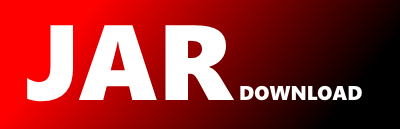
software.amazon.awssdk.services.ec2.model.CreateLaunchTemplateVersionRequest Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateLaunchTemplateVersionRequest extends Ec2Request implements
ToCopyableBuilder {
private static final SdkField DRY_RUN_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("DryRun")
.getter(getter(CreateLaunchTemplateVersionRequest::dryRun))
.setter(setter(Builder::dryRun))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DryRun")
.unmarshallLocationName("DryRun").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ClientToken")
.getter(getter(CreateLaunchTemplateVersionRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientToken")
.unmarshallLocationName("ClientToken").build()).build();
private static final SdkField LAUNCH_TEMPLATE_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LaunchTemplateId")
.getter(getter(CreateLaunchTemplateVersionRequest::launchTemplateId))
.setter(setter(Builder::launchTemplateId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchTemplateId")
.unmarshallLocationName("LaunchTemplateId").build()).build();
private static final SdkField LAUNCH_TEMPLATE_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("LaunchTemplateName")
.getter(getter(CreateLaunchTemplateVersionRequest::launchTemplateName))
.setter(setter(Builder::launchTemplateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchTemplateName")
.unmarshallLocationName("LaunchTemplateName").build()).build();
private static final SdkField SOURCE_VERSION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SourceVersion")
.getter(getter(CreateLaunchTemplateVersionRequest::sourceVersion))
.setter(setter(Builder::sourceVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceVersion")
.unmarshallLocationName("SourceVersion").build()).build();
private static final SdkField VERSION_DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("VersionDescription")
.getter(getter(CreateLaunchTemplateVersionRequest::versionDescription))
.setter(setter(Builder::versionDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionDescription")
.unmarshallLocationName("VersionDescription").build()).build();
private static final SdkField LAUNCH_TEMPLATE_DATA_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("LaunchTemplateData")
.getter(getter(CreateLaunchTemplateVersionRequest::launchTemplateData))
.setter(setter(Builder::launchTemplateData))
.constructor(RequestLaunchTemplateData::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchTemplateData")
.unmarshallLocationName("LaunchTemplateData").build()).build();
private static final SdkField RESOLVE_ALIAS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("ResolveAlias")
.getter(getter(CreateLaunchTemplateVersionRequest::resolveAlias))
.setter(setter(Builder::resolveAlias))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResolveAlias")
.unmarshallLocationName("ResolveAlias").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DRY_RUN_FIELD,
CLIENT_TOKEN_FIELD, LAUNCH_TEMPLATE_ID_FIELD, LAUNCH_TEMPLATE_NAME_FIELD, SOURCE_VERSION_FIELD,
VERSION_DESCRIPTION_FIELD, LAUNCH_TEMPLATE_DATA_FIELD, RESOLVE_ALIAS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("DryRun", DRY_RUN_FIELD);
put("ClientToken", CLIENT_TOKEN_FIELD);
put("LaunchTemplateId", LAUNCH_TEMPLATE_ID_FIELD);
put("LaunchTemplateName", LAUNCH_TEMPLATE_NAME_FIELD);
put("SourceVersion", SOURCE_VERSION_FIELD);
put("VersionDescription", VERSION_DESCRIPTION_FIELD);
put("LaunchTemplateData", LAUNCH_TEMPLATE_DATA_FIELD);
put("ResolveAlias", RESOLVE_ALIAS_FIELD);
}
});
private final Boolean dryRun;
private final String clientToken;
private final String launchTemplateId;
private final String launchTemplateName;
private final String sourceVersion;
private final String versionDescription;
private final RequestLaunchTemplateData launchTemplateData;
private final Boolean resolveAlias;
private CreateLaunchTemplateVersionRequest(BuilderImpl builder) {
super(builder);
this.dryRun = builder.dryRun;
this.clientToken = builder.clientToken;
this.launchTemplateId = builder.launchTemplateId;
this.launchTemplateName = builder.launchTemplateName;
this.sourceVersion = builder.sourceVersion;
this.versionDescription = builder.versionDescription;
this.launchTemplateData = builder.launchTemplateData;
this.resolveAlias = builder.resolveAlias;
}
/**
*
* Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*
*
* @return Checks whether you have the required permissions for the action, without actually making the request, and
* provides an error response. If you have the required permissions, the error response is
* DryRunOperation
. Otherwise, it is UnauthorizedOperation
.
*/
public final Boolean dryRun() {
return dryRun;
}
/**
*
* Unique, case-sensitive identifier you provide to ensure the idempotency of the request. For more information, see
* Ensuring
* idempotency.
*
*
* Constraint: Maximum 128 ASCII characters.
*
*
* @return Unique, case-sensitive identifier you provide to ensure the idempotency of the request. For more
* information, see Ensuring
* idempotency.
*
* Constraint: Maximum 128 ASCII characters.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* The ID of the launch template.
*
*
* You must specify either the launch template ID or the launch template name, but not both.
*
*
* @return The ID of the launch template.
*
* You must specify either the launch template ID or the launch template name, but not both.
*/
public final String launchTemplateId() {
return launchTemplateId;
}
/**
*
* The name of the launch template.
*
*
* You must specify either the launch template ID or the launch template name, but not both.
*
*
* @return The name of the launch template.
*
* You must specify either the launch template ID or the launch template name, but not both.
*/
public final String launchTemplateName() {
return launchTemplateName;
}
/**
*
* The version of the launch template on which to base the new version. Snapshots applied to the block device
* mapping are ignored when creating a new version unless they are explicitly included.
*
*
* If you specify this parameter, the new version inherits the launch parameters from the source version. If you
* specify additional launch parameters for the new version, they overwrite any corresponding launch parameters
* inherited from the source version.
*
*
* If you omit this parameter, the new version contains only the launch parameters that you specify for the new
* version.
*
*
* @return The version of the launch template on which to base the new version. Snapshots applied to the block
* device mapping are ignored when creating a new version unless they are explicitly included.
*
* If you specify this parameter, the new version inherits the launch parameters from the source version. If
* you specify additional launch parameters for the new version, they overwrite any corresponding launch
* parameters inherited from the source version.
*
*
* If you omit this parameter, the new version contains only the launch parameters that you specify for the
* new version.
*/
public final String sourceVersion() {
return sourceVersion;
}
/**
*
* A description for the version of the launch template.
*
*
* @return A description for the version of the launch template.
*/
public final String versionDescription() {
return versionDescription;
}
/**
*
* The information for the launch template.
*
*
* @return The information for the launch template.
*/
public final RequestLaunchTemplateData launchTemplateData() {
return launchTemplateData;
}
/**
*
* If true
, and if a Systems Manager parameter is specified for ImageId
, the AMI ID is
* displayed in the response for imageID
. For more information, see Use a Systems Manager parameter instead of an AMI ID in the Amazon EC2 User Guide.
*
*
* Default: false
*
*
* @return If true
, and if a Systems Manager parameter is specified for ImageId
, the AMI
* ID is displayed in the response for imageID
. For more information, see Use a Systems Manager parameter instead of an AMI ID in the Amazon EC2 User Guide.
*
* Default: false
*/
public final Boolean resolveAlias() {
return resolveAlias;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dryRun());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(launchTemplateId());
hashCode = 31 * hashCode + Objects.hashCode(launchTemplateName());
hashCode = 31 * hashCode + Objects.hashCode(sourceVersion());
hashCode = 31 * hashCode + Objects.hashCode(versionDescription());
hashCode = 31 * hashCode + Objects.hashCode(launchTemplateData());
hashCode = 31 * hashCode + Objects.hashCode(resolveAlias());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateLaunchTemplateVersionRequest)) {
return false;
}
CreateLaunchTemplateVersionRequest other = (CreateLaunchTemplateVersionRequest) obj;
return Objects.equals(dryRun(), other.dryRun()) && Objects.equals(clientToken(), other.clientToken())
&& Objects.equals(launchTemplateId(), other.launchTemplateId())
&& Objects.equals(launchTemplateName(), other.launchTemplateName())
&& Objects.equals(sourceVersion(), other.sourceVersion())
&& Objects.equals(versionDescription(), other.versionDescription())
&& Objects.equals(launchTemplateData(), other.launchTemplateData())
&& Objects.equals(resolveAlias(), other.resolveAlias());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateLaunchTemplateVersionRequest").add("DryRun", dryRun()).add("ClientToken", clientToken())
.add("LaunchTemplateId", launchTemplateId()).add("LaunchTemplateName", launchTemplateName())
.add("SourceVersion", sourceVersion()).add("VersionDescription", versionDescription())
.add("LaunchTemplateData", launchTemplateData()).add("ResolveAlias", resolveAlias()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DryRun":
return Optional.ofNullable(clazz.cast(dryRun()));
case "ClientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "LaunchTemplateId":
return Optional.ofNullable(clazz.cast(launchTemplateId()));
case "LaunchTemplateName":
return Optional.ofNullable(clazz.cast(launchTemplateName()));
case "SourceVersion":
return Optional.ofNullable(clazz.cast(sourceVersion()));
case "VersionDescription":
return Optional.ofNullable(clazz.cast(versionDescription()));
case "LaunchTemplateData":
return Optional.ofNullable(clazz.cast(launchTemplateData()));
case "ResolveAlias":
return Optional.ofNullable(clazz.cast(resolveAlias()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* Constraint: Maximum 128 ASCII characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientToken(String clientToken);
/**
*
* The ID of the launch template.
*
*
* You must specify either the launch template ID or the launch template name, but not both.
*
*
* @param launchTemplateId
* The ID of the launch template.
*
* You must specify either the launch template ID or the launch template name, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder launchTemplateId(String launchTemplateId);
/**
*
* The name of the launch template.
*
*
* You must specify either the launch template ID or the launch template name, but not both.
*
*
* @param launchTemplateName
* The name of the launch template.
*
* You must specify either the launch template ID or the launch template name, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder launchTemplateName(String launchTemplateName);
/**
*
* The version of the launch template on which to base the new version. Snapshots applied to the block device
* mapping are ignored when creating a new version unless they are explicitly included.
*
*
* If you specify this parameter, the new version inherits the launch parameters from the source version. If you
* specify additional launch parameters for the new version, they overwrite any corresponding launch parameters
* inherited from the source version.
*
*
* If you omit this parameter, the new version contains only the launch parameters that you specify for the new
* version.
*
*
* @param sourceVersion
* The version of the launch template on which to base the new version. Snapshots applied to the block
* device mapping are ignored when creating a new version unless they are explicitly included.
*
* If you specify this parameter, the new version inherits the launch parameters from the source version.
* If you specify additional launch parameters for the new version, they overwrite any corresponding
* launch parameters inherited from the source version.
*
*
* If you omit this parameter, the new version contains only the launch parameters that you specify for
* the new version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sourceVersion(String sourceVersion);
/**
*
* A description for the version of the launch template.
*
*
* @param versionDescription
* A description for the version of the launch template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder versionDescription(String versionDescription);
/**
*
* The information for the launch template.
*
*
* @param launchTemplateData
* The information for the launch template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder launchTemplateData(RequestLaunchTemplateData launchTemplateData);
/**
*
* The information for the launch template.
*
* This is a convenience method that creates an instance of the {@link RequestLaunchTemplateData.Builder}
* avoiding the need to create one manually via {@link RequestLaunchTemplateData#builder()}.
*
*
* When the {@link Consumer} completes, {@link RequestLaunchTemplateData.Builder#build()} is called immediately
* and its result is passed to {@link #launchTemplateData(RequestLaunchTemplateData)}.
*
* @param launchTemplateData
* a consumer that will call methods on {@link RequestLaunchTemplateData.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #launchTemplateData(RequestLaunchTemplateData)
*/
default Builder launchTemplateData(Consumer launchTemplateData) {
return launchTemplateData(RequestLaunchTemplateData.builder().applyMutation(launchTemplateData).build());
}
/**
*
* If true
, and if a Systems Manager parameter is specified for ImageId
, the AMI ID is
* displayed in the response for imageID
. For more information, see Use a Systems Manager parameter instead of an AMI ID in the Amazon EC2 User Guide.
*
*
* Default: false
*
*
* @param resolveAlias
* If true
, and if a Systems Manager parameter is specified for ImageId
, the
* AMI ID is displayed in the response for imageID
. For more information, see Use a Systems Manager parameter instead of an AMI ID in the Amazon EC2 User Guide.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder resolveAlias(Boolean resolveAlias);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends Ec2Request.BuilderImpl implements Builder {
private Boolean dryRun;
private String clientToken;
private String launchTemplateId;
private String launchTemplateName;
private String sourceVersion;
private String versionDescription;
private RequestLaunchTemplateData launchTemplateData;
private Boolean resolveAlias;
private BuilderImpl() {
}
private BuilderImpl(CreateLaunchTemplateVersionRequest model) {
super(model);
dryRun(model.dryRun);
clientToken(model.clientToken);
launchTemplateId(model.launchTemplateId);
launchTemplateName(model.launchTemplateName);
sourceVersion(model.sourceVersion);
versionDescription(model.versionDescription);
launchTemplateData(model.launchTemplateData);
resolveAlias(model.resolveAlias);
}
public final Boolean getDryRun() {
return dryRun;
}
public final void setDryRun(Boolean dryRun) {
this.dryRun = dryRun;
}
@Override
public final Builder dryRun(Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
public final String getClientToken() {
return clientToken;
}
public final void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
@Override
public final Builder clientToken(String clientToken) {
this.clientToken = clientToken;
return this;
}
public final String getLaunchTemplateId() {
return launchTemplateId;
}
public final void setLaunchTemplateId(String launchTemplateId) {
this.launchTemplateId = launchTemplateId;
}
@Override
public final Builder launchTemplateId(String launchTemplateId) {
this.launchTemplateId = launchTemplateId;
return this;
}
public final String getLaunchTemplateName() {
return launchTemplateName;
}
public final void setLaunchTemplateName(String launchTemplateName) {
this.launchTemplateName = launchTemplateName;
}
@Override
public final Builder launchTemplateName(String launchTemplateName) {
this.launchTemplateName = launchTemplateName;
return this;
}
public final String getSourceVersion() {
return sourceVersion;
}
public final void setSourceVersion(String sourceVersion) {
this.sourceVersion = sourceVersion;
}
@Override
public final Builder sourceVersion(String sourceVersion) {
this.sourceVersion = sourceVersion;
return this;
}
public final String getVersionDescription() {
return versionDescription;
}
public final void setVersionDescription(String versionDescription) {
this.versionDescription = versionDescription;
}
@Override
public final Builder versionDescription(String versionDescription) {
this.versionDescription = versionDescription;
return this;
}
public final RequestLaunchTemplateData.Builder getLaunchTemplateData() {
return launchTemplateData != null ? launchTemplateData.toBuilder() : null;
}
public final void setLaunchTemplateData(RequestLaunchTemplateData.BuilderImpl launchTemplateData) {
this.launchTemplateData = launchTemplateData != null ? launchTemplateData.build() : null;
}
@Override
public final Builder launchTemplateData(RequestLaunchTemplateData launchTemplateData) {
this.launchTemplateData = launchTemplateData;
return this;
}
public final Boolean getResolveAlias() {
return resolveAlias;
}
public final void setResolveAlias(Boolean resolveAlias) {
this.resolveAlias = resolveAlias;
}
@Override
public final Builder resolveAlias(Boolean resolveAlias) {
this.resolveAlias = resolveAlias;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateLaunchTemplateVersionRequest build() {
return new CreateLaunchTemplateVersionRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}