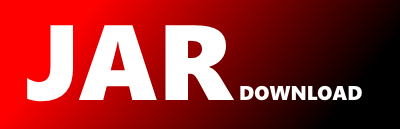
software.amazon.awssdk.services.ebs.EbsClient Maven / Gradle / Ivy
Show all versions of ebs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ebs;
import java.nio.file.Path;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ResponseBytes;
import software.amazon.awssdk.core.ResponseInputStream;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.sync.RequestBody;
import software.amazon.awssdk.core.sync.ResponseTransformer;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.ebs.model.AccessDeniedException;
import software.amazon.awssdk.services.ebs.model.CompleteSnapshotRequest;
import software.amazon.awssdk.services.ebs.model.CompleteSnapshotResponse;
import software.amazon.awssdk.services.ebs.model.ConcurrentLimitExceededException;
import software.amazon.awssdk.services.ebs.model.ConflictException;
import software.amazon.awssdk.services.ebs.model.EbsException;
import software.amazon.awssdk.services.ebs.model.GetSnapshotBlockRequest;
import software.amazon.awssdk.services.ebs.model.GetSnapshotBlockResponse;
import software.amazon.awssdk.services.ebs.model.InternalServerException;
import software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest;
import software.amazon.awssdk.services.ebs.model.ListChangedBlocksResponse;
import software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest;
import software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksResponse;
import software.amazon.awssdk.services.ebs.model.PutSnapshotBlockRequest;
import software.amazon.awssdk.services.ebs.model.PutSnapshotBlockResponse;
import software.amazon.awssdk.services.ebs.model.RequestThrottledException;
import software.amazon.awssdk.services.ebs.model.ResourceNotFoundException;
import software.amazon.awssdk.services.ebs.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.ebs.model.StartSnapshotRequest;
import software.amazon.awssdk.services.ebs.model.StartSnapshotResponse;
import software.amazon.awssdk.services.ebs.model.ValidationException;
import software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksIterable;
import software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksIterable;
/**
* Service client for accessing Amazon EBS. This can be created using the static {@link #builder()} method.
*
*
* You can use the Amazon Elastic Block Store (Amazon EBS) direct APIs to create Amazon EBS snapshots, write data
* directly to your snapshots, read data on your snapshots, and identify the differences or changes between two
* snapshots. If you’re an independent software vendor (ISV) who offers backup services for Amazon EBS, the EBS direct
* APIs make it more efficient and cost-effective to track incremental changes on your Amazon EBS volumes through
* snapshots. This can be done without having to create new volumes from snapshots, and then use Amazon Elastic Compute
* Cloud (Amazon EC2) instances to compare the differences.
*
*
* You can create incremental snapshots directly from data on-premises into volumes and the cloud to use for quick
* disaster recovery. With the ability to write and read snapshots, you can write your on-premises data to an snapshot
* during a disaster. Then after recovery, you can restore it back to Amazon Web Services or on-premises from the
* snapshot. You no longer need to build and maintain complex mechanisms to copy data to and from Amazon EBS.
*
*
* This API reference provides detailed information about the actions, data types, parameters, and errors of the EBS
* direct APIs. For more information about the elements that make up the EBS direct APIs, and examples of how to use
* them effectively, see Accessing the Contents of an
* Amazon EBS Snapshot in the Amazon Elastic Compute Cloud User Guide. For more information about the
* supported Amazon Web Services Regions, endpoints, and service quotas for the EBS direct APIs, see Amazon Elastic Block Store Endpoints and
* Quotas in the Amazon Web Services General Reference.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface EbsClient extends AwsClient {
String SERVICE_NAME = "ebs";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "ebs";
/**
*
* Seals and completes the snapshot after all of the required blocks of data have been written to it. Completing the
* snapshot changes the status to completed
. You cannot write new blocks to a snapshot after it has
* been completed.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param completeSnapshotRequest
* @return Result of the CompleteSnapshot operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.CompleteSnapshot
* @see AWS API
* Documentation
*/
default CompleteSnapshotResponse completeSnapshot(CompleteSnapshotRequest completeSnapshotRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
throw new UnsupportedOperationException();
}
/**
*
* Seals and completes the snapshot after all of the required blocks of data have been written to it. Completing the
* snapshot changes the status to completed
. You cannot write new blocks to a snapshot after it has
* been completed.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CompleteSnapshotRequest.Builder} avoiding the need
* to create one manually via {@link CompleteSnapshotRequest#builder()}
*
*
* @param completeSnapshotRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.CompleteSnapshotRequest.Builder} to create a request.
* @return Result of the CompleteSnapshot operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.CompleteSnapshot
* @see AWS API
* Documentation
*/
default CompleteSnapshotResponse completeSnapshot(Consumer completeSnapshotRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
return completeSnapshot(CompleteSnapshotRequest.builder().applyMutation(completeSnapshotRequest).build());
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param getSnapshotBlockRequest
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled
* GetSnapshotBlockResponse and an InputStream to the response content are provided as parameters to the
* callback. The callback may return a transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The data content of the block.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.GetSnapshotBlock
* @see AWS API
* Documentation
*/
default ReturnT getSnapshotBlock(GetSnapshotBlockRequest getSnapshotBlockRequest,
ResponseTransformer responseTransformer) throws AccessDeniedException,
ValidationException, ResourceNotFoundException, RequestThrottledException, ServiceQuotaExceededException,
InternalServerException, AwsServiceException, SdkClientException, EbsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link GetSnapshotBlockRequest#builder()}
*
*
* @param getSnapshotBlockRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.GetSnapshotBlockRequest.Builder} to create a request.
* @param responseTransformer
* Functional interface for processing the streamed response content. The unmarshalled
* GetSnapshotBlockResponse and an InputStream to the response content are provided as parameters to the
* callback. The callback may return a transformed type which will be the return value of this method. See
* {@link software.amazon.awssdk.core.sync.ResponseTransformer} for details on implementing this interface
* and for links to pre-canned implementations for common scenarios like downloading to a file. The service
* documentation for the response content is as follows '
*
* The data content of the block.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.GetSnapshotBlock
* @see AWS API
* Documentation
*/
default ReturnT getSnapshotBlock(Consumer getSnapshotBlockRequest,
ResponseTransformer responseTransformer) throws AccessDeniedException,
ValidationException, ResourceNotFoundException, RequestThrottledException, ServiceQuotaExceededException,
InternalServerException, AwsServiceException, SdkClientException, EbsException {
return getSnapshotBlock(GetSnapshotBlockRequest.builder().applyMutation(getSnapshotBlockRequest).build(),
responseTransformer);
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param getSnapshotBlockRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The data content of the block.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.GetSnapshotBlock
* @see #getSnapshotBlock(GetSnapshotBlockRequest, ResponseTransformer)
* @see AWS API
* Documentation
*/
default GetSnapshotBlockResponse getSnapshotBlock(GetSnapshotBlockRequest getSnapshotBlockRequest, Path destinationPath)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
return getSnapshotBlock(getSnapshotBlockRequest, ResponseTransformer.toFile(destinationPath));
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link GetSnapshotBlockRequest#builder()}
*
*
* @param getSnapshotBlockRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.GetSnapshotBlockRequest.Builder} to create a request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The data content of the block.
*
* '.
* @return The transformed result of the ResponseTransformer.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.GetSnapshotBlock
* @see #getSnapshotBlock(GetSnapshotBlockRequest, ResponseTransformer)
* @see AWS API
* Documentation
*/
default GetSnapshotBlockResponse getSnapshotBlock(Consumer getSnapshotBlockRequest,
Path destinationPath) throws AccessDeniedException, ValidationException, ResourceNotFoundException,
RequestThrottledException, ServiceQuotaExceededException, InternalServerException, AwsServiceException,
SdkClientException, EbsException {
return getSnapshotBlock(GetSnapshotBlockRequest.builder().applyMutation(getSnapshotBlockRequest).build(), destinationPath);
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param getSnapshotBlockRequest
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The data content of the block.
*
* '.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.GetSnapshotBlock
* @see #getObject(getSnapshotBlock, ResponseTransformer)
* @see AWS API
* Documentation
*/
default ResponseInputStream getSnapshotBlock(GetSnapshotBlockRequest getSnapshotBlockRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
return getSnapshotBlock(getSnapshotBlockRequest, ResponseTransformer.toInputStream());
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link GetSnapshotBlockRequest#builder()}
*
*
* @param getSnapshotBlockRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.GetSnapshotBlockRequest.Builder} to create a request.
* @return A {@link ResponseInputStream} containing data streamed from service. Note that this is an unmanaged
* reference to the underlying HTTP connection so great care must be taken to ensure all data if fully read
* from the input stream and that it is properly closed. Failure to do so may result in sub-optimal behavior
* and exhausting connections in the connection pool. The unmarshalled response object can be obtained via
* {@link ResponseInputStream#response()}. The service documentation for the response content is as follows
* '
*
* The data content of the block.
*
* '.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.GetSnapshotBlock
* @see #getObject(getSnapshotBlock, ResponseTransformer)
* @see AWS API
* Documentation
*/
default ResponseInputStream getSnapshotBlock(
Consumer getSnapshotBlockRequest) throws AccessDeniedException, ValidationException,
ResourceNotFoundException, RequestThrottledException, ServiceQuotaExceededException, InternalServerException,
AwsServiceException, SdkClientException, EbsException {
return getSnapshotBlock(GetSnapshotBlockRequest.builder().applyMutation(getSnapshotBlockRequest).build());
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param getSnapshotBlockRequest
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The data content of the block.
*
* '.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.GetSnapshotBlock
* @see #getObject(getSnapshotBlock, ResponseTransformer)
* @see AWS API
* Documentation
*/
default ResponseBytes getSnapshotBlockAsBytes(GetSnapshotBlockRequest getSnapshotBlockRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
return getSnapshotBlock(getSnapshotBlockRequest, ResponseTransformer.toBytes());
}
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link GetSnapshotBlockRequest#builder()}
*
*
* @param getSnapshotBlockRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.GetSnapshotBlockRequest.Builder} to create a request.
* @return A {@link ResponseBytes} that loads the data streamed from the service into memory and exposes it in
* convenient in-memory representations like a byte buffer or string. The unmarshalled response object can
* be obtained via {@link ResponseBytes#response()}. The service documentation for the response content is
* as follows '
*
* The data content of the block.
*
* '.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.GetSnapshotBlock
* @see #getObject(getSnapshotBlock, ResponseTransformer)
* @see AWS API
* Documentation
*/
default ResponseBytes getSnapshotBlockAsBytes(
Consumer getSnapshotBlockRequest) throws AccessDeniedException, ValidationException,
ResourceNotFoundException, RequestThrottledException, ServiceQuotaExceededException, InternalServerException,
AwsServiceException, SdkClientException, EbsException {
return getSnapshotBlockAsBytes(GetSnapshotBlockRequest.builder().applyMutation(getSnapshotBlockRequest).build());
}
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param listChangedBlocksRequest
* @return Result of the ListChangedBlocks operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListChangedBlocks
* @see AWS API
* Documentation
*/
default ListChangedBlocksResponse listChangedBlocks(ListChangedBlocksRequest listChangedBlocksRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListChangedBlocksRequest.Builder} avoiding the need
* to create one manually via {@link ListChangedBlocksRequest#builder()}
*
*
* @param listChangedBlocksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest.Builder} to create a request.
* @return Result of the ListChangedBlocks operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListChangedBlocks
* @see AWS API
* Documentation
*/
default ListChangedBlocksResponse listChangedBlocks(Consumer listChangedBlocksRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
return listChangedBlocks(ListChangedBlocksRequest.builder().applyMutation(listChangedBlocksRequest).build());
}
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a variant of
* {@link #listChangedBlocks(software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksIterable responses = client.listChangedBlocksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksIterable responses = client
* .listChangedBlocksPaginator(request);
* for (software.amazon.awssdk.services.ebs.model.ListChangedBlocksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksIterable responses = client.listChangedBlocksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChangedBlocks(software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest)} operation.
*
*
* @param listChangedBlocksRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListChangedBlocks
* @see AWS API
* Documentation
*/
default ListChangedBlocksIterable listChangedBlocksPaginator(ListChangedBlocksRequest listChangedBlocksRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
return new ListChangedBlocksIterable(this, listChangedBlocksRequest);
}
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a variant of
* {@link #listChangedBlocks(software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksIterable responses = client.listChangedBlocksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksIterable responses = client
* .listChangedBlocksPaginator(request);
* for (software.amazon.awssdk.services.ebs.model.ListChangedBlocksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListChangedBlocksIterable responses = client.listChangedBlocksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChangedBlocks(software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListChangedBlocksRequest.Builder} avoiding the need
* to create one manually via {@link ListChangedBlocksRequest#builder()}
*
*
* @param listChangedBlocksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.ListChangedBlocksRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListChangedBlocks
* @see AWS API
* Documentation
*/
default ListChangedBlocksIterable listChangedBlocksPaginator(
Consumer listChangedBlocksRequest) throws AccessDeniedException,
ValidationException, ResourceNotFoundException, RequestThrottledException, ServiceQuotaExceededException,
InternalServerException, AwsServiceException, SdkClientException, EbsException {
return listChangedBlocksPaginator(ListChangedBlocksRequest.builder().applyMutation(listChangedBlocksRequest).build());
}
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param listSnapshotBlocksRequest
* @return Result of the ListSnapshotBlocks operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
default ListSnapshotBlocksResponse listSnapshotBlocks(ListSnapshotBlocksRequest listSnapshotBlocksRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListSnapshotBlocksRequest.Builder} avoiding the
* need to create one manually via {@link ListSnapshotBlocksRequest#builder()}
*
*
* @param listSnapshotBlocksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest.Builder} to create a request.
* @return Result of the ListSnapshotBlocks operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
default ListSnapshotBlocksResponse listSnapshotBlocks(Consumer listSnapshotBlocksRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
return listSnapshotBlocks(ListSnapshotBlocksRequest.builder().applyMutation(listSnapshotBlocksRequest).build());
}
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a variant of
* {@link #listSnapshotBlocks(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksIterable responses = client.listSnapshotBlocksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksIterable responses = client
* .listSnapshotBlocksPaginator(request);
* for (software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksIterable responses = client.listSnapshotBlocksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSnapshotBlocks(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest)} operation.
*
*
* @param listSnapshotBlocksRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
default ListSnapshotBlocksIterable listSnapshotBlocksPaginator(ListSnapshotBlocksRequest listSnapshotBlocksRequest)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
return new ListSnapshotBlocksIterable(this, listSnapshotBlocksRequest);
}
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a variant of
* {@link #listSnapshotBlocks(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksIterable responses = client.listSnapshotBlocksPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksIterable responses = client
* .listSnapshotBlocksPaginator(request);
* for (software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.ebs.paginators.ListSnapshotBlocksIterable responses = client.listSnapshotBlocksPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSnapshotBlocks(software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListSnapshotBlocksRequest.Builder} avoiding the
* need to create one manually via {@link ListSnapshotBlocksRequest#builder()}
*
*
* @param listSnapshotBlocksRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.ListSnapshotBlocksRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
default ListSnapshotBlocksIterable listSnapshotBlocksPaginator(
Consumer listSnapshotBlocksRequest) throws AccessDeniedException,
ValidationException, ResourceNotFoundException, RequestThrottledException, ServiceQuotaExceededException,
InternalServerException, AwsServiceException, SdkClientException, EbsException {
return listSnapshotBlocksPaginator(ListSnapshotBlocksRequest.builder().applyMutation(listSnapshotBlocksRequest).build());
}
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param putSnapshotBlockRequest
* @param requestBody
* The content to send to the service. A {@link RequestBody} can be created using one of several factory
* methods for various sources of data. For example, to create a request body from a file you can do the
* following.
*
*
* {@code RequestBody.fromFile(new File("myfile.txt"))}
*
*
* See documentation in {@link RequestBody} for additional details and which sources of data are supported.
* The service documentation for the request content is as follows '
*
* The data to write to the block.
*
*
* The block data is not signed as part of the Signature Version 4 signing process. As a result, you must
* generate and provide a Base64-encoded SHA256 checksum for the block data using the x-amz-Checksum
* header. Also, you must specify the checksum algorithm using the x-amz-Checksum-Algorithm header.
* The checksum that you provide is part of the Signature Version 4 signing process. It is validated against
* a checksum generated by Amazon EBS to ensure the validity and authenticity of the data. If the checksums
* do not correspond, the request fails. For more information, see
* Using checksums with the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* '
* @return Result of the PutSnapshotBlock operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.PutSnapshotBlock
* @see AWS API
* Documentation
*/
default PutSnapshotBlockResponse putSnapshotBlock(PutSnapshotBlockRequest putSnapshotBlockRequest, RequestBody requestBody)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
throw new UnsupportedOperationException();
}
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link PutSnapshotBlockRequest#builder()}
*
*
* @param putSnapshotBlockRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.PutSnapshotBlockRequest.Builder} to create a request.
* @param requestBody
* The content to send to the service. A {@link RequestBody} can be created using one of several factory
* methods for various sources of data. For example, to create a request body from a file you can do the
* following.
*
*
* {@code RequestBody.fromFile(new File("myfile.txt"))}
*
*
* See documentation in {@link RequestBody} for additional details and which sources of data are supported.
* The service documentation for the request content is as follows '
*
* The data to write to the block.
*
*
* The block data is not signed as part of the Signature Version 4 signing process. As a result, you must
* generate and provide a Base64-encoded SHA256 checksum for the block data using the x-amz-Checksum
* header. Also, you must specify the checksum algorithm using the x-amz-Checksum-Algorithm header.
* The checksum that you provide is part of the Signature Version 4 signing process. It is validated against
* a checksum generated by Amazon EBS to ensure the validity and authenticity of the data. If the checksums
* do not correspond, the request fails. For more information, see
* Using checksums with the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* '
* @return Result of the PutSnapshotBlock operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.PutSnapshotBlock
* @see AWS API
* Documentation
*/
default PutSnapshotBlockResponse putSnapshotBlock(Consumer putSnapshotBlockRequest,
RequestBody requestBody) throws AccessDeniedException, ValidationException, ResourceNotFoundException,
RequestThrottledException, ServiceQuotaExceededException, InternalServerException, AwsServiceException,
SdkClientException, EbsException {
return putSnapshotBlock(PutSnapshotBlockRequest.builder().applyMutation(putSnapshotBlockRequest).build(), requestBody);
}
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param putSnapshotBlockRequest
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The data to write to the block.
*
*
* The block data is not signed as part of the Signature Version 4 signing process. As a result, you must
* generate and provide a Base64-encoded SHA256 checksum for the block data using the x-amz-Checksum
* header. Also, you must specify the checksum algorithm using the x-amz-Checksum-Algorithm header.
* The checksum that you provide is part of the Signature Version 4 signing process. It is validated against
* a checksum generated by Amazon EBS to ensure the validity and authenticity of the data. If the checksums
* do not correspond, the request fails. For more information, see
* Using checksums with the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* '
* @return Result of the PutSnapshotBlock operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.PutSnapshotBlock
* @see #putSnapshotBlock(PutSnapshotBlockRequest, RequestBody)
* @see AWS API
* Documentation
*/
default PutSnapshotBlockResponse putSnapshotBlock(PutSnapshotBlockRequest putSnapshotBlockRequest, Path sourcePath)
throws AccessDeniedException, ValidationException, ResourceNotFoundException, RequestThrottledException,
ServiceQuotaExceededException, InternalServerException, AwsServiceException, SdkClientException, EbsException {
return putSnapshotBlock(putSnapshotBlockRequest, RequestBody.fromFile(sourcePath));
}
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutSnapshotBlockRequest.Builder} avoiding the need
* to create one manually via {@link PutSnapshotBlockRequest#builder()}
*
*
* @param putSnapshotBlockRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.PutSnapshotBlockRequest.Builder} to create a request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* The data to write to the block.
*
*
* The block data is not signed as part of the Signature Version 4 signing process. As a result, you must
* generate and provide a Base64-encoded SHA256 checksum for the block data using the x-amz-Checksum
* header. Also, you must specify the checksum algorithm using the x-amz-Checksum-Algorithm header.
* The checksum that you provide is part of the Signature Version 4 signing process. It is validated against
* a checksum generated by Amazon EBS to ensure the validity and authenticity of the data. If the checksums
* do not correspond, the request fails. For more information, see
* Using checksums with the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
*
* '
* @return Result of the PutSnapshotBlock operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.PutSnapshotBlock
* @see #putSnapshotBlock(PutSnapshotBlockRequest, RequestBody)
* @see AWS API
* Documentation
*/
default PutSnapshotBlockResponse putSnapshotBlock(Consumer putSnapshotBlockRequest,
Path sourcePath) throws AccessDeniedException, ValidationException, ResourceNotFoundException,
RequestThrottledException, ServiceQuotaExceededException, InternalServerException, AwsServiceException,
SdkClientException, EbsException {
return putSnapshotBlock(PutSnapshotBlockRequest.builder().applyMutation(putSnapshotBlockRequest).build(), sourcePath);
}
/**
*
* Creates a new Amazon EBS snapshot. The new snapshot enters the pending
state after the request
* completes.
*
*
* After creating the snapshot, use PutSnapshotBlock to
* write blocks of data to the snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param startSnapshotRequest
* @return Result of the StartSnapshot operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws ConcurrentLimitExceededException
* You have reached the limit for concurrent API requests. For more information, see Optimizing performance of the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
* @throws ConflictException
* The request uses the same client token as a previous, but non-identical request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.StartSnapshot
* @see AWS API
* Documentation
*/
default StartSnapshotResponse startSnapshot(StartSnapshotRequest startSnapshotRequest) throws AccessDeniedException,
ValidationException, RequestThrottledException, ResourceNotFoundException, ServiceQuotaExceededException,
InternalServerException, ConcurrentLimitExceededException, ConflictException, AwsServiceException,
SdkClientException, EbsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Amazon EBS snapshot. The new snapshot enters the pending
state after the request
* completes.
*
*
* After creating the snapshot, use PutSnapshotBlock to
* write blocks of data to the snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link StartSnapshotRequest.Builder} avoiding the need to
* create one manually via {@link StartSnapshotRequest#builder()}
*
*
* @param startSnapshotRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ebs.model.StartSnapshotRequest.Builder} to create a request.
* @return Result of the StartSnapshot operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws ConcurrentLimitExceededException
* You have reached the limit for concurrent API requests. For more information, see Optimizing performance of the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
* @throws ConflictException
* The request uses the same client token as a previous, but non-identical request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EbsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EbsClient.StartSnapshot
* @see AWS API
* Documentation
*/
default StartSnapshotResponse startSnapshot(Consumer startSnapshotRequest)
throws AccessDeniedException, ValidationException, RequestThrottledException, ResourceNotFoundException,
ServiceQuotaExceededException, InternalServerException, ConcurrentLimitExceededException, ConflictException,
AwsServiceException, SdkClientException, EbsException {
return startSnapshot(StartSnapshotRequest.builder().applyMutation(startSnapshotRequest).build());
}
/**
* Create a {@link EbsClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static EbsClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link EbsClient}.
*/
static EbsClientBuilder builder() {
return new DefaultEbsClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default EbsServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}