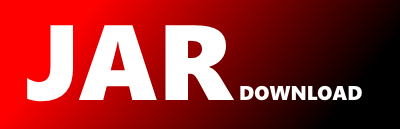
software.amazon.awssdk.services.databasemigration.model.ReplicationTaskStats Maven / Gradle / Ivy
Show all versions of dms Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.databasemigration.model;
import java.util.Objects;
import java.util.Optional;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.core.protocol.StructuredPojo;
import software.amazon.awssdk.services.databasemigration.transform.ReplicationTaskStatsMarshaller;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReplicationTaskStats implements StructuredPojo,
ToCopyableBuilder {
private final Integer fullLoadProgressPercent;
private final Long elapsedTimeMillis;
private final Integer tablesLoaded;
private final Integer tablesLoading;
private final Integer tablesQueued;
private final Integer tablesErrored;
private ReplicationTaskStats(BuilderImpl builder) {
this.fullLoadProgressPercent = builder.fullLoadProgressPercent;
this.elapsedTimeMillis = builder.elapsedTimeMillis;
this.tablesLoaded = builder.tablesLoaded;
this.tablesLoading = builder.tablesLoading;
this.tablesQueued = builder.tablesQueued;
this.tablesErrored = builder.tablesErrored;
}
/**
*
* The percent complete for the full load migration task.
*
*
* @return The percent complete for the full load migration task.
*/
public Integer fullLoadProgressPercent() {
return fullLoadProgressPercent;
}
/**
*
* The elapsed time of the task, in milliseconds.
*
*
* @return The elapsed time of the task, in milliseconds.
*/
public Long elapsedTimeMillis() {
return elapsedTimeMillis;
}
/**
*
* The number of tables loaded for this task.
*
*
* @return The number of tables loaded for this task.
*/
public Integer tablesLoaded() {
return tablesLoaded;
}
/**
*
* The number of tables currently loading for this task.
*
*
* @return The number of tables currently loading for this task.
*/
public Integer tablesLoading() {
return tablesLoading;
}
/**
*
* The number of tables queued for this task.
*
*
* @return The number of tables queued for this task.
*/
public Integer tablesQueued() {
return tablesQueued;
}
/**
*
* The number of errors that have occurred during this task.
*
*
* @return The number of errors that have occurred during this task.
*/
public Integer tablesErrored() {
return tablesErrored;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(fullLoadProgressPercent());
hashCode = 31 * hashCode + Objects.hashCode(elapsedTimeMillis());
hashCode = 31 * hashCode + Objects.hashCode(tablesLoaded());
hashCode = 31 * hashCode + Objects.hashCode(tablesLoading());
hashCode = 31 * hashCode + Objects.hashCode(tablesQueued());
hashCode = 31 * hashCode + Objects.hashCode(tablesErrored());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReplicationTaskStats)) {
return false;
}
ReplicationTaskStats other = (ReplicationTaskStats) obj;
return Objects.equals(fullLoadProgressPercent(), other.fullLoadProgressPercent())
&& Objects.equals(elapsedTimeMillis(), other.elapsedTimeMillis())
&& Objects.equals(tablesLoaded(), other.tablesLoaded()) && Objects.equals(tablesLoading(), other.tablesLoading())
&& Objects.equals(tablesQueued(), other.tablesQueued()) && Objects.equals(tablesErrored(), other.tablesErrored());
}
@Override
public String toString() {
return ToString.builder("ReplicationTaskStats").add("FullLoadProgressPercent", fullLoadProgressPercent())
.add("ElapsedTimeMillis", elapsedTimeMillis()).add("TablesLoaded", tablesLoaded())
.add("TablesLoading", tablesLoading()).add("TablesQueued", tablesQueued()).add("TablesErrored", tablesErrored())
.build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "FullLoadProgressPercent":
return Optional.ofNullable(clazz.cast(fullLoadProgressPercent()));
case "ElapsedTimeMillis":
return Optional.ofNullable(clazz.cast(elapsedTimeMillis()));
case "TablesLoaded":
return Optional.ofNullable(clazz.cast(tablesLoaded()));
case "TablesLoading":
return Optional.ofNullable(clazz.cast(tablesLoading()));
case "TablesQueued":
return Optional.ofNullable(clazz.cast(tablesQueued()));
case "TablesErrored":
return Optional.ofNullable(clazz.cast(tablesErrored()));
default:
return Optional.empty();
}
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
ReplicationTaskStatsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
*
* The percent complete for the full load migration task.
*
*
* @param fullLoadProgressPercent
* The percent complete for the full load migration task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder fullLoadProgressPercent(Integer fullLoadProgressPercent);
/**
*
* The elapsed time of the task, in milliseconds.
*
*
* @param elapsedTimeMillis
* The elapsed time of the task, in milliseconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder elapsedTimeMillis(Long elapsedTimeMillis);
/**
*
* The number of tables loaded for this task.
*
*
* @param tablesLoaded
* The number of tables loaded for this task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tablesLoaded(Integer tablesLoaded);
/**
*
* The number of tables currently loading for this task.
*
*
* @param tablesLoading
* The number of tables currently loading for this task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tablesLoading(Integer tablesLoading);
/**
*
* The number of tables queued for this task.
*
*
* @param tablesQueued
* The number of tables queued for this task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tablesQueued(Integer tablesQueued);
/**
*
* The number of errors that have occurred during this task.
*
*
* @param tablesErrored
* The number of errors that have occurred during this task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tablesErrored(Integer tablesErrored);
}
static final class BuilderImpl implements Builder {
private Integer fullLoadProgressPercent;
private Long elapsedTimeMillis;
private Integer tablesLoaded;
private Integer tablesLoading;
private Integer tablesQueued;
private Integer tablesErrored;
private BuilderImpl() {
}
private BuilderImpl(ReplicationTaskStats model) {
fullLoadProgressPercent(model.fullLoadProgressPercent);
elapsedTimeMillis(model.elapsedTimeMillis);
tablesLoaded(model.tablesLoaded);
tablesLoading(model.tablesLoading);
tablesQueued(model.tablesQueued);
tablesErrored(model.tablesErrored);
}
public final Integer getFullLoadProgressPercent() {
return fullLoadProgressPercent;
}
@Override
public final Builder fullLoadProgressPercent(Integer fullLoadProgressPercent) {
this.fullLoadProgressPercent = fullLoadProgressPercent;
return this;
}
public final void setFullLoadProgressPercent(Integer fullLoadProgressPercent) {
this.fullLoadProgressPercent = fullLoadProgressPercent;
}
public final Long getElapsedTimeMillis() {
return elapsedTimeMillis;
}
@Override
public final Builder elapsedTimeMillis(Long elapsedTimeMillis) {
this.elapsedTimeMillis = elapsedTimeMillis;
return this;
}
public final void setElapsedTimeMillis(Long elapsedTimeMillis) {
this.elapsedTimeMillis = elapsedTimeMillis;
}
public final Integer getTablesLoaded() {
return tablesLoaded;
}
@Override
public final Builder tablesLoaded(Integer tablesLoaded) {
this.tablesLoaded = tablesLoaded;
return this;
}
public final void setTablesLoaded(Integer tablesLoaded) {
this.tablesLoaded = tablesLoaded;
}
public final Integer getTablesLoading() {
return tablesLoading;
}
@Override
public final Builder tablesLoading(Integer tablesLoading) {
this.tablesLoading = tablesLoading;
return this;
}
public final void setTablesLoading(Integer tablesLoading) {
this.tablesLoading = tablesLoading;
}
public final Integer getTablesQueued() {
return tablesQueued;
}
@Override
public final Builder tablesQueued(Integer tablesQueued) {
this.tablesQueued = tablesQueued;
return this;
}
public final void setTablesQueued(Integer tablesQueued) {
this.tablesQueued = tablesQueued;
}
public final Integer getTablesErrored() {
return tablesErrored;
}
@Override
public final Builder tablesErrored(Integer tablesErrored) {
this.tablesErrored = tablesErrored;
return this;
}
public final void setTablesErrored(Integer tablesErrored) {
this.tablesErrored = tablesErrored;
}
@Override
public ReplicationTaskStats build() {
return new ReplicationTaskStats(this);
}
}
}