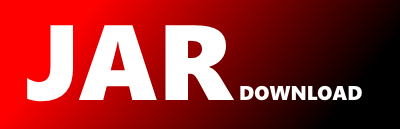
software.amazon.awssdk.services.ebs.EbsAsyncClient Maven / Gradle / Ivy
Show all versions of ebs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ebs;
import java.nio.file.Path;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.services.ebs.model.CompleteSnapshotRequest;
import software.amazon.awssdk.services.ebs.model.CompleteSnapshotResponse;
import software.amazon.awssdk.services.ebs.model.GetSnapshotBlockRequest;
import software.amazon.awssdk.services.ebs.model.GetSnapshotBlockResponse;
import software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest;
import software.amazon.awssdk.services.ebs.model.ListChangedBlocksResponse;
import software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest;
import software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksResponse;
import software.amazon.awssdk.services.ebs.model.PutSnapshotBlockRequest;
import software.amazon.awssdk.services.ebs.model.PutSnapshotBlockResponse;
import software.amazon.awssdk.services.ebs.model.StartSnapshotRequest;
import software.amazon.awssdk.services.ebs.model.StartSnapshotResponse;
import software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksPublisher;
import software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksPublisher;
/**
* Service client for accessing Amazon EBS asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* You can use the Amazon Elastic Block Store (Amazon EBS) direct APIs to create Amazon EBS snapshots, write data
* directly to your snapshots, read data on your snapshots, and identify the differences or changes between two
* snapshots. If you’re an independent software vendor (ISV) who offers backup services for Amazon EBS, the EBS direct
* APIs make it more efficient and cost-effective to track incremental changes on your Amazon EBS volumes through
* snapshots. This can be done without having to create new volumes from snapshots, and then use Amazon Elastic Compute
* Cloud (Amazon EC2) instances to compare the differences.
*
*
* You can create incremental snapshots directly from data on-premises into volumes and the cloud to use for quick
* disaster recovery. With the ability to write and read snapshots, you can write your on-premises data to an snapshot
* during a disaster. Then after recovery, you can restore it back to Amazon Web Services or on-premises from the
* snapshot. You no longer need to build and maintain complex mechanisms to copy data to and from Amazon EBS.
*
*
* This API reference provides detailed information about the actions, data types, parameters, and errors of the EBS
* direct APIs. For more information about the elements that make up the EBS direct APIs, and examples of how to use
* them effectively, see Accessing the Contents of an
* Amazon EBS Snapshot in the Amazon Elastic Compute Cloud User Guide. For more information about the
* supported Amazon Web Services Regions, endpoints, and service quotas for the EBS direct APIs, see Amazon Elastic Block Store Endpoints and
* Quotas in the Amazon Web Services General Reference.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface EbsAsyncClient extends SdkClient {
String SERVICE_NAME = "ebs";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "ebs";
/**
* Create a {@link EbsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static EbsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link EbsAsyncClient}.
*/
static EbsAsyncClientBuilder builder() {
return new DefaultEbsAsyncClientBuilder();
}
/**
*
* Seals and completes the snapshot after all of the required blocks of data have been written to it. Completing the
* snapshot changes the status to completed
. You cannot write new blocks to a snapshot after it has
* been completed.
*
*
* @param completeSnapshotRequest
* @return A Java Future containing the result of the CompleteSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.CompleteSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture completeSnapshot(CompleteSnapshotRequest completeSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Seals and completes the snapshot after all of the required blocks of data have been written to it. Completing the
* snapshot changes the status to completed
. You cannot write new blocks to a snapshot after it has
* been completed.
*
*
*
* This is a convenience which creates an instance of the {@link CompleteSnapshotRequest.Builder} avoiding the need
* to create one manually via {@link CompleteSnapshotRequest#builder()}
*
*
* @param completeSnapshotRequest
* A {@link Consumer} that will call methods on {@link CompleteSnapshotRequest.Builder} to create a request.
* @return A Java Future containing the result of the CompleteSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.CompleteSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture completeSnapshot(
Consumer completeSnapshotRequest) {
return completeSnapshot(CompleteSnapshotRequest.builder().applyMutation(completeSnapshotRequest).build());
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
* @param getSnapshotBlockRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The data content of the block.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.GetSnapshotBlock
* @see AWS API
* Documentation
*/
default CompletableFuture getSnapshotBlock(GetSnapshotBlockRequest getSnapshotBlockRequest,
AsyncResponseTransformer asyncResponseTransformer) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* This is a convenience which creates an instance of the {@link GetSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link GetSnapshotBlockRequest#builder()}
*
*
* @param getSnapshotBlockRequest
* A {@link Consumer} that will call methods on {@link GetSnapshotBlockRequest.Builder} to create a request.
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The data content of the block.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.GetSnapshotBlock
* @see AWS API
* Documentation
*/
default CompletableFuture getSnapshotBlock(
Consumer getSnapshotBlockRequest,
AsyncResponseTransformer asyncResponseTransformer) {
return getSnapshotBlock(GetSnapshotBlockRequest.builder().applyMutation(getSnapshotBlockRequest).build(),
asyncResponseTransformer);
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
* @param getSnapshotBlockRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The data content of the block.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.GetSnapshotBlock
* @see AWS API
* Documentation
*/
default CompletableFuture getSnapshotBlock(GetSnapshotBlockRequest getSnapshotBlockRequest,
Path destinationPath) {
return getSnapshotBlock(getSnapshotBlockRequest, AsyncResponseTransformer.toFile(destinationPath));
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* This is a convenience which creates an instance of the {@link GetSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link GetSnapshotBlockRequest#builder()}
*
*
* @param getSnapshotBlockRequest
* A {@link Consumer} that will call methods on {@link GetSnapshotBlockRequest.Builder} to create a request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The data content of the block.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.GetSnapshotBlock
* @see AWS API
* Documentation
*/
default CompletableFuture getSnapshotBlock(
Consumer getSnapshotBlockRequest, Path destinationPath) {
return getSnapshotBlock(GetSnapshotBlockRequest.builder().applyMutation(getSnapshotBlockRequest).build(), destinationPath);
}
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
* @param listChangedBlocksRequest
* @return A Java Future containing the result of the ListChangedBlocks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.ListChangedBlocks
* @see AWS API
* Documentation
*/
default CompletableFuture listChangedBlocks(ListChangedBlocksRequest listChangedBlocksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
*
* This is a convenience which creates an instance of the {@link ListChangedBlocksRequest.Builder} avoiding the need
* to create one manually via {@link ListChangedBlocksRequest#builder()}
*
*
* @param listChangedBlocksRequest
* A {@link Consumer} that will call methods on {@link ListChangedBlocksRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListChangedBlocks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.ListChangedBlocks
* @see AWS API
* Documentation
*/
default CompletableFuture listChangedBlocks(
Consumer listChangedBlocksRequest) {
return listChangedBlocks(ListChangedBlocksRequest.builder().applyMutation(listChangedBlocksRequest).build());
}
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
*
* This is a variant of
* {@link #listChangedBlocks(software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksPublisher publisher = client.listChangedBlocksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksPublisher publisher = client.listChangedBlocksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ebs.model.ListChangedBlocksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChangedBlocks(software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest)} operation.
*
*
* @param listChangedBlocksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.ListChangedBlocks
* @see AWS API
* Documentation
*/
default ListChangedBlocksPublisher listChangedBlocksPaginator(ListChangedBlocksRequest listChangedBlocksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
*
* This is a variant of
* {@link #listChangedBlocks(software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksPublisher publisher = client.listChangedBlocksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksPublisher publisher = client.listChangedBlocksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ebs.model.ListChangedBlocksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChangedBlocks(software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListChangedBlocksRequest.Builder} avoiding the need
* to create one manually via {@link ListChangedBlocksRequest#builder()}
*
*
* @param listChangedBlocksRequest
* A {@link Consumer} that will call methods on {@link ListChangedBlocksRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.ListChangedBlocks
* @see AWS API
* Documentation
*/
default ListChangedBlocksPublisher listChangedBlocksPaginator(
Consumer listChangedBlocksRequest) {
return listChangedBlocksPaginator(ListChangedBlocksRequest.builder().applyMutation(listChangedBlocksRequest).build());
}
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
* @param listSnapshotBlocksRequest
* @return A Java Future containing the result of the ListSnapshotBlocks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
default CompletableFuture listSnapshotBlocks(ListSnapshotBlocksRequest listSnapshotBlocksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
*
* This is a convenience which creates an instance of the {@link ListSnapshotBlocksRequest.Builder} avoiding the
* need to create one manually via {@link ListSnapshotBlocksRequest#builder()}
*
*
* @param listSnapshotBlocksRequest
* A {@link Consumer} that will call methods on {@link ListSnapshotBlocksRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListSnapshotBlocks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
default CompletableFuture listSnapshotBlocks(
Consumer listSnapshotBlocksRequest) {
return listSnapshotBlocks(ListSnapshotBlocksRequest.builder().applyMutation(listSnapshotBlocksRequest).build());
}
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
*
* This is a variant of
* {@link #listSnapshotBlocks(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksPublisher publisher = client.listSnapshotBlocksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksPublisher publisher = client.listSnapshotBlocksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSnapshotBlocks(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest)} operation.
*
*
* @param listSnapshotBlocksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
default ListSnapshotBlocksPublisher listSnapshotBlocksPaginator(ListSnapshotBlocksRequest listSnapshotBlocksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
*
* This is a variant of
* {@link #listSnapshotBlocks(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksPublisher publisher = client.listSnapshotBlocksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksPublisher publisher = client.listSnapshotBlocksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSnapshotBlocks(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListSnapshotBlocksRequest.Builder} avoiding the
* need to create one manually via {@link ListSnapshotBlocksRequest#builder()}
*
*
* @param listSnapshotBlocksRequest
* A {@link Consumer} that will call methods on {@link ListSnapshotBlocksRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
default ListSnapshotBlocksPublisher listSnapshotBlocksPaginator(
Consumer listSnapshotBlocksRequest) {
return listSnapshotBlocksPaginator(ListSnapshotBlocksRequest.builder().applyMutation(listSnapshotBlocksRequest).build());
}
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
* @param putSnapshotBlockRequest
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* The data to write to the block.
*
*
* The block data is not signed as part of the Signature Version 4 signing process. As a result, you must
* generate and provide a Base64-encoded SHA256 checksum for the block data using the x-amz-Checksum
* header. Also, you must specify the checksum algorithm using the x-amz-Checksum-Algorithm header.
* The checksum that you provide is part of the Signature Version 4 signing process. It is validated against
* a checksum generated by Amazon EBS to ensure the validity and authenticity of the data. If the checksums
* do not correspond, the request fails. For more information, see
* Using checksums with the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* '
* @return A Java Future containing the result of the PutSnapshotBlock operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.PutSnapshotBlock
* @see AWS API
* Documentation
*/
default CompletableFuture putSnapshotBlock(PutSnapshotBlockRequest putSnapshotBlockRequest,
AsyncRequestBody requestBody) {
throw new UnsupportedOperationException();
}
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
*
* This is a convenience which creates an instance of the {@link PutSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link PutSnapshotBlockRequest#builder()}
*
*
* @param putSnapshotBlockRequest
* A {@link Consumer} that will call methods on {@link PutSnapshotBlockRequest.Builder} to create a request.
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* The data to write to the block.
*
*
* The block data is not signed as part of the Signature Version 4 signing process. As a result, you must
* generate and provide a Base64-encoded SHA256 checksum for the block data using the x-amz-Checksum
* header. Also, you must specify the checksum algorithm using the x-amz-Checksum-Algorithm header.
* The checksum that you provide is part of the Signature Version 4 signing process. It is validated against
* a checksum generated by Amazon EBS to ensure the validity and authenticity of the data. If the checksums
* do not correspond, the request fails. For more information, see
* Using checksums with the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* '
* @return A Java Future containing the result of the PutSnapshotBlock operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.PutSnapshotBlock
* @see AWS API
* Documentation
*/
default CompletableFuture putSnapshotBlock(
Consumer putSnapshotBlockRequest, AsyncRequestBody requestBody) {
return putSnapshotBlock(PutSnapshotBlockRequest.builder().applyMutation(putSnapshotBlockRequest).build(), requestBody);
}
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
* @param putSnapshotBlockRequest
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The data to write to the block.
*
*
* The block data is not signed as part of the Signature Version 4 signing process. As a result, you must
* generate and provide a Base64-encoded SHA256 checksum for the block data using the x-amz-Checksum
* header. Also, you must specify the checksum algorithm using the x-amz-Checksum-Algorithm header.
* The checksum that you provide is part of the Signature Version 4 signing process. It is validated against
* a checksum generated by Amazon EBS to ensure the validity and authenticity of the data. If the checksums
* do not correspond, the request fails. For more information, see
* Using checksums with the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* '
* @return A Java Future containing the result of the PutSnapshotBlock operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.PutSnapshotBlock
* @see AWS API
* Documentation
*/
default CompletableFuture putSnapshotBlock(PutSnapshotBlockRequest putSnapshotBlockRequest,
Path sourcePath) {
return putSnapshotBlock(putSnapshotBlockRequest, AsyncRequestBody.fromFile(sourcePath));
}
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
*
* This is a convenience which creates an instance of the {@link PutSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link PutSnapshotBlockRequest#builder()}
*
*
* @param putSnapshotBlockRequest
* A {@link Consumer} that will call methods on {@link PutSnapshotBlockRequest.Builder} to create a request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The data to write to the block.
*
*
* The block data is not signed as part of the Signature Version 4 signing process. As a result, you must
* generate and provide a Base64-encoded SHA256 checksum for the block data using the x-amz-Checksum
* header. Also, you must specify the checksum algorithm using the x-amz-Checksum-Algorithm header.
* The checksum that you provide is part of the Signature Version 4 signing process. It is validated against
* a checksum generated by Amazon EBS to ensure the validity and authenticity of the data. If the checksums
* do not correspond, the request fails. For more information, see
* Using checksums with the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* '
* @return A Java Future containing the result of the PutSnapshotBlock operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - ResourceNotFoundException The specified resource does not exist.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.PutSnapshotBlock
* @see AWS API
* Documentation
*/
default CompletableFuture putSnapshotBlock(
Consumer putSnapshotBlockRequest, Path sourcePath) {
return putSnapshotBlock(PutSnapshotBlockRequest.builder().applyMutation(putSnapshotBlockRequest).build(), sourcePath);
}
/**
*
* Creates a new Amazon EBS snapshot. The new snapshot enters the pending
state after the request
* completes.
*
*
* After creating the snapshot, use PutSnapshotBlock to
* write blocks of data to the snapshot.
*
*
* @param startSnapshotRequest
* @return A Java Future containing the result of the StartSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ResourceNotFoundException The specified resource does not exist.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - ConcurrentLimitExceededException You have reached the limit for concurrent API requests. For more
* information, see Optimizing performance of the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* - ConflictException The request uses the same client token as a previous, but non-identical request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.StartSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture startSnapshot(StartSnapshotRequest startSnapshotRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Amazon EBS snapshot. The new snapshot enters the pending
state after the request
* completes.
*
*
* After creating the snapshot, use PutSnapshotBlock to
* write blocks of data to the snapshot.
*
*
*
* This is a convenience which creates an instance of the {@link StartSnapshotRequest.Builder} avoiding the need to
* create one manually via {@link StartSnapshotRequest#builder()}
*
*
* @param startSnapshotRequest
* A {@link Consumer} that will call methods on {@link StartSnapshotRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartSnapshot operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException The input fails to satisfy the constraints of the EBS direct APIs.
* - RequestThrottledException The number of API requests has exceed the maximum allowed API request
* throttling limit.
* - ResourceNotFoundException The specified resource does not exist.
* - ServiceQuotaExceededException Your current service quotas do not allow you to perform this action.
* - InternalServerException An internal error has occurred.
* - ConcurrentLimitExceededException You have reached the limit for concurrent API requests. For more
* information, see Optimizing performance of the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* - ConflictException The request uses the same client token as a previous, but non-identical request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EbsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EbsAsyncClient.StartSnapshot
* @see AWS API
* Documentation
*/
default CompletableFuture startSnapshot(Consumer startSnapshotRequest) {
return startSnapshot(StartSnapshotRequest.builder().applyMutation(startSnapshotRequest).build());
}
}