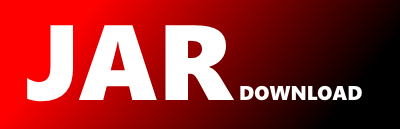
software.amazon.awssdk.services.dynamodb.model.CreateTableRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dynamodb.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the input of a CreateTable
operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateTableRequest extends DynamoDbRequest implements
ToCopyableBuilder {
private static final SdkField> ATTRIBUTE_DEFINITIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AttributeDefinitions")
.getter(getter(CreateTableRequest::attributeDefinitions))
.setter(setter(Builder::attributeDefinitions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AttributeDefinitions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AttributeDefinition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TABLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TableName").getter(getter(CreateTableRequest::tableName)).setter(setter(Builder::tableName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TableName").build()).build();
private static final SdkField> KEY_SCHEMA_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("KeySchema")
.getter(getter(CreateTableRequest::keySchema))
.setter(setter(Builder::keySchema))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeySchema").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(KeySchemaElement::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LOCAL_SECONDARY_INDEXES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LocalSecondaryIndexes")
.getter(getter(CreateTableRequest::localSecondaryIndexes))
.setter(setter(Builder::localSecondaryIndexes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LocalSecondaryIndexes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LocalSecondaryIndex::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> GLOBAL_SECONDARY_INDEXES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("GlobalSecondaryIndexes")
.getter(getter(CreateTableRequest::globalSecondaryIndexes))
.setter(setter(Builder::globalSecondaryIndexes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GlobalSecondaryIndexes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(GlobalSecondaryIndex::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField BILLING_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BillingMode").getter(getter(CreateTableRequest::billingModeAsString))
.setter(setter(Builder::billingMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BillingMode").build()).build();
private static final SdkField PROVISIONED_THROUGHPUT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ProvisionedThroughput")
.getter(getter(CreateTableRequest::provisionedThroughput)).setter(setter(Builder::provisionedThroughput))
.constructor(ProvisionedThroughput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProvisionedThroughput").build())
.build();
private static final SdkField STREAM_SPECIFICATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("StreamSpecification")
.getter(getter(CreateTableRequest::streamSpecification)).setter(setter(Builder::streamSpecification))
.constructor(StreamSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StreamSpecification").build())
.build();
private static final SdkField SSE_SPECIFICATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SSESpecification")
.getter(getter(CreateTableRequest::sseSpecification)).setter(setter(Builder::sseSpecification))
.constructor(SSESpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SSESpecification").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateTableRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ATTRIBUTE_DEFINITIONS_FIELD,
TABLE_NAME_FIELD, KEY_SCHEMA_FIELD, LOCAL_SECONDARY_INDEXES_FIELD, GLOBAL_SECONDARY_INDEXES_FIELD,
BILLING_MODE_FIELD, PROVISIONED_THROUGHPUT_FIELD, STREAM_SPECIFICATION_FIELD, SSE_SPECIFICATION_FIELD, TAGS_FIELD));
private final List attributeDefinitions;
private final String tableName;
private final List keySchema;
private final List localSecondaryIndexes;
private final List globalSecondaryIndexes;
private final String billingMode;
private final ProvisionedThroughput provisionedThroughput;
private final StreamSpecification streamSpecification;
private final SSESpecification sseSpecification;
private final List tags;
private CreateTableRequest(BuilderImpl builder) {
super(builder);
this.attributeDefinitions = builder.attributeDefinitions;
this.tableName = builder.tableName;
this.keySchema = builder.keySchema;
this.localSecondaryIndexes = builder.localSecondaryIndexes;
this.globalSecondaryIndexes = builder.globalSecondaryIndexes;
this.billingMode = builder.billingMode;
this.provisionedThroughput = builder.provisionedThroughput;
this.streamSpecification = builder.streamSpecification;
this.sseSpecification = builder.sseSpecification;
this.tags = builder.tags;
}
/**
* Returns true if the AttributeDefinitions property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasAttributeDefinitions() {
return attributeDefinitions != null && !(attributeDefinitions instanceof SdkAutoConstructList);
}
/**
*
* An array of attributes that describe the key schema for the table and indexes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAttributeDefinitions()} to see if a value was sent in this field.
*
*
* @return An array of attributes that describe the key schema for the table and indexes.
*/
public final List attributeDefinitions() {
return attributeDefinitions;
}
/**
*
* The name of the table to create.
*
*
* @return The name of the table to create.
*/
public final String tableName() {
return tableName;
}
/**
* Returns true if the KeySchema property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasKeySchema() {
return keySchema != null && !(keySchema instanceof SdkAutoConstructList);
}
/**
*
* Specifies the attributes that make up the primary key for a table or an index. The attributes in
* KeySchema
must also be defined in the AttributeDefinitions
array. For more information,
* see Data Model in
* the Amazon DynamoDB Developer Guide.
*
*
* Each KeySchemaElement
in the array is composed of:
*
*
* -
*
* AttributeName
- The name of this key attribute.
*
*
* -
*
* KeyType
- The role that the key attribute will assume:
*
*
* -
*
* HASH
- partition key
*
*
* -
*
* RANGE
- sort key
*
*
*
*
*
*
*
* The partition key of an item is also known as its hash attribute. The term "hash attribute" derives from
* the DynamoDB usage of an internal hash function to evenly distribute data items across partitions, based on their
* partition key values.
*
*
* The sort key of an item is also known as its range attribute. The term "range attribute" derives from the
* way DynamoDB stores items with the same partition key physically close together, in sorted order by the sort key
* value.
*
*
*
* For a simple primary key (partition key), you must provide exactly one element with a KeyType
of
* HASH
.
*
*
* For a composite primary key (partition key and sort key), you must provide exactly two elements, in this order:
* The first element must have a KeyType
of HASH
, and the second element must have a
* KeyType
of RANGE
.
*
*
* For more information, see Working with Tables in the Amazon DynamoDB Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasKeySchema()} to see if a value was sent in this field.
*
*
* @return Specifies the attributes that make up the primary key for a table or an index. The attributes in
* KeySchema
must also be defined in the AttributeDefinitions
array. For more
* information, see Data Model in
* the Amazon DynamoDB Developer Guide.
*
* Each KeySchemaElement
in the array is composed of:
*
*
* -
*
* AttributeName
- The name of this key attribute.
*
*
* -
*
* KeyType
- The role that the key attribute will assume:
*
*
* -
*
* HASH
- partition key
*
*
* -
*
* RANGE
- sort key
*
*
*
*
*
*
*
* The partition key of an item is also known as its hash attribute. The term "hash attribute"
* derives from the DynamoDB usage of an internal hash function to evenly distribute data items across
* partitions, based on their partition key values.
*
*
* The sort key of an item is also known as its range attribute. The term "range attribute" derives
* from the way DynamoDB stores items with the same partition key physically close together, in sorted order
* by the sort key value.
*
*
*
* For a simple primary key (partition key), you must provide exactly one element with a
* KeyType
of HASH
.
*
*
* For a composite primary key (partition key and sort key), you must provide exactly two elements, in this
* order: The first element must have a KeyType
of HASH
, and the second element
* must have a KeyType
of RANGE
.
*
*
* For more information, see Working with Tables in the Amazon DynamoDB Developer Guide.
*/
public final List keySchema() {
return keySchema;
}
/**
* Returns true if the LocalSecondaryIndexes property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasLocalSecondaryIndexes() {
return localSecondaryIndexes != null && !(localSecondaryIndexes instanceof SdkAutoConstructList);
}
/**
*
* One or more local secondary indexes (the maximum is 5) to be created on the table. Each index is scoped to a
* given partition key value. There is a 10 GB size limit per partition key value; otherwise, the size of a local
* secondary index is unconstrained.
*
*
* Each local secondary index in the array includes the following:
*
*
* -
*
* IndexName
- The name of the local secondary index. Must be unique only for this table.
*
*
* -
*
* KeySchema
- Specifies the key schema for the local secondary index. The key schema must begin with
* the same partition key as the table.
*
*
* -
*
* Projection
- Specifies attributes that are copied (projected) from the table into the index. These
* are in addition to the primary key attributes and index key attributes, which are automatically projected. Each
* attribute specification is composed of:
*
*
* -
*
* ProjectionType
- One of the following:
*
*
* -
*
* KEYS_ONLY
- Only the index and primary keys are projected into the index.
*
*
* -
*
* INCLUDE
- Only the specified table attributes are projected into the index. The list of projected
* attributes is in NonKeyAttributes
.
*
*
* -
*
* ALL
- All of the table attributes are projected into the index.
*
*
*
*
* -
*
* NonKeyAttributes
- A list of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes provided in NonKeyAttributes
, summed across all of
* the secondary indexes, must not exceed 100. If you project the same attribute into two different indexes, this
* counts as two distinct attributes when determining the total.
*
*
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasLocalSecondaryIndexes()} to see if a value was sent in this field.
*
*
* @return One or more local secondary indexes (the maximum is 5) to be created on the table. Each index is scoped
* to a given partition key value. There is a 10 GB size limit per partition key value; otherwise, the size
* of a local secondary index is unconstrained.
*
* Each local secondary index in the array includes the following:
*
*
* -
*
* IndexName
- The name of the local secondary index. Must be unique only for this table.
*
*
* -
*
* KeySchema
- Specifies the key schema for the local secondary index. The key schema must
* begin with the same partition key as the table.
*
*
* -
*
* Projection
- Specifies attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key attributes, which are automatically
* projected. Each attribute specification is composed of:
*
*
* -
*
* ProjectionType
- One of the following:
*
*
* -
*
* KEYS_ONLY
- Only the index and primary keys are projected into the index.
*
*
* -
*
* INCLUDE
- Only the specified table attributes are projected into the index. The list of
* projected attributes is in NonKeyAttributes
.
*
*
* -
*
* ALL
- All of the table attributes are projected into the index.
*
*
*
*
* -
*
* NonKeyAttributes
- A list of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes provided in NonKeyAttributes
, summed across
* all of the secondary indexes, must not exceed 100. If you project the same attribute into two different
* indexes, this counts as two distinct attributes when determining the total.
*
*
*
*
*/
public final List localSecondaryIndexes() {
return localSecondaryIndexes;
}
/**
* Returns true if the GlobalSecondaryIndexes property was specified by the sender (it may be empty), or false if
* the sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasGlobalSecondaryIndexes() {
return globalSecondaryIndexes != null && !(globalSecondaryIndexes instanceof SdkAutoConstructList);
}
/**
*
* One or more global secondary indexes (the maximum is 20) to be created on the table. Each global secondary index
* in the array includes the following:
*
*
* -
*
* IndexName
- The name of the global secondary index. Must be unique only for this table.
*
*
* -
*
* KeySchema
- Specifies the key schema for the global secondary index.
*
*
* -
*
* Projection
- Specifies attributes that are copied (projected) from the table into the index. These
* are in addition to the primary key attributes and index key attributes, which are automatically projected. Each
* attribute specification is composed of:
*
*
* -
*
* ProjectionType
- One of the following:
*
*
* -
*
* KEYS_ONLY
- Only the index and primary keys are projected into the index.
*
*
* -
*
* INCLUDE
- Only the specified table attributes are projected into the index. The list of projected
* attributes is in NonKeyAttributes
.
*
*
* -
*
* ALL
- All of the table attributes are projected into the index.
*
*
*
*
* -
*
* NonKeyAttributes
- A list of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes provided in NonKeyAttributes
, summed across all of
* the secondary indexes, must not exceed 100. If you project the same attribute into two different indexes, this
* counts as two distinct attributes when determining the total.
*
*
*
*
* -
*
* ProvisionedThroughput
- The provisioned throughput settings for the global secondary index,
* consisting of read and write capacity units.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasGlobalSecondaryIndexes()} to see if a value was sent in this field.
*
*
* @return One or more global secondary indexes (the maximum is 20) to be created on the table. Each global
* secondary index in the array includes the following:
*
* -
*
* IndexName
- The name of the global secondary index. Must be unique only for this table.
*
*
* -
*
* KeySchema
- Specifies the key schema for the global secondary index.
*
*
* -
*
* Projection
- Specifies attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key attributes, which are automatically
* projected. Each attribute specification is composed of:
*
*
* -
*
* ProjectionType
- One of the following:
*
*
* -
*
* KEYS_ONLY
- Only the index and primary keys are projected into the index.
*
*
* -
*
* INCLUDE
- Only the specified table attributes are projected into the index. The list of
* projected attributes is in NonKeyAttributes
.
*
*
* -
*
* ALL
- All of the table attributes are projected into the index.
*
*
*
*
* -
*
* NonKeyAttributes
- A list of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes provided in NonKeyAttributes
, summed across
* all of the secondary indexes, must not exceed 100. If you project the same attribute into two different
* indexes, this counts as two distinct attributes when determining the total.
*
*
*
*
* -
*
* ProvisionedThroughput
- The provisioned throughput settings for the global secondary index,
* consisting of read and write capacity units.
*
*
*/
public final List globalSecondaryIndexes() {
return globalSecondaryIndexes;
}
/**
*
* Controls how you are charged for read and write throughput and how you manage capacity. This setting can be
* changed later.
*
*
* -
*
* PROVISIONED
- We recommend using PROVISIONED
for predictable workloads.
* PROVISIONED
sets the billing mode to Provisioned Mode.
*
*
* -
*
* PAY_PER_REQUEST
- We recommend using PAY_PER_REQUEST
for unpredictable workloads.
* PAY_PER_REQUEST
sets the billing mode to On-Demand Mode.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMode} will
* return {@link BillingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingModeAsString}.
*
*
* @return Controls how you are charged for read and write throughput and how you manage capacity. This setting can
* be changed later.
*
* -
*
* PROVISIONED
- We recommend using PROVISIONED
for predictable workloads.
* PROVISIONED
sets the billing mode to Provisioned Mode.
*
*
* -
*
* PAY_PER_REQUEST
- We recommend using PAY_PER_REQUEST
for unpredictable
* workloads. PAY_PER_REQUEST
sets the billing mode to On-Demand Mode.
*
*
* @see BillingMode
*/
public final BillingMode billingMode() {
return BillingMode.fromValue(billingMode);
}
/**
*
* Controls how you are charged for read and write throughput and how you manage capacity. This setting can be
* changed later.
*
*
* -
*
* PROVISIONED
- We recommend using PROVISIONED
for predictable workloads.
* PROVISIONED
sets the billing mode to Provisioned Mode.
*
*
* -
*
* PAY_PER_REQUEST
- We recommend using PAY_PER_REQUEST
for unpredictable workloads.
* PAY_PER_REQUEST
sets the billing mode to On-Demand Mode.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #billingMode} will
* return {@link BillingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #billingModeAsString}.
*
*
* @return Controls how you are charged for read and write throughput and how you manage capacity. This setting can
* be changed later.
*
* -
*
* PROVISIONED
- We recommend using PROVISIONED
for predictable workloads.
* PROVISIONED
sets the billing mode to Provisioned Mode.
*
*
* -
*
* PAY_PER_REQUEST
- We recommend using PAY_PER_REQUEST
for unpredictable
* workloads. PAY_PER_REQUEST
sets the billing mode to On-Demand Mode.
*
*
* @see BillingMode
*/
public final String billingModeAsString() {
return billingMode;
}
/**
*
* Represents the provisioned throughput settings for a specified table or index. The settings can be modified using
* the UpdateTable
operation.
*
*
* If you set BillingMode as PROVISIONED
, you must specify this property. If you set BillingMode as
* PAY_PER_REQUEST
, you cannot specify this property.
*
*
* For current minimum and maximum provisioned throughput values, see Service, Account, and Table
* Quotas in the Amazon DynamoDB Developer Guide.
*
*
* @return Represents the provisioned throughput settings for a specified table or index. The settings can be
* modified using the UpdateTable
operation.
*
* If you set BillingMode as PROVISIONED
, you must specify this property. If you set
* BillingMode as PAY_PER_REQUEST
, you cannot specify this property.
*
*
* For current minimum and maximum provisioned throughput values, see Service, Account, and
* Table Quotas in the Amazon DynamoDB Developer Guide.
*/
public final ProvisionedThroughput provisionedThroughput() {
return provisionedThroughput;
}
/**
*
* The settings for DynamoDB Streams on the table. These settings consist of:
*
*
* -
*
* StreamEnabled
- Indicates whether DynamoDB Streams is to be enabled (true) or disabled (false).
*
*
* -
*
* StreamViewType
- When an item in the table is modified, StreamViewType
determines what
* information is written to the table's stream. Valid values for StreamViewType
are:
*
*
* -
*
* KEYS_ONLY
- Only the key attributes of the modified item are written to the stream.
*
*
* -
*
* NEW_IMAGE
- The entire item, as it appears after it was modified, is written to the stream.
*
*
* -
*
* OLD_IMAGE
- The entire item, as it appeared before it was modified, is written to the stream.
*
*
* -
*
* NEW_AND_OLD_IMAGES
- Both the new and the old item images of the item are written to the stream.
*
*
*
*
*
*
* @return The settings for DynamoDB Streams on the table. These settings consist of:
*
* -
*
* StreamEnabled
- Indicates whether DynamoDB Streams is to be enabled (true) or disabled
* (false).
*
*
* -
*
* StreamViewType
- When an item in the table is modified, StreamViewType
* determines what information is written to the table's stream. Valid values for
* StreamViewType
are:
*
*
* -
*
* KEYS_ONLY
- Only the key attributes of the modified item are written to the stream.
*
*
* -
*
* NEW_IMAGE
- The entire item, as it appears after it was modified, is written to the stream.
*
*
* -
*
* OLD_IMAGE
- The entire item, as it appeared before it was modified, is written to the
* stream.
*
*
* -
*
* NEW_AND_OLD_IMAGES
- Both the new and the old item images of the item are written to the
* stream.
*
*
*
*
*/
public final StreamSpecification streamSpecification() {
return streamSpecification;
}
/**
*
* Represents the settings used to enable server-side encryption.
*
*
* @return Represents the settings used to enable server-side encryption.
*/
public final SSESpecification sseSpecification() {
return sseSpecification;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of key-value pairs to label the table. For more information, see Tagging for DynamoDB.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return A list of key-value pairs to label the table. For more information, see Tagging for
* DynamoDB.
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hasAttributeDefinitions() ? attributeDefinitions() : null);
hashCode = 31 * hashCode + Objects.hashCode(tableName());
hashCode = 31 * hashCode + Objects.hashCode(hasKeySchema() ? keySchema() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLocalSecondaryIndexes() ? localSecondaryIndexes() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasGlobalSecondaryIndexes() ? globalSecondaryIndexes() : null);
hashCode = 31 * hashCode + Objects.hashCode(billingModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(provisionedThroughput());
hashCode = 31 * hashCode + Objects.hashCode(streamSpecification());
hashCode = 31 * hashCode + Objects.hashCode(sseSpecification());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateTableRequest)) {
return false;
}
CreateTableRequest other = (CreateTableRequest) obj;
return hasAttributeDefinitions() == other.hasAttributeDefinitions()
&& Objects.equals(attributeDefinitions(), other.attributeDefinitions())
&& Objects.equals(tableName(), other.tableName()) && hasKeySchema() == other.hasKeySchema()
&& Objects.equals(keySchema(), other.keySchema())
&& hasLocalSecondaryIndexes() == other.hasLocalSecondaryIndexes()
&& Objects.equals(localSecondaryIndexes(), other.localSecondaryIndexes())
&& hasGlobalSecondaryIndexes() == other.hasGlobalSecondaryIndexes()
&& Objects.equals(globalSecondaryIndexes(), other.globalSecondaryIndexes())
&& Objects.equals(billingModeAsString(), other.billingModeAsString())
&& Objects.equals(provisionedThroughput(), other.provisionedThroughput())
&& Objects.equals(streamSpecification(), other.streamSpecification())
&& Objects.equals(sseSpecification(), other.sseSpecification()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateTableRequest")
.add("AttributeDefinitions", hasAttributeDefinitions() ? attributeDefinitions() : null)
.add("TableName", tableName()).add("KeySchema", hasKeySchema() ? keySchema() : null)
.add("LocalSecondaryIndexes", hasLocalSecondaryIndexes() ? localSecondaryIndexes() : null)
.add("GlobalSecondaryIndexes", hasGlobalSecondaryIndexes() ? globalSecondaryIndexes() : null)
.add("BillingMode", billingModeAsString()).add("ProvisionedThroughput", provisionedThroughput())
.add("StreamSpecification", streamSpecification()).add("SSESpecification", sseSpecification())
.add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AttributeDefinitions":
return Optional.ofNullable(clazz.cast(attributeDefinitions()));
case "TableName":
return Optional.ofNullable(clazz.cast(tableName()));
case "KeySchema":
return Optional.ofNullable(clazz.cast(keySchema()));
case "LocalSecondaryIndexes":
return Optional.ofNullable(clazz.cast(localSecondaryIndexes()));
case "GlobalSecondaryIndexes":
return Optional.ofNullable(clazz.cast(globalSecondaryIndexes()));
case "BillingMode":
return Optional.ofNullable(clazz.cast(billingModeAsString()));
case "ProvisionedThroughput":
return Optional.ofNullable(clazz.cast(provisionedThroughput()));
case "StreamSpecification":
return Optional.ofNullable(clazz.cast(streamSpecification()));
case "SSESpecification":
return Optional.ofNullable(clazz.cast(sseSpecification()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function