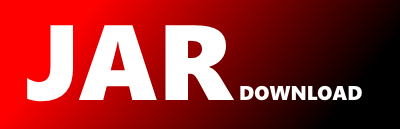
software.amazon.awssdk.services.dynamodb.model.GlobalSecondaryIndexDescription Maven / Gradle / Ivy
Show all versions of dynamodb Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dynamodb.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the properties of a global secondary index.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class GlobalSecondaryIndexDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField INDEX_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IndexName").getter(getter(GlobalSecondaryIndexDescription::indexName))
.setter(setter(Builder::indexName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IndexName").build()).build();
private static final SdkField> KEY_SCHEMA_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("KeySchema")
.getter(getter(GlobalSecondaryIndexDescription::keySchema))
.setter(setter(Builder::keySchema))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeySchema").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(KeySchemaElement::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PROJECTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Projection").getter(getter(GlobalSecondaryIndexDescription::projection))
.setter(setter(Builder::projection)).constructor(Projection::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Projection").build()).build();
private static final SdkField INDEX_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IndexStatus").getter(getter(GlobalSecondaryIndexDescription::indexStatusAsString))
.setter(setter(Builder::indexStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IndexStatus").build()).build();
private static final SdkField BACKFILLING_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Backfilling").getter(getter(GlobalSecondaryIndexDescription::backfilling))
.setter(setter(Builder::backfilling))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Backfilling").build()).build();
private static final SdkField PROVISIONED_THROUGHPUT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ProvisionedThroughput")
.getter(getter(GlobalSecondaryIndexDescription::provisionedThroughput))
.setter(setter(Builder::provisionedThroughput)).constructor(ProvisionedThroughputDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProvisionedThroughput").build())
.build();
private static final SdkField INDEX_SIZE_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("IndexSizeBytes").getter(getter(GlobalSecondaryIndexDescription::indexSizeBytes))
.setter(setter(Builder::indexSizeBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IndexSizeBytes").build()).build();
private static final SdkField ITEM_COUNT_FIELD = SdkField. builder(MarshallingType.LONG).memberName("ItemCount")
.getter(getter(GlobalSecondaryIndexDescription::itemCount)).setter(setter(Builder::itemCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ItemCount").build()).build();
private static final SdkField INDEX_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IndexArn").getter(getter(GlobalSecondaryIndexDescription::indexArn)).setter(setter(Builder::indexArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IndexArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(INDEX_NAME_FIELD,
KEY_SCHEMA_FIELD, PROJECTION_FIELD, INDEX_STATUS_FIELD, BACKFILLING_FIELD, PROVISIONED_THROUGHPUT_FIELD,
INDEX_SIZE_BYTES_FIELD, ITEM_COUNT_FIELD, INDEX_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final String indexName;
private final List keySchema;
private final Projection projection;
private final String indexStatus;
private final Boolean backfilling;
private final ProvisionedThroughputDescription provisionedThroughput;
private final Long indexSizeBytes;
private final Long itemCount;
private final String indexArn;
private GlobalSecondaryIndexDescription(BuilderImpl builder) {
this.indexName = builder.indexName;
this.keySchema = builder.keySchema;
this.projection = builder.projection;
this.indexStatus = builder.indexStatus;
this.backfilling = builder.backfilling;
this.provisionedThroughput = builder.provisionedThroughput;
this.indexSizeBytes = builder.indexSizeBytes;
this.itemCount = builder.itemCount;
this.indexArn = builder.indexArn;
}
/**
*
* The name of the global secondary index.
*
*
* @return The name of the global secondary index.
*/
public final String indexName() {
return indexName;
}
/**
* For responses, this returns true if the service returned a value for the KeySchema property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasKeySchema() {
return keySchema != null && !(keySchema instanceof SdkAutoConstructList);
}
/**
*
* The complete key schema for a global secondary index, which consists of one or more pairs of attribute names and
* key types:
*
*
* -
*
* HASH
- partition key
*
*
* -
*
* RANGE
- sort key
*
*
*
*
*
* The partition key of an item is also known as its hash attribute. The term "hash attribute" derives from
* DynamoDB's usage of an internal hash function to evenly distribute data items across partitions, based on their
* partition key values.
*
*
* The sort key of an item is also known as its range attribute. The term "range attribute" derives from the
* way DynamoDB stores items with the same partition key physically close together, in sorted order by the sort key
* value.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasKeySchema} method.
*
*
* @return The complete key schema for a global secondary index, which consists of one or more pairs of attribute
* names and key types:
*
* -
*
* HASH
- partition key
*
*
* -
*
* RANGE
- sort key
*
*
*
*
*
* The partition key of an item is also known as its hash attribute. The term "hash attribute"
* derives from DynamoDB's usage of an internal hash function to evenly distribute data items across
* partitions, based on their partition key values.
*
*
* The sort key of an item is also known as its range attribute. The term "range attribute" derives
* from the way DynamoDB stores items with the same partition key physically close together, in sorted order
* by the sort key value.
*
*/
public final List keySchema() {
return keySchema;
}
/**
*
* Represents attributes that are copied (projected) from the table into the global secondary index. These are in
* addition to the primary key attributes and index key attributes, which are automatically projected.
*
*
* @return Represents attributes that are copied (projected) from the table into the global secondary index. These
* are in addition to the primary key attributes and index key attributes, which are automatically
* projected.
*/
public final Projection projection() {
return projection;
}
/**
*
* The current state of the global secondary index:
*
*
* -
*
* CREATING
- The index is being created.
*
*
* -
*
* UPDATING
- The index is being updated.
*
*
* -
*
* DELETING
- The index is being deleted.
*
*
* -
*
* ACTIVE
- The index is ready for use.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #indexStatus} will
* return {@link IndexStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #indexStatusAsString}.
*
*
* @return The current state of the global secondary index:
*
* -
*
* CREATING
- The index is being created.
*
*
* -
*
* UPDATING
- The index is being updated.
*
*
* -
*
* DELETING
- The index is being deleted.
*
*
* -
*
* ACTIVE
- The index is ready for use.
*
*
* @see IndexStatus
*/
public final IndexStatus indexStatus() {
return IndexStatus.fromValue(indexStatus);
}
/**
*
* The current state of the global secondary index:
*
*
* -
*
* CREATING
- The index is being created.
*
*
* -
*
* UPDATING
- The index is being updated.
*
*
* -
*
* DELETING
- The index is being deleted.
*
*
* -
*
* ACTIVE
- The index is ready for use.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #indexStatus} will
* return {@link IndexStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #indexStatusAsString}.
*
*
* @return The current state of the global secondary index:
*
* -
*
* CREATING
- The index is being created.
*
*
* -
*
* UPDATING
- The index is being updated.
*
*
* -
*
* DELETING
- The index is being deleted.
*
*
* -
*
* ACTIVE
- The index is ready for use.
*
*
* @see IndexStatus
*/
public final String indexStatusAsString() {
return indexStatus;
}
/**
*
* Indicates whether the index is currently backfilling. Backfilling is the process of reading items from the
* table and determining whether they can be added to the index. (Not all items will qualify: For example, a
* partition key cannot have any duplicate values.) If an item can be added to the index, DynamoDB will do so. After
* all items have been processed, the backfilling operation is complete and Backfilling
is false.
*
*
* You can delete an index that is being created during the Backfilling
phase when
* IndexStatus
is set to CREATING and Backfilling
is true. You can't delete the index that
* is being created when IndexStatus
is set to CREATING and Backfilling
is false.
*
*
*
* For indexes that were created during a CreateTable
operation, the Backfilling
attribute
* does not appear in the DescribeTable
output.
*
*
*
* @return Indicates whether the index is currently backfilling. Backfilling is the process of reading items
* from the table and determining whether they can be added to the index. (Not all items will qualify: For
* example, a partition key cannot have any duplicate values.) If an item can be added to the index,
* DynamoDB will do so. After all items have been processed, the backfilling operation is complete and
* Backfilling
is false.
*
* You can delete an index that is being created during the Backfilling
phase when
* IndexStatus
is set to CREATING and Backfilling
is true. You can't delete the
* index that is being created when IndexStatus
is set to CREATING and Backfilling
* is false.
*
*
*
* For indexes that were created during a CreateTable
operation, the Backfilling
* attribute does not appear in the DescribeTable
output.
*
*/
public final Boolean backfilling() {
return backfilling;
}
/**
*
* Represents the provisioned throughput settings for the specified global secondary index.
*
*
* For current minimum and maximum provisioned throughput values, see Service, Account, and Table
* Quotas in the Amazon DynamoDB Developer Guide.
*
*
* @return Represents the provisioned throughput settings for the specified global secondary index.
*
* For current minimum and maximum provisioned throughput values, see Service, Account, and
* Table Quotas in the Amazon DynamoDB Developer Guide.
*/
public final ProvisionedThroughputDescription provisionedThroughput() {
return provisionedThroughput;
}
/**
*
* The total size of the specified index, in bytes. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
*
*
* @return The total size of the specified index, in bytes. DynamoDB updates this value approximately every six
* hours. Recent changes might not be reflected in this value.
*/
public final Long indexSizeBytes() {
return indexSizeBytes;
}
/**
*
* The number of items in the specified index. DynamoDB updates this value approximately every six hours. Recent
* changes might not be reflected in this value.
*
*
* @return The number of items in the specified index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
*/
public final Long itemCount() {
return itemCount;
}
/**
*
* The Amazon Resource Name (ARN) that uniquely identifies the index.
*
*
* @return The Amazon Resource Name (ARN) that uniquely identifies the index.
*/
public final String indexArn() {
return indexArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(indexName());
hashCode = 31 * hashCode + Objects.hashCode(hasKeySchema() ? keySchema() : null);
hashCode = 31 * hashCode + Objects.hashCode(projection());
hashCode = 31 * hashCode + Objects.hashCode(indexStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(backfilling());
hashCode = 31 * hashCode + Objects.hashCode(provisionedThroughput());
hashCode = 31 * hashCode + Objects.hashCode(indexSizeBytes());
hashCode = 31 * hashCode + Objects.hashCode(itemCount());
hashCode = 31 * hashCode + Objects.hashCode(indexArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GlobalSecondaryIndexDescription)) {
return false;
}
GlobalSecondaryIndexDescription other = (GlobalSecondaryIndexDescription) obj;
return Objects.equals(indexName(), other.indexName()) && hasKeySchema() == other.hasKeySchema()
&& Objects.equals(keySchema(), other.keySchema()) && Objects.equals(projection(), other.projection())
&& Objects.equals(indexStatusAsString(), other.indexStatusAsString())
&& Objects.equals(backfilling(), other.backfilling())
&& Objects.equals(provisionedThroughput(), other.provisionedThroughput())
&& Objects.equals(indexSizeBytes(), other.indexSizeBytes()) && Objects.equals(itemCount(), other.itemCount())
&& Objects.equals(indexArn(), other.indexArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GlobalSecondaryIndexDescription").add("IndexName", indexName())
.add("KeySchema", hasKeySchema() ? keySchema() : null).add("Projection", projection())
.add("IndexStatus", indexStatusAsString()).add("Backfilling", backfilling())
.add("ProvisionedThroughput", provisionedThroughput()).add("IndexSizeBytes", indexSizeBytes())
.add("ItemCount", itemCount()).add("IndexArn", indexArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "IndexName":
return Optional.ofNullable(clazz.cast(indexName()));
case "KeySchema":
return Optional.ofNullable(clazz.cast(keySchema()));
case "Projection":
return Optional.ofNullable(clazz.cast(projection()));
case "IndexStatus":
return Optional.ofNullable(clazz.cast(indexStatusAsString()));
case "Backfilling":
return Optional.ofNullable(clazz.cast(backfilling()));
case "ProvisionedThroughput":
return Optional.ofNullable(clazz.cast(provisionedThroughput()));
case "IndexSizeBytes":
return Optional.ofNullable(clazz.cast(indexSizeBytes()));
case "ItemCount":
return Optional.ofNullable(clazz.cast(itemCount()));
case "IndexArn":
return Optional.ofNullable(clazz.cast(indexArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function