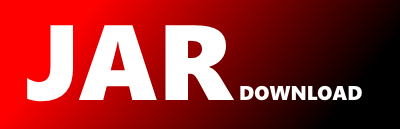
software.amazon.awssdk.services.ec2.model.CreateTransitGatewayMulticastDomainRequestOptions Maven / Gradle / Ivy
Show all versions of ec2 Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ec2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The options for the transit gateway multicast domain.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateTransitGatewayMulticastDomainRequestOptions
implements
SdkPojo,
Serializable,
ToCopyableBuilder {
private static final SdkField IGMPV2_SUPPORT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("Igmpv2Support")
.getter(getter(CreateTransitGatewayMulticastDomainRequestOptions::igmpv2SupportAsString))
.setter(setter(Builder::igmpv2Support))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Igmpv2Support")
.unmarshallLocationName("Igmpv2Support").build()).build();
private static final SdkField STATIC_SOURCES_SUPPORT_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("StaticSourcesSupport")
.getter(getter(CreateTransitGatewayMulticastDomainRequestOptions::staticSourcesSupportAsString))
.setter(setter(Builder::staticSourcesSupport))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StaticSourcesSupport")
.unmarshallLocationName("StaticSourcesSupport").build()).build();
private static final SdkField AUTO_ACCEPT_SHARED_ASSOCIATIONS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("AutoAcceptSharedAssociations")
.getter(getter(CreateTransitGatewayMulticastDomainRequestOptions::autoAcceptSharedAssociationsAsString))
.setter(setter(Builder::autoAcceptSharedAssociations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoAcceptSharedAssociations")
.unmarshallLocationName("AutoAcceptSharedAssociations").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(IGMPV2_SUPPORT_FIELD,
STATIC_SOURCES_SUPPORT_FIELD, AUTO_ACCEPT_SHARED_ASSOCIATIONS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Igmpv2Support", IGMPV2_SUPPORT_FIELD);
put("StaticSourcesSupport", STATIC_SOURCES_SUPPORT_FIELD);
put("AutoAcceptSharedAssociations", AUTO_ACCEPT_SHARED_ASSOCIATIONS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String igmpv2Support;
private final String staticSourcesSupport;
private final String autoAcceptSharedAssociations;
private CreateTransitGatewayMulticastDomainRequestOptions(BuilderImpl builder) {
this.igmpv2Support = builder.igmpv2Support;
this.staticSourcesSupport = builder.staticSourcesSupport;
this.autoAcceptSharedAssociations = builder.autoAcceptSharedAssociations;
}
/**
*
* Specify whether to enable Internet Group Management Protocol (IGMP) version 2 for the transit gateway multicast
* domain.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #igmpv2Support}
* will return {@link Igmpv2SupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #igmpv2SupportAsString}.
*
*
* @return Specify whether to enable Internet Group Management Protocol (IGMP) version 2 for the transit gateway
* multicast domain.
* @see Igmpv2SupportValue
*/
public final Igmpv2SupportValue igmpv2Support() {
return Igmpv2SupportValue.fromValue(igmpv2Support);
}
/**
*
* Specify whether to enable Internet Group Management Protocol (IGMP) version 2 for the transit gateway multicast
* domain.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #igmpv2Support}
* will return {@link Igmpv2SupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #igmpv2SupportAsString}.
*
*
* @return Specify whether to enable Internet Group Management Protocol (IGMP) version 2 for the transit gateway
* multicast domain.
* @see Igmpv2SupportValue
*/
public final String igmpv2SupportAsString() {
return igmpv2Support;
}
/**
*
* Specify whether to enable support for statically configuring multicast group sources for a domain.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #staticSourcesSupport} will return {@link StaticSourcesSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #staticSourcesSupportAsString}.
*
*
* @return Specify whether to enable support for statically configuring multicast group sources for a domain.
* @see StaticSourcesSupportValue
*/
public final StaticSourcesSupportValue staticSourcesSupport() {
return StaticSourcesSupportValue.fromValue(staticSourcesSupport);
}
/**
*
* Specify whether to enable support for statically configuring multicast group sources for a domain.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #staticSourcesSupport} will return {@link StaticSourcesSupportValue#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #staticSourcesSupportAsString}.
*
*
* @return Specify whether to enable support for statically configuring multicast group sources for a domain.
* @see StaticSourcesSupportValue
*/
public final String staticSourcesSupportAsString() {
return staticSourcesSupport;
}
/**
*
* Indicates whether to automatically accept cross-account subnet associations that are associated with the transit
* gateway multicast domain.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoAcceptSharedAssociations} will return
* {@link AutoAcceptSharedAssociationsValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #autoAcceptSharedAssociationsAsString}.
*
*
* @return Indicates whether to automatically accept cross-account subnet associations that are associated with the
* transit gateway multicast domain.
* @see AutoAcceptSharedAssociationsValue
*/
public final AutoAcceptSharedAssociationsValue autoAcceptSharedAssociations() {
return AutoAcceptSharedAssociationsValue.fromValue(autoAcceptSharedAssociations);
}
/**
*
* Indicates whether to automatically accept cross-account subnet associations that are associated with the transit
* gateway multicast domain.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #autoAcceptSharedAssociations} will return
* {@link AutoAcceptSharedAssociationsValue#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #autoAcceptSharedAssociationsAsString}.
*
*
* @return Indicates whether to automatically accept cross-account subnet associations that are associated with the
* transit gateway multicast domain.
* @see AutoAcceptSharedAssociationsValue
*/
public final String autoAcceptSharedAssociationsAsString() {
return autoAcceptSharedAssociations;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(igmpv2SupportAsString());
hashCode = 31 * hashCode + Objects.hashCode(staticSourcesSupportAsString());
hashCode = 31 * hashCode + Objects.hashCode(autoAcceptSharedAssociationsAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateTransitGatewayMulticastDomainRequestOptions)) {
return false;
}
CreateTransitGatewayMulticastDomainRequestOptions other = (CreateTransitGatewayMulticastDomainRequestOptions) obj;
return Objects.equals(igmpv2SupportAsString(), other.igmpv2SupportAsString())
&& Objects.equals(staticSourcesSupportAsString(), other.staticSourcesSupportAsString())
&& Objects.equals(autoAcceptSharedAssociationsAsString(), other.autoAcceptSharedAssociationsAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateTransitGatewayMulticastDomainRequestOptions")
.add("Igmpv2Support", igmpv2SupportAsString()).add("StaticSourcesSupport", staticSourcesSupportAsString())
.add("AutoAcceptSharedAssociations", autoAcceptSharedAssociationsAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Igmpv2Support":
return Optional.ofNullable(clazz.cast(igmpv2SupportAsString()));
case "StaticSourcesSupport":
return Optional.ofNullable(clazz.cast(staticSourcesSupportAsString()));
case "AutoAcceptSharedAssociations":
return Optional.ofNullable(clazz.cast(autoAcceptSharedAssociationsAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function