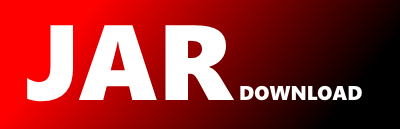
software.amazon.awssdk.services.dynamodb.model.TransactGetItemsResponse Maven / Gradle / Ivy
Show all versions of dynamodb Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dynamodb.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class TransactGetItemsResponse extends DynamoDbResponse implements
ToCopyableBuilder {
private static final SdkField> CONSUMED_CAPACITY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ConsumedCapacity")
.getter(getter(TransactGetItemsResponse::consumedCapacity))
.setter(setter(Builder::consumedCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConsumedCapacity").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ConsumedCapacity::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> RESPONSES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Responses")
.getter(getter(TransactGetItemsResponse::responses))
.setter(setter(Builder::responses))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Responses").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ItemResponse::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONSUMED_CAPACITY_FIELD,
RESPONSES_FIELD));
private final List consumedCapacity;
private final List responses;
private TransactGetItemsResponse(BuilderImpl builder) {
super(builder);
this.consumedCapacity = builder.consumedCapacity;
this.responses = builder.responses;
}
/**
* For responses, this returns true if the service returned a value for the ConsumedCapacity property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasConsumedCapacity() {
return consumedCapacity != null && !(consumedCapacity instanceof SdkAutoConstructList);
}
/**
*
* If the ReturnConsumedCapacity value was TOTAL
, this is an array of
* ConsumedCapacity
objects, one for each table addressed by TransactGetItem
objects in
* the TransactItems parameter. These ConsumedCapacity
objects report the read-capacity units
* consumed by the TransactGetItems
call in that table.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasConsumedCapacity} method.
*
*
* @return If the ReturnConsumedCapacity value was TOTAL
, this is an array of
* ConsumedCapacity
objects, one for each table addressed by TransactGetItem
* objects in the TransactItems parameter. These ConsumedCapacity
objects report the
* read-capacity units consumed by the TransactGetItems
call in that table.
*/
public final List consumedCapacity() {
return consumedCapacity;
}
/**
* For responses, this returns true if the service returned a value for the Responses property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasResponses() {
return responses != null && !(responses instanceof SdkAutoConstructList);
}
/**
*
* An ordered array of up to 25 ItemResponse
objects, each of which corresponds to the
* TransactGetItem
object in the same position in the TransactItems array. Each
* ItemResponse
object contains a Map of the name-value pairs that are the projected attributes of the
* requested item.
*
*
* If a requested item could not be retrieved, the corresponding ItemResponse
object is Null, or if the
* requested item has no projected attributes, the corresponding ItemResponse
object is an empty Map.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResponses} method.
*
*
* @return An ordered array of up to 25 ItemResponse
objects, each of which corresponds to the
* TransactGetItem
object in the same position in the TransactItems array. Each
* ItemResponse
object contains a Map of the name-value pairs that are the projected attributes
* of the requested item.
*
* If a requested item could not be retrieved, the corresponding ItemResponse
object is Null,
* or if the requested item has no projected attributes, the corresponding ItemResponse
object
* is an empty Map.
*/
public final List responses() {
return responses;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hasConsumedCapacity() ? consumedCapacity() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasResponses() ? responses() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TransactGetItemsResponse)) {
return false;
}
TransactGetItemsResponse other = (TransactGetItemsResponse) obj;
return hasConsumedCapacity() == other.hasConsumedCapacity()
&& Objects.equals(consumedCapacity(), other.consumedCapacity()) && hasResponses() == other.hasResponses()
&& Objects.equals(responses(), other.responses());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TransactGetItemsResponse")
.add("ConsumedCapacity", hasConsumedCapacity() ? consumedCapacity() : null)
.add("Responses", hasResponses() ? responses() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ConsumedCapacity":
return Optional.ofNullable(clazz.cast(consumedCapacity()));
case "Responses":
return Optional.ofNullable(clazz.cast(responses()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If a requested item could not be retrieved, the corresponding ItemResponse
object is
* Null, or if the requested item has no projected attributes, the corresponding
* ItemResponse
object is an empty Map.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder responses(Collection responses);
/**
*
* An ordered array of up to 25 ItemResponse
objects, each of which corresponds to the
* TransactGetItem
object in the same position in the TransactItems array. Each
* ItemResponse
object contains a Map of the name-value pairs that are the projected attributes of
* the requested item.
*
*
* If a requested item could not be retrieved, the corresponding ItemResponse
object is Null, or if
* the requested item has no projected attributes, the corresponding ItemResponse
object is an
* empty Map.
*
*
* @param responses
* An ordered array of up to 25 ItemResponse
objects, each of which corresponds to the
* TransactGetItem
object in the same position in the TransactItems array. Each
* ItemResponse
object contains a Map of the name-value pairs that are the projected
* attributes of the requested item.
*
* If a requested item could not be retrieved, the corresponding ItemResponse
object is
* Null, or if the requested item has no projected attributes, the corresponding
* ItemResponse
object is an empty Map.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder responses(ItemResponse... responses);
/**
*
* An ordered array of up to 25 ItemResponse
objects, each of which corresponds to the
* TransactGetItem
object in the same position in the TransactItems array. Each
* ItemResponse
object contains a Map of the name-value pairs that are the projected attributes of
* the requested item.
*
*
* If a requested item could not be retrieved, the corresponding ItemResponse
object is Null, or if
* the requested item has no projected attributes, the corresponding ItemResponse
object is an
* empty Map.
*
* This is a convenience method that creates an instance of the {@link List.Builder} avoiding the
* need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its
* result is passed to {@link #responses(List)}.
*
* @param responses
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #responses(List)
*/
Builder responses(Consumer... responses);
}
static final class BuilderImpl extends DynamoDbResponse.BuilderImpl implements Builder {
private List consumedCapacity = DefaultSdkAutoConstructList.getInstance();
private List responses = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(TransactGetItemsResponse model) {
super(model);
consumedCapacity(model.consumedCapacity);
responses(model.responses);
}
public final List getConsumedCapacity() {
List result = ConsumedCapacityMultipleCopier.copyToBuilder(this.consumedCapacity);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setConsumedCapacity(Collection consumedCapacity) {
this.consumedCapacity = ConsumedCapacityMultipleCopier.copyFromBuilder(consumedCapacity);
}
@Override
public final Builder consumedCapacity(Collection consumedCapacity) {
this.consumedCapacity = ConsumedCapacityMultipleCopier.copy(consumedCapacity);
return this;
}
@Override
@SafeVarargs
public final Builder consumedCapacity(ConsumedCapacity... consumedCapacity) {
consumedCapacity(Arrays.asList(consumedCapacity));
return this;
}
@Override
@SafeVarargs
public final Builder consumedCapacity(Consumer... consumedCapacity) {
consumedCapacity(Stream.of(consumedCapacity).map(c -> ConsumedCapacity.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final List getResponses() {
List result = ItemResponseListCopier.copyToBuilder(this.responses);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setResponses(Collection responses) {
this.responses = ItemResponseListCopier.copyFromBuilder(responses);
}
@Override
public final Builder responses(Collection responses) {
this.responses = ItemResponseListCopier.copy(responses);
return this;
}
@Override
@SafeVarargs
public final Builder responses(ItemResponse... responses) {
responses(Arrays.asList(responses));
return this;
}
@Override
@SafeVarargs
public final Builder responses(Consumer... responses) {
responses(Stream.of(responses).map(c -> ItemResponse.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
@Override
public TransactGetItemsResponse build() {
return new TransactGetItemsResponse(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}