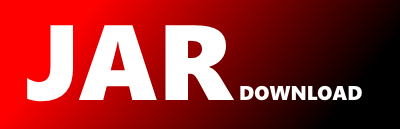
software.amazon.awssdk.services.dynamodb.model.DescribeLimitsResponse Maven / Gradle / Ivy
Show all versions of dynamodb Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dynamodb.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the output of a DescribeLimits
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeLimitsResponse extends DynamoDbResponse implements
ToCopyableBuilder {
private static final SdkField ACCOUNT_MAX_READ_CAPACITY_UNITS_FIELD = SdkField
. builder(MarshallingType.LONG)
.getter(getter(DescribeLimitsResponse::accountMaxReadCapacityUnits))
.setter(setter(Builder::accountMaxReadCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountMaxReadCapacityUnits")
.build()).build();
private static final SdkField ACCOUNT_MAX_WRITE_CAPACITY_UNITS_FIELD = SdkField
. builder(MarshallingType.LONG)
.getter(getter(DescribeLimitsResponse::accountMaxWriteCapacityUnits))
.setter(setter(Builder::accountMaxWriteCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountMaxWriteCapacityUnits")
.build()).build();
private static final SdkField TABLE_MAX_READ_CAPACITY_UNITS_FIELD = SdkField. builder(MarshallingType.LONG)
.getter(getter(DescribeLimitsResponse::tableMaxReadCapacityUnits)).setter(setter(Builder::tableMaxReadCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TableMaxReadCapacityUnits").build())
.build();
private static final SdkField TABLE_MAX_WRITE_CAPACITY_UNITS_FIELD = SdkField
. builder(MarshallingType.LONG)
.getter(getter(DescribeLimitsResponse::tableMaxWriteCapacityUnits))
.setter(setter(Builder::tableMaxWriteCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TableMaxWriteCapacityUnits").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
ACCOUNT_MAX_READ_CAPACITY_UNITS_FIELD, ACCOUNT_MAX_WRITE_CAPACITY_UNITS_FIELD, TABLE_MAX_READ_CAPACITY_UNITS_FIELD,
TABLE_MAX_WRITE_CAPACITY_UNITS_FIELD));
private final Long accountMaxReadCapacityUnits;
private final Long accountMaxWriteCapacityUnits;
private final Long tableMaxReadCapacityUnits;
private final Long tableMaxWriteCapacityUnits;
private DescribeLimitsResponse(BuilderImpl builder) {
super(builder);
this.accountMaxReadCapacityUnits = builder.accountMaxReadCapacityUnits;
this.accountMaxWriteCapacityUnits = builder.accountMaxWriteCapacityUnits;
this.tableMaxReadCapacityUnits = builder.tableMaxReadCapacityUnits;
this.tableMaxWriteCapacityUnits = builder.tableMaxWriteCapacityUnits;
}
/**
*
* The maximum total read capacity units that your account allows you to provision across all of your tables in this
* Region.
*
*
* @return The maximum total read capacity units that your account allows you to provision across all of your tables
* in this Region.
*/
public Long accountMaxReadCapacityUnits() {
return accountMaxReadCapacityUnits;
}
/**
*
* The maximum total write capacity units that your account allows you to provision across all of your tables in
* this Region.
*
*
* @return The maximum total write capacity units that your account allows you to provision across all of your
* tables in this Region.
*/
public Long accountMaxWriteCapacityUnits() {
return accountMaxWriteCapacityUnits;
}
/**
*
* The maximum read capacity units that your account allows you to provision for a new table that you are creating
* in this Region, including the read capacity units provisioned for its global secondary indexes (GSIs).
*
*
* @return The maximum read capacity units that your account allows you to provision for a new table that you are
* creating in this Region, including the read capacity units provisioned for its global secondary indexes
* (GSIs).
*/
public Long tableMaxReadCapacityUnits() {
return tableMaxReadCapacityUnits;
}
/**
*
* The maximum write capacity units that your account allows you to provision for a new table that you are creating
* in this Region, including the write capacity units provisioned for its global secondary indexes (GSIs).
*
*
* @return The maximum write capacity units that your account allows you to provision for a new table that you are
* creating in this Region, including the write capacity units provisioned for its global secondary indexes
* (GSIs).
*/
public Long tableMaxWriteCapacityUnits() {
return tableMaxWriteCapacityUnits;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(accountMaxReadCapacityUnits());
hashCode = 31 * hashCode + Objects.hashCode(accountMaxWriteCapacityUnits());
hashCode = 31 * hashCode + Objects.hashCode(tableMaxReadCapacityUnits());
hashCode = 31 * hashCode + Objects.hashCode(tableMaxWriteCapacityUnits());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeLimitsResponse)) {
return false;
}
DescribeLimitsResponse other = (DescribeLimitsResponse) obj;
return Objects.equals(accountMaxReadCapacityUnits(), other.accountMaxReadCapacityUnits())
&& Objects.equals(accountMaxWriteCapacityUnits(), other.accountMaxWriteCapacityUnits())
&& Objects.equals(tableMaxReadCapacityUnits(), other.tableMaxReadCapacityUnits())
&& Objects.equals(tableMaxWriteCapacityUnits(), other.tableMaxWriteCapacityUnits());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("DescribeLimitsResponse").add("AccountMaxReadCapacityUnits", accountMaxReadCapacityUnits())
.add("AccountMaxWriteCapacityUnits", accountMaxWriteCapacityUnits())
.add("TableMaxReadCapacityUnits", tableMaxReadCapacityUnits())
.add("TableMaxWriteCapacityUnits", tableMaxWriteCapacityUnits()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AccountMaxReadCapacityUnits":
return Optional.ofNullable(clazz.cast(accountMaxReadCapacityUnits()));
case "AccountMaxWriteCapacityUnits":
return Optional.ofNullable(clazz.cast(accountMaxWriteCapacityUnits()));
case "TableMaxReadCapacityUnits":
return Optional.ofNullable(clazz.cast(tableMaxReadCapacityUnits()));
case "TableMaxWriteCapacityUnits":
return Optional.ofNullable(clazz.cast(tableMaxWriteCapacityUnits()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function