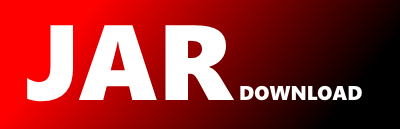
software.amazon.awssdk.services.dynamodb.model.ReplicaSettingsUpdate Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dynamodb.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the settings for a global table in a Region that will be modified.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReplicaSettingsUpdate implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField REGION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RegionName").getter(getter(ReplicaSettingsUpdate::regionName)).setter(setter(Builder::regionName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegionName").build()).build();
private static final SdkField REPLICA_PROVISIONED_READ_CAPACITY_UNITS_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("ReplicaProvisionedReadCapacityUnits")
.getter(getter(ReplicaSettingsUpdate::replicaProvisionedReadCapacityUnits))
.setter(setter(Builder::replicaProvisionedReadCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ReplicaProvisionedReadCapacityUnits").build()).build();
private static final SdkField REPLICA_PROVISIONED_READ_CAPACITY_AUTO_SCALING_SETTINGS_UPDATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ReplicaProvisionedReadCapacityAutoScalingSettingsUpdate")
.getter(getter(ReplicaSettingsUpdate::replicaProvisionedReadCapacityAutoScalingSettingsUpdate))
.setter(setter(Builder::replicaProvisionedReadCapacityAutoScalingSettingsUpdate))
.constructor(AutoScalingSettingsUpdate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ReplicaProvisionedReadCapacityAutoScalingSettingsUpdate").build()).build();
private static final SdkField> REPLICA_GLOBAL_SECONDARY_INDEX_SETTINGS_UPDATE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ReplicaGlobalSecondaryIndexSettingsUpdate")
.getter(getter(ReplicaSettingsUpdate::replicaGlobalSecondaryIndexSettingsUpdate))
.setter(setter(Builder::replicaGlobalSecondaryIndexSettingsUpdate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ReplicaGlobalSecondaryIndexSettingsUpdate").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ReplicaGlobalSecondaryIndexSettingsUpdate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField REPLICA_TABLE_CLASS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReplicaTableClass").getter(getter(ReplicaSettingsUpdate::replicaTableClassAsString))
.setter(setter(Builder::replicaTableClass))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicaTableClass").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REGION_NAME_FIELD,
REPLICA_PROVISIONED_READ_CAPACITY_UNITS_FIELD, REPLICA_PROVISIONED_READ_CAPACITY_AUTO_SCALING_SETTINGS_UPDATE_FIELD,
REPLICA_GLOBAL_SECONDARY_INDEX_SETTINGS_UPDATE_FIELD, REPLICA_TABLE_CLASS_FIELD));
private static final long serialVersionUID = 1L;
private final String regionName;
private final Long replicaProvisionedReadCapacityUnits;
private final AutoScalingSettingsUpdate replicaProvisionedReadCapacityAutoScalingSettingsUpdate;
private final List replicaGlobalSecondaryIndexSettingsUpdate;
private final String replicaTableClass;
private ReplicaSettingsUpdate(BuilderImpl builder) {
this.regionName = builder.regionName;
this.replicaProvisionedReadCapacityUnits = builder.replicaProvisionedReadCapacityUnits;
this.replicaProvisionedReadCapacityAutoScalingSettingsUpdate = builder.replicaProvisionedReadCapacityAutoScalingSettingsUpdate;
this.replicaGlobalSecondaryIndexSettingsUpdate = builder.replicaGlobalSecondaryIndexSettingsUpdate;
this.replicaTableClass = builder.replicaTableClass;
}
/**
*
* The Region of the replica to be added.
*
*
* @return The Region of the replica to be added.
*/
public final String regionName() {
return regionName;
}
/**
*
* The maximum number of strongly consistent reads consumed per second before DynamoDB returns a
* ThrottlingException
. For more information, see Specifying Read and Write Requirements in the Amazon DynamoDB Developer Guide.
*
*
* @return The maximum number of strongly consistent reads consumed per second before DynamoDB returns a
* ThrottlingException
. For more information, see Specifying Read and Write Requirements in the Amazon DynamoDB Developer Guide.
*/
public final Long replicaProvisionedReadCapacityUnits() {
return replicaProvisionedReadCapacityUnits;
}
/**
*
* Auto scaling settings for managing a global table replica's read capacity units.
*
*
* @return Auto scaling settings for managing a global table replica's read capacity units.
*/
public final AutoScalingSettingsUpdate replicaProvisionedReadCapacityAutoScalingSettingsUpdate() {
return replicaProvisionedReadCapacityAutoScalingSettingsUpdate;
}
/**
* For responses, this returns true if the service returned a value for the
* ReplicaGlobalSecondaryIndexSettingsUpdate property. This DOES NOT check that the value is non-empty (for which,
* you should check the {@code isEmpty()} method on the property). This is useful because the SDK will never return
* a null collection or map, but you may need to differentiate between the service returning nothing (or null) and
* the service returning an empty collection or map. For requests, this returns true if a value for the property was
* specified in the request builder, and false if a value was not specified.
*/
public final boolean hasReplicaGlobalSecondaryIndexSettingsUpdate() {
return replicaGlobalSecondaryIndexSettingsUpdate != null
&& !(replicaGlobalSecondaryIndexSettingsUpdate instanceof SdkAutoConstructList);
}
/**
*
* Represents the settings of a global secondary index for a global table that will be modified.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the
* {@link #hasReplicaGlobalSecondaryIndexSettingsUpdate} method.
*
*
* @return Represents the settings of a global secondary index for a global table that will be modified.
*/
public final List replicaGlobalSecondaryIndexSettingsUpdate() {
return replicaGlobalSecondaryIndexSettingsUpdate;
}
/**
*
* Replica-specific table class. If not specified, uses the source table's table class.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #replicaTableClass}
* will return {@link TableClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #replicaTableClassAsString}.
*
*
* @return Replica-specific table class. If not specified, uses the source table's table class.
* @see TableClass
*/
public final TableClass replicaTableClass() {
return TableClass.fromValue(replicaTableClass);
}
/**
*
* Replica-specific table class. If not specified, uses the source table's table class.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #replicaTableClass}
* will return {@link TableClass#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #replicaTableClassAsString}.
*
*
* @return Replica-specific table class. If not specified, uses the source table's table class.
* @see TableClass
*/
public final String replicaTableClassAsString() {
return replicaTableClass;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(regionName());
hashCode = 31 * hashCode + Objects.hashCode(replicaProvisionedReadCapacityUnits());
hashCode = 31 * hashCode + Objects.hashCode(replicaProvisionedReadCapacityAutoScalingSettingsUpdate());
hashCode = 31
* hashCode
+ Objects.hashCode(hasReplicaGlobalSecondaryIndexSettingsUpdate() ? replicaGlobalSecondaryIndexSettingsUpdate()
: null);
hashCode = 31 * hashCode + Objects.hashCode(replicaTableClassAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReplicaSettingsUpdate)) {
return false;
}
ReplicaSettingsUpdate other = (ReplicaSettingsUpdate) obj;
return Objects.equals(regionName(), other.regionName())
&& Objects.equals(replicaProvisionedReadCapacityUnits(), other.replicaProvisionedReadCapacityUnits())
&& Objects.equals(replicaProvisionedReadCapacityAutoScalingSettingsUpdate(),
other.replicaProvisionedReadCapacityAutoScalingSettingsUpdate())
&& hasReplicaGlobalSecondaryIndexSettingsUpdate() == other.hasReplicaGlobalSecondaryIndexSettingsUpdate()
&& Objects.equals(replicaGlobalSecondaryIndexSettingsUpdate(), other.replicaGlobalSecondaryIndexSettingsUpdate())
&& Objects.equals(replicaTableClassAsString(), other.replicaTableClassAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("ReplicaSettingsUpdate")
.add("RegionName", regionName())
.add("ReplicaProvisionedReadCapacityUnits", replicaProvisionedReadCapacityUnits())
.add("ReplicaProvisionedReadCapacityAutoScalingSettingsUpdate",
replicaProvisionedReadCapacityAutoScalingSettingsUpdate())
.add("ReplicaGlobalSecondaryIndexSettingsUpdate",
hasReplicaGlobalSecondaryIndexSettingsUpdate() ? replicaGlobalSecondaryIndexSettingsUpdate() : null)
.add("ReplicaTableClass", replicaTableClassAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RegionName":
return Optional.ofNullable(clazz.cast(regionName()));
case "ReplicaProvisionedReadCapacityUnits":
return Optional.ofNullable(clazz.cast(replicaProvisionedReadCapacityUnits()));
case "ReplicaProvisionedReadCapacityAutoScalingSettingsUpdate":
return Optional.ofNullable(clazz.cast(replicaProvisionedReadCapacityAutoScalingSettingsUpdate()));
case "ReplicaGlobalSecondaryIndexSettingsUpdate":
return Optional.ofNullable(clazz.cast(replicaGlobalSecondaryIndexSettingsUpdate()));
case "ReplicaTableClass":
return Optional.ofNullable(clazz.cast(replicaTableClassAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function