass;
}
/**
*
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with
* the VPC containing the replication instance.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Specifies the VPC security group to be used with the replication instance. The VPC security group must
* work with the VPC containing the replication instance.
*/
public List vpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
/**
*
* The EC2 Availability Zone that the replication instance will be created in.
*
*
* Default: A random, system-chosen Availability Zone in the endpoint's region.
*
*
* Example: us-east-1d
*
*
* @return The EC2 Availability Zone that the replication instance will be created in.
*
* Default: A random, system-chosen Availability Zone in the endpoint's region.
*
*
* Example: us-east-1d
*/
public String availabilityZone() {
return availabilityZone;
}
/**
*
* A subnet group to associate with the replication instance.
*
*
* @return A subnet group to associate with the replication instance.
*/
public String replicationSubnetGroupIdentifier() {
return replicationSubnetGroupIdentifier;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a random day
* of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
*
*
* @return The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a
* random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
*/
public String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* Specifies if the replication instance is a Multi-AZ deployment. You cannot set the AvailabilityZone
* parameter if the Multi-AZ parameter is set to true
.
*
*
* @return Specifies if the replication instance is a Multi-AZ deployment. You cannot set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*/
public Boolean multiAZ() {
return multiAZ;
}
/**
*
* The engine version number of the replication instance.
*
*
* @return The engine version number of the replication instance.
*/
public String engineVersion() {
return engineVersion;
}
/**
*
* Indicates that minor engine upgrades will be applied automatically to the replication instance during the
* maintenance window.
*
*
* Default: true
*
*
* @return Indicates that minor engine upgrades will be applied automatically to the replication instance during the
* maintenance window.
*
* Default: true
*/
public Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* Tags to be associated with the replication instance.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Tags to be associated with the replication instance.
*/
public List tags() {
return tags;
}
/**
*
* The KMS key identifier that will be used to encrypt the content on the replication instance. If you do not
* specify a value for the KmsKeyId parameter, then AWS DMS will use your default encryption key. AWS KMS creates
* the default encryption key for your AWS account. Your AWS account has a different default encryption key for each
* AWS region.
*
*
* @return The KMS key identifier that will be used to encrypt the content on the replication instance. If you do
* not specify a value for the KmsKeyId parameter, then AWS DMS will use your default encryption key. AWS
* KMS creates the default encryption key for your AWS account. Your AWS account has a different default
* encryption key for each AWS region.
*/
public String kmsKeyId() {
return kmsKeyId;
}
/**
*
* Specifies the accessibility options for the replication instance. A value of true
represents an
* instance with a public IP address. A value of false
represents an instance with a private IP
* address. The default value is true
.
*
*
* @return Specifies the accessibility options for the replication instance. A value of true
represents
* an instance with a public IP address. A value of false
represents an instance with a private
* IP address. The default value is true
.
*/
public Boolean publiclyAccessible() {
return publiclyAccessible;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(replicationInstanceIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(allocatedStorage());
hashCode = 31 * hashCode + Objects.hashCode(replicationInstanceClass());
hashCode = 31 * hashCode + Objects.hashCode(vpcSecurityGroupIds());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(replicationSubnetGroupIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(multiAZ());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(tags());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(publiclyAccessible());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateReplicationInstanceRequest)) {
return false;
}
CreateReplicationInstanceRequest other = (CreateReplicationInstanceRequest) obj;
return Objects.equals(replicationInstanceIdentifier(), other.replicationInstanceIdentifier())
&& Objects.equals(allocatedStorage(), other.allocatedStorage())
&& Objects.equals(replicationInstanceClass(), other.replicationInstanceClass())
&& Objects.equals(vpcSecurityGroupIds(), other.vpcSecurityGroupIds())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(replicationSubnetGroupIdentifier(), other.replicationSubnetGroupIdentifier())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(multiAZ(), other.multiAZ()) && Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(tags(), other.tags()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(publiclyAccessible(), other.publiclyAccessible());
}
@Override
public String toString() {
return ToString.builder("CreateReplicationInstanceRequest")
.add("ReplicationInstanceIdentifier", replicationInstanceIdentifier())
.add("AllocatedStorage", allocatedStorage()).add("ReplicationInstanceClass", replicationInstanceClass())
.add("VpcSecurityGroupIds", vpcSecurityGroupIds()).add("AvailabilityZone", availabilityZone())
.add("ReplicationSubnetGroupIdentifier", replicationSubnetGroupIdentifier())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow()).add("MultiAZ", multiAZ())
.add("EngineVersion", engineVersion()).add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade())
.add("Tags", tags()).add("KmsKeyId", kmsKeyId()).add("PubliclyAccessible", publiclyAccessible()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ReplicationInstanceIdentifier":
return Optional.ofNullable(clazz.cast(replicationInstanceIdentifier()));
case "AllocatedStorage":
return Optional.ofNullable(clazz.cast(allocatedStorage()));
case "ReplicationInstanceClass":
return Optional.ofNullable(clazz.cast(replicationInstanceClass()));
case "VpcSecurityGroupIds":
return Optional.ofNullable(clazz.cast(vpcSecurityGroupIds()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "ReplicationSubnetGroupIdentifier":
return Optional.ofNullable(clazz.cast(replicationSubnetGroupIdentifier()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "MultiAZ":
return Optional.ofNullable(clazz.cast(multiAZ()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "PubliclyAccessible":
return Optional.ofNullable(clazz.cast(publiclyAccessible()));
default:
return Optional.empty();
}
}
public interface Builder extends DatabaseMigrationRequest.Builder, CopyableBuilder {
/**
*
* The replication instance identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
*
*
* @param replicationInstanceIdentifier
* The replication instance identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationInstanceIdentifier(String replicationInstanceIdentifier);
/**
*
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*
*
* @param allocatedStorage
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder allocatedStorage(Integer allocatedStorage);
/**
*
* The compute and memory capacity of the replication instance as specified by the replication instance class.
*
*
* Valid Values:
* dms.t2.micro | dms.t2.small | dms.t2.medium | dms.t2.large | dms.c4.large | dms.c4.xlarge | dms.c4.2xlarge | dms.c4.4xlarge
*
*
* @param replicationInstanceClass
* The compute and memory capacity of the replication instance as specified by the replication instance
* class.
*
* Valid Values:
* dms.t2.micro | dms.t2.small | dms.t2.medium | dms.t2.large | dms.c4.large | dms.c4.xlarge | dms.c4.2xlarge | dms.c4.4xlarge
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationInstanceClass(String replicationInstanceClass);
/**
*
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work
* with the VPC containing the replication instance.
*
*
* @param vpcSecurityGroupIds
* Specifies the VPC security group to be used with the replication instance. The VPC security group must
* work with the VPC containing the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vpcSecurityGroupIds(Collection vpcSecurityGroupIds);
/**
*
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work
* with the VPC containing the replication instance.
*
*
* @param vpcSecurityGroupIds
* Specifies the VPC security group to be used with the replication instance. The VPC security group must
* work with the VPC containing the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder vpcSecurityGroupIds(String... vpcSecurityGroupIds);
/**
*
* The EC2 Availability Zone that the replication instance will be created in.
*
*
* Default: A random, system-chosen Availability Zone in the endpoint's region.
*
*
* Example: us-east-1d
*
*
* @param availabilityZone
* The EC2 Availability Zone that the replication instance will be created in.
*
* Default: A random, system-chosen Availability Zone in the endpoint's region.
*
*
* Example: us-east-1d
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder availabilityZone(String availabilityZone);
/**
*
* A subnet group to associate with the replication instance.
*
*
* @param replicationSubnetGroupIdentifier
* A subnet group to associate with the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationSubnetGroupIdentifier(String replicationSubnetGroupIdentifier);
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a random
* day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
*
*
* @param preferredMaintenanceWindow
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time
* (UTC).
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a
* random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder preferredMaintenanceWindow(String preferredMaintenanceWindow);
/**
*
* Specifies if the replication instance is a Multi-AZ deployment. You cannot set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*
*
* @param multiAZ
* Specifies if the replication instance is a Multi-AZ deployment. You cannot set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder multiAZ(Boolean multiAZ);
/**
*
* The engine version number of the replication instance.
*
*
* @param engineVersion
* The engine version number of the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder engineVersion(String engineVersion);
/**
*
* Indicates that minor engine upgrades will be applied automatically to the replication instance during the
* maintenance window.
*
*
* Default: true
*
*
* @param autoMinorVersionUpgrade
* Indicates that minor engine upgrades will be applied automatically to the replication instance during
* the maintenance window.
*
* Default: true
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder autoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade);
/**
*
* Tags to be associated with the replication instance.
*
*
* @param tags
* Tags to be associated with the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* Tags to be associated with the replication instance.
*
*
* @param tags
* Tags to be associated with the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* Tags to be associated with the replication instance.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to create
* one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its result
* is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(List)
*/
Builder tags(Consumer... tags);
/**
*
* The KMS key identifier that will be used to encrypt the content on the replication instance. If you do not
* specify a value for the KmsKeyId parameter, then AWS DMS will use your default encryption key. AWS KMS
* creates the default encryption key for your AWS account. Your AWS account has a different default encryption
* key for each AWS region.
*
*
* @param kmsKeyId
* The KMS key identifier that will be used to encrypt the content on the replication instance. If you do
* not specify a value for the KmsKeyId parameter, then AWS DMS will use your default encryption key. AWS
* KMS creates the default encryption key for your AWS account. Your AWS account has a different default
* encryption key for each AWS region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder kmsKeyId(String kmsKeyId);
/**
*
* Specifies the accessibility options for the replication instance. A value of true
represents an
* instance with a public IP address. A value of false
represents an instance with a private IP
* address. The default value is true
.
*
*
* @param publiclyAccessible
* Specifies the accessibility options for the replication instance. A value of true
* represents an instance with a public IP address. A value of false
represents an instance
* with a private IP address. The default value is true
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder publiclyAccessible(Boolean publiclyAccessible);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends DatabaseMigrationRequest.BuilderImpl implements Builder {
private String replicationInstanceIdentifier;
private Integer allocatedStorage;
private String replicationInstanceClass;
private List vpcSecurityGroupIds = DefaultSdkAutoConstructList.getInstance();
private String availabilityZone;
private String replicationSubnetGroupIdentifier;
private String preferredMaintenanceWindow;
private Boolean multiAZ;
private String engineVersion;
private Boolean autoMinorVersionUpgrade;
private List tags = DefaultSdkAutoConstructList.getInstance();
private String kmsKeyId;
private Boolean publiclyAccessible;
private BuilderImpl() {
}
private BuilderImpl(CreateReplicationInstanceRequest model) {
super(model);
replicationInstanceIdentifier(model.replicationInstanceIdentifier);
allocatedStorage(model.allocatedStorage);
replicationInstanceClass(model.replicationInstanceClass);
vpcSecurityGroupIds(model.vpcSecurityGroupIds);
availabilityZone(model.availabilityZone);
replicationSubnetGroupIdentifier(model.replicationSubnetGroupIdentifier);
preferredMaintenanceWindow(model.preferredMaintenanceWindow);
multiAZ(model.multiAZ);
engineVersion(model.engineVersion);
autoMinorVersionUpgrade(model.autoMinorVersionUpgrade);
tags(model.tags);
kmsKeyId(model.kmsKeyId);
publiclyAccessible(model.publiclyAccessible);
}
public final String getReplicationInstanceIdentifier() {
return replicationInstanceIdentifier;
}
@Override
public final Builder replicationInstanceIdentifier(String replicationInstanceIdentifier) {
this.replicationInstanceIdentifier = replicationInstanceIdentifier;
return this;
}
public final void setReplicationInstanceIdentifier(String replicationInstanceIdentifier) {
this.replicationInstanceIdentifier = replicationInstanceIdentifier;
}
public final Integer getAllocatedStorage() {
return allocatedStorage;
}
@Override
public final Builder allocatedStorage(Integer allocatedStorage) {
this.allocatedStorage = allocatedStorage;
return this;
}
public final void setAllocatedStorage(Integer allocatedStorage) {
this.allocatedStorage = allocatedStorage;
}
public final String getReplicationInstanceClass() {
return replicationInstanceClass;
}
@Override
public final Builder replicationInstanceClass(String replicationInstanceClass) {
this.replicationInstanceClass = replicationInstanceClass;
return this;
}
public final void setReplicationInstanceClass(String replicationInstanceClass) {
this.replicationInstanceClass = replicationInstanceClass;
}
public final Collection getVpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
@Override
public final Builder vpcSecurityGroupIds(Collection vpcSecurityGroupIds) {
this.vpcSecurityGroupIds = VpcSecurityGroupIdListCopier.copy(vpcSecurityGroupIds);
return this;
}
@Override
@SafeVarargs
public final Builder vpcSecurityGroupIds(String... vpcSecurityGroupIds) {
vpcSecurityGroupIds(Arrays.asList(vpcSecurityGroupIds));
return this;
}
public final void setVpcSecurityGroupIds(Collection vpcSecurityGroupIds) {
this.vpcSecurityGroupIds = VpcSecurityGroupIdListCopier.copy(vpcSecurityGroupIds);
}
public final String getAvailabilityZone() {
return availabilityZone;
}
@Override
public final Builder availabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
return this;
}
public final void setAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
}
public final String getReplicationSubnetGroupIdentifier() {
return replicationSubnetGroupIdentifier;
}
@Override
public final Builder replicationSubnetGroupIdentifier(String replicationSubnetGroupIdentifier) {
this.replicationSubnetGroupIdentifier = replicationSubnetGroupIdentifier;
return this;
}
public final void setReplicationSubnetGroupIdentifier(String replicationSubnetGroupIdentifier) {
this.replicationSubnetGroupIdentifier = replicationSubnetGroupIdentifier;
}
public final String getPreferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
@Override
public final Builder preferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
public final void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
public final Boolean getMultiAZ() {
return multiAZ;
}
@Override
public final Builder multiAZ(Boolean multiAZ) {
this.multiAZ = multiAZ;
return this;
}
public final void setMultiAZ(Boolean multiAZ) {
this.multiAZ = multiAZ;
}
public final String getEngineVersion() {
return engineVersion;
}
@Override
public final Builder engineVersion(String engineVersion) {
this.engineVersion = engineVersion;
return this;
}
public final void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
public final Boolean getAutoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
@Override
public final Builder autoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
return this;
}
public final void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
public final Collection getTags() {
return tags != null ? tags.stream().map(Tag::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
public final String getKmsKeyId() {
return kmsKeyId;
}
@Override
public final Builder kmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
return this;
}
public final void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
public final Boolean getPubliclyAccessible() {
return publiclyAccessible;
}
@Override
public final Builder publiclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
return this;
}
public final void setPubliclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateReplicationInstanceRequest build() {
return new CreateReplicationInstanceRequest(this);
}
}
}