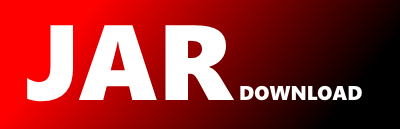
software.amazon.awssdk.services.ebs.DefaultEbsClient Maven / Gradle / Ivy
Show all versions of ebs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ebs;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.auth.signer.Aws4UnsignedPayloadSigner;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.runtime.transform.StreamingRequestMarshaller;
import software.amazon.awssdk.core.signer.Signer;
import software.amazon.awssdk.core.sync.RequestBody;
import software.amazon.awssdk.core.sync.ResponseTransformer;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.ebs.model.AccessDeniedException;
import software.amazon.awssdk.services.ebs.model.CompleteSnapshotRequest;
import software.amazon.awssdk.services.ebs.model.CompleteSnapshotResponse;
import software.amazon.awssdk.services.ebs.model.ConcurrentLimitExceededException;
import software.amazon.awssdk.services.ebs.model.ConflictException;
import software.amazon.awssdk.services.ebs.model.EbsException;
import software.amazon.awssdk.services.ebs.model.EbsRequest;
import software.amazon.awssdk.services.ebs.model.GetSnapshotBlockRequest;
import software.amazon.awssdk.services.ebs.model.GetSnapshotBlockResponse;
import software.amazon.awssdk.services.ebs.model.InternalServerException;
import software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest;
import software.amazon.awssdk.services.ebs.model.ListChangedBlocksResponse;
import software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest;
import software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksResponse;
import software.amazon.awssdk.services.ebs.model.PutSnapshotBlockRequest;
import software.amazon.awssdk.services.ebs.model.PutSnapshotBlockResponse;
import software.amazon.awssdk.services.ebs.model.RequestThrottledException;
import software.amazon.awssdk.services.ebs.model.ResourceNotFoundException;
import software.amazon.awssdk.services.ebs.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.ebs.model.StartSnapshotRequest;
import software.amazon.awssdk.services.ebs.model.StartSnapshotResponse;
import software.amazon.awssdk.services.ebs.model.ValidationException;
import software.amazon.awssdk.services.ebs.transform.CompleteSnapshotRequestMarshaller;
import software.amazon.awssdk.services.ebs.transform.GetSnapshotBlockRequestMarshaller;
import software.amazon.awssdk.services.ebs.transform.ListChangedBlocksRequestMarshaller;
import software.amazon.awssdk.services.ebs.transform.ListSnapshotBlocksRequestMarshaller;
import software.amazon.awssdk.services.ebs.transform.PutSnapshotBlockRequestMarshaller;
import software.amazon.awssdk.services.ebs.transform.StartSnapshotRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link EbsClient}.
*
* @see EbsClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultEbsClient implements EbsClient {
private static final Logger log = Logger.loggerFor(DefaultEbsClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final EbsServiceClientConfiguration serviceClientConfiguration;
protected DefaultEbsClient(EbsServiceClientConfiguration serviceClientConfiguration,
SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.serviceClientConfiguration = serviceClientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Seals and completes the snapshot after all of the required blocks of data have been written to it. Completing the
* snapshot changes the status to completed
. You cannot write new blocks to a snapshot after it has
* been completed.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param completeSnapshotRequest
* @return Result of the CompleteSnapshot operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.CompleteSnapshot
* @see AWS API
* Documentation
*/
@Override
public CompleteSnapshotResponse completeSnapshot(CompleteSnapshotRequest completeSnapshotRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CompleteSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, completeSnapshotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EBS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CompleteSnapshot");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CompleteSnapshot").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(completeSnapshotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CompleteSnapshotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param getSnapshotBlockRequest
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled
* GetSnapshotBlockResponse and an InputStream to the response content are provided as parameters to the
* callback. The callback may return a transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The data content of the block.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.GetSnapshotBlock
* @see AWS API
* Documentation
*/
@Override
public ReturnT getSnapshotBlock(GetSnapshotBlockRequest getSnapshotBlockRequest,
ResponseTransformer responseTransformer) throws AccessDeniedException,
ValidationException, ResourceNotFoundException, RequestThrottledException, ServiceQuotaExceededException,
InternalServerException, AwsServiceException, SdkClientException, EbsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(true)
.isPayloadJson(false).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetSnapshotBlockResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSnapshotBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EBS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSnapshotBlock");
return clientHandler.execute(
new ClientExecutionParams()
.withOperationName("GetSnapshotBlock").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getSnapshotBlockRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetSnapshotBlockRequestMarshaller(protocolFactory)), responseTransformer);
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param listChangedBlocksRequest
* @return Result of the ListChangedBlocks operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListChangedBlocks
* @see AWS API
* Documentation
*/
@Override
public ListChangedBlocksResponse listChangedBlocks(ListChangedBlocksRequest listChangedBlocksRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListChangedBlocksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listChangedBlocksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EBS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListChangedBlocks");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListChangedBlocks").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listChangedBlocksRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListChangedBlocksRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param listSnapshotBlocksRequest
* @return Result of the ListSnapshotBlocks operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
@Override
public ListSnapshotBlocksResponse listSnapshotBlocks(ListSnapshotBlocksRequest listSnapshotBlocksRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSnapshotBlocksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSnapshotBlocksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EBS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSnapshotBlocks");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListSnapshotBlocks").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listSnapshotBlocksRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSnapshotBlocksRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param putSnapshotBlockRequest
* @param requestBody
* The content to send to the service. A {@link RequestBody} can be created using one of several factory
* methods for various sources of data. For example, to create a request body from a file you can do the
* following.
*
*
* {@code RequestBody.fromFile(new File("myfile.txt"))}
*
*
* See documentation in {@link RequestBody} for additional details and which sources of data are supported.
* The service documentation for the request content is as follows '
*
* The data to write to the block.
*
*
* The block data is not signed as part of the Signature Version 4 signing process. As a result, you must
* generate and provide a Base64-encoded SHA256 checksum for the block data using the x-amz-Checksum
* header. Also, you must specify the checksum algorithm using the x-amz-Checksum-Algorithm header.
* The checksum that you provide is part of the Signature Version 4 signing process. It is validated against
* a checksum generated by Amazon EBS to ensure the validity and authenticity of the data. If the checksums
* do not correspond, the request fails. For more information, see
* Using checksums with the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* '
* @return Result of the PutSnapshotBlock operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.PutSnapshotBlock
* @see AWS API
* Documentation
*/
@Override
public PutSnapshotBlockResponse putSnapshotBlock(PutSnapshotBlockRequest putSnapshotBlockRequest, RequestBody requestBody)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
putSnapshotBlockRequest = applySignerOverride(putSnapshotBlockRequest, Aws4UnsignedPayloadSigner.create());
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutSnapshotBlockResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putSnapshotBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EBS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutSnapshotBlock");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutSnapshotBlock")
.withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler)
.withInput(putSnapshotBlockRequest)
.withMetricCollector(apiCallMetricCollector)
.withRequestBody(requestBody)
.withMarshaller(
StreamingRequestMarshaller.builder()
.delegateMarshaller(new PutSnapshotBlockRequestMarshaller(protocolFactory))
.requestBody(requestBody).transferEncoding(true).build()));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new Amazon EBS snapshot. The new snapshot enters the pending
state after the request
* completes.
*
*
* After creating the snapshot, use PutSnapshotBlock to
* write blocks of data to the snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param startSnapshotRequest
* @return Result of the StartSnapshot operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws ConcurrentLimitExceededException
* You have reached the limit for concurrent API requests. For more information, see Optimizing performance of the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
* @throws ConflictException
* The request uses the same client token as a previous, but non-identical request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.StartSnapshot
* @see AWS API
* Documentation
*/
@Override
public StartSnapshotResponse startSnapshot(StartSnapshotRequest startSnapshotRequest) throws AccessDeniedException,
ValidationException, RequestThrottledException, ResourceNotFoundException, ServiceQuotaExceededException,
InternalServerException, ConcurrentLimitExceededException, ConflictException, AwsServiceException,
SdkClientException, EbsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartSnapshotResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startSnapshotRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EBS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartSnapshot");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StartSnapshot").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startSnapshotRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartSnapshotRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private T applySignerOverride(T request, Signer signer) {
if (request.overrideConfiguration().flatMap(c -> c.signer()).isPresent()) {
return request;
}
Consumer signerOverride = b -> b.signer(signer).build();
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(signerOverride).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(signerOverride).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(EbsException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RequestThrottledException")
.exceptionBuilderSupplier(RequestThrottledException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConcurrentLimitExceededException")
.exceptionBuilderSupplier(ConcurrentLimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build());
}
@Override
public final EbsServiceClientConfiguration serviceClientConfiguration() {
return this.serviceClientConfiguration;
}
@Override
public void close() {
clientHandler.close();
}
}