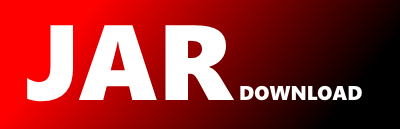
software.amazon.awssdk.services.ec2.model.CreateFlowLogsRequest Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateFlowLogsRequest extends Ec2Request implements
ToCopyableBuilder {
private static final SdkField DRY_RUN_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DryRun")
.getter(getter(CreateFlowLogsRequest::dryRun))
.setter(setter(Builder::dryRun))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DryRun")
.unmarshallLocationName("DryRun").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientToken")
.getter(getter(CreateFlowLogsRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken")
.unmarshallLocationName("ClientToken").build()).build();
private static final SdkField DELIVER_LOGS_PERMISSION_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DeliverLogsPermissionArn")
.getter(getter(CreateFlowLogsRequest::deliverLogsPermissionArn))
.setter(setter(Builder::deliverLogsPermissionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeliverLogsPermissionArn")
.unmarshallLocationName("DeliverLogsPermissionArn").build()).build();
private static final SdkField DELIVER_CROSS_ACCOUNT_ROLE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DeliverCrossAccountRole")
.getter(getter(CreateFlowLogsRequest::deliverCrossAccountRole))
.setter(setter(Builder::deliverCrossAccountRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeliverCrossAccountRole")
.unmarshallLocationName("DeliverCrossAccountRole").build()).build();
private static final SdkField LOG_GROUP_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LogGroupName")
.getter(getter(CreateFlowLogsRequest::logGroupName))
.setter(setter(Builder::logGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogGroupName")
.unmarshallLocationName("LogGroupName").build()).build();
private static final SdkField> RESOURCE_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ResourceIds")
.getter(getter(CreateFlowLogsRequest::resourceIds))
.setter(setter(Builder::resourceIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceId")
.unmarshallLocationName("ResourceId").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ResourceType")
.getter(getter(CreateFlowLogsRequest::resourceTypeAsString))
.setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType")
.unmarshallLocationName("ResourceType").build()).build();
private static final SdkField TRAFFIC_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("TrafficType")
.getter(getter(CreateFlowLogsRequest::trafficTypeAsString))
.setter(setter(Builder::trafficType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TrafficType")
.unmarshallLocationName("TrafficType").build()).build();
private static final SdkField LOG_DESTINATION_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LogDestinationType")
.getter(getter(CreateFlowLogsRequest::logDestinationTypeAsString))
.setter(setter(Builder::logDestinationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogDestinationType")
.unmarshallLocationName("LogDestinationType").build()).build();
private static final SdkField LOG_DESTINATION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LogDestination")
.getter(getter(CreateFlowLogsRequest::logDestination))
.setter(setter(Builder::logDestination))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogDestination")
.unmarshallLocationName("LogDestination").build()).build();
private static final SdkField LOG_FORMAT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LogFormat")
.getter(getter(CreateFlowLogsRequest::logFormat))
.setter(setter(Builder::logFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogFormat")
.unmarshallLocationName("LogFormat").build()).build();
private static final SdkField> TAG_SPECIFICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TagSpecifications")
.getter(getter(CreateFlowLogsRequest::tagSpecifications))
.setter(setter(Builder::tagSpecifications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TagSpecification")
.unmarshallLocationName("TagSpecification").build(),
ListTrait
.builder()
.memberLocationName("item")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TagSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Item").unmarshallLocationName("item").build()).build())
.build()).build();
private static final SdkField MAX_AGGREGATION_INTERVAL_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaxAggregationInterval")
.getter(getter(CreateFlowLogsRequest::maxAggregationInterval))
.setter(setter(Builder::maxAggregationInterval))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxAggregationInterval")
.unmarshallLocationName("MaxAggregationInterval").build()).build();
private static final SdkField DESTINATION_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("DestinationOptions")
.getter(getter(CreateFlowLogsRequest::destinationOptions))
.setter(setter(Builder::destinationOptions))
.constructor(DestinationOptionsRequest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DestinationOptions")
.unmarshallLocationName("DestinationOptions").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DRY_RUN_FIELD,
CLIENT_TOKEN_FIELD, DELIVER_LOGS_PERMISSION_ARN_FIELD, DELIVER_CROSS_ACCOUNT_ROLE_FIELD, LOG_GROUP_NAME_FIELD,
RESOURCE_IDS_FIELD, RESOURCE_TYPE_FIELD, TRAFFIC_TYPE_FIELD, LOG_DESTINATION_TYPE_FIELD, LOG_DESTINATION_FIELD,
LOG_FORMAT_FIELD, TAG_SPECIFICATIONS_FIELD, MAX_AGGREGATION_INTERVAL_FIELD, DESTINATION_OPTIONS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("DryRun", DRY_RUN_FIELD);
put("ClientToken", CLIENT_TOKEN_FIELD);
put("DeliverLogsPermissionArn", DELIVER_LOGS_PERMISSION_ARN_FIELD);
put("DeliverCrossAccountRole", DELIVER_CROSS_ACCOUNT_ROLE_FIELD);
put("LogGroupName", LOG_GROUP_NAME_FIELD);
put("ResourceId", RESOURCE_IDS_FIELD);
put("ResourceType", RESOURCE_TYPE_FIELD);
put("TrafficType", TRAFFIC_TYPE_FIELD);
put("LogDestinationType", LOG_DESTINATION_TYPE_FIELD);
put("LogDestination", LOG_DESTINATION_FIELD);
put("LogFormat", LOG_FORMAT_FIELD);
put("TagSpecification", TAG_SPECIFICATIONS_FIELD);
put("MaxAggregationInterval", MAX_AGGREGATION_INTERVAL_FIELD);
put("DestinationOptions", DESTINATION_OPTIONS_FIELD);
}
});
private final Boolean dryRun;
private final String clientToken;
private final String deliverLogsPermissionArn;
private final String deliverCrossAccountRole;
private final String logGroupName;
private final List resourceIds;
private final String resourceType;
private final String trafficType;
private final String logDestinationType;
private final String logDestination;
private final String logFormat;
private final List tagSpecifications;
private final Integer maxAggregationInterval;
private final DestinationOptionsRequest destinationOptions;
private CreateFlowLogsRequest(BuilderImpl builder) {
super(builder);
this.dryRun = builder.dryRun;
this.clientToken = builder.clientToken;
this.deliverLogsPermissionArn = builder.deliverLogsPermissionArn;
this.deliverCrossAccountRole = builder.deliverCrossAccountRole;
this.logGroupName = builder.logGroupName;
this.resourceIds = builder.resourceIds;
this.resourceType = builder.resourceType;
this.trafficType = builder.trafficType;
this.logDestinationType = builder.logDestinationType;
this.logDestination = builder.logDestination;
this.logFormat = builder.logFormat;
this.tagSpecifications = builder.tagSpecifications;
this.maxAggregationInterval = builder.maxAggregationInterval;
this.destinationOptions = builder.destinationOptions;
}
/**
*
* Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*
*
* @return Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*/
public final Boolean dryRun() {
return dryRun;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see How to ensure
* idempotency.
*
*
* @return Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see How
* to ensure idempotency.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* The ARN of the IAM role that allows Amazon EC2 to publish flow logs to the log destination.
*
*
* This parameter is required if the destination type is cloud-watch-logs
, or if the destination type
* is kinesis-data-firehose
and the delivery stream and the resources to monitor are in different
* accounts.
*
*
* @return The ARN of the IAM role that allows Amazon EC2 to publish flow logs to the log destination.
*
* This parameter is required if the destination type is cloud-watch-logs
, or if the
* destination type is kinesis-data-firehose
and the delivery stream and the resources to
* monitor are in different accounts.
*/
public final String deliverLogsPermissionArn() {
return deliverLogsPermissionArn;
}
/**
*
* The ARN of the IAM role that allows Amazon EC2 to publish flow logs across accounts.
*
*
* @return The ARN of the IAM role that allows Amazon EC2 to publish flow logs across accounts.
*/
public final String deliverCrossAccountRole() {
return deliverCrossAccountRole;
}
/**
*
* The name of a new or existing CloudWatch Logs log group where Amazon EC2 publishes your flow logs.
*
*
* This parameter is valid only if the destination type is cloud-watch-logs
.
*
*
* @return The name of a new or existing CloudWatch Logs log group where Amazon EC2 publishes your flow logs.
*
* This parameter is valid only if the destination type is cloud-watch-logs
.
*/
public final String logGroupName() {
return logGroupName;
}
/**
* For responses, this returns true if the service returned a value for the ResourceIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasResourceIds() {
return resourceIds != null && !(resourceIds instanceof SdkAutoConstructList);
}
/**
*
* The IDs of the resources to monitor. For example, if the resource type is VPC
, specify the IDs of
* the VPCs.
*
*
* Constraints: Maximum of 25 for transit gateway resource types. Maximum of 1000 for the other resource types.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasResourceIds} method.
*
*
* @return The IDs of the resources to monitor. For example, if the resource type is VPC
, specify the
* IDs of the VPCs.
*
* Constraints: Maximum of 25 for transit gateway resource types. Maximum of 1000 for the other resource
* types.
*/
public final List resourceIds() {
return resourceIds;
}
/**
*
* The type of resource to monitor.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link FlowLogsResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceTypeAsString}.
*
*
* @return The type of resource to monitor.
* @see FlowLogsResourceType
*/
public final FlowLogsResourceType resourceType() {
return FlowLogsResourceType.fromValue(resourceType);
}
/**
*
* The type of resource to monitor.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link FlowLogsResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceTypeAsString}.
*
*
* @return The type of resource to monitor.
* @see FlowLogsResourceType
*/
public final String resourceTypeAsString() {
return resourceType;
}
/**
*
* The type of traffic to monitor (accepted traffic, rejected traffic, or all traffic). This parameter is not
* supported for transit gateway resource types. It is required for the other resource types.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #trafficType} will
* return {@link TrafficType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #trafficTypeAsString}.
*
*
* @return The type of traffic to monitor (accepted traffic, rejected traffic, or all traffic). This parameter is
* not supported for transit gateway resource types. It is required for the other resource types.
* @see TrafficType
*/
public final TrafficType trafficType() {
return TrafficType.fromValue(trafficType);
}
/**
*
* The type of traffic to monitor (accepted traffic, rejected traffic, or all traffic). This parameter is not
* supported for transit gateway resource types. It is required for the other resource types.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #trafficType} will
* return {@link TrafficType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #trafficTypeAsString}.
*
*
* @return The type of traffic to monitor (accepted traffic, rejected traffic, or all traffic). This parameter is
* not supported for transit gateway resource types. It is required for the other resource types.
* @see TrafficType
*/
public final String trafficTypeAsString() {
return trafficType;
}
/**
*
* The type of destination for the flow log data.
*
*
* Default: cloud-watch-logs
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #logDestinationType} will return {@link LogDestinationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #logDestinationTypeAsString}.
*
*
* @return The type of destination for the flow log data.
*
* Default: cloud-watch-logs
* @see LogDestinationType
*/
public final LogDestinationType logDestinationType() {
return LogDestinationType.fromValue(logDestinationType);
}
/**
*
* The type of destination for the flow log data.
*
*
* Default: cloud-watch-logs
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #logDestinationType} will return {@link LogDestinationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #logDestinationTypeAsString}.
*
*
* @return The type of destination for the flow log data.
*
* Default: cloud-watch-logs
* @see LogDestinationType
*/
public final String logDestinationTypeAsString() {
return logDestinationType;
}
/**
*
* The destination for the flow log data. The meaning of this parameter depends on the destination type.
*
*
* -
*
* If the destination type is cloud-watch-logs
, specify the ARN of a CloudWatch Logs log group. For
* example:
*
*
* arn:aws:logs:region:account_id:log-group:my_group
*
*
* Alternatively, use the LogGroupName
parameter.
*
*
* -
*
* If the destination type is s3
, specify the ARN of an S3 bucket. For example:
*
*
* arn:aws:s3:::my_bucket/my_subfolder/
*
*
* The subfolder is optional. Note that you can't use AWSLogs
as a subfolder name.
*
*
* -
*
* If the destination type is kinesis-data-firehose
, specify the ARN of a Kinesis Data Firehose
* delivery stream. For example:
*
*
* arn:aws:firehose:region:account_id:deliverystream:my_stream
*
*
*
*
* @return The destination for the flow log data. The meaning of this parameter depends on the destination type.
*
* -
*
* If the destination type is cloud-watch-logs
, specify the ARN of a CloudWatch Logs log group.
* For example:
*
*
* arn:aws:logs:region:account_id:log-group:my_group
*
*
* Alternatively, use the LogGroupName
parameter.
*
*
* -
*
* If the destination type is s3
, specify the ARN of an S3 bucket. For example:
*
*
* arn:aws:s3:::my_bucket/my_subfolder/
*
*
* The subfolder is optional. Note that you can't use AWSLogs
as a subfolder name.
*
*
* -
*
* If the destination type is kinesis-data-firehose
, specify the ARN of a Kinesis Data Firehose
* delivery stream. For example:
*
*
* arn:aws:firehose:region:account_id:deliverystream:my_stream
*
*
*/
public final String logDestination() {
return logDestination;
}
/**
*
* The fields to include in the flow log record. List the fields in the order in which they should appear. If you
* omit this parameter, the flow log is created using the default format. If you specify this parameter, you must
* include at least one field. For more information about the available fields, see Flow log records in the
* Amazon VPC User Guide or Transit Gateway Flow Log
* records in the Amazon Web Services Transit Gateway Guide.
*
*
* Specify the fields using the ${field-id}
format, separated by spaces.
*
*
* @return The fields to include in the flow log record. List the fields in the order in which they should appear.
* If you omit this parameter, the flow log is created using the default format. If you specify this
* parameter, you must include at least one field. For more information about the available fields, see Flow log records in the
* Amazon VPC User Guide or Transit Gateway
* Flow Log records in the Amazon Web Services Transit Gateway Guide.
*
* Specify the fields using the ${field-id}
format, separated by spaces.
*/
public final String logFormat() {
return logFormat;
}
/**
* For responses, this returns true if the service returned a value for the TagSpecifications property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTagSpecifications() {
return tagSpecifications != null && !(tagSpecifications instanceof SdkAutoConstructList);
}
/**
*
* The tags to apply to the flow logs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagSpecifications} method.
*
*
* @return The tags to apply to the flow logs.
*/
public final List tagSpecifications() {
return tagSpecifications;
}
/**
*
* The maximum interval of time during which a flow of packets is captured and aggregated into a flow log record.
* The possible values are 60 seconds (1 minute) or 600 seconds (10 minutes). This parameter must be 60 seconds for
* transit gateway resource types.
*
*
* When a network interface is attached to a Nitro-based instance,
* the aggregation interval is always 60 seconds or less, regardless of the value that you specify.
*
*
* Default: 600
*
*
* @return The maximum interval of time during which a flow of packets is captured and aggregated into a flow log
* record. The possible values are 60 seconds (1 minute) or 600 seconds (10 minutes). This parameter must be
* 60 seconds for transit gateway resource types.
*
* When a network interface is attached to a Nitro-based
* instance, the aggregation interval is always 60 seconds or less, regardless of the value that you
* specify.
*
*
* Default: 600
*/
public final Integer maxAggregationInterval() {
return maxAggregationInterval;
}
/**
*
* The destination options.
*
*
* @return The destination options.
*/
public final DestinationOptionsRequest destinationOptions() {
return destinationOptions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dryRun());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(deliverLogsPermissionArn());
hashCode = 31 * hashCode + Objects.hashCode(deliverCrossAccountRole());
hashCode = 31 * hashCode + Objects.hashCode(logGroupName());
hashCode = 31 * hashCode + Objects.hashCode(hasResourceIds() ? resourceIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(resourceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(trafficTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(logDestinationTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(logDestination());
hashCode = 31 * hashCode + Objects.hashCode(logFormat());
hashCode = 31 * hashCode + Objects.hashCode(hasTagSpecifications() ? tagSpecifications() : null);
hashCode = 31 * hashCode + Objects.hashCode(maxAggregationInterval());
hashCode = 31 * hashCode + Objects.hashCode(destinationOptions());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateFlowLogsRequest)) {
return false;
}
CreateFlowLogsRequest other = (CreateFlowLogsRequest) obj;
return Objects.equals(dryRun(), other.dryRun()) && Objects.equals(clientToken(), other.clientToken())
&& Objects.equals(deliverLogsPermissionArn(), other.deliverLogsPermissionArn())
&& Objects.equals(deliverCrossAccountRole(), other.deliverCrossAccountRole())
&& Objects.equals(logGroupName(), other.logGroupName()) && hasResourceIds() == other.hasResourceIds()
&& Objects.equals(resourceIds(), other.resourceIds())
&& Objects.equals(resourceTypeAsString(), other.resourceTypeAsString())
&& Objects.equals(trafficTypeAsString(), other.trafficTypeAsString())
&& Objects.equals(logDestinationTypeAsString(), other.logDestinationTypeAsString())
&& Objects.equals(logDestination(), other.logDestination()) && Objects.equals(logFormat(), other.logFormat())
&& hasTagSpecifications() == other.hasTagSpecifications()
&& Objects.equals(tagSpecifications(), other.tagSpecifications())
&& Objects.equals(maxAggregationInterval(), other.maxAggregationInterval())
&& Objects.equals(destinationOptions(), other.destinationOptions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateFlowLogsRequest").add("DryRun", dryRun()).add("ClientToken", clientToken())
.add("DeliverLogsPermissionArn", deliverLogsPermissionArn())
.add("DeliverCrossAccountRole", deliverCrossAccountRole()).add("LogGroupName", logGroupName())
.add("ResourceIds", hasResourceIds() ? resourceIds() : null).add("ResourceType", resourceTypeAsString())
.add("TrafficType", trafficTypeAsString()).add("LogDestinationType", logDestinationTypeAsString())
.add("LogDestination", logDestination()).add("LogFormat", logFormat())
.add("TagSpecifications", hasTagSpecifications() ? tagSpecifications() : null)
.add("MaxAggregationInterval", maxAggregationInterval()).add("DestinationOptions", destinationOptions()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DryRun":
return Optional.ofNullable(clazz.cast(dryRun()));
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "DeliverLogsPermissionArn":
return Optional.ofNullable(clazz.cast(deliverLogsPermissionArn()));
case "DeliverCrossAccountRole":
return Optional.ofNullable(clazz.cast(deliverCrossAccountRole()));
case "LogGroupName":
return Optional.ofNullable(clazz.cast(logGroupName()));
case "ResourceIds":
return Optional.ofNullable(clazz.cast(resourceIds()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceTypeAsString()));
case "TrafficType":
return Optional.ofNullable(clazz.cast(trafficTypeAsString()));
case "LogDestinationType":
return Optional.ofNullable(clazz.cast(logDestinationTypeAsString()));
case "LogDestination":
return Optional.ofNullable(clazz.cast(logDestination()));
case "LogFormat":
return Optional.ofNullable(clazz.cast(logFormat()));
case "TagSpecifications":
return Optional.ofNullable(clazz.cast(tagSpecifications()));
case "MaxAggregationInterval":
return Optional.ofNullable(clazz.cast(maxAggregationInterval()));
case "DestinationOptions":
return Optional.ofNullable(clazz.cast(destinationOptions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function