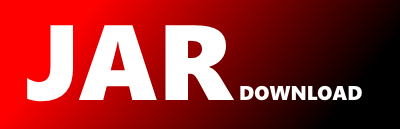
software.amazon.awssdk.services.dynamodb.model.ExpectedAttributeValue Maven / Gradle / Ivy
Show all versions of dynamodb Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dynamodb.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a condition to be compared with an attribute value. This condition can be used with
* DeleteItem
, PutItem
, or UpdateItem
operations; if the comparison evaluates to
* true, the operation succeeds; if not, the operation fails. You can use ExpectedAttributeValue
in one of
* two different ways:
*
*
* -
*
* Use AttributeValueList
to specify one or more values to compare against an attribute. Use
* ComparisonOperator
to specify how you want to perform the comparison. If the comparison evaluates to
* true, then the conditional operation succeeds.
*
*
* -
*
* Use Value
to specify a value that DynamoDB will compare against an attribute. If the values match, then
* ExpectedAttributeValue
evaluates to true and the conditional operation succeeds. Optionally, you can
* also set Exists
to false, indicating that you do not expect to find the attribute value in the
* table. In this case, the conditional operation succeeds only if the comparison evaluates to false.
*
*
*
*
* Value
and Exists
are incompatible with AttributeValueList
and
* ComparisonOperator
. Note that if you use both sets of parameters at once, DynamoDB will return a
* ValidationException
exception.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ExpectedAttributeValue implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField VALUE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(ExpectedAttributeValue::value)).setter(setter(Builder::value)).constructor(AttributeValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Value").build()).build();
private static final SdkField EXISTS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(ExpectedAttributeValue::exists)).setter(setter(Builder::exists))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Exists").build()).build();
private static final SdkField COMPARISON_OPERATOR_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ExpectedAttributeValue::comparisonOperatorAsString)).setter(setter(Builder::comparisonOperator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComparisonOperator").build())
.build();
private static final SdkField> ATTRIBUTE_VALUE_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ExpectedAttributeValue::attributeValueList))
.setter(setter(Builder::attributeValueList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AttributeValueList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AttributeValue::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VALUE_FIELD, EXISTS_FIELD,
COMPARISON_OPERATOR_FIELD, ATTRIBUTE_VALUE_LIST_FIELD));
private static final long serialVersionUID = 1L;
private final AttributeValue value;
private final Boolean exists;
private final String comparisonOperator;
private final List attributeValueList;
private ExpectedAttributeValue(BuilderImpl builder) {
this.value = builder.value;
this.exists = builder.exists;
this.comparisonOperator = builder.comparisonOperator;
this.attributeValueList = builder.attributeValueList;
}
/**
*
* Represents the data for the expected attribute.
*
*
* Each attribute value is described as a name-value pair. The name is the data type, and the value is the data
* itself.
*
*
* For more information, see Data Types in the Amazon DynamoDB Developer Guide.
*
*
* @return Represents the data for the expected attribute.
*
* Each attribute value is described as a name-value pair. The name is the data type, and the value is the
* data itself.
*
*
* For more information, see Data Types in the Amazon DynamoDB Developer Guide.
*/
public AttributeValue value() {
return value;
}
/**
*
* Causes DynamoDB to evaluate the value before attempting a conditional operation:
*
*
* -
*
* If Exists
is true
, DynamoDB will check to see if that attribute value already exists in
* the table. If it is found, then the operation succeeds. If it is not found, the operation fails with a
* ConditionCheckFailedException
.
*
*
* -
*
* If Exists
is false
, DynamoDB assumes that the attribute value does not exist in the
* table. If in fact the value does not exist, then the assumption is valid and the operation succeeds. If the value
* is found, despite the assumption that it does not exist, the operation fails with a
* ConditionCheckFailedException
.
*
*
*
*
* The default setting for Exists
is true
. If you supply a Value
all by
* itself, DynamoDB assumes the attribute exists: You don't have to set Exists
to true
,
* because it is implied.
*
*
* DynamoDB returns a ValidationException
if:
*
*
* -
*
* Exists
is true
but there is no Value
to check. (You expect a value to
* exist, but don't specify what that value is.)
*
*
* -
*
* Exists
is false
but you also provide a Value
. (You cannot expect an
* attribute to have a value, while also expecting it not to exist.)
*
*
*
*
* @return Causes DynamoDB to evaluate the value before attempting a conditional operation:
*
* -
*
* If Exists
is true
, DynamoDB will check to see if that attribute value already
* exists in the table. If it is found, then the operation succeeds. If it is not found, the operation fails
* with a ConditionCheckFailedException
.
*
*
* -
*
* If Exists
is false
, DynamoDB assumes that the attribute value does not exist in
* the table. If in fact the value does not exist, then the assumption is valid and the operation succeeds.
* If the value is found, despite the assumption that it does not exist, the operation fails with a
* ConditionCheckFailedException
.
*
*
*
*
* The default setting for Exists
is true
. If you supply a Value
all
* by itself, DynamoDB assumes the attribute exists: You don't have to set Exists
to
* true
, because it is implied.
*
*
* DynamoDB returns a ValidationException
if:
*
*
* -
*
* Exists
is true
but there is no Value
to check. (You expect a value
* to exist, but don't specify what that value is.)
*
*
* -
*
* Exists
is false
but you also provide a Value
. (You cannot expect
* an attribute to have a value, while also expecting it not to exist.)
*
*
*/
public Boolean exists() {
return exists;
}
/**
*
* A comparator for evaluating attributes in the AttributeValueList
. For example, equals, greater than,
* less than, etc.
*
*
* The following comparison operators are available:
*
*
* EQ | NE | LE | LT | GE | GT | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN | BETWEEN
*
*
* The following are descriptions of each comparison operator.
*
*
* -
*
* EQ
: Equal. EQ
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NE
: Not equal. NE
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number, Binary,
* String Set, Number Set, or Binary Set. If an item contains an AttributeValue
of a different type
* than the one provided in the request, the value does not match. For example, {"S":"6"}
does not
* equal {"N":"6"}
. Also, {"N":"6"}
does not equal {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LE
: Less than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If an item contains an AttributeValue
element of a different type than
* the one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LT
: Less than.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number, or
* Binary (not a set type). If an item contains an AttributeValue
element of a different type than the
* one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GE
: Greater than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If an item contains an AttributeValue
element of a different type than
* the one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GT
: Greater than.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If an item contains an AttributeValue
element of a different type than
* the one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NOT_NULL
: The attribute exists. NOT_NULL
is supported for all data types, including
* lists and maps.
*
*
*
* This operator tests for the existence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NOT_NULL
, the result is a Boolean
* true
. This result is because the attribute "a
" exists; its data type is not relevant to
* the NOT_NULL
comparison operator.
*
*
* -
*
* NULL
: The attribute does not exist. NULL
is supported for all data types, including
* lists and maps.
*
*
*
* This operator tests for the nonexistence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NULL
, the result is a Boolean false
.
* This is because the attribute "a
" exists; its data type is not relevant to the NULL
* comparison operator.
*
*
* -
*
* CONTAINS
: Checks for a subsequence, or value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If the target attribute of the comparison is of type String, then the operator checks
* for a substring match. If the target attribute of the comparison is of type Binary, then the operator looks for a
* subsequence of the target that matches the input. If the target attribute of the comparison is a set ("
* SS
", "NS
", or "BS
"), then the operator evaluates to true if it finds an
* exact match with any member of the set.
*
*
* CONTAINS is supported for lists: When evaluating "a CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* NOT_CONTAINS
: Checks for absence of a subsequence, or absence of a value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If the target attribute of the comparison is a String, then the operator checks for
* the absence of a substring match. If the target attribute of the comparison is Binary, then the operator checks
* for the absence of a subsequence of the target that matches the input. If the target attribute of the comparison
* is a set ("SS
", "NS
", or "BS
"), then the operator evaluates to true if it
* does not find an exact match with any member of the set.
*
*
* NOT_CONTAINS is supported for lists: When evaluating "a NOT CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* BEGINS_WITH
: Checks for a prefix.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String or Binary (not a
* Number or a set type). The target attribute of the comparison must be of type String or Binary (not a Number or a
* set type).
*
*
* -
*
* IN
: Checks for matching elements in a list.
*
*
* AttributeValueList
can contain one or more AttributeValue
elements of type String,
* Number, or Binary. These attributes are compared against an existing attribute of an item. If any elements of the
* input are equal to the item attribute, the expression evaluates to true.
*
*
* -
*
* BETWEEN
: Greater than or equal to the first value, and less than or equal to the second value.
*
*
* AttributeValueList
must contain two AttributeValue
elements of the same type, either
* String, Number, or Binary (not a set type). A target attribute matches if the target value is greater than, or
* equal to, the first element and less than, or equal to, the second element. If an item contains an
* AttributeValue
element of a different type than the one provided in the request, the value does not
* match. For example, {"S":"6"}
does not compare to {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #comparisonOperator} will return {@link ComparisonOperator#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #comparisonOperatorAsString}.
*
*
* @return A comparator for evaluating attributes in the AttributeValueList
. For example, equals,
* greater than, less than, etc.
*
* The following comparison operators are available:
*
*
* EQ | NE | LE | LT | GE | GT | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN | BETWEEN
*
*
* The following are descriptions of each comparison operator.
*
*
* -
*
* EQ
: Equal. EQ
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
* element of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NE
: Not equal. NE
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number,
* Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LE
: Less than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LT
: Less than.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number,
* or Binary (not a set type). If an item contains an AttributeValue
element of a different
* type than the one provided in the request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GE
: Greater than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GT
: Greater than.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NOT_NULL
: The attribute exists. NOT_NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the existence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NOT_NULL
, the result is a Boolean
* true
. This result is because the attribute "a
" exists; its data type is not
* relevant to the NOT_NULL
comparison operator.
*
*
* -
*
* NULL
: The attribute does not exist. NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the nonexistence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using NULL
, the result is a Boolean
* false
. This is because the attribute "a
" exists; its data type is not relevant
* to the NULL
comparison operator.
*
*
* -
*
* CONTAINS
: Checks for a subsequence, or value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If the target attribute of the comparison is of type String, then the
* operator checks for a substring match. If the target attribute of the comparison is of type Binary, then
* the operator looks for a subsequence of the target that matches the input. If the target attribute of the
* comparison is a set ("SS
", "NS
", or "BS
"), then the operator
* evaluates to true if it finds an exact match with any member of the set.
*
*
* CONTAINS is supported for lists: When evaluating "a CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* NOT_CONTAINS
: Checks for absence of a subsequence, or absence of a value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If the target attribute of the comparison is a String, then the
* operator checks for the absence of a substring match. If the target attribute of the comparison is
* Binary, then the operator checks for the absence of a subsequence of the target that matches the input.
* If the target attribute of the comparison is a set ("SS
", "NS
", or "
* BS
"), then the operator evaluates to true if it does not find an exact match with any
* member of the set.
*
*
* NOT_CONTAINS is supported for lists: When evaluating "a NOT CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* BEGINS_WITH
: Checks for a prefix.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String or Binary
* (not a Number or a set type). The target attribute of the comparison must be of type String or Binary
* (not a Number or a set type).
*
*
* -
*
* IN
: Checks for matching elements in a list.
*
*
* AttributeValueList
can contain one or more AttributeValue
elements of type
* String, Number, or Binary. These attributes are compared against an existing attribute of an item. If any
* elements of the input are equal to the item attribute, the expression evaluates to true.
*
*
* -
*
* BETWEEN
: Greater than or equal to the first value, and less than or equal to the second
* value.
*
*
* AttributeValueList
must contain two AttributeValue
elements of the same type,
* either String, Number, or Binary (not a set type). A target attribute matches if the target value is
* greater than, or equal to, the first element and less than, or equal to, the second element. If an item
* contains an AttributeValue
element of a different type than the one provided in the request,
* the value does not match. For example, {"S":"6"}
does not compare to {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
*
*
* @see ComparisonOperator
*/
public ComparisonOperator comparisonOperator() {
return ComparisonOperator.fromValue(comparisonOperator);
}
/**
*
* A comparator for evaluating attributes in the AttributeValueList
. For example, equals, greater than,
* less than, etc.
*
*
* The following comparison operators are available:
*
*
* EQ | NE | LE | LT | GE | GT | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN | BETWEEN
*
*
* The following are descriptions of each comparison operator.
*
*
* -
*
* EQ
: Equal. EQ
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NE
: Not equal. NE
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number, Binary,
* String Set, Number Set, or Binary Set. If an item contains an AttributeValue
of a different type
* than the one provided in the request, the value does not match. For example, {"S":"6"}
does not
* equal {"N":"6"}
. Also, {"N":"6"}
does not equal {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LE
: Less than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If an item contains an AttributeValue
element of a different type than
* the one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LT
: Less than.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number, or
* Binary (not a set type). If an item contains an AttributeValue
element of a different type than the
* one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GE
: Greater than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If an item contains an AttributeValue
element of a different type than
* the one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GT
: Greater than.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If an item contains an AttributeValue
element of a different type than
* the one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NOT_NULL
: The attribute exists. NOT_NULL
is supported for all data types, including
* lists and maps.
*
*
*
* This operator tests for the existence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NOT_NULL
, the result is a Boolean
* true
. This result is because the attribute "a
" exists; its data type is not relevant to
* the NOT_NULL
comparison operator.
*
*
* -
*
* NULL
: The attribute does not exist. NULL
is supported for all data types, including
* lists and maps.
*
*
*
* This operator tests for the nonexistence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NULL
, the result is a Boolean false
.
* This is because the attribute "a
" exists; its data type is not relevant to the NULL
* comparison operator.
*
*
* -
*
* CONTAINS
: Checks for a subsequence, or value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If the target attribute of the comparison is of type String, then the operator checks
* for a substring match. If the target attribute of the comparison is of type Binary, then the operator looks for a
* subsequence of the target that matches the input. If the target attribute of the comparison is a set ("
* SS
", "NS
", or "BS
"), then the operator evaluates to true if it finds an
* exact match with any member of the set.
*
*
* CONTAINS is supported for lists: When evaluating "a CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* NOT_CONTAINS
: Checks for absence of a subsequence, or absence of a value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String, Number,
* or Binary (not a set type). If the target attribute of the comparison is a String, then the operator checks for
* the absence of a substring match. If the target attribute of the comparison is Binary, then the operator checks
* for the absence of a subsequence of the target that matches the input. If the target attribute of the comparison
* is a set ("SS
", "NS
", or "BS
"), then the operator evaluates to true if it
* does not find an exact match with any member of the set.
*
*
* NOT_CONTAINS is supported for lists: When evaluating "a NOT CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* BEGINS_WITH
: Checks for a prefix.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String or Binary (not a
* Number or a set type). The target attribute of the comparison must be of type String or Binary (not a Number or a
* set type).
*
*
* -
*
* IN
: Checks for matching elements in a list.
*
*
* AttributeValueList
can contain one or more AttributeValue
elements of type String,
* Number, or Binary. These attributes are compared against an existing attribute of an item. If any elements of the
* input are equal to the item attribute, the expression evaluates to true.
*
*
* -
*
* BETWEEN
: Greater than or equal to the first value, and less than or equal to the second value.
*
*
* AttributeValueList
must contain two AttributeValue
elements of the same type, either
* String, Number, or Binary (not a set type). A target attribute matches if the target value is greater than, or
* equal to, the first element and less than, or equal to, the second element. If an item contains an
* AttributeValue
element of a different type than the one provided in the request, the value does not
* match. For example, {"S":"6"}
does not compare to {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #comparisonOperator} will return {@link ComparisonOperator#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #comparisonOperatorAsString}.
*
*
* @return A comparator for evaluating attributes in the AttributeValueList
. For example, equals,
* greater than, less than, etc.
*
* The following comparison operators are available:
*
*
* EQ | NE | LE | LT | GE | GT | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN | BETWEEN
*
*
* The following are descriptions of each comparison operator.
*
*
* -
*
* EQ
: Equal. EQ
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
* element of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NE
: Not equal. NE
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number,
* Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LE
: Less than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LT
: Less than.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number,
* or Binary (not a set type). If an item contains an AttributeValue
element of a different
* type than the one provided in the request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GE
: Greater than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GT
: Greater than.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NOT_NULL
: The attribute exists. NOT_NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the existence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NOT_NULL
, the result is a Boolean
* true
. This result is because the attribute "a
" exists; its data type is not
* relevant to the NOT_NULL
comparison operator.
*
*
* -
*
* NULL
: The attribute does not exist. NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the nonexistence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using NULL
, the result is a Boolean
* false
. This is because the attribute "a
" exists; its data type is not relevant
* to the NULL
comparison operator.
*
*
* -
*
* CONTAINS
: Checks for a subsequence, or value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If the target attribute of the comparison is of type String, then the
* operator checks for a substring match. If the target attribute of the comparison is of type Binary, then
* the operator looks for a subsequence of the target that matches the input. If the target attribute of the
* comparison is a set ("SS
", "NS
", or "BS
"), then the operator
* evaluates to true if it finds an exact match with any member of the set.
*
*
* CONTAINS is supported for lists: When evaluating "a CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* NOT_CONTAINS
: Checks for absence of a subsequence, or absence of a value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If the target attribute of the comparison is a String, then the
* operator checks for the absence of a substring match. If the target attribute of the comparison is
* Binary, then the operator checks for the absence of a subsequence of the target that matches the input.
* If the target attribute of the comparison is a set ("SS
", "NS
", or "
* BS
"), then the operator evaluates to true if it does not find an exact match with any
* member of the set.
*
*
* NOT_CONTAINS is supported for lists: When evaluating "a NOT CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* BEGINS_WITH
: Checks for a prefix.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String or Binary
* (not a Number or a set type). The target attribute of the comparison must be of type String or Binary
* (not a Number or a set type).
*
*
* -
*
* IN
: Checks for matching elements in a list.
*
*
* AttributeValueList
can contain one or more AttributeValue
elements of type
* String, Number, or Binary. These attributes are compared against an existing attribute of an item. If any
* elements of the input are equal to the item attribute, the expression evaluates to true.
*
*
* -
*
* BETWEEN
: Greater than or equal to the first value, and less than or equal to the second
* value.
*
*
* AttributeValueList
must contain two AttributeValue
elements of the same type,
* either String, Number, or Binary (not a set type). A target attribute matches if the target value is
* greater than, or equal to, the first element and less than, or equal to, the second element. If an item
* contains an AttributeValue
element of a different type than the one provided in the request,
* the value does not match. For example, {"S":"6"}
does not compare to {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
*
*
* @see ComparisonOperator
*/
public String comparisonOperatorAsString() {
return comparisonOperator;
}
/**
* Returns true if the AttributeValueList property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasAttributeValueList() {
return attributeValueList != null && !(attributeValueList instanceof SdkAutoConstructList);
}
/**
*
* One or more values to evaluate against the supplied attribute. The number of values in the list depends on the
* ComparisonOperator
being used.
*
*
* For type Number, value comparisons are numeric.
*
*
* String value comparisons for greater than, equals, or less than are based on ASCII character code values. For
* example, a
is greater than A
, and a
is greater than B
. For a
* list of code values, see http://en.wikipedia
* .org/wiki/ASCII#ASCII_printable_characters.
*
*
* For Binary, DynamoDB treats each byte of the binary data as unsigned when it compares binary values.
*
*
* For information on specifying data types in JSON, see JSON Data Format in
* the Amazon DynamoDB Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAttributeValueList()} to see if a value was sent in this field.
*
*
* @return One or more values to evaluate against the supplied attribute. The number of values in the list depends
* on the ComparisonOperator
being used.
*
* For type Number, value comparisons are numeric.
*
*
* String value comparisons for greater than, equals, or less than are based on ASCII character code values.
* For example, a
is greater than A
, and a
is greater than
* B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
*
* For Binary, DynamoDB treats each byte of the binary data as unsigned when it compares binary values.
*
*
* For information on specifying data types in JSON, see JSON Data
* Format in the Amazon DynamoDB Developer Guide.
*/
public List attributeValueList() {
return attributeValueList;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(value());
hashCode = 31 * hashCode + Objects.hashCode(exists());
hashCode = 31 * hashCode + Objects.hashCode(comparisonOperatorAsString());
hashCode = 31 * hashCode + Objects.hashCode(attributeValueList());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ExpectedAttributeValue)) {
return false;
}
ExpectedAttributeValue other = (ExpectedAttributeValue) obj;
return Objects.equals(value(), other.value()) && Objects.equals(exists(), other.exists())
&& Objects.equals(comparisonOperatorAsString(), other.comparisonOperatorAsString())
&& Objects.equals(attributeValueList(), other.attributeValueList());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ExpectedAttributeValue").add("Value", value()).add("Exists", exists())
.add("ComparisonOperator", comparisonOperatorAsString()).add("AttributeValueList", attributeValueList()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Value":
return Optional.ofNullable(clazz.cast(value()));
case "Exists":
return Optional.ofNullable(clazz.cast(exists()));
case "ComparisonOperator":
return Optional.ofNullable(clazz.cast(comparisonOperatorAsString()));
case "AttributeValueList":
return Optional.ofNullable(clazz.cast(attributeValueList()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Each attribute value is described as a name-value pair. The name is the data type, and the value is
* the data itself.
*
*
* For more information, see Data Types in the Amazon DynamoDB Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder value(AttributeValue value);
/**
*
* Represents the data for the expected attribute.
*
*
* Each attribute value is described as a name-value pair. The name is the data type, and the value is the data
* itself.
*
*
* For more information, see Data Types in the Amazon DynamoDB Developer Guide.
*
* This is a convenience that creates an instance of the {@link AttributeValue.Builder} avoiding the need to
* create one manually via {@link AttributeValue#builder()}.
*
* When the {@link Consumer} completes, {@link AttributeValue.Builder#build()} is called immediately and its
* result is passed to {@link #value(AttributeValue)}.
*
* @param value
* a consumer that will call methods on {@link AttributeValue.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #value(AttributeValue)
*/
default Builder value(Consumer value) {
return value(AttributeValue.builder().applyMutation(value).build());
}
/**
*
* Causes DynamoDB to evaluate the value before attempting a conditional operation:
*
*
* -
*
* If Exists
is true
, DynamoDB will check to see if that attribute value already
* exists in the table. If it is found, then the operation succeeds. If it is not found, the operation fails
* with a ConditionCheckFailedException
.
*
*
* -
*
* If Exists
is false
, DynamoDB assumes that the attribute value does not exist in the
* table. If in fact the value does not exist, then the assumption is valid and the operation succeeds. If the
* value is found, despite the assumption that it does not exist, the operation fails with a
* ConditionCheckFailedException
.
*
*
*
*
* The default setting for Exists
is true
. If you supply a Value
all by
* itself, DynamoDB assumes the attribute exists: You don't have to set Exists
to true
* , because it is implied.
*
*
* DynamoDB returns a ValidationException
if:
*
*
* -
*
* Exists
is true
but there is no Value
to check. (You expect a value to
* exist, but don't specify what that value is.)
*
*
* -
*
* Exists
is false
but you also provide a Value
. (You cannot expect an
* attribute to have a value, while also expecting it not to exist.)
*
*
*
*
* @param exists
* Causes DynamoDB to evaluate the value before attempting a conditional operation:
*
* -
*
* If Exists
is true
, DynamoDB will check to see if that attribute value
* already exists in the table. If it is found, then the operation succeeds. If it is not found, the
* operation fails with a ConditionCheckFailedException
.
*
*
* -
*
* If Exists
is false
, DynamoDB assumes that the attribute value does not exist
* in the table. If in fact the value does not exist, then the assumption is valid and the operation
* succeeds. If the value is found, despite the assumption that it does not exist, the operation fails
* with a ConditionCheckFailedException
.
*
*
*
*
* The default setting for Exists
is true
. If you supply a Value
* all by itself, DynamoDB assumes the attribute exists: You don't have to set Exists
to
* true
, because it is implied.
*
*
* DynamoDB returns a ValidationException
if:
*
*
* -
*
* Exists
is true
but there is no Value
to check. (You expect a
* value to exist, but don't specify what that value is.)
*
*
* -
*
* Exists
is false
but you also provide a Value
. (You cannot
* expect an attribute to have a value, while also expecting it not to exist.)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder exists(Boolean exists);
/**
*
* A comparator for evaluating attributes in the AttributeValueList
. For example, equals, greater
* than, less than, etc.
*
*
* The following comparison operators are available:
*
*
* EQ | NE | LE | LT | GE | GT | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN | BETWEEN
*
*
* The following are descriptions of each comparison operator.
*
*
* -
*
* EQ
: Equal. EQ
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
* element of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NE
: Not equal. NE
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number,
* Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LE
: Less than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a different
* type than the one provided in the request, the value does not match. For example, {"S":"6"}
does
* not equal {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LT
: Less than.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number, or
* Binary (not a set type). If an item contains an AttributeValue
element of a different type than
* the one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GE
: Greater than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a different
* type than the one provided in the request, the value does not match. For example, {"S":"6"}
does
* not equal {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GT
: Greater than.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a different
* type than the one provided in the request, the value does not match. For example, {"S":"6"}
does
* not equal {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NOT_NULL
: The attribute exists. NOT_NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the existence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NOT_NULL
, the result is a Boolean
* true
. This result is because the attribute "a
" exists; its data type is not
* relevant to the NOT_NULL
comparison operator.
*
*
* -
*
* NULL
: The attribute does not exist. NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the nonexistence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NULL
, the result is a Boolean
* false
. This is because the attribute "a
" exists; its data type is not relevant to
* the NULL
comparison operator.
*
*
* -
*
* CONTAINS
: Checks for a subsequence, or value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If the target attribute of the comparison is of type String, then the
* operator checks for a substring match. If the target attribute of the comparison is of type Binary, then the
* operator looks for a subsequence of the target that matches the input. If the target attribute of the
* comparison is a set ("SS
", "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
*
* CONTAINS is supported for lists: When evaluating "a CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* NOT_CONTAINS
: Checks for absence of a subsequence, or absence of a value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If the target attribute of the comparison is a String, then the operator
* checks for the absence of a substring match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match with any member of the set.
*
*
* NOT_CONTAINS is supported for lists: When evaluating "a NOT CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* BEGINS_WITH
: Checks for a prefix.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String or Binary
* (not a Number or a set type). The target attribute of the comparison must be of type String or Binary (not a
* Number or a set type).
*
*
* -
*
* IN
: Checks for matching elements in a list.
*
*
* AttributeValueList
can contain one or more AttributeValue
elements of type String,
* Number, or Binary. These attributes are compared against an existing attribute of an item. If any elements of
* the input are equal to the item attribute, the expression evaluates to true.
*
*
* -
*
* BETWEEN
: Greater than or equal to the first value, and less than or equal to the second value.
*
*
* AttributeValueList
must contain two AttributeValue
elements of the same type,
* either String, Number, or Binary (not a set type). A target attribute matches if the target value is greater
* than, or equal to, the first element and less than, or equal to, the second element. If an item contains an
* AttributeValue
element of a different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
*
*
*
*
* @param comparisonOperator
* A comparator for evaluating attributes in the AttributeValueList
. For example, equals,
* greater than, less than, etc.
*
* The following comparison operators are available:
*
*
* EQ | NE | LE | LT | GE | GT | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN | BETWEEN
*
*
* The following are descriptions of each comparison operator.
*
*
* -
*
* EQ
: Equal. EQ
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, Binary, String Set, Number Set, or Binary Set. If an item contains an
* AttributeValue
element of a different type than the one provided in the request, the
* value does not match. For example, {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NE
: Not equal. NE
is supported for all data types, including lists and
* maps.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String,
* Number, Binary, String Set, Number Set, or Binary Set. If an item contains an
* AttributeValue
of a different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LE
: Less than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If an item contains an AttributeValue
element
* of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LT
: Less than.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GE
: Greater than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If an item contains an AttributeValue
element
* of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GT
: Greater than.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If an item contains an AttributeValue
element
* of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NOT_NULL
: The attribute exists. NOT_NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using NOT_NULL
, the result is a
* Boolean true
. This result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
*
* -
*
* NULL
: The attribute does not exist. NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the nonexistence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using NULL
, the result is a
* Boolean false
. This is because the attribute "a
" exists; its data type is
* not relevant to the NULL
comparison operator.
*
*
* -
*
* CONTAINS
: Checks for a subsequence, or value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target attribute of the comparison is
* of type Binary, then the operator looks for a subsequence of the target that matches the input. If the
* target attribute of the comparison is a set ("SS
", "NS
", or "BS
* "), then the operator evaluates to true if it finds an exact match with any member of the set.
*
*
* CONTAINS is supported for lists: When evaluating "a CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* NOT_CONTAINS
: Checks for absence of a subsequence, or absence of a value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If the target attribute of the comparison is a String,
* then the operator checks for the absence of a substring match. If the target attribute of the
* comparison is Binary, then the operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set ("SS
", "
* NS
", or "BS
"), then the operator evaluates to true if it does not
* find an exact match with any member of the set.
*
*
* NOT_CONTAINS is supported for lists: When evaluating "a NOT CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* BEGINS_WITH
: Checks for a prefix.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String or
* Binary (not a Number or a set type). The target attribute of the comparison must be of type String or
* Binary (not a Number or a set type).
*
*
* -
*
* IN
: Checks for matching elements in a list.
*
*
* AttributeValueList
can contain one or more AttributeValue
elements of type
* String, Number, or Binary. These attributes are compared against an existing attribute of an item. If
* any elements of the input are equal to the item attribute, the expression evaluates to true.
*
*
* -
*
* BETWEEN
: Greater than or equal to the first value, and less than or equal to the second
* value.
*
*
* AttributeValueList
must contain two AttributeValue
elements of the same
* type, either String, Number, or Binary (not a set type). A target attribute matches if the target
* value is greater than, or equal to, the first element and less than, or equal to, the second element.
* If an item contains an AttributeValue
element of a different type than the one provided
* in the request, the value does not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
*
*
* @see ComparisonOperator
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComparisonOperator
*/
Builder comparisonOperator(String comparisonOperator);
/**
*
* A comparator for evaluating attributes in the AttributeValueList
. For example, equals, greater
* than, less than, etc.
*
*
* The following comparison operators are available:
*
*
* EQ | NE | LE | LT | GE | GT | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN | BETWEEN
*
*
* The following are descriptions of each comparison operator.
*
*
* -
*
* EQ
: Equal. EQ
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
* element of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NE
: Not equal. NE
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number,
* Binary, String Set, Number Set, or Binary Set. If an item contains an AttributeValue
of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LE
: Less than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a different
* type than the one provided in the request, the value does not match. For example, {"S":"6"}
does
* not equal {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LT
: Less than.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String, Number, or
* Binary (not a set type). If an item contains an AttributeValue
element of a different type than
* the one provided in the request, the value does not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GE
: Greater than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a different
* type than the one provided in the request, the value does not match. For example, {"S":"6"}
does
* not equal {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GT
: Greater than.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a different
* type than the one provided in the request, the value does not match. For example, {"S":"6"}
does
* not equal {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NOT_NULL
: The attribute exists. NOT_NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the existence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NOT_NULL
, the result is a Boolean
* true
. This result is because the attribute "a
" exists; its data type is not
* relevant to the NOT_NULL
comparison operator.
*
*
* -
*
* NULL
: The attribute does not exist. NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the nonexistence of an attribute, not its data type. If the data type of attribute "
* a
" is null, and you evaluate it using NULL
, the result is a Boolean
* false
. This is because the attribute "a
" exists; its data type is not relevant to
* the NULL
comparison operator.
*
*
* -
*
* CONTAINS
: Checks for a subsequence, or value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If the target attribute of the comparison is of type String, then the
* operator checks for a substring match. If the target attribute of the comparison is of type Binary, then the
* operator looks for a subsequence of the target that matches the input. If the target attribute of the
* comparison is a set ("SS
", "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
*
* CONTAINS is supported for lists: When evaluating "a CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* NOT_CONTAINS
: Checks for absence of a subsequence, or absence of a value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type String,
* Number, or Binary (not a set type). If the target attribute of the comparison is a String, then the operator
* checks for the absence of a substring match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match with any member of the set.
*
*
* NOT_CONTAINS is supported for lists: When evaluating "a NOT CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* BEGINS_WITH
: Checks for a prefix.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String or Binary
* (not a Number or a set type). The target attribute of the comparison must be of type String or Binary (not a
* Number or a set type).
*
*
* -
*
* IN
: Checks for matching elements in a list.
*
*
* AttributeValueList
can contain one or more AttributeValue
elements of type String,
* Number, or Binary. These attributes are compared against an existing attribute of an item. If any elements of
* the input are equal to the item attribute, the expression evaluates to true.
*
*
* -
*
* BETWEEN
: Greater than or equal to the first value, and less than or equal to the second value.
*
*
* AttributeValueList
must contain two AttributeValue
elements of the same type,
* either String, Number, or Binary (not a set type). A target attribute matches if the target value is greater
* than, or equal to, the first element and less than, or equal to, the second element. If an item contains an
* AttributeValue
element of a different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2", "1"]}
*
*
*
*
* @param comparisonOperator
* A comparator for evaluating attributes in the AttributeValueList
. For example, equals,
* greater than, less than, etc.
*
* The following comparison operators are available:
*
*
* EQ | NE | LE | LT | GE | GT | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN | BETWEEN
*
*
* The following are descriptions of each comparison operator.
*
*
* -
*
* EQ
: Equal. EQ
is supported for all data types, including lists and maps.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, Binary, String Set, Number Set, or Binary Set. If an item contains an
* AttributeValue
element of a different type than the one provided in the request, the
* value does not match. For example, {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NE
: Not equal. NE
is supported for all data types, including lists and
* maps.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String,
* Number, Binary, String Set, Number Set, or Binary Set. If an item contains an
* AttributeValue
of a different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LE
: Less than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If an item contains an AttributeValue
element
* of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* LT
: Less than.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String,
* Number, or Binary (not a set type). If an item contains an AttributeValue
element of a
* different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GE
: Greater than or equal.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If an item contains an AttributeValue
element
* of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* GT
: Greater than.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If an item contains an AttributeValue
element
* of a different type than the one provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also, {"N":"6"}
does not
* compare to {"NS":["6", "2", "1"]}
.
*
*
* -
*
* NOT_NULL
: The attribute exists. NOT_NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using NOT_NULL
, the result is a
* Boolean true
. This result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
*
* -
*
* NULL
: The attribute does not exist. NULL
is supported for all data types,
* including lists and maps.
*
*
*
* This operator tests for the nonexistence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using NULL
, the result is a
* Boolean false
. This is because the attribute "a
" exists; its data type is
* not relevant to the NULL
comparison operator.
*
*
* -
*
* CONTAINS
: Checks for a subsequence, or value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target attribute of the comparison is
* of type Binary, then the operator looks for a subsequence of the target that matches the input. If the
* target attribute of the comparison is a set ("SS
", "NS
", or "BS
* "), then the operator evaluates to true if it finds an exact match with any member of the set.
*
*
* CONTAINS is supported for lists: When evaluating "a CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* NOT_CONTAINS
: Checks for absence of a subsequence, or absence of a value in a set.
*
*
* AttributeValueList
can contain only one AttributeValue
element of type
* String, Number, or Binary (not a set type). If the target attribute of the comparison is a String,
* then the operator checks for the absence of a substring match. If the target attribute of the
* comparison is Binary, then the operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set ("SS
", "
* NS
", or "BS
"), then the operator evaluates to true if it does not
* find an exact match with any member of the set.
*
*
* NOT_CONTAINS is supported for lists: When evaluating "a NOT CONTAINS b
", "a
* " can be a list; however, "b
" cannot be a set, a map, or a list.
*
*
* -
*
* BEGINS_WITH
: Checks for a prefix.
*
*
* AttributeValueList
can contain only one AttributeValue
of type String or
* Binary (not a Number or a set type). The target attribute of the comparison must be of type String or
* Binary (not a Number or a set type).
*
*
* -
*
* IN
: Checks for matching elements in a list.
*
*
* AttributeValueList
can contain one or more AttributeValue
elements of type
* String, Number, or Binary. These attributes are compared against an existing attribute of an item. If
* any elements of the input are equal to the item attribute, the expression evaluates to true.
*
*
* -
*
* BETWEEN
: Greater than or equal to the first value, and less than or equal to the second
* value.
*
*
* AttributeValueList
must contain two AttributeValue
elements of the same
* type, either String, Number, or Binary (not a set type). A target attribute matches if the target
* value is greater than, or equal to, the first element and less than, or equal to, the second element.
* If an item contains an AttributeValue
element of a different type than the one provided
* in the request, the value does not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare to
* {"NS":["6", "2", "1"]}
*
*
* @see ComparisonOperator
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComparisonOperator
*/
Builder comparisonOperator(ComparisonOperator comparisonOperator);
/**
*
* One or more values to evaluate against the supplied attribute. The number of values in the list depends on
* the ComparisonOperator
being used.
*
*
* For type Number, value comparisons are numeric.
*
*
* String value comparisons for greater than, equals, or less than are based on ASCII character code values. For
* example, a
is greater than A
, and a
is greater than B
.
* For a list of code values, see http://
* en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
*
* For Binary, DynamoDB treats each byte of the binary data as unsigned when it compares binary values.
*
*
* For information on specifying data types in JSON, see JSON Data Format
* in the Amazon DynamoDB Developer Guide.
*
*
* @param attributeValueList
* One or more values to evaluate against the supplied attribute. The number of values in the list
* depends on the ComparisonOperator
being used.
*
* For type Number, value comparisons are numeric.
*
*
* String value comparisons for greater than, equals, or less than are based on ASCII character code
* values. For example, a
is greater than A
, and a
is greater than
* B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
*
* For Binary, DynamoDB treats each byte of the binary data as unsigned when it compares binary values.
*
*
* For information on specifying data types in JSON, see JSON Data
* Format in the Amazon DynamoDB Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder attributeValueList(Collection attributeValueList);
/**
*
* One or more values to evaluate against the supplied attribute. The number of values in the list depends on
* the ComparisonOperator
being used.
*
*
* For type Number, value comparisons are numeric.
*
*
* String value comparisons for greater than, equals, or less than are based on ASCII character code values. For
* example, a
is greater than A
, and a
is greater than B
.
* For a list of code values, see http://
* en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
*
* For Binary, DynamoDB treats each byte of the binary data as unsigned when it compares binary values.
*
*
* For information on specifying data types in JSON, see JSON Data Format
* in the Amazon DynamoDB Developer Guide.
*
*
* @param attributeValueList
* One or more values to evaluate against the supplied attribute. The number of values in the list
* depends on the ComparisonOperator
being used.
*
* For type Number, value comparisons are numeric.
*
*
* String value comparisons for greater than, equals, or less than are based on ASCII character code
* values. For example, a
is greater than A
, and a
is greater than
* B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
*
* For Binary, DynamoDB treats each byte of the binary data as unsigned when it compares binary values.
*
*
* For information on specifying data types in JSON, see JSON Data
* Format in the Amazon DynamoDB Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder attributeValueList(AttributeValue... attributeValueList);
/**
*
* One or more values to evaluate against the supplied attribute. The number of values in the list depends on
* the ComparisonOperator
being used.
*
*
* For type Number, value comparisons are numeric.
*
*
* String value comparisons for greater than, equals, or less than are based on ASCII character code values. For
* example, a
is greater than A
, and a
is greater than B
.
* For a list of code values, see http://
* en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
*
* For Binary, DynamoDB treats each byte of the binary data as unsigned when it compares binary values.
*
*
* For information on specifying data types in JSON, see JSON Data Format
* in the Amazon DynamoDB Developer Guide.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need
* to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and
* its result is passed to {@link #attributeValueList(List)}.
*
* @param attributeValueList
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #attributeValueList(List)
*/
Builder attributeValueList(Consumer... attributeValueList);
}
static final class BuilderImpl implements Builder {
private AttributeValue value;
private Boolean exists;
private String comparisonOperator;
private List attributeValueList = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(ExpectedAttributeValue model) {
value(model.value);
exists(model.exists);
comparisonOperator(model.comparisonOperator);
attributeValueList(model.attributeValueList);
}
public final AttributeValue.Builder getValue() {
return value != null ? value.toBuilder() : null;
}
@Override
public final Builder value(AttributeValue value) {
this.value = value;
return this;
}
public final void setValue(AttributeValue.BuilderImpl value) {
this.value = value != null ? value.build() : null;
}
public final Boolean getExists() {
return exists;
}
@Override
public final Builder exists(Boolean exists) {
this.exists = exists;
return this;
}
public final void setExists(Boolean exists) {
this.exists = exists;
}
public final String getComparisonOperator() {
return comparisonOperator;
}
@Override
public final Builder comparisonOperator(String comparisonOperator) {
this.comparisonOperator = comparisonOperator;
return this;
}
@Override
public final Builder comparisonOperator(ComparisonOperator comparisonOperator) {
this.comparisonOperator(comparisonOperator == null ? null : comparisonOperator.toString());
return this;
}
public final void setComparisonOperator(String comparisonOperator) {
this.comparisonOperator = comparisonOperator;
}
public final Collection getAttributeValueList() {
return attributeValueList != null ? attributeValueList.stream().map(AttributeValue::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder attributeValueList(Collection attributeValueList) {
this.attributeValueList = AttributeValueListCopier.copy(attributeValueList);
return this;
}
@Override
@SafeVarargs
public final Builder attributeValueList(AttributeValue... attributeValueList) {
attributeValueList(Arrays.asList(attributeValueList));
return this;
}
@Override
@SafeVarargs
public final Builder attributeValueList(Consumer... attributeValueList) {
attributeValueList(Stream.of(attributeValueList).map(c -> AttributeValue.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setAttributeValueList(Collection attributeValueList) {
this.attributeValueList = AttributeValueListCopier.copyFromBuilder(attributeValueList);
}
@Override
public ExpectedAttributeValue build() {
return new ExpectedAttributeValue(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}