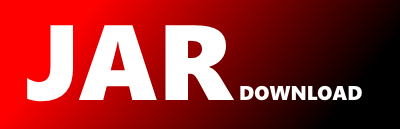
software.amazon.awssdk.services.ec2.model.CreateCapacityReservationRequest Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateCapacityReservationRequest extends Ec2Request implements
ToCopyableBuilder {
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientToken")
.getter(getter(CreateCapacityReservationRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken")
.unmarshallLocationName("ClientToken").build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InstanceType")
.getter(getter(CreateCapacityReservationRequest::instanceType))
.setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType")
.unmarshallLocationName("InstanceType").build()).build();
private static final SdkField INSTANCE_PLATFORM_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InstancePlatform")
.getter(getter(CreateCapacityReservationRequest::instancePlatformAsString))
.setter(setter(Builder::instancePlatform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstancePlatform")
.unmarshallLocationName("InstancePlatform").build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AvailabilityZone")
.getter(getter(CreateCapacityReservationRequest::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone")
.unmarshallLocationName("AvailabilityZone").build()).build();
private static final SdkField AVAILABILITY_ZONE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AvailabilityZoneId")
.getter(getter(CreateCapacityReservationRequest::availabilityZoneId))
.setter(setter(Builder::availabilityZoneId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZoneId")
.unmarshallLocationName("AvailabilityZoneId").build()).build();
private static final SdkField TENANCY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Tenancy")
.getter(getter(CreateCapacityReservationRequest::tenancyAsString))
.setter(setter(Builder::tenancy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tenancy")
.unmarshallLocationName("Tenancy").build()).build();
private static final SdkField INSTANCE_COUNT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("InstanceCount")
.getter(getter(CreateCapacityReservationRequest::instanceCount))
.setter(setter(Builder::instanceCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceCount")
.unmarshallLocationName("InstanceCount").build()).build();
private static final SdkField EBS_OPTIMIZED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EbsOptimized")
.getter(getter(CreateCapacityReservationRequest::ebsOptimized))
.setter(setter(Builder::ebsOptimized))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsOptimized")
.unmarshallLocationName("EbsOptimized").build()).build();
private static final SdkField EPHEMERAL_STORAGE_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EphemeralStorage")
.getter(getter(CreateCapacityReservationRequest::ephemeralStorage))
.setter(setter(Builder::ephemeralStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EphemeralStorage")
.unmarshallLocationName("EphemeralStorage").build()).build();
private static final SdkField END_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("EndDate")
.getter(getter(CreateCapacityReservationRequest::endDate))
.setter(setter(Builder::endDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndDate")
.unmarshallLocationName("EndDate").build()).build();
private static final SdkField END_DATE_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EndDateType")
.getter(getter(CreateCapacityReservationRequest::endDateTypeAsString))
.setter(setter(Builder::endDateType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndDateType")
.unmarshallLocationName("EndDateType").build()).build();
private static final SdkField INSTANCE_MATCH_CRITERIA_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("InstanceMatchCriteria")
.getter(getter(CreateCapacityReservationRequest::instanceMatchCriteriaAsString))
.setter(setter(Builder::instanceMatchCriteria))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceMatchCriteria")
.unmarshallLocationName("InstanceMatchCriteria").build()).build();
private static final SdkField> TAG_SPECIFICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TagSpecifications")
.getter(getter(CreateCapacityReservationRequest::tagSpecifications))
.setter(setter(Builder::tagSpecifications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSpecifications")
.unmarshallLocationName("TagSpecifications").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TagSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField DRY_RUN_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DryRun")
.getter(getter(CreateCapacityReservationRequest::dryRun))
.setter(setter(Builder::dryRun))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DryRun")
.unmarshallLocationName("DryRun").build()).build();
private static final SdkField OUTPOST_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("OutpostArn")
.getter(getter(CreateCapacityReservationRequest::outpostArn))
.setter(setter(Builder::outpostArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutpostArn")
.unmarshallLocationName("OutpostArn").build()).build();
private static final SdkField PLACEMENT_GROUP_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PlacementGroupArn")
.getter(getter(CreateCapacityReservationRequest::placementGroupArn))
.setter(setter(Builder::placementGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlacementGroupArn")
.unmarshallLocationName("PlacementGroupArn").build()).build();
private static final List> SDK_FIELDS = Collections
.unmodifiableList(Arrays.asList(CLIENT_TOKEN_FIELD, INSTANCE_TYPE_FIELD, INSTANCE_PLATFORM_FIELD,
AVAILABILITY_ZONE_FIELD, AVAILABILITY_ZONE_ID_FIELD, TENANCY_FIELD, INSTANCE_COUNT_FIELD,
EBS_OPTIMIZED_FIELD, EPHEMERAL_STORAGE_FIELD, END_DATE_FIELD, END_DATE_TYPE_FIELD,
INSTANCE_MATCH_CRITERIA_FIELD, TAG_SPECIFICATIONS_FIELD, DRY_RUN_FIELD, OUTPOST_ARN_FIELD,
PLACEMENT_GROUP_ARN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("ClientToken", CLIENT_TOKEN_FIELD);
put("InstanceType", INSTANCE_TYPE_FIELD);
put("InstancePlatform", INSTANCE_PLATFORM_FIELD);
put("AvailabilityZone", AVAILABILITY_ZONE_FIELD);
put("AvailabilityZoneId", AVAILABILITY_ZONE_ID_FIELD);
put("Tenancy", TENANCY_FIELD);
put("InstanceCount", INSTANCE_COUNT_FIELD);
put("EbsOptimized", EBS_OPTIMIZED_FIELD);
put("EphemeralStorage", EPHEMERAL_STORAGE_FIELD);
put("EndDate", END_DATE_FIELD);
put("EndDateType", END_DATE_TYPE_FIELD);
put("InstanceMatchCriteria", INSTANCE_MATCH_CRITERIA_FIELD);
put("TagSpecifications", TAG_SPECIFICATIONS_FIELD);
put("DryRun", DRY_RUN_FIELD);
put("OutpostArn", OUTPOST_ARN_FIELD);
put("PlacementGroupArn", PLACEMENT_GROUP_ARN_FIELD);
}
});
private final String clientToken;
private final String instanceType;
private final String instancePlatform;
private final String availabilityZone;
private final String availabilityZoneId;
private final String tenancy;
private final Integer instanceCount;
private final Boolean ebsOptimized;
private final Boolean ephemeralStorage;
private final Instant endDate;
private final String endDateType;
private final String instanceMatchCriteria;
private final List tagSpecifications;
private final Boolean dryRun;
private final String outpostArn;
private final String placementGroupArn;
private CreateCapacityReservationRequest(BuilderImpl builder) {
super(builder);
this.clientToken = builder.clientToken;
this.instanceType = builder.instanceType;
this.instancePlatform = builder.instancePlatform;
this.availabilityZone = builder.availabilityZone;
this.availabilityZoneId = builder.availabilityZoneId;
this.tenancy = builder.tenancy;
this.instanceCount = builder.instanceCount;
this.ebsOptimized = builder.ebsOptimized;
this.ephemeralStorage = builder.ephemeralStorage;
this.endDate = builder.endDate;
this.endDateType = builder.endDateType;
this.instanceMatchCriteria = builder.instanceMatchCriteria;
this.tagSpecifications = builder.tagSpecifications;
this.dryRun = builder.dryRun;
this.outpostArn = builder.outpostArn;
this.placementGroupArn = builder.placementGroupArn;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensure
* Idempotency.
*
*
* @return Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensure
* Idempotency.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* The instance type for which to reserve capacity. For more information, see Instance types in the
* Amazon EC2 User Guide.
*
*
* @return The instance type for which to reserve capacity. For more information, see Instance types in the
* Amazon EC2 User Guide.
*/
public final String instanceType() {
return instanceType;
}
/**
*
* The type of operating system for which to reserve capacity.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instancePlatform}
* will return {@link CapacityReservationInstancePlatform#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #instancePlatformAsString}.
*
*
* @return The type of operating system for which to reserve capacity.
* @see CapacityReservationInstancePlatform
*/
public final CapacityReservationInstancePlatform instancePlatform() {
return CapacityReservationInstancePlatform.fromValue(instancePlatform);
}
/**
*
* The type of operating system for which to reserve capacity.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instancePlatform}
* will return {@link CapacityReservationInstancePlatform#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the
* service is available from {@link #instancePlatformAsString}.
*
*
* @return The type of operating system for which to reserve capacity.
* @see CapacityReservationInstancePlatform
*/
public final String instancePlatformAsString() {
return instancePlatform;
}
/**
*
* The Availability Zone in which to create the Capacity Reservation.
*
*
* @return The Availability Zone in which to create the Capacity Reservation.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The ID of the Availability Zone in which to create the Capacity Reservation.
*
*
* @return The ID of the Availability Zone in which to create the Capacity Reservation.
*/
public final String availabilityZoneId() {
return availabilityZoneId;
}
/**
*
* Indicates the tenancy of the Capacity Reservation. A Capacity Reservation can have one of the following tenancy
* settings:
*
*
* -
*
* default
- The Capacity Reservation is created on hardware that is shared with other Amazon Web
* Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservation is created on single-tenant hardware that is dedicated to a
* single Amazon Web Services account.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tenancy} will
* return {@link CapacityReservationTenancy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #tenancyAsString}.
*
*
* @return Indicates the tenancy of the Capacity Reservation. A Capacity Reservation can have one of the following
* tenancy settings:
*
* -
*
* default
- The Capacity Reservation is created on hardware that is shared with other Amazon
* Web Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservation is created on single-tenant hardware that is dedicated
* to a single Amazon Web Services account.
*
*
* @see CapacityReservationTenancy
*/
public final CapacityReservationTenancy tenancy() {
return CapacityReservationTenancy.fromValue(tenancy);
}
/**
*
* Indicates the tenancy of the Capacity Reservation. A Capacity Reservation can have one of the following tenancy
* settings:
*
*
* -
*
* default
- The Capacity Reservation is created on hardware that is shared with other Amazon Web
* Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservation is created on single-tenant hardware that is dedicated to a
* single Amazon Web Services account.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tenancy} will
* return {@link CapacityReservationTenancy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #tenancyAsString}.
*
*
* @return Indicates the tenancy of the Capacity Reservation. A Capacity Reservation can have one of the following
* tenancy settings:
*
* -
*
* default
- The Capacity Reservation is created on hardware that is shared with other Amazon
* Web Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservation is created on single-tenant hardware that is dedicated
* to a single Amazon Web Services account.
*
*
* @see CapacityReservationTenancy
*/
public final String tenancyAsString() {
return tenancy;
}
/**
*
* The number of instances for which to reserve capacity.
*
*
* Valid range: 1 - 1000
*
*
* @return The number of instances for which to reserve capacity.
*
* Valid range: 1 - 1000
*/
public final Integer instanceCount() {
return instanceCount;
}
/**
*
* Indicates whether the Capacity Reservation supports EBS-optimized instances. This optimization provides dedicated
* throughput to Amazon EBS and an optimized configuration stack to provide optimal I/O performance. This
* optimization isn't available with all instance types. Additional usage charges apply when using an EBS- optimized
* instance.
*
*
* @return Indicates whether the Capacity Reservation supports EBS-optimized instances. This optimization provides
* dedicated throughput to Amazon EBS and an optimized configuration stack to provide optimal I/O
* performance. This optimization isn't available with all instance types. Additional usage charges apply
* when using an EBS- optimized instance.
*/
public final Boolean ebsOptimized() {
return ebsOptimized;
}
/**
*
* Deprecated.
*
*
* @return Deprecated.
*/
public final Boolean ephemeralStorage() {
return ephemeralStorage;
}
/**
*
* The date and time at which the Capacity Reservation expires. When a Capacity Reservation expires, the reserved
* capacity is released and you can no longer launch instances into it. The Capacity Reservation's state changes to
* expired
when it reaches its end date and time.
*
*
* You must provide an EndDate
value if EndDateType
is limited
. Omit
* EndDate
if EndDateType
is unlimited
.
*
*
* If the EndDateType
is limited
, the Capacity Reservation is cancelled within an hour
* from the specified time. For example, if you specify 5/31/2019, 13:30:55, the Capacity Reservation is guaranteed
* to end between 13:30:55 and 14:30:55 on 5/31/2019.
*
*
* @return The date and time at which the Capacity Reservation expires. When a Capacity Reservation expires, the
* reserved capacity is released and you can no longer launch instances into it. The Capacity Reservation's
* state changes to expired
when it reaches its end date and time.
*
* You must provide an EndDate
value if EndDateType
is limited
. Omit
* EndDate
if EndDateType
is unlimited
.
*
*
* If the EndDateType
is limited
, the Capacity Reservation is cancelled within an
* hour from the specified time. For example, if you specify 5/31/2019, 13:30:55, the Capacity Reservation
* is guaranteed to end between 13:30:55 and 14:30:55 on 5/31/2019.
*/
public final Instant endDate() {
return endDate;
}
/**
*
* Indicates the way in which the Capacity Reservation ends. A Capacity Reservation can have one of the following
* end types:
*
*
* -
*
* unlimited
- The Capacity Reservation remains active until you explicitly cancel it. Do not provide
* an EndDate
if the EndDateType
is unlimited
.
*
*
* -
*
* limited
- The Capacity Reservation expires automatically at a specified date and time. You must
* provide an EndDate
value if the EndDateType
value is limited
.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #endDateType} will
* return {@link EndDateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #endDateTypeAsString}.
*
*
* @return Indicates the way in which the Capacity Reservation ends. A Capacity Reservation can have one of the
* following end types:
*
* -
*
* unlimited
- The Capacity Reservation remains active until you explicitly cancel it. Do not
* provide an EndDate
if the EndDateType
is unlimited
.
*
*
* -
*
* limited
- The Capacity Reservation expires automatically at a specified date and time. You
* must provide an EndDate
value if the EndDateType
value is limited
.
*
*
* @see EndDateType
*/
public final EndDateType endDateType() {
return EndDateType.fromValue(endDateType);
}
/**
*
* Indicates the way in which the Capacity Reservation ends. A Capacity Reservation can have one of the following
* end types:
*
*
* -
*
* unlimited
- The Capacity Reservation remains active until you explicitly cancel it. Do not provide
* an EndDate
if the EndDateType
is unlimited
.
*
*
* -
*
* limited
- The Capacity Reservation expires automatically at a specified date and time. You must
* provide an EndDate
value if the EndDateType
value is limited
.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #endDateType} will
* return {@link EndDateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #endDateTypeAsString}.
*
*
* @return Indicates the way in which the Capacity Reservation ends. A Capacity Reservation can have one of the
* following end types:
*
* -
*
* unlimited
- The Capacity Reservation remains active until you explicitly cancel it. Do not
* provide an EndDate
if the EndDateType
is unlimited
.
*
*
* -
*
* limited
- The Capacity Reservation expires automatically at a specified date and time. You
* must provide an EndDate
value if the EndDateType
value is limited
.
*
*
* @see EndDateType
*/
public final String endDateTypeAsString() {
return endDateType;
}
/**
*
* Indicates the type of instance launches that the Capacity Reservation accepts. The options include:
*
*
* -
*
* open
- The Capacity Reservation automatically matches all instances that have matching attributes
* (instance type, platform, and Availability Zone). Instances that have matching attributes run in the Capacity
* Reservation automatically without specifying any additional parameters.
*
*
* -
*
* targeted
- The Capacity Reservation only accepts instances that have matching attributes (instance
* type, platform, and Availability Zone), and explicitly target the Capacity Reservation. This ensures that only
* permitted instances can use the reserved capacity.
*
*
*
*
* Default: open
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #instanceMatchCriteria} will return {@link InstanceMatchCriteria#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #instanceMatchCriteriaAsString}.
*
*
* @return Indicates the type of instance launches that the Capacity Reservation accepts. The options include:
*
* -
*
* open
- The Capacity Reservation automatically matches all instances that have matching
* attributes (instance type, platform, and Availability Zone). Instances that have matching attributes run
* in the Capacity Reservation automatically without specifying any additional parameters.
*
*
* -
*
* targeted
- The Capacity Reservation only accepts instances that have matching attributes
* (instance type, platform, and Availability Zone), and explicitly target the Capacity Reservation. This
* ensures that only permitted instances can use the reserved capacity.
*
*
*
*
* Default: open
* @see InstanceMatchCriteria
*/
public final InstanceMatchCriteria instanceMatchCriteria() {
return InstanceMatchCriteria.fromValue(instanceMatchCriteria);
}
/**
*
* Indicates the type of instance launches that the Capacity Reservation accepts. The options include:
*
*
* -
*
* open
- The Capacity Reservation automatically matches all instances that have matching attributes
* (instance type, platform, and Availability Zone). Instances that have matching attributes run in the Capacity
* Reservation automatically without specifying any additional parameters.
*
*
* -
*
* targeted
- The Capacity Reservation only accepts instances that have matching attributes (instance
* type, platform, and Availability Zone), and explicitly target the Capacity Reservation. This ensures that only
* permitted instances can use the reserved capacity.
*
*
*
*
* Default: open
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #instanceMatchCriteria} will return {@link InstanceMatchCriteria#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #instanceMatchCriteriaAsString}.
*
*
* @return Indicates the type of instance launches that the Capacity Reservation accepts. The options include:
*
* -
*
* open
- The Capacity Reservation automatically matches all instances that have matching
* attributes (instance type, platform, and Availability Zone). Instances that have matching attributes run
* in the Capacity Reservation automatically without specifying any additional parameters.
*
*
* -
*
* targeted
- The Capacity Reservation only accepts instances that have matching attributes
* (instance type, platform, and Availability Zone), and explicitly target the Capacity Reservation. This
* ensures that only permitted instances can use the reserved capacity.
*
*
*
*
* Default: open
* @see InstanceMatchCriteria
*/
public final String instanceMatchCriteriaAsString() {
return instanceMatchCriteria;
}
/**
* For responses, this returns true if the service returned a value for the TagSpecifications property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTagSpecifications() {
return tagSpecifications != null && !(tagSpecifications instanceof SdkAutoConstructList);
}
/**
*
* The tags to apply to the Capacity Reservation during launch.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagSpecifications} method.
*
*
* @return The tags to apply to the Capacity Reservation during launch.
*/
public final List tagSpecifications() {
return tagSpecifications;
}
/**
*
* Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*
*
* @return Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*/
public final Boolean dryRun() {
return dryRun;
}
/**
*
* The Amazon Resource Name (ARN) of the Outpost on which to create the Capacity Reservation.
*
*
* @return The Amazon Resource Name (ARN) of the Outpost on which to create the Capacity Reservation.
*/
public final String outpostArn() {
return outpostArn;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster placement group in which to create the Capacity Reservation. For
* more information, see Capacity
* Reservations for cluster placement groups in the Amazon EC2 User Guide.
*
*
* @return The Amazon Resource Name (ARN) of the cluster placement group in which to create the Capacity
* Reservation. For more information, see Capacity Reservations for cluster
* placement groups in the Amazon EC2 User Guide.
*/
public final String placementGroupArn() {
return placementGroupArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(instancePlatformAsString());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZoneId());
hashCode = 31 * hashCode + Objects.hashCode(tenancyAsString());
hashCode = 31 * hashCode + Objects.hashCode(instanceCount());
hashCode = 31 * hashCode + Objects.hashCode(ebsOptimized());
hashCode = 31 * hashCode + Objects.hashCode(ephemeralStorage());
hashCode = 31 * hashCode + Objects.hashCode(endDate());
hashCode = 31 * hashCode + Objects.hashCode(endDateTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(instanceMatchCriteriaAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasTagSpecifications() ? tagSpecifications() : null);
hashCode = 31 * hashCode + Objects.hashCode(dryRun());
hashCode = 31 * hashCode + Objects.hashCode(outpostArn());
hashCode = 31 * hashCode + Objects.hashCode(placementGroupArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateCapacityReservationRequest)) {
return false;
}
CreateCapacityReservationRequest other = (CreateCapacityReservationRequest) obj;
return Objects.equals(clientToken(), other.clientToken()) && Objects.equals(instanceType(), other.instanceType())
&& Objects.equals(instancePlatformAsString(), other.instancePlatformAsString())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(availabilityZoneId(), other.availabilityZoneId())
&& Objects.equals(tenancyAsString(), other.tenancyAsString())
&& Objects.equals(instanceCount(), other.instanceCount()) && Objects.equals(ebsOptimized(), other.ebsOptimized())
&& Objects.equals(ephemeralStorage(), other.ephemeralStorage()) && Objects.equals(endDate(), other.endDate())
&& Objects.equals(endDateTypeAsString(), other.endDateTypeAsString())
&& Objects.equals(instanceMatchCriteriaAsString(), other.instanceMatchCriteriaAsString())
&& hasTagSpecifications() == other.hasTagSpecifications()
&& Objects.equals(tagSpecifications(), other.tagSpecifications()) && Objects.equals(dryRun(), other.dryRun())
&& Objects.equals(outpostArn(), other.outpostArn())
&& Objects.equals(placementGroupArn(), other.placementGroupArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateCapacityReservationRequest").add("ClientToken", clientToken())
.add("InstanceType", instanceType()).add("InstancePlatform", instancePlatformAsString())
.add("AvailabilityZone", availabilityZone()).add("AvailabilityZoneId", availabilityZoneId())
.add("Tenancy", tenancyAsString()).add("InstanceCount", instanceCount()).add("EbsOptimized", ebsOptimized())
.add("EphemeralStorage", ephemeralStorage()).add("EndDate", endDate()).add("EndDateType", endDateTypeAsString())
.add("InstanceMatchCriteria", instanceMatchCriteriaAsString())
.add("TagSpecifications", hasTagSpecifications() ? tagSpecifications() : null).add("DryRun", dryRun())
.add("OutpostArn", outpostArn()).add("PlacementGroupArn", placementGroupArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "InstancePlatform":
return Optional.ofNullable(clazz.cast(instancePlatformAsString()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "AvailabilityZoneId":
return Optional.ofNullable(clazz.cast(availabilityZoneId()));
case "Tenancy":
return Optional.ofNullable(clazz.cast(tenancyAsString()));
case "InstanceCount":
return Optional.ofNullable(clazz.cast(instanceCount()));
case "EbsOptimized":
return Optional.ofNullable(clazz.cast(ebsOptimized()));
case "EphemeralStorage":
return Optional.ofNullable(clazz.cast(ephemeralStorage()));
case "EndDate":
return Optional.ofNullable(clazz.cast(endDate()));
case "EndDateType":
return Optional.ofNullable(clazz.cast(endDateTypeAsString()));
case "InstanceMatchCriteria":
return Optional.ofNullable(clazz.cast(instanceMatchCriteriaAsString()));
case "TagSpecifications":
return Optional.ofNullable(clazz.cast(tagSpecifications()));
case "DryRun":
return Optional.ofNullable(clazz.cast(dryRun()));
case "OutpostArn":
return Optional.ofNullable(clazz.cast(outpostArn()));
case "PlacementGroupArn":
return Optional.ofNullable(clazz.cast(placementGroupArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function