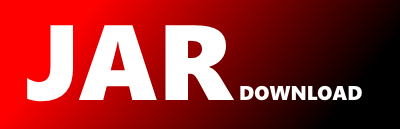
software.amazon.awssdk.services.dynamodb.model.ExportDescription Maven / Gradle / Ivy
Show all versions of dynamodb Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.dynamodb.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the properties of the exported table.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ExportDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField EXPORT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExportArn").getter(getter(ExportDescription::exportArn)).setter(setter(Builder::exportArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExportArn").build()).build();
private static final SdkField EXPORT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExportStatus").getter(getter(ExportDescription::exportStatusAsString))
.setter(setter(Builder::exportStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExportStatus").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTime").getter(getter(ExportDescription::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTime").getter(getter(ExportDescription::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField EXPORT_MANIFEST_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExportManifest").getter(getter(ExportDescription::exportManifest))
.setter(setter(Builder::exportManifest))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExportManifest").build()).build();
private static final SdkField TABLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TableArn").getter(getter(ExportDescription::tableArn)).setter(setter(Builder::tableArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TableArn").build()).build();
private static final SdkField TABLE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TableId").getter(getter(ExportDescription::tableId)).setter(setter(Builder::tableId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TableId").build()).build();
private static final SdkField EXPORT_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ExportTime").getter(getter(ExportDescription::exportTime)).setter(setter(Builder::exportTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExportTime").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClientToken").getter(getter(ExportDescription::clientToken)).setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken").build()).build();
private static final SdkField S3_BUCKET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("S3Bucket").getter(getter(ExportDescription::s3Bucket)).setter(setter(Builder::s3Bucket))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3Bucket").build()).build();
private static final SdkField S3_BUCKET_OWNER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("S3BucketOwner").getter(getter(ExportDescription::s3BucketOwner)).setter(setter(Builder::s3BucketOwner))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3BucketOwner").build()).build();
private static final SdkField S3_PREFIX_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("S3Prefix").getter(getter(ExportDescription::s3Prefix)).setter(setter(Builder::s3Prefix))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3Prefix").build()).build();
private static final SdkField S3_SSE_ALGORITHM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("S3SseAlgorithm").getter(getter(ExportDescription::s3SseAlgorithmAsString))
.setter(setter(Builder::s3SseAlgorithm))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3SseAlgorithm").build()).build();
private static final SdkField S3_SSE_KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("S3SseKmsKeyId").getter(getter(ExportDescription::s3SseKmsKeyId)).setter(setter(Builder::s3SseKmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3SseKmsKeyId").build()).build();
private static final SdkField FAILURE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureCode").getter(getter(ExportDescription::failureCode)).setter(setter(Builder::failureCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureCode").build()).build();
private static final SdkField FAILURE_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureMessage").getter(getter(ExportDescription::failureMessage))
.setter(setter(Builder::failureMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureMessage").build()).build();
private static final SdkField EXPORT_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExportFormat").getter(getter(ExportDescription::exportFormatAsString))
.setter(setter(Builder::exportFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExportFormat").build()).build();
private static final SdkField BILLED_SIZE_BYTES_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("BilledSizeBytes").getter(getter(ExportDescription::billedSizeBytes))
.setter(setter(Builder::billedSizeBytes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BilledSizeBytes").build()).build();
private static final SdkField ITEM_COUNT_FIELD = SdkField. builder(MarshallingType.LONG).memberName("ItemCount")
.getter(getter(ExportDescription::itemCount)).setter(setter(Builder::itemCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ItemCount").build()).build();
private static final SdkField EXPORT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExportType").getter(getter(ExportDescription::exportTypeAsString)).setter(setter(Builder::exportType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExportType").build()).build();
private static final SdkField INCREMENTAL_EXPORT_SPECIFICATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("IncrementalExportSpecification")
.getter(getter(ExportDescription::incrementalExportSpecification))
.setter(setter(Builder::incrementalExportSpecification))
.constructor(IncrementalExportSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncrementalExportSpecification")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EXPORT_ARN_FIELD,
EXPORT_STATUS_FIELD, START_TIME_FIELD, END_TIME_FIELD, EXPORT_MANIFEST_FIELD, TABLE_ARN_FIELD, TABLE_ID_FIELD,
EXPORT_TIME_FIELD, CLIENT_TOKEN_FIELD, S3_BUCKET_FIELD, S3_BUCKET_OWNER_FIELD, S3_PREFIX_FIELD,
S3_SSE_ALGORITHM_FIELD, S3_SSE_KMS_KEY_ID_FIELD, FAILURE_CODE_FIELD, FAILURE_MESSAGE_FIELD, EXPORT_FORMAT_FIELD,
BILLED_SIZE_BYTES_FIELD, ITEM_COUNT_FIELD, EXPORT_TYPE_FIELD, INCREMENTAL_EXPORT_SPECIFICATION_FIELD));
private static final long serialVersionUID = 1L;
private final String exportArn;
private final String exportStatus;
private final Instant startTime;
private final Instant endTime;
private final String exportManifest;
private final String tableArn;
private final String tableId;
private final Instant exportTime;
private final String clientToken;
private final String s3Bucket;
private final String s3BucketOwner;
private final String s3Prefix;
private final String s3SseAlgorithm;
private final String s3SseKmsKeyId;
private final String failureCode;
private final String failureMessage;
private final String exportFormat;
private final Long billedSizeBytes;
private final Long itemCount;
private final String exportType;
private final IncrementalExportSpecification incrementalExportSpecification;
private ExportDescription(BuilderImpl builder) {
this.exportArn = builder.exportArn;
this.exportStatus = builder.exportStatus;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.exportManifest = builder.exportManifest;
this.tableArn = builder.tableArn;
this.tableId = builder.tableId;
this.exportTime = builder.exportTime;
this.clientToken = builder.clientToken;
this.s3Bucket = builder.s3Bucket;
this.s3BucketOwner = builder.s3BucketOwner;
this.s3Prefix = builder.s3Prefix;
this.s3SseAlgorithm = builder.s3SseAlgorithm;
this.s3SseKmsKeyId = builder.s3SseKmsKeyId;
this.failureCode = builder.failureCode;
this.failureMessage = builder.failureMessage;
this.exportFormat = builder.exportFormat;
this.billedSizeBytes = builder.billedSizeBytes;
this.itemCount = builder.itemCount;
this.exportType = builder.exportType;
this.incrementalExportSpecification = builder.incrementalExportSpecification;
}
/**
*
* The Amazon Resource Name (ARN) of the table export.
*
*
* @return The Amazon Resource Name (ARN) of the table export.
*/
public final String exportArn() {
return exportArn;
}
/**
*
* Export can be in one of the following states: IN_PROGRESS, COMPLETED, or FAILED.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportStatus} will
* return {@link ExportStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportStatusAsString}.
*
*
* @return Export can be in one of the following states: IN_PROGRESS, COMPLETED, or FAILED.
* @see ExportStatus
*/
public final ExportStatus exportStatus() {
return ExportStatus.fromValue(exportStatus);
}
/**
*
* Export can be in one of the following states: IN_PROGRESS, COMPLETED, or FAILED.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportStatus} will
* return {@link ExportStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportStatusAsString}.
*
*
* @return Export can be in one of the following states: IN_PROGRESS, COMPLETED, or FAILED.
* @see ExportStatus
*/
public final String exportStatusAsString() {
return exportStatus;
}
/**
*
* The time at which the export task began.
*
*
* @return The time at which the export task began.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* The time at which the export task completed.
*
*
* @return The time at which the export task completed.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* The name of the manifest file for the export task.
*
*
* @return The name of the manifest file for the export task.
*/
public final String exportManifest() {
return exportManifest;
}
/**
*
* The Amazon Resource Name (ARN) of the table that was exported.
*
*
* @return The Amazon Resource Name (ARN) of the table that was exported.
*/
public final String tableArn() {
return tableArn;
}
/**
*
* Unique ID of the table that was exported.
*
*
* @return Unique ID of the table that was exported.
*/
public final String tableId() {
return tableId;
}
/**
*
* Point in time from which table data was exported.
*
*
* @return Point in time from which table data was exported.
*/
public final Instant exportTime() {
return exportTime;
}
/**
*
* The client token that was provided for the export task. A client token makes calls to
* ExportTableToPointInTimeInput
idempotent, meaning that multiple identical calls have the same effect
* as one single call.
*
*
* @return The client token that was provided for the export task. A client token makes calls to
* ExportTableToPointInTimeInput
idempotent, meaning that multiple identical calls have the
* same effect as one single call.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* The name of the Amazon S3 bucket containing the export.
*
*
* @return The name of the Amazon S3 bucket containing the export.
*/
public final String s3Bucket() {
return s3Bucket;
}
/**
*
* The ID of the Amazon Web Services account that owns the bucket containing the export.
*
*
* @return The ID of the Amazon Web Services account that owns the bucket containing the export.
*/
public final String s3BucketOwner() {
return s3BucketOwner;
}
/**
*
* The Amazon S3 bucket prefix used as the file name and path of the exported snapshot.
*
*
* @return The Amazon S3 bucket prefix used as the file name and path of the exported snapshot.
*/
public final String s3Prefix() {
return s3Prefix;
}
/**
*
* Type of encryption used on the bucket where export data is stored. Valid values for S3SseAlgorithm
* are:
*
*
* -
*
* AES256
- server-side encryption with Amazon S3 managed keys
*
*
* -
*
* KMS
- server-side encryption with KMS managed keys
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #s3SseAlgorithm}
* will return {@link S3SseAlgorithm#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #s3SseAlgorithmAsString}.
*
*
* @return Type of encryption used on the bucket where export data is stored. Valid values for
* S3SseAlgorithm
are:
*
* -
*
* AES256
- server-side encryption with Amazon S3 managed keys
*
*
* -
*
* KMS
- server-side encryption with KMS managed keys
*
*
* @see S3SseAlgorithm
*/
public final S3SseAlgorithm s3SseAlgorithm() {
return S3SseAlgorithm.fromValue(s3SseAlgorithm);
}
/**
*
* Type of encryption used on the bucket where export data is stored. Valid values for S3SseAlgorithm
* are:
*
*
* -
*
* AES256
- server-side encryption with Amazon S3 managed keys
*
*
* -
*
* KMS
- server-side encryption with KMS managed keys
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #s3SseAlgorithm}
* will return {@link S3SseAlgorithm#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #s3SseAlgorithmAsString}.
*
*
* @return Type of encryption used on the bucket where export data is stored. Valid values for
* S3SseAlgorithm
are:
*
* -
*
* AES256
- server-side encryption with Amazon S3 managed keys
*
*
* -
*
* KMS
- server-side encryption with KMS managed keys
*
*
* @see S3SseAlgorithm
*/
public final String s3SseAlgorithmAsString() {
return s3SseAlgorithm;
}
/**
*
* The ID of the KMS managed key used to encrypt the S3 bucket where export data is stored (if applicable).
*
*
* @return The ID of the KMS managed key used to encrypt the S3 bucket where export data is stored (if applicable).
*/
public final String s3SseKmsKeyId() {
return s3SseKmsKeyId;
}
/**
*
* Status code for the result of the failed export.
*
*
* @return Status code for the result of the failed export.
*/
public final String failureCode() {
return failureCode;
}
/**
*
* Export failure reason description.
*
*
* @return Export failure reason description.
*/
public final String failureMessage() {
return failureMessage;
}
/**
*
* The format of the exported data. Valid values for ExportFormat
are DYNAMODB_JSON
or
* ION
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportFormat} will
* return {@link ExportFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportFormatAsString}.
*
*
* @return The format of the exported data. Valid values for ExportFormat
are
* DYNAMODB_JSON
or ION
.
* @see ExportFormat
*/
public final ExportFormat exportFormat() {
return ExportFormat.fromValue(exportFormat);
}
/**
*
* The format of the exported data. Valid values for ExportFormat
are DYNAMODB_JSON
or
* ION
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportFormat} will
* return {@link ExportFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportFormatAsString}.
*
*
* @return The format of the exported data. Valid values for ExportFormat
are
* DYNAMODB_JSON
or ION
.
* @see ExportFormat
*/
public final String exportFormatAsString() {
return exportFormat;
}
/**
*
* The billable size of the table export.
*
*
* @return The billable size of the table export.
*/
public final Long billedSizeBytes() {
return billedSizeBytes;
}
/**
*
* The number of items exported.
*
*
* @return The number of items exported.
*/
public final Long itemCount() {
return itemCount;
}
/**
*
* The type of export that was performed. Valid values are FULL_EXPORT
or
* INCREMENTAL_EXPORT
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportType} will
* return {@link ExportType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportTypeAsString}.
*
*
* @return The type of export that was performed. Valid values are FULL_EXPORT
or
* INCREMENTAL_EXPORT
.
* @see ExportType
*/
public final ExportType exportType() {
return ExportType.fromValue(exportType);
}
/**
*
* The type of export that was performed. Valid values are FULL_EXPORT
or
* INCREMENTAL_EXPORT
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportType} will
* return {@link ExportType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportTypeAsString}.
*
*
* @return The type of export that was performed. Valid values are FULL_EXPORT
or
* INCREMENTAL_EXPORT
.
* @see ExportType
*/
public final String exportTypeAsString() {
return exportType;
}
/**
*
* Optional object containing the parameters specific to an incremental export.
*
*
* @return Optional object containing the parameters specific to an incremental export.
*/
public final IncrementalExportSpecification incrementalExportSpecification() {
return incrementalExportSpecification;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(exportArn());
hashCode = 31 * hashCode + Objects.hashCode(exportStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(exportManifest());
hashCode = 31 * hashCode + Objects.hashCode(tableArn());
hashCode = 31 * hashCode + Objects.hashCode(tableId());
hashCode = 31 * hashCode + Objects.hashCode(exportTime());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(s3Bucket());
hashCode = 31 * hashCode + Objects.hashCode(s3BucketOwner());
hashCode = 31 * hashCode + Objects.hashCode(s3Prefix());
hashCode = 31 * hashCode + Objects.hashCode(s3SseAlgorithmAsString());
hashCode = 31 * hashCode + Objects.hashCode(s3SseKmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(failureCode());
hashCode = 31 * hashCode + Objects.hashCode(failureMessage());
hashCode = 31 * hashCode + Objects.hashCode(exportFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(billedSizeBytes());
hashCode = 31 * hashCode + Objects.hashCode(itemCount());
hashCode = 31 * hashCode + Objects.hashCode(exportTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(incrementalExportSpecification());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ExportDescription)) {
return false;
}
ExportDescription other = (ExportDescription) obj;
return Objects.equals(exportArn(), other.exportArn())
&& Objects.equals(exportStatusAsString(), other.exportStatusAsString())
&& Objects.equals(startTime(), other.startTime()) && Objects.equals(endTime(), other.endTime())
&& Objects.equals(exportManifest(), other.exportManifest()) && Objects.equals(tableArn(), other.tableArn())
&& Objects.equals(tableId(), other.tableId()) && Objects.equals(exportTime(), other.exportTime())
&& Objects.equals(clientToken(), other.clientToken()) && Objects.equals(s3Bucket(), other.s3Bucket())
&& Objects.equals(s3BucketOwner(), other.s3BucketOwner()) && Objects.equals(s3Prefix(), other.s3Prefix())
&& Objects.equals(s3SseAlgorithmAsString(), other.s3SseAlgorithmAsString())
&& Objects.equals(s3SseKmsKeyId(), other.s3SseKmsKeyId()) && Objects.equals(failureCode(), other.failureCode())
&& Objects.equals(failureMessage(), other.failureMessage())
&& Objects.equals(exportFormatAsString(), other.exportFormatAsString())
&& Objects.equals(billedSizeBytes(), other.billedSizeBytes()) && Objects.equals(itemCount(), other.itemCount())
&& Objects.equals(exportTypeAsString(), other.exportTypeAsString())
&& Objects.equals(incrementalExportSpecification(), other.incrementalExportSpecification());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ExportDescription").add("ExportArn", exportArn()).add("ExportStatus", exportStatusAsString())
.add("StartTime", startTime()).add("EndTime", endTime()).add("ExportManifest", exportManifest())
.add("TableArn", tableArn()).add("TableId", tableId()).add("ExportTime", exportTime())
.add("ClientToken", clientToken()).add("S3Bucket", s3Bucket()).add("S3BucketOwner", s3BucketOwner())
.add("S3Prefix", s3Prefix()).add("S3SseAlgorithm", s3SseAlgorithmAsString())
.add("S3SseKmsKeyId", s3SseKmsKeyId()).add("FailureCode", failureCode()).add("FailureMessage", failureMessage())
.add("ExportFormat", exportFormatAsString()).add("BilledSizeBytes", billedSizeBytes())
.add("ItemCount", itemCount()).add("ExportType", exportTypeAsString())
.add("IncrementalExportSpecification", incrementalExportSpecification()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ExportArn":
return Optional.ofNullable(clazz.cast(exportArn()));
case "ExportStatus":
return Optional.ofNullable(clazz.cast(exportStatusAsString()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "ExportManifest":
return Optional.ofNullable(clazz.cast(exportManifest()));
case "TableArn":
return Optional.ofNullable(clazz.cast(tableArn()));
case "TableId":
return Optional.ofNullable(clazz.cast(tableId()));
case "ExportTime":
return Optional.ofNullable(clazz.cast(exportTime()));
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "S3Bucket":
return Optional.ofNullable(clazz.cast(s3Bucket()));
case "S3BucketOwner":
return Optional.ofNullable(clazz.cast(s3BucketOwner()));
case "S3Prefix":
return Optional.ofNullable(clazz.cast(s3Prefix()));
case "S3SseAlgorithm":
return Optional.ofNullable(clazz.cast(s3SseAlgorithmAsString()));
case "S3SseKmsKeyId":
return Optional.ofNullable(clazz.cast(s3SseKmsKeyId()));
case "FailureCode":
return Optional.ofNullable(clazz.cast(failureCode()));
case "FailureMessage":
return Optional.ofNullable(clazz.cast(failureMessage()));
case "ExportFormat":
return Optional.ofNullable(clazz.cast(exportFormatAsString()));
case "BilledSizeBytes":
return Optional.ofNullable(clazz.cast(billedSizeBytes()));
case "ItemCount":
return Optional.ofNullable(clazz.cast(itemCount()));
case "ExportType":
return Optional.ofNullable(clazz.cast(exportTypeAsString()));
case "IncrementalExportSpecification":
return Optional.ofNullable(clazz.cast(incrementalExportSpecification()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function