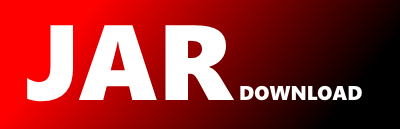
software.amazon.awssdk.services.ec2.model.CapacityBlockOffering Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The recommended Capacity Block that fits your search requirements.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CapacityBlockOffering implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CAPACITY_BLOCK_OFFERING_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CapacityBlockOfferingId")
.getter(getter(CapacityBlockOffering::capacityBlockOfferingId))
.setter(setter(Builder::capacityBlockOfferingId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CapacityBlockOfferingId")
.unmarshallLocationName("capacityBlockOfferingId").build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InstanceType")
.getter(getter(CapacityBlockOffering::instanceType))
.setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType")
.unmarshallLocationName("instanceType").build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AvailabilityZone")
.getter(getter(CapacityBlockOffering::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone")
.unmarshallLocationName("availabilityZone").build()).build();
private static final SdkField INSTANCE_COUNT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("InstanceCount")
.getter(getter(CapacityBlockOffering::instanceCount))
.setter(setter(Builder::instanceCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceCount")
.unmarshallLocationName("instanceCount").build()).build();
private static final SdkField START_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("StartDate")
.getter(getter(CapacityBlockOffering::startDate))
.setter(setter(Builder::startDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartDate")
.unmarshallLocationName("startDate").build()).build();
private static final SdkField END_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("EndDate")
.getter(getter(CapacityBlockOffering::endDate))
.setter(setter(Builder::endDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndDate")
.unmarshallLocationName("endDate").build()).build();
private static final SdkField CAPACITY_BLOCK_DURATION_HOURS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("CapacityBlockDurationHours")
.getter(getter(CapacityBlockOffering::capacityBlockDurationHours))
.setter(setter(Builder::capacityBlockDurationHours))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CapacityBlockDurationHours")
.unmarshallLocationName("capacityBlockDurationHours").build()).build();
private static final SdkField UPFRONT_FEE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("UpfrontFee")
.getter(getter(CapacityBlockOffering::upfrontFee))
.setter(setter(Builder::upfrontFee))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpfrontFee")
.unmarshallLocationName("upfrontFee").build()).build();
private static final SdkField CURRENCY_CODE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CurrencyCode")
.getter(getter(CapacityBlockOffering::currencyCode))
.setter(setter(Builder::currencyCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrencyCode")
.unmarshallLocationName("currencyCode").build()).build();
private static final SdkField TENANCY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Tenancy")
.getter(getter(CapacityBlockOffering::tenancyAsString))
.setter(setter(Builder::tenancy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tenancy")
.unmarshallLocationName("tenancy").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
CAPACITY_BLOCK_OFFERING_ID_FIELD, INSTANCE_TYPE_FIELD, AVAILABILITY_ZONE_FIELD, INSTANCE_COUNT_FIELD,
START_DATE_FIELD, END_DATE_FIELD, CAPACITY_BLOCK_DURATION_HOURS_FIELD, UPFRONT_FEE_FIELD, CURRENCY_CODE_FIELD,
TENANCY_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("CapacityBlockOfferingId", CAPACITY_BLOCK_OFFERING_ID_FIELD);
put("InstanceType", INSTANCE_TYPE_FIELD);
put("AvailabilityZone", AVAILABILITY_ZONE_FIELD);
put("InstanceCount", INSTANCE_COUNT_FIELD);
put("StartDate", START_DATE_FIELD);
put("EndDate", END_DATE_FIELD);
put("CapacityBlockDurationHours", CAPACITY_BLOCK_DURATION_HOURS_FIELD);
put("UpfrontFee", UPFRONT_FEE_FIELD);
put("CurrencyCode", CURRENCY_CODE_FIELD);
put("Tenancy", TENANCY_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String capacityBlockOfferingId;
private final String instanceType;
private final String availabilityZone;
private final Integer instanceCount;
private final Instant startDate;
private final Instant endDate;
private final Integer capacityBlockDurationHours;
private final String upfrontFee;
private final String currencyCode;
private final String tenancy;
private CapacityBlockOffering(BuilderImpl builder) {
this.capacityBlockOfferingId = builder.capacityBlockOfferingId;
this.instanceType = builder.instanceType;
this.availabilityZone = builder.availabilityZone;
this.instanceCount = builder.instanceCount;
this.startDate = builder.startDate;
this.endDate = builder.endDate;
this.capacityBlockDurationHours = builder.capacityBlockDurationHours;
this.upfrontFee = builder.upfrontFee;
this.currencyCode = builder.currencyCode;
this.tenancy = builder.tenancy;
}
/**
*
* The ID of the Capacity Block offering.
*
*
* @return The ID of the Capacity Block offering.
*/
public final String capacityBlockOfferingId() {
return capacityBlockOfferingId;
}
/**
*
* The instance type of the Capacity Block offering.
*
*
* @return The instance type of the Capacity Block offering.
*/
public final String instanceType() {
return instanceType;
}
/**
*
* The Availability Zone of the Capacity Block offering.
*
*
* @return The Availability Zone of the Capacity Block offering.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The number of instances in the Capacity Block offering.
*
*
* @return The number of instances in the Capacity Block offering.
*/
public final Integer instanceCount() {
return instanceCount;
}
/**
*
* The start date of the Capacity Block offering.
*
*
* @return The start date of the Capacity Block offering.
*/
public final Instant startDate() {
return startDate;
}
/**
*
* The end date of the Capacity Block offering.
*
*
* @return The end date of the Capacity Block offering.
*/
public final Instant endDate() {
return endDate;
}
/**
*
* The amount of time of the Capacity Block reservation in hours.
*
*
* @return The amount of time of the Capacity Block reservation in hours.
*/
public final Integer capacityBlockDurationHours() {
return capacityBlockDurationHours;
}
/**
*
* The total price to be paid up front.
*
*
* @return The total price to be paid up front.
*/
public final String upfrontFee() {
return upfrontFee;
}
/**
*
* The currency of the payment for the Capacity Block.
*
*
* @return The currency of the payment for the Capacity Block.
*/
public final String currencyCode() {
return currencyCode;
}
/**
*
* The tenancy of the Capacity Block.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tenancy} will
* return {@link CapacityReservationTenancy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #tenancyAsString}.
*
*
* @return The tenancy of the Capacity Block.
* @see CapacityReservationTenancy
*/
public final CapacityReservationTenancy tenancy() {
return CapacityReservationTenancy.fromValue(tenancy);
}
/**
*
* The tenancy of the Capacity Block.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tenancy} will
* return {@link CapacityReservationTenancy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #tenancyAsString}.
*
*
* @return The tenancy of the Capacity Block.
* @see CapacityReservationTenancy
*/
public final String tenancyAsString() {
return tenancy;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(capacityBlockOfferingId());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(instanceCount());
hashCode = 31 * hashCode + Objects.hashCode(startDate());
hashCode = 31 * hashCode + Objects.hashCode(endDate());
hashCode = 31 * hashCode + Objects.hashCode(capacityBlockDurationHours());
hashCode = 31 * hashCode + Objects.hashCode(upfrontFee());
hashCode = 31 * hashCode + Objects.hashCode(currencyCode());
hashCode = 31 * hashCode + Objects.hashCode(tenancyAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CapacityBlockOffering)) {
return false;
}
CapacityBlockOffering other = (CapacityBlockOffering) obj;
return Objects.equals(capacityBlockOfferingId(), other.capacityBlockOfferingId())
&& Objects.equals(instanceType(), other.instanceType())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(instanceCount(), other.instanceCount()) && Objects.equals(startDate(), other.startDate())
&& Objects.equals(endDate(), other.endDate())
&& Objects.equals(capacityBlockDurationHours(), other.capacityBlockDurationHours())
&& Objects.equals(upfrontFee(), other.upfrontFee()) && Objects.equals(currencyCode(), other.currencyCode())
&& Objects.equals(tenancyAsString(), other.tenancyAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CapacityBlockOffering").add("CapacityBlockOfferingId", capacityBlockOfferingId())
.add("InstanceType", instanceType()).add("AvailabilityZone", availabilityZone())
.add("InstanceCount", instanceCount()).add("StartDate", startDate()).add("EndDate", endDate())
.add("CapacityBlockDurationHours", capacityBlockDurationHours()).add("UpfrontFee", upfrontFee())
.add("CurrencyCode", currencyCode()).add("Tenancy", tenancyAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CapacityBlockOfferingId":
return Optional.ofNullable(clazz.cast(capacityBlockOfferingId()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "InstanceCount":
return Optional.ofNullable(clazz.cast(instanceCount()));
case "StartDate":
return Optional.ofNullable(clazz.cast(startDate()));
case "EndDate":
return Optional.ofNullable(clazz.cast(endDate()));
case "CapacityBlockDurationHours":
return Optional.ofNullable(clazz.cast(capacityBlockDurationHours()));
case "UpfrontFee":
return Optional.ofNullable(clazz.cast(upfrontFee()));
case "CurrencyCode":
return Optional.ofNullable(clazz.cast(currencyCode()));
case "Tenancy":
return Optional.ofNullable(clazz.cast(tenancyAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function