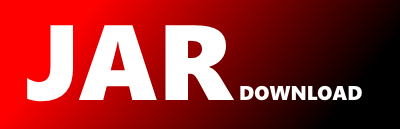
software.amazon.awssdk.services.ec2.model.CreateFleetRequest Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateFleetRequest extends Ec2Request implements
ToCopyableBuilder {
private static final SdkField DRY_RUN_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DryRun")
.getter(getter(CreateFleetRequest::dryRun))
.setter(setter(Builder::dryRun))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DryRun")
.unmarshallLocationName("DryRun").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientToken")
.getter(getter(CreateFleetRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken")
.unmarshallLocationName("ClientToken").build()).build();
private static final SdkField SPOT_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("SpotOptions")
.getter(getter(CreateFleetRequest::spotOptions))
.setter(setter(Builder::spotOptions))
.constructor(SpotOptionsRequest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SpotOptions")
.unmarshallLocationName("SpotOptions").build()).build();
private static final SdkField ON_DEMAND_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("OnDemandOptions")
.getter(getter(CreateFleetRequest::onDemandOptions))
.setter(setter(Builder::onDemandOptions))
.constructor(OnDemandOptionsRequest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OnDemandOptions")
.unmarshallLocationName("OnDemandOptions").build()).build();
private static final SdkField EXCESS_CAPACITY_TERMINATION_POLICY_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ExcessCapacityTerminationPolicy")
.getter(getter(CreateFleetRequest::excessCapacityTerminationPolicyAsString))
.setter(setter(Builder::excessCapacityTerminationPolicy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExcessCapacityTerminationPolicy")
.unmarshallLocationName("ExcessCapacityTerminationPolicy").build()).build();
private static final SdkField> LAUNCH_TEMPLATE_CONFIGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LaunchTemplateConfigs")
.getter(getter(CreateFleetRequest::launchTemplateConfigs))
.setter(setter(Builder::launchTemplateConfigs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchTemplateConfigs")
.unmarshallLocationName("LaunchTemplateConfigs").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(FleetLaunchTemplateConfigRequest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField TARGET_CAPACITY_SPECIFICATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("TargetCapacitySpecification")
.getter(getter(CreateFleetRequest::targetCapacitySpecification))
.setter(setter(Builder::targetCapacitySpecification))
.constructor(TargetCapacitySpecificationRequest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetCapacitySpecification")
.unmarshallLocationName("TargetCapacitySpecification").build()).build();
private static final SdkField TERMINATE_INSTANCES_WITH_EXPIRATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("TerminateInstancesWithExpiration")
.getter(getter(CreateFleetRequest::terminateInstancesWithExpiration))
.setter(setter(Builder::terminateInstancesWithExpiration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TerminateInstancesWithExpiration")
.unmarshallLocationName("TerminateInstancesWithExpiration").build()).build();
private static final SdkField TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Type")
.getter(getter(CreateFleetRequest::typeAsString))
.setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type")
.unmarshallLocationName("Type").build()).build();
private static final SdkField VALID_FROM_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("ValidFrom")
.getter(getter(CreateFleetRequest::validFrom))
.setter(setter(Builder::validFrom))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ValidFrom")
.unmarshallLocationName("ValidFrom").build()).build();
private static final SdkField VALID_UNTIL_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("ValidUntil")
.getter(getter(CreateFleetRequest::validUntil))
.setter(setter(Builder::validUntil))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ValidUntil")
.unmarshallLocationName("ValidUntil").build()).build();
private static final SdkField REPLACE_UNHEALTHY_INSTANCES_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ReplaceUnhealthyInstances")
.getter(getter(CreateFleetRequest::replaceUnhealthyInstances))
.setter(setter(Builder::replaceUnhealthyInstances))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplaceUnhealthyInstances")
.unmarshallLocationName("ReplaceUnhealthyInstances").build()).build();
private static final SdkField> TAG_SPECIFICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TagSpecifications")
.getter(getter(CreateFleetRequest::tagSpecifications))
.setter(setter(Builder::tagSpecifications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSpecification")
.unmarshallLocationName("TagSpecification").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TagSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField CONTEXT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Context")
.getter(getter(CreateFleetRequest::context))
.setter(setter(Builder::context))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Context")
.unmarshallLocationName("Context").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DRY_RUN_FIELD,
CLIENT_TOKEN_FIELD, SPOT_OPTIONS_FIELD, ON_DEMAND_OPTIONS_FIELD, EXCESS_CAPACITY_TERMINATION_POLICY_FIELD,
LAUNCH_TEMPLATE_CONFIGS_FIELD, TARGET_CAPACITY_SPECIFICATION_FIELD, TERMINATE_INSTANCES_WITH_EXPIRATION_FIELD,
TYPE_FIELD, VALID_FROM_FIELD, VALID_UNTIL_FIELD, REPLACE_UNHEALTHY_INSTANCES_FIELD, TAG_SPECIFICATIONS_FIELD,
CONTEXT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("DryRun", DRY_RUN_FIELD);
put("ClientToken", CLIENT_TOKEN_FIELD);
put("SpotOptions", SPOT_OPTIONS_FIELD);
put("OnDemandOptions", ON_DEMAND_OPTIONS_FIELD);
put("ExcessCapacityTerminationPolicy", EXCESS_CAPACITY_TERMINATION_POLICY_FIELD);
put("LaunchTemplateConfigs", LAUNCH_TEMPLATE_CONFIGS_FIELD);
put("TargetCapacitySpecification", TARGET_CAPACITY_SPECIFICATION_FIELD);
put("TerminateInstancesWithExpiration", TERMINATE_INSTANCES_WITH_EXPIRATION_FIELD);
put("Type", TYPE_FIELD);
put("ValidFrom", VALID_FROM_FIELD);
put("ValidUntil", VALID_UNTIL_FIELD);
put("ReplaceUnhealthyInstances", REPLACE_UNHEALTHY_INSTANCES_FIELD);
put("TagSpecification", TAG_SPECIFICATIONS_FIELD);
put("Context", CONTEXT_FIELD);
}
});
private final Boolean dryRun;
private final String clientToken;
private final SpotOptionsRequest spotOptions;
private final OnDemandOptionsRequest onDemandOptions;
private final String excessCapacityTerminationPolicy;
private final List launchTemplateConfigs;
private final TargetCapacitySpecificationRequest targetCapacitySpecification;
private final Boolean terminateInstancesWithExpiration;
private final String type;
private final Instant validFrom;
private final Instant validUntil;
private final Boolean replaceUnhealthyInstances;
private final List tagSpecifications;
private final String context;
private CreateFleetRequest(BuilderImpl builder) {
super(builder);
this.dryRun = builder.dryRun;
this.clientToken = builder.clientToken;
this.spotOptions = builder.spotOptions;
this.onDemandOptions = builder.onDemandOptions;
this.excessCapacityTerminationPolicy = builder.excessCapacityTerminationPolicy;
this.launchTemplateConfigs = builder.launchTemplateConfigs;
this.targetCapacitySpecification = builder.targetCapacitySpecification;
this.terminateInstancesWithExpiration = builder.terminateInstancesWithExpiration;
this.type = builder.type;
this.validFrom = builder.validFrom;
this.validUntil = builder.validUntil;
this.replaceUnhealthyInstances = builder.replaceUnhealthyInstances;
this.tagSpecifications = builder.tagSpecifications;
this.context = builder.context;
}
/**
*
* Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*
*
* @return Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*/
public final Boolean dryRun() {
return dryRun;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensuring
* idempotency.
*
*
* @return Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensuring
* idempotency.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* Describes the configuration of Spot Instances in an EC2 Fleet.
*
*
* @return Describes the configuration of Spot Instances in an EC2 Fleet.
*/
public final SpotOptionsRequest spotOptions() {
return spotOptions;
}
/**
*
* Describes the configuration of On-Demand Instances in an EC2 Fleet.
*
*
* @return Describes the configuration of On-Demand Instances in an EC2 Fleet.
*/
public final OnDemandOptionsRequest onDemandOptions() {
return onDemandOptions;
}
/**
*
* Indicates whether running instances should be terminated if the total target capacity of the EC2 Fleet is
* decreased below the current size of the EC2 Fleet.
*
*
* Supported only for fleets of type maintain
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #excessCapacityTerminationPolicy} will return
* {@link FleetExcessCapacityTerminationPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #excessCapacityTerminationPolicyAsString}.
*
*
* @return Indicates whether running instances should be terminated if the total target capacity of the EC2 Fleet is
* decreased below the current size of the EC2 Fleet.
*
* Supported only for fleets of type maintain
.
* @see FleetExcessCapacityTerminationPolicy
*/
public final FleetExcessCapacityTerminationPolicy excessCapacityTerminationPolicy() {
return FleetExcessCapacityTerminationPolicy.fromValue(excessCapacityTerminationPolicy);
}
/**
*
* Indicates whether running instances should be terminated if the total target capacity of the EC2 Fleet is
* decreased below the current size of the EC2 Fleet.
*
*
* Supported only for fleets of type maintain
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #excessCapacityTerminationPolicy} will return
* {@link FleetExcessCapacityTerminationPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #excessCapacityTerminationPolicyAsString}.
*
*
* @return Indicates whether running instances should be terminated if the total target capacity of the EC2 Fleet is
* decreased below the current size of the EC2 Fleet.
*
* Supported only for fleets of type maintain
.
* @see FleetExcessCapacityTerminationPolicy
*/
public final String excessCapacityTerminationPolicyAsString() {
return excessCapacityTerminationPolicy;
}
/**
* For responses, this returns true if the service returned a value for the LaunchTemplateConfigs property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasLaunchTemplateConfigs() {
return launchTemplateConfigs != null && !(launchTemplateConfigs instanceof SdkAutoConstructList);
}
/**
*
* The configuration for the EC2 Fleet.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLaunchTemplateConfigs} method.
*
*
* @return The configuration for the EC2 Fleet.
*/
public final List launchTemplateConfigs() {
return launchTemplateConfigs;
}
/**
*
* The number of units to request.
*
*
* @return The number of units to request.
*/
public final TargetCapacitySpecificationRequest targetCapacitySpecification() {
return targetCapacitySpecification;
}
/**
*
* Indicates whether running instances should be terminated when the EC2 Fleet expires.
*
*
* @return Indicates whether running instances should be terminated when the EC2 Fleet expires.
*/
public final Boolean terminateInstancesWithExpiration() {
return terminateInstancesWithExpiration;
}
/**
*
* The fleet type. The default value is maintain
.
*
*
* -
*
* maintain
- The EC2 Fleet places an asynchronous request for your desired capacity, and continues to
* maintain your desired Spot capacity by replenishing interrupted Spot Instances.
*
*
* -
*
* request
- The EC2 Fleet places an asynchronous one-time request for your desired capacity, but does
* submit Spot requests in alternative capacity pools if Spot capacity is unavailable, and does not maintain Spot
* capacity if Spot Instances are interrupted.
*
*
* -
*
* instant
- The EC2 Fleet places a synchronous one-time request for your desired capacity, and returns
* errors for any instances that could not be launched.
*
*
*
*
* For more information, see EC2 Fleet request
* types in the Amazon EC2 User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link FleetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The fleet type. The default value is maintain
.
*
* -
*
* maintain
- The EC2 Fleet places an asynchronous request for your desired capacity, and
* continues to maintain your desired Spot capacity by replenishing interrupted Spot Instances.
*
*
* -
*
* request
- The EC2 Fleet places an asynchronous one-time request for your desired capacity,
* but does submit Spot requests in alternative capacity pools if Spot capacity is unavailable, and does not
* maintain Spot capacity if Spot Instances are interrupted.
*
*
* -
*
* instant
- The EC2 Fleet places a synchronous one-time request for your desired capacity, and
* returns errors for any instances that could not be launched.
*
*
*
*
* For more information, see EC2 Fleet request
* types in the Amazon EC2 User Guide.
* @see FleetType
*/
public final FleetType type() {
return FleetType.fromValue(type);
}
/**
*
* The fleet type. The default value is maintain
.
*
*
* -
*
* maintain
- The EC2 Fleet places an asynchronous request for your desired capacity, and continues to
* maintain your desired Spot capacity by replenishing interrupted Spot Instances.
*
*
* -
*
* request
- The EC2 Fleet places an asynchronous one-time request for your desired capacity, but does
* submit Spot requests in alternative capacity pools if Spot capacity is unavailable, and does not maintain Spot
* capacity if Spot Instances are interrupted.
*
*
* -
*
* instant
- The EC2 Fleet places a synchronous one-time request for your desired capacity, and returns
* errors for any instances that could not be launched.
*
*
*
*
* For more information, see EC2 Fleet request
* types in the Amazon EC2 User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link FleetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The fleet type. The default value is maintain
.
*
* -
*
* maintain
- The EC2 Fleet places an asynchronous request for your desired capacity, and
* continues to maintain your desired Spot capacity by replenishing interrupted Spot Instances.
*
*
* -
*
* request
- The EC2 Fleet places an asynchronous one-time request for your desired capacity,
* but does submit Spot requests in alternative capacity pools if Spot capacity is unavailable, and does not
* maintain Spot capacity if Spot Instances are interrupted.
*
*
* -
*
* instant
- The EC2 Fleet places a synchronous one-time request for your desired capacity, and
* returns errors for any instances that could not be launched.
*
*
*
*
* For more information, see EC2 Fleet request
* types in the Amazon EC2 User Guide.
* @see FleetType
*/
public final String typeAsString() {
return type;
}
/**
*
* The start date and time of the request, in UTC format (for example,
* YYYY-MM-DDTHH:MM:SSZ). The default is to start fulfilling the request
* immediately.
*
*
* @return The start date and time of the request, in UTC format (for example,
* YYYY-MM-DDTHH:MM:SSZ). The default is to start fulfilling the
* request immediately.
*/
public final Instant validFrom() {
return validFrom;
}
/**
*
* The end date and time of the request, in UTC format (for example,
* YYYY-MM-DDTHH:MM:SSZ). At this point, no new EC2 Fleet requests are
* placed or able to fulfill the request. If no value is specified, the request remains until you cancel it.
*
*
* @return The end date and time of the request, in UTC format (for example,
* YYYY-MM-DDTHH:MM:SSZ). At this point, no new EC2 Fleet requests
* are placed or able to fulfill the request. If no value is specified, the request remains until you cancel
* it.
*/
public final Instant validUntil() {
return validUntil;
}
/**
*
* Indicates whether EC2 Fleet should replace unhealthy Spot Instances. Supported only for fleets of type
* maintain
. For more information, see EC2
* Fleet health checks in the Amazon EC2 User Guide.
*
*
* @return Indicates whether EC2 Fleet should replace unhealthy Spot Instances. Supported only for fleets of type
* maintain
. For more information, see EC2 Fleet health checks in the Amazon EC2 User Guide.
*/
public final Boolean replaceUnhealthyInstances() {
return replaceUnhealthyInstances;
}
/**
* For responses, this returns true if the service returned a value for the TagSpecifications property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTagSpecifications() {
return tagSpecifications != null && !(tagSpecifications instanceof SdkAutoConstructList);
}
/**
*
* The key-value pair for tagging the EC2 Fleet request on creation. For more information, see Tag your resources.
*
*
* If the fleet type is instant
, specify a resource type of fleet
to tag the fleet or
* instance
to tag the instances at launch.
*
*
* If the fleet type is maintain
or request
, specify a resource type of fleet
* to tag the fleet. You cannot specify a resource type of instance
. To tag instances at launch,
* specify the tags in a launch template.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagSpecifications} method.
*
*
* @return The key-value pair for tagging the EC2 Fleet request on creation. For more information, see Tag your
* resources.
*
* If the fleet type is instant
, specify a resource type of fleet
to tag the fleet
* or instance
to tag the instances at launch.
*
*
* If the fleet type is maintain
or request
, specify a resource type of
* fleet
to tag the fleet. You cannot specify a resource type of instance
. To tag
* instances at launch, specify the tags in a launch template.
*/
public final List tagSpecifications() {
return tagSpecifications;
}
/**
*
* Reserved.
*
*
* @return Reserved.
*/
public final String context() {
return context;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dryRun());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(spotOptions());
hashCode = 31 * hashCode + Objects.hashCode(onDemandOptions());
hashCode = 31 * hashCode + Objects.hashCode(excessCapacityTerminationPolicyAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasLaunchTemplateConfigs() ? launchTemplateConfigs() : null);
hashCode = 31 * hashCode + Objects.hashCode(targetCapacitySpecification());
hashCode = 31 * hashCode + Objects.hashCode(terminateInstancesWithExpiration());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(validFrom());
hashCode = 31 * hashCode + Objects.hashCode(validUntil());
hashCode = 31 * hashCode + Objects.hashCode(replaceUnhealthyInstances());
hashCode = 31 * hashCode + Objects.hashCode(hasTagSpecifications() ? tagSpecifications() : null);
hashCode = 31 * hashCode + Objects.hashCode(context());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateFleetRequest)) {
return false;
}
CreateFleetRequest other = (CreateFleetRequest) obj;
return Objects.equals(dryRun(), other.dryRun()) && Objects.equals(clientToken(), other.clientToken())
&& Objects.equals(spotOptions(), other.spotOptions())
&& Objects.equals(onDemandOptions(), other.onDemandOptions())
&& Objects.equals(excessCapacityTerminationPolicyAsString(), other.excessCapacityTerminationPolicyAsString())
&& hasLaunchTemplateConfigs() == other.hasLaunchTemplateConfigs()
&& Objects.equals(launchTemplateConfigs(), other.launchTemplateConfigs())
&& Objects.equals(targetCapacitySpecification(), other.targetCapacitySpecification())
&& Objects.equals(terminateInstancesWithExpiration(), other.terminateInstancesWithExpiration())
&& Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(validFrom(), other.validFrom())
&& Objects.equals(validUntil(), other.validUntil())
&& Objects.equals(replaceUnhealthyInstances(), other.replaceUnhealthyInstances())
&& hasTagSpecifications() == other.hasTagSpecifications()
&& Objects.equals(tagSpecifications(), other.tagSpecifications()) && Objects.equals(context(), other.context());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateFleetRequest").add("DryRun", dryRun()).add("ClientToken", clientToken())
.add("SpotOptions", spotOptions()).add("OnDemandOptions", onDemandOptions())
.add("ExcessCapacityTerminationPolicy", excessCapacityTerminationPolicyAsString())
.add("LaunchTemplateConfigs", hasLaunchTemplateConfigs() ? launchTemplateConfigs() : null)
.add("TargetCapacitySpecification", targetCapacitySpecification())
.add("TerminateInstancesWithExpiration", terminateInstancesWithExpiration()).add("Type", typeAsString())
.add("ValidFrom", validFrom()).add("ValidUntil", validUntil())
.add("ReplaceUnhealthyInstances", replaceUnhealthyInstances())
.add("TagSpecifications", hasTagSpecifications() ? tagSpecifications() : null).add("Context", context()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DryRun":
return Optional.ofNullable(clazz.cast(dryRun()));
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "SpotOptions":
return Optional.ofNullable(clazz.cast(spotOptions()));
case "OnDemandOptions":
return Optional.ofNullable(clazz.cast(onDemandOptions()));
case "ExcessCapacityTerminationPolicy":
return Optional.ofNullable(clazz.cast(excessCapacityTerminationPolicyAsString()));
case "LaunchTemplateConfigs":
return Optional.ofNullable(clazz.cast(launchTemplateConfigs()));
case "TargetCapacitySpecification":
return Optional.ofNullable(clazz.cast(targetCapacitySpecification()));
case "TerminateInstancesWithExpiration":
return Optional.ofNullable(clazz.cast(terminateInstancesWithExpiration()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "ValidFrom":
return Optional.ofNullable(clazz.cast(validFrom()));
case "ValidUntil":
return Optional.ofNullable(clazz.cast(validUntil()));
case "ReplaceUnhealthyInstances":
return Optional.ofNullable(clazz.cast(replaceUnhealthyInstances()));
case "TagSpecifications":
return Optional.ofNullable(clazz.cast(tagSpecifications()));
case "Context":
return Optional.ofNullable(clazz.cast(context()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* If the fleet type is instant
, specify a resource type of fleet
to tag the
* fleet or instance
to tag the instances at launch.
*
*
* If the fleet type is maintain
or request
, specify a resource type of
* fleet
to tag the fleet. You cannot specify a resource type of instance
. To
* tag instances at launch, specify the tags in a launch template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tagSpecifications(Collection tagSpecifications);
/**
*
* The key-value pair for tagging the EC2 Fleet request on creation. For more information, see Tag your
* resources.
*
*
* If the fleet type is instant
, specify a resource type of fleet
to tag the fleet or
* instance
to tag the instances at launch.
*
*
* If the fleet type is maintain
or request
, specify a resource type of
* fleet
to tag the fleet. You cannot specify a resource type of instance
. To tag
* instances at launch, specify the tags in a launch template.
*
*
* @param tagSpecifications
* The key-value pair for tagging the EC2 Fleet request on creation. For more information, see Tag your
* resources.
*
* If the fleet type is instant
, specify a resource type of fleet
to tag the
* fleet or instance
to tag the instances at launch.
*
*
* If the fleet type is maintain
or request
, specify a resource type of
* fleet
to tag the fleet. You cannot specify a resource type of instance
. To
* tag instances at launch, specify the tags in a launch template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tagSpecifications(TagSpecification... tagSpecifications);
/**
*
* The key-value pair for tagging the EC2 Fleet request on creation. For more information, see Tag your
* resources.
*
*
* If the fleet type is instant
, specify a resource type of fleet
to tag the fleet or
* instance
to tag the instances at launch.
*
*
* If the fleet type is maintain
or request
, specify a resource type of
* fleet
to tag the fleet. You cannot specify a resource type of instance
. To tag
* instances at launch, specify the tags in a launch template.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ec2.model.TagSpecification.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.ec2.model.TagSpecification#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.ec2.model.TagSpecification.Builder#build()} is called immediately and
* its result is passed to {@link #tagSpecifications(List)}.
*
* @param tagSpecifications
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.ec2.model.TagSpecification.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tagSpecifications(java.util.Collection)
*/
Builder tagSpecifications(Consumer... tagSpecifications);
/**
*
* Reserved.
*
*
* @param context
* Reserved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder context(String context);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends Ec2Request.BuilderImpl implements Builder {
private Boolean dryRun;
private String clientToken;
private SpotOptionsRequest spotOptions;
private OnDemandOptionsRequest onDemandOptions;
private String excessCapacityTerminationPolicy;
private List launchTemplateConfigs = DefaultSdkAutoConstructList.getInstance();
private TargetCapacitySpecificationRequest targetCapacitySpecification;
private Boolean terminateInstancesWithExpiration;
private String type;
private Instant validFrom;
private Instant validUntil;
private Boolean replaceUnhealthyInstances;
private List tagSpecifications = DefaultSdkAutoConstructList.getInstance();
private String context;
private BuilderImpl() {
}
private BuilderImpl(CreateFleetRequest model) {
super(model);
dryRun(model.dryRun);
clientToken(model.clientToken);
spotOptions(model.spotOptions);
onDemandOptions(model.onDemandOptions);
excessCapacityTerminationPolicy(model.excessCapacityTerminationPolicy);
launchTemplateConfigs(model.launchTemplateConfigs);
targetCapacitySpecification(model.targetCapacitySpecification);
terminateInstancesWithExpiration(model.terminateInstancesWithExpiration);
type(model.type);
validFrom(model.validFrom);
validUntil(model.validUntil);
replaceUnhealthyInstances(model.replaceUnhealthyInstances);
tagSpecifications(model.tagSpecifications);
context(model.context);
}
public final Boolean getDryRun() {
return dryRun;
}
public final void setDryRun(Boolean dryRun) {
this.dryRun = dryRun;
}
@Override
public final Builder dryRun(Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
public final String getClientToken() {
return clientToken;
}
public final void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
@Override
public final Builder clientToken(String clientToken) {
this.clientToken = clientToken;
return this;
}
public final SpotOptionsRequest.Builder getSpotOptions() {
return spotOptions != null ? spotOptions.toBuilder() : null;
}
public final void setSpotOptions(SpotOptionsRequest.BuilderImpl spotOptions) {
this.spotOptions = spotOptions != null ? spotOptions.build() : null;
}
@Override
public final Builder spotOptions(SpotOptionsRequest spotOptions) {
this.spotOptions = spotOptions;
return this;
}
public final OnDemandOptionsRequest.Builder getOnDemandOptions() {
return onDemandOptions != null ? onDemandOptions.toBuilder() : null;
}
public final void setOnDemandOptions(OnDemandOptionsRequest.BuilderImpl onDemandOptions) {
this.onDemandOptions = onDemandOptions != null ? onDemandOptions.build() : null;
}
@Override
public final Builder onDemandOptions(OnDemandOptionsRequest onDemandOptions) {
this.onDemandOptions = onDemandOptions;
return this;
}
public final String getExcessCapacityTerminationPolicy() {
return excessCapacityTerminationPolicy;
}
public final void setExcessCapacityTerminationPolicy(String excessCapacityTerminationPolicy) {
this.excessCapacityTerminationPolicy = excessCapacityTerminationPolicy;
}
@Override
public final Builder excessCapacityTerminationPolicy(String excessCapacityTerminationPolicy) {
this.excessCapacityTerminationPolicy = excessCapacityTerminationPolicy;
return this;
}
@Override
public final Builder excessCapacityTerminationPolicy(FleetExcessCapacityTerminationPolicy excessCapacityTerminationPolicy) {
this.excessCapacityTerminationPolicy(excessCapacityTerminationPolicy == null ? null : excessCapacityTerminationPolicy
.toString());
return this;
}
public final List getLaunchTemplateConfigs() {
List result = FleetLaunchTemplateConfigListRequestCopier
.copyToBuilder(this.launchTemplateConfigs);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setLaunchTemplateConfigs(Collection launchTemplateConfigs) {
this.launchTemplateConfigs = FleetLaunchTemplateConfigListRequestCopier.copyFromBuilder(launchTemplateConfigs);
}
@Override
public final Builder launchTemplateConfigs(Collection launchTemplateConfigs) {
this.launchTemplateConfigs = FleetLaunchTemplateConfigListRequestCopier.copy(launchTemplateConfigs);
return this;
}
@Override
@SafeVarargs
public final Builder launchTemplateConfigs(FleetLaunchTemplateConfigRequest... launchTemplateConfigs) {
launchTemplateConfigs(Arrays.asList(launchTemplateConfigs));
return this;
}
@Override
@SafeVarargs
public final Builder launchTemplateConfigs(Consumer... launchTemplateConfigs) {
launchTemplateConfigs(Stream.of(launchTemplateConfigs)
.map(c -> FleetLaunchTemplateConfigRequest.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final TargetCapacitySpecificationRequest.Builder getTargetCapacitySpecification() {
return targetCapacitySpecification != null ? targetCapacitySpecification.toBuilder() : null;
}
public final void setTargetCapacitySpecification(
TargetCapacitySpecificationRequest.BuilderImpl targetCapacitySpecification) {
this.targetCapacitySpecification = targetCapacitySpecification != null ? targetCapacitySpecification.build() : null;
}
@Override
public final Builder targetCapacitySpecification(TargetCapacitySpecificationRequest targetCapacitySpecification) {
this.targetCapacitySpecification = targetCapacitySpecification;
return this;
}
public final Boolean getTerminateInstancesWithExpiration() {
return terminateInstancesWithExpiration;
}
public final void setTerminateInstancesWithExpiration(Boolean terminateInstancesWithExpiration) {
this.terminateInstancesWithExpiration = terminateInstancesWithExpiration;
}
@Override
public final Builder terminateInstancesWithExpiration(Boolean terminateInstancesWithExpiration) {
this.terminateInstancesWithExpiration = terminateInstancesWithExpiration;
return this;
}
public final String getType() {
return type;
}
public final void setType(String type) {
this.type = type;
}
@Override
public final Builder type(String type) {
this.type = type;
return this;
}
@Override
public final Builder type(FleetType type) {
this.type(type == null ? null : type.toString());
return this;
}
public final Instant getValidFrom() {
return validFrom;
}
public final void setValidFrom(Instant validFrom) {
this.validFrom = validFrom;
}
@Override
public final Builder validFrom(Instant validFrom) {
this.validFrom = validFrom;
return this;
}
public final Instant getValidUntil() {
return validUntil;
}
public final void setValidUntil(Instant validUntil) {
this.validUntil = validUntil;
}
@Override
public final Builder validUntil(Instant validUntil) {
this.validUntil = validUntil;
return this;
}
public final Boolean getReplaceUnhealthyInstances() {
return replaceUnhealthyInstances;
}
public final void setReplaceUnhealthyInstances(Boolean replaceUnhealthyInstances) {
this.replaceUnhealthyInstances = replaceUnhealthyInstances;
}
@Override
public final Builder replaceUnhealthyInstances(Boolean replaceUnhealthyInstances) {
this.replaceUnhealthyInstances = replaceUnhealthyInstances;
return this;
}
public final List getTagSpecifications() {
List result = TagSpecificationListCopier.copyToBuilder(this.tagSpecifications);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTagSpecifications(Collection tagSpecifications) {
this.tagSpecifications = TagSpecificationListCopier.copyFromBuilder(tagSpecifications);
}
@Override
public final Builder tagSpecifications(Collection tagSpecifications) {
this.tagSpecifications = TagSpecificationListCopier.copy(tagSpecifications);
return this;
}
@Override
@SafeVarargs
public final Builder tagSpecifications(TagSpecification... tagSpecifications) {
tagSpecifications(Arrays.asList(tagSpecifications));
return this;
}
@Override
@SafeVarargs
public final Builder tagSpecifications(Consumer... tagSpecifications) {
tagSpecifications(Stream.of(tagSpecifications).map(c -> TagSpecification.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final String getContext() {
return context;
}
public final void setContext(String context) {
this.context = context;
}
@Override
public final Builder context(String context) {
this.context = context;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateFleetRequest build() {
return new CreateFleetRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}